- C Data Types
- C Operators
- C Input and Output
- C Control Flow
- C Functions
- C Preprocessors
- C File Handling
- C Cheatsheet
- C Interview Questions
- Solve Coding Problems
- getch() function in C with Examples
- C Program to Print Armstrong Numbers Between 1 to 1000
- rand() in C
- Else without IF and L-Value Required Error in C
- C program to check if a given string is Keyword or not
- Reverse String in C
- Discrete Fourier Transform and its Inverse using C
- time.h header file in C with Examples
- How to use gotoxy() in codeblocks?
- Program For Newton’s Forward Interpolation
- How to avoid Structure Padding in C?
- C Program to Reverse a String Using Recursion
- C Program To Remove Duplicates From Sorted Array
- C Program to Find Determinant of a Matrix
- Concatenating Two Strings in C
- C Program to Convert Decimal to Octal
- Convert Binary to Decimal in C
- C Program to Add N Distances Given in inch-feet System using Structures
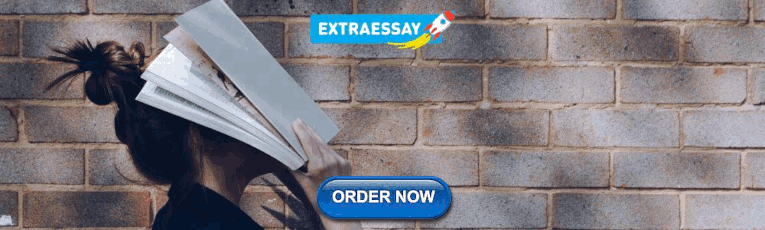
C Exercises – Practice Questions with Solutions for C Programming
The best way to learn C programming language is by hands-on practice. This C Exercise page contains the top 30 C exercise questions with solutions that are designed for both beginners and advanced programmers. It covers all major concepts like arrays, pointers, for-loop, and many more.
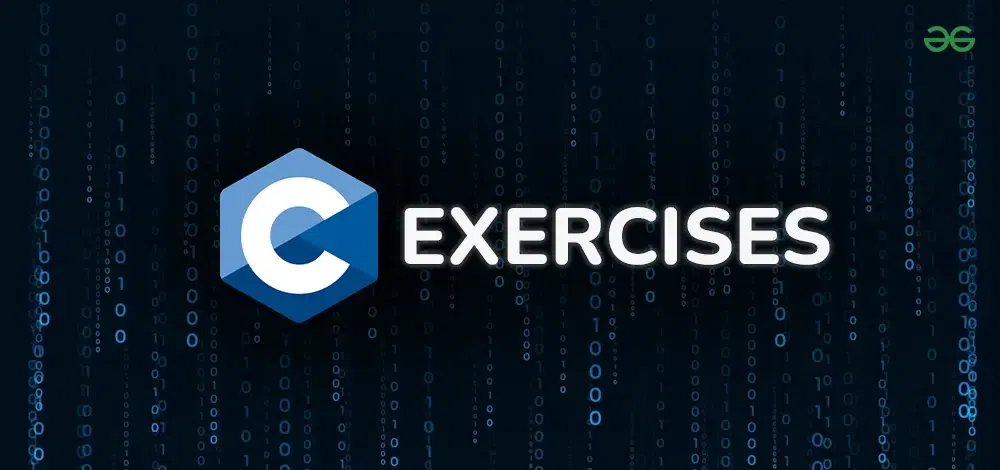
So, Keep it Up! Solve topic-wise C exercise questions to strengthen your weak topics.
C Programming Exercises
The following are the top 30 programming exercises with solutions to help you practice online and improve your coding efficiency in the C language. You can solve these questions online in GeeksforGeeks IDE.
Q1: Write a Program to Print “Hello World!” on the Console.
In this problem, you have to write a simple program that prints “Hello World!” on the console screen.
For Example,
Click here to view the solution.
Q2: write a program to find the sum of two numbers entered by the user..
In this problem, you have to write a program that adds two numbers and prints their sum on the console screen.
Q3: Write a Program to find the size of int, float, double, and char.
In this problem, you have to write a program to print the size of the variable.
Q4: Write a Program to Swap the values of two variables.
In this problem, you have to write a program that swaps the values of two variables that are entered by the user.
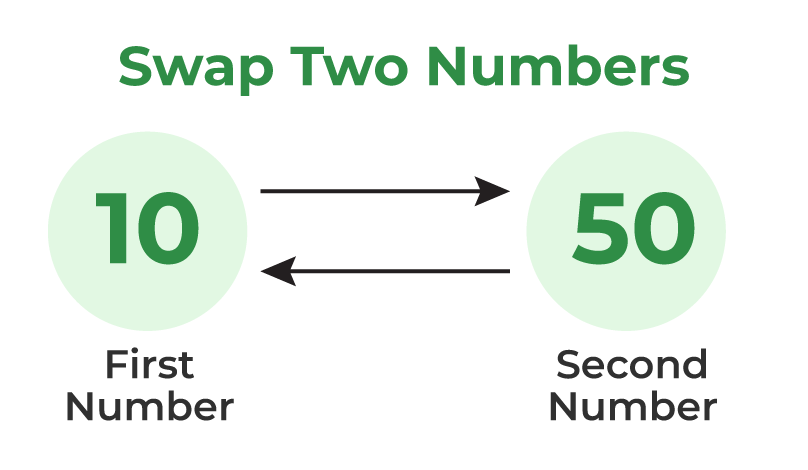
Swap two numbers
Q5: Write a Program to calculate Compound Interest.
