A continuous assignment drives a value into a net.
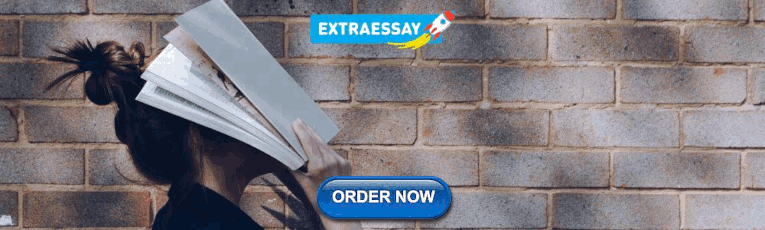
Description:
Continuous assignments model combinational logic. Each time the expression changes on the right-hand side, the right-hand side is re-evaluated, and the result is assigned to the net on the left-hand side.
The implicit continuous assignment combines the net declaration (see Net data type) and continuous assignment into one statement. The explicit assignment require two statements: one to declare the net (see Net data type), and one to continuously assign a value to it.
Continuous assignments are not the same as procedural continuous assignments. Continuous assignments are declared outside of procedural blocks. They automatically become active at time zero, and are evaluated concurrently with procedural blocks, module instances, and primitive instances.
Net data type , Procedural continuous assignment
Using Continuous Assignment to Model Combinational Logic in Verilog
In this post, we talk about continuous assignment in verilog using the assign keyword. We then look at how we can model basic logic gates and multiplexors in verilog using continuous assignment.
There are two main classes of digital circuit which we can model in verilog – combinational and sequential .
Combinational logic is the simplest of the two, consisting solely of basic logic gates, such as ANDs, ORs and NOTs. When the circuit input changes, the output changes almost immediately (there is a small delay as signals propagate through the circuit).
In contrast, sequential circuits use a clock and require storage elements such as flip flops . As a result, output changes are synchronized to the circuit clock and are not immediate.
In this post, we talk about the techniques we can use to design combinational logic circuits in verilog. In the next post, we will discuss the techniques we use to model basic sequential circuits .
Continuous Assignment in Verilog
We use continuous assignment to drive data onto verilog net types in our designs. As a result of this, we often use continuous assignment to model combinational logic circuits.
We can actually use two different methods to implement continuous assignment in verilog.
The first of these is known as explicit continuous assignment. This is the most commonly used method for continuous assignment in verilog.
In addition, we can also use implicit continuous assignment, or net declaration assignment as it is also known. This method is less common but it can allow us to write less code.
Let's look at both of these techniques in more detail.
- Explicit Continuous Assignment
We normally use the assign keyword when we want to use continuous assignment in verilog. This approach is known as explicit continuous assignment.
The verilog code below shows the general syntax for continuous assignment using the assign keyword.
The <variable> field in the code above is the name of the signal which we are assigning data to. We can only use continuous assignment to assign data to net type variables.
The <value> field can be a fixed value or we can create an expression using the verilog operators we discussed in a previous post. We can use either variable or net types in this expression.
When we use continuous assignment, the <variable> value changes whenever one of the signals in the <value> field changes state.
The code snippet below shows the most basic example of continuous assignment in verilog. In this case, whenever the b signal changes states, the value of a is updated so that it is equal to b.
- Net Declaration Assignment
We can also use implicit continuous assignment in our verilog designs. This approach is also commonly known as net declaration assignment in verilog.
When we use net declaration assignment, we place a continuous assignment in the statement which declares our signal. This can allow us to reduce the amount of code we have to write.
To use net declaration assignment in verilog, we use the = symbol to assign a value to a signal when we declare it.
The code snippet below shows the general syntax we use for net declaration assignment.
The variable and value fields have the same function for both explicit continuous assignment and net declaration assignment.
As an example, the verilog code below shows how we would use net declaration assignment to assign the value of b to signal a.
Modelling Combinational Logic Circuits in Verilog
We use continuous assignment and the verilog operators to model basic combinational logic circuits in verilog.
To show we would do this, let's look at the very basic example of a three input and gate as shown below.
To model this circuit in verilog, we use the assign keyword to drive the data on to the and_out output. This means that the and_out signal must be declared as a net type variable, such as a wire.
We can then use the bit wise and operator (&) to model the behavior of the and gate.
The code snippet below shows how we would model this three input and gate in verilog.
This example shows how simple it is to design basic combinational logic circuits in verilog. If we need to change the functionality of the logic gate, we can simply use a different verilog bit wise operator .
If we need to build a more complex combinational logic circuit, it is also possible for us to use a mixture of different bit wise operators.
To demonstrate this, let's consider the basic circuit shown below as an example.
To model this circuit in verilog, we need to use a mixture of the bit wise and (&) and or (|) operators. The code snippet below shows how we would implement this circuit in verilog.
Again, this code is relatively straight forward to understand as it makes use of the verilog bit wise operators which we discussed in the last post.
However, we need to make sure that we use brackets to model more complex logic circuit. Not only does this ensure that the circuit operates properly, it also makes our code easier to read and maintain.
Modelling Multiplexors in Verilog
Multiplexors are another component which are commonly used in combinational logic circuits.
In verilog, there are a number of ways we can model these components.
One of these methods uses a construct known as an always block . We normally use this construct to model sequential logic circuits, which is the topic of the next post in this series. Therefore, we will look at this approach in more detail the next blog post.
In the rest of this post, we will look at the other methods we can use to model multiplexors.
- Verilog Conditional Operator
As we talked about in a previous blog, there is a conditional operator in verilog . This functions in the same way as the conditional operator in the C programming language.
To use the conditional operator, we write a logical expression before the ? operator which is then evaluated to see if it is true or false.
The output is assigned to one of two values depending on whether the expression is true or false.
The verilog code below shows the general syntax which the conditional operator uses.
From this example, it is clear how we can create a basic two to one multiplexor using this operator.
However, let's look at the example of a simple 2 to 1 multiplexor as shown in the circuit diagram below.
The code snippet below shows how we would use the conditional operator to model this multiplexor in verilog.
- Nested Conditional Operators
Although this is not common, we can also write code to build larger multiplexors by nesting conditional operators.
To show how this is done, let's consider a basic 4 to 1 multiplexor as shown in the circuit below.
To model this in verilog using the conditional operator, we treat the multiplexor circuit as if it were a pair of two input multiplexors.
