Home » Top 3 Practical Ways to Perform Javascript String Concatenation
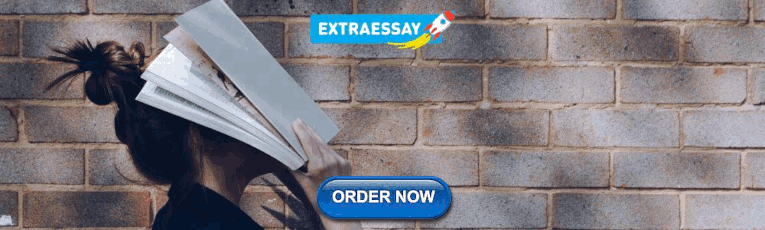
Top 3 Practical Ways to Perform Javascript String Concatenation
Summary : in this tutorial, you’ll learn how to concatenate strings in JavaScript.
JavaScript provides various options that allow you to concatenate two or more strings:
- Use the concat() method
- Use the + and += operators
- Use the template literals
1) Using the concat() method
The String.prototype.concat() concatenates two or more string arguments and returns a new string:
The concat() accepts a varied number of string arguments and returns a new string containing the combined string arguments.
If you pass non-string arguments into the concat() , it will convert these arguments to a string before concatenating. For example:
This example concatenates strings into a new string:
The following example concatenates all string elements in an array into a string:
2) Using the + and += operators
The operator + allows you to concatenate two strings. For example:
To compose a string piece by piece, you use the += operator:
According to a performance test on some modern web browsers, the + and += operators perform faster than the concat() method.
3) Using template literals
ES6 introduces the template literals that allow you to perform string interpolation.
The following example shows how to concatenate three strings:
In this tutorial, you have learned how to concatenate strings in JavaScript using the concat() method, + and += operator, and template literals.
3 Ways to Concatenate Strings in JavaScript
There are 3 ways to concatenate strings in JavaScript. In this tutorial, you'll the different ways and the tradeoffs between them.
The + Operator
The same + operator you use for adding two numbers can be used to concatenate two strings.
You can also use += , where a += b is a shorthand for a = a + b .
If the left hand side of the + operator is a string, JavaScript will coerce the right hand side to a string. That means it is safe to concatenate objects, numbers, null , and undefined .
The + and += operators are fast on modern JavaScript engines , so no need to worry about something like Java's StringBuilder class .
Array#join()
The Array#join() function creates a new string from concatenating all elements in an array. For example:
The first parameter to join() is called the separator . By default, the separator is a single comma ',' .
You can pass in any separator you want. Separators make Array#join() the preferred choice for concatenating strings if you find yourself repeating the same character over and over again. For example, you can use ' ' as the separator to join an array of words:
Or you can use '/' to join together URL fragments:
Separators make Array#join() a very flexible way to concatenate strings. If you want to join together a variable number of strings, you should generally use join() rather than a for loop with + .
String#concat()
JavaScript strings have a built-in concat() method . The concat() function takes one or more parameters, and returns the modified string. Strings in JavaScript are immutable, so concat() doesn't modify the string in place.
The downside of using concat() is that you must be certain str1 is a string. You can pass non-string parameters to concat() , but you will get a TypeError if str == null .
The concat() function is rarely used because it has more error cases than the + operator. For example, you would get unexpected behavior if you call concat() on a value that happens to be an array . You should use + instead of concat() unless you have a very good reason.
If you must use concat() , it is usually best to call it on an empty string:
More Fundamentals Tutorials
- Check if a Date is Valid in JavaScript
- Encode base64 in JavaScript
- Check if URL Contains a String
- JavaScript Add Month to Date
- JavaScript Add Days to Date
- 3 Patterns to Merge Arrays in JavaScript
- Convert a BigInt to a Number in JavaScript
How to Join/Concatenate Strings in JavaScript
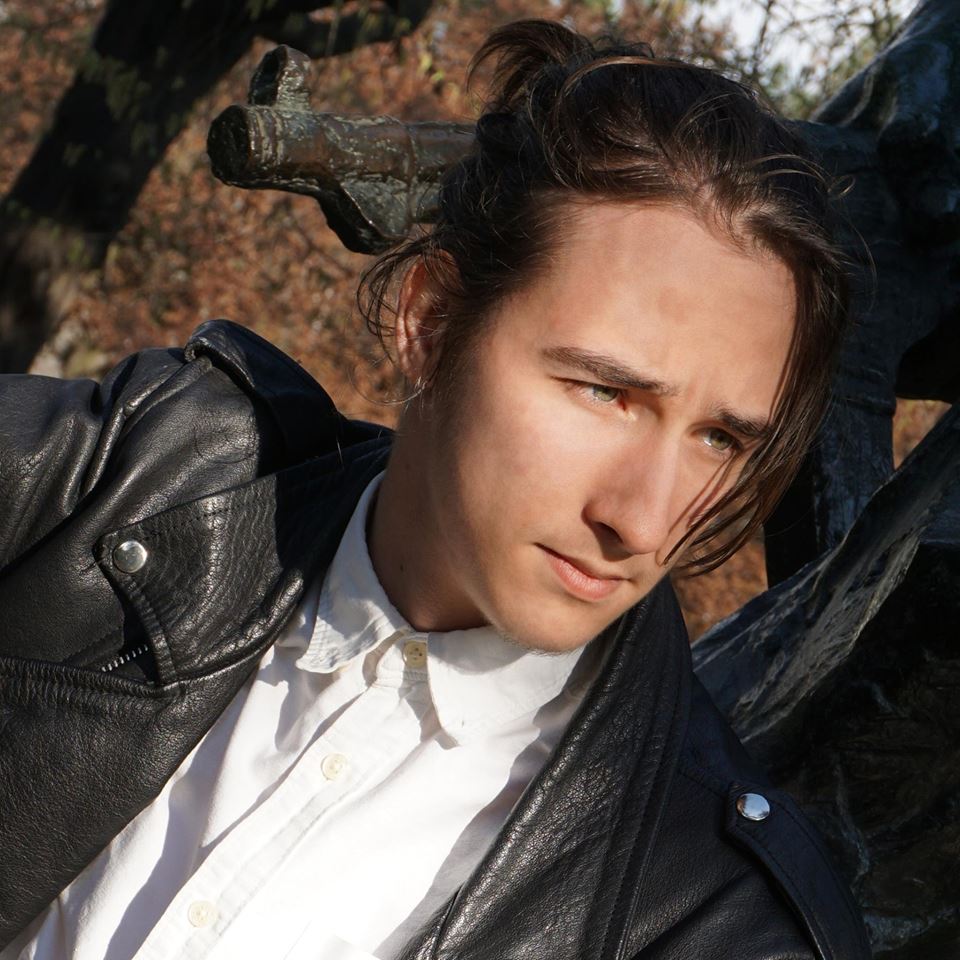
- Introduction
Strings are one of the most common data types used in software - they represent strings of characters that we parse as words.