In this problem, you have to write a program that takes principal, time, and rate as user input and calculates the compound interest.
Q6: Write a Program to check if the given number is Even or Odd.
In this problem, you have to write a program to check whether the given number is even or odd.
Q7: Write a Program to find the largest number among three numbers.
In this problem, you have to write a program to take three numbers from the user as input and print the largest number among them.
Q8: Write a Program to make a simple calculator.
In this problem, you have to write a program to make a simple calculator that accepts two operands and an operator to perform the calculation and prints the result.
Q9: Write a Program to find the factorial of a given number.
In this problem, you have to write a program to calculate the factorial (product of all the natural numbers less than or equal to the given number n) of a number entered by the user.
Q10: Write a Program to Convert Binary to Decimal.
In this problem, you have to write a program to convert the given binary number entered by the user into an equivalent decimal number.
Q11: Write a Program to print the Fibonacci series using recursion.
In this problem, you have to write a program to print the Fibonacci series(the sequence where each number is the sum of the previous two numbers of the sequence) till the number entered by the user using recursion.
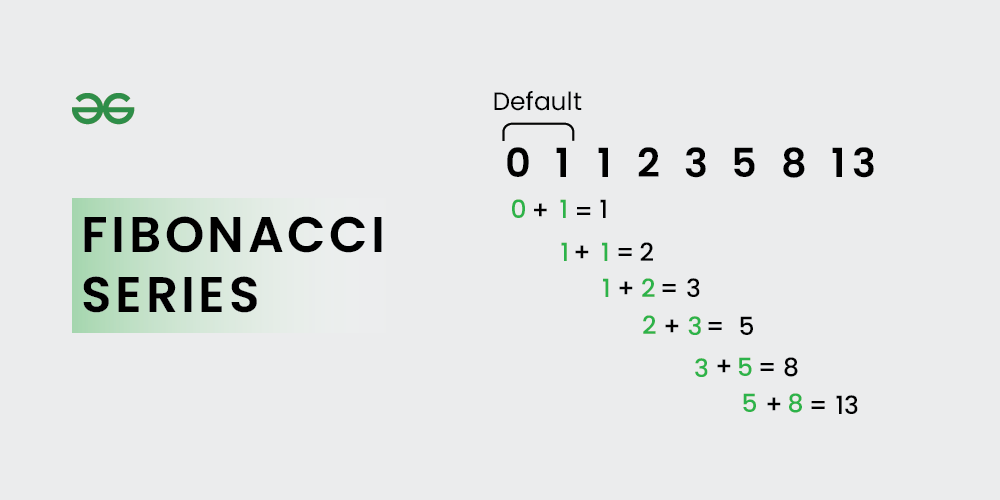
Fibonacci Series
Q12: Write a Program to Calculate the Sum of Natural Numbers using recursion.
In this problem, you have to write a program to calculate the sum of natural numbers up to a given number n.
Q13: Write a Program to find the maximum and minimum of an Array.
In this problem, you have to write a program to find the maximum and the minimum element of the array of size N given by the user.
Q14: Write a Program to Reverse an Array.
In this problem, you have to write a program to reverse an array of size n entered by the user. Reversing an array means changing the order of elements so that the first element becomes the last element and the second element becomes the second last element and so on.
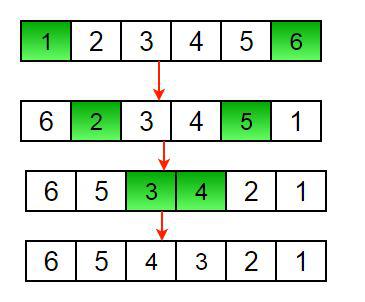
Reverse an array
Q15: Write a Program to rotate the array to the left.
In this problem, you have to write a program that takes an array arr[] of size N from the user and rotates the array to the left (counter-clockwise direction) by D steps, where D is a positive integer.
Q16: Write a Program to remove duplicates from the Sorted array.
In this problem, you have to write a program that takes a sorted array arr[] of size N from the user and removes the duplicate elements from the array.
Q17: Write a Program to search elements in an array (using Binary Search).
In this problem, you have to write a program that takes an array arr[] of size N and a target value to be searched by the user. Search the target value using binary search if the target value is found print its index else print ‘element is not present in array ‘.
Q18: Write a Program to reverse a linked list.
In this problem, you have to write a program that takes a pointer to the head node of a linked list, you have to reverse the linked list and print the reversed linked list.
Q18: Write a Program to create a dynamic array in C.
In this problem, you have to write a program to create an array of size n dynamically then take n elements of an array one by one by the user. Print the array elements.
Q19: Write a Program to find the Transpose of a Matrix.
In this problem, you have to write a program to find the transpose of a matrix for a given matrix A with dimensions m x n and print the transposed matrix. The transpose of a matrix is formed by interchanging its rows with columns.
Q20: Write a Program to concatenate two strings.
In this problem, you have to write a program to read two strings str1 and str2 entered by the user and concatenate these two strings. Print the concatenated string.
Q21: Write a Program to check if the given string is a palindrome string or not.
In this problem, you have to write a program to read a string str entered by the user and check whether the string is palindrome or not. If the str is palindrome print ‘str is a palindrome’ else print ‘str is not a palindrome’. A string is said to be palindrome if the reverse of the string is the same as the string.
Q22: Write a program to print the first letter of each word.
