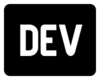
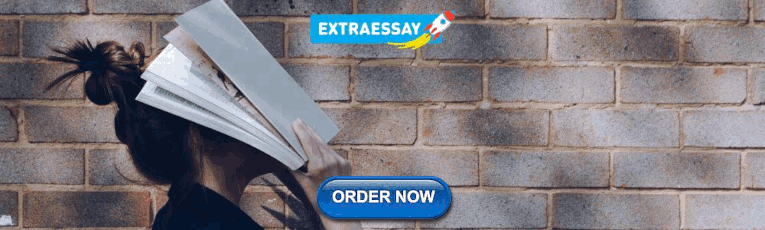
DEV Community

Posted on Jun 1, 2023
How to Avoid Unchecked Casts in Java Programs
Unchecked cast refers to the process of converting a variable of one data type to another data type without checks by the Java compiler.
This operation is unchecked because the compiler does not verify if the operation is valid or safe. Unchecked casts can lead to runtime errors, such as ClassCastException, when the program tries to assign an object to a variable of an incompatible type.
Hence, it is important to avoid unchecked casts in Java programs to prevent potential errors and ensure the program's reliability.
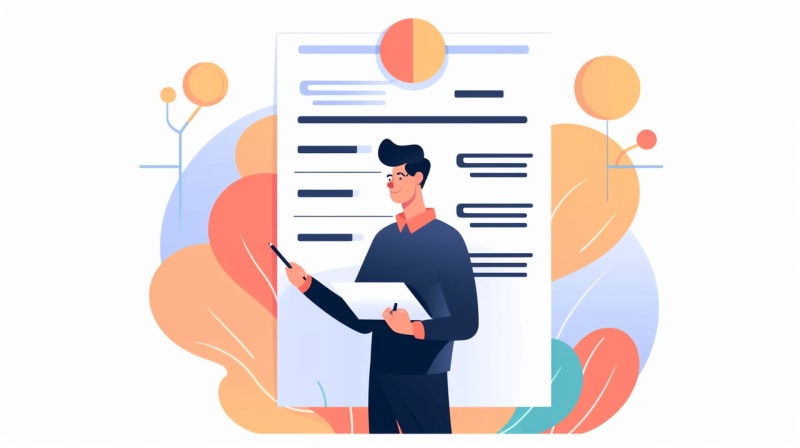
Consequences of Unchecked Casts
In Java programs, unchecked casts can lead to several issues. The most common problem is a ClassCastException at runtime, which occurs when we try to cast an object to a wrong type. This can cause the program to crash or behave unexpectedly.
Unchecked casts also violate the type safety of the Java language, which can lead to bugs that are difficult to detect and debug. Additionally, unchecked casts can make the code less readable and maintainable, as they hide the true type of objects and dependencies between components.
Therefore, it is important to avoid unchecked casts and use other mechanisms, such as generics or polymorphism, to ensure type safety and code quality in Java programs.
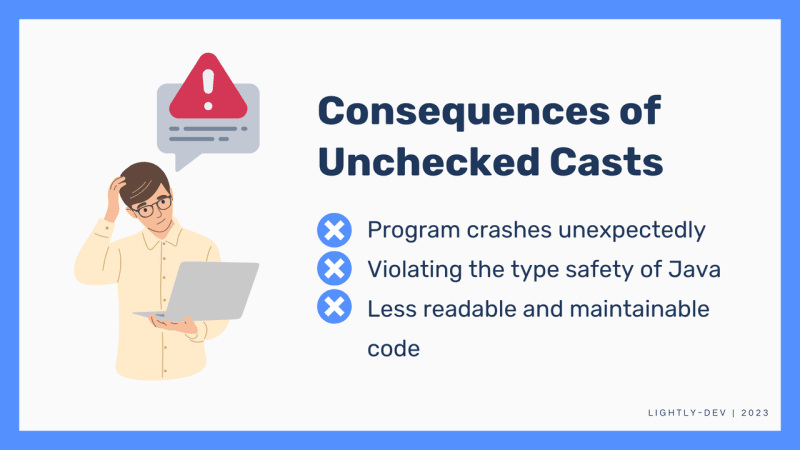
How Unchecked Casts Occur
Unchecked casts in Java programs occur when an object of one type is assigned to a reference of another type without proper type checking. This can happen when a programmer assumes that a reference to a superclass is actually a reference to its subclass and tries to cast it into that subclass. If the assumption is incorrect, the cast will result in a ClassCastException at runtime.
