- How it works
- Homework answers
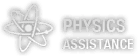
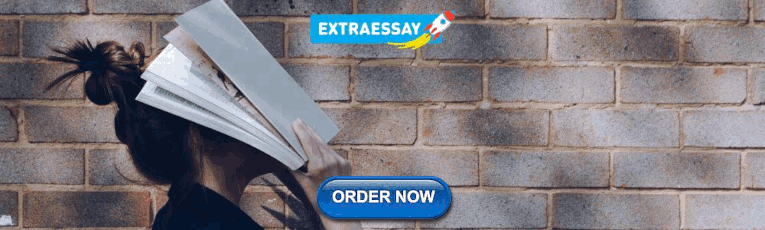
Answer to Question #165334 in Python for hemanth
Given a sentence S, write a program to print the frequency of each word in S. The output order should correspond with the word's input order of appearance.
The input will be a single line containing a sentence S.
The output contains multiple lines, with each line containing a word and its count separated by ": " with the order of appearance in the given sentence.
Python Output:
Need a fast expert's response?
and get a quick answer at the best price
for any assignment or question with DETAILED EXPLANATIONS !
Leave a comment
Ask your question, related questions.
- 1. Prefix SuffixWrite a program to check the overlapping of one string's suffix with the prefix of
- 2. PolynomialGiven polynomial, write a program that prints polynomial in Cix^Pi + Ci-1x^Pi-1 + .... + C
- 3. Alphabetic SymbolWrite a program to print the right alphabetic triangle up to the given N rows.Input
- 4. After selecting the random experiment, write a Python program that performs the following:(The e
- 5. Abhinav and Anjali are playing the Tic-Tac-Toe game. Tic-Tac-Toe is a game played on a grid that
- 6. Write a program to create a menu-driven calculator that performs basic arithmetic operations (+, -,
- 7. Given a sentence S, write a program to print the frequency of each word in S, where words are sorted
- Programming
- Engineering
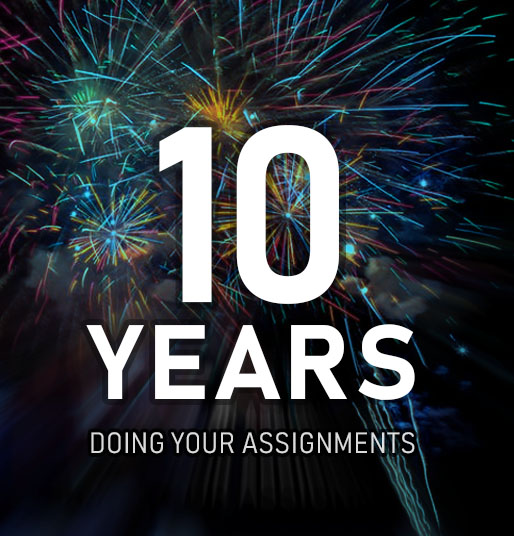
How to Create a Word Count Program in Python
In this guide, we will explore how to create a word count program in Python. You've come to the right place! We'll break down the process into small, easy-to-understand blocks and provide a detailed discussion of each step. By the end of this guide, you'll have a solid understanding of how to analyze text and perform word count operations using Python. Whether you're a seasoned programmer or just starting your Python journey, this guide is designed to be accessible and informative. We'll equip you with the skills to tackle text analysis projects with confidence, making Python your tool of choice for word counting and beyond.
- A Step-by-Step: Building a Word Count Program in Python
Demystifying Word Count in Python
Explore our comprehensive guide on how to create word count programs in Python. Whether you're a seasoned coder or just starting, this guide is designed to provide valuable insights and practical skills for word counting. From understanding the basics to mastering advanced techniques, you'll be well-prepared to excel in Python text analysis. We're here to help with your Python assignment , ensuring you have the knowledge and confidence to tackle text analysis projects effectively. Whether it's for academic assignments or real-world applications, this guide equips you with the tools you need for success in Python programming.
Block 1 - `text_to_words` Function
This function takes a text string (`the_text`) as input and processes it to return a list of words. It performs the following steps:
- It defines a translation table (`my_substitutions`) using `str.maketrans` to replace uppercase letters, numbers, and various punctuation characters with lowercase letters or spaces.
- It applies this translation to the input text to remove punctuation and convert the text to lowercase.
- It splits the cleaned text into a list of words and returns it.
Block 2 - `load_string_from_file` Function
This function takes a filename as input and reads the content of the specified file. It returns the content as a single string. It uses the `with` statement to ensure that the file is properly closed after reading its content.
Block 3 - `getWordCount` Function
This function takes the content of a text file (`filetext`) and calculates the word count. It uses the `text_to_words` function to obtain a list of words from the file text and then returns the length of this list, which represents the total word count. Duplicate words are counted as well.
Block 4 - `getDict` Function
This function takes the content of a text file (`filetext`) and generates a dictionary where each word is a key, and the corresponding value is the frequency of that word in the text. It first uses the `text_to_words` function to obtain a list of words, and then it iterates through this list to populate the dictionary.
Block 5 - `getvocabulary` Function
This function takes the content of a text file (`filetext`) and returns a list containing the unique words (vocabulary) found in the text. It uses the `text_to_words` function to obtain a list of words and then converts this list into a set to remove duplicates. Finally, it converts the set back to a list before returning it.
Block 6 - Main Execution
This part of the code represents the main execution. It reads the content of a file named 'brooks.txt' using the `load_string_from_file` function and stores the content in the `file_content` variable. It then performs the following tasks:
- Prints the content of the file.
- Counts and prints the total number of words in the text.
- Prints a word dictionary where words are keys and their frequencies are values.
- Prints the vocabulary, which is a list of unique words in the text.
In conclusion, this guide has equipped you with the essential knowledge and practical skills to create word count programs in Python. By breaking down the process into manageable blocks, we've demystified text analysis and word counting. Whether you're a beginner or an experienced programmer, you now have the tools to confidently navigate Python for text analysis. The ability to count words and analyze text is a fundamental skill in various applications, from data processing to content optimization. With this newfound expertise, you're well-prepared to embark on more advanced text analysis projects and harness the power of Python in your programming journey.
Get Free Quote
A step-by-step: building a word count program in python submit your homework, attached files.
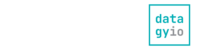
- Learn Python
- Python Lists
- Python Dictionaries
- Python Strings
- Python Functions
- Learn Pandas & NumPy
- Pandas Tutorials
- Numpy Tutorials
- Learn Data Visualization
- Python Seaborn
- Python Matplotlib
Python: Count Words in a String or File
- April 4, 2022 December 16, 2022
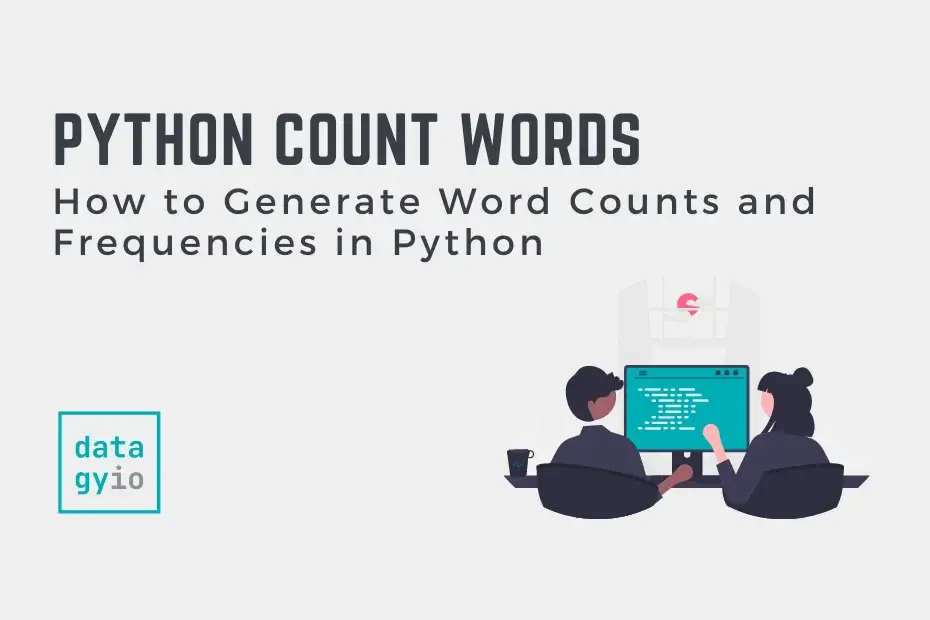
In this tutorial, you’ll learn how to use Python to count the number of words and word frequencies in both a string and a text file . Being able to count words and word frequencies is a useful skill. For example, knowing how to do this can be important in text classification machine learning algorithms.
By the end of this tutorial, you’ll have learned:
- How to count the number of words in a string
- How to count the number of words in a text file
- How to calculate word frequencies using Python
Table of Contents
Reading a Text File in Python
The processes to count words and calculate word frequencies shown below are the same for whether you’re considering a string or an entire text file. Because of this, this section will briefly describe how to read a text file in Python.
If you want a more in-depth guide on how to read a text file in Python, check out this tutorial here . Here is a quick piece of code that you can use to load the contents of a text file into a Python string:
I encourage you to check out the tutorial to learn why and how this approach works. However, if you’re in a hurry, just know that the process opens the file, reads its contents, and then closes the file again.
Count Number of Words In Python Using split()
One of the simplest ways to count the number of words in a Python string is by using the split() function . The split function looks like this:
By default, Python will consider runs of consecutive whitespace to be a single separator. This means that if our string had multiple spaces, they’d only be considered a single delimiter. Let’s see what this method returns:
We can see that the method now returns a list of items. Because we can use the len() function to count the number of items in a list, we’re able to generate a word count. Let’s see what this looks like:
Count Number of Words In Python Using Regex
Another simple way to count the number of words in a Python string is to use the regular expressions library, re . The library comes with a function, findall() , which lets you search for different patterns of strings.
Because we can use regular expression to search for patterns, we must first define our pattern. In this case, we want patterns of alphanumeric characters that are separated by whitespace.
For this, we can use the pattern \w+ , where \w represents any alphanumeric character and the + denotes one or more occurrences. Once the pattern encounters whitespace, such as a space, it will stop the pattern there.
Let’s see how we can use this method to generate a word count using the regular expressions library, re :
Calculating Word Frequencies in Python
In order to calculate word frequencies, we can use either the defaultdict class or the Counter class. Word frequencies represent how often a given word appears in a piece of text.