In this problem, you have to write a simple program to read a string str entered by the user and print the first letter of each word in a string.
Q23: Write a program to reverse a string using recursion
In this problem, you have to write a program to read a string str entered by the user, and reverse that string means changing the order of characters in the string so that the last character becomes the first character of the string using recursion.
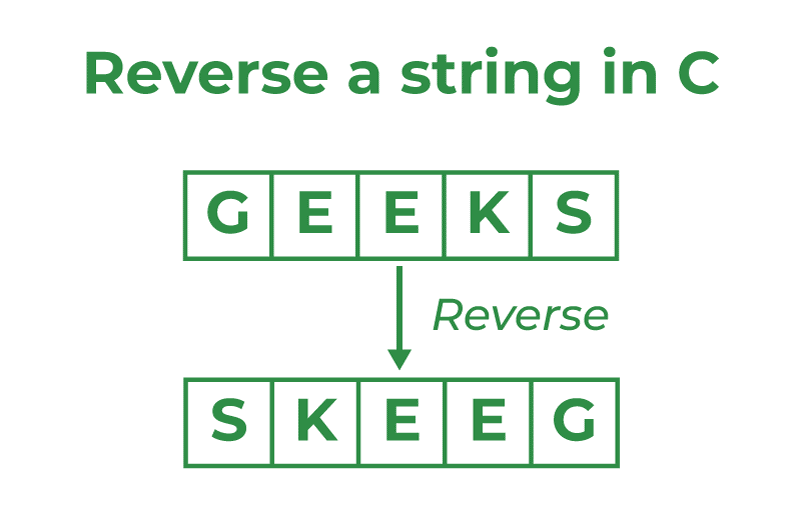
reverse a string
Q24: Write a program to Print Half half-pyramid pattern.
In this problem, you have to write a simple program to read the number of rows (n) entered by the user and print the half-pyramid pattern of numbers. Half pyramid pattern looks like a right-angle triangle of numbers having a hypotenuse on the right side.
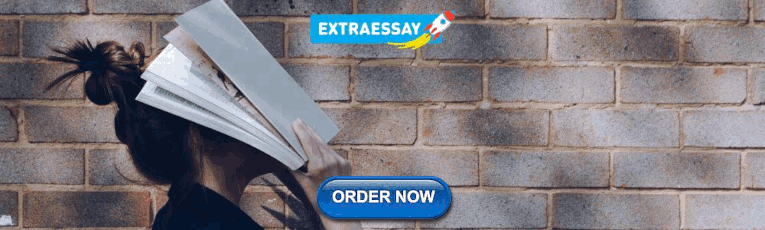
Q25: Write a program to print Pascal’s triangle pattern.
In this problem, you have to write a simple program to read the number of rows (n) entered by the user and print Pascal’s triangle pattern. Pascal’s Triangle is a pattern in which the first row has a single number 1 all rows begin and end with the number 1. The numbers in between are obtained by adding the two numbers directly above them in the previous row.
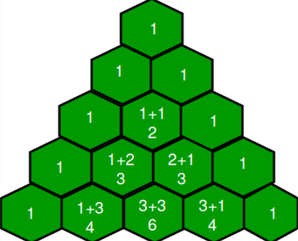
Pascal’s Triangle
Q26: Write a program to sort an array using Insertion Sort.
In this problem, you have to write a program that takes an array arr[] of size N from the user and sorts the array elements in ascending or descending order using insertion sort.
Q27: Write a program to sort an array using Quick Sort.
In this problem, you have to write a program that takes an array arr[] of size N from the user and sorts the array elements in ascending order using quick sort.
Q28: Write a program to sort an array of strings.
In this problem, you have to write a program that reads an array of strings in which all characters are of the same case entered by the user and sort them alphabetically.
Q29: Write a program to copy the contents of one file to another file.
In this problem, you have to write a program that takes user input to enter the filenames for reading and writing. Read the contents of one file and copy the content to another file. If the file specified for reading does not exist or cannot be opened, display an error message “Cannot open file: file_name” and terminate the program else print “Content copied to file_name”
Q30: Write a program to store information on students using structure.
In this problem, you have to write a program that stores information about students using structure. The program should create various structures, each representing a student’s record. Initialize the records with sample data having data members’ Names, Roll Numbers, Ages, and Total Marks. Print the information for each student.
We hope after completing these C exercises you have gained a better understanding of C concepts. Learning C language is made easier with this exercise sheet as it helps you practice all major C concepts. Solving these C exercise questions will take you a step closer to becoming a C programmer.
Frequently Asked Questions (FAQs)
Q1. what are some common mistakes to avoid while doing c programming exercises.
Some of the most common mistakes made by beginners doing C programming exercises can include missing semicolons, bad logic loops, uninitialized pointers, and forgotten memory frees etc.
Q2. What are the best practices for beginners starting with C programming exercises?
Best practices for beginners starting with C programming exercises: Start with easy codes Practice consistently Be creative Think before you code Learn from mistakes Repeat!
Q3. How do I debug common errors in C programming exercises?
You can use the following methods to debug a code in C programming exercises Read the error message carefully Read code line by line Try isolating the error code Look for Missing elements, loops, pointers, etc Check error online
Please Login to comment...
