- How it works
- Homework answers
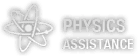
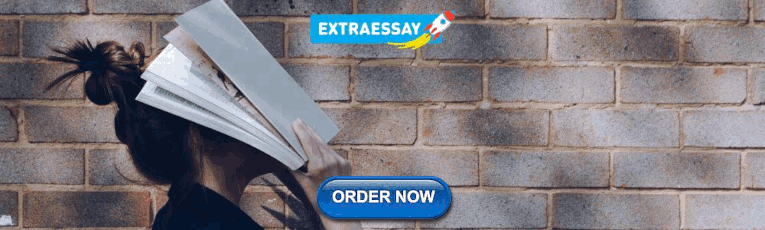
Answer to Question #240532 in Python for sai krishna
given an MxN integer matrix write a program to
Find all zeros and replace them with the sum of their neighboring elements.
After replacing the zeros set all other elements the corresponding row and column with zeros (excluding the elements which where previously zeros)
Note: consider the upper ,lower,right and left elements as neighboring elements
The first line of input is two space seperated integeres M and N
The next M lines of input contain N space seperated integers
the output should be an MxN matrix
sample input 1
sample output 1
sample input 2
sample output 2
Need a fast expert's response?
and get a quick answer at the best price
for any assignment or question with DETAILED EXPLANATIONS !
Leave a comment
Ask your question, related questions.
- 1. you given a list of prices where prices[i] is the price of a given stock o the i th day write a prog
- 2. given a sentence S. and a positive integer N. write a program to shift the sentence by N words to th
- 3. Activity #5 – Applying Functions The output of this activity will be the same as activity #3 but i
- 4. Activity #3 – Strings and LengthsCreate a program that will accept an integer X from the user, fol
- 5. Activity #2 – Smallest and DivisibilityCreate a program that will accept 3 integers from the user.
- 6. Roll the Dice, where each dice has from 1 to 6 dots on each side. Two dice will be rolled. Use th
- 7. Describe the difference between a chained conditional and a nested conditional. Give your own exa
- Programming
- Engineering
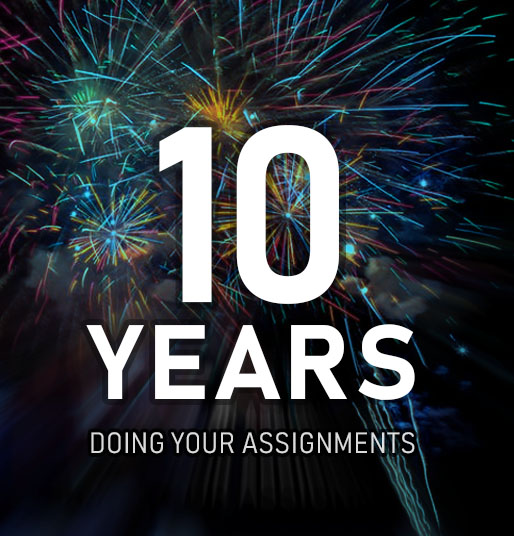
Learn Python practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn python interactively, python introduction.
- Getting Started
- Keywords and Identifier
- Python Comments
- Python Variables
- Python Data Types
- Python Type Conversion
- Python I/O and Import
- Python Operators
- Python Namespace
Python Flow Control
- Python if...else
- Python for Loop
- Python while Loop
- Python break and continue
- Python Pass
Python Functions
- Python Function
- Function Argument
- Python Recursion
- Anonymous Function
- Global, Local and Nonlocal
- Python Global Keyword
- Python Modules
- Python Package
Python Datatypes
- Python Numbers
- Python List
- Python Tuple
- Python String
- Python Dictionary
Python Files
- Python File Operation
- Python Directory
- Python Exception
- Exception Handling
- User-defined Exception
Python Object & Class
- Classes & Objects
- Python Inheritance
- Multiple Inheritance
- Operator Overloading
Python Advanced Topics
- Python Iterator
- Python Generator
- Python Closure
- Python Decorators
- Python Property
- Python RegEx
Python Date and time
- Python datetime Module
- Python datetime.strftime()
- Python datetime.strptime()
- Current date & time
- Get current time
- Timestamp to datetime
- Python time Module
- Python time.sleep()
Python Tutorials
Python Array
Python List copy()
Python Shallow Copy and Deep Copy
- Python abs()
- Python List Comprehension
Python Matrices and NumPy Arrays
A matrix is a two-dimensional data structure where numbers are arranged into rows and columns. For example:
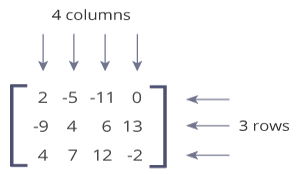
This matrix is a 3x4 (pronounced "three by four") matrix because it has 3 rows and 4 columns.
- Python Matrix
Python doesn't have a built-in type for matrices. However, we can treat a list of a list as a matrix. For example:
We can treat this list of a list as a matrix having 2 rows and 3 columns.
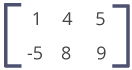
Be sure to learn about Python lists before proceed this article.