String concatenation is a pretty standard task, especially since we don't always deal with static strings. We often create strings dynamically, such as with ES6 Templates, or simply concatenate strings to form new ones.
If you'd like to read more about creating dynamic formatted strings - read our Guide to ES6 Templates/String Literals in Node.js !
In this tutorial, we'll take a look at how to join/append/concatenate strings in JavaScript.
Note: Strings are immutable , meaning they can't really be changed. Whenever you call a changing operation on a string, a copy is constructed with the changes applied and it's returned instead of the original one.
- Concatenate With the + Operator
The easiest, and probably most intuitive approach to string concatenation is using the + operator:
Running this short piece of code results in:
It is possible to assign a string in memory to a reference variable, and reference the object instead of concatenating it directly:
This also results in:
Note: In most cases, you can reasonably replace a = and + with the shorthand += , which lets you omit the reference to the first operand , which will be replaced with the variable you're assigning the result to.
Instead, you could be writing the short-hand version:
It's worth noting that other data types can be thrown into the mix as well, and whenever implicit conversion is possible - JavaScript will "match" the data types into strings.
For instance, integers are easily converted to strings so if you try concatenating an integer onto a string - the integer is converted before being appended. A string may not be converted into an integer at all times - such as when it doesn't represent a number or has odd formatting, so generally, it's easier to convert to a string, than from a string:
This results in both the boolean and integer being converted into string representations and then appended to the "hello" string:
- String.prototype.concat()
The concat() method was created specifically for this task - to concatenate strings. It can be invoked over any string, and accepts another string to be concatenated to the calling one.
In a sense, it's similar to using the short-hand += operator:
Check out our hands-on, practical guide to learning Git, with best-practices, industry-accepted standards, and included cheat sheet. Stop Googling Git commands and actually learn it!
This results in:
Additionally, you can specify a delimiter as the first argument, which is then added in-between all of the concatenated strings:
- Array.prototype.join()
The join() method of the Array class is used to join the elements of an array into a string, with a default delimiter of a comma ( , ).
This allows us to deal with a larger number of strings in a much more concise way, and it's not unheard of to read a file into an array, or to work with an array of strings you'd like to join together.
If you don't pass in any arguments into the method - the default value will be used to delimit the newly joined strings. Let's pass in an empty string to skip that and just join them similar to what we've seen before:
- Which Approach to Use?
It's advised to prefer the concat() function instead of the + operator , due to performance benefits. However, this doesn't actually always hold and takes in several assumptions into mind.
First of all - the number of strings you're concatenating plays a role in this, as well as other factors you might not even be aware of. Let's benchmark the concat() method as well as the + operator:
But wait, let's switch the environment and run this on a different machine:
And let's run that on the same machine again:
The execution times vary wildly (although, all of them are acceptable in speed). It's also worth noting the official statement from MDN , regarding the performance benefits:
It is strongly recommended that the assignment operators (+, +=) are used instead of the concat() method.
Which might seem odd, given the fact that concat() outperforms the operators in the tests or at worst is the same speed. What gives? Well, benchmarking code like this isn't as easy as simply running it and observing the results.
Your browser, its version, as well as the optimizer it uses may vary from machine to machine, and properties like those really impact the performance. For instance, we've used different strings in the concatenation, the ones generated from iteration. If we were to use the same string, an optimizer such as Google's V8 would further optimize the usage of the string.
Test and verify your own code instead of taking advice at face value. Not all machines and environments are the same, and what works great on one, might not work great on another. Take this into consideration when creating applications as well.
We've taken a look at how to concatenate strings in JavaScript using the + operator, the join() method of the Arrays class as well as the concat() method of the String class.
We've taken a look at the official stance and ran our own tests to see which approach is the most performant - and as usual, it depends on various factors.
You might also like...
- JavaScript: Check if First Letter of a String is Upper Case
- Using Mocks for Testing in JavaScript with Sinon.js
- For-each Over an Array in JavaScript
- Commenting Code in JavaScript - Types and Best Practices
Improve your dev skills!
Get tutorials, guides, and dev jobs in your inbox.
No spam ever. Unsubscribe at any time. Read our Privacy Policy.
Entrepreneur, Software and Machine Learning Engineer, with a deep fascination towards the application of Computation and Deep Learning in Life Sciences (Bioinformatics, Drug Discovery, Genomics), Neuroscience (Computational Neuroscience), robotics and BCIs.
Great passion for accessible education and promotion of reason, science, humanism, and progress.
In this article
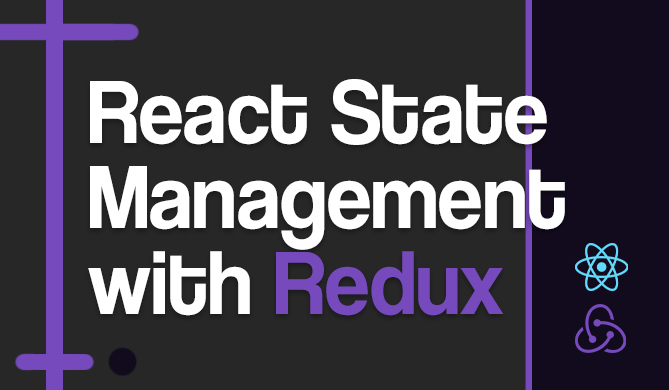
React State Management with Redux and Redux-Toolkit
Coordinating state and keeping components in sync can be tricky. If components rely on the same data but do not communicate with each other when...