- WhatsApp To Launch New App Lock Feature
- Top Design Resources for Icons
- Node.js 21 is here: What’s new
- Zoom: World’s Most Innovative Companies of 2024
- 30 OOPs Interview Questions and Answers (2024)
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
- Skip to main content
- Skip to primary sidebar
- Skip to footer
Additional menu
Khan Academy Blog
Free Math Worksheets — Over 100k free practice problems on Khan Academy
Looking for free math worksheets.
You’ve found something even better!
That’s because Khan Academy has over 100,000 free practice questions. And they’re even better than traditional math worksheets – more instantaneous, more interactive, and more fun!
Just choose your grade level or topic to get access to 100% free practice questions:
Kindergarten, basic geometry, pre-algebra, algebra basics, high school geometry.
- Trigonometry
Statistics and probability
High school statistics, ap®︎/college statistics, precalculus, differential calculus, integral calculus, ap®︎/college calculus ab, ap®︎/college calculus bc, multivariable calculus, differential equations, linear algebra.
- Addition and subtraction
- Place value (tens and hundreds)
- Addition and subtraction within 20
- Addition and subtraction within 100
- Addition and subtraction within 1000
- Measurement and data
- Counting and place value
- Measurement and geometry
- Place value
- Measurement, data, and geometry
- Add and subtract within 20
- Add and subtract within 100
- Add and subtract within 1,000
- Money and time
- Measurement
- Intro to multiplication
- 1-digit multiplication
- Addition, subtraction, and estimation
- Intro to division
- Understand fractions
- Equivalent fractions and comparing fractions
- More with multiplication and division
- Arithmetic patterns and problem solving
- Quadrilaterals
- Represent and interpret data
- Multiply by 1-digit numbers
- Multiply by 2-digit numbers
- Factors, multiples and patterns
- Add and subtract fractions
- Multiply fractions
- Understand decimals
- Plane figures
- Measuring angles
- Area and perimeter
- Units of measurement
- Decimal place value
- Add decimals
- Subtract decimals
- Multi-digit multiplication and division
- Divide fractions
- Multiply decimals
- Divide decimals
- Powers of ten
- Coordinate plane
- Algebraic thinking
- Converting units of measure
- Properties of shapes
- Ratios, rates, & percentages
- Arithmetic operations
- Negative numbers
- Properties of numbers
- Variables & expressions
- Equations & inequalities introduction
- Data and statistics
- Negative numbers: addition and subtraction
- Negative numbers: multiplication and division
- Fractions, decimals, & percentages
- Rates & proportional relationships
- Expressions, equations, & inequalities
- Numbers and operations
- Solving equations with one unknown
- Linear equations and functions
- Systems of equations
- Geometric transformations
- Data and modeling
- Volume and surface area
- Pythagorean theorem
- Transformations, congruence, and similarity
- Arithmetic properties
- Factors and multiples
- Reading and interpreting data
- Negative numbers and coordinate plane
- Ratios, rates, proportions
- Equations, expressions, and inequalities
- Exponents, radicals, and scientific notation
- Foundations
- Algebraic expressions
- Linear equations and inequalities
- Graphing lines and slope
- Expressions with exponents
- Quadratics and polynomials
- Equations and geometry
- Algebra foundations
- Solving equations & inequalities
- Working with units
- Linear equations & graphs
- Forms of linear equations
- Inequalities (systems & graphs)
- Absolute value & piecewise functions
- Exponents & radicals
- Exponential growth & decay
- Quadratics: Multiplying & factoring
- Quadratic functions & equations
- Irrational numbers
- Performing transformations
- Transformation properties and proofs
- Right triangles & trigonometry
- Non-right triangles & trigonometry (Advanced)
- Analytic geometry
- Conic sections
- Solid geometry
- Polynomial arithmetic
- Complex numbers
- Polynomial factorization
- Polynomial division
- Polynomial graphs
- Rational exponents and radicals
- Exponential models
- Transformations of functions
- Rational functions
- Trigonometric functions
- Non-right triangles & trigonometry
- Trigonometric equations and identities
- Analyzing categorical data
- Displaying and comparing quantitative data
- Summarizing quantitative data
- Modeling data distributions
- Exploring bivariate numerical data
- Study design
- Probability
- Counting, permutations, and combinations
- Random variables
- Sampling distributions
- Confidence intervals
- Significance tests (hypothesis testing)
- Two-sample inference for the difference between groups
- Inference for categorical data (chi-square tests)
- Advanced regression (inference and transforming)
- Analysis of variance (ANOVA)
- Scatterplots
- Data distributions
- Two-way tables
- Binomial probability
- Normal distributions
- Displaying and describing quantitative data
- Inference comparing two groups or populations
- Chi-square tests for categorical data
- More on regression
- Prepare for the 2020 AP®︎ Statistics Exam
- AP®︎ Statistics Standards mappings
- Polynomials
- Composite functions
- Probability and combinatorics
- Limits and continuity
- Derivatives: definition and basic rules
- Derivatives: chain rule and other advanced topics
- Applications of derivatives
- Analyzing functions
- Parametric equations, polar coordinates, and vector-valued functions
- Applications of integrals
- Differentiation: definition and basic derivative rules
- Differentiation: composite, implicit, and inverse functions
- Contextual applications of differentiation
- Applying derivatives to analyze functions
- Integration and accumulation of change
- Applications of integration
- AP Calculus AB solved free response questions from past exams
- AP®︎ Calculus AB Standards mappings
- Infinite sequences and series
- AP Calculus BC solved exams
- AP®︎ Calculus BC Standards mappings
- Integrals review
- Integration techniques
- Thinking about multivariable functions
- Derivatives of multivariable functions
- Applications of multivariable derivatives
- Integrating multivariable functions
- Green’s, Stokes’, and the divergence theorems
- First order differential equations
- Second order linear equations
- Laplace transform
- Vectors and spaces
- Matrix transformations
- Alternate coordinate systems (bases)
Frequently Asked Questions about Khan Academy and Math Worksheets
Why is khan academy even better than traditional math worksheets.