Let's see how to work with a nested list.
When we run the program, the output will be:
Here are few more examples related to Python matrices using nested lists.
- Add two matrices
- Transpose a Matrix
- Multiply two matrices
Using nested lists as a matrix works for simple computational tasks, however, there is a better way of working with matrices in Python using NumPy package.
- NumPy Array
NumPy is a package for scientific computing which has support for a powerful N-dimensional array object. Before you can use NumPy, you need to install it. For more info,
- Visit: How to install NumPy?
- If you are on Windows, download and install anaconda distribution of Python. It comes with NumPy and other several packages related to data science and machine learning.
Once NumPy is installed, you can import and use it.
NumPy provides multidimensional array of numbers (which is actually an object). Let's take an example:
As you can see, NumPy's array class is called ndarray .
How to create a NumPy array?
There are several ways to create NumPy arrays.
1. Array of integers, floats and complex Numbers
When you run the program, the output will be:
2. Array of zeros and ones
Here, we have specified dtype to 32 bits (4 bytes). Hence, this array can take values from -2 -31 to 2 -31 -1 .
3. Using arange() and shape()
Learn more about other ways of creating a NumPy array .
Matrix Operations
Above, we gave you 3 examples: addition of two matrices, multiplication of two matrices and transpose of a matrix. We used nested lists before to write those programs. Let's see how we can do the same task using NumPy array.
Addition of Two Matrices
We use + operator to add corresponding elements of two NumPy matrices.
Multiplication of Two Matrices
To multiply two matrices, we use dot() method. Learn more about how numpy.dot works.
Note: * is used for array multiplication (multiplication of corresponding elements of two arrays) not matrix multiplication.
Transpose of a Matrix
We use numpy.transpose to compute transpose of a matrix.
As you can see, NumPy made our task much easier.
Access matrix elements, rows and columns
Access matrix elements
Similar like lists, we can access matrix elements using index. Let's start with a one-dimensional NumPy array.
Now, let's see how we can access elements of a two-dimensional array (which is basically a matrix).
Access rows of a Matrix
Access columns of a Matrix
If you don't know how this above code works, read slicing of a matrix section of this article.
Slicing of a Matrix
Slicing of a one-dimensional NumPy array is similar to a list. If you don't know how slicing for a list works, visit Understanding Python's slice notation .
Let's take an example:
Now, let's see how we can slice a matrix.
As you can see, using NumPy (instead of nested lists) makes it a lot easier to work with matrices, and we haven't even scratched the basics. We suggest you to explore NumPy package in detail especially if you trying to use Python for data science/analytics.
NumPy Resources you might find helpful:
- NumPy Tutorial
- NumPy Reference
Table of Contents
- What is a matrix?
- Numbers(integers, float, complex etc.) Array
- Zeros and Ones Array
- Array Using arange() and shape()
- Multiplication
- Access elements
- Access rows
- Access columns
- Slicing of Matrix
- Useful Resources
Sorry about that.
Related Tutorials
Python Tutorial
Python Library
5 Best Ways to Explain Python Matrix with Examples
💡 Problem Formulation: Matrices are fundamental for a multitude of operations in software development, data analysis, and scientific computing. In Python, a matrix can be represented and manipulated in various ways. This article solves the problem of how to create, modify, and perform operations on Python matrices with practical examples. Imagine you want to represent 2D data like pixel values in an image or distances between cities; such tasks require creating and managing a matrix efficiently.
Method 1: Using Nested Lists
In Python, one of the most straightforward ways to represent a matrix is using nested lists. Each inner list represents a row in the matrix, and the elements of these lists are the matrix elements. Accessing, updating, and iterating over these matrices is intuitive and requires no additional libraries.
Here’s an example:
The code snippet creates a 3×3 matrix with nested lists. It then accesses and prints the element on the second row and third column, which is the number 6. This method is easy to understand and works well without additional dependencies, but can become inefficient for large matrices or complex operations.
Method 2: Using NumPy Arrays
NumPy is a powerful library for numerical computing in Python. Its array object is more efficient and convenient for large matrices and supports a wide range of mathematical operations. NumPy arrays are homogeneous, which can lead to better performance compared to nested lists.
This example demonstrates creating a NumPy array to represent a matrix and performing an element-wise addition with another matrix. NumPy arrays offer more efficient storage and better functionality for large-scale operations than lists.
Method 3: Using pandas DataFrame
pandas is another library that’s extremely useful for data analysis, and it provides the DataFrame object which can be thought of as a matrix with more functionality like labeled rows and columns. DataFrames are great for handling tabular data and can be created from lists, dicts, or even reading from files like CSV.
This code snippet creates a 3×3 matrix as a pandas DataFrame with labeled rows and columns. It selects and prints the element from row labeled ‘Y’ and column labeled ‘B’. pandas DataFrames are ideal for complex data manipulation but may be an overkill for simple matrix operations.
Method 4: Using List Comprehensions
List comprehensions provide a concise way to create lists including matrices. They are elegant and can be used to initialize, transform, and even transpose matrices with readable and compact code. List comprehensions are a Pythonic way to operate with matrices represented by lists.