This means one multiplexor will select between inputs A and B whilst the other selects between C and D. Both of these multiplexors use the LSB of the address signal as the address pin.
To create the full four input multiplexor, we would then need another multiplexor.
This takes the outputs from the first two multiplexors and uses the MSB of the address signal to select between them.
The code snippet below shows the simplest way to do this. This code uses the signals mux1 and mux2 which we defined in the last example.
However, we could easily remove the mux1 and mux2 signals from this code and instead use nested conditional operators.
This reduces the amount of code that we would have to write without affecting the functionality.
The code snippet below shows how we would do this.
As we can see from this example, when we use conditional operators to model multiplexors in verilog, the code can quickly become difficult to understand. Therefore, we should only use this method to model small multiplexors.
- Arrays as Multiplexors
It is also possible for us to use verilog arrays to build simple multiplexors.
To do this we combine all of the multiplexor inputs into a single array type and use the address to point at an element in the array.
To get a better idea of how this works in practise, let's consider a basic four to one multiplexor as an example.
The first thing we must do is combine our input signals into an array. There are two ways in which we can do this.
Firstly, we can declare an array and then assign all of the individual bits, as shown in the verilog code below.
Alternatively we can use the verilog concatenation operator , which allows us to assign the entire array in one line of code.
To do this, we use a pair of curly braces - { } - and list the elements we wish to include in the array inside of them.
When we use the concatenation operator we can also declare and assign the variable in one statement, as long as we use a net type.
The verilog code below shows how we can use the concatenation operator to populate an array.
As verilog is a loosely typed language , we can use the two bit addr signal as if it were an integer type. This signal then acts as a pointer that determines which of the four elements to select.
The code snippet below demonstrates this method in practise. As the mux output is a wire, we must use continuous assignment in this instance.
What is the difference between implicit and explicit continuous assignment?
When we use implicit continuous assignment we assign the variable a value when we declare. When we use explicit continuous assignment we use the assign keyword to assign a value.
Write the code for a 2 to 1 multiplexor using any of the methods discussed we discussed.
Write the code for circuit below using both implicit and explicit continuous assignment.
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
Table of Contents
Sign up free for exclusive content.
Don't Miss Out
We are about to launch exclusive video content. Sign up to hear about it first.
VHDL Concurrent Conditional Assignment
The Conditional Signal Assignment statement is concurrent because it is assigned in the concurrent section of the architecture. It is possible to implement the same code in a sequential version, as we will see next.
The conditional signal assignment statement is a process that assigns values to a signal.
It is a concurrent statement; this means that you must use it only in concurrent code sections.
The statement that performs the same operation in a sequential environment is the “ if ” statement.
The syntax for a conditional signal assignment statement is:
This is a simple example of a two-way mux as reported here:
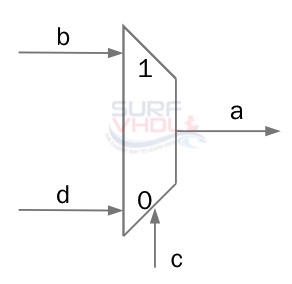
The output “ a ” is equal to “ b ” when the selector “ c ” is “1” else is equal to “ d ”
Concurrent Conditional Signal Assignment Example 1
This example extends the previous one. This is a 4-way mux, implemented as concurrent code.
The architecture declarative section is empty. As you can notice, we don’t care about how the mux is implemented.
In this moment we don’t’ talk about logic gate, and or nand ect, we are describing the behavior of circuit using a high level description.
A graphical representation can be this one.
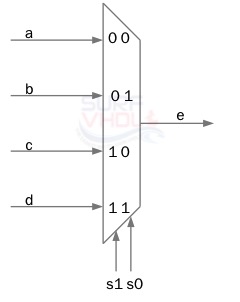
It is up to the synthesizer to implement the best architecture on the selected technology in terms of logic gates. In fact if you are using FPGA the synthesizer will use LUT to map the VHDL functions, if you are implementing an ASIC the synthesized logic will depend on differ technology and will be implemented using, for instance, NAND, OR, NOR gate, depending on the technology.
Running the RTL compiler on Altera Quartus II , this is the output of the RTL viewer, if we try to layout this mux4 .
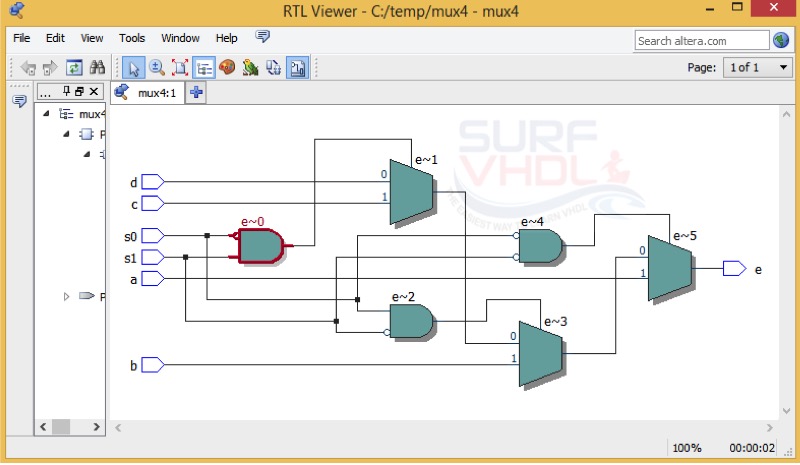
As clear, the RTL translation is implemented in terms of AND gate and 2-way mux. The output “ e ” is generated by cascading 3 two-way mux.
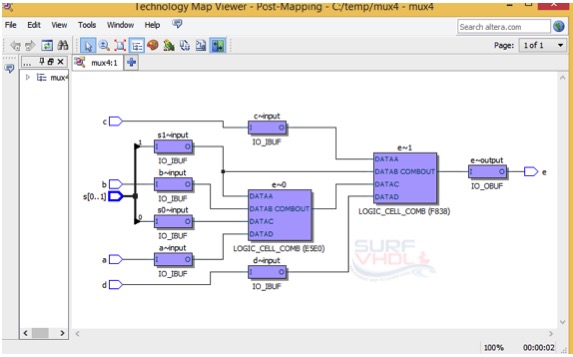
This is the output of the Altera MAP viewer selecting Cyclone IV FPGA technology. Our mux4 is implemented using LOGIC_COMB_CELL Look Up Table present in the Cyclone IV FPGA . This example should clarify the meaning of “technology dependent”.