Khan Academy’s 100,000+ free practice questions give instant feedback, don’t need to be graded, and don’t require a printer.
What do Khan Academy’s interactive math worksheets look like?
Here’s an example:
What are teachers saying about Khan Academy’s interactive math worksheets?
“My students love Khan Academy because they can immediately learn from their mistakes, unlike traditional worksheets.”
Is Khan Academy free?
Khan Academy’s practice questions are 100% free—with no ads or subscriptions.
What do Khan Academy’s interactive math worksheets cover?
Our 100,000+ practice questions cover every math topic from arithmetic to calculus, as well as ELA, Science, Social Studies, and more.
Is Khan Academy a company?
Khan Academy is a nonprofit with a mission to provide a free, world-class education to anyone, anywhere.
Want to get even more out of Khan Academy?
Then be sure to check out our teacher tools . They’ll help you assign the perfect practice for each student from our full math curriculum and track your students’ progress across the year. Plus, they’re also 100% free — with no subscriptions and no ads.
Get Khanmigo
The best way to learn and teach with AI is here. Ace the school year with our AI-powered guide, Khanmigo.
For learners For teachers For parents
"Hello World!" in C Easy C (Basic) Max Score: 5 Success Rate: 85.66%
Playing with characters easy c (basic) max score: 5 success rate: 84.18%, sum and difference of two numbers easy c (basic) max score: 5 success rate: 94.55%, functions in c easy c (basic) max score: 10 success rate: 95.94%, pointers in c easy c (basic) max score: 10 success rate: 96.54%, conditional statements in c easy c (basic) max score: 10 success rate: 96.94%, for loop in c easy c (basic) max score: 10 success rate: 93.63%, sum of digits of a five digit number easy c (basic) max score: 15 success rate: 98.66%, bitwise operators easy c (basic) max score: 15 success rate: 94.84%, printing pattern using loops medium c (basic) max score: 30 success rate: 95.89%.
O Level Biology MCQ (PDF) Questions Answers Bank | IGCSE GCSE Biology MCQs e-Book Download
Quiz questions chapter 1-20 & practice tests with answers key | o level biology textbook notes, mcqs & study guide, publisher description.
The e-Book O Level Biology Multiple Choice Questions (MCQ Quiz) with Answers PDF Download (IGCSE GCSE PDF Book): MCQ Questions Chapter 1-20 & Practice Tests with Answers Key (Class 9-10 Biology Questions Bank, MCQs & Notes) includes revision guide for problem solving with hundreds of solved MCQs. O Level Biology MCQ with Answers PDF book covers basic concepts, analytical and practical assessment tests. "O Level Biology MCQ" PDF book helps to practice test questions from exam prep notes. The eBook O Level Biology MCQs with Answers PDF includes revision guide with verbal, quantitative, and analytical past papers, solved MCQs. O Level Biology Multiple Choice Questions and Answers (MCQs) PDF Download , a book covers solved quiz questions and answers on chapters: Biotechnology, co-ordination and response, animal receptor organs, hormones and endocrine glands, nervous system in mammals, drugs, ecology, effects of human activity on ecosystem, excretion, homeostasis, microorganisms and applications in biotechnology, nutrition in general, nutrition in mammals, nutrition in plants, reproduction in plants, respiration, sexual reproduction in animals, transport in mammals, transport of materials in flowering plants, enzymes and what is biology tests for school and college revision guide. O Level Biology Quiz Questions and Answers PDF download, free eBook’s sample covers beginner's solved questions, textbook's study notes to practice tests. The e-Book IGCSE GCSE Biology MCQs Chapter 1-20 PDF includes high school question papers to review practice tests for exams. O Level Biology Multiple Choice Questions (MCQ) with Answers PDF digital edition eBook, a study guide with textbook chapters' tests for IGCSE/NEET/MCAT/MDCAT/SAT/ACT competitive exam. GCSE Biology Practice Tests Chapter 1-20 PDF covers problem solving exam tests from biology textbook and practical eBook chapter-wise as: Chapter 1: Biotechnology MCQ Chapter 2: Animal Receptor Organs MCQ Chapter 3: Hormones and Endocrine Glands MCQ Chapter 4: Nervous System in Mammals MCQ Chapter 5: Drugs MCQ Chapter 6: Ecology MCQ Chapter 7: Effects of Human Activity on Ecosystem MCQ Chapter 8: Excretion MCQ Chapter 9: Homeostasis MCQ Chapter 10: Microorganisms and Applications in Biotechnology MCQ Chapter 11: Nutrition in General MCQ Chapter 12: Nutrition in Mammals MCQ Chapter 13: Nutrition in Plants MCQ Chapter 14: Reproduction in Plants MCQ Chapter 15: Respiration MCQ Chapter 16: Sexual Reproduction in Animals MCQ Chapter 17: Transport in Mammals MCQ Chapter 18: Transport of Materials in Flowering Plants MCQ Chapter 19: Enzymes MCQ Chapter 20: What is Biology MCQ The e-Book "Biotechnology MCQ" PDF, chapter 1 practice test to solve MCQ questions: Branches of biotechnology and introduction to biotechnology. The e-Book "Animal Receptor Organs MCQ" PDF, chapter 