The given code uses a list comprehension to create a 3×3 identity matrix, a matrix with 1s on the diagonal and 0s elsewhere. List comprehensions are a compact and readable method to create matrices, but can become less readable for very complex operations.
Bonus One-Liner Method 5: Using zip() and * Operator
Transposing a matrix, which is flipping it over its diagonal, can be elegantly achieved in Python by using the zip() function in conjunction with the star operator * . This one-liner is very readable and takes advantage of Python’s unpacking feature.
The code takes a 3×2 matrix and transposes it to a 2×3 matrix using the zip() function. The star operator unpacks the rows of the original matrix such that they are passed as separate arguments to zip() , which pairs elements of the same index from these arguments. This is a short and efficient one-liner for transposing matrices but may be less obvious to beginners.
Summary/Discussion
- Method 1: Nested Lists. Easily understandable. Not as efficient for large or complex matrices.
- Method 2: Using NumPy Arrays. Highly efficient and versatile. Requires additional knowledge of NumPy.
- Method 3: Using pandas DataFrames. Great for complex data manipulations with labeled axes. Overly powerful for simple tasks.
- Method 4: List Comprehensions. Elegant and Pythonic. Can become unwieldy for complex operations.
- Bonus Method 5: Using zip() and * Operator. Succinct for transposing. Less intuitive for those unfamiliar with argument unpacking.
Emily Rosemary Collins is a tech enthusiast with a strong background in computer science, always staying up-to-date with the latest trends and innovations. Apart from her love for technology, Emily enjoys exploring the great outdoors, participating in local community events, and dedicating her free time to painting and photography. Her interests and passion for personal growth make her an engaging conversationalist and a reliable source of knowledge in the ever-evolving world of technology.
- MapReduce Algorithm
- Linear Programming using Pyomo
- Networking and Professional Development for Machine Learning Careers in the USA
- Predicting Employee Churn in Python
- Airflow Operators

Solving Assignment Problem using Linear Programming in Python
Learn how to use Python PuLP to solve Assignment problems using Linear Programming.
In earlier articles, we have seen various applications of Linear programming such as transportation, transshipment problem, Cargo Loading problem, and shift-scheduling problem. Now In this tutorial, we will focus on another model that comes under the class of linear programming model known as the Assignment problem. Its objective function is similar to transportation problems. Here we minimize the objective function time or cost of manufacturing the products by allocating one job to one machine.
If we want to solve the maximization problem assignment problem then we subtract all the elements of the matrix from the highest element in the matrix or multiply the entire matrix by –1 and continue with the procedure. For solving the assignment problem, we use the Assignment technique or Hungarian method, or Flood’s technique.
The transportation problem is a special case of the linear programming model and the assignment problem is a special case of transportation problem, therefore it is also a special case of the linear programming problem.
In this tutorial, we are going to cover the following topics:
Assignment Problem
A problem that requires pairing two sets of items given a set of paired costs or profit in such a way that the total cost of the pairings is minimized or maximized. The assignment problem is a special case of linear programming.
For example, an operation manager needs to assign four jobs to four machines. The project manager needs to assign four projects to four staff members. Similarly, the marketing manager needs to assign the 4 salespersons to 4 territories. The manager’s goal is to minimize the total time or cost.
Problem Formulation
A manager has prepared a table that shows the cost of performing each of four jobs by each of four employees. The manager has stated his goal is to develop a set of job assignments that will minimize the total cost of getting all 4 jobs.
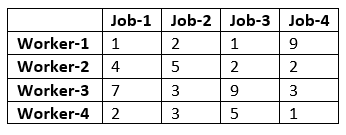
Initialize LP Model
In this step, we will import all the classes and functions of pulp module and create a Minimization LP problem using LpProblem class.
Define Decision Variable
In this step, we will define the decision variables. In our problem, we have two variable lists: workers and jobs. Let’s create them using LpVariable.dicts() class. LpVariable.dicts() used with Python’s list comprehension. LpVariable.dicts() will take the following four values:
- First, prefix name of what this variable represents.
- Second is the list of all the variables.
- Third is the lower bound on this variable.
- Fourth variable is the upper bound.
- Fourth is essentially the type of data (discrete or continuous). The options for the fourth parameter are LpContinuous or LpInteger .
Let’s first create a list route for the route between warehouse and project site and create the decision variables using LpVariable.dicts() the method.
Define Objective Function
In this step, we will define the minimum objective function by adding it to the LpProblem object. lpSum(vector)is used here to define multiple linear expressions. It also used list comprehension to add multiple variables.
Define the Constraints
Here, we are adding two types of constraints: Each job can be assigned to only one employee constraint and Each employee can be assigned to only one job. We have added the 2 constraints defined in the problem by adding them to the LpProblem object.
Solve Model
In this step, we will solve the LP problem by calling solve() method. We can print the final value by using the following for loop.
From the above results, we can infer that Worker-1 will be assigned to Job-1, Worker-2 will be assigned to job-3, Worker-3 will be assigned to Job-2, and Worker-4 will assign with job-4.