Using defaultdict To Calculate Word Frequencies in Python
Let’s see how we can use defaultdict to calculate word frequencies in Python. The defaultdict extend on the regular Python dictionary by providing helpful functions to initialize missing keys.
Because of this, we can loop over a piece of text and count the occurrences of each word. Let’s see how we can use it to create word frequencies for a given string:
Let’s break down what we did here:
- We imported both the defaultdict function and the re library
- We loaded some text and instantiated a defaultdict using the int factory function
- We then looped over each word in the word list and added one for each time it occurred
Using Counter to Create Word Frequencies in Python
Another way to do this is to use the Counter class. The benefit of this approach is that we can even easily identify the most frequent word. Let’s see how we can use this approach:
- We imported our required libraries and classes
- We passed the resulting list from the findall() function into the Counter class
- We printed the result of this class
One of the perks of this is that we can easily find the most common word by using the .most_common() function. The function returns a sorted list of tuples, ordering the items from most common to least common. Because of this, we can simply access the 0th index to find the most common word:
In this tutorial, you learned how to generate word counts and word frequencies using Python. You learned a number of different ways to count words including using the .split() method and the re library. Then, you learned different ways to generate word frequencies using defaultdict and Counter . Using the Counter method, you were able to find the most frequent word in a string.
Additional Resources
To learn more about related topics, check out the tutorials below:
- Python str.split() – Official Documentation
- Python Defaultdict: Overview and Examples
- Python: Count Number of Occurrences in List (6 Ways)
- Python: Count Number of Occurrences in a String (4 Ways!)
Nik Piepenbreier
Nik is the author of datagy.io and has over a decade of experience working with data analytics, data science, and Python. He specializes in teaching developers how to use Python for data science using hands-on tutorials. View Author posts
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
- Free Python 3 Tutorial
- Control Flow
- Exception Handling
- Python Programs
- Python Projects
- Python Interview Questions
- Python Database
- Data Science With Python
- Machine Learning with Python
- Solve Coding Problems
- Python Program that Displays Letters that are not common in two strings
- Python - Integers String to Integer List
- Python - Character Replacement Combination
- Python - String uncommon characters
- Python | Ways to split strings using newline delimiter
- Python - Characters Index occurrences in String
- Python - Split a String by Custom Lengths
- Python - Test substring order
- Python - Right and Left Shift characters in String
- Python program to extract numeric suffix from string
- Python | Check whether string contains only numbers or not
- Python | Get numeric prefix of given string
- Python - Check if Elements delimited by K
- Python - Maximum occurring Substring from list
- Python - Convert Delimiter separated list to Number
- Python - Case insensitive string replacement
- Python | Check if string ends with any string in given list
- Python - Specific Characters Frequency in String List
- Python - Construct Grades List
Python program to count words in a sentence
Data preprocessing is an important task in text classification. With the emergence of Python in the field of data science, it is essential to have certain shorthands to have the upper hand among others. This article discusses ways to count words in a sentence, it starts with space-separated words but also includes ways to in presence of special characters as well. Let’s discuss certain ways to perform this.
Quick Ninja Methods: One line Code to find count words in a sentence with Static and Dynamic Inputs.
Method #1: Using split() split function is quite useful and usually quite generic method to get words out of the list, but this approach fails once we introduce special characters in the list.
Method #2 : Using regex(findall()) Regular expressions have to be used in case we require to handle the cases of punctuation marks or special characters in the string. This is the most elegant way in which this task can be performed.
Method #3 : Using sum() + strip() + split() This method performs this particular task without using regex. In this method we first check all the words consisting of all the alphabets, if so they are added to sum and then returned.
Method #4: Using count() method
Method #5 : Using the shlex module:
Here is a new approach using the split() method in shlex module:
The shlex module provides a lexical analyzer for simple shell-like syntaxes. It can be used to split a string into a list of words while taking into account quotes, escapes, and other special characters. This makes it a good choice for counting words in a sentence that may contain such characters.
Note: The shlex.split function returns a list of words, so you can use the len function to count the number of words in the list. The count method can also be used on the list to achieve the same result.
Method #6: Using operator.countOf() method
The time complexity of this approach is O(n), where n is the length of the input string. The Auxiliary space is also O(n), as the shlex.split function creates a new list of words from the input string. This approach is efficient for small to medium-sized inputs, but may not be suitable for very large inputs due to the use of additional memory.
Method #7:Using reduce()
- Initialize a variable res to 1 to account for the first word in the string.
- For each character ch in the string, do the following: a. If ch is a space, increment res by 1.
- Return the value of res as the result.
The time complexity of the algorithm for counting the number of words in a string using the count method or reduce function is O(n), where n is the length of the string. This is because we iterate over each character in the string once to count the number of spaces.
The auxiliary space of the algorithm is O(1), since we only need to store a few variables (res and ch) at any given time during the execution of the algorithm. The space required is independent of the length of the input string.
Method #8: Using numpy:
- Initialize the input string ‘test_string’
- Print the original string
- Use the numpy ‘char.count()’ method to count the number of spaces in the string and add 1 to it to get the count of words.
- Print the count of words.
Time complexity: O(n) The time complexity of the ‘char.count()’ method is O(n), where n is the length of the input string. The addition operation takes constant time. Therefore, the time complexity of the code is O(n).
Auxiliary Space: O(1) The space complexity of the code is constant, as we are not using any additional data structures or variables that are dependent on the input size. Therefore, the space complexity of the code is O(1).
Please Login to comment...
- Python string-programs
- WhatsApp To Launch New App Lock Feature
- Top Design Resources for Icons
- Node.js 21 is here: What’s new
- Zoom: World’s Most Innovative Companies of 2024
- 30 OOPs Interview Questions and Answers (2024)
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Create a Word Counter in Python
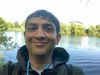
Shantnu Tiwari
Intro: Start here
Beginners Start Here:
An introduction to Numpy and Matplotlib
Introduction to Pandas with Practical Examples (New)
Image and Video Processing in Python
Data Analysis with Pandas
Audio and Digital Signal Processing (DSP)
Machine Learning Section
Machine Learning with an Amazon like Recommendation Engine
This chapter is for those new to Python, but I recommend everyone go through it, just so that we are all on equal footing.
Baby steps: Read and print a file
Okay folks, we are going to start gentle. We will build a simple utility called word counter. Those of you who have used Linux will know this as the wc utility. On Linux, you can type:
to get the number of words, lines and characters in a file. The wc utility is quite advanced, of course, since it has been around for a long time. We are going to build a baby version of that. This is more interesting than just printing Hello World to the screen.
With that in mind, let’s start. The file we are working with is read_file.py, which is in the folder Wordcount.
The first line that starts with a #! is used mainly on Linux systems. It tells the shell that this is a Python file, and should be run as such. It also tells Linux which interpreter to use (Python in our case). It doesn’t do any harm on Windows (as anything that starts with a # is a comment in Python), so we keep it in.
Let’s start looking at the code.
We simply open a file called “birds.txt”. It must exist in the current directory (ie, the directory you are running the code from). Later on, we will cover reading from the command line, but for now, the path is hard coded. The r means the file will be opened in a read only mode. Other common modes are w for write, a for append. You can also read/write binary files, however we won’t go into that for the moment. Our files are plain text.
After opening the file, we read its contents into a variable called data, and close the file.
And we print the file. And now, to test our code. If you are on Linux, you can just type:
to run it. You might have to make the file executable. On Windows, you’ll need to do:
Go to the folder called WordCount, and run the file there:
And there you go. Your First Python program.
Count words and lines
Okay, so we can read a file and print it on the screen. Now, to count the number of words. We’ll be using the file count_words.py in the WordCount folder.
These lines should be familiar by now. We open the file and read it.
Python has several in built functions for strings. One is the split() function which splits the string on the given parameter. In the example above, we are splitting on a space. The function returns a list (which is what Python calls arrays) of the string split on space.
To see how this works, I’ll fire up an IPython console.
I took a sentence “I am a boy” and split it on a space. Python returned a list with four elements: [‘I’, ‘am’, ‘a’, ‘boy’] . We can split on anything. Here, I split on a comma:
Coming back to our example:
You should know what we are doing now. We are splitting the file we read on spaces. This should give us the number of words, as in English, words are separated by space (as if you didn’t know already).
So we print the words that we found. Next, we call the len() function, which returns the length of a list. Remember I said the split() function breaks the string into a list? Well, by using the len() function, we can find out how many elements the list has, and hence the number of words.
Next, we find the number of lines by using the same method.
We do the same thing, except this we split on the newline character (“\n”). For those who don’t know, the newline character is the code that tells the editor to insert a new line, a return. By counting the number of newline characters, we can get the number of lines in the program.
Run the file count_words.py , and see the results.
Now open the file birds.txt and count the number of lines by hand. You’ll find the answers are different. That’s because there is a bug in our code. It is counting empty lines as well. We need to fix that now.
Count lines fixed
This is the old code, the one we need to correct.
For loop in Python
The syntax of the for loop is:
A few key things. There is a colon (:) after the for instruction. And in Python, there are no brackets {} or start-end keywords. For example, if you come from a C/C++/Java/C# type world, this is how you would write your for loop:
The curly braces {} tell the compiler that this code is under the for loop. Python doesn’t have these braces. Instead, it uses white space/indentation. If you don’t use indentation, Python will complain. Example:
The correct way to do it is:
How much indentation to use? Four spaces is recommended. If you are using a good text editor like Sublime Text, it will do that automatically. Coming back to our code,
Let’s go over this line by line.
We are looping over our list lines . l will contain each line as Python is looping over them.
As a side note, those of you who come from a C/C++ background, you will be surprised by us not using arrays. We don’t need to— Python will do that for us automatically. Python will take the list lines and automatically loop over it. We don’t need to do lines[0] , lines[1] , lines[2] etc like you would do in C/C++. In fact, doing so is an anti-pattern.
So now we have each line. We need to now check if it is empty. There are many ways to do it. One is:
This checks if the current line has a length of 0, which is fine, but there is a more elegant way of doing it.
The not keyword in Python will automatically check for emptiness for us. If the line is empty, we remove it from the list using the remove() command.
Again, like the for loop, we need to give four spaces to let Python know that this instruction is under the if condition.
We should now have the correct number of lines. Run count_lines_fixed.py to see the results.
Bringing it all together
Now we need to tie it all together. word_count.py is our final file.
The only new thing here is the import sys command. This is needed to read from the command line.
We will beak our code into functions now. The way to write a function in Python is:
def defines a function. Notice the colon (:) and the white space? Like loops and if conditions, you need to use indentation for code under the for loop.