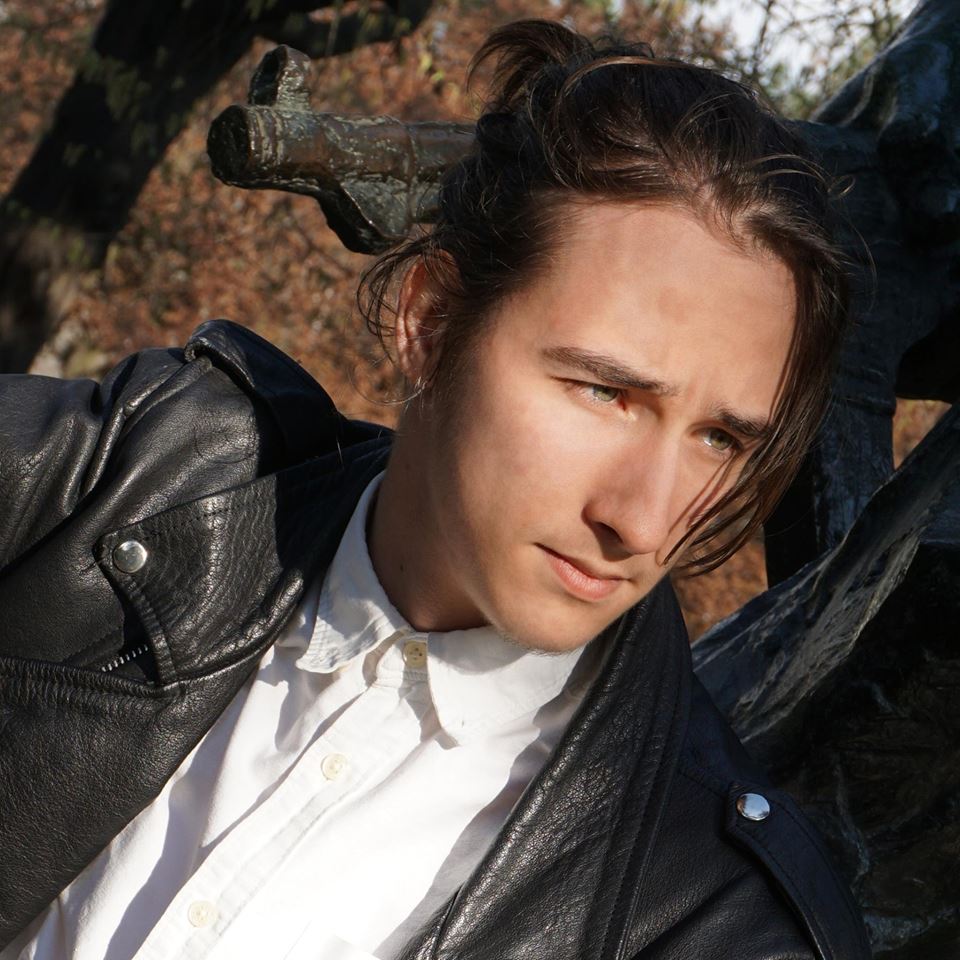
Getting Started with AWS in Node.js
Build the foundation you'll need to provision, deploy, and run Node.js applications in the AWS cloud. Learn Lambda, EC2, S3, SQS, and more!
© 2013- 2024 Stack Abuse. All rights reserved.
- Skip to main content
- Select language
- Skip to search
- Assignment operators
An assignment operator assigns a value to its left operand based on the value of its right operand.
The basic assignment operator is equal ( = ), which assigns the value of its right operand to its left operand. That is, x = y assigns the value of y to x . The other assignment operators are usually shorthand for standard operations, as shown in the following definitions and examples.
Simple assignment operator which assigns a value to a variable. The assignment operation evaluates to the assigned value. Chaining the assignment operator is possible in order to assign a single value to multiple variables. See the example.
Addition assignment
The addition assignment operator adds the value of the right operand to a variable and assigns the result to the variable. The types of the two operands determine the behavior of the addition assignment operator. Addition or concatenation is possible. See the addition operator for more details.
Subtraction assignment
The subtraction assignment operator subtracts the value of the right operand from a variable and assigns the result to the variable. See the subtraction operator for more details.
Multiplication assignment
The multiplication assignment operator multiplies a variable by the value of the right operand and assigns the result to the variable. See the multiplication operator for more details.
Division assignment
The division assignment operator divides a variable by the value of the right operand and assigns the result to the variable. See the division operator for more details.
Remainder assignment
The remainder assignment operator divides a variable by the value of the right operand and assigns the remainder to the variable. See the remainder operator for more details.
Exponentiation assignment
This is an experimental technology, part of the ECMAScript 2016 (ES7) proposal. Because this technology's specification has not stabilized, check the compatibility table for usage in various browsers. Also note that the syntax and behavior of an experimental technology is subject to change in future version of browsers as the spec changes.
The exponentiation assignment operator evaluates to the result of raising first operand to the power second operand. See the exponentiation operator for more details.
Left shift assignment
The left shift assignment operator moves the specified amount of bits to the left and assigns the result to the variable. See the left shift operator for more details.
Right shift assignment
The right shift assignment operator moves the specified amount of bits to the right and assigns the result to the variable. See the right shift operator for more details.
Unsigned right shift assignment
The unsigned right shift assignment operator moves the specified amount of bits to the right and assigns the result to the variable. See the unsigned right shift operator for more details.
Bitwise AND assignment
The bitwise AND assignment operator uses the binary representation of both operands, does a bitwise AND operation on them and assigns the result to the variable. See the bitwise AND operator for more details.
Bitwise XOR assignment
The bitwise XOR assignment operator uses the binary representation of both operands, does a bitwise XOR operation on them and assigns the result to the variable. See the bitwise XOR operator for more details.
Bitwise OR assignment
The bitwise OR assignment operator uses the binary representation of both operands, does a bitwise OR operation on them and assigns the result to the variable. See the bitwise OR operator for more details.