Concurrent Conditional Signal Assignment Example 2
This example is the same 4-way mux as the previous one, in which we used a different syntax to implement the selector. In this case, we have introduced the statement “with select”.
In the architecture declarative section, we declared a signal “ sel ” of type integer used to address the mux. The signal “ sel ” is coded as binary to integer.
The statement “ with select ” allows compacting the syntax of the mux code. Note the introduction of the “ other ” keyword. It is necessary because the mux assignment cover only 3 of the 2^32 possible integer values. If we try to layout the code, it is interesting to see how RTL viewer interprets the VHDL code:
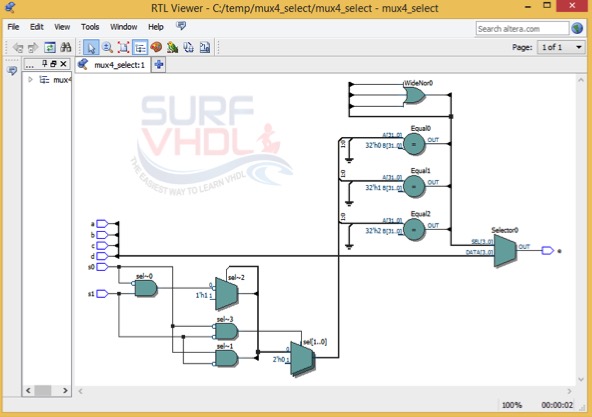
This case is different from the previous one. We can notice that the VHDL relative to the signal sel is decoded in order to implement mux selection and that the output mux is implemented as 4-way mux. So the RTL view of the RTL code is totally different from the previous one.
The FPGA used in this example is the same as the previous example, in fact the output of Altera MAP viewer have the same implementation of the previous RTL code as clear if we make a comparison between the two implementations.
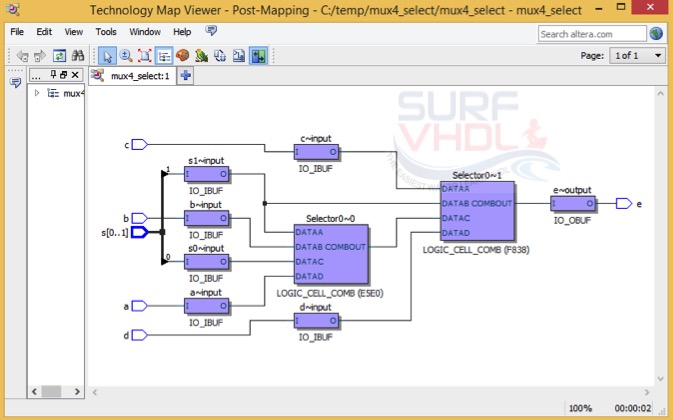
These two examples should clarify the meaning of behavioral. We have seen two different implementations of a simple mux mapped on the same hardware :
implementation of different RTL code can generate the same hardware logic.
Of course, gaining the sensibility to write good VHDL/RTL code is only a matter of time and experience . If you will follow the course, you will find good advices in order to gain all the shortcuts useful reduce this amount of time.
Previous – Next
The same designs make using SystemVerilog VHDL and Verilog
Every design contains top module + 3 modules that have the same functionality but written on SystemVerilog, VHDL, and Verilog:
- VHDL top Entity that contains 3 component
- SystemVerilog component
- Verilog component
- VHDL component
The simulation was written on SystemVerilog. Some simulation projects contain external stimulus files and files with expected simulation results.
In additionals presents
- RTL schematics
- Synthesis schematics
- Implementation schematics
- Simulations waveforms
All projects are available in https://github.com/SystemVerilog-VHDL-Verilog/VHDL_SV_Verilog
The projects made in Vivado v2019.1.1 (64-bit)
The unexpected results of RTL, Synthesis, Implementation, or Simulations marked by Orange color .
VHDL (Part 2)
- First Online: 20 March 2019
Cite this chapter
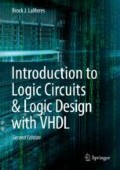
- Brock J. LaMeres 2
120k Accesses
In Chap. 5 VHDL was presented as a way to describe the behavior of concurrent systems. The modeling techniques presented were appropriate for combinational logic because these types of circuits have outputs dependent only on the current values of their inputs. This means a model that continuously performs signal assignments provides an accurate model of this circuit behavior. In Chap. 7 sequential logic storage devices were presented that did not continuously update their outputs based on the instantaneous values of their inputs. Instead, sequential storage devices only update their outputs based upon an event, most often the edge of a clock signal. The modeling techniques presented in Chap. 5 are unable to accurately describe this type of behavior. In this chapter we describe the VHDL constructs to model signal assignments that are triggered by an event in order to accurately model sequential logic. We can then use these techniques to describe more complex sequential logic circuits such as finite state machines and register transfer level systems. This chapter will also present how to create test benches and look at commonly used packages that increase the capability and accuracy with which VHDL can model modern systems. The goal of this chapter is to give an understanding of the full capability of hardware description languages.
This is a preview of subscription content, log in via an institution to check access.
Access this chapter
- Available as PDF
- Read on any device
- Instant download
- Own it forever
- Available as EPUB and PDF
- Compact, lightweight edition
- Dispatched in 3 to 5 business days
- Free shipping worldwide - see info
Tax calculation will be finalised at checkout
Purchases are for personal use only
Institutional subscriptions
Author information
Authors and affiliations.
Department of Electrical & Computer Engineering, Montana State University, Bozeman, MT, USA
Brock J. LaMeres
You can also search for this author in PubMed Google Scholar
Rights and permissions
Reprints and permissions
Copyright information
© 2019 Springer Nature Switzerland AG
About this chapter
LaMeres, B.J. (2019). VHDL (Part 2). In: Introduction to Logic Circuits & Logic Design with VHDL . Springer, Cham. https://doi.org/10.1007/978-3-030-12489-2_8
Download citation
DOI : https://doi.org/10.1007/978-3-030-12489-2_8
Published : 20 March 2019
Publisher Name : Springer, Cham
Print ISBN : 978-3-030-12488-5
Online ISBN : 978-3-030-12489-2
eBook Packages : Engineering Engineering (R0)
Share this chapter
Anyone you share the following link with will be able to read this content:
Sorry, a shareable link is not currently available for this article.