Our first function counts the number of words:
It takes in the list data, and returns the number of words. Keep in mind this is the exact same code as before, the only difference is now it is in a function.
The function to count lines is similar:
The next part is one of the most Googled lines:
There are two ways to call Python files:
You can call the file directly, python filename , which is what we’ve been doing.
We haven’t covered calling the file as a library yet. If you wanted to use the function count_words in another file, you would do this:
This will take the function count_words and make it available in the new file. You can also do:
This will import all functions and variables, but generally, this approach isn’t recommended. You should only import what you need.
Now, sometimes you’ll have code you only want to run if the file is being called directly, ie, without an import. If so, you can put it under this line:
This means (in simple English): Only run this code if I am running this file from the command line (or something similar). If we import this file in another, all of this code will be ignored.
By using this syntax, you can ensure your function only runs when someone calls your program directly, and not imports is as a module.
__name__ is an an internal variable set to __main__ by the Python interpreter when we are running the program standalone.
Now in our examples, we have been calling the files directly, but I thought I’d show you this syntax in case you ever saw it on the web. It is optional in our case, but good practice in case you want to turn your code into a library. Even if you don’t want to at the moment, it’s a good idea to use the command as it’s only one line.
Remember we imported the sys library? It contains several system calls, one of which is the sys.argv command, which returns the command line arguments. You already know our old friend len() . We check if the number of command line arguments is less than two (the first is always the name of the file), and if so, print a message and exit. This is what happens:
The next line:
As I said, the first element of sys.argv (or argv[0]) will be the name of the file itself (word_count.py in our case). The second will be the file the user entered. We read that.
We read the data from the file.
And now we call our functions to count the number of words and lines, and print the results. Voila! A simple word counter.
The word counter isn’t perfect, and if you try it with different files, you will find many bugs. But it’s good enough for us to move on to the next chapter.
Sign up to know when the next post is out, and get exclusive content
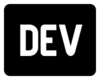
DEV Community

Posted on Oct 13, 2022 • Updated on Sep 6, 2023
Introduction to Python Programming - A Word-counter Program
Introduction.
In the last article, we looked at controlling program execution with if-elif-else statements, match statements, for and while loops, pass statements, and try-except statements. In this, we will create some programs.
A Word Counter Program
Building a word counter will allow us to put some of the concepts we have learned into practice.
Assuming you have a string variable declared literally or a text read from a file, you may decide to know the unique words as well as count them. Let us create a variable called text .
If you have a file in the same directory/folder as your python file, you can replace the above variable with
Since Python is case-sensitive, words like "The" and "the" are not the same. So, convert the text to lowercase.
To get the individual words in the text , use the split() method. Optionally, you can sort the text_split list using the list's sort() method.
View the unique words in the list using the set() function.
Notice that some words like file and skills end with a period (.). Let us remove them using the map() function.
The map() takes a function and an iterable/sequence and applies the function to every element of the sequence. The lambda you see above is used to create a one-time-use function. We will see more of map and lambda in my next article on functions :) The expression within the lambda function x[: -1] if x.endswith(".") else x is an example of an if expression. You will find it in the previous article. If the word ends with a period take a slice excluding the last [: -1] . If not, give me back the word.
Next, create an empty dictionary to hold the words and their count. Let us call the variable summary .
Using a for loop, we will go through the text_split list and if we see a word already in the dictionary, we will increase its count by 1 else, we will add the variable to the dictionary and set its count to 1. For example
The word in summary checks if the word is already a key in the dictionary.
Here is a shorter way to re-write the code above using the dictionary's get() method.
The summary and summary have the same content.
summary2.get(word, 0) + 1 get the value/count associated with word . If there is nothing there that is, the word is not a key in the dictionary, give me zero (0) as the count. Add 1 to either set the summary[word] to 1 or increment the value contained in summary[word] .
Finally, print the content of the summary . The order of the content may differ from yours but they will be the same.
To obtain a much prettier print, use the pp() method in the pprint module. We have not discussed modules so do not worry about it. Just type it for now.
Now, here is the full program.
In this article, we created a word-counter program. If we had read a file, we could include the number of lines and the size of the file in bytes just like the wc command in a linux shell. In the next article, we will deal with functions. Thanks for reading.
Top comments (0)
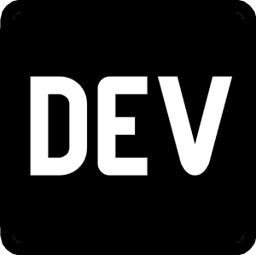
Templates let you quickly answer FAQs or store snippets for re-use.
Are you sure you want to hide this comment? It will become hidden in your post, but will still be visible via the comment's permalink .
Hide child comments as well
For further actions, you may consider blocking this person and/or reporting abuse
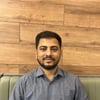
An Introduction to HubSpot Operations Hub
Rahul - Feb 21
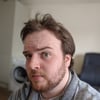
Learning Rust: Structuring Data with Structs
Andrew Bone - Mar 18
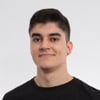
Announcing TechSchool: A free and open-source platform to learn programming
Daniel Bergholz - Mar 5
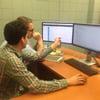
How to upgrade a Ruby on Rails app
Fernando - Mar 14
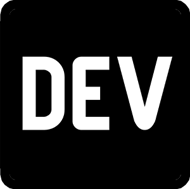
We're a place where coders share, stay up-to-date and grow their careers.

Python Word Count (Filter out Punctuation, Dictionary Manipulation, and Sorting Lists)

Michael Galarnyk
For the text below, count how many times each word occurs.
Clean Punctuation and Transform All Words to Lowercase.
Output a list of word count pairs (sorted from highest to lowest).
Approach 1: Collections Module
This is the easiest way to do this, but it requires knowing which library to use.
Approach 2: Using For Loops
This approach uses the dictionary method get. If you want to learn more about using the method, see the tutorial Python Dictionary and Dictionary Methods .
Next, reverse the key and values so they can be sorted using tuples.
Next, sort from highest to lowest.
Approach 3: Not using Dictionary Get Method
Approach 4: Using sorted
Concluding Remarks
Using any of the four methods should give you an output like
I should note that the code used in this blog post and in the video above is available on my github . Please let me know if you have any questions either here, on youtube , or through Twitter ! If you want to learn how to utilize the Pandas, Matplotlib, or Seaborn libraries, please consider taking my Python for Data Visualization LinkedIn Learning course .

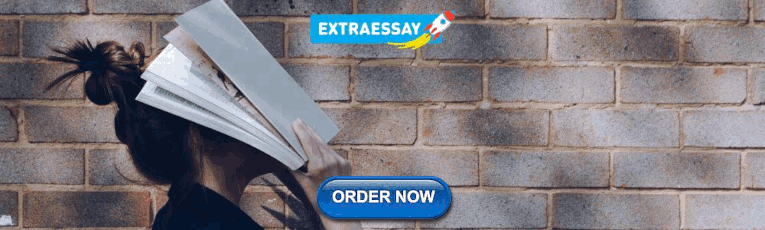
Written by Michael Galarnyk
Data Scientist https://www.linkedin.com/in/michaelgalarnyk/
More from Michael Galarnyk and codeburst
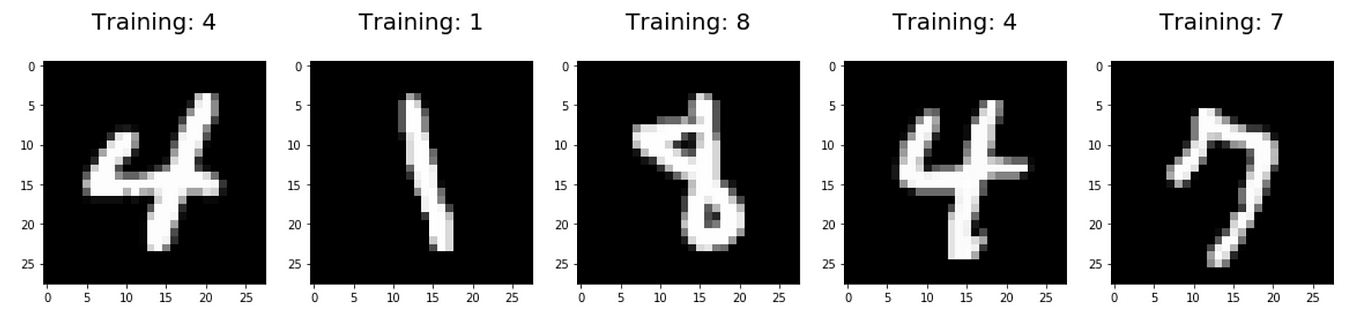
Towards Data Science
Logistic Regression using Python (scikit-learn)
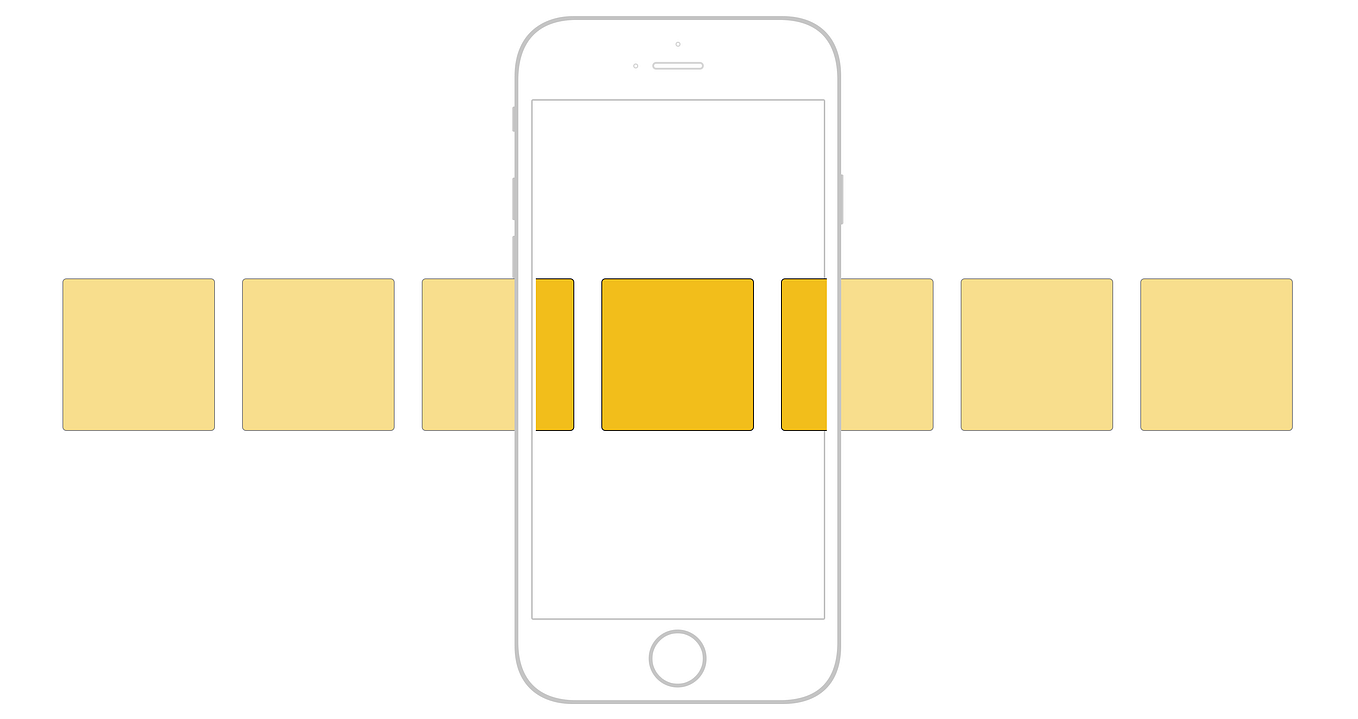
How To Create Horizontal Scrolling Containers
As a front end developer, more and more frequently i am given designs that include a horizontal scrolling component. this has become….
