Java Tutorial
Java methods, java classes, java file handling, java how to, java reference, java examples, java operators.
Operators are used to perform operations on variables and values.
In the example below, we use the + operator to add together two values:
Try it Yourself »
Although the + operator is often used to add together two values, like in the example above, it can also be used to add together a variable and a value, or a variable and another variable:
Java divides the operators into the following groups:
- Arithmetic operators
- Assignment operators
- Comparison operators
- Logical operators
- Bitwise operators
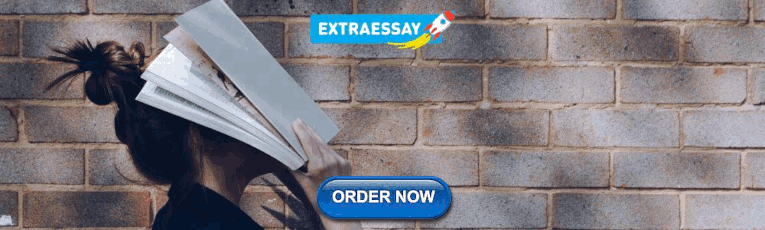
Arithmetic Operators
Arithmetic operators are used to perform common mathematical operations.
Advertisement
Java Assignment Operators
Assignment operators are used to assign values to variables.
In the example below, we use the assignment operator ( = ) to assign the value 10 to a variable called x :
The addition assignment operator ( += ) adds a value to a variable:
A list of all assignment operators:
Java Comparison Operators
Comparison operators are used to compare two values (or variables). This is important in programming, because it helps us to find answers and make decisions.
The return value of a comparison is either true or false . These values are known as Boolean values , and you will learn more about them in the Booleans and If..Else chapter.
In the following example, we use the greater than operator ( > ) to find out if 5 is greater than 3:
Java Logical Operators
You can also test for true or false values with logical operators.
Logical operators are used to determine the logic between variables or values:
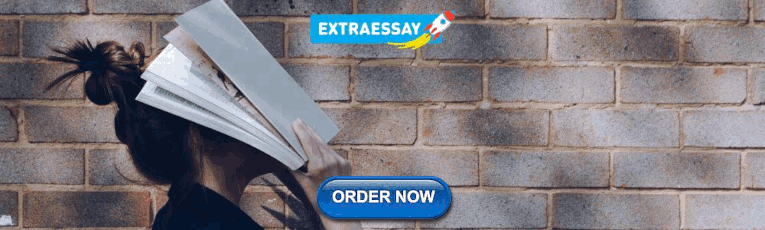
Java Bitwise Operators
Bitwise operators are used to perform binary logic with the bits of an integer or long integer.
Note: The Bitwise examples above use 4-bit unsigned examples, but Java uses 32-bit signed integers and 64-bit signed long integers. Because of this, in Java, ~5 will not return 10. It will return -6. ~00000000000000000000000000000101 will return 11111111111111111111111111111010
In Java, 9 >> 1 will not return 12. It will return 4. 00000000000000000000000000001001 >> 1 will return 00000000000000000000000000000100
Test Yourself With Exercises
Multiply 10 with 5 , and print the result.
Start the Exercise

COLOR PICKER

Report Error
If you want to report an error, or if you want to make a suggestion, do not hesitate to send us an e-mail:
Top Tutorials
Top references, top examples, get certified.
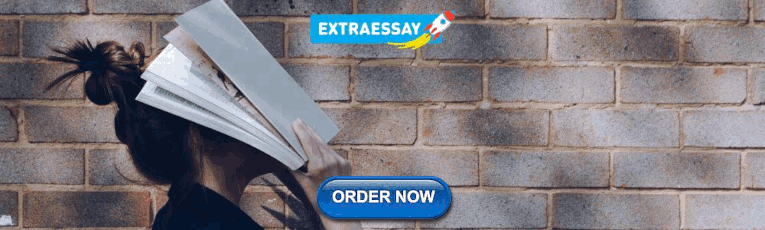
IMAGES
VIDEO
COMMENTS
Java Comparison Operators. Comparison operators are used to compare two values (or variables). This is important in programming, because it helps us to find answers and make decisions. The return value of a comparison is either true or false. These values are known as Boolean values, and you will learn more about them in the Booleans and If ...