Left operand with another assignment operator
In unusual situations, the assignment operator (e.g. x += y ) is not identical to the meaning expression (here x = x + y ). When the left operand of an assignment operator itself contains an assignment operator, the left operand is evaluated only once. For example:
Specifications
Browser compatibility.
- Arithmetic operators
Document Tags and Contributors
- JavaScript basics
- JavaScript first steps
- JavaScript building blocks
- Introducing JavaScript objects
- Introduction
- Grammar and types
- Control flow and error handling
- Loops and iteration
- Expressions and operators
- Numbers and dates
- Text formatting
- Regular expressions
- Indexed collections
- Keyed collections
- Working with objects
- Details of the object model
- Iterators and generators
- Meta programming
- A re-introduction to JavaScript
- JavaScript data structures
- Equality comparisons and sameness
- Inheritance and the prototype chain
- Strict mode
- JavaScript typed arrays
- Memory Management
- Concurrency model and Event Loop
- References:
- ArrayBuffer
- AsyncFunction
- Float32Array
- Float64Array
- GeneratorFunction
- InternalError
- Intl.Collator
- Intl.DateTimeFormat
- Intl.NumberFormat
- ParallelArray
- ReferenceError
- SIMD.Bool16x8
- SIMD.Bool32x4
- SIMD.Bool64x2
- SIMD.Bool8x16
- SIMD.Float32x4
- SIMD.Float64x2
- SIMD.Int16x8
- SIMD.Int32x4
- SIMD.Int8x16
- SIMD.Uint16x8
- SIMD.Uint32x4
- SIMD.Uint8x16
- SharedArrayBuffer
- StopIteration
- SyntaxError
- Uint16Array
- Uint32Array
- Uint8ClampedArray
- WebAssembly
- decodeURI()
- decodeURIComponent()
- encodeURI()
- encodeURIComponent()
- parseFloat()
- Array comprehensions
- Bitwise operators
- Comma operator
- Comparison operators
- Conditional (ternary) Operator
- Destructuring assignment
- Expression closures
- Generator comprehensions
- Grouping operator
- Legacy generator function expression
- Logical Operators
- Object initializer
- Operator precedence
- Property accessors
- Spread syntax
- async function expression
- class expression
- delete operator
- function expression
- function* expression
- in operator
- new operator
- void operator
- Legacy generator function
- async function
- for each...in
- function declaration
- try...catch
- Arguments object
- Arrow functions
- Default parameters
- Method definitions
- Rest parameters
- constructor
- element loaded from a different domain for which you violated the same-origin policy.">Error: Permission denied to access property "x"
- InternalError: too much recursion
- RangeError: argument is not a valid code point
- RangeError: invalid array length
- RangeError: invalid date
- RangeError: precision is out of range
- RangeError: radix must be an integer
- RangeError: repeat count must be less than infinity
- RangeError: repeat count must be non-negative
- ReferenceError: "x" is not defined
- ReferenceError: assignment to undeclared variable "x"
- ReferenceError: deprecated caller or arguments usage
- ReferenceError: invalid assignment left-hand side
- ReferenceError: reference to undefined property "x"
- SyntaxError: "0"-prefixed octal literals and octal escape seq. are deprecated
- SyntaxError: "use strict" not allowed in function with non-simple parameters
- SyntaxError: "x" is a reserved identifier
- SyntaxError: JSON.parse: bad parsing
- SyntaxError: Malformed formal parameter
- SyntaxError: Unexpected token
- SyntaxError: Using //@ to indicate sourceURL pragmas is deprecated. Use //# instead
- SyntaxError: a declaration in the head of a for-of loop can't have an initializer
- SyntaxError: applying the 'delete' operator to an unqualified name is deprecated
- SyntaxError: for-in loop head declarations may not have initializers
- SyntaxError: function statement requires a name
- SyntaxError: identifier starts immediately after numeric literal
- SyntaxError: illegal character
- SyntaxError: invalid regular expression flag "x"
- SyntaxError: missing ) after argument list
- SyntaxError: missing ) after condition
- SyntaxError: missing : after property id
- SyntaxError: missing ; before statement
- SyntaxError: missing = in const declaration
- SyntaxError: missing ] after element list
- SyntaxError: missing formal parameter
- SyntaxError: missing name after . operator
- SyntaxError: missing variable name
- SyntaxError: missing } after function body
- SyntaxError: missing } after property list
- SyntaxError: redeclaration of formal parameter "x"
- SyntaxError: return not in function
- SyntaxError: test for equality (==) mistyped as assignment (=)?
- SyntaxError: unterminated string literal
- TypeError: "x" has no properties
- TypeError: "x" is (not) "y"
- TypeError: "x" is not a constructor
- TypeError: "x" is not a function
- TypeError: "x" is not a non-null object
- TypeError: "x" is read-only
- TypeError: More arguments needed
- TypeError: can't access dead object
- TypeError: can't define property "x": "obj" is not extensible
- TypeError: can't redefine non-configurable property "x"
- TypeError: cyclic object value
- TypeError: invalid 'in' operand "x"
- TypeError: invalid Array.prototype.sort argument
- TypeError: invalid arguments
- TypeError: invalid assignment to const "x"
- TypeError: property "x" is non-configurable and can't be deleted
- TypeError: setting getter-only property "x"
- TypeError: variable "x" redeclares argument
- URIError: malformed URI sequence
- Warning: -file- is being assigned a //# sourceMappingURL, but already has one
- Warning: 08/09 is not a legal ECMA-262 octal constant
- Warning: Date.prototype.toLocaleFormat is deprecated
- Warning: JavaScript 1.6's for-each-in loops are deprecated
- Warning: String.x is deprecated; use String.prototype.x instead
- Warning: expression closures are deprecated
- Warning: unreachable code after return statement
- JavaScript technologies overview
- Lexical grammar
- Enumerability and ownership of properties
- Iteration protocols
- Transitioning to strict mode
- Template literals
- Deprecated features
- ECMAScript 2015 support in Mozilla
- ECMAScript 5 support in Mozilla
- ECMAScript Next support in Mozilla
- Firefox JavaScript changelog
- New in JavaScript 1.1
- New in JavaScript 1.2
- New in JavaScript 1.3
- New in JavaScript 1.4
- New in JavaScript 1.5
- New in JavaScript 1.6
- New in JavaScript 1.7
- New in JavaScript 1.8
- New in JavaScript 1.8.1
- New in JavaScript 1.8.5
- Documentation:
- All pages index
- Methods index
- Properties index
- Pages tagged "JavaScript"
- JavaScript doc status
- The MDN project

JAVASCRIPT ASSIGNMENT OPERATORS
In this tutorial, you will learn about all the different assignment operators in javascript and how to use them in javascript.