Provided by the Springer Nature SharedIt content-sharing initiative
- Publish with us
Policies and ethics
- Find a journal
- Track your research
Verilog vs VHDL: A Comprehensive Comparison
19 Sep, 2023
Fig 1 - Printed Circuit Board Chips and Radio Components
Unveiling the Duel of Digital Design - A Comprehensive Exploration of History, Syntax, and Applications of the two popular hardware description languages
Introduction.
Verilog and VHDL are two primary hardware description languages (HDLs) engineers and designers use to model, simulate, and synthesize digital systems. These languages are crucial in developing integrated circuits (ICs), field-programmable gate arrays (FPGAs), and other digital hardware.
While both languages serve similar purposes, they have distinct syntax, design methodologies, and features that can influence the choice between them for a specific project. This article aims to provide a comprehensive Verilog and VHDL comparison, delving into their history, syntax, design methodologies, features, tools, industry adoption, and applications.
Understanding the differences and similarities between Verilog vs VHDL will allow designers to pick the right tools for their projects and gain insights into the intricacies of digital hardware design. Hence, these languages form the base for optimized FPGA design for customizable digital logic designs.
Further Reading: FPGA Design: A Comprehensive Guide to Mastering Field-Programmable Gate Arrays (wevolver.com)
What are Hardware Description Languages?
Hardware Description Language (HDL) describes a digital circuit's behavioral or structural orientation. These languages can simulate digital circuits, allowing designers to analyze circuit responses before fabrication. Moreover, both Verilog and VHDL are used to formulate digital circuits to produce ASIC or FPGA-based systems .
For instance, consider the following example, where an HDL code produces a simplistic digital circuit design.
The trend for HDLs started in the 1980s as digital design companies explored the possibilities of languages that could shape digital circuits. While several HDLs like Java HDL (JHDL), Cypress SemiConductor Corporation's Active HHDL, etc., Verilog and VHDL have been widely accepted and endorsed by IEEE (Institute of Electrical and Electronics Engineers) standards.
Historical Development of the Two Languages
History of verilog.
Verilog was initially developed in the late 1980s by Gateway Design Automation as a proprietary language for simulating and verifying digital circuits. It was one of the first hardware description languages developed from 1983 to 1984. The language quickly gained popularity due to its simplicity and ease of use.
In 1990, Cadence Design Systems acquired Gateway Design Automation and subsequently released the Verilog language as an open standard. This decision spurred the growth of the Verilog user community and the development of third-party tools for simulation and synthesis.
The first official standard for Verilog, known as IEEE Standard 1364-1995, was published in 1995. This standard introduced several enhancements to the language, including support for behavioral modeling and testbenches. In 2001, the IEEE released an updated standard, IEEE 1364-2001, which expanded the language's capabilities by adding constructs for representing complex digital systems and improving support for verification.
In 2005, the IEEE merged the Verilog standard with SystemVerilog, a Verilog superset incorporating advanced features for system-level modeling, verification, and design reuse. The combined standard, IEEE 1800, has since become the de facto standard for digital design using Verilog. Today, Verilog is widely used in the semiconductor industry for designing application-specific integrated circuits (ASICs) , FPGAs, and other digital systems.
History of VHDL
VHDL, which stands for VHSIC (Very High-Speed Integrated Circuit) Hardware Description Language, was developed in the early 1980s by the U.S. Department of Defense (DoD) as part of the VHSIC program. The program's primary goal was to create a standardized language for the design and verification of digital systems, particularly for military applications. VHDL was designed to be a powerful, flexible, and portable language that could accurately represent complex digital systems at various levels of abstraction.
In 1987, the Institute of Electrical and Electronics Engineers (IEEE) adopted VHDL as an official standard, IEEE 1076. This standardization helped promote VHDL's widespread adoption in both military and commercial sectors. Over the years, the language has undergone several revisions to expand its capabilities and address the evolving needs of the digital design community. Notable revisions include IEEE 1076-1993, which introduced support for synthesis and testbenches, and IEEE 1076-2008, which added improved modeling and verification features.
VHDL has become a popular choice for digital design in various industries, including aerospace, defense, telecommunications, and consumer electronics. Its strong typing, modularity, and support for concurrent design make it well-suited for large-scale projects and safety-critical applications. Today, VHDL is widely used for designing ASICs, FPGAs, and other digital systems, with a robust ecosystem of simulation, synthesis, and verification tools available to support the design process.
Language Syntax and Structure
Verilog syntax.
Verilog's syntax is based on the C programming language, making it familiar and accessible for those with a background in C or C-like languages. The basic building blocks of Verilog are modules, which represent individual components of a digital system. A module can contain inputs, outputs, internal signals, and instances of other modules, allowing for hierarchical design.
Data Types in Verilog
In Verilog, data types include wire, reg, integer, and real. Wire data types represent physical connections between components, while reg data types store values in registers. The integer and real data types are used for arithmetic operations and modeling.
Verilog supports various operators, such as arithmetic, relational, logical, and bitwise operators. These operators enable designers to perform calculations, comparisons, and manipulations of data within the language.
A key feature of Verilog syntax is the use of procedural and continuous assignments. Procedural assignments are used within "always" blocks, which describe the behavior of a digital system in response to changes in input signals or internal states. Continuous assignments, on the other hand, are used to model combinational logic and define the relationship between input and output signals.
Here's an example of a simple Verilog module that implements a 2-input AND gate:
In this example, the module named "and_gate" has two input wires, "a" and "b," and one output wire, "y." The continuous assignment statement "assign y = a & b;" defines the relationship between the input and output signals, implementing the AND gate functionality.
VHDL Syntax
The Ada programming language inspires VHDL syntax and is more verbose than Verilog. The primary building blocks of VHDL are entities and architectures, which define the behavior and structure of a digital system. An entity describes the interface of a component, including its input and output ports, while an architecture defines the internal behavior and interconnections of the component.
VHDL Data Types
VHDL has a rich set of data types, including bit, bit_vector, integer, real, and enumerated types. The bit and bit_vector data types represent binary values and arrays of binary values, respectively. Integer and real data types are used for arithmetic operations and modeling purposes, while enumerated types allow designers to define custom data types with a finite set of values.