In this article, we have learned about Assignment problems, Problem Formulation, and implementation using the python PuLp library. We have solved the Assignment problem using a Linear programming problem in Python. Of course, this is just a simple case study, we can add more constraints to it and make it more complicated. You can also run other case studies on Cargo Loading problems , Staff scheduling problems . In upcoming articles, we will write more on different optimization problems such as transshipment problem, balanced diet problem. You can revise the basics of mathematical concepts in this article and learn about Linear Programming in this article .
- Solving Blending Problem in Python using Gurobi
- Transshipment Problem in Python Using PuLP
You May Also Like
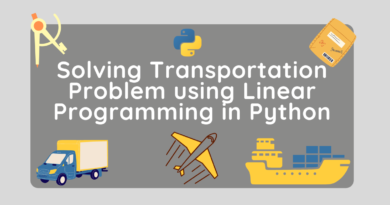
Solving Transportation Problem using Linear Programming in Python
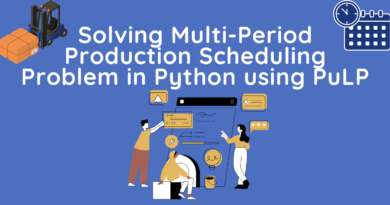
Solving Multi-Period Production Scheduling Problem in Python using PuLP
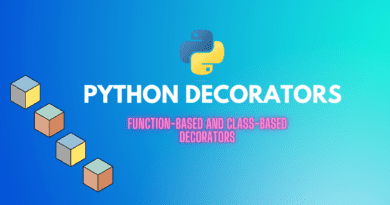
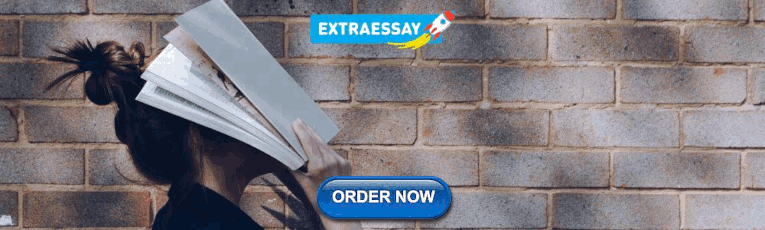
Python Decorators
- Free Python 3 Tutorial
- Control Flow
- Exception Handling
- Python Programs
- Python Projects
- Python Interview Questions
- Python Database
- Data Science With Python
- Machine Learning with Python
- Convert characters of a string to opposite case
- | | Question 35
- Handling Click events in Button | Android
- IoT- Recreating Healthcare
- Tokenization Using Spacy library
- | | Question 34
- SQL DELETE Statement
- Check whether Python shell is executing in 32bit or 64bit mode on OS
- Introduction of Optical Computing
- Who lost it - Apple or FBI?
- GATE | GATE CS 2019 | Question 25
- | | Question 33
- | | Question 32
- GATE CSE Test Series - 2019 | Sudo GATE
- UPSC Mains 2023 General Studies Paper 4 - Question Paper PDF Download (Free)
- SIM Card Formats
- Happy New Year!
- Forward chaining in AI with FOL proof
- | | Question 36
Python – Matrix
Here we will discuss different ways how we can form a matrix using Python within this tutorial we will also discuss the various operation that can be performed on a matrix. we will also cover the external module Numpy to form a matrix and its operations in Python .
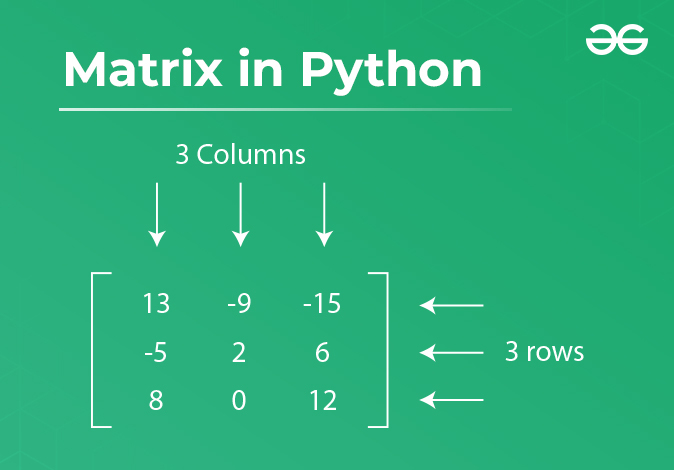
What is the matrix?
A matrix is a collection of numbers arranged in a rectangular array in rows and columns. In the fields of engineering, physics, statistics, and graphics, matrices are widely used to express picture rotations and other types of transformations. The matrix is referred to as an m by n matrix, denoted by the symbol “m x n” if there are m rows and n columns.
Creating a simple matrix using Python
Method 1: creating a matrix with a list of list.
Here, we are going to create a matrix using the list of lists.
Method 2: Take Matrix input from user in Python
Here, we are taking a number of rows and columns from the user and printing the Matrix.