Unchecked casts can also occur when dealing with raw types, which are generic types without any type parameters specified. In such cases, the compiler cannot perform type checking and the programmer must ensure that the proper type conversions are made. Failing to do so can result in unchecked casts and potential runtime errors.
Why unchecked casts are problematic
In Java, unchecked casts allow a programmer to cast any object reference to any other reference type without providing any type information at compile-time. While this flexibility may seem useful, it can lead to serious run-time errors. If the object being casted is not actually of the type specified, a ClassCastException will occur at run-time.
Unchecked casts can cause difficult-to-debug errors in large and complex codebases, as it may not be immediately clear where the error originated. Additionally, unchecked casts can undermine Java's type system, creating code that is harder to read, maintain, and reason about. As a result, avoiding unchecked casts should be a priority when writing Java programs.
Examples of Unchecked Casts in Java
Unchecked casts are a common source of Java program errors. Here are some examples of unchecked casts:
This cast statement above can result in a class cast exception if the object referred to by obj is not a List.
In this case, the cast could fail at runtime if the array contains objects of a type other than String.
Finally, this cast could fail if the object referred to by obj is not a Map.
Using Generics to Avoid Unchecked Casts in Java
In Java, Generics is a powerful feature that allows you to write classes and methods that are parameterized by one or more types. Generics are a way of making your code more type-safe and reusable. With generics, you can define classes and methods that work on a variety of types, without having to write separate code for each type.
Using generics in Java programs has several advantages. It enables type safety at compile-time, which can prevent ClassCastException errors at runtime. With generics, the compiler can detect type mismatches and prevent them from happening, which leads to more robust and reliable code. It also allows for code reuse without sacrificing type safety and improve performance by avoiding unnecessary casting and allowing for more efficient code generation.
Generics allow Java developers to create classes and methods that can work with different data types. For example, a List can be defined to hold any type of object using generics. Here's an example:
In this example, we create a List that holds String objects. We can add String objects to the list and iterate over them using a for-each loop. The use of generics allows us to ensure type safety and avoid unchecked casts. Another example is the Map interface, which can be used to map keys to values of any data type using generics.
Using the instanceof operator to Avoid Unchecked Casts in Java
The instanceof operator is a built-in operator in Java that is used to check whether an object is an instance of a particular class or interface. The operator returns a boolean value - true if the object is an instance of the specified class or interface, and false otherwise.
The instanceof operator is defined as follows:
where object is the object that is being checked, and class/interface is the class or interface that is being tested against.
The instanceof operator can be useful in situations where we need to perform different operations based on the type of an object. It provides a way to check the type of an object at runtime, which can help prevent errors that can occur when performing unchecked casts.
Here are some examples of using the instanceof operator:
In this example, we use the instanceof operator to check whether the object obj is an instance of the String class. If it is, we perform an explicit cast to convert the object to a String and call the toUpperCase() method on it.
In this example, we use the instanceof operator to check whether the List object passed as a parameter is an instance of the ArrayList or LinkedList classes. If it is, we perform an explicit cast to convert the List to the appropriate class and perform different operations on it depending on its type.
Overall, using the instanceof operator can help us write more robust and flexible code. However, it should be used judiciously as it can also make code harder to read and understand.
Using Polymorphism to Avoid Unchecked Casts in Java
Polymorphism is a fundamental concept in object-oriented programming. It refers to the ability of an object or method to take on multiple forms. It allows us to write code that can work with objects of different classes as long as they inherit from a common superclass or implement a common interface. This helps to reduce code duplication and makes our programs more modular and extensible.
Some of the advantages of using polymorphism are:
- Code reusability: We can write code that can work with multiple objects without having to rewrite it for each specific class.
