Python Tutorial
File handling, python modules, python numpy, python pandas, python matplotlib, python scipy, machine learning, python mysql, python mongodb, python reference, module reference, python how to, python examples, python arrays.
Note: Python does not have built-in support for Arrays, but Python Lists can be used instead.
Note: This page shows you how to use LISTS as ARRAYS, however, to work with arrays in Python you will have to import a library, like the NumPy library .
Arrays are used to store multiple values in one single variable:
Create an array containing car names:
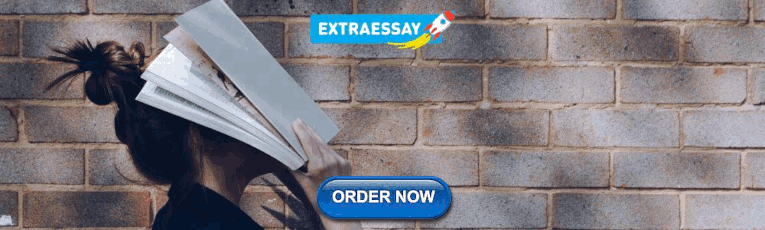
What is an Array?
An array is a special variable, which can hold more than one value at a time.
If you have a list of items (a list of car names, for example), storing the cars in single variables could look like this:
However, what if you want to loop through the cars and find a specific one? And what if you had not 3 cars, but 300?
The solution is an array!
An array can hold many values under a single name, and you can access the values by referring to an index number.
Access the Elements of an Array
You refer to an array element by referring to the index number .
Get the value of the first array item:
Modify the value of the first array item:
The Length of an Array
Use the len() method to return the length of an array (the number of elements in an array).
Return the number of elements in the cars array:
Note: The length of an array is always one more than the highest array index.
Advertisement
Looping Array Elements
You can use the for in loop to loop through all the elements of an array.
Print each item in the cars array:
Adding Array Elements
You can use the append() method to add an element to an array.
Add one more element to the cars array:
Removing Array Elements
You can use the pop() method to remove an element from the array.
Delete the second element of the cars array:
You can also use the remove() method to remove an element from the array.
Delete the element that has the value "Volvo":
Note: The list's remove() method only removes the first occurrence of the specified value.
Array Methods
Python has a set of built-in methods that you can use on lists/arrays.

COLOR PICKER

Report Error
If you want to report an error, or if you want to make a suggestion, do not hesitate to send us an e-mail:
Top Tutorials
Top references, top examples, get certified.

Nick McCullum
Software Developer & Professional Explainer
NumPy Indexing and Assignment
Hey - Nick here! This page is a free excerpt from my $199 course Python for Finance, which is 50% off for the next 50 students.
If you want the full course, click here to sign up.
In this lesson, we will explore indexing and assignment in NumPy arrays.
The Array I'll Be Using In This Lesson
As before, I will be using a specific array through this lesson. This time it will be generated using the np.random.rand method. Here's how I generated the array:
Here is the actual array:
To make this array easier to look at, I will round every element of the array to 2 decimal places using NumPy's round method:
Here's the new array:
How To Return A Specific Element From A NumPy Array
We can select (and return) a specific element from a NumPy array in the same way that we could using a normal Python list: using square brackets.
An example is below:
We can also reference multiple elements of a NumPy array using the colon operator. For example, the index [2:] selects every element from index 2 onwards. The index [:3] selects every element up to and excluding index 3. The index [2:4] returns every element from index 2 to index 4, excluding index 4. The higher endpoint is always excluded.
A few example of indexing using the colon operator are below.
Element Assignment in NumPy Arrays
We can assign new values to an element of a NumPy array using the = operator, just like regular python lists. A few examples are below (note that this is all one code block, which means that the element assignments are carried forward from step to step).
arr[2:5] = 0.5
Returns array([0. , 0. , 0.5, 0.5, 0.5])
As you can see, modifying second_new_array also changed the value of new_array .
Why is this?
By default, NumPy does not create a copy of an array when you reference the original array variable using the = assignment operator. Instead, it simply points the new variable to the old variable, which allows the second variable to make modification to the original variable - even if this is not your intention.
This may seem bizarre, but it does have a logical explanation. The purpose of array referencing is to conserve computing power. When working with large data sets, you would quickly run out of RAM if you created a new array every time you wanted to work with a slice of the array.
Fortunately, there is a workaround to array referencing. You can use the copy method to explicitly copy a NumPy array.
An example of this is below.
As you can see below, making modifications to the copied array does not alter the original.
So far in the lesson, we have only explored how to reference one-dimensional NumPy arrays. We will now explore the indexing of two-dimensional arrays.
Indexing Two-Dimensional NumPy Arrays
To start, let's create a two-dimensional NumPy array named mat :
There are two ways to index a two-dimensional NumPy array:
- mat[row, col]
- mat[row][col]
I personally prefer to index using the mat[row][col] nomenclature because it is easier to visualize in a step-by-step fashion. For example:
You can also generate sub-matrices from a two-dimensional NumPy array using this notation:
Array referencing also applies to two-dimensional arrays in NumPy, so be sure to use the copy method if you want to avoid inadvertently modifying an original array after saving a slice of it into a new variable name.
Conditional Selection Using NumPy Arrays
NumPy arrays support a feature called conditional selection , which allows you to generate a new array of boolean values that state whether each element within the array satisfies a particular if statement.
An example of this is below (I also re-created our original arr variable since its been awhile since we've seen it):
You can also generate a new array of values that satisfy this condition by passing the condition into the square brackets (just like we do for indexing).
An example of this is below:
Conditional selection can become significantly more complex than this. We will explore more examples in this section's associated practice problems.
In this lesson, we explored NumPy array indexing and assignment in thorough detail. We will solidify your knowledge of these concepts further by working through a batch of practice problems in the next section.
- Python »
- 3.12.2 Documentation »
- The Python Standard Library »
- Data Types »
- array — Efficient arrays of numeric values
- Theme Auto Light Dark |
array — Efficient arrays of numeric values ¶
This module defines an object type which can compactly represent an array of basic values: characters, integers, floating point numbers. Arrays are sequence types and behave very much like lists, except that the type of objects stored in them is constrained. The type is specified at object creation time by using a type code , which is a single character. The following type codes are defined:
It can be 16 bits or 32 bits depending on the platform.
Changed in version 3.9: array('u') now uses wchar_t as C type instead of deprecated Py_UNICODE . This change doesn’t affect its behavior because Py_UNICODE is alias of wchar_t since Python 3.3.
Deprecated since version 3.3, will be removed in version 4.0.
The actual representation of values is determined by the machine architecture (strictly speaking, by the C implementation). The actual size can be accessed through the array.itemsize attribute.
The module defines the following item:
A string with all available type codes.
The module defines the following type:
A new array whose items are restricted by typecode , and initialized from the optional initializer value, which must be a bytes or bytearray object, a Unicode string, or iterable over elements of the appropriate type.
If given a bytes or bytearray object, the initializer is passed to the new array’s frombytes() method; if given a Unicode string, the initializer is passed to the fromunicode() method; otherwise, the initializer’s iterator is passed to the extend() method to add initial items to the array.
Array objects support the ordinary sequence operations of indexing, slicing, concatenation, and multiplication. When using slice assignment, the assigned value must be an array object with the same type code; in all other cases, TypeError is raised. Array objects also implement the buffer interface, and may be used wherever bytes-like objects are supported.
Raises an auditing event array.__new__ with arguments typecode , initializer .
The typecode character used to create the array.
The length in bytes of one array item in the internal representation.
Append a new item with value x to the end of the array.
Return a tuple (address, length) giving the current memory address and the length in elements of the buffer used to hold array’s contents. The size of the memory buffer in bytes can be computed as array.buffer_info()[1] * array.itemsize . This is occasionally useful when working with low-level (and inherently unsafe) I/O interfaces that require memory addresses, such as certain ioctl() operations. The returned numbers are valid as long as the array exists and no length-changing operations are applied to it.
When using array objects from code written in C or C++ (the only way to effectively make use of this information), it makes more sense to use the buffer interface supported by array objects. This method is maintained for backward compatibility and should be avoided in new code. The buffer interface is documented in Buffer Protocol .
“Byteswap” all items of the array. This is only supported for values which are 1, 2, 4, or 8 bytes in size; for other types of values, RuntimeError is raised. It is useful when reading data from a file written on a machine with a different byte order.
Return the number of occurrences of x in the array.
Append items from iterable to the end of the array. If iterable is another array, it must have exactly the same type code; if not, TypeError will be raised. If iterable is not an array, it must be iterable and its elements must be the right type to be appended to the array.
Appends items from the bytes-like object , interpreting its content as an array of machine values (as if it had been read from a file using the fromfile() method).
New in version 3.2: fromstring() is renamed to frombytes() for clarity.
Read n items (as machine values) from the file object f and append them to the end of the array. If less than n items are available, EOFError is raised, but the items that were available are still inserted into the array.
Append items from the list. This is equivalent to for x in list: a.append(x) except that if there is a type error, the array is unchanged.
Extends this array with data from the given Unicode string. The array must have type code 'u' ; otherwise a ValueError is raised. Use array.frombytes(unicodestring.encode(enc)) to append Unicode data to an array of some other type.
Return the smallest i such that i is the index of the first occurrence of x in the array. The optional arguments start and stop can be specified to search for x within a subsection of the array. Raise ValueError if x is not found.
Changed in version 3.10: Added optional start and stop parameters.
Insert a new item with value x in the array before position i . Negative values are treated as being relative to the end of the array.
Removes the item with the index i from the array and returns it. The optional argument defaults to -1 , so that by default the last item is removed and returned.
Remove the first occurrence of x from the array.
Reverse the order of the items in the array.
Convert the array to an array of machine values and return the bytes representation (the same sequence of bytes that would be written to a file by the tofile() method.)