VHDL supports various arithmetic, relational, logical, and bitwise operators. These operators enable designers to perform calculations, comparisons, and data manipulations within the language.
In VHDL, concurrent and sequential statements are used to describe the behavior of digital systems. Concurrent statements, such as signal assignments and component instantiations, describe the relationships between signals and components that co-occur. Sequential statements, on the other hand, are used within process blocks to describe the behavior of a digital system in a sequential manner, similar to traditional programming languages.
Here's an example of a simple VHDL entity and architecture that implements a 2-input AND gate:
In this example, the entity "and_gate" defines the interface with two input ports, "a" and "b," and one output port, "y." The architecture "behavior" describes the internal behavior of the AND gate using a concurrent signal assignment statement "y <= a and b;", which defines the relationship between the input and output signals.
Digital Design Methodologies
Design methodology refers to designing, implementing, and verifying digital electronic systems. It encompasses a set of principles, practices, and techniques engineers follow to ensure that the final digital circuit meets its intended functionality, performance, and reliability requirements. Some of the critical aspects and steps commonly involved in a digital circuit design methodology include:
Specification and Requirements Analysis - understanding the requirements and specifications of the digital circuit.
High-Level Design - Create a high-level or architectural design that defines the overall structure and functionality of the digital system.
Functional Verification - Verification that the high-level design meets the specified requirements. It involves simulations and modeling to ensure that the system functions as intended.
RTL (Register-Transfer Level) Design - Creating a detailed RTL design using a hardware description language (HDL) like Verilog or VHDL. Further Reading: RTL Design: A Comprehensive Guide to Unlocking the Power of Register-Transfer Level Design (wevolver.com)
Synthesis - Using synthesis tools to convert the RTL description into a gate-level netlist.
Gate-Level Simulation - Simulating gate-level netlist to verify that it meets functional and timing requirements after synthesis.
Physical Design - Performing physical design tasks, including floor planning, placement, and routing, to generate a physical representation of the circuit. This step is crucial for manufacturing the chip.
Timing Analysis - Analyzing the circuit for timing violations and ensuring the design meets the specified timing constraints.
Functional Verification (again) - Conducting thorough verification tests to ensure that the synthesized and physically designed circuit behaves correctly.
Design for Testability (DFT) - Implementing testability features to facilitate manufacturing testing, such as built-in self-test (BIST) or scan chains.
Manufacturing - Sending the final design to a fabrication facility (foundry) for chip manufacturing.
Testing - Testing manufactured chips to identify any defects or manufacturing errors.
Debugging and Validation - Debugging any issues that arise during testing and validating that the chips meet the original specifications.
Documentation - Maintaining comprehensive documentation throughout the design process, including design specifications, test plans, and design reviews.
Post-Silicon Debug - If the design is implemented in an FPGA or ASIC, post-silicon validation may be necessary to ensure the design works correctly in the physical device.
Iteration and Optimization - Iterating on the design as needed to address any issues, optimizing performance, or make improvements based on feedback or changing requirements.
Verilog Design Methodology
Verilog design methodology revolves around the concept of modules and hierarchy. Modules are the fundamental building blocks of a digital system, encapsulating functionality and interconnections. A module can instantiate other modules, creating a hierarchical design that promotes modularity, reusability, and ease of understanding.
Designers typically start by creating a top-level module representing the entire digital system. This module instantiates and interconnects lower-level modules, which may instantiate even lower-level modules.
This hierarchical approach allows designers to break down complex systems into manageable, reusable components.
Testbenches in Verilog
Testbenches play a crucial role in the Verilog design methodology. A testbench is a separate module that instantiates the design under test (DUT) and applies input stimuli to verify its functionality. Testbenches can be written using behavioral Verilog, which allows designers to describe the desired behavior of the system without specifying its implementation details. This abstraction enables efficient verification of the design's functionality and performance under various conditions.
Simulation and synthesis tools are essential components of the Verilog design process. Simulation tools, such as ModelSim and VCS, enable designers to execute and debug their Verilog code, providing insights into the behavior of the digital system under different scenarios.
Synthesis tools, such as Synopsys Design Compiler and Xilinx Vivado, convert the Verilog code into a gate-level netlist or an FPGA bitstream, which can then be used for implementation on an ASIC or FPGA, respectively. These tools also perform optimizations to meet design constraints, such as timing, area, and power requirements.
VHDL Design Methodology
The VHDL design methodology focuses on entities, architectures, and hierarchy. Entities define the interfaces of components, while architectures describe their internal behavior and interconnections. Like Verilog, VHDL promotes a hierarchical design approach, allowing designers to break down complex systems into smaller, reusable components.
In a typical VHDL design process, designers create a top-level entity representing the entire digital system. This entity instantiates and interconnects lower-level entities, which may further instantiate even lower-level entities. This hierarchical structure enables modularity and reusability, making managing and understanding complex designs easier.
Testbenches in VHDL
Testbenches are an integral part of the VHDL design methodology. A testbench is a separate entity that instantiates the design under test (DUT) and applies input stimuli to verify its functionality. Testbenches can be written using behavioral VHDL, which allows designers to describe the desired behavior of the system without specifying its implementation details. This abstraction efficiently verifies the design's functionality and performance under various conditions.
Essential Language Features
Verilog features.
Verilog offers several vital features enabling designers to effectively model and verify digital systems. Some of the most notable features include.
Procedural Assignments
Procedural assignments are used within 'always' blocks to describe the behavior of a digital system in response to changes in input signals or internal states. These assignments allow designers to model sequential logic, such as flip-flops and state machines, using familiar programming constructs like if-else statements and loops.
Continuous Assignments
Continuous assignments are used to model combinational logic and define the relationship between input and output signals. These assignments are evaluated continuously, ensuring that the output signals are always up-to-date concerning their input signals.
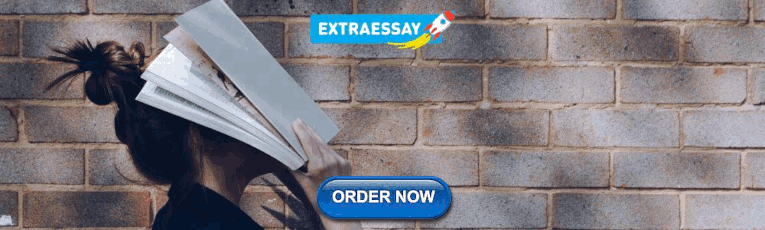
Gate-level Modeling
Verilog supports gate-level modeling, which allows designers to describe digital systems using primitive gates, such as AND, OR, and NOT gates. This feature enables designers to model and simulate digital systems at a low level of abstraction, providing insights into the underlying hardware implementation.