2 practice test to solve MCQ questions: Controlling entry of light, internal structure of eye, and mammalian eye. The e-Book "Hormones and Endocrine Glands MCQ" PDF, chapter 3 practice test to solve MCQ questions: Glycogen, hormones, and endocrine glands thyroxin function. The e-Book "Nervous System in Mammals MCQ" PDF, chapter 4 practice test to solve MCQ questions: Brain of mammal, forebrain, hindbrain, central nervous system, meningitis, nervous tissue, sensitivity, sensory neurons, spinal cord, nerves, spinal nerves, voluntary, and reflex actions. The e-Book "Drugs MCQ" PDF, chapter 5 practice test to solve MCQ questions: Anesthetics and analgesics, cell biology, drugs of abuse, effects of alcohol, heroin effects, medical drugs, antibiotics, pollution, carbon monoxide, poppies, opium and heroin, smoking related diseases, lung cancer, tea, coffee, and types of drugs. The e-Book "Ecology MCQ" PDF, chapter 6 practice test to solve MCQ questions: Biological science, biotic and abiotic environment, biotic and abiotic in ecology, carbon cycle, fossil fuels, decomposition, ecology and environment, energy types in ecological pyramids, food chain and web, glucose formation, habitat specialization due to salinity, mineral salts, nutrients, parasite diseases, parasitism, malarial pathogen, physical environment, ecology, water, and pyramid of energy. The e-Book "Effects of Human Activity on Ecosystem MCQ" PDF, chapter 7 practice test to solve MCQ questions: Atmospheric pollution, carboxyhemoglobin, conservation, fishing grounds, forests and renewable resources, deforestation and pollution, air and water pollution, eutrophication, herbicides, human biology, molecular biology, pesticides, pollution causes, bod and eutrophication, carbon monoxide, causes of pollution, inorganic wastes as cause, pesticides and DDT, sewage, smog, recycling, waste disposal, and soil erosion. The e-Book "Excretion MCQ" PDF, chapter 8 practice test to solve MCQ questions: Body muscles, excretion, egestion, formation of urine, function of ADH, human biology, kidneys as osmoregulators, mammalian urinary system, size and position of kidneys, structure of nephron, and ultrafiltration. The e-Book "Homeostasis MCQ" PDF, chapter 9 practice test to solve MCQ questions: Diabetes, epidermis and homeostasis, examples of homeostasis in man, heat loss prevention, layers of epidermis, mammalian skin, protein sources, structure of mammalian skin and nephron, ultrafiltration, and selective reabsorption. The e-Book "Microorganisms and Applications in Biotechnology MCQ" PDF, chapter 10 practice test to solve MCQ questions: Biotechnology and fermentation products, microorganisms, antibiotics: penicillin production, fungi: mode of life, decomposers in nature, parasite diseases, genetic engineering, viruses, and biochemical parasites. The e-Book "Nutrition in General MCQ" PDF, chapter 11 practice test to solve MCQ questions: Amino acid, anemia and minerals, average daily mineral intake, balanced diet and food values, basal metabolism, biological molecules, biological science, fats, body muscles, carbohydrates, cellulose digestion, characteristics of energy, condensation reaction, daily energy requirements, disaccharides and complex sugars, disadvantages of excess vitamins, disease caused by protein deficiency, energy requirements, energy units, fat rich foods, fats and health, fructose and disaccharides, functions and composition, general nutrition, glucose formation, glycerol, glycogen, health pyramid, heat loss prevention, human heart, hydrolysis, internal skeleton, lactose, liver, mineral nutrition in plants, molecular biology, mucus, nutrients, nutrition vitamins, glycogen, nutrition, protein sources, proteins, red blood cells and hemoglobin, simple carbohydrates, starch, starvation and muscle waste, structure and function, formation and test, thyroxin function, vitamin deficiency, vitamins, minerals, vitamin D, weight reduction program, and nutrition. The e-Book "Nutrition in Mammals MCQ" PDF, chapter 12 practice test to solve MCQ questions: Adaptations in small intestine, amino acid, bile, origination and functions, biological molecules, fats, caecum and chyle, cell biology, digestion process, function of assimilation, pepsin, trypsinogen, function of enzymes, functions and composition, functions of liver, functions of stomach, gastric juice, glycerol, holozoic nutrition, liver, mammalian digestive system, molecular biology, mouth and buccal cavity, esophagus, proteins, red blood cells and hemoglobin, stomach and pancreas, structure and function and nutrition. The e-Book "Nutrition in Plants MCQ" PDF, chapter 13 practice test to solve MCQ questions: Amino acid, carbohydrate, conditions essential for photosynthesis, digestion process, function of enzyme, pepsin, function of enzymes, glycerol, holozoic nutrition, leaf adaptations for photosynthesis, limiting factors, mineral nutrition in plants, mineral salts, molecular biology, photolysis, photons in photosynthesis, photosynthesis in plants, photosynthesis, starch, stomata and functions, storage