Learn Java practically and Get Certified .
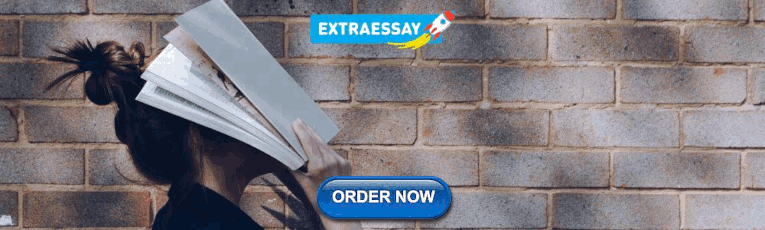
Popular Tutorials
Popular examples, reference materials, learn java interactively, java introduction.
- Java Hello World
- Java JVM, JRE and JDK
- Java Variables and Literals
- Java Data Types
- Java Operators
- Java Input and Output
- Java Expressions & Blocks
- Java Comment
Java Flow Control
- Java if...else
Java switch Statement
- Java for Loop
- Java for-each Loop
- Java while Loop
Java break Statement
- Java continue Statement
- Java Arrays
- Multidimensional Array
- Java Copy Array
Java OOP (I)
- Java Class and Objects
- Java Methods
- Java Method Overloading
- Java Constructor
- Java Strings
- Java Access Modifiers
- Java this keyword
- Java final keyword
- Java Recursion
- Java instanceof Operator
Java OOP (II)
- Java Inheritance
- Java Method Overriding
- Java super Keyword
- Abstract Class & Method
- Java Interfaces
- Java Polymorphism
- Java Encapsulation
Java OOP (III)
- Nested & Inner Class
- Java Static Class
- Java Anonymous Class
- Java Singleton
- Java enum Class
- Java enum Constructor
- Java enum String
- Java Reflection
- Java Exception Handling
- Java Exceptions
- Java try...catch
- Java throw and throws
- Java catch Multiple Exceptions
- Java try-with-resources
- Java Annotations
- Java Annotation Types
- Java Logging
- Java Assertions
- Java Collections Framework
- Java Collection Interface
- Java List Interface
- Java ArrayList
- Java Vector
- Java Queue Interface
- Java PriorityQueue
- Java Deque Interface
- Java LinkedList
- Java ArrayDeque
- Java BlockingQueue Interface
- Java ArrayBlockingQueue
- Java LinkedBlockingQueue
- Java Map Interface
- Java HashMap
- Java LinkedHashMap
- Java WeakHashMap
- Java EnumMap
- Java SortedMap Interface
- Java NavigableMap Interface
- Java TreeMap
- Java ConcurrentMap Interface
- Java ConcurrentHashMap
- Java Set Interface
- Java HashSet
- Java EnumSet
- Java LinkedhashSet
- Java SortedSet Interface
- Java NavigableSet Interface
- Java TreeSet
- Java Algorithms
- Java Iterator
- Java ListIterator
- Java I/O Streams
- Java InputStream
- Java OutputStream
- Java FileInputStream
- Java FileOutputStream
- Java ByteArrayInputStream
- Java ByteArrayOutputStream
- Java ObjectInputStream
- Java ObjectOutputStream
- Java BufferedInputStream
- Java BufferedOutputStream
- Java PrintStream
Java Reader/Writer
- Java Reader
- Java Writer
- Java InputStreamReader
- Java OutputStreamWriter
- Java FileReader
- Java FileWriter
- Java BufferedReader
- Java BufferedWriter
- Java StringReader
- Java StringWriter
- Java PrintWriter
Additional Topics
- Java Scanner Class
- Java Type Casting
- Java autoboxing and unboxing
- Java Lambda Expression
- Java Generics
- Java File Class
- Java Wrapper Class
- Java Command Line Arguments
Java Tutorials
Java Ternary Operator
Java Expressions, Statements and Blocks
Java if...else Statement
In programming, we use the if..else statement to run a block of code among more than one alternatives.
For example, assigning grades (A, B, C) based on the percentage obtained by a student.
- if the percentage is above 90 , assign grade A
- if the percentage is above 75 , assign grade B
- if the percentage is above 65 , assign grade C
1. Java if (if-then) Statement
The syntax of an if-then statement is:
Here, condition is a boolean expression such as age >= 18 .
- if condition evaluates to true , statements are executed
- if condition evaluates to false , statements are skipped
Working of if Statement
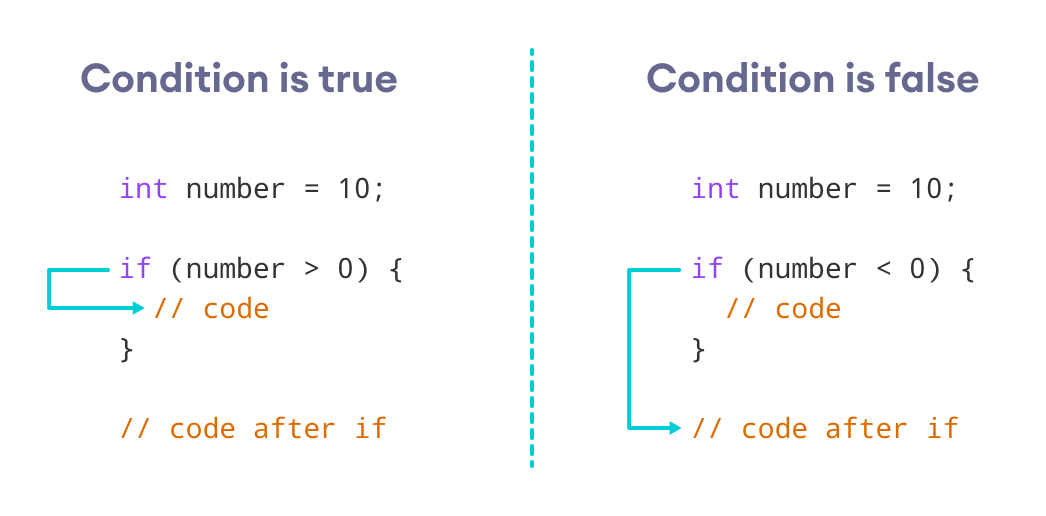
Example 1: Java if Statement
In the program, number < 0 is false . Hence, the code inside the body of the if statement is skipped .
Note: If you want to learn more about about test conditions, visit Java Relational Operators and Java Logical Operators .
We can also use Java Strings as the test condition.
Example 2: Java if with String
In the above example, we are comparing two strings in the if block.
2. Java if...else (if-then-else) Statement
The if statement executes a certain section of code if the test expression is evaluated to true . However, if the test expression is evaluated to false , it does nothing.
In this case, we can use an optional else block. Statements inside the body of else block are executed if the test expression is evaluated to false . This is known as the if-...else statement in Java.
The syntax of the if...else statement is:
Here, the program will do one task (codes inside if block) if the condition is true and another task (codes inside else block) if the condition is false .
How the if...else statement works?
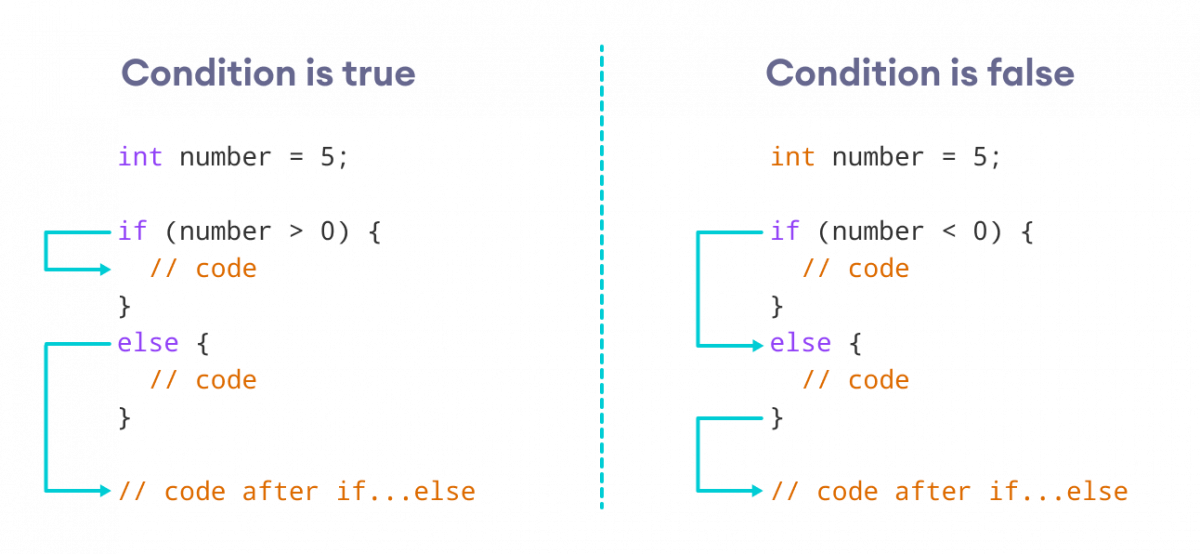
Example 3: Java if...else Statement
In the above example, we have a variable named number . Here, the test expression number > 0 checks if number is greater than 0.
Since the value of the number is 10 , the test expression evaluates to true . Hence code inside the body of if is executed.
Now, change the value of the number to a negative integer. Let's say -5 .
If we run the program with the new value of number , the output will be:
Here, the value of number is -5 . So the test expression evaluates to false . Hence code inside the body of else is executed.
3. Java if...else...if Statement
In Java, we have an if...else...if ladder, that can be used to execute one block of code among multiple other blocks.
Here, if statements are executed from the top towards the bottom. When the test condition is true , codes inside the body of that if block is executed. And, program control jumps outside the if...else...if ladder.
If all test expressions are false , codes inside the body of else are executed.
How the if...else...if ladder works?
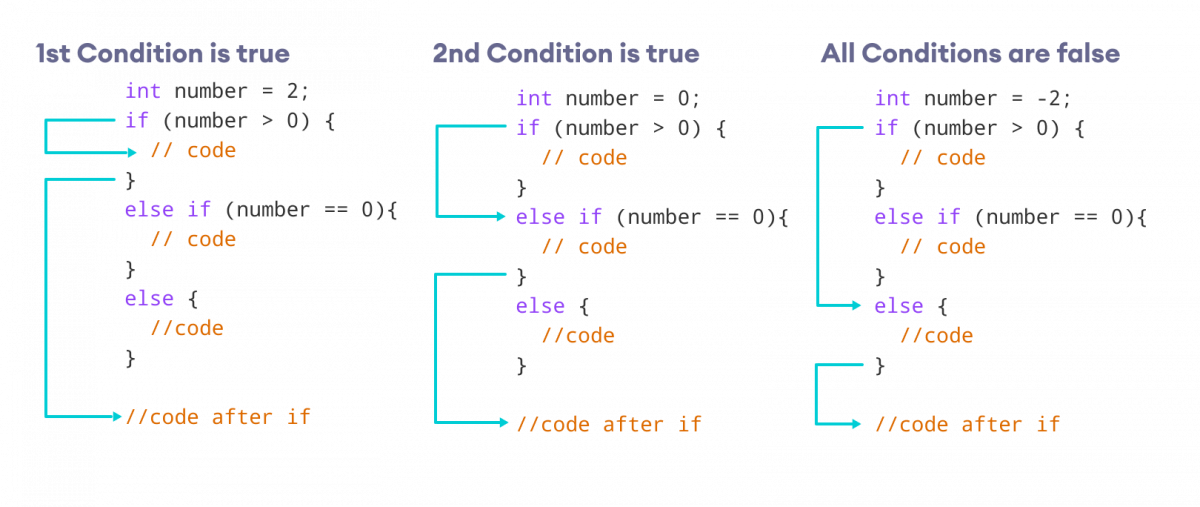
Example 4: Java if...else...if Statement
In the above example, we are checking whether number is positive, negative, or zero . Here, we have two condition expressions:
- number > 0 - checks if number is greater than 0
- number < 0 - checks if number is less than 0
Here, the value of number is 0 . So both the conditions evaluate to false . Hence the statement inside the body of else is executed.
Note : Java provides a special operator called ternary operator , which is a kind of shorthand notation of if...else...if statement. To learn about the ternary operator, visit Java Ternary Operator .
4. Java Nested if..else Statement
In Java, it is also possible to use if..else statements inside an if...else statement. It's called the nested if...else statement.
Here's a program to find the largest of 3 numbers using the nested if...else statement.
Example 5: Nested if...else Statement
In the above programs, we have assigned the value of variables ourselves to make this easier.
However, in real-world applications, these values may come from user input data, log files, form submission, etc.
Table of Contents
- Introduction
- Java if (if-then) Statement
- Example: Java if Statement
- Java if...else (if-then-else) Statement
- Example: Java if else Statement
- Java if..else..if Statement
- Example: Java if..else..if Statement
- Java Nested if..else Statement
Sorry about that.
Related Tutorials
Java Tutorial
The Java Tutorials have been written for JDK 8. Examples and practices described in this page don't take advantage of improvements introduced in later releases and might use technology no longer available. See Java Language Changes for a summary of updated language features in Java SE 9 and subsequent releases. See JDK Release Notes for information about new features, enhancements, and removed or deprecated options for all JDK releases.
The if-then and if-then-else Statements
The if-then statement.
The if-then statement is the most basic of all the control flow statements. It tells your program to execute a certain section of code only if a particular test evaluates to true . For example, the Bicycle class could allow the brakes to decrease the bicycle's speed only if the bicycle is already in motion. One possible implementation of the applyBrakes method could be as follows:
If this test evaluates to false (meaning that the bicycle is not in motion), control jumps to the end of the if-then statement.
In addition, the opening and closing braces are optional, provided that the "then" clause contains only one statement:
Deciding when to omit the braces is a matter of personal taste. Omitting them can make the code more brittle. If a second statement is later added to the "then" clause, a common mistake would be forgetting to add the newly required braces. The compiler cannot catch this sort of error; you'll just get the wrong results.
The if-then-else Statement
The if-then-else statement provides a secondary path of execution when an "if" clause evaluates to false . You could use an if-then-else statement in the applyBrakes method to take some action if the brakes are applied when the bicycle is not in motion. In this case, the action is to simply print an error message stating that the bicycle has already stopped.
The following program, IfElseDemo , assigns a grade based on the value of a test score: an A for a score of 90% or above, a B for a score of 80% or above, and so on.
The output from the program is:
You may have noticed that the value of testscore can satisfy more than one expression in the compound statement: 76 >= 70 and 76 >= 60 . However, once a condition is satisfied, the appropriate statements are executed (grade = 'C';) and the remaining conditions are not evaluated.
About Oracle | Contact Us | Legal Notices | Terms of Use | Your Privacy Rights
Copyright © 1995, 2022 Oracle and/or its affiliates. All rights reserved.
Java Tutorial
Java methods, java classes, java file handling, java how to, java reference, java examples, java short hand if...else (ternary operator), short hand if...else.
There is also a short-hand if else , which is known as the ternary operator because it consists of three operands.
It can be used to replace multiple lines of code with a single line, and is most often used to replace simple if else statements:
Instead of writing:
Try it Yourself »
You can simply write:
Test Yourself With Exercises
Insert the missing parts to complete the following "short hand if...else" statement:
Start the Exercise