New in version 3.2: tostring() is renamed to tobytes() for clarity.
Write all items (as machine values) to the file object f .
Convert the array to an ordinary list with the same items.
Convert the array to a Unicode string. The array must have a type 'u' ; otherwise a ValueError is raised. Use array.tobytes().decode(enc) to obtain a Unicode string from an array of some other type.
The string representation of array objects has the form array(typecode, initializer) . The initializer is omitted if the array is empty, otherwise it is a Unicode string if the typecode is 'u' , otherwise it is a list of numbers. The string representation is guaranteed to be able to be converted back to an array with the same type and value using eval() , so long as the array class has been imported using from array import array . Variables inf and nan must also be defined if it contains corresponding floating point values. Examples:
Packing and unpacking of heterogeneous binary data.
Packing and unpacking of External Data Representation (XDR) data as used in some remote procedure call systems.
The NumPy package defines another array type.
Previous topic
bisect — Array bisection algorithm
weakref — Weak references
- Report a Bug
- Show Source
Guide to Arrays in Python

- Introduction
Imagine you have a playlist of your favorite songs on your phone. This playlist is a list where each song is placed in a specific order. You can play the first song, skip to the second, jump to the fifth, and so on. This playlist is a lot like an array in computer programming.
Arrays stand as one of the most fundamental and widely used data structures.
In essence, an array is a structured way to store multiple items (like numbers, characters, or even other arrays) in a specific order, and you can quickly access, modify, or remove any item if you know its position (index).
In this guide, we'll give you a comprehensive overview of the array data structure. First of all, we'll take a look at what arrays are and what are their main characteristics. We'll then transition into the world of Python, exploring how arrays are implemented, manipulated, and applied in real-world scenarios.
- Understanding the Array Data Structure
Arrays are among the oldest and most fundamental data structures used in computer science and programming. Their simplicity, combined with their efficiency in certain operations, makes them a staple topic for anyone delving into the realm of data management and manipulation.
An array is a collection of items, typically of the same type , stored in contiguous memory locations .
This contiguous storage allows arrays to provide constant-time access to any element, given its index. Each item in an array is called an element , and the position of an element in the array is defined by its index , which usually starts from zero .
For instance, consider an array of integers: [10, 20, 30, 40, 50] . Here, the element 20 has an index of 1 :
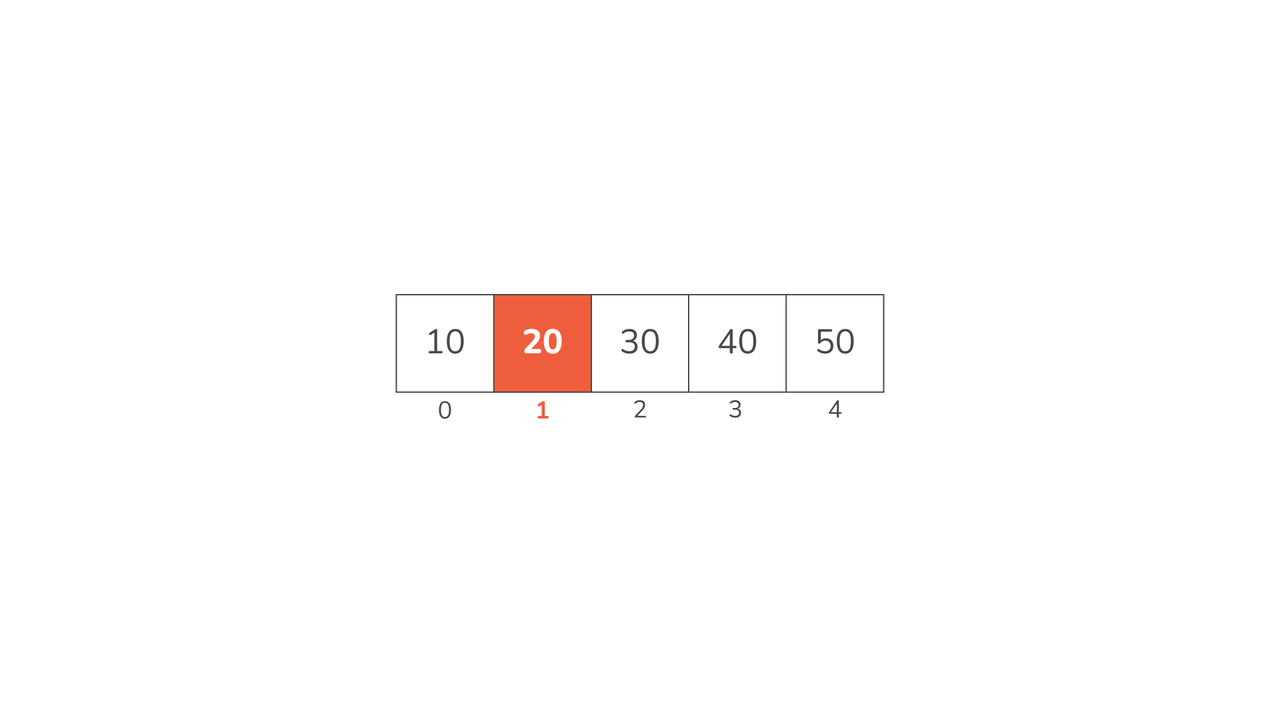
There are multiple advantages of using arrays to store our data. For example, due to their memory layout, arrays allow for O(1) (constant) time complexity when accessing an element by its index. This is particularly beneficial when we need random access to elements. Additionally, arrays are stored in contiguous memory locations , which can lead to better cache locality and overall performance improvements in certain operations. Another notable advantage of using arrays is that, since arrays have a fixed size once declared, it's easier to manage memory and avoid unexpected overflows or out-of-memory errors.
Note : Arrays are especially useful in scenarios where the size of the collection is known in advance and remains constant , or where random access is more frequent than insertions and deletions.
On the other side, arrays come with their own set of limitations . One of the primary limitations of traditional arrays is their fixed size . Once an array is created, its size cannot be changed. This can lead to issues like wasted memory (if the array is too large) or the need for resizing (if the array is too small). Besides that, inserting or deleting an element in the middle of an array requires shifting of elements, leading to O(n) time complexity for these operations.
To sum this all up, let's illustrate the main characteristics of arrays using the song playlist example from the beginning of this guide. An array is a data structure that:
Is Indexed: Just like each song on your playlist has a number (1, 2, 3, ...), each element in an array has an index. But, in most programming languages, the index starts at 0. So, the first item is at index 0, the second at index 1, and so on.
Has Fixed Size : When you create a playlist for, say, 10 songs, you can't add an 11th song without removing one first. Similarly, arrays have a fixed size. Once you create an array of a certain size, you can't add more items than its capacity.
Is Homogeneous : All songs in your playlist are music tracks. Similarly, all elements in an array are of the same type. If you have an array of integers, you can't suddenly store a text string in it.
Has Direct Access : If you want to listen to the 7th song in your playlist, you can jump directly to it. Similarly, with arrays, you can instantly access any element if you know its index.
Contiguous Memory : This is a bit more technical. When an array is created in a computer's memory, it occupies a continuous block of memory. Think of it like a row of adjacent lockers in school. Each locker is next to the other, with no gaps in between.
- Python and Arrays
Python, known for its flexibility and ease of use, offers multiple ways to work with arrays. While Python does not have a native array data structure like some other languages, it provides powerful alternatives that can function similarly and even offer extended capabilities.
At first glance, Python's list might seem synonymous with an array, but there are subtle differences and nuances to consider:
Looking at this table, it comes naturally to ask - "When to use which?" . Well, if you need a collection that can grow or shrink dynamically and can hold mixed data types, Python's list is the way to go. However, for scenarios requiring a more memory-efficient collection with elements of the same type, you might consider using Python's array module or external libraries like NumPy.
- The array Module in Python
When most developers think of arrays in Python, they often default to thinking about lists. However, Python offers a more specialized array structure through its built-in array module. This module provides a space-efficient storage of basic C-style data types in Python.
While Python lists are incredibly versatile and can store any type of object, they can sometimes be overkill, especially when you only need to store a collection of basic data types, like integers or floats. The array module provides a way to create arrays that are more memory efficient than lists for specific data types.
- Creating an Array
To use the array module, you first need to import it:
Once imported, you can create an array using the array() constructor:
Here, the 'i' argument indicates that the array will store signed integers . There are several other type codes available, such as 'f' for floats and 'd' for doubles.
- Accessing and Modifying Elements
You can access and modify elements in an array just like you would with a list:
And now, let's modify the element by changing it's value to 6 :
- Array Methods
The array module provides several methods to manipulate arrays:
append() - Adds an element to the end of the array:
extend() - Appends iterable elements to the end:
pop() - Removes and returns the element at the given position:
remove() : Removes the first occurrence of the specified value:
reverse() : Reverses the order of the array:
Note: There are more methods than we listed here. Refer to the official Python documentation to see a list of all available methods in the array module.
While the array module offers a more memory-efficient way to store basic data types, it's essential to remember its limitations . Unlike lists, arrays are homogeneous . This means all elements in the array must be of the same type. Also, you can only store basic C-style data types in arrays. If you need to store custom objects or other Python types, you'll need to use a list or another data structure.
- NumPy Arrays
NumPy, short for Numerical Python, is a foundational package for numerical computations in Python. One of its primary features is its powerful N-dimensional array object , which offers fast operations on arrays, including mathematical, logical, shape manipulation, and more.
NumPy arrays are more versatile than Python's built-in array module and are a staple in data science and machine learning projects.
- Why Use NumPy Arrays?
The first thing that comes to mind is performance . NumPy arrays are implemented in C and allow for efficient memory storage and faster operations due to optimized algorithms and the benefits of contiguous memory storage.