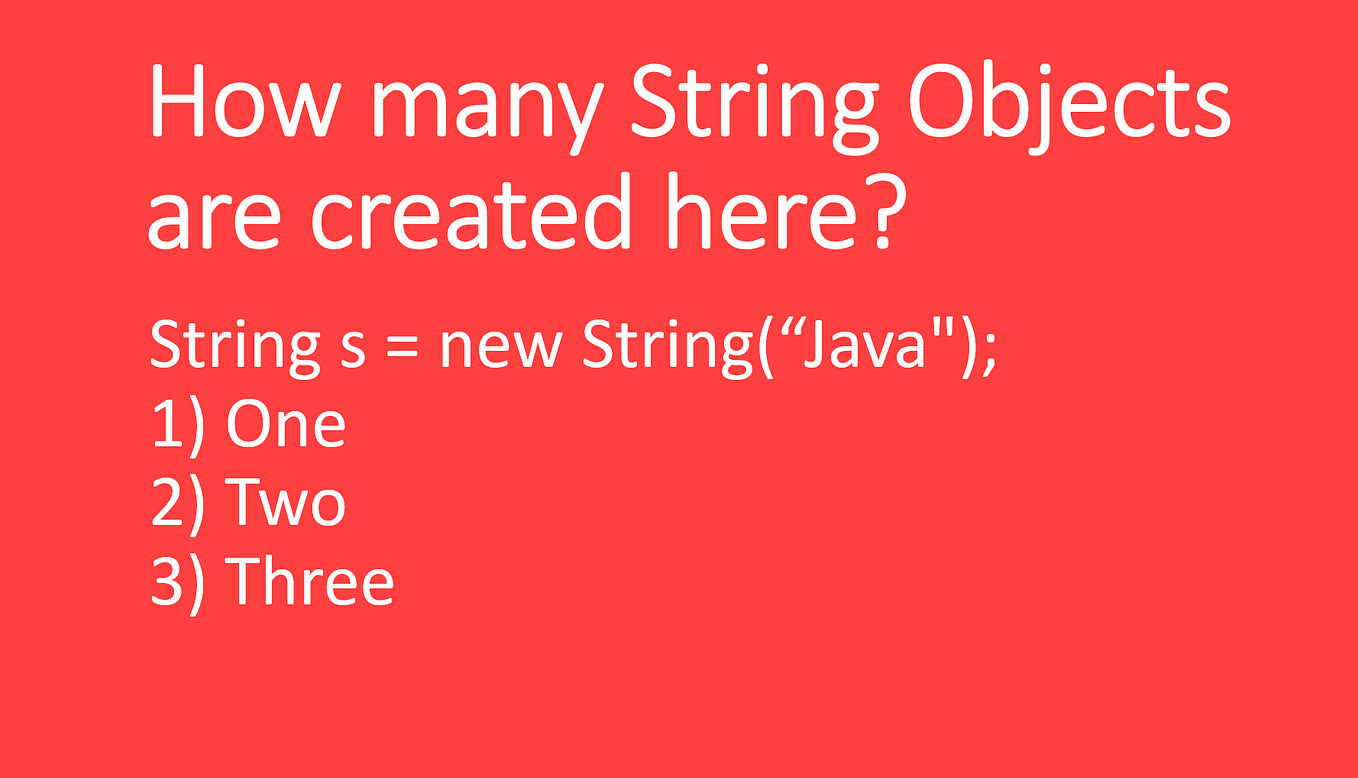
Top 50 Java Interview Questions for Beginners and Junior Developers
A list of frequently asked java questions and answers from programming job interviews of java developers of different experience..
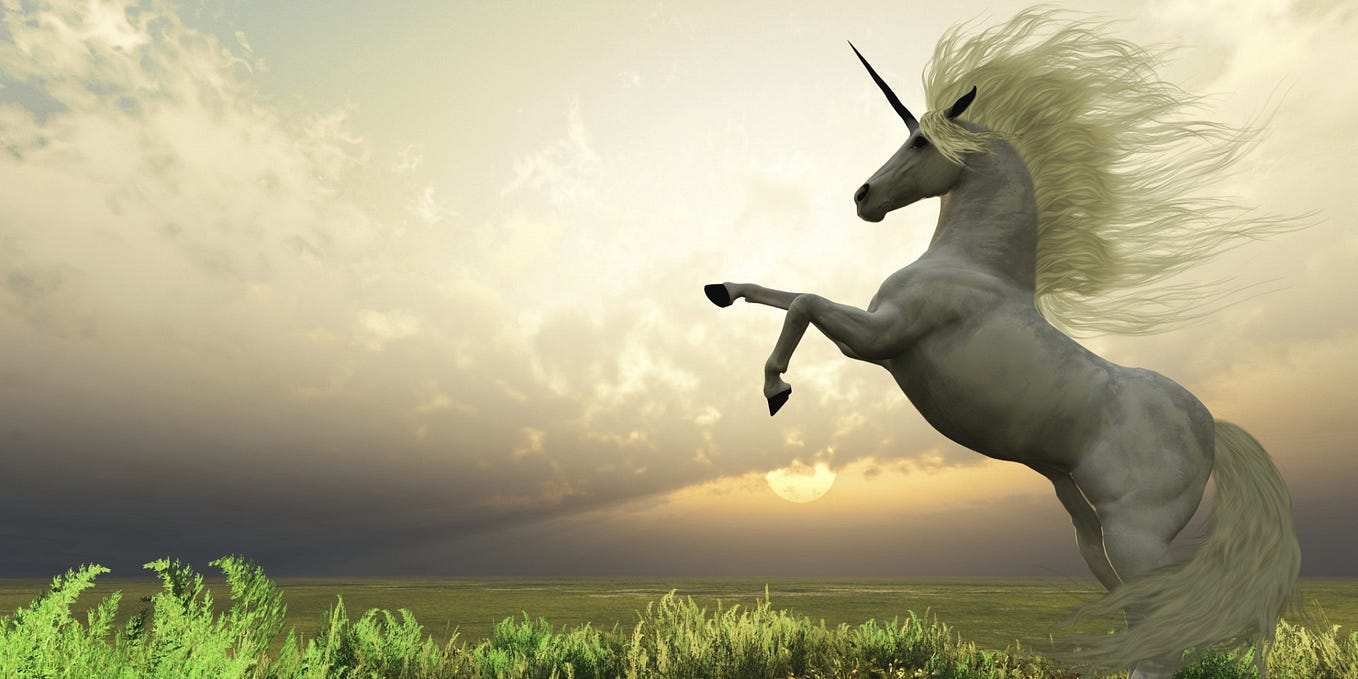
How to Build a Data Science Portfolio
Recommended from medium.
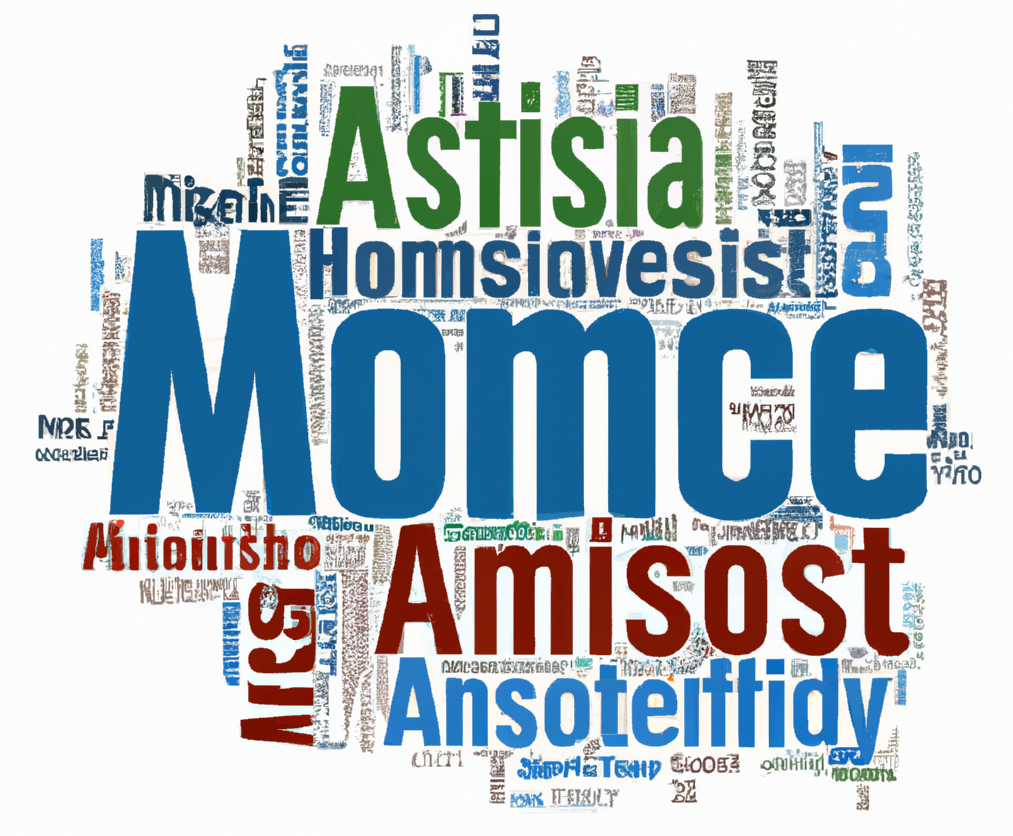
Omer Duskin
Python in Plain English
How to use TF-IDF to retrieve Most Significant Words of a file — A Practical Python Guide
What is tf-idf.