- Flexibility: Polymorphism allows us to write code that can adapt to different types of objects at runtime.
- Ease of maintenance: By using polymorphism, changes made to a superclass or interface are automatically propagated to all its subclasses.
Here are a few examples of how you can use polymorphism to avoid unchecked casts in Java:
Example 1: Shape Hierarchy
In this example, the abstract class Shape defines the common behavior draw(), which is implemented by the concrete classes Circle and Rectangle. By using the Shape reference type, we can invoke the draw() method on different objects without the need for unchecked casts.
Example 2: Polymorphic Method Parameter
In this example, the makeAnimalSound() method accepts an Animal parameter. We can pass different Animal objects, such as Dog or Cat, without the need for unchecked casts. The appropriate implementation of the makeSound() method will be invoked based on the dynamic type of the object.
By utilizing polymorphism in these examples, we achieve type safety and avoid unchecked casts, allowing for cleaner and more flexible code.
Tips to Avoid Unchecked Casts in Java Programs
Unchecked casts in Java programs can introduce runtime errors and compromise type safety. Fortunately, there are several techniques and best practices you can employ to avoid unchecked casts and ensure a more robust codebase. Here are some effective tips to help you write Java programs that are type-safe and free from unchecked cast exceptions.
- Use generic classes, interfaces, and methods to ensure that your code handles compatible types without relying on casting.
- Embrace polymorphism by utilizing abstract classes and interfaces, define common behavior and interact with objects through their common type.
- Check the type of an object using the instanceof operator. This allows you to verify that an object is of the expected type before proceeding with the cast.
- Favor composition over inheritance, where classes contain references to other classes as instance variables.
- Familiarize yourself with design patterns that promote type safety and avoid unchecked casts. Patterns such as Factory Method, Builder, and Strategy provide alternative approaches to object creation and behavior, often eliminating the need for explicit casting.
- Clearly define the contracts and preconditions for your methods. A well-defined contract helps ensure that the method is called with appropriate types, improving overall code safety.
- Refactor your code and improve its overall design. Look for opportunities to apply the aforementioned tips, such as utilizing generics, polymorphism, or design patterns.
Unchecked casts in Java programs can introduce runtime errors and undermine type safety. By adopting practices like using generics, leveraging polymorphism, checking types with instanceof, favoring composition over inheritance, reviewing design patterns, employing design by contract, and improving code design, you can avoid unchecked casts and enhance the robustness of your Java programs. Prioritizing type safety will result in more reliable code and a smoother development process.
Lightly IDE as a Programming Learning Platform
So, you want to learn a new programming language? Don't worry, it's not like climbing Mount Everest. With Lightly IDE, you'll feel like a coding pro in no time. With Lightly IDE , you don't need to be a coding wizard to start programming.
One of its standout features is its intuitive design, which makes it easy to use even if you're a technologically challenged unicorn. With just a few clicks, you can become a programming wizard in Lightly IDE. It's like magic, but with less wands and more code.
If you're looking to dip your toes into the world of programming or just want to pretend like you know what you're doing, Lightly IDE's online Java compiler is the perfect place to start. It's like a playground for programming geniuses in the making! Even if you're a total newbie, this platform will make you feel like a coding superstar in no time.
Read more: How to Avoid Unchecked Casts in Java Programs
Top comments (0)
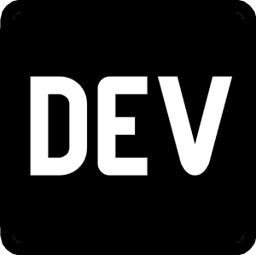
Templates let you quickly answer FAQs or store snippets for re-use.
Are you sure you want to hide this comment? It will become hidden in your post, but will still be visible via the comment's permalink .
Hide child comments as well
For further actions, you may consider blocking this person and/or reporting abuse
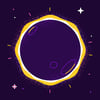
استكشاف المستقبل الرقمي: التقارب بين البلوكتشين والعقود الذكية في BitNest
Aa A - Apr 25
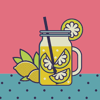
AA A - Apr 25
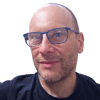
Descansa en Paz, Z80
Baltasar García Perez-Schofield - Apr 25
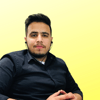
My Experience with Finding the Ideal Car Detailer for My Mercedes E-Class
alex - Apr 25
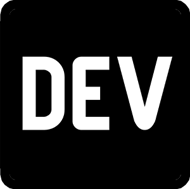
We're a place where coders share, stay up-to-date and grow their careers.