While Python's built-in arrays are one-dimensional, NumPy arrays can be multi-dimensional , making them ideal for representing matrices or tensors.
Check out our hands-on, practical guide to learning Git, with best-practices, industry-accepted standards, and included cheat sheet. Stop Googling Git commands and actually learn it!
Finally, NumPy provides a vast array of functions to operate on these arrays, from basic arithmetic to advanced mathematical operations, reshaping, splitting, and more.
Note: When you know the size of the data in advance, pre-allocating memory for arrays (especially in NumPy) can lead to performance improvements.
- Creating a NumPy Array
To use NumPy, you first need to install it ( pip install numpy ) and then import it:
Once imported, you can create a NumPy array using the array() function:
You can also create multi-dimensional arrays:
This will give us:
Besides these basic ways we can create arrays, NumPy provides us with other clever ways we can create arrays. One of which is the arange() method. It creates arrays with regularly incrementing values:
Another one is the linspace() method, which creates arrays with a specified number of elements, spaced equally between specified beginning and end values:
Accessing and modifying elements in a NumPy array is intuitive:
Doing pretty much the same for multi-dimensional arrays:
Will change the value of the element in the second row (index 1 ) and the third column (index 2 ):
- Changing the Shape of an Array
NumPy offers many functions and methods to manipulate and operate on arrays. For example, you can use the reshape() method to change the shape of an array . Say we have a simple array:
And we want to reshape it to a 3x4 matrix. All you need to do is use the reshape() method with desired dimensions passed as arguments:
This will result in:
- Matrix Multiplication
The numpy.dot() method is used for matrix multiplication . It returns the dot product of two arrays. For one-dimensional arrays, it is the inner product of the arrays. For 2-dimensional arrays, it is equivalent to matrix multiplication , and for N-D, it is a sum product over the last axis of the first array and the second-to-last of the second array.
Let's see how it works. First, let's compute the dot product of two 1-D arrays (the inner product of the vectors):
32 is, in fact, the inner product of the two arrays - (1 4 + 2 5 + 3*6) . Next, we can perform matrix multiplication of two 2-D arrays:
Which will give us:
NumPy arrays are a significant step up from Python's built-in lists and the array module, especially for scientific and mathematical computations. Their efficiency, combined with the rich functionality provided by the NumPy library, makes them an indispensable tool for anyone looking to do numerical operations in Python.
Advice: This is just a quick overview of what you can do with the NumPy library. For more information about the library, you can read our "NumPy Tutorial: A Simple Example-Based Guide"
Arrays, a cornerstone of computer science and programming, have proven their worth time and again across various applications and domains. In Python, this fundamental data structure, through its various incarnations like lists, the array module, and the powerful NumPy arrays, offers developers a blend of efficiency, versatility, and simplicity.
Throughout this guide, we've journeyed from the foundational concepts of arrays to their practical applications in Python. We've seen how arrays, with their memory-contiguous nature, provide rapid access times, and how Python's dynamic lists bring an added layer of flexibility. We've also delved into the specialized world of NumPy, where arrays transform into powerful tools for numerical computation.
You might also like...
- Guide to Hash Tables in Python
- Dictionaries vs Arrays in Python - Deep Dive
- Modified Preorder Tree Traversal in Django
- Stacks and Queues in Python
- Python Performance Optimization
Improve your dev skills!
Get tutorials, guides, and dev jobs in your inbox.
No spam ever. Unsubscribe at any time. Read our Privacy Policy.
In this article
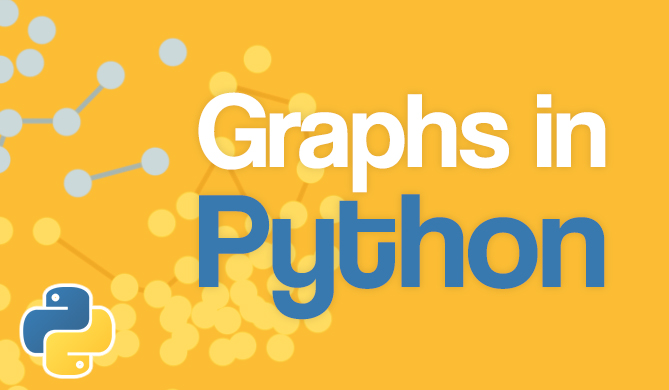
Graphs in Python - Theory and Implementation
Graphs are an extremely versatile data structure. More so than most people realize! Graphs can be used to model practically anything, given their nature of...
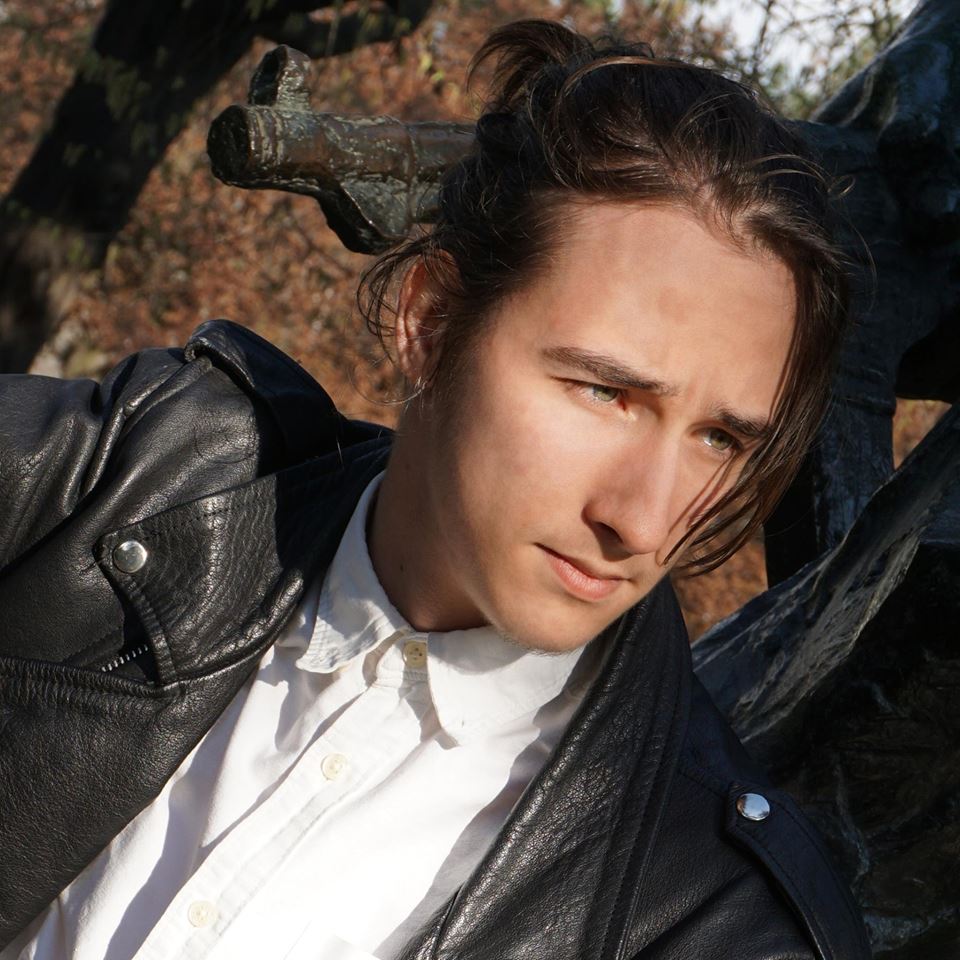
Data Visualization in Python with Matplotlib and Pandas
Data Visualization in Python with Matplotlib and Pandas is a course designed to take absolute beginners to Pandas and Matplotlib, with basic Python knowledge, and...
© 2013- 2024 Stack Abuse. All rights reserved.
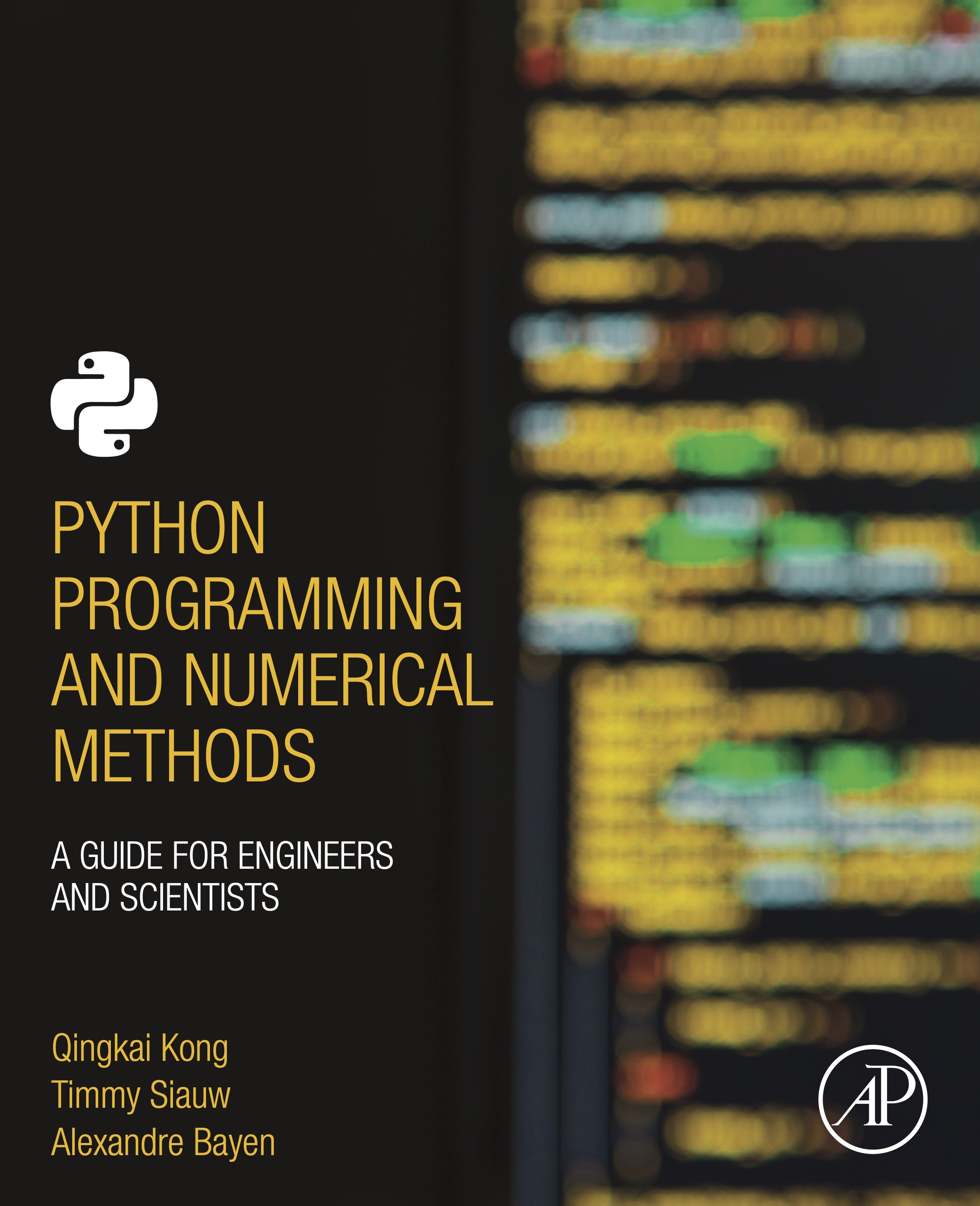
Python Numerical Methods

This notebook contains an excerpt from the Python Programming and Numerical Methods - A Guide for Engineers and Scientists , the content is also available at Berkeley Python Numerical Methods .