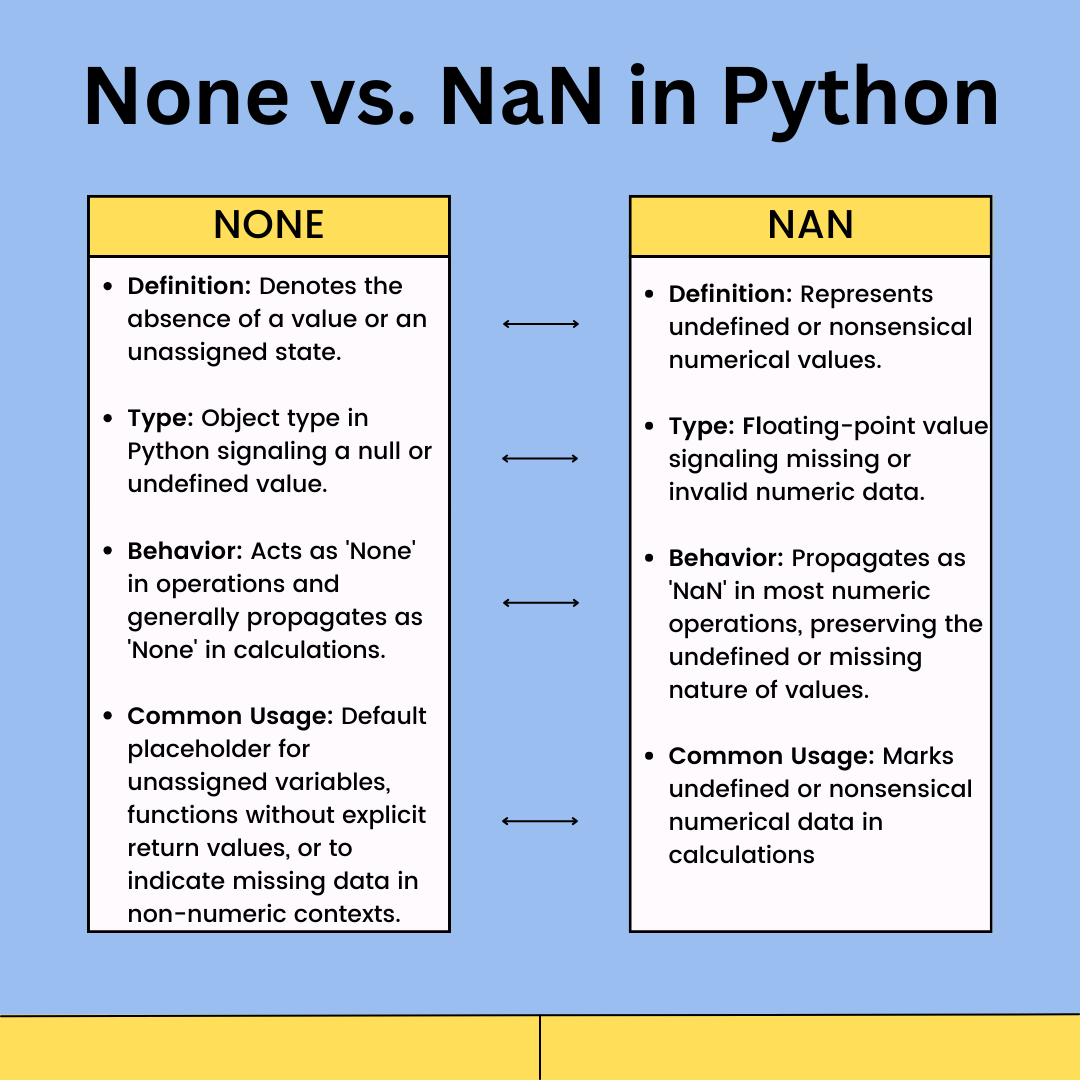
Annette Dolph
Data And Beyond
None, NaN, Null, and Zero in Python
A beginning analyst’s guide to essential data distinctions.
Coding & Development

Predictive Modeling w/ Python

Practical Guides to Machine Learning

Aysel Aydin
1 — Text Preprocessing Techniques for NLP
In this article, we will cover the following topics:.
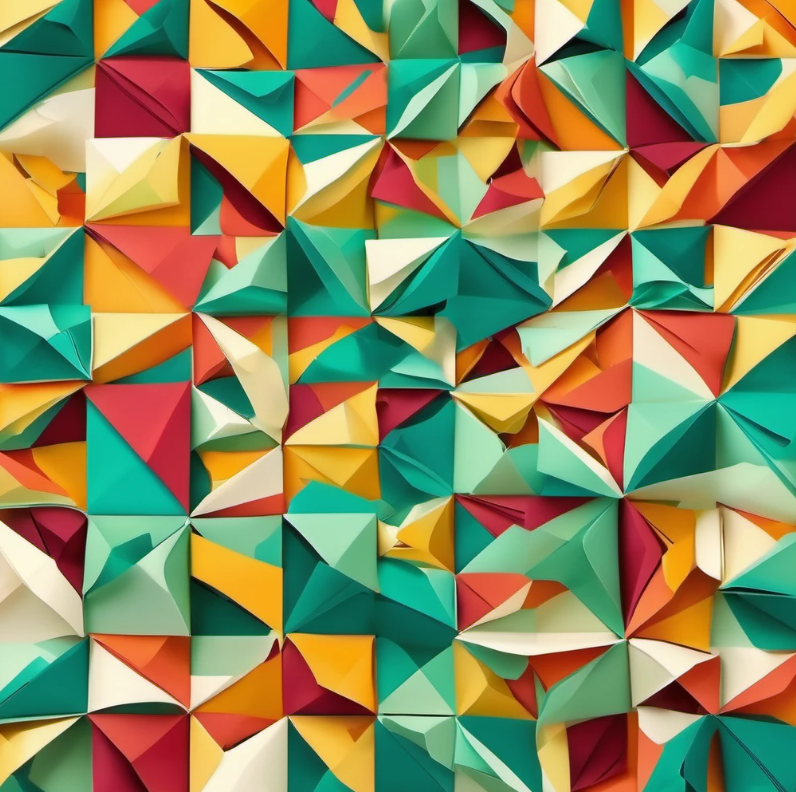
Mohamad Mahmood
Pointwise Mutual Information calculation
Using (1) list of tuples and (2) numpy array.
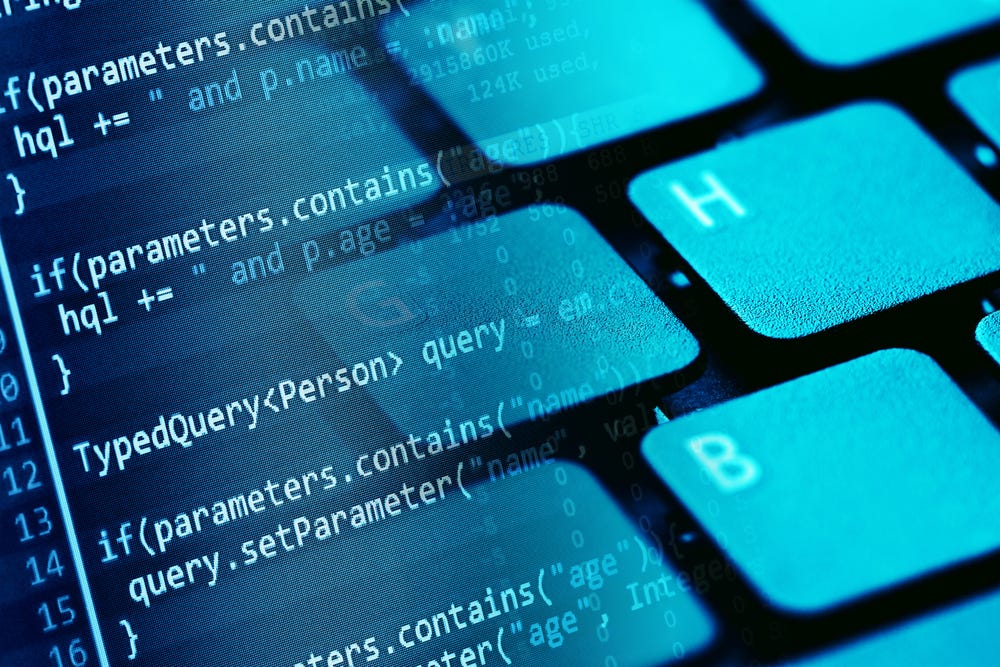
BeyondVerse
Algorithms for String Manipulation and Matching
In the realm of computer science, string manipulation and matching algorithms play a pivotal role in processing and analyzing textual …...