of excess amino acids, structure and function, structure of lamina, formation and test, vitamins and minerals, water transport in plants, and nutrition. The e-Book "Reproduction in Plants MCQ" PDF, chapter 14 practice test to solve MCQ questions: Transport in flowering plants, artificial methods of vegetative reproduction, asexual reproduction, dormancy and seed germination, epigeal and hypogeal germination, fertilization and post fertilization changes, insect pollination, natural vegetative propagation in flowering plants, ovary and pistil, parts of flower, pollination in flowers, pollination, seed dispersal, dispersal by animals, seed dispersal, sexual and asexual reproduction, structure of a wind pollinated flower, structure of an insect pollinated flower, types of flowers, vegetative reproduction in plants, wind dispersed fruits and seeds, and wind pollination. The e-Book "Respiration MCQ" PDF, chapter 15 practice test to solve MCQ questions: Aerobic respiration and waste, biological science, human biology, human respiration, molecular biology, oxidation and respiration, oxygen debt, tissue respiration, gas exchange, breathing, and respiration. The e-Book "Sexual Reproduction in Animals MCQ" PDF, chapter 16 practice test to solve MCQ questions: Features of sexual reproduction in animals, and male reproductive system. The e-Book "Transport in Mammals MCQ" PDF, chapter 17 practice test to solve MCQ questions: Acclimatization to high attitudes, anemia and minerals, blood and plasma, blood clotting, blood platelets, blood pressure testing, blood pressures, carboxyhemoglobin, circulatory system, double circulation in mammals, function and shape of RBCS, heart, human biology, human heart, main arteries of body, main veins of body, mode of action of heart, organ transplantation and rejection, production of antibodies, red blood cells, hemoglobin, red blood cells in mammals, role of blood in transportation, fibrinogen, and white blood cells. The e-Book "Transport of Materials in Flowering Plants MCQ" PDF, chapter 18 practice test to solve MCQ questions: Transport in flowering plants, cell biology, cell structure and function, epidermis and homeostasis, functions and composition, herbaceous and woody plants, mineral salts, molecular biology, piliferous layer, stomata and functions, structure of root, sugar types, formation and test, water transport in plants, and transpiration. The e-Book "Enzymes MCQ" PDF, chapter 19 practice test to solve MCQ questions: Amino acid, biological science, characteristics of enzymes, classification of enzymes, denaturation of enzymes, digestion process, digestion, catalyzed process, effects of pH, effects of temperature, enzymes, factors affecting enzymes, hydrolysis, rate of reaction, enzyme activity, and specifity of enzymes. The e-Book "What is Biology MCQ" PDF, chapter 20 practice test to solve MCQ questions: Biology basics, cell biology, cell structure, cell structure and function, cells, building blocks of life, tissues, excretion, human respiration, red blood cells and hemoglobin, sensitivity, structure of cell and protoplasm, centrioles, mitochondrion, nucleus, protoplasm, vacuoles, system of classification, vitamins, minerals and nutrition.
More Books by Arshad Iqbal
Other books in this series.
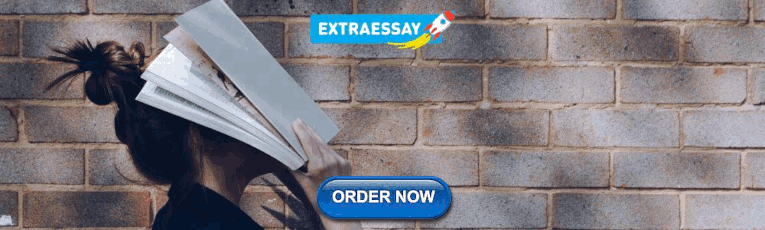
IMAGES
VIDEO
COMMENTS
The best way to learn C programming language is by hands-on practice. This C Exercise page contains the top 30 C exercise questions with solutions that are designed for both beginners and advanced programmers. It covers all major concepts like arrays, pointers, for-loop, and many more. So, Keep it Up!
10. Possible answer: Three more than two-thirds the number of hours Laura worked last week is the same as five-sixths times the hours she worked this week decreased by seven-eighths. x = 23 1 4 hours Practice and Problem Solving: D 1. 4 2. 6 8 99 91 x =
Find step-by-step solutions and answers to Algebra 1 Practice and Problem Solving Workbook - 9780133688771, as well as thousands of textbooks so you can move forward with confidence.
Find step-by-step solutions and answers to enVision Algebra 1 - 9780328931576, as well as thousands of textbooks so you can move forward with confidence. ... Key Features of a Quadratic Function. Section 8-2: Quadratic Functions in Vertex Form. Section 8-3: ... Practice and Problem Solving. Exercise 1a. Exercise 1b. Exercise 2a. Exercise 2b ...
Practice and Problem Solving: D 1. 19.6 cm2 2. 379.9 in.2 3. 28.3 mm2 4. 78.5 in2 5. 132.7 cm2 6. 162.8 yd2 7. 36π cm2 8. 90.25π in2 9. 12.25π yd2 10. 121 π yd2 ... Practice and Problem Solving: A/B Answers may vary for Exercises 1 and 2. 1. 21 ft2 2. 24 ft2 3. 90 ft2 4. 208 m2 5. 140 ft2 6. 23.13 m2 7. 100 ft2
Practice and Problem Solving: C 1. v = 1 2 2. x = 3 3. r = −3 4. m = −4 5. x = −1 6. t = −5 7. 12 − 2n = 8(n + 4); n ... Sample answer: Erin has already saved $60. She plans to save an additional $25 per week. Robin has already saved $20 and plans to save an additional $35 per week. After how many weeks will Robin and Erin have the ...