The copyright of the book belongs to Elsevier. We also have this interactive book online for a better learning experience. The code is released under the MIT license . If you find this content useful, please consider supporting the work on Elsevier or Amazon !
< 2.6 Data Structure - Dictionaries | Contents | 2.8 Summary and Problems >
Introducing Numpy Arrays ¶
In the 2nd part of this book, we will study the numerical methods by using Python. We will use array/matrix a lot later in the book. Therefore, here we are going to introduce the most common way to handle arrays in Python using the Numpy module . Numpy is probably the most fundamental numerical computing module in Python.
NumPy is important in scientific computing, it is coded both in Python and C (for speed). On its website, a few important features for Numpy is listed:
a powerful N-dimensional array object
sophisticated (broadcasting) functions
tools for integrating C/C++ and Fortran code
useful linear algebra, Fourier transform, and random number capabilities
Here we will only introduce you the Numpy array which is related to the data structure, but we will gradually touch on other aspects of Numpy in the following chapters.
In order to use Numpy module, we need to import it first. A conventional way to import it is to use “np” as a shortened name.
WARNING! Of course, you could call it any name, but conventionally, “np” is accepted by the whole community and it is a good practice to use it for obvious purposes.
To define an array in Python, you could use the np.array function to convert a list.
TRY IT! Create the following arrays:
\(x = \begin{pmatrix} 1 & 4 & 3 \\ \end{pmatrix}\)
\(y = \begin{pmatrix} 1 & 4 & 3 \\ 9 & 2 & 7 \\ \end{pmatrix}\)
NOTE! A 2-D array could use a nested lists to represent, with the inner list represent each row.
Many times we would like to know the size or length of an array. The array shape attribute is called on an array M and returns a 2 × 3 array where the first element is the number of rows in the matrix M and the second element is the number of columns in M. Note that the output of the shape attribute is a tuple. The size attribute is called on an array M and returns the total number of elements in matrix M.
TRY IT! Find the rows, columns and the total size for array y.
NOTE! You may notice the difference that we only use y.shape instead of y.shape() , this is because shape is an attribute rather than a method in this array object. We will introduce more of the object-oriented programming in a later chapter. For now, you need to remember that when we call a method in an object, we need to use the parentheses, while the attribute don’t.
Very often we would like to generate arrays that have a structure or pattern. For instance, we may wish to create the array z = [1 2 3 … 2000]. It would be very cumbersome to type the entire description of z into Python. For generating arrays that are in order and evenly spaced, it is useful to use the arange function in Numpy.
TRY IT! Create an array z from 1 to 2000 with an increment 1.
Using the np.arange , we could create z easily. The first two numbers are the start and end of the sequence, and the last one is the increment. Since it is very common to have an increment of 1, if an increment is not specified, Python will use a default value of 1. Therefore np.arange(1, 2000) will have the same result as np.arange(1, 2000, 1) . Negative or noninteger increments can also be used. If the increment “misses” the last value, it will only extend until the value just before the ending value. For example, x = np.arange(1,8,2) would be [1, 3, 5, 7].
TRY IT! Generate an array with [0.5, 1, 1.5, 2, 2.5].
Sometimes we want to guarantee a start and end point for an array but still have evenly spaced elements. For instance, we may want an array that starts at 1, ends at 8, and has exactly 10 elements. For this purpose you can use the function np.linspace . linspace takes three input values separated by commas. So A = linspace(a,b,n) generates an array of n equally spaced elements starting from a and ending at b.
TRY IT! Use linspace to generate an array starting at 3, ending at 9, and containing 10 elements.
Getting access to the 1D numpy array is similar to what we described for lists or tuples, it has an index to indicate the location. For example:
For 2D arrays, it is slightly different, since we have rows and columns. To get access to the data in a 2D array M, we need to use M[r, c], that the row r and column c are separated by comma. This is referred to as array indexing. The r and c could be single number, a list and so on. If you only think about the row index or the column index, than it is similar to the 1D array. Let’s use the \(y = \begin{pmatrix} 1 & 4 & 3 \\ 9 & 2 & 7 \\ \end{pmatrix}\) as an example.
TRY IT! Get the element at first row and 2nd column of array y .
TRY IT! Get the first row of array y .
TRY IT! Get the last column of array y .
TRY IT! Get the first and third column of array y .
There are some predefined arrays that are really useful. For example, the np.zeros , np.ones , and np.empty are 3 useful functions. Let’s see the examples.
TRY IT! Generate a 3 by 5 array with all the as 0.
TRY IT! Generate a 5 by 3 array with all the element as 1.
NOTE! The shape of the array is defined in a tuple with row as the first item, and column as the second. If you only need a 1D array, then it could be only one number as the input: np.ones(5) .
TRY IT! Generate a 1D empty array with 3 elements.
NOTE! The empty array is not really empty, it is filled with random very small numbers.
You can reassign a value of an array by using array indexing and the assignment operator. You can reassign multiple elements to a single number using array indexing on the left side. You can also reassign multiple elements of an array as long as both the number of elements being assigned and the number of elements assigned is the same. You can create an array using array indexing.
TRY IT! Let a = [1, 2, 3, 4, 5, 6]. Reassign the fourth element of A to 7. Reassign the first, second, and thrid elements to 1. Reassign the second, third, and fourth elements to 9, 8, and 7.
TRY IT! Create a zero array b with shape 2 by 2, and set \(b = \begin{pmatrix} 1 & 2 \\ 3 & 4 \\ \end{pmatrix}\) using array indexing.
WARNING! Although you can create an array from scratch using indexing, we do not advise it. It can confuse you and errors will be harder to find in your code later. For example, b[1, 1] = 1 will give the result \(b = \begin{pmatrix} 0 & 0 \\ 0 & 1 \\ \end{pmatrix}\) , which is strange because b[0, 0], b[0, 1], and b[1, 0] were never specified.
Basic arithmetic is defined for arrays. However, there are operations between a scalar (a single number) and an array and operations between two arrays. We will start with operations between a scalar and an array. To illustrate, let c be a scalar, and b be a matrix.
b + c , b − c , b * c and b / c adds a to every element of b, subtracts c from every element of b, multiplies every element of b by c, and divides every element of b by c, respectively.
TRY IT! Let \(b = \begin{pmatrix} 1 & 2 \\ 3 & 4 \\ \end{pmatrix}\) . Add and substract 2 from b. Multiply and divide b by 2. Square every element of b. Let c be a scalar. On your own, verify the reflexivity of scalar addition and multiplication: b + c = c + b and cb = bc.
Describing operations between two matrices is more complicated. Let b and d be two matrices of the same size. b − d takes every element of b and subtracts the corresponding element of d. Similarly, b + d adds every element of d to the corresponding element of b.
TRY IT! Let \(b = \begin{pmatrix} 1 & 2 \\ 3 & 4 \\ \end{pmatrix}\) and \(d = \begin{pmatrix} 3 & 4 \\ 5 & 6 \\ \end{pmatrix}\) . Compute b + d and b - d.
There are two different kinds of matrix multiplication (and division). There is element-by-element matrix multiplication and standard matrix multiplication. For this section, we will only show how element-by-element matrix multiplication and division work. Standard matrix multiplication will be described in later chapter on Linear Algebra. Python takes the * symbol to mean element-by-element multiplication. For matrices b and d of the same size, b * d takes every element of b and multiplies it by the corresponding element of d. The same is true for / and **.
TRY IT! Compute b * d, b / d, and b**d.
The transpose of an array, b, is an array, d, where b[i, j] = d[j, i]. In other words, the transpose switches the rows and the columns of b. You can transpose an array in Python using the array method T .
TRY IT! Compute the transpose of array b.
Numpy has many arithmetic functions, such as sin, cos, etc., can take arrays as input arguments. The output is the function evaluated for every element of the input array. A function that takes an array as input and performs the function on it is said to be vectorized .
TRY IT! Compute np.sqrt for x = [1, 4, 9, 16].