Assignment Operators
In javascript, there are 16 different assignment operators that are used to assign value to the variable. It is shorthand of other operators which is recommended to use.
The assignment operators are used to assign value based on the right operand to its left operand.
The left operand must be a variable while the right operand may be a variable, number, boolean, string, expression, object, or combination of any other.
One of the most basic assignment operators is equal = , which is used to directly assign a value.
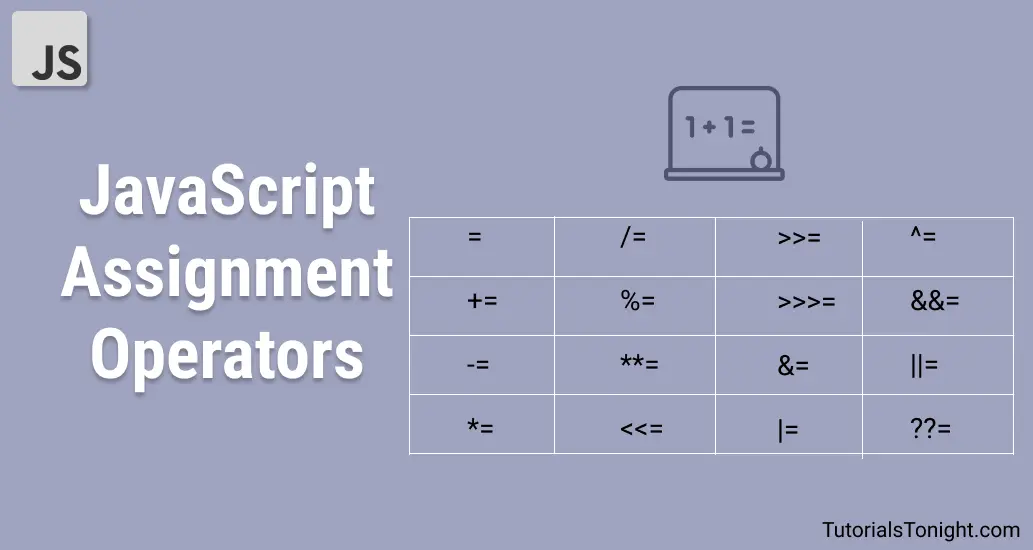
Assignment Operators List
Here is the list of all assignment operators in JavaScript:
In the following table if variable a is not defined then assume it to be 10.
Assignment operator
The assignment operator = is the simplest value assigning operator which assigns a given value to a variable.
The assignment operators support chaining, which means you can assign a single value in multiple variables in a single line.
Addition assignment operator
The addition assignment operator += is used to add the value of the right operand to the value of the left operand and assigns the result to the left operand.
On the basis of the data type of variable, the addition assignment operator may add or concatenate the variables.
Subtraction assignment operator
The subtraction assignment operator -= subtracts the value of the right operand from the value of the left operand and assigns the result to the left operand.
If the value can not be subtracted then it results in a NaN .
Multiplication assignment operator
The multiplication assignment operator *= assigns the result to the left operand after multiplying values of the left and right operand.
Division assignment operator
The division assignment operator /= divides the value of the left operand by the value of the right operand and assigns the result to the left operand.
Remainder assignment operator
The remainder assignment operator %= assigns the remainder to the left operand after dividing the value of the left operand by the value of the right operand.
Exponentiation assignment operator
The exponential assignment operator **= assigns the result of exponentiation to the left operand after exponentiating the value of the left operand by the value of the right operand.
Left shift assignment
The left shift assignment operator <<= assigns the result of the left shift to the left operand after shifting the value of the left operand by the value of the right operand.
Right shift assignment
The right shift assignment operator >>= assigns the result of the right shift to the left operand after shifting the value of the left operand by the value of the right operand.
Unsigned right shift assignment
The unsigned right shift assignment operator >>>= assigns the result of the unsigned right shift to the left operand after shifting the value of the left operand by the value of the right operand.
Bitwise AND assignment
The bitwise AND assignment operator &= assigns the result of bitwise AND to the left operand after ANDing the value of the left operand by the value of the right operand.
Bitwise OR assignment
The bitwise OR assignment operator |= assigns the result of bitwise OR to the left operand after ORing the value of left operand by the value of the right operand.
Bitwise XOR assignment
The bitwise XOR assignment operator ^= assigns the result of bitwise XOR to the left operand after XORing the value of the left operand by the value of the right operand.
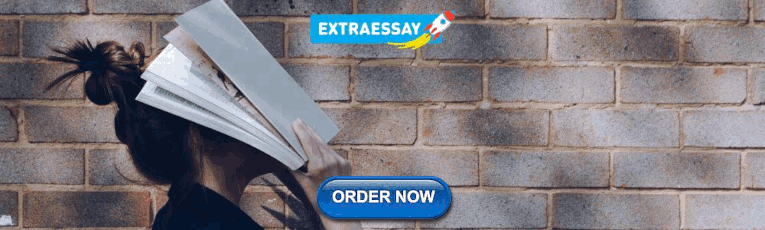
Logical AND assignment
The logical AND assignment operator &&= assigns value to left operand only when it is truthy .
Note : A truthy value is a value that is considered true when encountered in a boolean context.
Logical OR assignment
The logical OR assignment operator ||= assigns value to left operand only when it is falsy .
Note : A falsy value is a value that is considered false when encountered in a boolean context.
Logical nullish assignment
The logical nullish assignment operator ??= assigns value to left operand only when it is nullish ( null or undefined ).
4 ways to concatenate strings in JavaScript
Concat() method, the + operator, template literals, join() method.
String concatenation is a common task in any programming language. There are 4 ways to combine strings in JavaScript.
In this article, we'll look at all these methods to perform string concatenation in JavaScript.
JavaScript String object has a built-in concat() method. As the name suggests, this method joins or merges two or more strings.
The concat() method doesn't modify the string in place. Instead, it creates and returns a new string containing the text of the joined strings.
If the parameters are not of the type string, they are automatically converted to string values before concatenating.
The same + operator that we used to add two numbers can also be used to combine two strings:
The above method creates a brand new string. To mutate the existing string, you could use the shorthand += operator:
The assignment operators ( + , += ) perform faster on modern JavaScript engines, so there is no need to use the concat() method.
However, if you prefer readability over performance, use template literals (explained below).
Template literals provides a modern way to work with strings in JavaScript. They are primarily string literals with embedded expressions. You can use string interpolation, multi-line strings , and tagged expressions with them.
I personally use template literals in JavaScript (and even in Node.js) to combine strings. Because they're more readable with no awkward backslashes to escape quotes, no empty spaces, and no more plus operators. You write exactly how you want your string to appear.
The join() method concatenates all elements in an array and returns a new string:
The first parameter of the join() method is the separator. If skipped, a comma ( , ) is used as a default separator:
You can pass in any separator you want to combine strings:
✌️ Like this article? Follow me on Twitter and LinkedIn . You can also subscribe to RSS Feed .
You might also like...
- Get the length of a Map in JavaScript
- Delete an element from a Map in JavaScript
- Get the first element of a Map in JavaScript
- Get an element from a Map using JavaScript
- Update an element in a Map using JavaScript
- Add an element to a Map in JavaScript
The simplest cloud platform for developers & teams. Start with a $200 free credit.
Buy me a coffee ☕
If you enjoy reading my articles and want to help me out paying bills, please consider buying me a coffee ($5) or two ($10). I will be highly grateful to you ✌️
Enter the number of coffees below:
✨ Learn to build modern web applications using JavaScript and Spring Boot
I started this blog as a place to share everything I have learned in the last decade. I write about modern JavaScript, Node.js, Spring Boot, core Java, RESTful APIs, and all things web development.
The newsletter is sent every week and includes early access to clear, concise, and easy-to-follow tutorials, and other stuff I think you'd enjoy! No spam ever, unsubscribe at any time.
- JavaScript, Node.js & Spring Boot
- In-depth tutorials
- Super-handy protips
- Cool stuff around the web
- 1-click unsubscribe
- No spam, free-forever!
JS Tutorial
Js versions, js functions, js html dom, js browser bom, js web apis, js vs jquery, js graphics, js examples, js references, javascript assignment, javascript assignment operators.
Assignment operators assign values to JavaScript variables.
Shift Assignment Operators
Bitwise assignment operators, logical assignment operators, the = operator.
The Simple Assignment Operator assigns a value to a variable.
Simple Assignment Examples
The += operator.
The Addition Assignment Operator adds a value to a variable.
Addition Assignment Examples
The -= operator.
The Subtraction Assignment Operator subtracts a value from a variable.
Subtraction Assignment Example
The *= operator.
The Multiplication Assignment Operator multiplies a variable.
Multiplication Assignment Example
The **= operator.
The Exponentiation Assignment Operator raises a variable to the power of the operand.
Exponentiation Assignment Example
The /= operator.
The Division Assignment Operator divides a variable.
Division Assignment Example
The %= operator.
The Remainder Assignment Operator assigns a remainder to a variable.
Remainder Assignment Example
Advertisement
The <<= Operator
The Left Shift Assignment Operator left shifts a variable.
Left Shift Assignment Example
The >>= operator.
The Right Shift Assignment Operator right shifts a variable (signed).
Right Shift Assignment Example
The >>>= operator.
The Unsigned Right Shift Assignment Operator right shifts a variable (unsigned).
Unsigned Right Shift Assignment Example
The &= operator.
The Bitwise AND Assignment Operator does a bitwise AND operation on two operands and assigns the result to the the variable.
Bitwise AND Assignment Example
The |= operator.
The Bitwise OR Assignment Operator does a bitwise OR operation on two operands and assigns the result to the variable.
Bitwise OR Assignment Example
The ^= operator.
The Bitwise XOR Assignment Operator does a bitwise XOR operation on two operands and assigns the result to the variable.
Bitwise XOR Assignment Example
The &&= operator.
The Logical AND assignment operator is used between two values.
If the first value is true, the second value is assigned.
Logical AND Assignment Example
The &&= operator is an ES2020 feature .
The ||= Operator
The Logical OR assignment operator is used between two values.
If the first value is false, the second value is assigned.
Logical OR Assignment Example
The ||= operator is an ES2020 feature .
The ??= Operator
The Nullish coalescing assignment operator is used between two values.
If the first value is undefined or null, the second value is assigned.
Nullish Coalescing Assignment Example
The ??= operator is an ES2020 feature .
Test Yourself With Exercises
Use the correct assignment operator that will result in x being 15 (same as x = x + y ).
Start the Exercise

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
- DSA with JS - Self Paced
- JS Tutorial
- JS Exercise
- JS Interview Questions
- JS Operator
- JS Projects
- JS Examples
- JS Free JS Course
- JS A to Z Guide
- JS Formatter
- JavaScript Tutorial
JavaScript Basics
- Introduction to JavaScript
- JavaScript Versions
- How to Add JavaScript in HTML Document?
- JavaScript Statements
- JavaScript Syntax
- JavaScript Output
- JavaScript Comments
JS Variables & Datatypes
- Variables and Datatypes in JavaScript
- Global and Local variables in JavaScript
- JavaScript Let
- JavaScript Const
- JavaScript var
JS Operators
Javascript operators.
- Operator precedence in JavaScript
JavaScript Arithmetic Operators
Javascript assignment operators, javascript comparison operators, javascript logical operators, javascript bitwise operators.