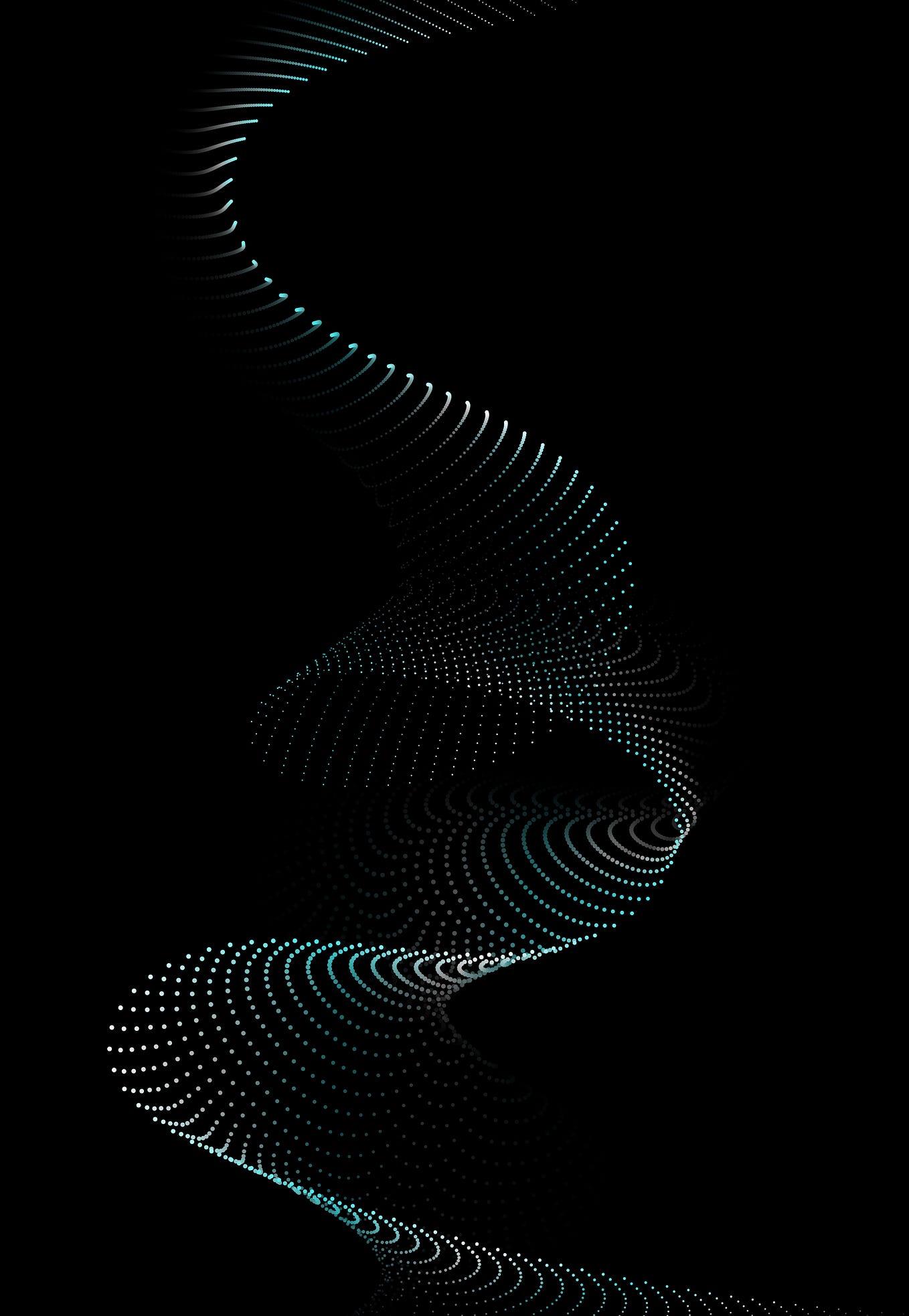
Stackademic
Python Mastery: 90 Tips for Efficiently Looping Through Data in Python
Efficient looping is important in programming for several reasons, and it becomes particularly crucial when working with large datasets….
Text to speech
- Table of Contents
- Scratch ActiveCode
- Chapter 2. Values and Variables (Single Pieces of Data)
- 1. Introduction
- 2. Values and Variables (Single Pieces of Data)
- 3. Control Structures
- 4. Functions
- 5. Strings, Lists, and Files (Multiple Pieces of Data)
- 6. Pandas: Series
- 7. Pandas: Dataframes
- 8. Statistics
- 9. Visualization with Seaborn
- 10. Data Cleaning
- 11. Classes (Defining New Kinds of Objects)
- Contributions
2.8. Word Count Example Program (Again) ¶
We can use much of what we’ve covered in this chapter to help make some sense of the word count example program from the introduction. Here it is again:
Here is what we can understand so far, just based on the contents of this chapter:
Right away, we can see that this line is an example of the assignment statement syntax pattern . We can interpret this as “The countchar variable gets the value 'w' ,” and we know that 'w' is a string value.
Again, we have an assignment statement. It’s creating a variable called file and assigning it… What? Well, we don’t know exactly what that does yet, but from the context we can guess that it has something to do with the file named atotc_opening.txt . We’ll cover the open() function later.
More assignment statements, this time creating a text variable and a words variable. The file variable from the line above is used here, but again, the details of what, precisely, these lines do will come later in the book. The variable names help us understand the code, though, because they were chosen well . It’s possible to guess that this will “read” the file that was “opened” earlier, that the text variable will contain the text of that file, and that words will contain words from that text.
Another simple assignment statement. Here, count gets the value 0 . Many programs start with a series of variable assignments like these, creating variables and giving them initial values for the rest of the program to use.
The for and if keywords used in these lines will be covered in the very next chapter. For now, form a guess about what they mean based on what you know of the variables involved, the common English meaning of the words used, and what you can see the program output. You can compare your guess to the formal definitions when you reach them in a few sections.
Here we have an assignment statement with an expression on the right hand side. This is an example of a variable update, and specifically it is an increment . This line sets the count variable to get its current value plus one.
In these remaining lines, we see a print statement, but with an oddly-formed string, and something to do with the file variable above. The meaning of these can be guessed from the names used and the context, but we have no precise interpretation for them yet.
Create a Wordcounter in Python: How to Count Words and Lines in a File using Python?
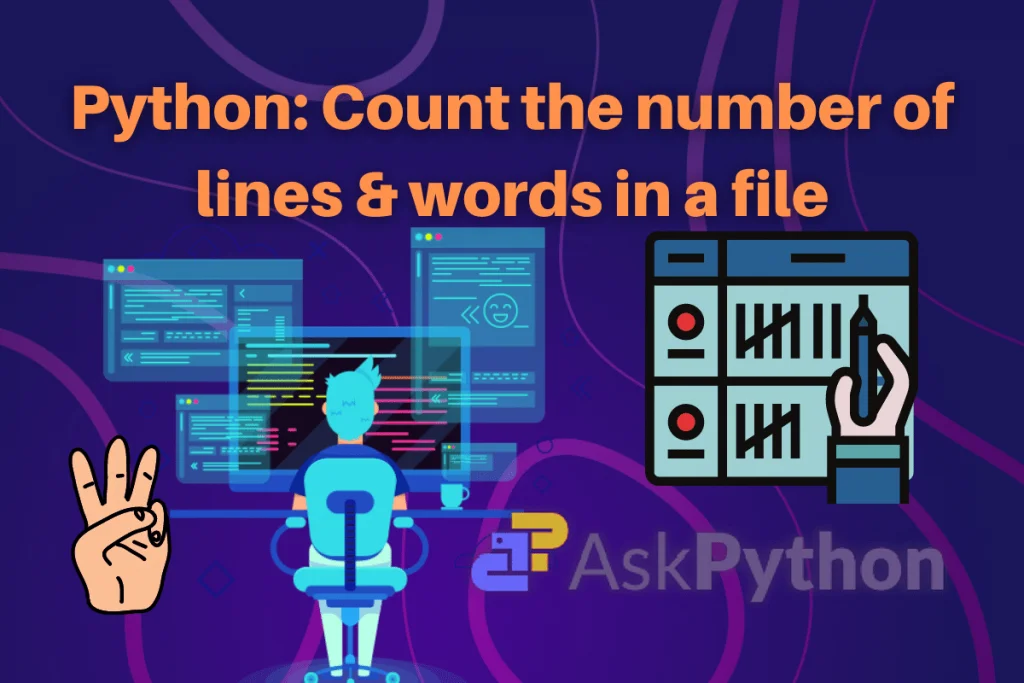
Hello readers! In this tutorial, we are going to discuss how to count the number of lines and words in a file using Python programming.
Also read: How to read a file in Python?
How to Count the Words and Lines – Python Wordcounter
Suppose you have a large file and need to figure out the number of words within the file. Along with that, you also want to find out how many lines of text are present within it. You can create a wordcounter program that does counts the words and the lines using Python.
1. Create a sample text file
In this process of creating a text file, we will first create a variable and assign a string to it. Then we will create a file in write only mode (‘w’) using the open() function and write the content of the string variable to the newly created text file. Finally, close the text file. Let’s write a Python program to create a text file.
2. Display the content of the sample text file
As we have successfully created a text file, we will now read the content of the sample text file into a variable using the read() function in read-only mode (‘ r ‘). Then we will print the content of the Python variable to view the text from our file. Finally, as a good practice, we will close the opened text to avoid any memory leaks in our code. Let’s see the Python code to read a given text file.
3. Algorithm to count the number of lines and words in a file
To count the number of lines and words in a file, we have to follow the steps given below:
- Create two variables say line_count & word_count and initialize them with zero.
- Create another variable say file_path and initialize it with the full path of the given text file.
- Open the given text file in read only mode (‘ r ‘) using the open() function.
- Read the opened text file line by line and keep incrementing the line_count by one in each iteration.
- Count the number of words in each line being read using the len() and split() functions.
- Add the number of words in each line to the word_count .
- Close the opened text file using close() function.
- Print the final values of line_count and word_count variables.
4. Python code to count the number of lines and words in a file
Let’s implement the above algorithm to count the number of lines and words through Python code.
In this tutorial, we have learned the following things:
- How to create a text file using Python?
- How to read the content of a text file in Python?
- Algorithm to count the number of lines and words in a given text file.
- How to count the number of lines and words in a text file using Python?
Hope you are clear and are ready to perform these tasks on your own. Thank you and stay tuned with us for more such Python tutorials.
5 Best Ways to Count Words in a Python Program
💡 Problem Formulation: Counting words in a sentence is a common problem tackled in text analysis and processing. It involves determining the number of individual words present in a string of text. For example, the input “Python is awesome!” should yield an output indicating that there are 3 words.
Method 1: Using String’s split() Method
This Python method involves using the built-in string function split() , which divides a string into a list where each word is a list item. The default behavior of split() is to split by white spaces, which makes it an efficient option to count words in a sentence.
Here’s an example:
This code snippet defines a string sentence and uses the split() method to divide it into a list of words. By getting the length of this list using len() , we find the number of words in the original string.
Method 2: Regular Expression with re.findall()
The re.findall() method from Python’s re module can be used to count words by matching against a regular expression pattern that defines a word. This method is especially useful for more complex word definitions that may include apostrophes, hyphens, and other punctuation.
In this snippet, re.findall() finds all occurrences of the pattern which represents full words (denoted by the regex \b\w+\b ), then we count the returned list of words.
Method 3: Using Collections with Counter
The Counter class from Python’s collections module provides a way to count occurrences of elements in a list. It can be used for word count by first splitting the sentence into words, then counting each word’s occurrences in the sentence.
After splitting the sentence into words, Counter is used to tally each word. The sum() of the values in the Counter object gives the total word count.
Method 4: Iterating With a Loop
For educational purposes or fine-grained control, manually iterating over a string to count words can be a good approach. This method requires more lines of code but can be customized to handle specific criteria for word demarcation.
Here, we iterate through the list of words generated by sentence.split() and increment word_count for each iteration, therefore counting the number of words.
Bonus One-Liner Method 5: Using List Comprehension and split()
A more Pythonic and concise way to count words is to use list comprehension in combination with split() . This one-liner approach reduces the iteration and word counting process down to a single line.