Logical operations are only defined between a scalar and an array and between two arrays of the same size. Between a scalar and an array, the logical operation is conducted between the scalar and each element of the array. Between two arrays, the logical operation is conducted element-by-element.
TRY IT! Check which elements of the array x = [1, 2, 4, 5, 9, 3] are larger than 3. Check which elements in x are larger than the corresponding element in y = [0, 2, 3, 1, 2, 3].
Python can index elements of an array that satisfy a logical expression.
TRY IT! Let x be the same array as in the previous example. Create a variable y that contains all the elements of x that are strictly bigger than 3. Assign all the values of x that are bigger than 3, the value 0.
Learn Python practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn python interactively, python introduction.
- Getting Started
- Keywords and Identifier
- Python Comments
- Python Variables
- Python Data Types
- Python Type Conversion
- Python I/O and Import
- Python Operators
- Python Namespace
Python Flow Control
- Python if...else
- Python for Loop
- Python while Loop
- Python break and continue
- Python Pass
Python Functions
- Python Function
- Function Argument
- Python Recursion
- Anonymous Function
- Global, Local and Nonlocal
- Python Global Keyword
- Python Modules
- Python Package
Python Datatypes
- Python Numbers
Python List
- Python Tuple
- Python String
- Python Dictionary
Python Files
- Python File Operation
- Python Directory
- Python Exception
- Exception Handling
- User-defined Exception
Python Object & Class
- Classes & Objects
- Python Inheritance
- Multiple Inheritance
- Operator Overloading
Python Advanced Topics
- Python Iterator
- Python Generator
- Python Closure
- Python Decorators
- Python Property
- Python RegEx
Python Date and time
- Python datetime Module
- Python datetime.strftime()
- Python datetime.strptime()
- Current date & time
- Get current time
- Timestamp to datetime
- Python time Module
- Python time.sleep()
Python Tutorials
Python Matrices and NumPy Arrays
Python Numbers, Type Conversion and Mathematics
Python bytearray()
- Python bytes()
Python Array
In this tutorial, we will focus on a module named array . The array module allows us to store a collection of numeric values.
Note: When people say arrays in Python, more often than not, they are talking about Python lists . If that's the case, visit the Python list tutorial.
- Creating Python Arrays
To create an array of numeric values, we need to import the array module. For example:
Here, we created an array of float type. The letter d is a type code. This determines the type of the array during creation.
Commonly used type codes are listed as follows:
We will not discuss different C types in this article. We will use two type codes in this entire article: i for integers and d for floats.
Note : The u type code for Unicode characters is deprecated since version 3.3. Avoid using as much as possible.
- Accessing Python Array Elements
We use indices to access elements of an array:
Note : The index starts from 0 (not 1) similar to lists.
- Slicing Python Arrays
We can access a range of items in an array by using the slicing operator : .
- Changing and Adding Elements
Arrays are mutable; their elements can be changed in a similar way as lists.
We can add one item to the array using the append() method, or add several items using the extend() method.
We can also concatenate two arrays using + operator.
- Removing Python Array Elements
We can delete one or more items from an array using Python's del statement.
We can use the remove() method to remove the given item, and pop() method to remove an item at the given index.
Check this page to learn more about Python array and array methods .
- Python Lists Vs Arrays
In Python, we can treat lists as arrays. However, we cannot constrain the type of elements stored in a list. For example:
If you create arrays using the array module, all elements of the array must be of the same numeric type.
- When to use arrays?
Lists are much more flexible than arrays. They can store elements of different data types including strings. And, if you need to do mathematical computation on arrays and matrices, you are much better off using something like NumPy .
So, what are the uses of arrays created from the Python array module?
The array.array type is just a thin wrapper on C arrays which provides space-efficient storage of basic C-style data types. If you need to allocate an array that you know will not change, then arrays can be faster and use less memory than lists.
Unless you don't really need arrays (array module may be needed to interface with C code), the use of the array module is not recommended.
Table of Contents
- Introduction
Sorry about that.
Related Tutorials
Python Tutorial
Python Library
NumPy: Get and set values in an array using various indexing
This article explains how to get and set values, such as individual elements or subarrays (e.g., rows or columns), in a NumPy array ( ndarray ) using various indexing.
- Indexing on ndarrays — NumPy v1.26 Manual
Basics of selecting values in an ndarray
Specify with integers, specify with slices, specify with a list of boolean values: boolean indexing, specify with a list of integers: fancy indexing, combine different specification formats, assign new values to selected ranges, views and copies.
See the following articles for information on deleting, concatenating, and adding to ndarray .
- NumPy: Delete rows/columns from an array with np.delete()
- NumPy: Concatenate arrays with np.concatenate, np.stack, etc.
- NumPy: Insert elements, rows, and columns into an array with np.insert()
The NumPy version used in this article is as follows. Note that functionality may vary between versions.
Individual elements or subarrays (such as rows or columns) in an ndarray can be selected by specifying their positions or ranges in each dimension with commas, as in [○, ○, ○, ...] . The trailing : can be omitted, making [○, ○, :, :] equivalent to [○, ○] .
For a 2D array, [i] selects the ith row, and [:, i] selects the ith column (indexes start from 0 ). More details will be provided later.
Positions in each dimension can be specified not only as integers but also in other formats such as lists or slices, allowing for the selection of any subarray.
Positions (indexes) are specified as integers ( int ).
Indexes start from 0 , and negative values can be used to specify positions from the end ( -1 represents the last). Specifying a non-existent position results in an error.
The same applies to multi-dimensional arrays. Positions are specified for each dimension.
You can omit the specification of later dimensions.
In a 2D array, [i] , equivalent to [i, :] , selects the ith row as a 1D array, and [:, i] selects the ith column.
Ranges can be selected with slices ( start:end:step ).
- NumPy: Slicing ndarray
Example with a 1D array:
Example with a 2D array:
The trailing : can be omitted.
Using a slice i:i+1 selects a single row or column, preserving the array's dimensions, unlike selection with an integer ( int ), which reduces the dimensions.
- NumPy: Get the number of dimensions, shape, and size of ndarray
Slices preserve the original array's dimensions, while integers reduce them. This difference can affect outcomes or cause errors in operations like concatenation, even with the same range selected.
Specifying a list or ndarray of Boolean values ( True or False ) matching the dimensions' sizes selects True positions, similar to masking.
An error occurs if the sizes do not match.
Rows or columns can be extracted using a slice : .
Note that specifying a list of Boolean values for multiple dimensions simultaneously does not yield the expected result. Using np.ix_() is necessary.
- numpy.ix_ — NumPy v1.26 Manual
As with slices, selecting a range of width 1 (a single row or column) preserves the original array's number of dimensions.
A comparison on an ndarray yields a Boolean ndarray . Using this for indexing with [] selects True values, producing a flattened 1D array.
- NumPy: Compare two arrays element-wise
Specify multiple conditions using & (AND), | (OR), and ~ (NOT) with parentheses () . Using and , or , not , or omitting parentheses results in an error.
- How to fix "ValueError: The truth value ... is ambiguous" in NumPy, pandas
For methods of extracting rows or columns that meet certain conditions using Boolean indexing, refer to the following article.
- NumPy: Extract or delete elements, rows, and columns that satisfy the conditions
It is also possible to select ranges with a list or ndarray of integers.
Order can be inverted or repeated, and using negative values is allowed. Essentially, it involves creating a new array by selecting specific positions from the original array.
As with Boolean indexing, specifying lists for multiple dimensions simultaneously does not yield the expected result. Using np.ix_() is necessary.
As in the 1D example, the order can be inverted or repeated, and negative values are also permissible.
When selecting with a list of one element, the original array's number of dimensions is preserved, in contrast to specifying with an integer.
Different formats can be used to specify each dimension.
A combination of a list of Boolean values and a list of integers requires the use of np.ix_() .
Note that np.ix_() can only accept 1D lists or arrays.
For example, when specifying multiple lists for arrays of three dimensions or more, np.ix_() is required. However, it cannot be combined with integers or slices in the same selection operation.
Integers can be specified as lists containing a single element. In this case, the resulting array retains the same number of dimensions as the original ndarray .
You can use range() to achieve similar functionality as slices. For example, to simulate slicing, retrieve the size of the target dimension using the shape attribute and pass it to range() as in range(a.shape[n])[::] .
- How to use range() in Python
You can assign new values to selected ranges in an ndarray .
Specifying a scalar value on the right side assigns that value to all elements in the selected range on the left side.
Arrays can also be specified on the right side.
If the shape of the selected range on the left side matches that of the array on the right side, it is directly assigned. Non-contiguous locations pose no problem.
If the shape of the selected range on the left side does not match that of the array on the right side, it is assigned through broadcasting.
An error occurs if the shapes cannot be broadcast.
For more information on broadcasting, refer to the following article.
- NumPy: Broadcasting rules and examples
The specification format used for each dimension when selecting subarrays determines whether a view or a copy of the original array is returned.
For example, using slices returns a view.
Whether two arrays refer to the same memory can be checked using np.shares_memory() .
- NumPy: Views and copies of arrays
In the case of a view, changing the value in the selected subarray also changes the value in the original array, and vice versa.
Boolean indexing or fancy indexing returns a copy.
In this case, changing the value in the selected subarray does not affect the original array, and vice versa.
To create a copy of a subarray selected with a slice and process it separately from the original ndarray , use the copy() method.
When combining different specification formats, using Boolean or fancy indexing returns a copy.
Using integers and slices returns a view.
Related Categories
Related articles.
- NumPy: Functions ignoring NaN (np.nansum, np.nanmean, etc.)