Time Complexity: O(n*n) Auxiliary Space: O(n*n)
Method 3: Create a matrix using list comprehension
List comprehension is an elegant way to define and create a list in Python, we are using the range function for printing 4 rows and 4 columns.
Assigning Value in a matrix
Method 1: assign value to an individual cell in matrix.
Here we are replacing and assigning value to an individual cell (1 row and 1 column = 11) in the Matrix.
Method 2: Assign a value to an individual cell using negative indexing in Matrix
Here we are replacing and assigning value to an individual cell (-2 row and -1 column = 21) in the Matrix.
Accessing Value in a matrix
Method 1: accessing matrix values.
Here, we are accessing elements of a Matrix by passing its row and column.
Method 2: Accessing Matrix values using negative indexing
Here, we are accessing elements of a Matrix by passing its row and column on negative indexing.
Mathematical Operations with Matrix in Python
Example 1: adding values to a matrix with a for loop in python.
Here, we are adding two matrices using the Python for-loop.
Example 2: Adding and subtracting values to a matrix with list comprehension
Performing the Basic addition and subtraction using list comprehension.
Example 3: Python program to multiply and divide two matrices
Performing the Basic multiplication and division using Python loop.
Transpose in matrix
Example: python program to transpose a matrix using loop.
Transpose of a matrix is obtained by changing rows to columns and columns to rows. In other words, transpose of A[][] is obtained by changing A[i][j] to A[j][i].
Matrix using Numpy
Create a matrix using numpy .
Here we are creating a Numpy array using numpy.random and a random module .
Matrix mathematical operations in Python Using Numpy
Here we are covering different mathematical operations such as addition subtraction, multiplication, and division using Numpy.
Dot and cross product with Matrix
Here, we will find the inner, outer, and cross products of matrices and vectors using NumPy in Python.
Matrix transpose in Python using Numpy
To perform transpose operation in matrix we can use the numpy.transpose() method.
Create an empty matrix with NumPy in Python
Initializing an empty array, using the np.zeros() .
Slicing in Matrix using Numpy
Slicing is the process of choosing specific rows and columns from a matrix and then creating a new matrix by removing all of the non-selected elements. In the first example, we are printing the whole matrix, in the second we are passing 2 as an initial index, 3 as the last index, and index jump as 1. The same is used in the next print we have just changed the index jump to 2.
Delete rows and columns using Numpy
Here, we are trying to delete rows using the np.delete() function . In the code, we first tried to delete the 0 th row, then we tried to delete the 2 nd row, and then the 3 rd row.
Add row/columns in the Numpy array
We added one more column at the 4 th position using np.hstack .
Please Login to comment...
Similar reads.
- 10 Best Todoist Alternatives in 2024 (Free)
- How to Get Spotify Premium Free Forever on iOS/Android
- Yahoo Acquires Instagram Co-Founders' AI News Platform Artifact
- OpenAI Introduces DALL-E Editor Interface
- Top 10 R Project Ideas for Beginners in 2024
Improve your Coding Skills with Practice
What kind of Experience do you want to share?

The Assignment Problem & Calculating the Minimum Matrix Sum (Python)

Too Long; Didn't Read
Company mentioned.
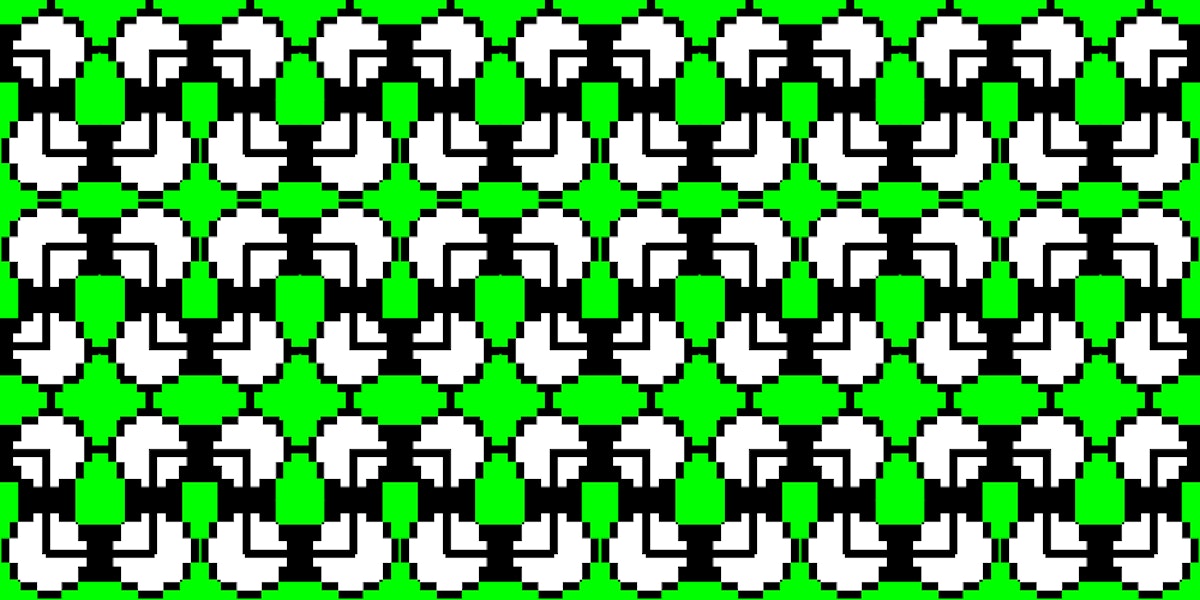
Ethan Jarrell
@ ethan.jarrell
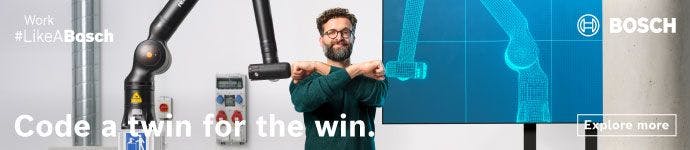
About Author
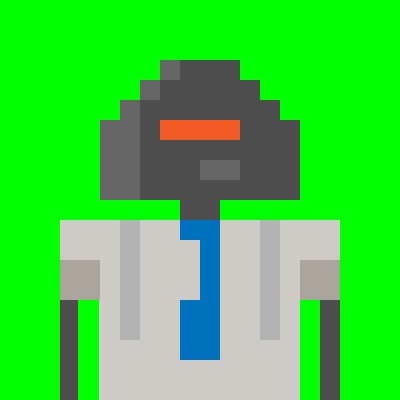
THIS ARTICLE WAS FEATURED IN ...

RELATED STORIES
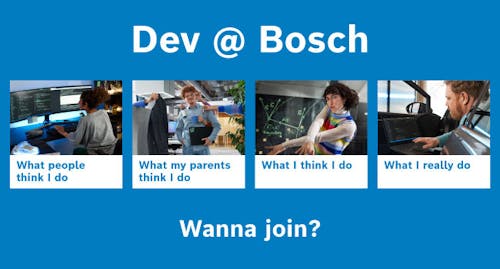
CopyAssignment
We are Python language experts, a community to solve Python problems, we are a 1.2 Million community on Instagram, now here to help with our blogs.
Ordered Matrix in Python
Problem statement:.
In the ordered matrix in python, we are given a matrix of integers. We need to convert the given matrix to an ordered matrix and print it. An ordered matrix is a matrix that is sorted row-wise.
Code for Ordered Matrix in Python:
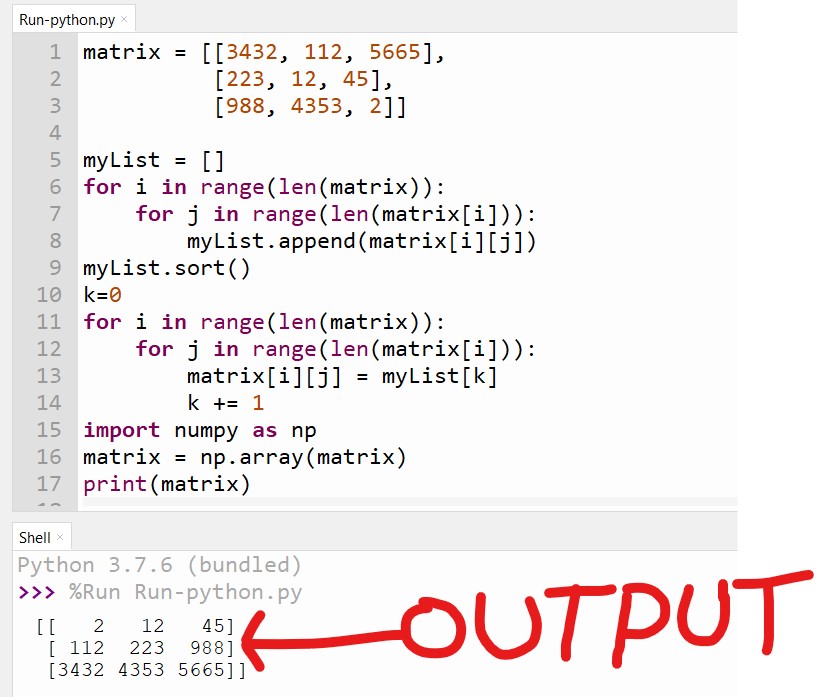
- Hyphenate Letters in Python
- Earthquake in Python | Easy Calculation
- Striped Rectangle in Python
- Perpendicular Words in Python
- Free shipping in Python
- Raj has ordered two electronic items Python | Assignment Expert
- Team Points in Python
- Ticket selling in Cricket Stadium using Python | Assignment Expert
- Split the sentence in Python
- String Slicing in JavaScript
- First and Last Digits in Python | Assignment Expert
- List Indexing in Python
- Date Format in Python | Assignment Expert
- New Year Countdown in Python
- Add Two Polynomials in Python
- Sum of even numbers in Python | Assignment Expert
- Evens and Odds in Python
- A Game of Letters in Python
- Sum of non-primes in Python
- Smallest Missing Number in Python
- String Rotation in Python
- Secret Message in Python
- Word Mix in Python
- Single Digit Number in Python
- Shift Numbers in Python | Assignment Expert
- Weekend in Python
- Temperature Conversion in Python
- Special Characters in Python
- Sum of Prime Numbers in the Input in Python
Author: Harry

Search….