- JavaScript Ternary Operator
- JavaScript Comma Operator
JavaScript Unary Operators
- JavaScript Relational operators
JavaScript String Operators
- JavaScript Loops
- 7 Loops of JavaScript
- JavaScript For Loop
- JavaScript While Loop
- JavaScript for-in Loop
- JavaScript for...of Loop
- JavaScript do...while Loop
JS Perfomance & Debugging
- JavaScript | Performance
- Debugging in JavaScript
- JavaScript Errors Throw and Try to Catch
- Objects in Javascript
- Introduction to Object Oriented Programming in JavaScript
- JavaScript Objects
- Creating objects in JavaScript (4 Different Ways)
- JavaScript JSON Objects
- JavaScript Object Reference
JS Function
- Functions in JavaScript
- How to write a function in JavaScript ?
- JavaScript Function Call
- Different ways of writing functions in JavaScript
- Difference between Methods and Functions in JavaScript
- Explain the Different Function States in JavaScript
- JavaScript Function Complete Reference
- JavaScript Arrays
- JavaScript Array Methods
- Best-Known JavaScript Array Methods
- What are the Important Array Methods of JavaScript ?
- JavaScript Array Reference
- JavaScript Strings
- JavaScript String Methods
- JavaScript String Reference
- JavaScript Numbers
- How numbers are stored in JavaScript ?
- How to create a Number object using JavaScript ?
- JavaScript Number Reference
- JavaScript Math Object
- What is the use of Math object in JavaScript ?
- JavaScript Math Reference
- JavaScript Map
- What is JavaScript Map and how to use it ?
- JavaScript Map Reference
- Sets in JavaScript
- How are elements ordered in a Set in JavaScript ?
- How to iterate over Set elements in JavaScript ?
- How to sort a set in JavaScript ?
- JavaScript Set Reference
- JavaScript Date
- JavaScript Promise
- JavaScript BigInt
- JavaScript Boolean
- JavaScript Proxy/Handler
- JavaScript WeakMap
- JavaScript WeakSet
- JavaScript Function Generator
- JavaScript JSON
- Arrow functions in JavaScript
- JavaScript this Keyword
- Strict mode in JavaScript
- Introduction to ES6
- JavaScript Hoisting
- Async and Await in JavaScript
JavaScript Exercises
- JavaScript Exercises, Practice Questions and Solutions
JavaScript operators are symbols used to operate the operands. Operators are used to perform specific mathematical and logical computations on operands.
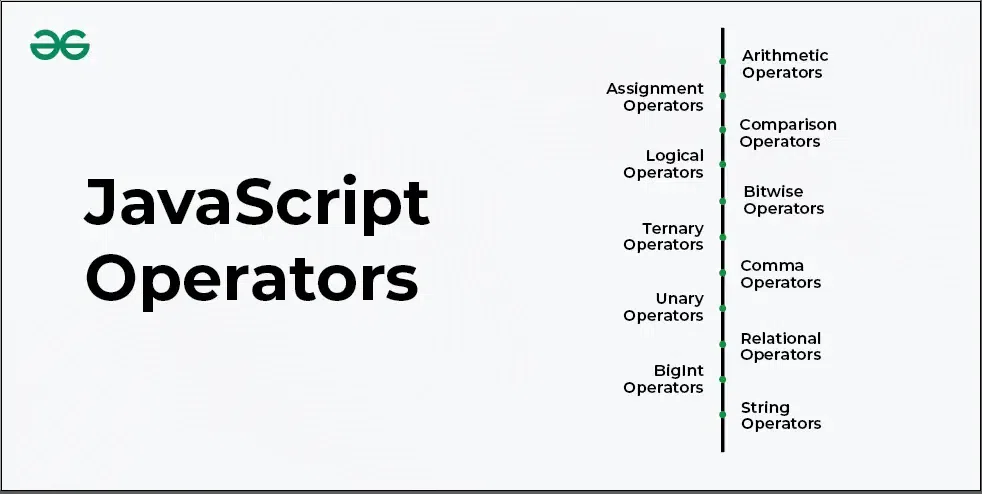
JavaScript Operators: There are various operators supported by JavaScript.
Table of Content
JavaScript Ternary Operators
Javascript comma operators, javascript relational operators, javascript bigint operators.
JavaScript Arithmetic Operators perform arithmetic operations: addition (+), subtraction (-), multiplication (*), division (/), modulus (%), and exponentiation (**).
The assignment operation evaluates the assigned value. Chaining the assignment operator is possible in order to assign a single value to multiple variables
Comparison operators are mainly used to perform the logical operations that determine the equality or difference between the values.
JavaScript Logical Operators perform logical operations: AND (&&), OR (||), and NOT (!), evaluating expressions and returning boolean values.
The bitwise operator in JavaScript is used to convert the number to a 32-bit binary number and perform the bitwise operation. The number is converted back to the 64-bit number after the result.
The ternary operator has three operands. It is the simplified operator of if/else.
Comma Operator (,) mainly evaluates its operands from left to right sequentially and returns the value of the rightmost operand.
A unary operation is an operation with only one operand.
JavaScript Relational operators are used to compare its operands and determine the relationship between them. They return a Boolean value (true or false) based on the comparison result.
JavaScript BigInt operators support arithmetic operations on BigInt data type, including addition, subtraction, multiplication, division, and exponentiation. Most operators that can be used between numbers can be used between BigInt values as well.
JavaScript String Operators include concatenation (+) and concatenation assignment (+=), used to join strings or combine strings with other data types.
We have a list of JavaScript Operators reference where you can know more about these operators.
Please Login to comment...
Similar reads.