- NumPy: Generate random numbers with np.random
- NumPy: Ellipsis (...) for ndarray
- List of NumPy articles
- NumPy: arange() and linspace() to generate evenly spaced values
- NumPy: Trigonometric functions (sin, cos, tan, arcsin, arccos, arctan)
- NumPy: np.sign(), np.signbit(), np.copysign()
- NumPy: Set the display format for ndarray
- NumPy: Meaning of the axis parameter (0, 1, -1)
- NumPy: Read and write CSV files (np.loadtxt, np.genfromtxt, np.savetxt)
- OpenCV, NumPy: Rotate and flip image
- NumPy: Set whether to print full or truncated ndarray
- NumPy: Calculate cumulative sum and product (np.cumsum, np.cumprod)
- Free Python 3 Tutorial
- Control Flow
- Exception Handling
- Python Programs
- Python Projects
- Python Interview Questions
- Python Database
- Data Science With Python
- Machine Learning with Python
- Python Tutorial | Learn Python Programming
- Python Data Types
Python Arrays
- Python String
- Python Numbers
- Boolean data type in Python
- Dictionaries in Python
- Python Lists
- Python Tuples
- Python Sets
For simplicity, we can think of an array a fleet of stairs where on each step is placed a value (let’s say one of your friends). Here, you can identify the location of any of your friends by simply knowing the count of the steps they are on. The array can be handled in Python by a module named array. They can be useful when we have to manipulate only specific data type values. A user can treat lists as arrays. However, the user cannot constrain the type of elements stored in a list. If you create arrays using the array module, all elements of the array must be of the same type.
What is an Array in Python?
An array is a collection of items stored at contiguous memory locations. The idea is to store multiple items of the same type together. This makes it easier to calculate the position of each element by simply adding an offset to a base value, i.e., the memory location of the first element of the array (generally denoted by the name of the array).
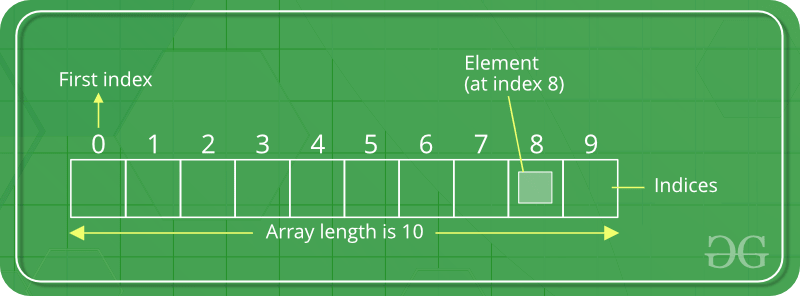
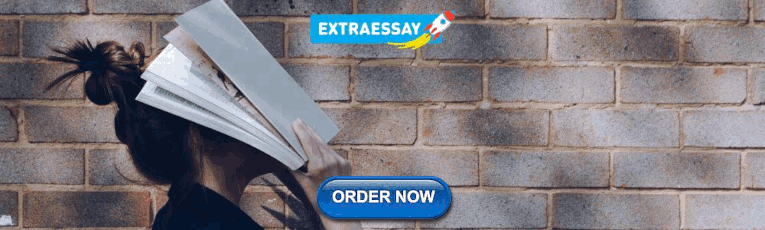
Creating an Array
Array in Python can be created by importing an array module. array( data_type , value_list ) is used to create an array with data type and value list specified in its arguments.
This code creates two arrays: one of integers and one of doubles . It then prints the contents of each array to the console.
Complexities for Creation of Arrays:
Time Complexity: O(1) Auxiliary Space: O(n)
Some of the data types are mentioned below which will help in creating an array of different data types.
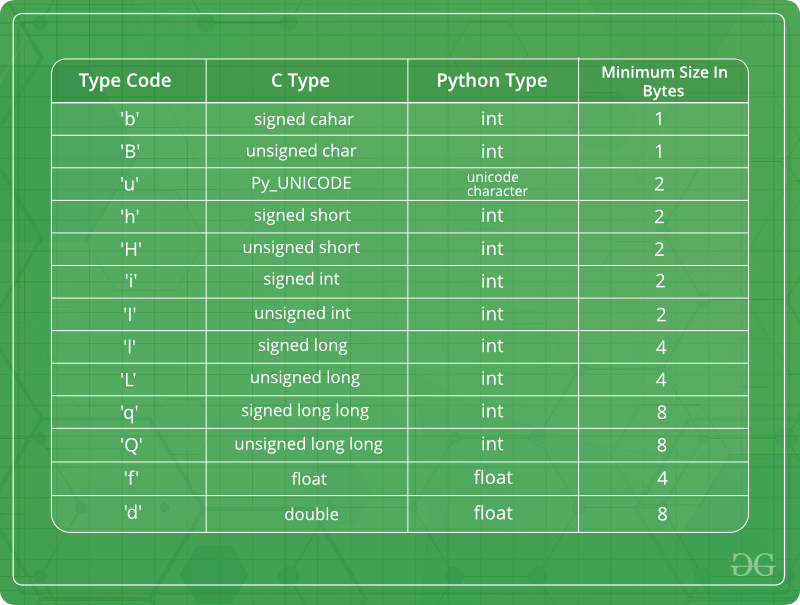
Adding Elements to a Array
Elements can be added to the Array by using built-in insert() function. Insert is used to insert one or more data elements into an array. Based on the requirement, a new element can be added at the beginning, end, or any given index of array. append() is also used to add the value mentioned in its arguments at the end of the array.
The code first imports the `array` module as `arr` . Then, it creates an array of integers named `a` with elements `[1, 2, 3]` . The array is printed before and after inserting the integer `4` at index 1. Similarly, an array of doubles named `b` with elements `[2.5, 3.2, 3.3]` is created and printed before and after appending the double ` 4.4` to the array.
Complexities for Adding elements to the Arrays
Time Complexity: O(1)/O(n) ( O(1) – for inserting elements at the end of the array, O(n) – for inserting elements at the beginning of the array and to the full array Auxiliary Space: O(1)
Accessing Elements from the Array
In order to access the array items refer to the index number. Use the index operator [ ] to access an item in a array. The index must be an integer.
This code demonstrates the use of indexing to access elements in arrays. The a[0] expression accesses the first element of the array a , which is 1. The a[3] expression accesses the fourth element of the array a , which is 4. Similarly, the b[1] expression accesses the second element of the array b , which is 3.2, and the b[2] expression accesses the third element of the array b , which is 3.3.
Output :
Complexities for accessing elements in the Arrays
Time Complexity: O(1) Auxiliary Space: O(1)
Removing Elements from the Array
Elements can be removed from the array by using built-in remove() function but an Error arises if element doesn’t exist in the set. Remove() method only removes one element at a time, to remove range of elements, iterator is used. pop() function can also be used to remove and return an element from the array, but by default it removes only the last element of the array, to remove element from a specific position of the array, index of the element is passed as an argument to the pop() method. Note – Remove method in List will only remove the first occurrence of the searched element.
This code demonstrates how to create, print, remove elements from, and access elements of an array in Python. It imports the array module, which is used to work with arrays. It creates an array of integers and prints the original array. It then removes an element from the array and prints the modified array. Finally, it removes all occurrences of a specific element from the array and prints the updated array
Complexities for Removing elements in the Arrays
Time Complexity: O(1)/O(n) ( O(1) – for removing elements at the end of the array, O(n) – for removing elements at the beginning of the array and to the full array Auxiliary Space: O(1)
Slicing of an Array
In Python array, there are multiple ways to print the whole array with all the elements, but to print a specific range of elements from the array, we use Slice operation . Slice operation is performed on array with the use of colon(:). To print elements from beginning to a range use [:Index], to print elements from end use [:-Index], to print elements from specific Index till the end use [Index:], to print elements within a range, use [Start Index:End Index] and to print whole List with the use of slicing operation, use [:]. Further, to print whole array in reverse order, use [::-1].
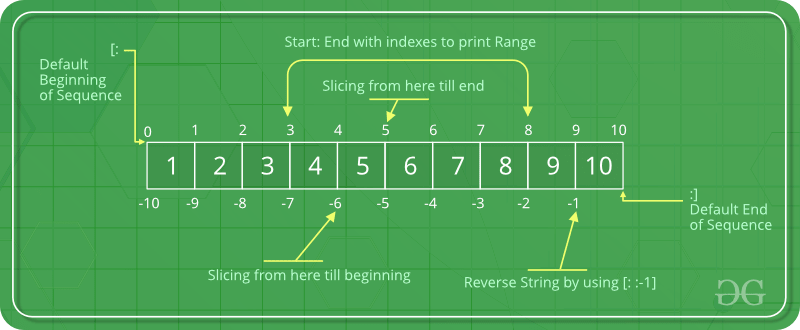
This code employs slicing to extract elements or subarrays from an array. It starts with an initial array of integers and creates an array from the list. The code slices the array to extract elements from index 3 to 8, from index 5 to the end, and the entire array. The sliced arrays are then printed to demonstrate the slicing operations.
Searching Element in an Array
In order to search an element in the array we use a python in-built index() method. This function returns the index of the first occurrence of value mentioned in arguments.
Example: The code demonstrates how to create an array in Python, print its elements, and find the indices of specific elements. It imports the array module, creates an array of integers, prints the array using a for loop, and then uses the index() method to find the indices of the first occurrences of the integers 2 and 1.
Complexities for searching elements in the Arrays
Time Complexity: O(n) Auxiliary Space: O(1)
Updating Elements in a Array
In order to update an element in the array we simply reassign a new value to the desired index we want to update.
Example: This code illustrates the functionality of modifying elements within an array using indexing. It imports the array module, creates an array of integers, and prints the initial array. Then, it modifies two elements of the array at specific indexes and prints the updated array. This serves to demonstrate how indexing allows for dynamic manipulation of array contents.
Complexities for updating elements in the Arrays
Different operations on python arrays, counting elements in a array.
In order to count elements in an array we need to use count method.