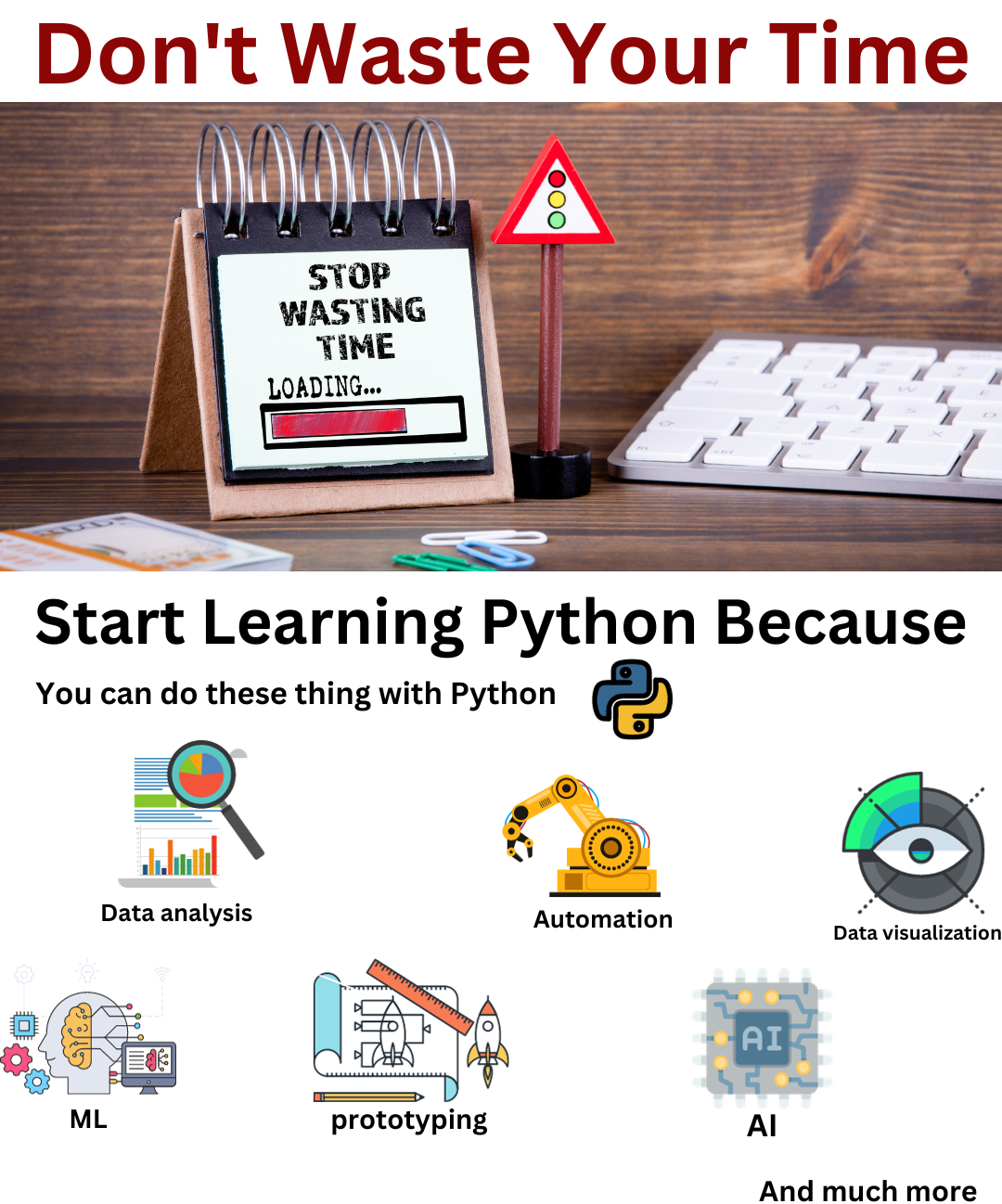
Machine Learning
Data Structures and Algorithms(Python)
Python Turtle
Games with Python
All Blogs On-Site
Python Compiler(Interpreter)
Online Java Editor
Online C++ Editor
Online C Editor
All Editors
Services(Freelancing)
Recent Posts
- Most Underrated Database Trick | Life-Saving SQL Command
- Python List Methods
- Top 5 Free HTML Resume Templates in 2024 | With Source Code
- How to See Connected Wi-Fi Passwords in Windows?