User-defined Primitives (UDPs)
Verilog allows designers to create user-defined primitives, custom gate-level components that can be instantiated and used in a design. UDPs enable designers to model complex or specialized logic elements that may not be available as built-in primitives.
Behavioral Modeling
Verilog supports behavioral modeling, which allows designers to describe the desired behavior of a digital system without specifying its implementation details. This abstraction enables efficient verification of the design's functionality and performance under various conditions.
For example, consider a simple 4-bit counter implemented in Verilog:
In this example, the counter uses procedural assignments within an always block to model the sequential behavior of the counter. The count signal is updated on the rising edge of the clock, and the counter is reset when the reset signal is asserted. This demonstrates the use of Verilog features such as procedural assignments, behavioral modeling, and data types like reg and wire.
VHDL Features
VHDL offers a rich set of features that enable designers to model, simulate, and verify digital systems effectively. Some of the most notable features include:
Process Statements
Process statements are used to describe the behavior of a digital system in a sequential manner, similar to traditional programming languages. These statements are executed within a process block, which is sensitive to specific signals or events. Process statements allow designers to model sequential logic, such as flip-flops and state machines, using familiar programming constructs like if-else statements and loops.
Concurrent Statements
Concurrent statements, such as signal assignments and component instantiations, describe the relationships between signals and components that occur simultaneously. These statements enable designers to model combinational logic and define the interconnections between components in a digital system.
Component Instantiation
VHDL supports component instantiation, which allows designers to create reusable components and instantiate them within a higher-level architecture. This feature promotes modularity and reusability, making it easier to manage and understand complex designs.
Generics and Configurations
Generics and configurations are powerful VHDL features that enable designers to create parameterized components and customize their behavior or structure. Generics allow designers to define parameters for a component, while configurations enable designers to specify the mapping between entities and architectures, as well as the values of generics for specific instances.
Packages and Libraries
VHDL supports packages and libraries, which allow designers to organize and reuse code across multiple projects. Packages can contain declarations of data types, subprograms, and components, while libraries are used to store and manage packages and design units.
For example, consider a simple 4-bit counter implemented in VHDL:
In this example, the counter uses a process statement to model the sequential behavior of the counter. The count_internal signal is updated on the rising edge of the clock, and the counter is reset when the reset signal is asserted. This demonstrates the use of VHDL features such as process statements, concurrent statements, and data types like std_logic and unsigned.
Simulation and Synthesis Tools
When it comes to design and synthesis tools, most of the popular applications provides support for both VHDL and Verilog. Some of the most widely used tools include:
ModelSim is a high-performance digital simulation tool developed by Mentor Graphics (now part of Siemens EDA). It supports both Verilog and VHDL languages and offers advanced debugging features, such as waveform viewing, source code stepping, and breakpoint setting.
There are advanced debugging features, such as waveform viewing, source code stepping, and breakpoint setting. It is widely used in the industry for functional verification and timing analysis of digital designs.
VCS (Verilog Compiled Simulator)
VCS, developed by Synopsys, is a high-performance Verilog simulator that provides fast simulation and advanced debugging capabilities.
VCS supports both Verilog and SystemVerilog languages and offers features like waveform viewing, source code stepping, and breakpoint setting. It is widely used for functional verification and performance analysis of digital designs.
Synopsys Design Compiler
Design Compiler is a synthesis tool developed by Synopsys that translates Verilog code into a gate-level netlist for ASIC implementation.
It performs optimizations for timing, area, and power, ensuring that the synthesized design meets the specified constraints. Design Compiler is widely used in the industry for ASIC synthesis and optimization.
GHDL is an open-source VHDL simulator that provides a fast and efficient way to simulate and verify VHDL designs. It supports the IEEE 1076 standard and offers basic debugging capabilities, such as waveform viewing and source code stepping.
It is a popular choice for designers looking for a free and open-source alternative to commercial VHDL simulators.
Xilinx Vivado
Vivado is a comprehensive design suite developed by Xilinx for FPGA design, synthesis, and implementation. It supports both Verilog and VHDL languages and offers advanced features like high-level synthesis, IP integration, and design optimization. Vivado is widely used for FPGA design and implementation, targeting Xilinx FPGA devices.
Industry Adoption and Applications
Verilog adoption.
Verilog has been widely adopted in various industries due to its simplicity, ease of use, and compatibility with the C programming language. Some of the key industries and applications where Verilog is commonly used include:
Semiconductor Industry
Verilog is extensively used in the design and verification of application-specific integrated circuits (ASICs) and field-programmable gate arrays (FPGAs). Its syntax and features make it suitable for modeling complex digital systems at different levels of abstraction, from gate-level to behavioral-level descriptions.
Telecommunications
In the telecommunications industry, Verilog is used to design and verify digital signal processing (DSP) components, such as filters, modulators, and demodulators. These components are critical for the efficient transmission and reception of data in modern communication systems.
Consumer Electronics
Verilog is employed in the design of various consumer electronic devices, such as smartphones, tablets, and gaming consoles. It is used to develop custom ICs and FPGAs that provide the necessary processing power, graphics capabilities, and connectivity features for these devices.
Automotive Industry
With the increasing demand for advanced driver assistance systems (ADAS) and autonomous vehicles, Verilog has found applications in the automotive industry. It is used to design and verify digital systems responsible for sensor processing, decision-making, and control in modern vehicles.
One notable example of a successful project that utilized Verilog is the design of the RISC-V open-source processor architecture. RISC-V was developed at the University of California, Berkeley, and has gained significant attention due to its flexibility, modularity, and open-source nature. Verilog was used to describe the RTL (register-transfer level) design of the RISC-V processor, enabling its implementation on various ASIC and FPGA platforms.
Another notable application of Verilog HDL comes in the form of a lane changing prediction system that provides assistance to the driver for changing lanes on a straight road. The system uses FPGA Zybo 700 to analyze driver performance and predict directions using eye and face detection.