Example: The code demonstrates how to determine the frequency of a particular element within an array. It imports the array module, creates an array of integers, counts the occurrences of the number 2 using the count() method, and finally prints the result. This code snippet effectively showcases the ability to analyze the distribution of elements in arrays.
Complexities for counting elements in the Arrays
Reversing elements in a array.
In order to reverse elements of an array we need to simply use reverse method.
Example: The presented code demonstrates the functionality of reversing the order of elements within an array using the reverse() method. It imports the array module, creates an array of integers, prints the original array, reverses the order of elements using reverse() , and then prints the reversed array. This effectively illustrates the ability to modify the arrangement of elements in an array.
Complexities for reversing elements in the Arrays:
Time Complexity: O(n) Auxiiary Space: O(1)
Extend Element from Array
In the article,we will cover the python list extend() and try to understand the Python list extend().
What is extend element from array?
In Python, an array is used to store multiple values or elements of the same datatype in a single variable. The extend() function is simply used to attach an item from iterable to the end of the array. In simpler terms, this method is used to add an array of values to the end of a given or existing array.
Syntax of list extend()
The syntax of the extend() method:
Here,all the element of iterable are added to the end of list1
The provided code demonstrates the capability of extending an array to include additional elements. It imports the array module using an alias, creates an array of integers, prints the array before extension, extends the array using the extend() method, and finally prints the extended array. This concisely illustrates the ability to add elements to an existing array structure
The provided code demonstrates the capacity to extend arrays with various data types, including integers and floats. It utilizes the array module, creates arrays of both data types, and extends them using the extend() method. The arrays are then printed before and after extension to illustrate the changes. This effectively showcases the ability to append elements to arrays of different data representations.
Complexities for extend element from array:
Time Complexity : O(1) Auxiliary Space: O(1)
More Information Resource Related to Python Array:
- Array in Python | Set 1 (Introduction and Functions)
- Array in Python | Set 2 (Important Functions) an
Please Login to comment...
- Python-array
- How to Delete Whatsapp Business Account?
- Discord vs Zoom: Select The Efficienct One for Virtual Meetings?
- Otter AI vs Dragon Speech Recognition: Which is the best AI Transcription Tool?
- Google Messages To Let You Send Multiple Photos
- 30 OOPs Interview Questions and Answers (2024)
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
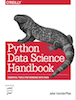
The text is released under the CC-BY-NC-ND license , and code is released under the MIT license . If you find this content useful, please consider supporting the work by buying the book !
The Basics of NumPy Arrays
< Understanding Data Types in Python | Contents | Computation on NumPy Arrays: Universal Functions >
Data manipulation in Python is nearly synonymous with NumPy array manipulation: even newer tools like Pandas ( Chapter 3 ) are built around the NumPy array. This section will present several examples of using NumPy array manipulation to access data and subarrays, and to split, reshape, and join the arrays. While the types of operations shown here may seem a bit dry and pedantic, they comprise the building blocks of many other examples used throughout the book. Get to know them well!
We'll cover a few categories of basic array manipulations here:
- Attributes of arrays : Determining the size, shape, memory consumption, and data types of arrays
- Indexing of arrays : Getting and setting the value of individual array elements
- Slicing of arrays : Getting and setting smaller subarrays within a larger array
- Reshaping of arrays : Changing the shape of a given array
- Joining and splitting of arrays : Combining multiple arrays into one, and splitting one array into many
NumPy Array Attributes ¶
First let's discuss some useful array attributes. We'll start by defining three random arrays, a one-dimensional, two-dimensional, and three-dimensional array. We'll use NumPy's random number generator, which we will seed with a set value in order to ensure that the same random arrays are generated each time this code is run:
Each array has attributes ndim (the number of dimensions), shape (the size of each dimension), and size (the total size of the array):
Another useful attribute is the dtype , the data type of the array (which we discussed previously in Understanding Data Types in Python ):
Other attributes include itemsize , which lists the size (in bytes) of each array element, and nbytes , which lists the total size (in bytes) of the array:
In general, we expect that nbytes is equal to itemsize times size .
Array Indexing: Accessing Single Elements ¶
If you are familiar with Python's standard list indexing, indexing in NumPy will feel quite familiar. In a one-dimensional array, the $i^{th}$ value (counting from zero) can be accessed by specifying the desired index in square brackets, just as with Python lists:
To index from the end of the array, you can use negative indices:
In a multi-dimensional array, items can be accessed using a comma-separated tuple of indices:
Values can also be modified using any of the above index notation:
Keep in mind that, unlike Python lists, NumPy arrays have a fixed type. This means, for example, that if you attempt to insert a floating-point value to an integer array, the value will be silently truncated. Don't be caught unaware by this behavior!
Array Slicing: Accessing Subarrays ¶
Just as we can use square brackets to access individual array elements, we can also use them to access subarrays with the slice notation, marked by the colon ( : ) character. The NumPy slicing syntax follows that of the standard Python list; to access a slice of an array x , use this:
If any of these are unspecified, they default to the values start=0 , stop= size of dimension , step=1 . We'll take a look at accessing sub-arrays in one dimension and in multiple dimensions.
One-dimensional subarrays ¶
A potentially confusing case is when the step value is negative. In this case, the defaults for start and stop are swapped. This becomes a convenient way to reverse an array:
Multi-dimensional subarrays ¶
Multi-dimensional slices work in the same way, with multiple slices separated by commas. For example:
Finally, subarray dimensions can even be reversed together:
Accessing array rows and columns ¶
One commonly needed routine is accessing of single rows or columns of an array. This can be done by combining indexing and slicing, using an empty slice marked by a single colon ( : ):
In the case of row access, the empty slice can be omitted for a more compact syntax:
Subarrays as no-copy views ¶
One important–and extremely useful–thing to know about array slices is that they return views rather than copies of the array data. This is one area in which NumPy array slicing differs from Python list slicing: in lists, slices will be copies. Consider our two-dimensional array from before:
Let's extract a $2 \times 2$ subarray from this:
Now if we modify this subarray, we'll see that the original array is changed! Observe:
This default behavior is actually quite useful: it means that when we work with large datasets, we can access and process pieces of these datasets without the need to copy the underlying data buffer.
Creating copies of arrays ¶
Despite the nice features of array views, it is sometimes useful to instead explicitly copy the data within an array or a subarray. This can be most easily done with the copy() method:
If we now modify this subarray, the original array is not touched:
Reshaping of Arrays ¶
Another useful type of operation is reshaping of arrays. The most flexible way of doing this is with the reshape method. For example, if you want to put the numbers 1 through 9 in a $3 \times 3$ grid, you can do the following:
Note that for this to work, the size of the initial array must match the size of the reshaped array. Where possible, the reshape method will use a no-copy view of the initial array, but with non-contiguous memory buffers this is not always the case.
Another common reshaping pattern is the conversion of a one-dimensional array into a two-dimensional row or column matrix. This can be done with the reshape method, or more easily done by making use of the newaxis keyword within a slice operation:
We will see this type of transformation often throughout the remainder of the book.
Array Concatenation and Splitting ¶
All of the preceding routines worked on single arrays. It's also possible to combine multiple arrays into one, and to conversely split a single array into multiple arrays. We'll take a look at those operations here.
Concatenation of arrays ¶
Concatenation, or joining of two arrays in NumPy, is primarily accomplished using the routines np.concatenate , np.vstack , and np.hstack . np.concatenate takes a tuple or list of arrays as its first argument, as we can see here:
You can also concatenate more than two arrays at once:
It can also be used for two-dimensional arrays:
For working with arrays of mixed dimensions, it can be clearer to use the np.vstack (vertical stack) and np.hstack (horizontal stack) functions:
Similary, np.dstack will stack arrays along the third axis.
Splitting of arrays ¶
The opposite of concatenation is splitting, which is implemented by the functions np.split , np.hsplit , and np.vsplit . For each of these, we can pass a list of indices giving the split points:
Notice that N split-points, leads to N + 1 subarrays. The related functions np.hsplit and np.vsplit are similar:
Similarly, np.dsplit will split arrays along the third axis.
- Skip to main content
- Skip to secondary menu
- Skip to primary sidebar
- Skip to secondary sidebar
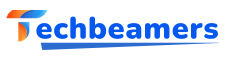
TechBeamers
Tutorials for Beginners to Learn Python, Java, Selenium, C Programming, CSharp, SQL, Linux, Agile, and Software Testing
Python Arrays Explained With Examples
Oct 29, 2023 by Meenakshi Agarwal Leave a Comment
Python arrays are homogenous data structures. They are used to store multiple items but allow only the same type of data. They are available in Python by importing the array module.
Python Arrays – A Beginners Guide
List, a built-in type in Python, is also capable of storing multiple values. But they are different from arrays because they are not bound to any specific type.
So, to summarize, arrays are not fundamental types, but lists are internal to Python. An array accepts values of one kind while lists are independent of the data type. In order to know more about lists, check out our tutorial on Python List .
However, in this tutorial, you’ll get to know how to create an array, add/update, index, remove, and slice.
What is Array in Python?
An array is a container used to contain a fixed number of items. But, there is an exception that values should be of the same type. The following are two terms often used with arrays.
- Array element – Every value in an array represents an element.
- Array index – Every element has some position in the array known as the index.
Let’s now see how Python represents an array.
Array Illustration
The array is made up of multiple parts. And each section of the array is an element. We can access all the values by specifying the corresponding integer index.
The first element starts at index 0 and so on. At the 9th index, the 10th item would appear. Check the below graphical illustration.
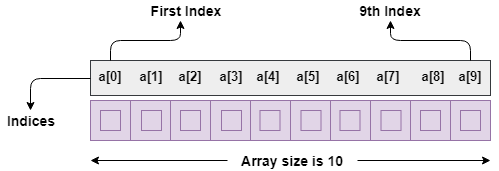
Declare Array in Python
You have first to import the array module in your Python script. After that, declare the array variable as per the below syntax.