Every 51 cups of flour weighs 1 9 pounds. Use a unit rate to show. 2 16 how you could determine if there are more than or less than 35 cups of flour in a 10-pound bag of flour. 6. One tank is filling at a rate of 5 gallon per. 7 hour. A second tank is. 10 filling at rate of 5 gallon per 2 hour.
Find step-by-step solutions and answers to Geometry Common Core Practice and Problem Solving Workbook - 9780133185966, as well as thousands of textbooks so you can move forward with confidence. Scheduled maintenance: March 23, 2024 from 11:00 PM to 12:00 AM
a reflection across the y-axis. Draw the image of the given figure after the two transformations. 4. Translate 8 units right and 1 unit up. 5. Reflect across the y-axis. Reflect across the x-axis. Translate 2 units left and 5 units up. 3.
Look at the equations below. Notice how the value on each side of the = sign is the same for each equation: 5 + 7 = 8 + 4 19 − 7 = 12 42 = 3 • 14 If an equation contains a variable, and the variable is replaced by a value that keeps the equation equal, that value is called a solution of the equation. y.
Practice and Problem Solving: C Write an algebraic rule to describe each transformation of figure ... Possible answer: (x, y) → (x + 2, y) 6. (0, −3); rotation of 90° clockwise Practice and Problem Solving: D 1.
Write an equation to solve the problem and answer the question. 7 Write a real-world problem that you could represent with the equation 4 x Possible equation: 15 1 5 5 37. Solve the equation to Þ nd the answer to your question. 8 Without solving, explain how you can tell whether the solution to 0.5 x 5 10 is less than 1 or greater than 1. M M ...
Two-Step Equations Practice Problems with Answers. Hone your skills in solving two-step equations because it will serve as your foundation when solving multi-step equations. I prepared eight (8) two-step equations problems with complete solutions to get you rolling. My advice is for you to solve them by hand using a pencil or pen and paper.
Chapter 14: Chapter Fourteen. Page 370: Think About a Plan. Page 371: Practice. Page 373: Standardized Test Prep. Find step-by-step solutions and answers to Algebra 2 Practice and Problem Solving Workbook - 9780133688894, as well as thousands of textbooks so you can move forward with confidence.
Khan Academy's 100,000+ free practice questions give instant feedback, don't need to be graded, and don't require a printer. Math Worksheets. Khan Academy. Math worksheets take forever to hunt down across the internet. Khan Academy is your one-stop-shop for practice from arithmetic to calculus. Math worksheets can vary in quality from ...
Join over 16 million developers in solving code challenges on HackerRank, one of the best ways to prepare for programming interviews. ... "Hello World!" in C. Easy C (Basic) Max Score: 5 Success Rate: 85.66%. Solve Challenge. Playing With Characters. Easy C (Basic) Max Score: 5 Success Rate: 84.18%. Solve Challenge. Sum and Difference of Two ...
Get hands-on experience with Practice C programming practice problem course on CodeChef. Solve a wide range of Practice C coding challenges and boost your confidence in programming. ... CodeChef Learn Problem Solving: 287: Multiple Choice Question: NA: Learning SQL: 339: Multiple Choice Question: NA: Parking Charges: 416: 2. Conditionals. A ...
3. A: circle; B and C: ellipses or ovals; D: a plane of length, h, the cylinder's height, and width, d, the cylinder's diameter 4. Area A < Area B < Area C < Area D Practice and Problem Solving: D 1. a triangle that is similar to the base 2. a rectangle or a square 3. a trapezoid 4. a circle 5. Drawings will vary, but the cross section
Now, with expert-verified solutions from Ready Mathematics: Practice and Problem Solving Grade 7 , you'll learn how to solve your toughest homework problems. Our resource for Ready Mathematics: Practice and Problem Solving Grade 7 includes answers to chapter exercises, as well as detailed information to walk you through the process step by step.
6. C 1(n) = 2n + 4, C 2(n) = 2.5n + 2 7. The functions represent the rates charged by 2 different dog walkers. The variable represents the number of dogs. 8. Yes Practice and Problem Solving: C 1. = 1 2 y x 2. Sample answer: The number of bales of hay needed to feed 3 elephants. 3. Sample answer: Let x = number of bales of hay; Let y = the ...
Solutions Key 1 Foundations for Geometry CHAPTER ARE YOU READY? PAGE 3 1. C 2. E 3. A 4. D 5. 7 1_ in. 2 ... Possible answer: string c. Possible answer: grid formed by string 23. M 24. R S T 25. U 26. U 27. U 28. ... PRACTICE AND PROBLEM SOLVING 11. DB = ...
Our resource for Integrated Math 2: Practice Workbook includes answers to chapter exercises, as well as detailed information to walk you through the process step by step. With Expert Solutions for thousands of practice problems, you can take the guesswork out of studying and move forward with confidence. Find step-by-step solutions and answers ...
The e-Book O Level Biology Multiple Choice Questions (MCQ Quiz) with Answers PDF Download (IGCSE GCSE PDF Book): MCQ Questions Chapter 1-20 & Practice Tests with Answers Key (Class 9-10 Biology Questions Bank, MCQs & Notes) includes revision guide for problem solving with hundreds of solved MCQs.O Level Biology MCQ with Answers PDF book covers basic concepts, analytical and practical ...
Practice and Problem Solving. Exercise a. Exercise b. At Quizlet, we're giving you the tools you need to take on any subject without having to carry around solutions manuals or printing out PDFs! Now, with expert-verified solutions from enVision Geometry 1st Edition, you'll learn how to solve your toughest homework problems.