COLOR PICKER

Report Error
If you want to report an error, or if you want to make a suggestion, do not hesitate to send us an e-mail:
Top Tutorials
Top references, top examples, get certified.

Java Tutorial
Control statements, java object class, java inheritance, java polymorphism, java abstraction, java encapsulation, java oops misc.
- Send your Feedback to [email protected]
Help Others, Please Share

Learn Latest Tutorials

Transact-SQL

Reinforcement Learning

R Programming

React Native

Python Design Patterns

Python Pillow

Python Turtle

Preparation

Verbal Ability

Interview Questions

Company Questions
Trending Technologies

Artificial Intelligence

Cloud Computing

Data Science

Machine Learning

B.Tech / MCA

Data Structures

Operating System

Computer Network

Compiler Design

Computer Organization

Discrete Mathematics

Ethical Hacking

Computer Graphics

Software Engineering

Web Technology

Cyber Security

C Programming

Control System

Data Mining

Data Warehouse

- Java One Line if Statement
- Java Howtos
Ternary Operator in Java
One line if-else statement using filter in java 8.
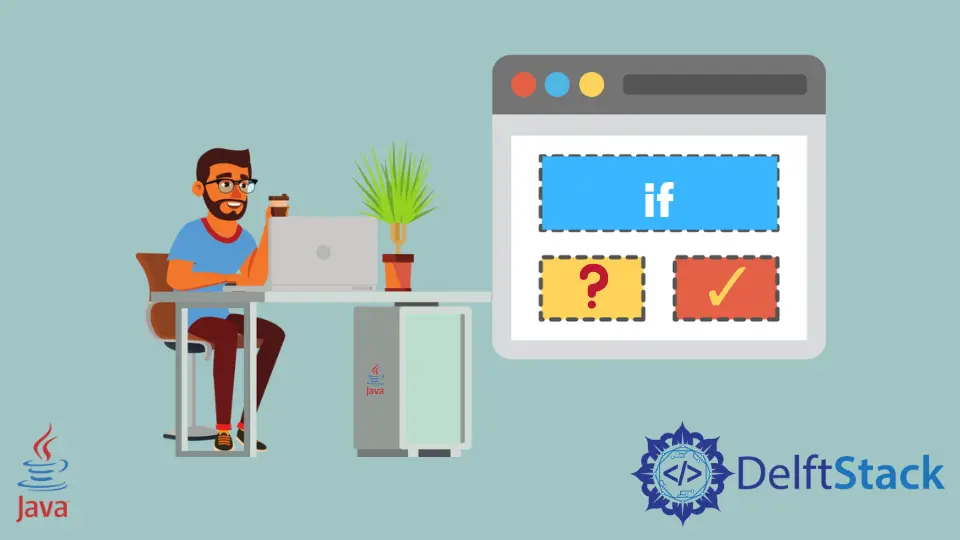
The if-else statement in Java is a fundamental construct used to conditionally execute blocks of code based on certain conditions. However, it often requires multiple lines to define a simple if-else block, which may not always be ideal, especially for concise and readable code.
Fortunately, Java provides a shorthand form called the ternary operator, which allows us to write a one-line if-else statement.
The ternary operator in Java, denoted as ? : , provides a compact way to represent an if-else statement. Its syntax is as follows:
Here, condition is evaluated first. If condition is true, expression1 is executed; otherwise, expression2 is executed.
Example 1: Assigning a Value Based on a Condition
This code uses the ternary operator to determine if a student has made a distinction based on their exam marks.
If marks is greater than 70 , the string Yes is assigned to the distinction variable and the output will be:
If marks is less than or equal to 70 , the string No is assigned to the distinction variable and the output will be:
Example 2: Printing a Message Conditionally
This code defines a Boolean variable isRaining with the value true and uses a ternary operator to print a message based on the value of isRaining .
If isRaining is true , it prints Bring an umbrella ; otherwise, it prints No need for an umbrella .
Example 3: Returning a Value from a Method
In this code, we’re assigning the maximum of a and b to the variable max using a ternary operator. The maximum value is then printed to the console.
You can try it yourself by replacing the values of a and b with your desired values to find the maximum between them.
Java 8 introduced streams and the filter method, which operates similarly to an if-else statement. It allows us to filter elements based on a condition.
Here’s its syntax:
Predicate is a functional interface that takes an argument and returns a Boolean. It’s often used with lambda expressions to define the condition for filtering.
Here’s a demonstration of a one-line if-else -like usage using the filter method in Java 8 streams.
As we can see, this example demonstrates a one-line usage of an if-else -like structure using the filter method. It first defines a list of words and then applies a stream to this list.
Within the map function, a lambda expression is used to check if each word starts with the letter b . If a word meets this condition, it remains unchanged; otherwise, it is replaced with the string Not available .
Finally, the resulting stream is collected back into a list using collect(Collectors.toList()) . The output shows the effect of this one-line if-else -like usage, modifying the words based on the specified condition.
Let’s see another example.
This example code also showcases the use of Java 8 streams to filter and print elements from a list based on a condition. The code begins by importing necessary packages and defining a class named Java8Streams .
Inside the main method, a list of strings, stringList , is created with elements 1 and 2 . The stream is then created from this list using the stream() method.
Next, the filter method is applied to this stream, utilizing a lambda expression as the predicate. The lambda expression checks if the string is equal to 1 .
If this condition is true, the string is allowed to pass through the filter; otherwise, it is discarded.
Finally, the forEach method is used to iterate over the filtered stream and print each element that passed the filter. It uses a method reference System.out::println to achieve this.
In summary, one-line if statements in Java offer a compact and efficient way to handle simple conditional logic. By understanding the syntax and appropriate usage, you can write concise code while maintaining readability and clarity.
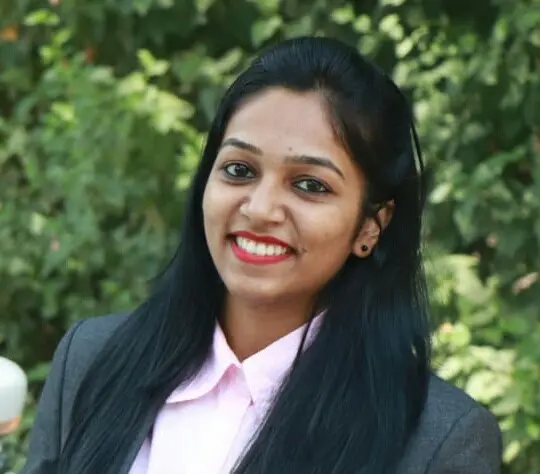
Rashmi is a professional Software Developer with hands on over varied tech stack. She has been working on Java, Springboot, Microservices, Typescript, MySQL, Graphql and more. She loves to spread knowledge via her writings. She is keen taking up new things and adopt in her career.
Related Article - Java Statement
- Difference Between break and continue Statements in Java
- The continue Statement in Java
We Love Servers.
- WHY IOFLOOD?
- BARE METAL CLOUD
- DEDICATED SERVERS
If-Else Java: Mastering Decision Making in Code
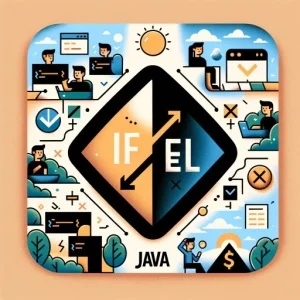
Ever found yourself puzzled on how to make your Java program make decisions? You’re not alone. Many developers find themselves at crossroads when it comes to controlling the flow of their Java programs. Think of the ‘if-else’ statement in Java as a traffic light – it regulates the flow of your program, directing it where to go based on certain conditions.
This guide will take you through the journey of mastering the ‘if-else’ statement in Java , from the basics to more advanced usage. We’ll cover everything from writing your first if-else statement, understanding its flow, to handling more complex scenarios with nested if-else and if-else-if ladder. We’ll also explore alternative approaches to control the flow of a Java program.
So, let’s dive in and start mastering the if-else statement in Java!
TL;DR: How Do I Use the If-Else Statement in Java?
An ‘if-else’ statement is used to perform different actions based on different conditions, defined with the syntax: if (condition) {// code if condition is true} else {code if condition is false} It’s like a fork in the road, directing your program based on the conditions you set.
Here’s a simple example:
In this example, the ‘if-else’ statement checks a condition. If the condition is true, it executes the code within the ‘if’ block. If the condition is false, it executes the code within the ‘else’ block. It’s a simple yet powerful way to control the flow of your program.
But there’s so much more to the ‘if-else’ statement in Java. Continue reading for a deeper understanding, more advanced usage scenarios, and alternative approaches.
Table of Contents
Beginner’s Guide to If-Else in Java
Intermediate-level if-else in java, exploring alternatives to if-else in java, troubleshooting java if-else statements, control flow: the heart of programming, if-else in larger java projects, wrapping up: mastering if-else statements in java.
The ‘if-else’ statement in Java is a cornerstone of decision-making in your program. It allows you to execute different blocks of code based on specific conditions. Let’s break down how to use it step by step.
Writing Your First If-Else Statement
Here’s a simple example of how to use the ‘if-else’ statement:
In this example, we’re checking if the score is greater than or equal to 90. If it is, the program will print ‘Excellent!’, otherwise it will print ‘Good job, but there’s room for improvement.’
Advantages of Using If-Else
The ‘if-else’ statement is a powerful tool for controlling your program’s flow. It allows you to specify different actions for different conditions, making your program more flexible and adaptable.
Pitfalls to Watch Out For
While the ‘if-else’ statement is incredibly useful, it’s important to use it correctly. Be careful not to write conditions that are always true or always false, as this can lead to unexpected behavior. Additionally, remember that the order of your conditions matters. The program will check the conditions in the order they appear, so make sure you’re considering all possible scenarios.
As you gain more experience with Java, you’ll find situations where a simple ‘if-else’ statement isn’t enough. This is where more complex structures like nested ‘if-else’ and ‘if-else-if’ ladders come in.
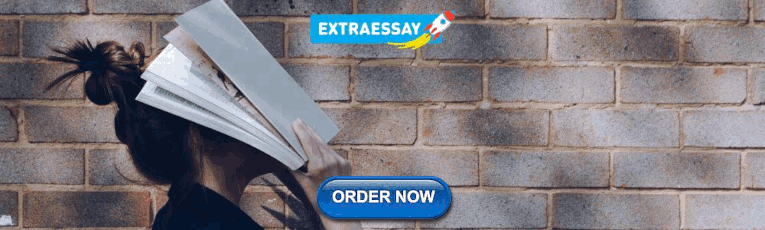
Nested If-Else Statements
A nested ‘if-else’ statement is an ‘if-else’ statement within another ‘if-else’ statement. It allows for more complex decision-making in your program.
Here’s an example:
In this example, if the score is not greater than or equal to 90, the program checks another condition: if the score is greater than or equal to 80. If it is, it prints ‘Very Good!’. If not, it prints ‘Good job, but there’s room for improvement.’
If-Else-If Ladder
An ‘if-else-if’ ladder is a series of conditions checked one after the other. It’s useful when you have multiple conditions to check.
In this example, the program checks the conditions in order. If the score is not greater than or equal to 90, it checks if the score is greater than or equal to 80. If it is, it prints ‘Very Good!’. If not, it prints ‘Good job, but there’s room for improvement.’
While ‘if-else’ statements are a fundamental part of controlling flow in Java, they aren’t the only tool at your disposal. Another powerful tool is the ‘switch’ statement.
The Power of Switch Statements
The ‘switch’ statement in Java allows you to select one of many code blocks to be executed. It’s a more readable and efficient alternative to the ‘if-else-if’ ladder when dealing with multiple conditions.
In this example, the ‘switch’ statement checks the value of ‘day’. Depending on the value, it assigns a different string to ‘dayOfWeek’. If ‘day’ is not a value between 1 and 7, it assigns ‘Invalid day’ to ‘dayOfWeek’.
Advantages and Drawbacks of Switch Statements
Switch statements can make your code cleaner and easier to read when dealing with multiple conditions. However, they are less flexible than ‘if-else’ statements. They can only be used with discrete values and cannot handle ranges or complex conditions.
Making the Right Choice
When deciding whether to use ‘if-else’ or ‘switch’, consider the complexity of your conditions. If you’re dealing with ranges or complex conditions, ‘if-else’ is the way to go. If you’re dealing with multiple discrete values, consider using ‘switch’ for cleaner, more readable code.
Like any tool, ‘if-else’ statements in Java can present challenges, especially when used in complex ways. Let’s look at some common issues and their solutions.
Dealing with Syntax Errors
One of the most common issues when using ‘if-else’ statements is syntax errors. These can occur if you forget a bracket, misspell a keyword, or make other typographical errors. For example:
In this case, the opening bracket after the ‘if’ statement is missing, causing a syntax error. The solution is to correct the syntax:
Handling Logical Errors
Another common issue is logical errors, where the code runs without errors, but doesn’t produce the expected result. This usually happens due to incorrect conditions in the ‘if-else’ statement.
For example, consider this code:
Even though the score is 85, the program prints ‘Excellent!’ because the condition checks if the score is less than or equal to 90, not greater than or equal to. The solution is to correct the condition:
These are just a few examples of the challenges you might face when using ‘if-else’ statements in Java. Remember, the key to effective troubleshooting is understanding the problem, reading error messages carefully, and knowing how ‘if-else’ statements work.
Control flow is a fundamental concept in programming. It’s the order in which your program executes instructions. In Java, control flow is managed using conditional statements like ‘if-else’, loops, and other constructs.
The Role of If-Else in Control Flow
The ‘if-else’ statement is a conditional statement that plays a pivotal role in control flow. It allows your program to choose a path of execution based on whether a condition is true or false.
Here’s a simple ‘if-else’ statement:
In this example, the program checks the condition. If it’s true, it executes the code within the ‘if’ block. If it’s false, it executes the code within the ‘else’ block.
Understanding Conditional Statements
Conditional statements are the building blocks of control flow. They allow your program to make decisions, repeat actions, and handle complex logic. The ‘if-else’ statement is one of the most common conditional statements, but there are others, like ‘switch’ and ‘for’, each with its unique use cases.
By mastering ‘if-else’ and other conditional statements, you’ll be able to write Java programs that can handle complex tasks, making you a more effective and versatile developer.
The ‘if-else’ statement isn’t just for simple programs or exercises. It’s a fundamental tool that you’ll use in almost every Java project, no matter how large or complex.
Integrating If-Else with Loops
In larger projects, you’ll often find ‘if-else’ statements used in conjunction with loops. For example, you might use a ‘for’ or ‘while’ loop to iterate over a collection of items, and an ‘if-else’ statement to perform different actions based on each item.
In this example, the program uses a ‘for’ loop to iterate over the numbers 0 through 9. For each number, it uses an ‘if-else’ statement to check if the number is even or odd, and prints a message accordingly.
If-Else and Exception Handling
‘If-else’ statements are also often used in exception handling, a crucial part of any Java program. You might use an ‘if-else’ statement to check if a certain condition is met before performing an action that could throw an exception.
In this example, the program uses an ‘if-else’ statement to check if ‘str’ is not null before trying to get its length. If ‘str’ is null, it prints a warning message instead of throwing a NullPointerException.
Further Resources for Mastering If-Else in Java
If you want to dive deeper into the ‘if-else’ statement in Java and related topics, here are some resources to check out:
- IOFlood’s Complete Guide to Java Loops explores nested loops for complex iteration patterns in Java.
Java Switch Statement – Explore the switch statement in Java for multi-branch decision-making.
Using Else-If in Java – Master the else-if construct for complex decision-making in Java programs.
Java if-else statement by Oracle : Oracle’s official Java tutorials offer exhaustive information on if-else statements.
Java if-else by JavaTpoint explains using if-else conditions in Java, with easy-to-understand examples.
Java if-else statement with examples by GeeksforGeeks includes a variety of examples and use cases.
In this comprehensive guide, we’ve explored the ins and outs of ‘if-else’ statements in Java, from the basics to more advanced usage scenarios.
We began with the basics, learning how to use ‘if-else’ statements to control the flow of a Java program. We then delved deeper, tackling more complex structures like nested ‘if-else’ and ‘if-else-if’ ladders. Along the way, we also discussed common issues that you might encounter when using ‘if-else’ statements and provided solutions to these problems.
We didn’t stop at ‘if-else’ statements, though. We also explored alternative approaches to control flow in Java, such as using ‘switch’ statements. We compared these methods, giving you a sense of the broader landscape of tools for managing control flow in Java.
Here’s a quick comparison of the methods we’ve discussed:
Whether you’re just starting out with Java or you’re looking to level up your control flow skills, we hope this guide has given you a deeper understanding of ‘if-else’ statements and their alternatives.
With a solid grasp of ‘if-else’ statements, you’ll be well-equipped to write Java programs that can handle complex decision-making. Happy coding!
About Author

Gabriel Ramuglia
Gabriel is the owner and founder of IOFLOOD.com , an unmanaged dedicated server hosting company operating since 2010.Gabriel loves all things servers, bandwidth, and computer programming and enjoys sharing his experience on these topics with readers of the IOFLOOD blog.
Related Posts
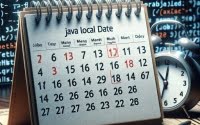
- Java Arrays
- Java Strings
- Java Collection
- Java 8 Tutorial
- Java Multithreading
- Java Exception Handling
- Java Programs
- Java Project
- Java Collections Interview
- Java Interview Questions
- Spring Boot
- Java Tutorial
Overview of Java
- Introduction to Java
- The Complete History of Java Programming Language
- C++ vs Java vs Python
- How to Download and Install Java for 64 bit machine?
- Setting up the environment in Java
- How to Download and Install Eclipse on Windows?
- JDK in Java
- How JVM Works - JVM Architecture?
- Differences between JDK, JRE and JVM
- Just In Time Compiler
- Difference between JIT and JVM in Java
- Difference between Byte Code and Machine Code
- How is Java platform independent?
Basics of Java
- Java Basic Syntax
- Java Hello World Program
- Java Data Types
- Primitive data type vs. Object data type in Java with Examples
- Java Identifiers
Operators in Java
- Java Variables
- Scope of Variables In Java
Wrapper Classes in Java
Input/output in java.
- How to Take Input From User in Java?
- Scanner Class in Java
- Java.io.BufferedReader Class in Java
- Difference Between Scanner and BufferedReader Class in Java
- Ways to read input from console in Java
- System.out.println in Java
- Difference between print() and println() in Java
- Formatted Output in Java using printf()
- Fast I/O in Java in Competitive Programming
Flow Control in Java
- Decision Making in Java (if, if-else, switch, break, continue, jump)
- Java if statement with Examples
- Java if-else
- Java if-else-if ladder with Examples
- Loops in Java
- For Loop in Java
- Java while loop with Examples
- Java do-while loop with Examples
- For-each loop in Java
- Continue Statement in Java
- Break statement in Java
- Usage of Break keyword in Java
- return keyword in Java
- Java Arithmetic Operators with Examples
- Java Unary Operator with Examples
Java Assignment Operators with Examples
- Java Relational Operators with Examples
- Java Logical Operators with Examples
- Java Ternary Operator with Examples
- Bitwise Operators in Java
- Strings in Java
- String class in Java
- Java.lang.String class in Java | Set 2
- Why Java Strings are Immutable?
- StringBuffer class in Java
- StringBuilder Class in Java with Examples
- String vs StringBuilder vs StringBuffer in Java
- StringTokenizer Class in Java
- StringTokenizer Methods in Java with Examples | Set 2
- StringJoiner Class in Java
- Arrays in Java
- Arrays class in Java
- Multidimensional Arrays in Java
- Different Ways To Declare And Initialize 2-D Array in Java
- Jagged Array in Java
- Final Arrays in Java
- Reflection Array Class in Java
- util.Arrays vs reflect.Array in Java with Examples
OOPS in Java
- Object Oriented Programming (OOPs) Concept in Java
- Why Java is not a purely Object-Oriented Language?
- Classes and Objects in Java
- Naming Conventions in Java
- Java Methods
Access Modifiers in Java
- Java Constructors
- Four Main Object Oriented Programming Concepts of Java
Inheritance in Java
Abstraction in java, encapsulation in java, polymorphism in java, interfaces in java.
- 'this' reference in Java
- Inheritance and Constructors in Java
- Java and Multiple Inheritance
- Interfaces and Inheritance in Java
- Association, Composition and Aggregation in Java
- Comparison of Inheritance in C++ and Java
- abstract keyword in java
- Abstract Class in Java
- Difference between Abstract Class and Interface in Java
- Control Abstraction in Java with Examples
- Difference Between Data Hiding and Abstraction in Java
- Difference between Abstraction and Encapsulation in Java with Examples
- Difference between Inheritance and Polymorphism
- Dynamic Method Dispatch or Runtime Polymorphism in Java
- Difference between Compile-time and Run-time Polymorphism in Java
Constructors in Java
- Copy Constructor in Java
- Constructor Overloading in Java
- Constructor Chaining In Java with Examples
- Private Constructors and Singleton Classes in Java
Methods in Java
- Static methods vs Instance methods in Java
- Abstract Method in Java with Examples
- Overriding in Java
- Method Overloading in Java
- Difference Between Method Overloading and Method Overriding in Java
- Differences between Interface and Class in Java
- Functional Interfaces in Java
- Nested Interface in Java
- Marker interface in Java
- Comparator Interface in Java with Examples
- Need of Wrapper Classes in Java
- Different Ways to Create the Instances of Wrapper Classes in Java
- Character Class in Java
- Java.Lang.Byte class in Java
- Java.Lang.Short class in Java
- Java.lang.Integer class in Java
- Java.Lang.Long class in Java
- Java.Lang.Float class in Java
- Java.Lang.Double Class in Java
- Java.lang.Boolean Class in Java
- Autoboxing and Unboxing in Java
- Type conversion in Java with Examples
Keywords in Java
- Java Keywords
- Important Keywords in Java
- Super Keyword in Java
- final Keyword in Java
- static Keyword in Java
- enum in Java
- transient keyword in Java
- volatile Keyword in Java
- final, finally and finalize in Java
- Public vs Protected vs Package vs Private Access Modifier in Java
- Access and Non Access Modifiers in Java
Memory Allocation in Java
- Java Memory Management
- How are Java objects stored in memory?
- Stack vs Heap Memory Allocation
- How many types of memory areas are allocated by JVM?
- Garbage Collection in Java
- Types of JVM Garbage Collectors in Java with implementation details
- Memory leaks in Java
- Java Virtual Machine (JVM) Stack Area
Classes of Java
- Understanding Classes and Objects in Java
- Singleton Method Design Pattern in Java
- Object Class in Java
- Inner Class in Java
- Throwable Class in Java with Examples
Packages in Java
- Packages In Java
- How to Create a Package in Java?
- Java.util Package in Java
- Java.lang package in Java
- Java.io Package in Java
- Java Collection Tutorial
Exception Handling in Java
- Exceptions in Java
- Types of Exception in Java with Examples
- Checked vs Unchecked Exceptions in Java
- Java Try Catch Block
- Flow control in try catch finally in Java
- throw and throws in Java
- User-defined Custom Exception in Java
- Chained Exceptions in Java
- Null Pointer Exception In Java
- Exception Handling with Method Overriding in Java
- Multithreading in Java
- Lifecycle and States of a Thread in Java
- Java Thread Priority in Multithreading
- Main thread in Java
- Java.lang.Thread Class in Java
- Runnable interface in Java
- Naming a thread and fetching name of current thread in Java
- What does start() function do in multithreading in Java?
- Difference between Thread.start() and Thread.run() in Java
- Thread.sleep() Method in Java With Examples
- Synchronization in Java
- Importance of Thread Synchronization in Java
- Method and Block Synchronization in Java
- Lock framework vs Thread synchronization in Java
- Difference Between Atomic, Volatile and Synchronized in Java
- Deadlock in Java Multithreading
- Deadlock Prevention And Avoidance
- Difference Between Lock and Monitor in Java Concurrency
- Reentrant Lock in Java
File Handling in Java
- Java.io.File Class in Java
- Java Program to Create a New File
- Different ways of Reading a text file in Java
- Java Program to Write into a File
- Delete a File Using Java
- File Permissions in Java
- FileWriter Class in Java
- Java.io.FileDescriptor in Java
- Java.io.RandomAccessFile Class Method | Set 1
- Regular Expressions in Java
- Regex Tutorial - How to write Regular Expressions?
- Matcher pattern() method in Java with Examples
- Pattern pattern() method in Java with Examples
- Quantifiers in Java
- java.lang.Character class methods | Set 1
- Java IO : Input-output in Java with Examples
- Java.io.Reader class in Java
- Java.io.Writer Class in Java
- Java.io.FileInputStream Class in Java
- FileOutputStream in Java
- Java.io.BufferedOutputStream class in Java
- Java Networking
- TCP/IP Model
- User Datagram Protocol (UDP)
- Differences between IPv4 and IPv6
- Difference between Connection-oriented and Connection-less Services
- Socket Programming in Java
- java.net.ServerSocket Class in Java
- URL Class in Java with Examples
JDBC - Java Database Connectivity
- Introduction to JDBC (Java Database Connectivity)
- JDBC Drivers
- Establishing JDBC Connection in Java
- Types of Statements in JDBC
- JDBC Tutorial
- Java 8 Features - Complete Tutorial
Operators constitute the basic building block of any programming language. Java too provides many types of operators which can be used according to the need to perform various calculations and functions, be it logical, arithmetic, relational, etc. They are classified based on the functionality they provide.
Types of Operators:
- Arithmetic Operators
- Unary Operators
- Assignment Operator
- Relational Operators
- Logical Operators
- Ternary Operator
- Bitwise Operators
- Shift Operators
This article explains all that one needs to know regarding Assignment Operators.
Assignment Operators
These operators are used to assign values to a variable. The left side operand of the assignment operator is a variable, and the right side operand of the assignment operator is a value. The value on the right side must be of the same data type of the operand on the left side. Otherwise, the compiler will raise an error. This means that the assignment operators have right to left associativity, i.e., the value given on the right-hand side of the operator is assigned to the variable on the left. Therefore, the right-hand side value must be declared before using it or should be a constant. The general format of the assignment operator is,
Types of Assignment Operators in Java
The Assignment Operator is generally of two types. They are:
1. Simple Assignment Operator: The Simple Assignment Operator is used with the “=” sign where the left side consists of the operand and the right side consists of a value. The value of the right side must be of the same data type that has been defined on the left side.
2. Compound Assignment Operator: The Compound Operator is used where +,-,*, and / is used along with the = operator.
Let’s look at each of the assignment operators and how they operate:
1. (=) operator:
This is the most straightforward assignment operator, which is used to assign the value on the right to the variable on the left. This is the basic definition of an assignment operator and how it functions.
Syntax:
Example:
2. (+=) operator:
This operator is a compound of ‘+’ and ‘=’ operators. It operates by adding the current value of the variable on the left to the value on the right and then assigning the result to the operand on the left.
Note: The compound assignment operator in Java performs implicit type casting. Let’s consider a scenario where x is an int variable with a value of 5. int x = 5; If you want to add the double value 4.5 to the integer variable x and print its value, there are two methods to achieve this: Method 1: x = x + 4.5 Method 2: x += 4.5 As per the previous example, you might think both of them are equal. But in reality, Method 1 will throw a runtime error stating the “i ncompatible types: possible lossy conversion from double to int “, Method 2 will run without any error and prints 9 as output.
Reason for the Above Calculation
Method 1 will result in a runtime error stating “incompatible types: possible lossy conversion from double to int.” The reason is that the addition of an int and a double results in a double value. Assigning this double value back to the int variable x requires an explicit type casting because it may result in a loss of precision. Without the explicit cast, the compiler throws an error. Method 2 will run without any error and print the value 9 as output. The compound assignment operator += performs an implicit type conversion, also known as an automatic narrowing primitive conversion from double to int . It is equivalent to x = (int) (x + 4.5) , where the result of the addition is explicitly cast to an int . The fractional part of the double value is truncated, and the resulting int value is assigned back to x . It is advisable to use Method 2 ( x += 4.5 ) to avoid runtime errors and to obtain the desired output.
Same automatic narrowing primitive conversion is applicable for other compound assignment operators as well, including -= , *= , /= , and %= .
3. (-=) operator:
This operator is a compound of ‘-‘ and ‘=’ operators. It operates by subtracting the variable’s value on the right from the current value of the variable on the left and then assigning the result to the operand on the left.
4. (*=) operator:
This operator is a compound of ‘*’ and ‘=’ operators. It operates by multiplying the current value of the variable on the left to the value on the right and then assigning the result to the operand on the left.
5. (/=) operator:
This operator is a compound of ‘/’ and ‘=’ operators. It operates by dividing the current value of the variable on the left by the value on the right and then assigning the quotient to the operand on the left.
6. (%=) operator:
This operator is a compound of ‘%’ and ‘=’ operators. It operates by dividing the current value of the variable on the left by the value on the right and then assigning the remainder to the operand on the left.
Please Login to comment...
Similar reads.
- Java-Operators
- 10 Ways to Use Slack for Effective Communication
- 10 Ways to Use Google Docs for Collaborative Writing
- NEET MDS 2024 Result: Toppers List, Category-wise Cutoff, and Important Dates
- NDA Admit Card 2024 Live Updates: Download Your Hall Ticket Soon on upsc.gov.in!
- 30 OOPs Interview Questions and Answers (2024)
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
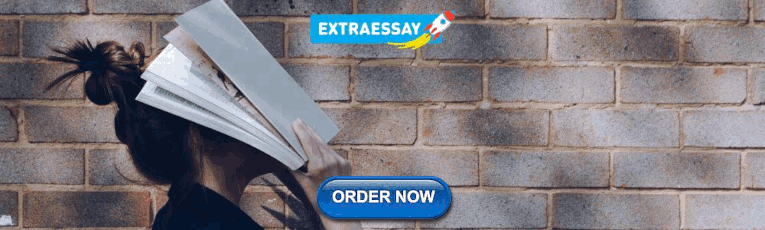
COMMENTS
Stack Overflow Public questions & answers; Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers; Talent Build your employer brand ; Advertising Reach developers & technologists worldwide; Labs The future of collective knowledge sharing; About the company
Output. The number is positive. Statement outside if...else block. In the above example, we have a variable named number.Here, the test expression number > 0 checks if number is greater than 0.. Since the value of the number is 10, the test expression evaluates to true.Hence code inside the body of if is executed.. Now, change the value of the number to a negative integer.
Java has the following conditional statements: Use if to specify a block of code to be executed, if a specified condition is true. Use else to specify a block of code to be executed, if the same condition is false. Use else if to specify a new condition to test, if the first condition is false. Use switch to specify many alternative blocks of ...
Java if-else. Decision-making in Java helps to write decision-driven statements and execute a particular set of code based on certain conditions. The if statement alone tells us that if a condition is true it will execute a block of statements and if the condition is false it won't. In this article, we will learn about Java if-else.
The if-then Statement. The if-then statement is the most basic of all the control flow statements. It tells your program to execute a certain section of code only if a particular test evaluates to true.For example, the Bicycle class could allow the brakes to decrease the bicycle's speed only if the bicycle is already in motion. One possible implementation of the applyBrakes method could be as ...
The if-else statement is the most basic of all control structures, and it's likely also the most common decision-making statement in programming. It allows us to execute a certain code section only if a specific condition is met. 2. Syntax of If-Else. The if statement always needs a boolean expression as its parameter.
The if-else statement in Java is the most basic of all the flow control statements.An if-else statement tells the program to execute a certain block only if a particular test evaluates to true, else execute the alternate block if the condition is false.. The if and else are reserved keywords in Java, and cannot be used as other identifiers.. 1. Syntax. A simple if-else statement is written as ...
Else. An else clause can be added to an if statement. The else statement executes a block of code when the condition inside the if statement is false: If the condition evaluates to true, code in the if part is executed. If the condition evaluates to false, code in the else part is executed. The else statement is always the last condition.
Overall, the if-else statement is a fundamental tool in programming that provides a way to control the flow of a program based on conditions. It helps to improve the readability, reusability, debuggability, and flexibility of the code. Related Articles: Decision Making in Java; Java if-else statement with Examples; Java if-else-if ladder with ...
Java Short Hand If...Else (Ternary Operator) Previous Next Short Hand If...Else. There is also a short-hand if else, which is known as the ternary operator because it consists of three operands. It can be used to replace multiple lines of code with a single line, and is most often used to replace simple if else statements: ...
Java If-else Statement. The Java if statement is used to test the condition. It checks boolean condition: true or false.There are various types of if statement in Java. if statement; if-else statement; if-else-if ladder; nested if statement
Ternary Operator in Java One Line if-else Statement Using filter in Java 8 ; Conclusion The if-else statement in Java is a fundamental construct used to conditionally execute blocks of code based on certain conditions. However, it often requires multiple lines to define a simple if-else block, which may not always be ideal, especially for concise and readable code.
Java if-else statement by Oracle: Oracle's official Java tutorials offer exhaustive information on if-else statements. Java if-else by JavaTpoint explains using if-else conditions in Java, with easy-to-understand examples. Java if-else statement with examples by GeeksforGeeks includes a variety of examples and use cases.
There are two methods I know of that you can declare a variable's value by conditions. Method 1: If the condition evaluates to true, the value on the left side of the column would be assigned to the variable. If the condition evaluates to false the condition on the right will be assigned to the variable. You can also nest many conditions into ...
These are used to cause the flow of execution to advance and branch based on changes to the state of a program. Java's Selection statements: if. if-else. nested-if. if-else-if. switch-case. jump - break, continue, return. 1. if: if statement is the most simple decision-making statement.
Write a Java program to solve quadratic equations (use if, else if and else). Test Data Input a: 1 Input b: 5 Input c: 1 Expected Output: The roots are -0.20871215252208009 and -4.7912878474779195 Click me to see the solution. 3. Write a Java program that takes three numbers from the user and prints the greatest number. Test Data Input the 1st ...
Decision Making in Java helps to write decision-driven statements and execute a particular set of code based on certain conditions. Java if-else-if ladder is used to decide among multiple options. The if statements are executed from the top down. As soon as one of the conditions controlling the if is true, the statement associated with that if is executed, and the rest of the ladder is bypassed.
Assignment in a conditional statement is valid in javascript, because your just asking "if assignment is valid, do something which possibly includes the result of the assignment". But indeed, assigning before the conditional is also valid, not too verbose, and more commonly used. - okdewit.
My assignment is to create several methods for the program. I seem to be stuck on these two the most. Specifications are as follows: Need to use if n else statement name: setZipCode arguments: 1 int returns: boolean. what it does: stores its argument as the address' zip code. If the zip code is not a number between 90000 and 92000 (inclusive ...
Java string assignment from if/else statement. Ask Question Asked 11 years ago. Modified 11 years ago. Viewed 1k times 0 I'm fairly new to java, and I've been trying to debug this code for some time now. I figured there must be something that I don't understand fully about Strings, so I thought I'd bite the bullet and ask on Stackoverflow.
Note: The compound assignment operator in Java performs implicit type casting. Let's consider a scenario where x is an int variable with a value of 5. int x = 5; If you want to add the double value 4.5 to the integer variable x and print its value, there are two methods to achieve this: Method 1: x = x + 4.5. Method 2: x += 4.5.