- javascript-operators
- Web Technologies
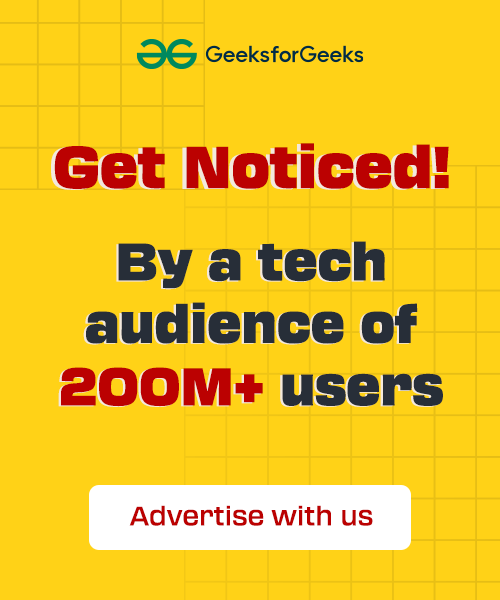
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
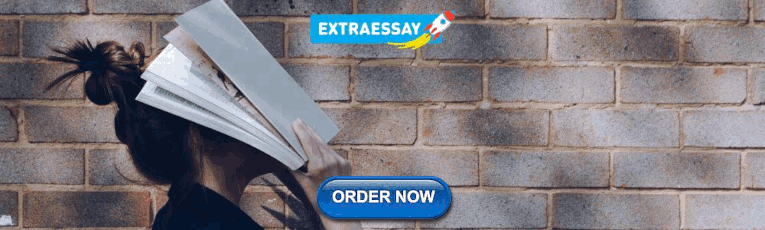
IMAGES
VIDEO
COMMENTS
Please see below code. str += '<br>Multiply & assign: ' + initB; I get that the += operator, when dealing with strings concatenates the two operands and when dealing with numbers they add the values of the operands and reassign to the computed value to first operand. I also get that str is being initialised as 'Add & assign string ' + msg; and ...
This chapter describes JavaScript's expressions and operators, including assignment, comparison, arithmetic, bitwise, logical, string, ternary and more. At a high level, an expression is a valid unit of code that resolves to a value. There are two types of expressions: those that have side effects (such as assigning values) and those that ...
JavaScript Assignment Operators. Assignment operators assign values to JavaScript variables. The Addition Assignment Operator (+=) adds a value to a variable. ... When used on strings, the + operator is called the concatenation operator. Adding Strings and Numbers. Adding two numbers, will return the sum, but adding a number and a string will ...
169. Seems based on benchmarks at JSPerf that using += is the fastest method, though not necessarily in every browser. For building strings in the DOM, it seems to be better to concatenate the string first and then add to the DOM, rather then iteratively add it to the dom. You should benchmark your own case though.
The shorthand assignment operator += can also be used to concatenate strings. For example, var mystring = 'alpha'; mystring += 'bet'; // evaluates to "alphabet" and assigns this value to mystring. Conditional (ternary) operator. The conditional operator is the only JavaScript operator that takes three operands. The operator can have one of two ...
1) Using the concat() method. The String.prototype.concat() concatenates two or more string arguments and returns a new string: let newString = string.concat(...str); Code language: JavaScript (javascript) The concat() accepts a varied number of string arguments and returns a new string containing the combined string arguments.
There are 3 ways to concatenate strings in JavaScript. In this tutorial, you'll the different ways and the tradeoffs between them. The + Operator. The same + operator you use for adding two numbers can be used to concatenate two strings.. const str = 'Hello' + ' ' + 'World'; str; // 'Hello World'. You can also use +=, where a += b is a shorthand for a = a + b. ...
Concatenating with Operator: 2.030ms Concatenating with Function: 0.934ms The execution times vary wildly (although, all of them are acceptable in speed). It's also worth noting the official statement from MDN, regarding the performance benefits: It is strongly recommended that the assignment operators (+, +=) are used instead of the concat ...
An assignment operator assigns a value to its left operand based on the value of its right operand.. Overview. The basic assignment operator is equal (=), which assigns the value of its right operand to its left operand.That is, x = y assigns the value of y to x.The other assignment operators are usually shorthand for standard operations, as shown in the following definitions and examples.
The assignment operator is completely different from the equals (=) sign used as syntactic separators in other locations, which include:Initializers of var, let, and const declarations; Default values of destructuring; Default parameters; Initializers of class fields; All these places accept an assignment expression on the right-hand side of the =, so if you have multiple equals signs chained ...
In JavaScript, when the + operator is used with a String value, it is called the concatenation operator. You can build a new string out of other strings by concatenating them together. Example 'My name is Alan,' + ' I concatenate.' Note: Watch out for spaces. Concatenation does not add spaces between concatenated strings, so you'll need to add ...
The addition assignment (+=) operator performs addition (which is either numeric addition or string concatenation) on the two operands and assigns the result to the left operand. Try it Syntax
Addition Assignment Operator(+=) The Addition assignment operator adds the value to the right operand to a variable and assigns the result to the variable. Addition or concatenation is possible. In case of concatenation then we use the string as an operand.
Addition assignment operator. The addition assignment operator += is used to add the value of the right operand to the value of the left operand and assigns the result to the left operand. On the basis of the data type of variable, the addition assignment operator may add or concatenate the variables.
The assignment operators (+, +=) perform faster on modern JavaScript engines, so there is no need to use the concat() method. However, if you prefer readability over performance, use template literals (explained below). Template Literals. Template literals provides a modern way to work with strings in JavaScript. They are primarily string ...
In this, we perform a concatenation assignment by using the '+=' operator to add the value of a variable or string to an existing string variable. Syntax: str1 += str2. ... JavaScript Assignment Operators V. vishalkumar2204. Follow. Article Tags : javascript-basics; javascript-operators; JavaScript; Web Technologies
JavaScript Addition assignment operator (+=) adds a value to a variable, The Addition Assignment (+ =) Sums up left and right operand values and then assigns the result to the left operand. The two major operations that can be performed using this operator are the addition of numbers and the concatenation of strings. Syntax: Example 1: In this ...
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. ... The Bitwise AND Assignment Operator does a bitwise AND operation on two operands and assigns the result to the the variable.
JavaScript Assignment Operators. The assignment operation evaluates the assigned value. Chaining the assignment operator is possible in order to assign a single value to multiple variables ... concatenation operator (+) It concatenates two string values together, returning another string that is the union of the two operand strings. str1 + str2 ...
Combining Operators. We can combine assignment operators with all mathematical operators, which include arithmetic operators and bitwise operators. For example, if we have x set to 1 and y set to 2, and we want to set x to the sum of x and y, we write x += y, which is the same as x += y. JavaScript has many operators. They allow us to do a lot ...
The addition assignment operator `(+=)` adds a value to a variable. `x += y` means `x = x + y` The `+=` assignment operator can also be used to add (concatenate) strings: Example: txt1 = "What a very "; txt1 += "nice day"; The result of txt1 will be: What a very nice day On the other hand adding empty String `''` will make javascript to confuse ...