VHDL Adoption
VHDL has been widely adopted in various industries due to its strong typing, modularity, and support for concurrent design. Some of the key industries and applications where VHDL is commonly used include:
Aerospace and Defense
VHDL is extensively used in the aerospace and defense industries for the design and verification of safety-critical digital systems, such as avionics, radar, and missile guidance systems. Its strong typing and formal semantics make it well-suited for large-scale projects with stringent reliability and safety requirements.
As FPGA designs have profound applications in unmanned systems too, VHDL becomes a primary component in the design of FPGA-based aircrafts and unmanned vehicles for enhancing their security especially for long-term deployments.
Machine Learning and AI Acceleration
FPGAs are constantly being adopted in machine learning and AI as they are capable of parallel processing. Hence, specially designed libraries allow engineers to deploy accelerated AI models with unmatchable performance and efficiency, enabling AI powered products and services for regular consumers.
Similar to Verilog, VHDL is used in the telecommunications industry to design and verify digital signal processing (DSP) components, such as filters, modulators, and demodulators. These components play a crucial role in the efficient transmission and reception of data in modern communication systems.
VHDL is employed in the design of various consumer electronic devices, such as digital cameras, set-top boxes, and home automation systems. It is used to develop custom ICs and FPGAs that provide the necessary processing power, graphics capabilities, and connectivity features for these devices.
Industrial Automation
In the industrial automation sector, VHDL is used to design and verify digital systems responsible for process control, monitoring, and data acquisition. These systems are critical for ensuring the efficient operation and safety of industrial processes and equipment.
One notable example of a successful project that uses VHDL is the design of the LEON processor, an open-source, fault-tolerant, and radiation-hardened processor based on the SPARC architecture.
Developed by the European Space Agency (ESA), the LEON processor has been used in various space missions, such as the Mars Express and the BepiColombo mission to Mercury. VHDL was used to describe the RTL (register-transfer level) design of the LEON processor, enabling its implementation on various ASIC and FPGA platforms with the required fault-tolerance and radiation-hardening features.
Choosing Between Verilog and VHDL
When deciding between Verilog and VHDL for a project, several factors should be considered to ensure the most suitable language is chosen. Some of the key factors include:
Design Complexity
Both Verilog and VHDL can handle complex digital designs, but their syntax and features may influence the choice depending on the specific requirements of the project. Verilog's C-like syntax and simpler constructs may be more suitable for smaller projects or designers with a background in C programming. On the other hand, VHDL's strong typing and support for concurrent design make it well-suited for large-scale projects and safety-critical applications.
Industry and application
The choice between Verilog and VHDL may be influenced by the specific industry or application domain. Verilog is widely used in the semiconductor industry, while VHDL is more prevalent in the aerospace and defense sectors.
Also, the familiarity with the language commonly used in a particular industry can be beneficial for designers seeking employment or collaboration opportunities.
Tool support
The availability and compatibility of simulation and synthesis tools can also influence the choice between Verilog and VHDL. While most modern tools support both languages, some tools may offer better performance or features for one language over the other. Team expertise
The expertise and experience of the design team can play a significant role in the choice between Verilog and VHDL. If the team is already familiar with one language, it may be more efficient to continue using that language to leverage existing knowledge and skills.
Design Reuse and IP integration
The availability of reusable design components or intellectual property (IP) cores can also influence the choice between Verilog and VHDL. Hence, designers must consider the availability of IP cores and libraries in their target language and choose the language that offers the best resources for their specific project requirements.
The choice between Verilog and VHDL depends on various factors, such as design complexity, industry, tool support, team expertise, and design reuse. By carefully considering these factors, designers can select the most appropriate language for their projects and ensure a smooth and efficient design process.
This article provides a comprehensive comparison of Verilog and VHDL, two prominent hardware description languages used in digital system design. We have explored their history, syntax, design methodologies, features, simulation and synthesis tools, and industry adoption.
By understanding the differences and similarities between these languages, designers can make informed decisions when choosing the right language for their projects and gain insights into the intricacies of digital hardware design.
Frequently Asked Questions (FAQs)
1. can verilog and vhdl be used together in a single project.
Yes, it is possible to use both Verilog and VHDL in a single project by employing mixed-language simulation and synthesis tools. These tools can handle designs written in both languages, allowing designers to leverage the strengths of each language and reuse existing IP cores or libraries.
2. Which language is easier to learn, Verilog or VHDL?
The ease of learning Verilog or VHDL depends on the individual's background and preferences. Verilog's syntax is similar to C, making it easier for those with a background in C or C-like languages. VHDL, on the other hand, has a more verbose syntax and strong typing, which some designers may find more structured and easier to understand.
3. Are there any open-source tools available for Verilog and VHDL?
Yes, there are several open-source tools available for both Verilog and VHDL, such as Icarus Verilog and GHDL for simulation, and Yosys for synthesis. These tools provide an alternative to commercial tools and can be used for learning, experimentation, or even production designs.
4. How do I choose between an ASIC and an FPGA for my digital design project?
The choice between an ASIC and an FPGA depends on factors such as design complexity, performance requirements, production volume, and cost. ASICs offer higher performance and lower power consumption but have higher upfront costs and longer development times. FPGAs provide more flexibility and shorter development times but may have lower performance and higher power consumption compared to ASICs.
5. What are some popular FPGA vendors that support Verilog and VHDL?
Some popular FPGA vendors that support both Verilog and VHDL include Xilinx, Intel (formerly Altera), Microchip (formerly Microsemi), and Lattice Semiconductor. These vendors provide design tools, IP cores, and libraries that support both languages, enabling designers to choose the language that best suits their needs.
The History of Verilog - HardwareBee
Hardware Description Languages - Introduction to Digital Systems: Modeling, Synthesis, and Simulation Using VHDL [Book] (oreilly.com)
Manish Vishnoi, Karan Pathak; Satyam Kumar; Sushain Bhat, (2017), “FPGA based real-time implementation of driver assistance system” https://ieeexplore.ieee.org/document/8342564/keywords#keywords
FPGA Design | Emerging Methodologies & Tools (vlsifirst.com)
ESA - LEON: the space chip that Europe built
Search for articles and topics on Wevolver
More by muhammad sufyan.
Meet Sufyan - an accomplished Electronics Engineer and dedicated Educator who teaches at Mohammad Ali Jinnah University in Karachi, Pakistan. With a Master's degree in Electrical Engineering, Sufyan specializes in teaching courses on Microcontrollers, Digital Electronics, and Control Systems. He has ...