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement . We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Unchecked assignment: 'java.util.List' to 'java.util.List<ua.lv.hoy.entity.Customer>' #184
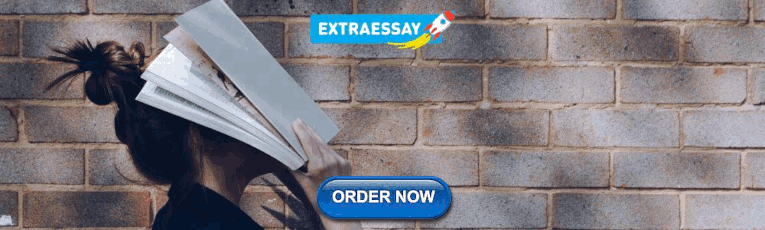
ghost commented Feb 8, 2018
The text was updated successfully, but these errors were encountered:
halyafg commented Feb 10, 2018
Sorry, something went wrong.
ghost commented Feb 26, 2018
Halyafg commented feb 27, 2018.
No branches or pull requests
- Unchecked Cast in Java
- Java Howtos
What Is the Unchecked Cast Warning in Java
Understanding unchecked cast warnings in java, preventing unchecked cast warnings, best practices for preventing unchecked cast warnings in java.
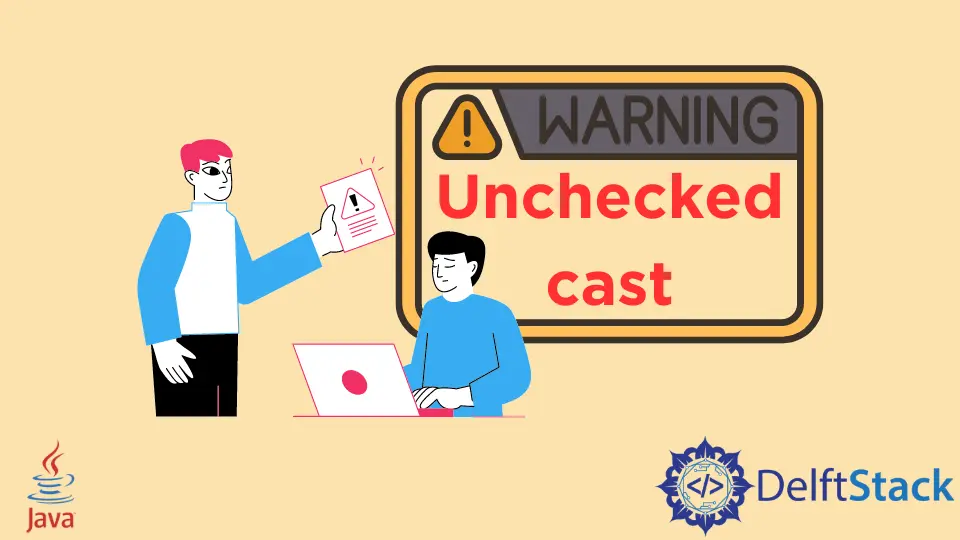
Java is a programming language that enforces type safety, which means that we should always specify the type of data that we are going to store or use and cannot store incompatible types in them.
Discover how to prevent unchecked cast warnings in Java. Explore the causes, solutions, and best practices for ensuring type safety and reliability in your code.
An unchecked cast warning in Java occurs when the compiler cannot ensure type safety during a casting operation. It warns the developer about potential runtime errors, such as ClassCastException , that may occur due to type mismatches.
Unchecked cast warnings typically arise when casting from a generic type to a specific type or when casting to a parameterized type without proper type checking. Addressing these warnings is crucial to ensure code reliability and prevent unexpected runtime errors.
In Java programming, unchecked cast warnings are common occurrences that indicate potential type safety issues in your code. Let’s delve into two significant causes of unchecked cast warnings:
Understanding these causes is crucial for maintaining code quality and preventing unexpected runtime errors. Let’s explore solutions to address unchecked cast warnings in Java code.
In Java programming, unchecked cast warnings signify potential type safety issues that can lead to runtime errors if not addressed properly. Direct casting from raw types and casting without type checking are common scenarios where unchecked cast warnings occur.
Understanding how to prevent these warnings is crucial for maintaining code integrity and preventing unexpected runtime errors. In this section, we’ll explore effective techniques to prevent unchecked cast warnings in Java by addressing direct casting from raw types and casting without proper type checking.
Code Example:
The code example provided illustrates two common scenarios in Java where unchecked cast warnings can arise: direct casting from raw types and casting without proper type checking.
In the first scenario, a raw ArrayList named rawList is instantiated, and a String ( Hello ) is added to it. Initially, an attempt is made to directly cast rawList to a parameterized type List<String> .
Such direct casting from a raw type can trigger unchecked cast warnings as it bypasses type safety checks. To address this, we adopt a safer approach by creating a new ArrayList , stringList1 .
By passing rawList as a parameter to its constructor, we ensure that stringList1 maintains the correct generic type. This action effectively prevents unchecked cast warnings, ensuring type safety throughout the code.
In the second scenario, an Object ( obj ) is assigned the value World . There is an initial attempt to cast obj directly to List<String> without performing proper type checking.
Such casting without type checking can lead to unchecked cast warnings as it lacks verification of type compatibility. To mitigate this risk, we instantiate a new ArrayList ( stringList2 ) and add obj after performing necessary type checking and casting.
By ensuring that the object being added to stringList2 is indeed a String type, we maintain type safety and avoid unchecked cast warnings.
By employing these techniques, developers can effectively prevent unchecked cast warnings in their Java code, thereby enhancing type safety and reducing the likelihood of unexpected runtime errors.
The code will produce the following output:

By following the demonstrated techniques, developers can effectively prevent unchecked cast warnings in Java code, ensuring type safety and reducing the risk of unexpected runtime errors. Understanding and implementing these practices are essential for maintaining code reliability and integrity in Java applications.
Use Generics Consistently
Utilize parameterized types (generics) consistently throughout your code to ensure type safety and prevent unchecked cast warnings.
Perform Type Checking Before Casting
Always perform proper type checking before casting objects to parameterized types to ensure compatibility and prevent unchecked cast warnings.
Avoid Raw Types
Minimize the use of raw types and prefer parameterized types whenever possible to maintain type safety and prevent unchecked cast warnings.
Consider Type Inference
Leverage type inference where applicable to automatically determine generic types and reduce the likelihood of unchecked cast warnings.
Review and Test Code
Regularly review and test your code to identify and address any instances of unchecked cast warnings, ensuring robustness and reliability.
Unchecked cast warnings in Java signal potential type safety issues arising from direct casting from raw types and casting without proper type checking. The causes include direct casting from raw types and casting without type checking.
Solutions involve creating new parameterized types instead of directly casting from raw types and performing type checking before casting. Best practices include using generics consistently, avoiding raw types, considering type inference, and regularly reviewing and testing code.
By implementing these strategies, developers can ensure type safety and prevent unchecked cast warnings in Java programs.
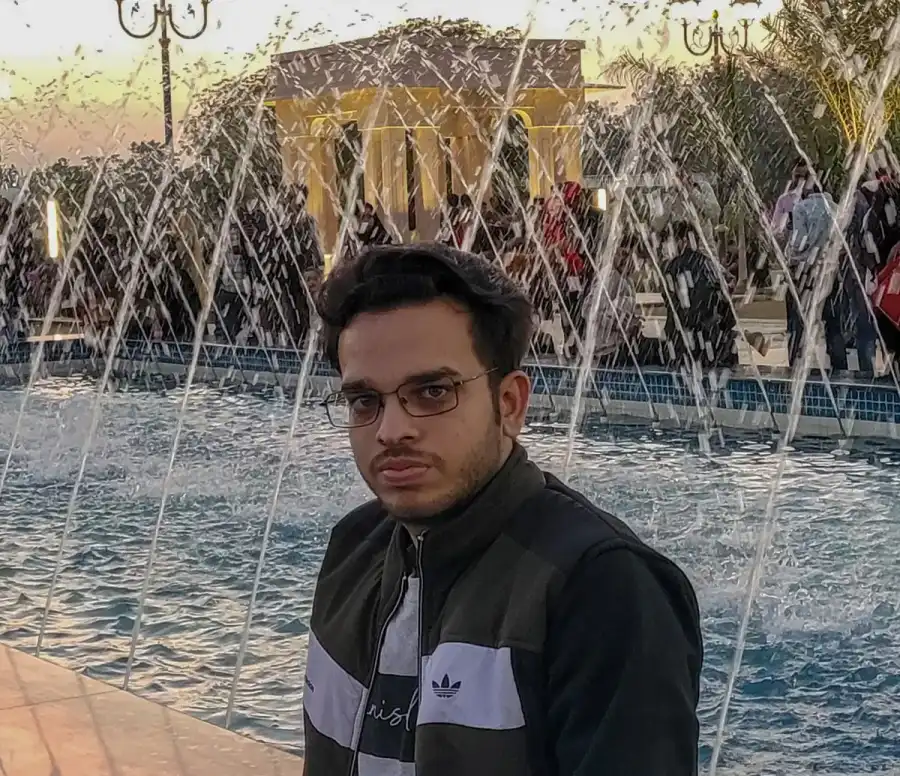
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
Unchecked assignment: ‘java.util.List’ to ‘java.util.Collection’
I am having an adapter where i have two lists one list is for InvestorsList where it comes with the list of investors and the other list is called investorListFull which is used to filter results when searching.
Below is how i have declared the lists
Below is how the lists are assigned in my recyclerview adapter constructor
Below is how i am filtering results in investors list
I am getting Unchecked assignment error in publish results investorList.addAll((List) filterResults.values);
Advertisement
I am getting Unchecked cast error in publish results investorList.addAll((List) filterResults.values);
That’s because you’re doing an unchecked cast. Actually, you’re doing both a checked and an unchecked cast there.
(List) filterResults.values is a checked cast. This means that the Java compiler will insert a checkcast instruction into the bytecode to ensure that filterResults.values (an Object ) really is an instance of List .
However, investorList.addAll expects a List<Investor> , not a List . List is a raw type . You can pass a raw-typed List to a method expecting a List<Something> ; but this is flagged as unsafe because the compiler can’t guarantee that it really is a List<Something> – there is nothing about the List that makes it a “list of Something “, because of type erasure. The compiler can insert a checkcast instruction to ensure it’s a List ; but not one to ensure it’s a List<Something> : this fact is unchecked .
What it’s saying with the warning is “there may be something wrong here; I just can’t prove it’s wrong”. If you know for sure – by construction – that filterResults.values really is a List<Investor> , casting it to List<Investor> is safe.
You should write the line as:
Note that this will still give you an unchecked cast warning, because it’s still an unchecked cast – you just avoid the use of raw types as well.
If you feel confident in suppressing the warning, declare a variable, so you can suppress the warning specifically on that variable, rather than having to add the suppression to the method or class; and document why it’s safe to suppress there:
Trying to understand an unchecked conversion warning

How to use checkedList method in java.util.Collections
Best java code snippets using java.util . collections . checkedlist (showing top 20 results out of 432).
Class LinkedList<E>
All of the operations perform as could be expected for a doubly-linked list. Operations that index into the list will traverse the list from the beginning or the end, whichever is closer to the specified index.
Note that this implementation is not synchronized. If multiple threads access a linked list concurrently, and at least one of the threads modifies the list structurally, it must be synchronized externally. (A structural modification is any operation that adds or deletes one or more elements; merely setting the value of an element is not a structural modification.) This is typically accomplished by synchronizing on some object that naturally encapsulates the list. If no such object exists, the list should be "wrapped" using the Collections.synchronizedList method. This is best done at creation time, to prevent accidental unsynchronized access to the list: List list = Collections.synchronizedList(new LinkedList(...));
The iterators returned by this class's iterator and listIterator methods are fail-fast : if the list is structurally modified at any time after the iterator is created, in any way except through the Iterator's own remove or add methods, the iterator will throw a ConcurrentModificationException . Thus, in the face of concurrent modification, the iterator fails quickly and cleanly, rather than risking arbitrary, non-deterministic behavior at an undetermined time in the future.