This code uses list comprehension to iterate over the words and sum the count of iterations, which directly corresponds to the number of words.
Summary/Discussion
- Method 1: Using String’s split() Method. Strengths: Simple and straightforward. Weaknesses: Assumes words are only separated by whitespace, which may not cover all punctuation and language rules.
- Method 2: Regular Expression with re.findall() . Strengths: More precise and adaptable to different word definitions. Weaknesses: Requires understanding of regular expressions and may have slower performance for large texts.
- Method 3: Using Collections with Counter . Strengths: Efficient for counting word frequencies as well. Weaknesses: Overkill for just counting total words, and slightly more complex.
- Method 4: Iterating With a Loop. Strengths: Offers the most control. Weaknesses: Verbose, and not the most efficient or Pythonic solution.
- Bonus One-Liner Method 5: Using List Comprehension + split() . Strengths: Elegant and compact. Weaknesses: May sacrifice a bit of readability for brevity.
Emily Rosemary Collins is a tech enthusiast with a strong background in computer science, always staying up-to-date with the latest trends and innovations. Apart from her love for technology, Emily enjoys exploring the great outdoors, participating in local community events, and dedicating her free time to painting and photography. Her interests and passion for personal growth make her an engaging conversationalist and a reliable source of knowledge in the ever-evolving world of technology.
Donate to Programming Historian today!
Programming historian, counting word frequencies with python, william j. turkel and adam crymble.
Counting the frequency of specific words in a list can provide illustrative data. This lesson will teach you Python’s easy way to count such frequencies.
Peer-reviewed
- Miriam Posner
reviewed by
- Jim Clifford
- Frederik Elwert
Donate today!
Great Open Access tutorials cost money to produce. Join the growing number of people supporting Programming Historian so we can continue to share knowledge free of charge.
Files Needed For This Lesson
Frequencies, python dictionaries, word-frequency pairs, removing stop words, putting it all together, code syncing, lesson goals.
Your list is now clean enough that you can begin analyzing its contents in meaningful ways. Counting the frequency of specific words in the list can provide illustrative data. Python has an easy way to count frequencies, but it requires the use of a new type of variable: the dictionary . Before you begin working with a dictionary, consider the processes used to calculate frequencies in a list.
If you do not have these files, you can download a ( zip ) file containing all of the code from the previous lessons in this series.
Now we want to count the frequency of each word in our list. You’ve already seen that it is easy to process a list by using a for loop. Try saving and executing the following example. Recall that += tells the program to append something to the end of an existing variable.
Here, we start with a string and split it into a list, as we’ve done before. We then create an (initially empty) list called wordfreq , go through each word in the wordlist , and count the number of times that word appears in the whole list. We then add each word’s count to our wordfreq list. Using the zip operation, we are able to match the first word of the word list with the first number in the frequency list, the second word and second frequency, and so on. We end up with a list of word and frequency pairs. The str function converts any object to a string so that it can be printed.
You should get something like this:
It will pay to study the above code until you understand it before moving on.
Python also includes a very convenient tool called a list comprehension , which can be used to do the same thing as the for loop more economically.
If you study this list comprehension carefully, you will discover that it does exactly the same thing as the for loop in the previous example, but in a condensed manner. Either method will work fine, so use the version that you are most comfortable with.
Generally it is wise to use code you understand rather than code that runs quickest.
At this point we have a list of pairs, where each pair contains a word and its frequency. This list is a bit redundant. If ‘the’ occurs 500 times, then this list contains five hundred copies of the pair (‘the’, 500). The list is also ordered by the words in the original text, rather than listing the words in order from most to least frequent. We can solve both problems by converting it into a dictionary, then printing out the dictionary in order from the most to the least commonly occurring item.
Both strings and lists are sequentially ordered, which means that you can access their contents by using an index, a number that starts at 0. If you have a list containing strings, you can use a pair of indexes to access first a particular string in the list, and then a particular character within that string. Study the examples below.
To keep track of frequencies, we’re going to use another type of Python object, a dictionary. The dictionary is an unordered collection of objects. That means that you can’t use an index to retrieve elements from it. You can, however, look them up by using a key (hence the name “dictionary”). Study the following example.
Dictionaries might be a bit confusing to a new programmer. Try to think of it like a language dictionary. If you don’t know (or remember) exactly how “bijection” differs from “surjection” you can look the two terms up in the Oxford English Dictionary . The same principle applies when you print(d['hello']); except, rather than print a literary definition it prints the value associated with the keyword ‘hello’, as defined by you when you created the dictionary named d . In this case, that value is “0”.
Note that you use curly braces to define a dictionary, but square brackets to access things within it. The keys operation returns a list of keys that are defined in the dictionary.
Building on what we have so far, we want a function that can convert a list of words into a dictionary of word-frequency pairs. The only new command that we will need is dict , which makes a dictionary from a list of pairs. Copy the following and add it to the obo.py module.
We are also going to want a function that can sort a dictionary of word-frequency pairs by descending frequency. Copy this and add it to the obo.py module, too.
We can now write a program which takes a URL and returns word-frequency pairs for the web page, sorted in order of descending frequency. Copy the following program into Komodo Edit, save it as html-to-freq.py and execute it. Study the program and its output carefully before continuing.
When we look at the output of our html-to-freq.py program, we see that a lot of the most frequent words in the text are function words like “the”, “of”, “to” and “and”.
These words are usually the most common in any English language text, so they don’t tell us much that is distinctive about Bowsey’s trial. In general, we are more interested in finding the words that will help us differentiate this text from texts that are about different subjects. So we’re going to filter out the common function words. Words that are ignored like this are known as stop words. We’re going to use the following list, adapted from one posted online by computer scientists at Glasgow . Copy it and put it at the beginning of the obo.py library that you are building.
Now getting rid of the stop words in a list is as easy as using another list comprehension. Add this function to the obo.py module, too.
Now we have everything we need to determine word frequencies for web pages. Copy the following to Komodo Edit, save it as html-to-freq-2.py and execute it.
If all went well, your output should look like this:
Suggested Readings
Lutz, Learning Python
- Ch. 9: Tuples, Files, and Everything Else
- Ch. 11: Assignment, Expressions, and print
- Ch. 12: if Tests
- Ch. 13: while and for Loops
Pilgrim, Diving into Python
- Ch. 7: Regular Expressions
To follow along with future lessons it is important that you have the right files and programs in your “programming-historian” directory. At the end of each lesson in this series you can download the “programming-historian” zip file to make sure you have the correct code.
- programming-historian-5 ( zip sync )
About the authors
Suggested citation.
William J. Turkel and Adam Crymble, "Counting Word Frequencies with Python," Programming Historian 1 (2012), https://doi.org/10.46430/phen0003.
Introduction
You teach English as a foreign language to high school students.
You've decided to base your entire curriculum on TV shows. You need to analyze which words are used, and how often they're repeated.
This will let you choose the simplest shows to start with, and to gradually increase the difficulty as time passes.
Instructions
Your task is to count how many times each word occurs in a subtitle of a drama.
The subtitles from these dramas use only ASCII characters.
The characters often speak in casual English, using contractions like they're or it's . Though these contractions come from two words (e.g. we are ), the contraction ( we're ) is considered a single word.
Words can be separated by any form of punctuation (e.g. ":", "!", or "?") or whitespace (e.g. "\t", "\n", or " "). The only punctuation that does not separate words is the apostrophe in contractions.
Numbers are considered words. If the subtitles say It costs 100 dollars. then 100 will be its own word.
Words are case insensitive. For example, the word you occurs three times in the following sentence:
You come back, you hear me? DO YOU HEAR ME?
The ordering of the word counts in the results doesn't matter.
Here's an example that incorporates several of the elements discussed above:
- simple words
- contractions
- case insensitive words
- punctuation (including apostrophes) to separate words
- different forms of whitespace to separate words
"That's the password: 'PASSWORD 123'!", cried the Special Agent.\nSo I fled.
The mapping for this subtitle would be:
Ready to start Word Count?
Sign up to Exercism to learn and master Python with 17 concepts , 140 exercises , and real human mentoring, all for free.
CopyAssignment
We are Python language experts, a community to solve Python problems, we are a 1.2 Million community on Instagram, now here to help with our blogs.
Word count in Python
Problem statement:.
In this problem of word count in python , we are given a sentence and we need to find the count of all words from the sentence and print them in alphabetical order. For example, sentence=> this is a game of game , result=> a: 1 game: 2 is: 1 of: 1 this: 1
Code for Word count in Python:
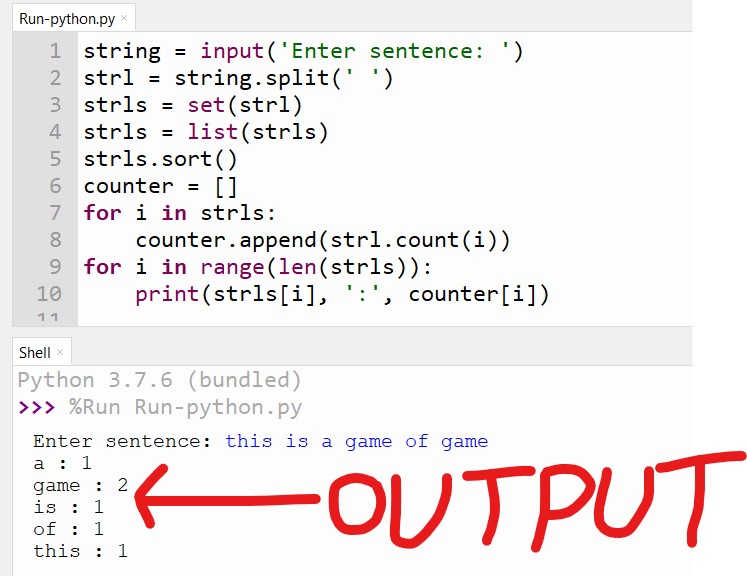
- Hyphenate Letters in Python
- Earthquake in Python | Easy Calculation
- Striped Rectangle in Python
- Perpendicular Words in Python
- Free shipping in Python
- Raj has ordered two electronic items Python | Assignment Expert
- Team Points in Python
- Ticket selling in Cricket Stadium using Python | Assignment Expert
- Split the sentence in Python
- String Slicing in JavaScript
- First and Last Digits in Python | Assignment Expert
- List Indexing in Python
- Date Format in Python | Assignment Expert
- New Year Countdown in Python
- Add Two Polynomials in Python
- Sum of even numbers in Python | Assignment Expert
- Evens and Odds in Python
- A Game of Letters in Python
- Sum of non-primes in Python
- Smallest Missing Number in Python
- String Rotation in Python
- Secret Message in Python
- Word Mix in Python
- Single Digit Number in Python
- Shift Numbers in Python | Assignment Expert
- Weekend in Python
- Temperature Conversion in Python
- Special Characters in Python
- Sum of Prime Numbers in the Input in Python
Author: Harry

Search….