Pick and Place Robots: An In-Depth Guide to Their Functionality and Applica...
15 minutes read
Rigid Flex PCB: Revolutionizing Modern Electronics Design
13 minutes read
Tapeout in Semiconductor Manufacturing: An In-depth Exploration
12 minutes read
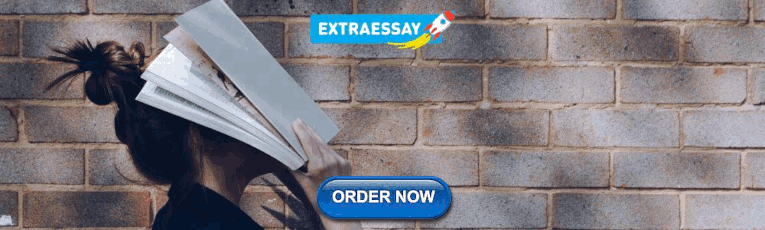
IMAGES
VIDEO
COMMENTS
In you not working example x_delayed (0) <= x; is aquvalent to. process(x) begin. x_delayed(0) <= x; end process; So the process will assign x_delayed (0) only when x changes. Because this is a signal asignment the x_delayed (0) will not change immediatly, it will change after a delta cycle.
Conditional Signal Assignment or the "When/Else" Statement. The "when/else" statement is another way to describe the concurrent signal assignments similar to those in Examples 1 and 2. Since the syntax of this type of signal assignment is quite descriptive, let's first see the VHDL code of a one-bit 4-to-1 multiplexer using the ...
Description: Continuous assignments model combinational logic. Each time the expression changes on the right-hand side, the right-hand side is re-evaluated, and the result is assigned to the net on the left-hand side. The implicit continuous assignment combines the net declaration (see Net data type) and continuous assignment into one statement.
The VHDL code shown below uses one of the logical operators to implement this basic circuit. and_out <= a and b; Although this code is simple, there are a couple of important concepts to consider. The first of these is the VHDL assignment operator (<=) which must be used for all signals.
A bus is a collection of wires related in some way by function or clock domain. Examples would be an address bus or data bus. In VHDL we refer to busses as a vector. For example: --8-bit bus consisting of 8 wires carrying signals of -- type std_logic --all these wires may be referred to by the name big_bus. SIGNAL big_bus : STD_LOGIC_VECTOR(7 ...
Signal assignment Concurrent VHDL Statements 6.111 Lecture # 6 . inb WHEN OTHERS; inb WHEN '1', outc <= ina WHEN '0', WITH inc SELECT Select conditions must be mutually exclusive and exhaustive With-Select-When (Note: always use OTHERS as there are values other than '0' and '1') Or:
Do not make assignments to the same signal in more than one always statement or continuous assignment statement. VHDL. 1. Use process(clk) and nonblocking assignments to model synchronous sequential logic. process(clk) begin if rising_edge(clk) then n1 <= d; -- nonblocking q <= n1; -- nonblocking end if; end process; 2.
In this post, we talk about continuous assignment in verilog using the assign keyword. We then look at how we can model basic logic gates and multiplexors in verilog using continuous assignment.. There are two main classes of digital circuit which we can model in verilog - combinational and sequential. Combinational logic is the simplest of the two, consisting solely of basic logic gates ...
rent signal assignments, and component instantiations (described in Laboratory No. 8). This laboratory work presents the format and use of sequential and concurrent statements. As examples some basic combinational and sequential circuits are described, such as multiplexers, decoders, flip-flops, regis-ters, and counters. 6.1. Sequential Statements
A continuous assignment in Verilog is a statement that executes every time the right hand side of the an assignment changes. This is wholly equivalent to the concurrent signal assignment in VHDL with inertial delays. ... i.e it does not matter what order you make the assignments in a procedural dataflow. VHDL assignments are inherently non ...
The conditional signal assignment statement is a process that assigns values to a signal. It is a concurrent statement; this means that you must use it only in concurrent code sections. The statement that performs the same operation in a sequential environment is the " if " statement. The syntax for a conditional signal assignment statement is:
This chapter contains sections titled: Combinational versus sequential circuits Simple signal assignment statement Conditional signal assignment statement Selected signal assignment statement...
Continuous Assignments. A continuous assignment in Verilog is a statement that executes every time the right hand side of the an assignment changes. This is wholly equivalent to the concurrent signal assignment in VHDL with inertial delays. Phone: 540-953-3390 | Email: Sales Office.
1. The Inside_process and Outside_process versions behave differently. If both designs work, it is mostly out of luck, because in this case Out_signal simply lags half a clock cycle when declared inside the process. Out_signal is assigned when the process triggers, which in this case occurs on rising and falling edges of clk.
The same designs make using SystemVerilog VHDL and Verilog. Every design contains top module + 3 modules that have the same functionality but written on SystemVerilog, VHDL, and Verilog: ... Verilog continuous assignment statements (assign) and VHDL concurrent assignment statements (<=) are reevaluated any time any of the inputs on the right ...
assignments allow a process to model register transfer level behavior where a signal can be used as both the operand of an assignment and the destination of a different assignment within the same process. VHDL provides two techniques to trigger a process, the sensitivity list and the wait statement. 8.1.1 Sensitivity List
• P. Chu, RTL Hardware Design using VHDL Chapter 4, Concurrent Signal Assignment Statements of VHDL Chapter 6.3, Realization of VHDL Data Types. 3 Components and interconnects structural VHDL Descriptions dataflow Concurrent statements behavioral (sequential)
The simplest solution is to change the datatype of burst_mode to integer with range 0 to 1, and then use some math: library ieee; use ieee.std_logic_1164.all; use ieee.numeric_std.all; entity bridge is. generic (. burst_mode :integer range 0 to 1 := 0. ); end entity;
The continuous assignment statement "assign y = a & b;" defines the relationship between the input and output signals, implementing the AND gate functionality. VHDL Syntax The Ada programming language inspires VHDL syntax and is more verbose than Verilog.
And using a different tool yields: ghdl -a async.vhdl. async.vhdl:32:5: a generate statement must have a label. async.vhdl:32:22: 'generate' is expected instead of 'loop'. In a place appropriate for a concurrent statement in an architecture body the only statement that can have a for keyword is a generate statement, which requires a label.