Note that the fail-fast behavior of an iterator cannot be guaranteed as it is, generally speaking, impossible to make any hard guarantees in the presence of unsynchronized concurrent modification. Fail-fast iterators throw ConcurrentModificationException on a best-effort basis. Therefore, it would be wrong to write a program that depended on this exception for its correctness: the fail-fast behavior of iterators should be used only to detect bugs.
This class is a member of the Java Collections Framework .
- Serialized Form
Field Summary
Fields declared in class java.util. abstractlist, constructor summary, method summary, methods declared in class java.util. abstractsequentiallist, methods declared in class java.util. abstractlist, methods declared in class java.util. abstractcollection, methods declared in class java.lang. object, methods declared in interface java.util. collection, methods declared in interface java.util. deque, methods declared in interface java.lang. iterable, methods declared in interface java.util. list, constructor details, method details, removefirst.
This method is equivalent to add(E) .
This method is equivalent to addLast(E) .
- AbstractCollection.add(Object)
lastIndexOf
This method is equivalent to addFirst(E) .
This method is equivalent to removeFirst() .
removeFirstOccurrence
Removelastoccurrence, listiterator.
The list-iterator is fail-fast : if the list is structurally modified at any time after the Iterator is created, in any way except through the list-iterator's own remove or add methods, the list-iterator will throw a ConcurrentModificationException . Thus, in the face of concurrent modification, the iterator fails quickly and cleanly, rather than risking arbitrary, non-deterministic behavior at an undetermined time in the future.
- List.listIterator(int)
descendingIterator
The returned array will be "safe" in that no references to it are maintained by this list. (In other words, this method must allocate a new array). The caller is thus free to modify the returned array.
This method acts as bridge between array-based and collection-based APIs.
- Arrays.asList(Object[])
If the list fits in the specified array with room to spare (i.e., the array has more elements than the list), the element in the array immediately following the end of the list is set to null . (This is useful in determining the length of the list only if the caller knows that the list does not contain any null elements.)
Like the toArray() method, this method acts as bridge between array-based and collection-based APIs. Further, this method allows precise control over the runtime type of the output array, and may, under certain circumstances, be used to save allocation costs.
Suppose x is a list known to contain only strings. The following code can be used to dump the list into a newly allocated array of String : String[] y = x.toArray(new String[0]); Note that toArray(new Object[0]) is identical in function to toArray() .
spliterator
The Spliterator reports Spliterator.SIZED and Spliterator.ORDERED . Overriding implementations should document the reporting of additional characteristic values.
Modifications to the reversed view are permitted and will be propagated to this list. In addition, modifications to this list will be visible in the reversed view.
Scripting on this page tracks web page traffic, but does not change the content in any way.
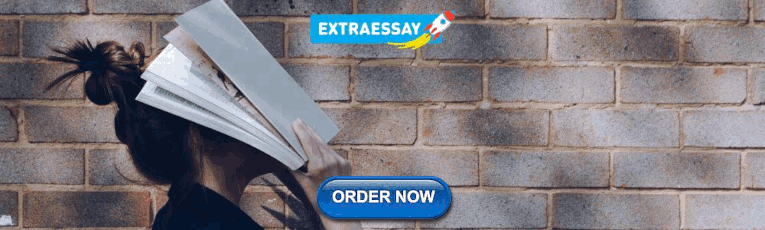
IMAGES
VIDEO
COMMENTS
Unchecked assignment: 'java.util.List[]' to 'java.util.List<java.lang.String>[]' java; Share. Improve this question. Follow edited Nov 12, 2020 at 15:46. Mark Rotteveel. 106k 211 211 gold badges 148 148 silver badges 204 204 bronze badges. asked Nov 12, 2020 at 10:09.
This assignment is allowed by the compiler because the compiler has to allow this assignment to preserve backward compatibility with older Java versions that do not support generics.. An example will explain it quickly. Let's say we have a simple method to return a raw type List:. public class UncheckedConversion { public static List getRawList() { List result = new ArrayList(); result.add ...
The "unchecked cast" is a compile-time warning . Simply put, we'll see this warning when casting a raw type to a parameterized type without type checking. An example can explain it straightforwardly. Let's say we have a simple method to return a raw type Map: public class UncheckedCast {. public static Map getRawMap() {.
Unchecked assignment: java.util.List to java.util.List<String> Unfortunately, there's no way to fix that problem. I've tried adding <String>before the dot and after, but neither works.
Unchecked casts are a common source of Java program errors. Here are some examples of unchecked casts: List names = (List) obj; // cast Object to List. This cast statement above can result in a class cast exception if the object referred to by obj is not a List.
MacBook:Homework Brienna$ javac Orders.java -Xlint:unchecked Orders.java:152: warning: [unchecked] unchecked cast orders = (ArrayList<Vehicle>) in.readObject(); ^ required: ArrayList<Vehicle> found: Object 1 warning I always try to improve my code instead of ignoring or suppressing warnings. In this case, I have come up with a solution, but I'm ...
具体告警为:Unchecked assignment:'java.util.List' to 'java.util.List<java.lang.String>',即从List转为List<String>是未检查的分配。 JSONObject.parseObject(jsonString, List.class)fastjson反序列化时指定的类型是List,反序列化出来的自然是List,但是却用一个List<Srting>接收,就告警了。
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
The code example provided illustrates two common scenarios in Java where unchecked cast warnings can arise: direct casting from raw types and casting without proper type checking.. In the first scenario, a raw ArrayList named rawList is instantiated, and a String (Hello) is added to it.Initially, an attempt is made to directly cast rawList to a parameterized type List<String>.
Actually, you're doing both a checked and an unchecked cast there. (List) filterResults.values is a checked cast. This means that the Java compiler will insert a checkcast instruction into the bytecode to ensure that filterResults.values (an Object) really is an instance of List. However, investorList.addAll expects a List<Investor>, not a ...
In the process of using some of these classes I get an unchecked conversion warning and I am unsure exactly what it is trying to tell me. 350: warning: [unchecked] unchecked conversion. found : java.util.List. required: java.util.List<org.jfree.data.xy.XYDataItem>. item = data.getItems ();
Best Java code snippets using java.util. Collections.checkedList (Showing top 20 results out of 432) java.util Collections checkedList. Collections.addAll (innerList, elements); return Collections.checkedList (innerList, String.class);
An unchecked cast warning in Java occurs when the compiler cannot verify that a cast is safe at compile time. This can happen when you are casting an object to a type that is not a supertype or subtype of the object's actual type. To address an unchecked cast warning, you can either suppress the warning using the @SuppressWarnings("unchecked ...
Everything works fine I'm just getting a warning when invoking .addAll () method on the listOfObjects variable. This warning states : Unchecked assignment, java.util.List to java.util.Collection<? extends T> Reason, paginatedResponse.getPaginationData () has raw type so the result of getPaginatedObjectList () is erased.
Returns a list-iterator of the elements in this list (in proper sequence), starting at the specified position in the list. Obeys the general contract of List.listIterator(int).. The list-iterator is fail-fast: if the list is structurally modified at any time after the Iterator is created, in any way except through the list-iterator's own remove or add methods, the list-iterator will throw a ...
Warning:(20, 39) Unchecked assignment: 'java.util.List' to 'java.util.List<com.flowplanner.persistence.Transaction>'. Reason: 'new CsvToBeanBuilder(fr).withType(Transaction.class).build()' has raw type, so result of parse is erased. The code in question is intended to import a simple CSV to java beans. The pojo field names map perfectly to the ...
Of course that's an unchecked assignment. You seem to be implementing a heterogeneous map. Java (and any other strongly-typed language) has no way to express the value type of your map in a statically-type-safe way. That is because the element types are only known at runtime.