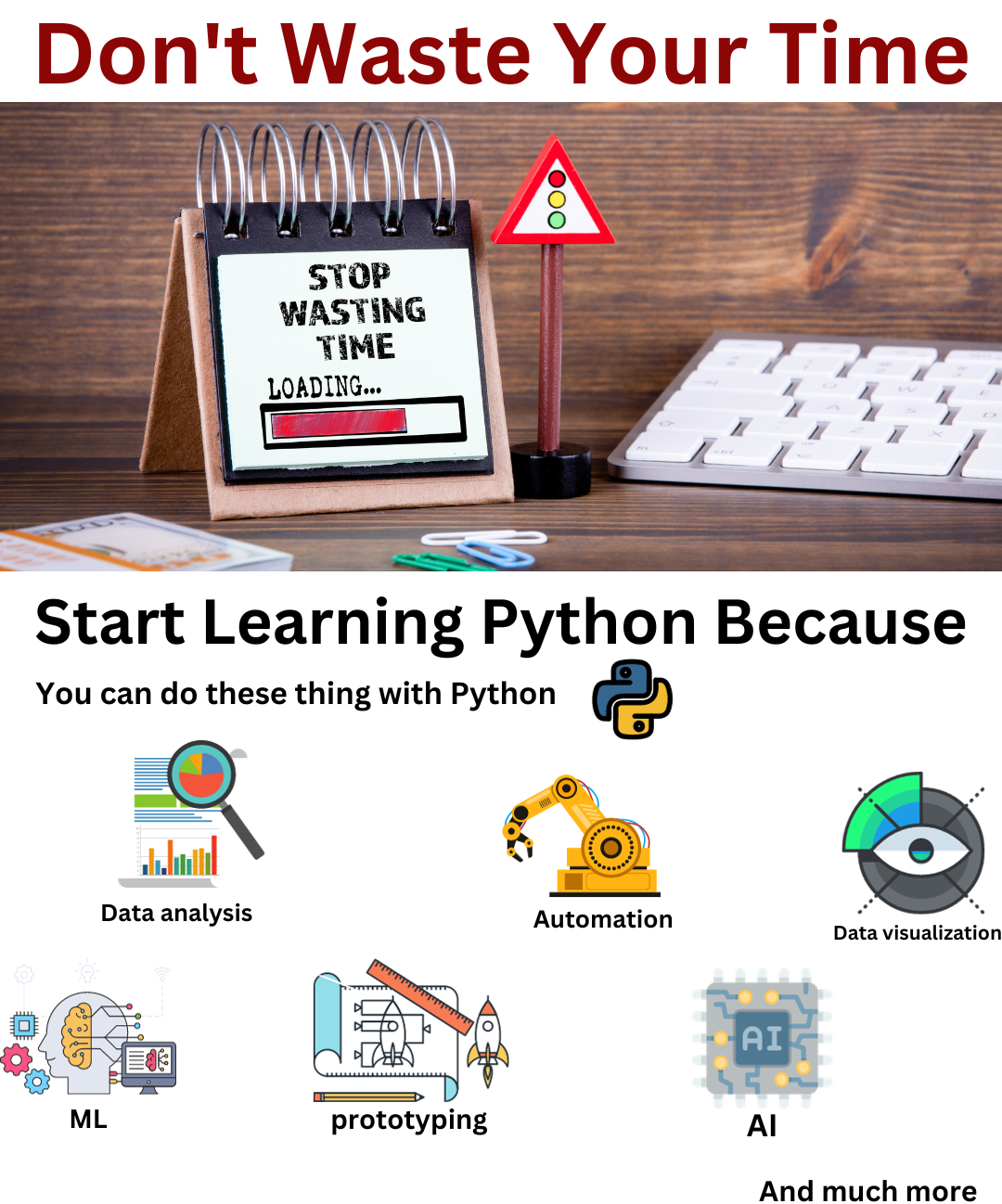
Machine Learning
Data Structures and Algorithms(Python)
Python Turtle
Games with Python
All Blogs On-Site
Python Compiler(Interpreter)
Online Java Editor
Online C++ Editor
Online C Editor
All Editors
Services(Freelancing)
Recent Posts
- Most Underrated Database Trick | Life-Saving SQL Command
- Python List Methods
- Top 5 Free HTML Resume Templates in 2024 | With Source Code
- How to See Connected Wi-Fi Passwords in Windows?
- 2023 Merry Christmas using Python Turtle
© Copyright 2019-2023 www.copyassignment.com. All rights reserved. Developed by copyassignment
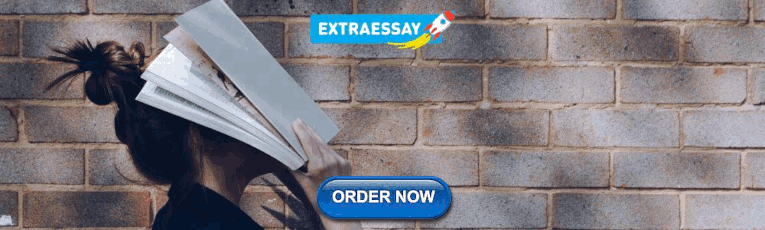
IMAGES
VIDEO
COMMENTS
Question #226370. Given a sentence S, write a program to print the frequency of each word in S. The output order should correspond with the word's input order of appearance.Input. The input will be a single line containing a sentence S.Output. The output contains multiple lines, with each line containing a word and its count separated by ...
Question #166090. Word Count - 2. Given a sentence S, write a program to print the frequency of each word in S, where words are sorted in alphabetical order. Input. The input will be a single line containing a string S. Output. The output contains multiple lines, with each line containing a word and frequency of each word in the given string ...
Answer to Question #165334 in Python for hemanth. Given a sentence S, write a program to print the frequency of each word in S. The output order should correspond with the word's input order of appearance. The output contains multiple lines, with each line containing a word and its count separated by ": " with the order of appearance in the ...
Block 3 - `getWordCount` Function. ```python def getWordCount (filetext): """Return the number of words extracted from the filetext. Note that the duplicate words are also counted. """ words = text_to_words (filetext) return len (words) ```. This function takes the content of a text file (`filetext`) and calculates the word count.
One of the simplest ways to count the number of words in a Python string is by using the split () function. The split function looks like this: sep= None # The delimiter to split on. maxsplit=- 1 # The number of times to split. By default, Python will consider runs of consecutive whitespace to be a single separator.
Note: The shlex.split function returns a list of words, so you can use the len function to count the number of words in the list. The count method can also be used on the list to achieve the same result. Method #6: Using operator.countOf() method
We will build a simple utility called word counter. Those of you who have used Linux will know this as the wc utility. On Linux, you can type: wc <filename>. to get the number of words, lines and characters in a file. The wc utility is quite advanced, of course, since it has been around for a long time.
summary = {} Using a for loop, we will go through the text_split list and if we see a word already in the dictionary, we will increase its count by 1 else, we will add the variable to the dictionary and set its count to 1. For example. for word in text_split: if word in summary: summary[word] = summary[word] + 1 else: summary[word] = 1.
Explore other people's solutions to Word Count in Python, and learn how others have solved the exercise. Learn. Language Tracks. Upskill in 65+ languages #48in24 Challenge. ... Develop fluency in 69 programming languages with our unique blend of learning, practice and mentoring. Exercism is fun, effective and 100% free, forever.
List of 2 element tuples (count, word) I should note that the code used in this blog post and in the video above is available on my github.Please let me know if you have any questions either here, on youtube, or through Twitter!If you want to learn how to utilize the Pandas, Matplotlib, or Seaborn libraries, please consider taking my Python for Data Visualization LinkedIn Learning course.
More assignment statements, this time creating a text variable and a words variable. The file variable from the line above is used here, but again, the details of what, precisely, these lines do will come later in the book. The variable names help us understand the code, though, because they were chosen well.It's possible to guess that this will "read" the file that was "opened ...
RATE COMMENT AND SUBSCRIBE!Website: http://www.syntheticprogramming.comPatreon: https://www.patreon.com/syntheticprogrammingFacebook: https://www.facebook.co...
4. Python code to count the number of lines and words in a file. Let's implement the above algorithm to count the number of lines and words through Python code. # Create two counter variables. # And initialize them with zero. line_count = 0. word_count = 0. # Open the given sample text file using open() function.
Method 2: Regular Expression with re.findall () The re.findall () method from Python's re module can be used to count words by matching against a regular expression pattern that defines a word. This method is especially useful for more complex word definitions that may include apostrophes, hyphens, and other punctuation.
Now we want to count the frequency of each word in our list. You've already seen that it is easy to process a list by using a for loop. Try saving and executing the following example. Recall that += tells the program to append something to the end of an existing variable. # count-list-items-1.py.
In Python 3, use just items where you'd previously use iteritems. The new items() returns a dictionary view object that supports iteration as well as len and in. And of course, in top_words = (words.iteritems(), ... you forgot to call the sorted function.
Your task is to count how many times each word occurs in a subtitle of a drama. The subtitles from these dramas use only ASCII characters. The characters often speak in casual English, using contractions like they're or it's . Though these contractions come from two words (e.g. we are ), the contraction ( we're) is considered a single word.
\$\begingroup\$ I understand this is a Python exercise, but note that in real text word counting is not that easy. 'fish/meat' should be two words but 'A/C' only one. 'Los Angeles' should possibly be one word, while 'The Angels' are two words. It gets worse when you want to split sentences. nltk is a Python library that can help you with this if you're interested in natural language processing ...
In this problem of word count in python, we are given a sentence and we need to find the count of all words from the sentence and print them in alphabetical order. For example, sentence=> this is a game of game, result=> a: 1game: 2is: 1of: 1this: 1.
Python word count program from txt file. I'm trying to write a program that counts the 5 most common words in a txt file. if word not in wordcount: wordcount[word] = 1. else: wordcount[word] += 1. print (k, v) The program as it is counts every word in the .txt file. My question is how to make it so it only counts the 5 most common words in the ...
Word Count Program using Python Streaming Interface - Big Data Analytics Tutorial #WordCountProgram#PythonStreamingInterfacehadoop mapreduce wordcount exampl...