- 2023 Merry Christmas using Python Turtle
© Copyright 2019-2023 www.copyassignment.com. All rights reserved. Developed by copyassignment
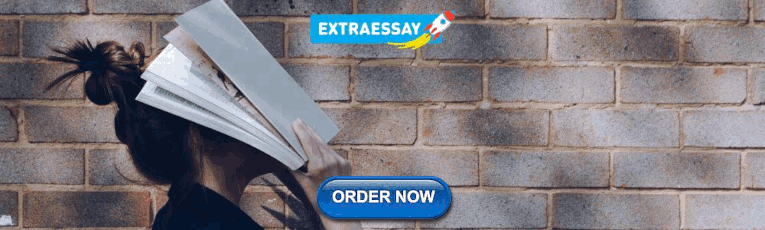
IMAGES
VIDEO
COMMENTS
Question #314508. Matrix Problem. you are given an m*n matrix.write a program to compute the perimeter of the matrix and print the result.Perimeter of a matrix is defined as the sum of all elements of the four edges of the matrix. Input. The first line of input containing the positive integer. The second line of input containing the positive ...
Question #182874. Ordered Matrix. Given a M x N matrix, write a program to print the matrix after ordering all the elements of the matrix in increasing order.Input. The first line of input will contain two space-separated integers, denoting the M and N. The next M following lines will contain N space-separated integers, denoting the elements of ...
Question #240532. given an MxN integer matrix write a program to. Find all zeros and replace them with the sum of their neighboring elements. After replacing the zeros set all other elements the corresponding row and column with zeros (excluding the elements which where previously zeros) Note: consider the upper ,lower,right and left elements ...
Assignment Operators; Relational Operators; Logical Operators; Ternary Operators; Flow Control in Java. ... Top 50 Array Problems; Top 50 String Problems; Top 50 DP Problems; Top 50 Graph Problems; ... In python matrix can be implemented as 2D list or 2D Array. Forming matrix from latter, gives the additional functionalities for performing ...
Python Matrix. Python doesn't have a built-in type for matrices. However, we can treat a list of a list as a matrix. For example: A = [[1, 4, 5], [-5, 8, 9]] We can treat this list of a list as a matrix having 2 rows and 3 columns. Be sure to learn about Python lists before proceed this article.
To understand the idea behind the inverse of a matrix, start by recalling the concept of the multiplicative inverse of a number. When you multiply a number by its inverse, you get 1 as the result. Take 3 as an example. The inverse of 3 is 1/3, and when you multiply these numbers, you get 3 × 1/3 = 1.
Method 1: Using Nested Lists. In Python, one of the most straightforward ways to represent a matrix is using nested lists. Each inner list represents a row in the matrix, and the elements of these lists are the matrix elements. Accessing, updating, and iterating over these matrices is intuitive and requires no additional libraries.
In this step, we will solve the LP problem by calling solve () method. We can print the final value by using the following for loop. From the above results, we can infer that Worker-1 will be assigned to Job-1, Worker-2 will be assigned to job-3, Worker-3 will be assigned to Job-2, and Worker-4 will assign with job-4.
A matrix is a collection of numbers arranged in a rectangular array in rows and columns. In the fields of engineering, physics, statistics, and graphics, matrices are widely used to express picture rotations and other types of transformations. The matrix is referred to as an m by n matrix, denoted by the symbol "m x n" if there are m rows ...
The Assignment Problem & Calculating the Minimum Matrix Sum (Python) by @ethan.jarrell. 13,831 reads. 13,831 reads. The Assignment Problem & Calculating the Minimum Matrix Sum (Python) by Ethan Jarrell 13m March 23rd, 2018. Too Long; Didn't Read. Company Mentioned. Ethan Jarrell @ ethan.jarrell. Share Your Thoughts. About Author.
6. No, NumPy contains no such function. Combinatorial optimization is outside of NumPy's scope. It may be possible to do it with one of the optimizers in scipy.optimize but I have a feeling that the constraints may not be of the right form. NetworkX probably also includes algorithms for assignment problems.
The Hungarian method is a combinatorial optimization algorithm that solves the assignment problem in polynomial time and which anticipated later primal-dual methods. It was developed and published ...
Our Teligram group Link 🔗https://t.me/shivatechcareerhub Instagram id : https://instagram.com/mr_chiva?igshid=YmMyMTA2M2Y=Cal me on Calme4 app:id: shivapra...
We are Python language experts, a community to solve Python problems, we are a 1.2 Million community on Instagram, now here to help with our blogs. ... Ordered Matrix in Python Harry September 7, 2022. Problem Statement: In the ordered matrix in python, we are given a matrix of integers. We need to convert the given matrix to an ordered matrix ...
1. This row: matrix[n, m] = sum(1 for item in b if item==(i)) counts the occurrences of i in b and saves the result to matrix [n, m]. Each cell of the matrix will contain either the number of 1's in b (i.e. 2) or the number of 2's in b (i.e. 2) or the number of 3's in b (i.e. 6). Notice that this value is completely independent of j, which ...
I have wrote this piece of code and need to generate a matrix and save it. But, when assigning the matrix values, it says "KeyError: 0"!! ... Construct an assignment matrix - Python. 0. Assignning value with for loop in two dimensional arrays (matrixes in python) ... system stand going back in time (when applying winter time for example)? Going ...
The Assignment Problem is a special type of Linear Programming Problem based on the following assumptions: However, solving this task for increasing number of jobs and/or resources calls for…