In the above statements, “array_var” is the name of the array variable. And we’ve used the array() function which takes two parameters. “TypeCode” is the type of array whereas “Initializers” are the values to set in the array.
The argument “TypeCode” can be any value from the below chart.
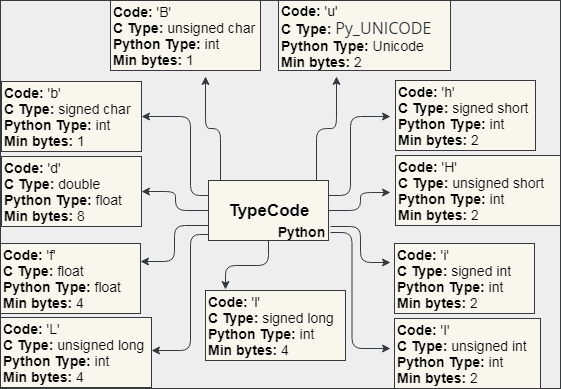
In the above diagram, we’ve listed down all possible type codes for Python and C Types. But we’ll only be using the Python Types “i” for integers and “d” for floats here in our examples.
Also, note that there is one Unicode type shown in the chart. Its support ended with Python version 3.3. So, it is best not to use it in your programs.
Let’s consider a simple case to create an array of 10 integers.
We first imported the array module and then used the Python range() function to produce ten integers. We’ve also printed the numbers that our array variable would hold. Here is the outcome of the above program.
In the next sections, we’ll cover all actions that can be performed using arrays.
Array Operations
Indexing an array.
We can use indices to retrieve elements of an array. See the below example:
Arrays have their first element stored at the zeroth index. Also, you can see that if we use the -ve index, then it gives us elements from the tail end.
The output is:
Slicing arrays
The slice operator “:” is commonly used to slice strings and lists . However, it does work for the arrays also. Let’s see with the help of examples.
When you execute the above script, it produces the following output:
The following two points, you should note down:
- When you pass both the left and right operands to the slice operator, then they act as the indexes.
- If you take one of them whether the left or right one, then it represents the no. of elements.
Add/Update an array
We can make changes to an array in different ways. Some of these are as follows:
- Assignment operator to change or update an array
- Append() method to add one element
- Extend() method to add multiple items
We’ll now understand each of these approaches with the help of examples.
Let’s begin by using the assignment operator to update an existing array variable.
Now, we’ll apply the append() and extend() methods on a given array. These work the same for lists in Python. See the tutorial below.
Difference Between List Append() and Extend()
This program yields the following:
The point to note is that both append() and extend() add elements to the end. The next tip is an interesting one. We can join two or more arrays using the “+” operator. If interested, learn about the different operators available in Python .
The above script shows the following result after execution:
Remove array elements
There are multiple ways that we can follow to remove elements from an array. Here are these:
- Python del operator
- Remove() method
- Pop() method
Let’s first check how Python del works to delete array members.
The output is as follows:
Now, let’s try to utilize the remove() and pop() methods. The former removes the given value from the array whereas the latter deletes the item at a specified index.
After running this code, we get the below result:
Reverse array
Last but not least is how we can reverse the elements of an array in Python. There can be many approaches to this. However, we’ll take the following two:
- Slice operator in Python
- Python List comprehension
Check out the below sample code to invert the element in a given array.
The above code produces the following output after running:
Now, we are mentioning a bonus method to reverse the array using the reversed() call. This function inverts the elements and returns a “list_reverseiterator” type object. However, if you want more info, read our in-depth tutorial on 7 ways to reverse a list in Python .
Here is the output of the above example.
We hope that after wrapping up this tutorial, you should feel comfortable using Python arrays. However, you may practice more with examples to gain confidence.
Also, to learn Python from scratch to depth, do read our step-by-step Python tutorial .
Reader Interactions
Leave a reply.
Your email address will not be published. Required fields are marked *
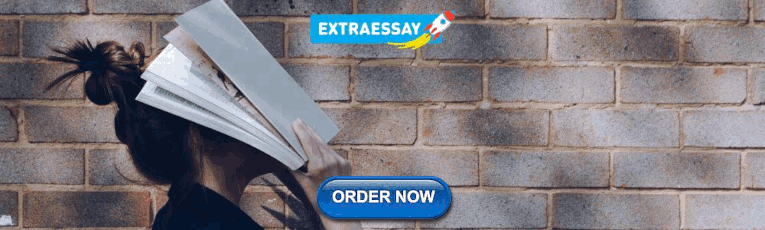
IMAGES
VIDEO
COMMENTS
with each iteration it assign the first to char to uuidVal (ex: 52, 22, 87) and the last two char to distVal (ex: 60, 90, 81) Note: using .append concatenate the values, for example if used with distArray like distArray.append (distVal) the values will be like this [60, 6090, 609081] @michaelpri Nope, they have values.
temp = z[:] This will create a shallow copy -- mutations to elements in the list will show up in the elements in z, but not changes to temp directly. For more general purposes, python has a copy module that you can use: temp = copy.copy(z) Or, possibly: temp = copy.deepcopy(z) answered Oct 10, 2013 at 15:43. mgilson.
Here, variable represents a generic Python variable, while expression represents any Python object that you can provide as a concrete value—also known as a literal—or an expression that evaluates to a value. To execute an assignment statement like the above, Python runs the following steps: Evaluate the right-hand expression to produce a concrete value or object.
Python Variables Variable Names Assign Multiple Values Output Variables Global Variables Variable Exercises. ... however, to work with arrays in Python you will have to import a library, like the NumPy library. Arrays are used to store multiple values in one single variable: ... The solution is an array! An array can hold many values under a ...
In this article, you'll learn how to use Python arrays. You'll see how to define them and the different methods commonly used for performing operations on them. ... You can change the value of a specific element by speficying its position and assigning it a new value: import array as arr #original array numbers = arr.array('i',[10,20,30]) # ...
Element Assignment in NumPy Arrays. We can assign new values to an element of a NumPy array using the = operator, just like regular python lists. A few examples are below (note that this is all one code block, which means that the element assignments are carried forward from step to step).
Keep Learning. In this tutorial, you'll dive deep into working with numeric arrays in Python, an efficient tool for handling binary data. Along the way, you'll explore low-level data types exposed by the array module, emulate custom types, and even pass a Python array to C for high-performance processing.
This module defines an object type which can compactly represent an array of basic values: characters, integers, floating point numbers. Arrays are sequence types and behave very much like lists, except that the type of objects stored in them is constrained. The type is specified at object creation time by using a type code, which is a single ...
Unpack a tuple and list in Python; You can also swap the values of multiple variables in the same way. See the following article for details: Swap values in a list or values of variables in Python; Assign the same value to multiple variables. You can assign the same value to multiple variables by using = consecutively.
While the array module offers a more memory-efficient way to store basic data types, it's essential to remember its limitations.Unlike lists, arrays are homogeneous.This means all elements in the array must be of the same type. Also, you can only store basic C-style data types in arrays. If you need to store custom objects or other Python types, you'll need to use a list or another data structure.
To define an array in Python, you could use the np.array function to convert a list ... You can reassign a value of an array by using array indexing and the assignment operator. ... Create a variable y that contains all the elements of x that are strictly bigger than 3. Assign all the values of x that are bigger than 3, the value 0. y = x [x ...
The alternative is to store all these values in an array. Arrays are useful for storing and manipulating multiple values of the same data type. They act like a variable that can hold a collection of values, all of which are the same type. These values are stored together in bordering memory. Python Array Methods
In this tutorial, you'll learn about Python array module, the difference between arrays and lists, and how and when to use them with the help of examples. Courses Tutorials Examples . ... To create an array of numeric values, we need to import the array module. For example: import array as arr a = arr.array('d', [1.1, 3.5, 4.5]) print(a) Output.
This article explains how to get and set values, such as individual elements or subarrays (e.g., rows or columns), in a NumPy array ( ndarray) using various indexing. See the following articles for information on deleting, concatenating, and adding to ndarray. The NumPy version used in this article is as follows.
Array in Python can be created by importing an array module. array (data_type, value_list) is used to create an array with data type and value list specified in its arguments. Unmute. This code creates two arrays: one of integers and one of doubles. It then prints the contents of each array to the console. Python3.
In this method, we use the array () function from the array module to create an array in Python. In Python, you can declare arrays using the Python Array Module, Python List as an Array, or Python NumPy Array. The Python Array Module and NumPy Array offer more efficient memory usage and specific data types, while Python lists provide ...
The NumPy slicing syntax follows that of the standard Python list; to access a slice of an array x, use this: x[start:stop:step] If any of these are unspecified, they default to the values start=0, stop= size of dimension, step=1 . We'll take a look at accessing sub-arrays in one dimension and in multiple dimensions.
Here's the syntax to create an array in Python: import array as arr numbers = arr.array(typecode, [values]) As the array data type is not built into Python by default, you have to import it from the array module. We import this module as arr. Using the array method of arr, we can create an array by specifying a typecode (data type of the values ...
$\begingroup$ Have a look at the description/hove of tag python: Programming questions are off-topic here. (There are "no" arrays in Python: you show list s. Roughly, sequence * int means sequence repeated int times - while the result with immutable s is unsurprising, with object s it means referring to the same object in multiple places , with ...
What is Array in Python? An array is a container used to contain a fixed number of items. But, there is an exception that values should be of the same type. The following are two terms often used with arrays. Array element - Every value in an array represents an element. Array index - Every element has some position in the array known as ...
Let's say I have the following empty two dimensional array in Python: q = [[None]*5]*4 I want to assign a value of 5 to the first row in the first column of q. Instinctively, I do the following: q[0][0] = 5 However, this produces: