- General Solutions
- Ruby On Rails
- Jackson (JSON Object Mapper)
- GSON (JSON Object Mapper)
- JSON-Lib (JSON Object Mapper)
- Flexjson (JSON Object Mapper)
- References and future reading
- Microservices Security
- Microservices based Security Arch Doc
- Mobile Application Security
- Multifactor Authentication
- NPM Security
- Network Segmentation
- NodeJS Docker
- Nodejs Security
- OS Command Injection Defense
- PHP Configuration
- Password Storage
- Prototype Pollution Prevention
- Query Parameterization
- REST Assessment
- REST Security
- Ruby on Rails
- SAML Security
- SQL Injection Prevention
- Secrets Management
- Secure Cloud Architecture
- Secure Product Design
- Securing Cascading Style Sheets
- Server Side Request Forgery Prevention
- Session Management
- TLS Cipher String
- Third Party Javascript Management
- Threat Modeling
- Transaction Authorization
- Transport Layer Protection
- Transport Layer Security
- Unvalidated Redirects and Forwards
- User Privacy Protection
- Virtual Patching
- Vulnerability Disclosure
- Vulnerable Dependency Management
- Web Service Security
- XML External Entity Prevention
- XML Security
- XSS Filter Evasion
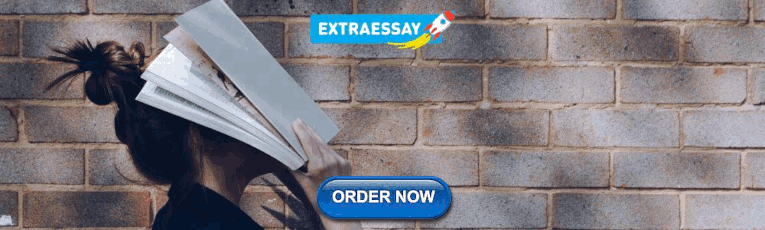
Mass Assignment Cheat Sheet ¶
Introduction ¶, definition ¶.
Software frameworks sometime allow developers to automatically bind HTTP request parameters into program code variables or objects to make using that framework easier on developers. This can sometimes cause harm.
Attackers can sometimes use this methodology to create new parameters that the developer never intended which in turn creates or overwrites new variable or objects in program code that was not intended.
This is called a Mass Assignment vulnerability.
Alternative Names ¶
Depending on the language/framework in question, this vulnerability can have several alternative names :
- Mass Assignment: Ruby on Rails, NodeJS.
- Autobinding: Spring MVC, ASP NET MVC.
- Object injection: PHP.
Example ¶
Suppose there is a form for editing a user's account information:
Here is the object that the form is binding to:
Here is the controller handling the request:
Here is the typical request:
And here is the exploit in which we set the value of the attribute isAdmin of the instance of the class User :
Exploitability ¶
This functionality becomes exploitable when:
- Attacker can guess common sensitive fields.
- Attacker has access to source code and can review the models for sensitive fields.
- AND the object with sensitive fields has an empty constructor.
GitHub case study ¶
In 2012, GitHub was hacked using mass assignment. A user was able to upload his public key to any organization and thus make any subsequent changes in their repositories. GitHub's Blog Post .
Solutions ¶
- Allow-list the bindable, non-sensitive fields.
- Block-list the non-bindable, sensitive fields.
- Use Data Transfer Objects (DTOs).
General Solutions ¶
An architectural approach is to create Data Transfer Objects and avoid binding input directly to domain objects. Only the fields that are meant to be editable by the user are included in the DTO.
Language & Framework specific solutions ¶
Spring mvc ¶, allow-listing ¶.
Take a look here for the documentation.
Block-listing ¶
Nodejs + mongoose ¶, ruby on rails ¶, django ¶, asp net ¶, php laravel + eloquent ¶, grails ¶, play ¶, jackson (json object mapper) ¶.
Take a look here and here for the documentation.
GSON (JSON Object Mapper) ¶
Take a look here and here for the document.
JSON-Lib (JSON Object Mapper) ¶
Flexjson (json object mapper) ¶, references and future reading ¶.
- Mass Assignment, Rails and You
Back to all guides
A brief guide on API Mass Assignment Vulnerability
Tue May 24 2022

API vulnerabilities are a common thing that can break down your whole system if not patched. Hackers can leverage them to add additional code to your app or get access to your database. This can turn into a huge fiasco real quick. So it is always a good idea to put additional measures.
According to the Open Web Application Security Project (OWASP), there are ten API vulnerabilities that should be taken care of when you build an API. In this piece, let’s look at one of them.
API Mass Assignment
It is a severe API threat that arises when you save the request body as it is on the server instead of getting values from it one by one. It allows the user to initialize or overwrite server-side variables that the application does not intend.
Generally, it is easy to spread an object to create its copy and save it in the database, but this practice should be avoided. It is because if someone figures out the request payload, they can send more key values that can alter their presence on the web application.
A more appropriate way to do it would be to create a new object on the server-side by extracting only the fields you need from the request body and saving that object.
How To Prevent It?
You can prevent API mass assignment in multiple ways.
- As described earlier, you should not explicitly bind incoming data and internet objects because the user can send more data than required.
- Write a schema to define all the types and patterns you will accept in the request and then implement it on runtime.
- You should set the read-only property to true for all the fields that can be retrieved from the API request body but should not be modified by the user.
- You should also explicitly define the request body and query parameters you are expecting from an API request.
Continuously monitor web applications for vulnerabilities at scale with Cobalt Dynamic Application Security Testing (DAST).
- Application Pentest
- Secure Code Review
- Threat Modeling
- Network Pentest
- Red Teaming
- Digital Risk Management
- Social Engineering
- Device Hardening
- IoT Testing
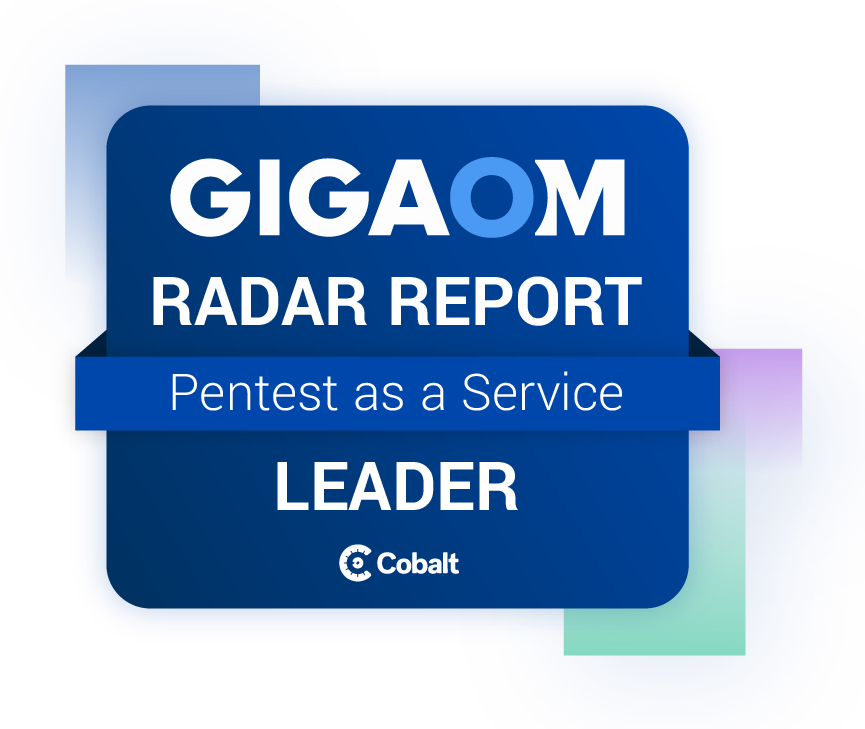
- get started

Mass Assignment & APIs - Exploitation in the Wild
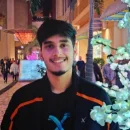
The APIs (Application Programmable Interfaces) are widely used to power applications, and one of the popular choices for implementing API is REST APIs . With this increase in popularity and usage, many security risks also come into the picture.
APIs have their own OWASP API Top 10 list, which describes the vulnerabilities commonly found in the APIs, including Mass Assignment.
This blog will dive deeply into understanding and exploiting mass assignment vulnerabilities.
Mass Assignment - A 20ft Overview:
Modern frameworks allow developers a convenient mass assignment functionality that lets developers directly take a “user-supplied Key-Value Pair” input to the object database. This reduces the requirement of writing code for such custom Key-Value pairs and increases the development efficiency but at the cost of security risks if not implemented correctly.
A mass assignment without a whitelist of allowed “Key-Value Pairs” could allow an attacker to use arbitrary values to create or update the resources abusing the applications’ regular workflow. Privilege escalation is one of the most common vulnerabilities arising from Mass Assignment vulnerability.
According to OWASPthis vulnerability depends on the language/framework in question can have several alternative names:
Mass Assignment: Ruby on Rails, NodeJS.
Autobinding: Spring MVC, ASP NET MVC.
Object injection: PHP.
For example, consider an API that allows users to update their profile information. The API may accept a JSON payload that contains multiple fields such as name, email, and address. Without proper validation, an attacker can add additional fields such as "isAdmin":true” or "isSuperUser":true and gain elevated privileges as an admin or superuser.
Let’s understand this attack further with the help of a vulnerable code snippet as described below:
const express = require('express');
const app = express();
app.post('/users', (req, res) => {
const newUser = {
username: req.body.username,
password: req.body.password,
isAdmin: req.body.isAdmin
};
// Save new user to database
app.listen(3000, () => {
console.log('Server started on port 3000');
In the above code, the “newUser” object is created from the request body without validation or filtering. An attacker can attempt to craft a request with an additional field named “isAdmin”:true and send it to the server to escalate the privileges.
To remotely exploit this issue, an attacker can send a POST request with an additional "isAdmin" field set to "true" to register as an administrator. In this case, isAdmin is an optional body parameter.
POST /users HTTP/1.1
Host: example.com
Content-Type: application/json
"username": "attacker",
"password": "password",
"isAdmin": true
Now, to mitigate this issue, simply adding a check to ensure that only the user with an admin session can trigger this parameter will fix the underlying vulnerability as described in below code:
Const port = 3000;
password: req.body.password
if (req.user.isAdmin && req.body.isAdmin) {
// Only admins can set isAdmin field
newUser.isAdmin = req.body.isAdmin;
app.listen(port, () => {
console.log(`Server started on port {port}`);
Hunting for Mass Assignment Attack in the Wild - A Practical Approach
Mass Assignment is not necessarily to be found in the user profile to perform privilege escalations. You can find it on any API endpoint, which could be using a parameter of interest to the attacker, causing significant damage to the application and its user’s reputation.
Note: Always read the API documentation to understand and identify interesting parameters/key-value pairs that could cause significant impact.
Let’s understand how to approach the Mass Assignment Attack in a black-box/grey-box assessment with the help of the “crAPI” Demo Lab.
Locally set up the crAPI demo lab .
Navigate to the shop - http://127.0.0.1:8888/shop
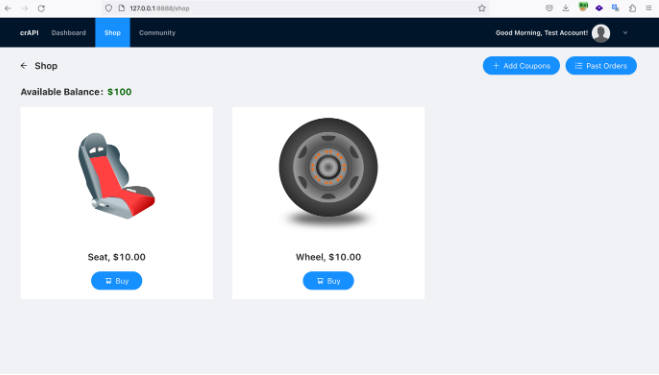
Note that the Available Balance by default is $100, and now Buy any item while capturing the request in Burp Suite or another proxy tool.
Send the Request to the repeater for later use.
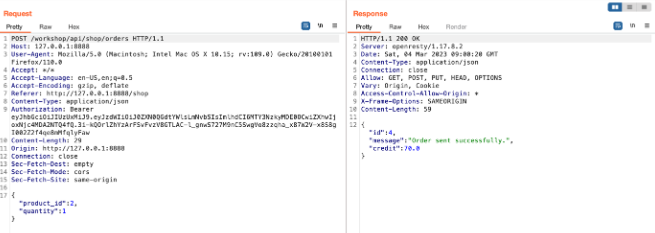
Observe after purchasing the items; Available Balance is changed.
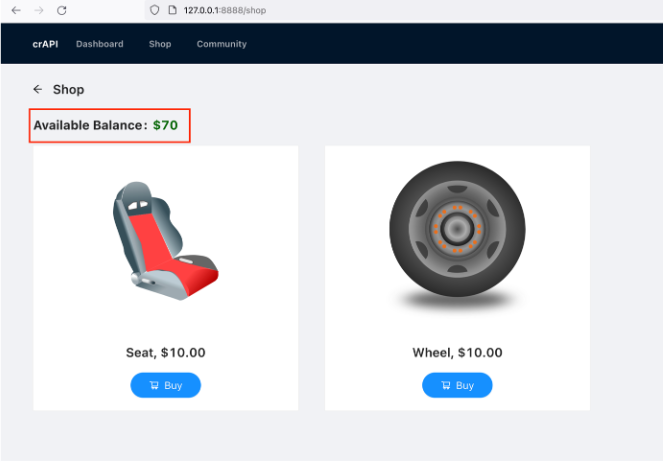
In the repeater tab, modify the request by changing the request method to GET and adding “/all” route to retrieve the information of all orders.
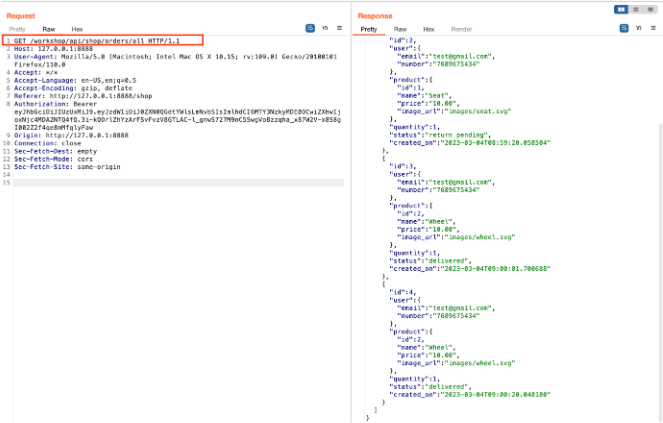
Observe that the application has returned all information about past orders.
Modify the request, and change the “all” to any random order ID.
Send the request, and observe the methods allowed and the order status.
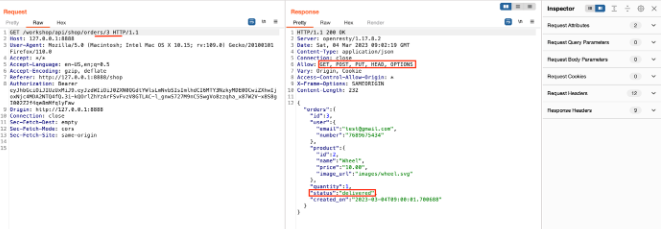
Again, modify the request by changing the request method to PUT and adding the status as a return.
Send the request and observe the error message in the response.
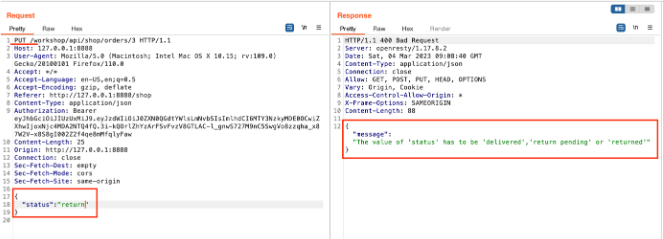
Send the request again by adding the status as returned and observing that the order status has changed to returned.
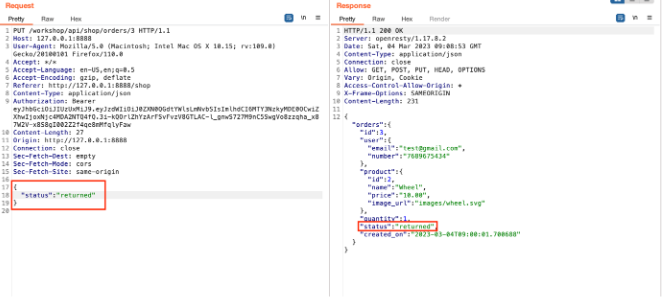
Navigate to the shop, and observe that credit transfers to the account.
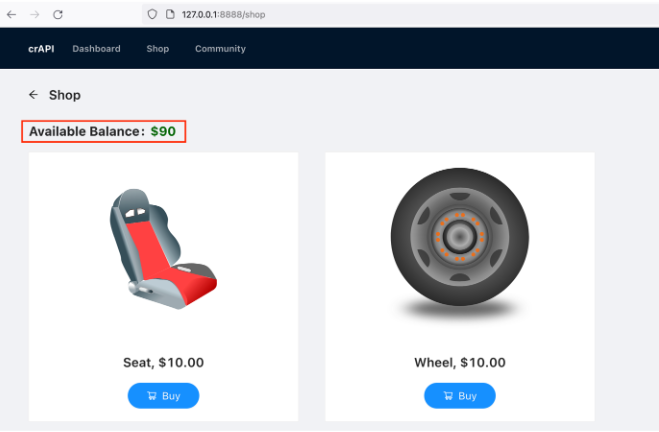
In the above lab scenario, as an attacker, it was possible to mark a delivered item as returned to get the cashback allowing an attacker to financially abuse the application with the help of a mass assignment attack.
Since now you know what chaos this attack can bring to the organization from the user integrity and the financial aspects, it is essential to understand how to implement a fix to prevent such attacks.
Fixing Mass Assignment - Remediation Approach
Some common ways to fix mass assignment issues include:
- Disable Automatic Property Mapping: Ensure that your applications have the automatic mapping disabled and always map the properties manually.
- Read-Only Key-Value Pairs: Ensure to set the fields retrieved from the “request body” that is not present in the “request body” should be read-only, and a user should not be allowed to tamper them.
You can find a detailed remediation guide here .
References and Further Reads
https://cheatsheetseries.owasp.org/cheatsheets/Mass_Assignment_Cheat_Sheet.html
https://www.impart.security/post/mass-assignment-101
https://crashtest-security.com/api-mass-assignment/
https://www.wallarm.com/what/mass-assignment
About Harsh Bothra
Related resources.
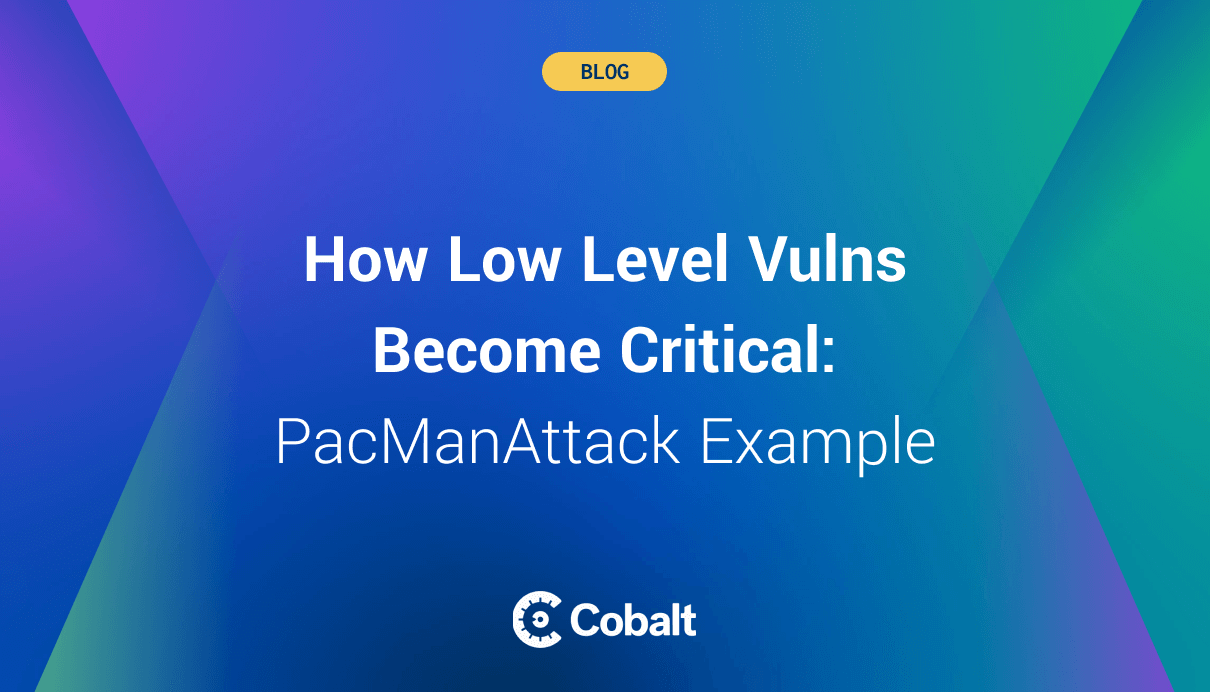
How Low Severity Vulns Become Critical: PACMAN Attack Example
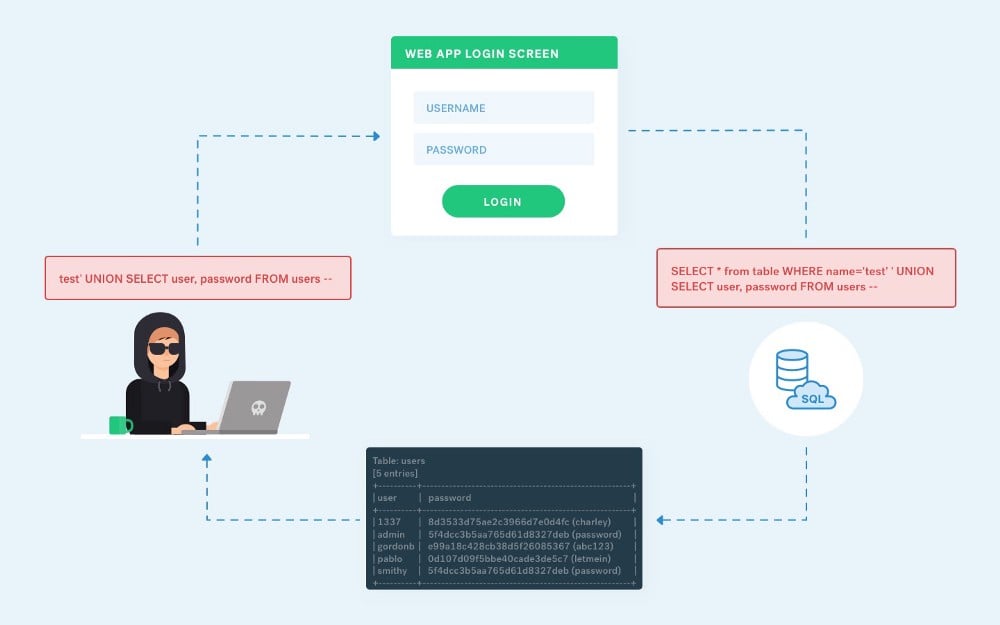
A Pentester’s Guide to SQL Injection (SQLi)
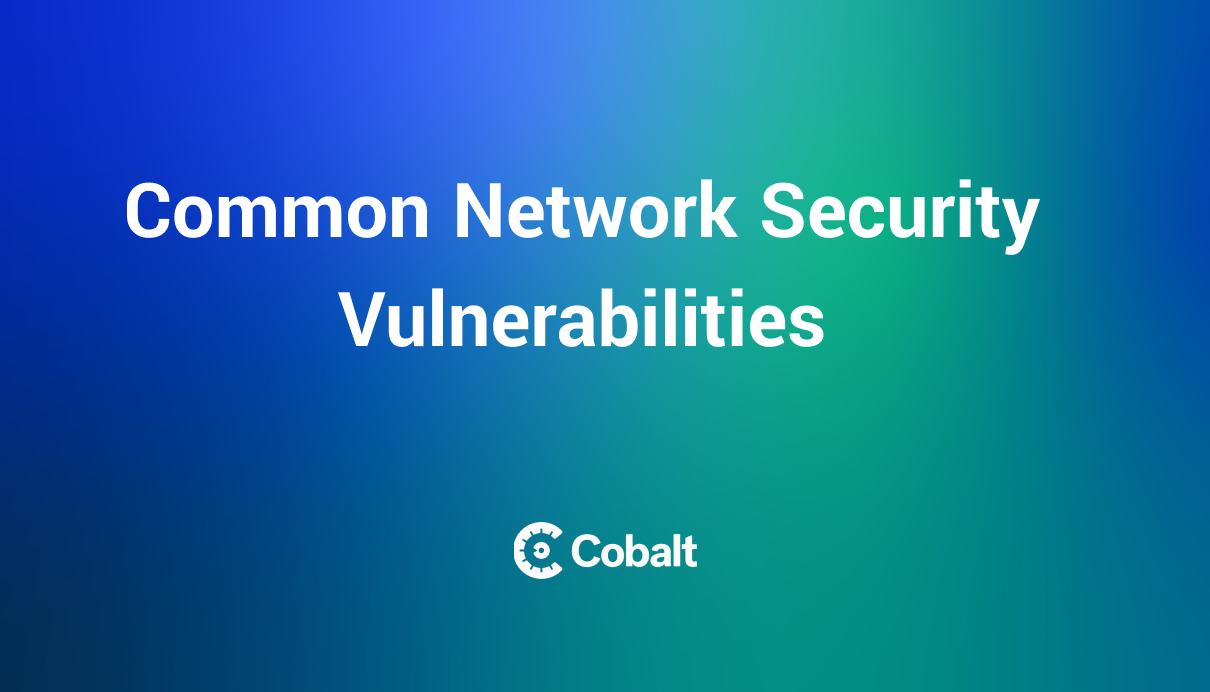
Common Network Security Vulnerabilities
Never miss a story.
- schedule a demo
- Cobalt Platform
- Offensive Security
- Application Security
- Network Security
- Cloud Security
- Brand Protection
- Device Security
- Core Community
- Product Documentation
- Resource Library
- Events & Webinars
- Vulnerability Wiki
- Trust Center

This is a title

- © 2024 Cobalt
- Terms of use
- Your privacy settings
- Do not sell my data
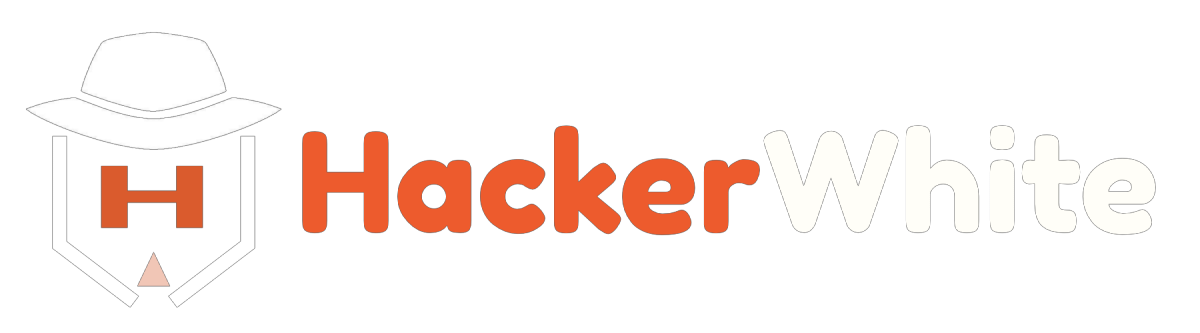
Point of Contact
Mass assignment vulnerability: understanding & mitigating the risks in api.
Mass assignment vulnerability is a critical security concern that often goes unnoticed in API development. Understanding the risks associated with this vulnerability is crucial for protecting sensitive user data. In this article, we will delve into the details of mass assignment vulnerabilities and explore effective mitigation strategies.
- 10+ Years of Experience
- 120+ Assessments Completed
- 800+ Vulnerabilities Uncovered
Start a 10-day Trial at just $1,000
- Vulnerability 101
Table of Contents
Introduction:.
The "Mass Assignment" vulnerability is a security flaw that occurs when an application assigns user input directly to model attributes without proper validation or sanitization. This can lead to unauthorized access and modification of sensitive data, potentially compromising the security of the application and its users.
Addressing the "Mass Assignment" vulnerability is crucial for developers as it can have serious consequences, including data breaches, unauthorized access, and legal implications. Understanding and mitigating this vulnerability is essential to ensure the integrity and security of an application.
Understanding the "Mass Assignment" Vulnerability:
The "Mass Assignment" vulnerability occurs when an attacker is able to manipulate the values of model attributes by submitting unexpected or malicious data. This can happen when developers use frameworks or libraries that automatically map user input to object properties without proper validation or filtering.
Common scenarios where developers may unintentionally introduce the "Mass Assignment" vulnerability include:
- Using frameworks or libraries that provide automatic mapping of user input to object properties without considering the security implications.
- Allowing users to submit data that directly maps to sensitive attributes without proper validation.
- Failing to implement proper input validation and sanitization techniques.
The impact of the "Mass Assignment" vulnerability can be severe. Attackers can exploit this vulnerability to gain unauthorized access to sensitive data, modify user privileges, or even execute arbitrary code on the server. This can lead to data breaches, compromised user accounts, and potential legal issues.
Common Examples of "Mass Assignment":
There are several common examples of the "Mass Assignment" vulnerability. Let's explore a few of them:
User Profile Update: Suppose an application allows users to update their profile information, including their email address and password. If the application blindly maps user input to the corresponding model attributes without proper validation, an attacker can manipulate the request to update other sensitive fields such as admin privileges.
Role-Based Access Control: In applications with role-based access control, developers often use a single parameter to assign roles to users. If this parameter is not properly validated, an attacker can modify it to gain unauthorized access to sensitive functionality or elevate their privileges.
API Endpoints: APIs that accept JSON or XML payloads are also susceptible to the "Mass Assignment" vulnerability. If the API endpoint maps the incoming request directly to model attributes without proper validation, an attacker can manipulate the payload to modify sensitive data or gain unauthorized access.
These examples highlight the importance of implementing proper validation and sanitization techniques to mitigate the risks associated with the "Mass Assignment" vulnerability.
Risks and Consequences:
The "Mass Assignment" vulnerability poses significant risks and consequences for both developers and users. Some of the potential risks and consequences include:
Data Breaches: Exploiting the "Mass Assignment" vulnerability can lead to unauthorized access to sensitive data, including personal information, financial records, and confidential business data. This can result in serious privacy breaches and financial losses.
Unauthorized Access and Privilege Escalation: Attackers can manipulate the values of model attributes to gain unauthorized access to restricted functionality or elevate their privileges within the application. This can lead to unauthorized actions, such as modifying critical settings, accessing sensitive data, or impersonating other users.
Reputation Damage: Security breaches resulting from the "Mass Assignment" vulnerability can severely damage the reputation of the application and its developers. Users lose trust in the application's ability to protect their data, leading to a loss of user base and potential legal consequences.
Legal Implications: Depending on the nature of the application and the data involved, security breaches resulting from the "Mass Assignment" vulnerability can have legal implications. Developers may face legal actions, regulatory fines, and potential lawsuits for failing to protect user data adequately.
Real-world examples of security breaches resulting from the "Mass Assignment" vulnerability include the 2012 GitHub incident, where an attacker exploited the vulnerability to gain administrative access to repositories. This incident highlighted the severity and impact of this vulnerability.
Best Practices for Mitigating the "Mass Assignment" Vulnerability:
To mitigate the risks associated with the "Mass Assignment" vulnerability, developers should follow these best practices:
Whitelist Input Validation: Developers should implement strong input validation techniques to ensure that only expected and valid data is accepted. This includes whitelisting allowed attributes and rejecting any unexpected or malicious input.
Use Role-Based Access Control (RBAC): Implement RBAC to control user privileges and access to sensitive functionality. Do not rely solely on user input to determine roles and permissions.
Implement Attribute-Level Access Controls: Instead of blindly mapping all user input to corresponding attributes, developers should implement attribute-level access controls. This ensures that only authorized users can modify specific attributes.
Sanitize and Filter User Input: Before assigning user input to model attributes, developers should sanitize and filter the data to remove any potential malicious content. This includes validating data types, length restrictions, and ensuring data integrity.
Implement Secure Coding Practices: Follow secure coding practices, such as avoiding dynamic attribute assignment, using strong encryption for sensitive data, and regularly updating frameworks and libraries to their latest secure versions.
Regular Security Testing and Auditing: Conduct regular security testing and auditing of the application to identify and mitigate any vulnerabilities, including the "Mass Assignment" vulnerability. This includes penetration testing, code review, and vulnerability scanning.
Tools and Resources:
To aid developers in addressing the "Mass Assignment" vulnerability, the following tools, libraries, and resources can be helpful:
OWASP Cheat Sheet - Mass Assignment: The OWASP Cheat Sheet provides guidelines and recommendations for securing web applications against the "Mass Assignment" vulnerability. It offers practical advice and code snippets for developers to implement secure coding practices.
Security-Focused Libraries and Frameworks: Many programming languages and frameworks provide security-focused libraries and modules that can help mitigate the "Mass Assignment" vulnerability. Examples include Django's ModelForm, Laravel's Mass Assignment Protection, and Ruby on Rails' Strong Parameters.
Platform-Specific Security Guidelines: Developers should refer to platform-specific security guidelines and resources provided by the framework or platform they are using. These guidelines often include best practices and recommendations for securing applications against common vulnerabilities, including "Mass Assignment."
Code Review and Testing Tools: Developers should leverage code review and testing tools to identify and mitigate the "Mass Assignment" vulnerability. Tools like SonarQube, OWASP ZAP, and Burp Suite can help identify security flaws in the code and test the application for vulnerabilities.
The Role of Security Testing and Auditing:
Regular security testing and auditing play a crucial role in identifying and mitigating the "Mass Assignment" vulnerability. Various testing techniques can be employed, including:
Penetration Testing: Conducting penetration tests can help identify vulnerabilities and potential attack vectors, including the "Mass Assignment" vulnerability. Ethical hackers simulate real-world attacks to identify security weaknesses and provide recommendations for improvement.
Code Review: Manual code review or automated tools can help identify insecure coding practices, including instances of the "Mass Assignment" vulnerability. Developers should review their code regularly and ensure it follows best practices for secure coding.
Vulnerability Scanning: Automated vulnerability scanning tools can scan the application for known vulnerabilities, including the "Mass Assignment" vulnerability. These tools can help identify potential weaknesses and provide guidance on how to address them.
By employing these testing techniques, developers can proactively identify and mitigate the "Mass Assignment" vulnerability, ensuring the security and integrity of their applications.
Conclusion:
Addressing the "Mass Assignment" vulnerability is crucial for developers to protect the integrity and security of their applications. By understanding the definition, risks, and consequences of the vulnerability, developers can take proactive measures to mitigate its impact.
Implementing best practices, such as whitelisting input validation, utilizing role-based access control, and regular security testing and auditing, can significantly reduce the risks associated with the "Mass Assignment" vulnerability.
Secured High Growth Companies Worldwide
Let's find out if we are a good fit with a 30-min intro call
Plans start from $1,000. No Contracts, Cancel Anytime.
Mass Assignment
Introduction.
Software frameworks sometime allow developers to automatically bind HTTP request parameters into program code variables or objects to make using that framework easier on developers. This can sometimes cause harm.
Attackers can sometimes use this methodology to create new parameters that the developer never intended which in turn creates or overwrites new variable or objects in program code that was not intended.
This is called a Mass Assignment vulnerability.
Alternative Names
Depending on the language/framework in question, this vulnerability can have several alternative names :
- Mass Assignment: Ruby on Rails, NodeJS.
- Autobinding: Spring MVC, ASP NET MVC.
- Object injection: PHP.
Suppose there is a form for editing a user's account information:
Here is the object that the form is binding to:
Here is the controller handling the request:
Here is the typical request:
And here is the exploit in which we set the value of the attribute isAdmin of the instance of the class User :
Exploitability
This functionality becomes exploitable when:
- Attacker can guess common sensitive fields.
- Attacker has access to source code and can review the models for sensitive fields.
- AND the object with sensitive fields has an empty constructor.
GitHub case study
In 2012, GitHub was hacked using mass assignment. A user was able to upload his public key to any organization and thus make any subsequent changes in their repositories. GitHub's Blog Post .
- Whitelist the bindable, non-sensitive fields.
- Blacklist the non-bindable, sensitive fields.
- Use Data Transfer Objects (DTOs).
General Solutions
An architectural approach is to create Data Transfer Objects and avoid binding input directly to domain objects. Only the fields that are meant to be editable by the user are included in the DTO.
Language & Framework specific solutions
Whitelisting.
Take a look here for the documentation.
Blacklisting
Nodejs + mongoose, ruby on rails, php laravel + eloquent, jackson (json object mapper).
Take a look here and here for the documentation.
GSON (JSON Object Mapper)
Take a look here and here for the document.
JSON-Lib (JSON Object Mapper)
Flexjson (json object mapper), references and future reading.
- Mass Assignment, Rails and You
Authors and Primary Editors
Abashkin Anton - [email protected]
results matching " "
No results matching " ".
Burp Scanner
Burp Suite's web vulnerability scanner
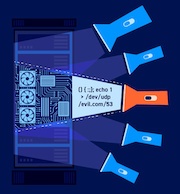
Product comparison
What's the difference between Pro and Enterprise Edition?
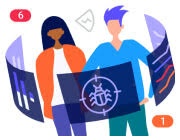
Download the latest version of Burp Suite.
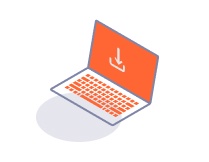
- Web Security Academy
- API testing
Lab: Exploiting a mass assignment vulnerability
PRACTITIONER
To solve the lab, find and exploit a mass assignment vulnerability to buy a Lightweight l33t Leather Jacket . You can log in to your own account using the following credentials: wiener:peter .
Required knowledge
To solve this lab, you'll need to know:
- What mass assignment is.
- Why mass assignment may result in hidden parameters.
- How to identify hidden parameters.
- How to exploit mass assignment vulnerabilities.
These points are covered in our API Testing Academy topic.
Launching labs may take some time, please hold on while we build your environment.
In Burp's browser, log in to the application using the credentials wiener:peter .
Click on the Lightweight "l33t" Leather Jacket product and add it to your basket.
Go to your basket and click Place order . Notice that you don't have enough credit for the purchase.
In Proxy > HTTP history , notice both the GET and POST API requests for /api/checkout .
Notice that the response to the GET request contains the same JSON structure as the POST request. Observe that the JSON structure in the GET response includes a chosen_discount parameter, which is not present in the POST request.
Right-click the POST /api/checkout request and select Send to Repeater .
In Repeater, add the chosen_discount parameter to the request. The JSON should look like the following:
{ "chosen_discount":{ "percentage":0 }, "chosen_products":[ { "product_id":"1", "quantity":1 } ] }
Send the request. Notice that adding the chosen_discount parameter doesn't cause an error.
Change the chosen_discount value to the string "x" , then send the request. Observe that this results in an error message as the parameter value isn't a number. This may indicate that the user input is being processed.
Change the chosen_discount percentage to 100 , then send the request to solve the lab.
Register for free to track your learning progress
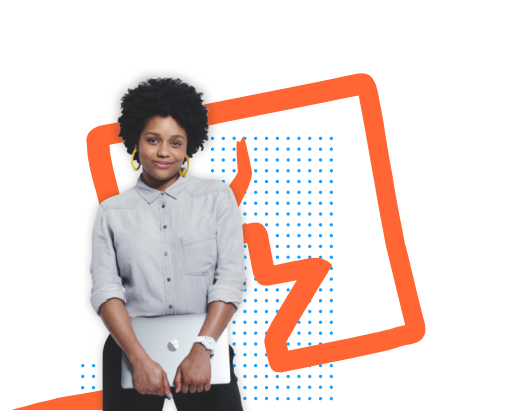
Practise exploiting vulnerabilities on realistic targets.
Record your progression from Apprentice to Expert.
See where you rank in our Hall of Fame.
Already got an account? Login here
Test APIs for vulnerabilities using Burp Suite
- Knowledge Base
- Pentest services
Application Penetration Testing
- Cross-domain Referer Leakage
- Pentesting Basic Authentication
- Username Enumeration
- iOS Frida Objection Pentesting Cheat Sheet
- URL Redirection – Attack and Defense
- Jailbreaking iOS 13 with unc0ver
- X-Runtime Header Timing Attacks
- wkhtmltopdf File Inclusion Vulnerability
API Mass Assignment Vulnerability
- Web Server TRACE Enabled
AWS Pentesting
- HTTP Request Smuggling (AWS)
- Create an AWS Read-Only Access Token
- ScoutSuite Quickstart
- Protecting S3 buckets using IAM and KMS
- Misconfigured S3 Bucket
- S3 Storage Does Not Require Authentication
DevOps Security
- Securing Travis CI
- SSH Weak Key Exchange Algorithms Enabled
- SSH Weak MAC Algorithms Enabled
- TLS 1.0 Initialization Vector Implementation Information Disclosure Vulnerability
- OpenSSL ‘ChangeCipherSpec’ (CCS) MiTM Vulnerability
- Null Ciphers Supported
- ‘Export Ciphers’ Enabled
Network Penetration Testing
- F5 BIG-IP Cookie Remote Information Disclosure
- DNS Server Dynamic Update Record Injection
- rlogin Service Enabled
- Unauthenticated MongoDB – Attack and Defense
- SNMP ‘GETBULK’ Denial of Service
- Responder / MultiRelay Pentesting Cheatsheet
- NTP Mode 6 Vulnerabilities
- Cisco Information Disclosure (CVE-2014-3398 – CSCuq65542)
- SSH Tunneling for Pentesters
- .NET Handler Enumeration
- TLS_FALLBACK_SCSV Not Supported
- PHP Easter Eggs Enabled
- MySQL Multiple Vulnerabilities
- Debian Predictable Random Number Generator Weakness
- Cisco IKE Fragmentation Vulnerability
Pentesting Fundamentals
- GET vs POST
- Cache Controls Explained
- Cookie Security Attributes
- Essential Wireshark Skills for Pentesting
- Testing Cookie Based Session Management
Windows Hardening
- Resolving “Windows NetBIOS / SMB Remote Host Information Disclosure” (2020)
Home » API Mass Assignment Vulnerability
Table of Contents
- 1. API Mass Assignment
API Mass Assignment
Mass assignment vulnerabilites occur when a user is able to initialize or overwrite server-side variables for which are not intended by the application. By manually crafting a request to include additional parameters in a request, a malicious user may adversely affect application functionality.
Common root causes of mass assignment vulnerabilities may include the following:
Framework-level “autobinding” features. Spring and .NET MVC are two of many frameworks that allow HTTP parameters to be directly mapped to model objects. While this feature is useful to easily set server-side values, it does not prevent arbitrary parameters from being injected.
- https://agrrrdog.blogspot.com/2017/03/autobinding-vulns-and-spring-mvc.html
Parsing a request body as an object. Although copying an object is easier than selecting numerous individual values within that object, this practice should be avoided. When using data formats such as JSON, developers should only extract values that are intended to be modified by users.
Below shows a common example of this vulnerability in a user registration endpoint: POST /api/register HTTP/1.1 [..] {“email”:”[email protected]”}
HTTP/1.1 200 OK [..] {”userid”:”112345”,“email”:”[email protected]”,”email_verified”:false}
A malicious user may want to bypass email verification for a number of reasons. To attack this endpoint, a value is inserted into the request body:
POST /api/register HTTP/1.1 [..] {“email”:”[email protected]”,”email_verified”:true}
HTTP/1.1 200 OK [..] {”userid”:”112346”,“email”:”[email protected]”,”email_verified”:true}
Developers should also ensure that values do not include nested objects or arrays which may undermine application logic. Below shows another style of attack leveraging JSON arrays:
POST /api/register HTTP/1.1 [..] {“email”:[”[email protected]”,”[email protected]”]}
HTTP/1.1 200 OK [..] {”userid”:”112347”,“email”:[”[email protected]”,”[email protected]”],”email_verified”:false}
In the above example an array is provided where a single string value was expected. This would likely have significant security implications for account access and associations.
- Application
- Share this article:
API6:2019 — Mass assignment
The API takes data that client provides and stores it without proper filtering for whitelisted properties. Attackers can try to guess object properties or provide additional object properties in their requests, read the documentation, or check out API endpoints for clues where to find the openings to modify properties they are not supposed to on the data objects stored in the backend.
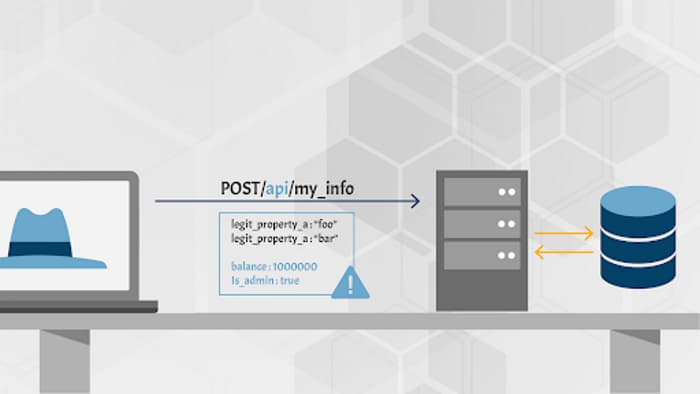
- The API works with the data structures without proper filtering.
- NodeJS: var user = new User(req.body); user.save();
- Rails: @user = User.new(params[:user])
- Attackers can guess the fields by looking at the GET request data.
How to prevent
- Do not automatically bind incoming data and internal objects.
- Explicitly define all the parameters and payloads you are expecting.
- Use the readOnly property set to true in object schemas for all properties that can be retrieved through APIs but should never be modified.
- Precisely define the schemas, types, and patterns you will accept in requests at design time and enforce them at runtime.
Copyright 42Crunch 2021
Test Library
Search for API Security Tests
API Security
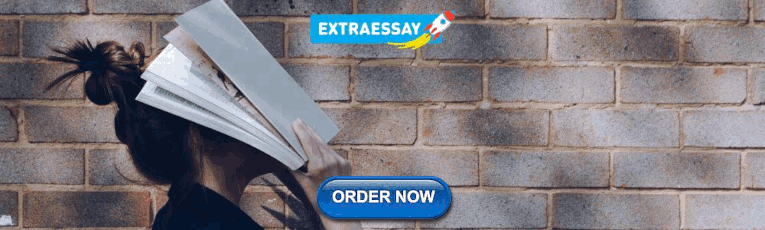
How to Test Mass Assignment in APIs using Akto
This blog is about learning mass assignment vulnerability, how to find it manually, how to test for it using Akto and finally how to prevent it.

In 2017, Equifax, an American multinational consumer credit reporting agency, experienced a data breach . Hackers exploited a vulnerability in Equifax's web application that allowed them to access sensitive personal information of millions of customers. The vulnerability was caused by an unpatched version of Apache Struts, a popular open-source framework used for building web applications. The hackers were able to exploit the vulnerability to gain access to sensitive data by modifying the values of certain parameters using a technique called mass assignment . The result was a massive data breach that compromised the personal information of millions of people, causing widespread damage and leading to numerous lawsuits and investigations.
This highlights the importance of properly securing APIs, particularly from mass assignment vulnerabilities, through regular testing and validation of user input, as well as limiting the properties that can be modified through user input. In this blog, we will cover the following:
1. What is Mass Assignment?
2. How to find Mass Assignment Vulnerability?
3. Automation with Akto
4. How to prevent mass assignment?
What is Mass Assignment?
Mass Assignment vulnerability is a security flaw that can occur in API when user input is directly used to modify the properties of an object. This can allow attackers to modify data and perform unauthorized actions on an application. To prevent this vulnerability, it is important to validate and sanitize user input, and to limit the properties that can be modified through user input. Mass Assignment is one of the OWASP Top 10 API vulnerabilities. Therefore, it is crucial to test for it from a security standpoint.
OWASP API Top 10: https://owasp.org/www-project-api-security/
How to find Mass Assignment Vulnerability?
The most effective method to discover mass assignment vulnerabilities in an API endpoint is by analyzing an it’s requests and responses. The recommended tool for this task is a web application scanner, such as BurpSuite.
Steps to find APIs with potential Mass Assignment vulnerability:
1. Turn on the Burp Suite proxy and start visiting every endpoint in a web application. Focus on endpoints that allow a user to create resources into the application such as creating account, updating, creating wallet or inviting a user.
2. Once you have added the target to the scope in BurpSuite, try to interact with the application in a variety of ways. This could include submitting different types of input or performing different actions within the application.
3. If you encounter any parameters or variables in the response that are being assigned to the user, take note of them. These variables can often be manipulated to gain access to sensitive data or functionality. For example, variables like " role:authenticated ", " role:customer ", " balance:0 ", " timestamp:XX ", " user_id:XXX ", etc.
4. Additionally, make sure to explore all of the functionality of the application, including any areas that may not be immediately obvious. This can help you identify additional vulnerabilities that may not be apparent at first glance.
Let's assume that, after account creation, you find a variable in the response such as " role:customer ". The server assigns this "role" variable to identify the user's role and assign privileges accordingly.
How to exploit manually?
To perform this test, follow the steps below:
1. Search for the request that assigns a role to the user on the server-side.
2. Once you have found the request, try modifying the JSON data of the request by changing the value of the variable that assigns the user's role (e.g., "admin").
3. Analyze the response from the server. If the response returns " role:admin ", it means that the variable has been successfully overwritten by the user.
4. Another way to exploit is to attempt various HTTP methods such as POST, PUT, and PATCH, and send the request.
5. To confirm the vulnerability, return to the application and check if there are any additional features or pages that you can now access. For example, you may be able to access a page that previously displayed a 403 error, or access the admin dashboard.
6. If you can access additional features or pages, it is likely that the application is vulnerable to a privilege escalation attack, and further investigation is required.
Test for Mass Assignment using the best proactive API Security product
Our customers love us for our proactive approach and world class API Security test templates. Try Akto's test library yourself in your testing playground. Play with the default test or add your own.
Start Testing
How much time does it take?
Finding and exploiting vulnerabilities in an API endpoint can take hours or even days to complete. The duration depends on the number of API endpoints and their complexity which is too cumbersome.
Automation with Akto
Each step I described above takes time and requires proper analysis of requests and responses. Doing so manually, could take hours or even days, depending on the complexity of the APIs. Just imagine doing this for thousands of APIs! It sounds difficult, right?
Akto can make this task easier for you by scanning thousands of endpoints with just one click!
If you have not yet installed Akto, you can do so from the Akto GitHub page along with the Akto extension in Burp Suite. For demonstration purposes, we will use OWASP Juice Shop .
Akto Burp Extension
There are multiple ways to create an API inventory in Akto that work in both the Community and Professional editions. You can do this by importing a .har file or forwarding traffic from BurpSuite.
Steps to Create API Inventory using Akto Extension:-
1. Run Akto in docker
2. Launch BurpSuite
3. Download Akto Burp extension. Check this out for setup.
4. Turn on burp proxy and browse the target application

While browsing, you will soon notice that the Akto extension captures many requests. To filter the requests you are interested in, simply left-click on the target request and choose "Use Request.path Value as Log Filter". This will automatically add a filter to the filter bar since the extension captures all requests from the proxy tab.

Open the Akto Dashboard, where you will be able to see that an API Inventory is created automatically with a name. The default name of the collection is " burp ," but it can be changed through the "options" menu in the Akto burp extension.
Exploitation: Run Test

Click on "Run Test". A "Configure Test" box will pop up, asking for the vulnerabilities you want to test for. Select and deselect as needed, then run the test. Afterward, move to the "Testing" tab to see the status of the test.
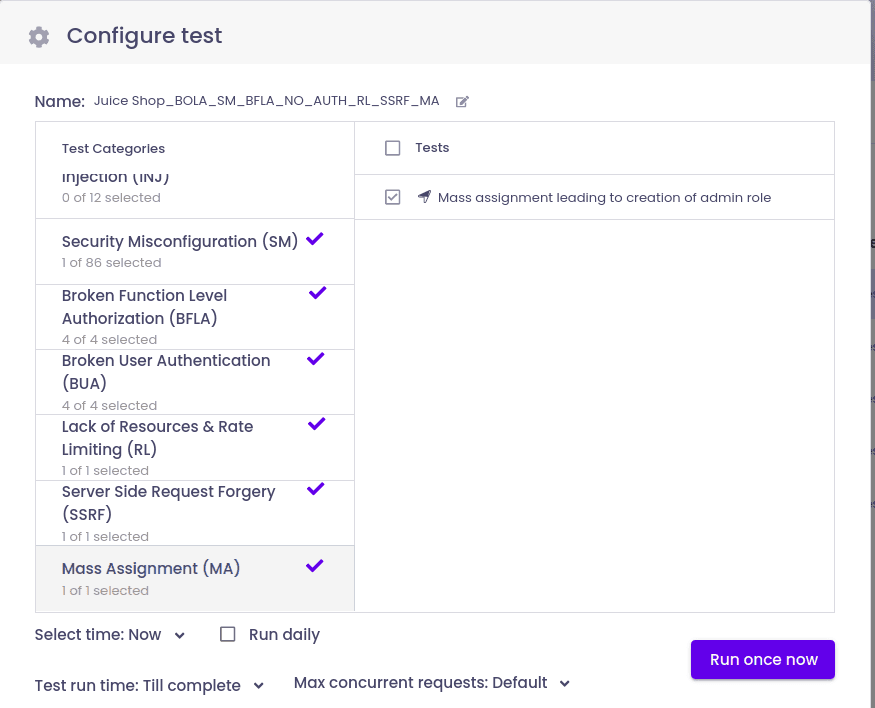
As you can see, I've selected Mass Assignment for my testing and left the other default settings as they are.
Findings: Test Results
Move to the Testing tab to see test results

Below, you can see a list of endpoints that were tested, along with their severity. Since I wanted to test for mass assignment, I selected "Mass Assignment" from the " Issue Category " tab to filter the endpoints that are vulnerable to mass assignment.
Clicking on the first request, I can see three options – “Description, Original, Attempt”.
Description: Information about the found vulnerability.
Original : Intended or normal client request and response.
Attempt : Akto attempt to exploit the vulnerability.

In the original tab request, we can see that the response says "role:customer". Therefore, this is the variable that the server is using to assign roles and privileges. In this case, it's a customer role with fewer privileges.

Now switch to the attempt option, the Akto engine was able to identify the variable used and hence tried assigning " role:admin " by sending a request to the server. The server updated the variable value to "admin," leading to a successful mass assignment exploitation. Now, any normal user can become an admin just by assigning a variable in the client request, which is a critical vulnerability We found it in just seconds.
When to use Burp vs Akto?
Both manual and automated testing are helpful for detecting vulnerabilities in an API endpoint. When you have just deployed a new API inventory, start by testing in Akto to cover all the popular mass assignment vulnerability attacks. After you finish testing, manually explore the critical APIs in Burp to find vulnerabilities you couldn’t find using Akto. This approach can help you save time and focus on critical endpoints.
How to Prevent it?
APIs take input inside the JSON body and set data according to it. Therefore, it is vulnerable to injection attacks. The most important step is input sanitization to prevent such attacks. Also, here are some additional points to keep in mind while writing code.
Limit the properties that can be modified by the user. It can be done by ensuring that the payload meets the defined schema, and rejecting any payload that does not.
Whitelist or blacklist to specify which properties can or cannot be updated by the client. If your system allows it, try making properties read-only using the @read-only annotation.
If possible, use a separate API endpoint for admin functionality instead of using parameters to assign roles to the user in the same API endpoint.
Try to avoid using functions like unserialize() in PHP applications.
When using ASP.NET Core or Apache Structs, automatic binding of request parameters into objects can sometimes cause issues. In such cases, use the [Bind] attribute model to select only bindable attributes.
Provide explicit definitions for all the parameters that the server is expecting, as well as those that it is not expecting.
Getting Started
Start your API testing with Akto. You can download it from the GitHub page and follow the instructions for a successful installation. Also, don't forget the BurpSuite Akto extension, which you can download by following steps from here .
Looking forward to hearing from you. Please let us know if you have any ideas that you would like us to include.
Happy API security testing!
Follow us for more updates
Monthly product updates in your inbox. no spam., table of contents, keep reading.

Developer best practices
Top 34 Cyber security Certifications to Grow Your Career
This guide provides an overview of 34 of the most popular and respected cybersecurity certifications. We have organized them by career stage and specialism, so you can easily find the ones that are most relevant to you.

API security breaches
Roku Data Breach - 15000 Customers affected!
Roku revealed a data breach that affected more than 15,000 customers for unauthorized purchases of hardware and streaming subscriptions.

February Product News: Akto’s Istio Connector, Sensitive Data in URLs and more
This is the February product newsletter for Akto. This month, we launched some exciting features, including Akto’s Istio Traffic Connector, Sensitive Data Detection in URLs and more.
- Cloud login
OWASP API security – 6: Mass assignment
Publish on 27 May, 2022 - by Justin Fletcher Last updated: 27 Dec, 2023 --> Last updated: 27 Dec, 2023
Introduction
1: Broken object level authorisation
2: Broken user authentication
3: Excessive data exposure
4: Lack of resources & rate limiting
5: Broken function level Authorization
6: Mass assignment
7: Security misconfiguration
8: Injection
9: Improper assets management
10: Insufficient logging & monitoring
The mass assignment vulnerability is a lack of data input validation that allows an attacker to modify data or elevate privileges by manipulating payload data. In the case of an object database, for example, if the payload maps directly to the stored data and is inserted directly, without input validation compared against authorisation levels, then the attacker can craft a payload that alters data not intended to be altered.
To prevent against mass assignment APIs need to validate input against a blacklist or preferably a whitelist so that only the expected fields of the payload are evaluated, coupled with authorisation validation to ensure the client has the correct privileges on those fields. This ensures that data can only be modified by a client that is granted the rights to modify that data.
OWASP summary
Source: OWASP mass assignment
APIM context
While, in general, mass assignment is an API issue to solve an APIM can facilitate the process. Validation of the payload through, for example, a JSON schema ensures that the payload contains only the expected fields.
Additionally an APIM can validate authentication and authorisation by scope. This ensures that the client has the correct credentials before the API processes the request.
Tyk vs Apollo
Powerful security
Do you always want to redeploy the whole stack if you change a security policy? What if you need the flexibility to expose the same API to different groups of users? How do you model this with a single annotated schema?
Tyk approach
Payload validation can be implemented in various ways with the Tyk APIM.
- JSON schema validation to ensure the payload meets the defined schema, and rejects payloads that do not.
- Body transformation allows using string template syntax, which is a powerful tool for generating the desired output from the input.
- Custom plugins for more complex cases or logic not satisfied by the first 2, users can write custom plugins in a variety of languages, either directly or through gRPC calls, to implement their requirements.
- Request method transformation while not directly a Mass Assignment prevention, it is a feature that can help ensure that APIs are called with the correct methods.
In addition to the technical solutions mentioned above, Tyk also recommends considering splitting Admin APIs from client facing APIs. By having the admin usage of APIs separate from client facing APIs then different policies, payload validation methods, authentication and authorisation checks in the APIM can be separated and managed under different governance models providing a clear split between the roles.
API Market & Industry Research API platform governance & optimisation Create, secure & test APIs Monitor, troubleshoot & update APIs
Share with your network
Privacy Overview
Search code, repositories, users, issues, pull requests...
Provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
How to prevent mass assignment in ASP.NET Core
One of the many security risks which you should consider is a mass assignment vulnerability ( cheatsheet ) also know as overposting. While it’s not in OWASP Top 10 it’s still considered important. Read on to understand the issue and find out possible ways of fixing it.
Mass assignment explained
ASP.NET Core allows automatic model binding of request parameters into variables or objects to make developers life easier. It can be as simple as binding id parameter via route:
It can also be more complicated, for example we can bind a bunch of GET parameters into custom model:
This behavior can be harmful. Attacker can guess parameter names and overwrite variables which should remain intact.
Let’s see how this works by example. Let’s assume that we have a simple web application where users can change login and password.
This is our database model:
This is the view with password change form:
And this is how our action looks like:
If the user found out that we’re having Role property, he could try to overwrite it and set it to “admin”.
Now, what would happen if attacker crafted a malformed request like this:
In this scenario our evil user could promote his account to admin, because there is nothing in our code to prevent that.
We may think that we’re safe because we haven’t included Role property in our form or we made it hidden, but it doesn’t prevent proper binding.
How to deal with it?
This vulnerability happens during model binding when someone is trying to create request in a way which allows overriding model properties.
There are a couple of security countermeasures to help us prevent mass assignment.
As we’ll see there are two groups of solutions for this problem. First is to manually specify which properties should or shouldn’t be binded (whitelisting/blacklisting) using data annotations. Second is to add another layer to our application (view models).
The bad approach
While you could technically fix this problem by manually nulling/zeroing all other variables, you shouln’t follow this way. It’s error prone, verbose and we’ve better solutions available out of the box.
So I strongly advise against doing something like this:
BindAttribute
One way to prevent binding of unwated properties is to use [Bind] attribute on model.
We can use it to pick only bindable properties:
Other model properties would simply be ignored, so even if someone posted Role property it wouldn’t be binded to model. We’re basically whitelisting properties here.
Unfortunately this solution uses magic strings, so if you decide to change your property name (e.g. Login to Username ) you will have to remember and change it manually.
Pretty error prone solution if you ask me, but since we have the nameof operator there is a way to make it a litte bit better (and more verbose):
EditableAttribute / BindNeverAttribute / ReadOnlyAtribute
Next possibility is to use one of property attributes which will prevent binding:
- [BindNever]
- [Editable(false)]
Those attributes has to be used directly on model fields like so:
Most appropriate in my opinion would be BindNever , the other one seems to not be appropriate in this case.
TryUpdateModelAsync
Controllers have a neat little method called TryUpdateModelAsync which helps us update only specified fields:
The drawback of this solution is that TryUpdateModelAsync is tied to controller, so if you (want to) have a separate database services layer it will cause some trouble.
Also you may dislike this solution because it tends to put too much database related operations into controller.
ViewModel / Data Transfer Object
Many would say that it’s best to provide additional layer which would be responsible for data exchange between controller and view.
Separation of concerns is a good idea, after all database model is not the same as view model.
Besides introducing data transfer objects / view models layer to our app can help us prevent mass assignment vulnerabilities.
This way we have view-models with minimum required properties which are mapped to EntityFramework models afterwards.
Providing additional layer has its own drawback which is extra work. It takes some time to create additional classes and view model to model mapping, but in return we’re having a better layered application.
We can use solutions like Automapper to help us map values from view-model to database model and back.
If you’re building an (JSON) API: attributes won’t work with [FromBody] !
Model binding using different formatters (JSON, XML, etc) won’t take standard attributes into account.
Simply model binding knows nothing about your serializer/deserializer, so regular attributes won’t apply.
You can try to check if your serializer has build-in attributes which could replace [BindNever] e.g. Json.NET has [JsonIgnore] which could be used to prevent binding.
What you can do in this case:
- use data attributes provided by your serializer/deserializer
- write custom binder which would take attributes into account
- use data transfer object approach.
In my opinion it’s best not to bind directly to database model. Personally I avoid attribute based solutions.
I prefer to have separate view-model layer and binding directly to entity models doesn’t appeal to me. In return we have separation of concerns and we’re hiding internal (database) data structures away from users.
Though most people would probably use attribute based approach for basic stuff and view-models for advanced apps.
Don’t forget that you may need different security measures when you’re accepting JSON (or other) formatted requests.
Author Zbigniew
LastMod 2018-03-02

LRQA Nettitude Command & Control Framework - Free and Open Source
Vulnerability research, regular vulnerability disclosures, red team training, learn to be the best, from the best, explaining mass assignment vulnerabilities.
Programming frameworks have gained popularity due to their ability to make software development easier than using the underlying language alone. However, when developers don’t fully understand how framework functionality can be abused by attackers, vulnerabilities are often introduced.
One commonly used framework feature is known as mass assignment, a technique designed to help match front end variables to their back end fields, for easy model updating.
Implementing mass assignment
We’ll be using PHP/Laravel as an example to demonstrate how mass assignment works via the Laravel framework. Let’s imagine you have a form which allows a user to update some of their profile details, and that form contains the following fields:
Within the Laravel controller, one way to update those fields would be as follows:
An alternative way to do this would be to take advantage of mass assignment, which would look something like this:
This code updates the User model with the values from the Request (in this case the HTML fields for name, email, address and phone) assuming that the input names match the models fields. This obviously saves superfluous lines of code, since all fields can be updated at once, instead of specifying individually.
The mass assignment vulnerability
So, how might an attacker exploit this?
As may be evident from the code above, the framework is taking all the input fields from the Request variable and updating the model without performing any kind of validation. Therefore, its trusting that all the fields provided are intended to be updateable.
Although the example currently only provides options for updating fields such as name and email, there are usually more columns in the User table which aren’t displayed on the front end. In this case, lets imagine that there is also a field named role , which determines the privilege of the user. The role field wasn’t displayed in the HTML form because the developers didn’t want users changing their own role.
However, with our understanding that the controller is simply trusting all input provided by the request to update the User model, an attacker can inject their own HTML into the page to add a field for role , simply by using built in browser tools. This can also be done by intercepting the request using a proxy and appending the field name and value, or by any other technique that allows client side modification.
This time, when the controller is reached, the user model will be updated with the expected fields (name, email, address, phone) as well as the additional role field provided. In this case, the vulnerability leads to privilege escalation.
This particular example demonstrates how mass assignment can be exploited to achieve privilege escalation, however it is often possible to bypass other controls using the same technique. For example, an application might prevent a username from being edited when updating profile information, to ensure integrity and accountability across audit trails. Using this attack, a user could perform malicious actions under the guise of one username before switching to another.
Countermeasures
There are several ways to protect against mass assignment attacks. Most frameworks provide defensive techniques such as those discussed in this section.
The general idea is to validate input before updating the model. The safest way to do this is to somewhat fall back to the original and more convoluted process of specifying each field individually. This also has the added benefit of providing the ability to add additional validation to each field beyond ensuring only expected fields are updated.
In Laravel, one way to do this would be as shown below; include some validation such as the maximum number of permissible characters for the name field, and then update the User model with the validated data. As the validate() function lists the exact fields expected, if the role field was appended as demonstrated in our previous sample attack, it would be ignored and have no effect.
An alternative method is to utilize allow lists and deny lists to explicitly state what fields can and cannot be mass assigned. In Laravel, this can be done by setting the $fillable property on the User model to state which fields may be updated in this way. The code below lists the four original fields from the HTML form, so if an attacker tried to append the role field, since its not in the $fillable allow list, it won’t be updated.
Similarly, deny lists can be used to specify which fields cannot be updated via mass assignment. In Laravel, this can be done using the $guarded property in the model instead. Using the following code would have the same effect as the above, since the role parameter has been deny listed.
Mass assignment vulnerabilities are important issues to check for during software development and during penetration tests because they are often not picked up by automated tools, due to them often having a logic component. For example, a tool will not likely have the context to understand if a user has managed to escalate their privilege after a specially crafted request.
They are also often overlooked by developers, partly due to lack of awareness for how certain features can be exploited, but also due to pressure to complete projects since its faster to use mass assignment without performing input validation.
It’s important to understand that mass assignment vulnerabilities exist and can be exploited with high impact. A strong software development lifecycle and associated testing regime will reduce the likelihood of these vulnerabilities appearing in code.
Share This Story, Choose Your Platform!
Related posts.
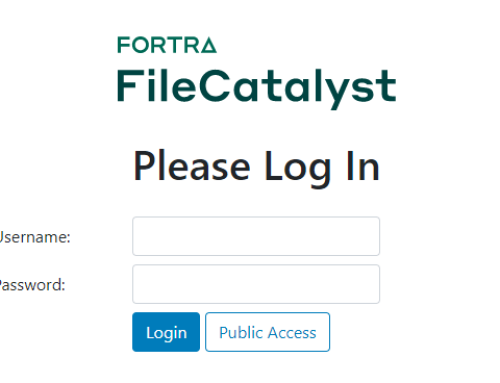
- Browse topics
Mass assignment
Be careful with parameters that are automatically bound from requests to objects, select your ecosystem, mass assignment: the basics, what are mass assignment vulnerabilities.
To make it easier to save data submitted via an HTML form into a database or object, many web application frameworks have included libraries to automatically bind HTTP request parameters (typically sent via forms) to the fields of database models or members of an object, requiring only minimal coding.
Let’s say we have a (very simple) HTML form:
When the form is submitted to the web application, it will send the form data as HTTP request parameters, and the backend code will have to read each parameter individually into a corresponding variable. Then, once all the fields have been read, the application will usually execute a database update or insert operation to save the data.
Mass Assignment makes it possible to write less code to handle this process - think about how much coding this technique could save if it was an object that had dozens of fields, and multiply this across a complex application that has many of these objects in its database.
Mass assignment vulnerabilities occur when the database model that is being assigned contains security-relevant fields, and the application user can supply values in the POST request that are saved to those fields, even though they are not present in the HTML form.
For example, if the User model contained a field isAdmin: Boolean , the user could add the POST body parameter isAdmin=true and make themselves an administrator.
For this to occur, an attacker would need to guess the names of the sensitive fields, or the source code for the vulnerable application would have to be available to the attacker (allowing them to see what sensitive fields are present in the data model).
Impacts of this attack can include bypassing authentication or authorization logic or elevation of privilege. This could then result in the destruction or disclosure of data within the application.
About this lesson
In this lesson, you will learn how mass assignment vulnerabilities work and how to protect your applications against them. We will begin by exploiting a Mass Assignment vulnerability in a simple application. Then we will analyze the vulnerable code and explore some options for remediation and prevention.
Mass assignment in the wild
In 2012, a GitHub user exploited a Mass Assignment vulnerability in GitHub’s public key update form. The flaw allowed the user to add their public key to another organization they were not a member of. The user added their key to the Ruby on Rails organization. To demonstrate proof of the exploit, the user added a file to the Rails project repository. GitHub responded, quickly fixing the vulnerability and they conducted a wide audit of their code to ensure the issue was detected and fixed if it existed anywhere else.
Mass assignment in action
New SaaS startup SuperCloudCRM recently launched their web platform designed to help businesses boost their sales and marketing efforts.
Setting the stage
SuperCloudCRM recently launched its web platform. Unfortunately, they suffered a security breach, resulting in data being leaked. What went wrong?
Mass assignment details
As mentioned, SuperCloudCRM’s developers had been logging request data for API endpoints like the POST /user/create endpoint, which creates new user accounts when a user submits the signup form.
A typical JSON payload in the request sent to the /user/create endpoint was supposed to look like this:
But a search of the /user/create endpoint’s logs for the [email protected] account around the time the user was created, found JSON POST data starting with the following excerpt:
It was different to the normal requests, and had a long request body with dozens more fields all starting with the letter r . What was the attacker doing? All of these weird field names that weren’t part of the user model schema, which was:
After doing some testing like the scenario above showed, a few things were discovered.
First, the new user account’s password was apparently being saved to the database in plaintext. Not good! But what stuck out was that the application ignored the non-existent fields and just assigned the fields that were actually part of the User model schema.
The data from the new User document was sent back to the API client and the attacker could then infer which of the list of fields starting with r were part of the User model schema, because if a field existed it was saved and echoed back in the response with the other user data.
A search of the /user/create endpoint’s request log entries around the same time revealed that thousands of similar requests had been sent. Each request testing lists of possible field names in the User model schema.
It was concluded that the attackers had brute-forced HTTP requests with various field name guesses to enumerate the organization and role fields in the schema. Despite them not being referred to anywhere in the client-side JavaScript code, the attackers were able to discover these security-related field names.
So, if the attackers knew these field names, what would they do then? Well, this could have led to a possible mass assignment attack. After hours of reviewing logs for the POST /user/create and POST /user/update endpoints the incident response team found dozens of requests had been submitted to the application, which looked similar to:
The requests appeared to be successful. Each of the requests changed the organization to a different customer, essentially giving the attackers access to each of them as admins. The last request was:
This seemed to explain why [email protected] was an administrator in the Cowmoo Industries organization.
By exploiting this mass assignment vulnerability and adding themselves as the administrator for various customers, the attackers were able to access the organizations’ data within SuperCloudCRM and steal it.
Mass assignment by different names
The concept of mass assignment is known by different names in various programming languages or frameworks. NodeJS and Ruby on Rails call it mass assignment. It is referred to as autobinding in Java Spring MVC and ASP NET MVC. PHP calls it object injection.
Mass assignment under the hood
Let’s have a look at this vulnerable application in more detail by going through the server-side code.
The schema for the User model is defined here, with the user’s credentials, email address, plus their role and organization they belong to. During signup, the credentials and email address are the only values that are supposed to be supplied by the user and accepted by the application.
Firstly, let's recap what took place in the example above.
- The User schema consisted of several fields: username, password, email, role and organization.
- Only the username, password and email fields were sent from the web browser to the /user/create endpoint
- The API endpoint used mass assignment to blindly assign any field from the POST request’s JSON data to the User model in the database (if the field existed in the User schema).
- The attackers were able to determine the names of security-related fields in the schema (role and organization)
- The attackers could supply arbitrary values for these fields when creating or updating a user account
- This let the attackers add themselves to other organizations and elevate their privileges to those of an administrator
Here ( $user = new User($request->post()); ), the endpoint creates a new User object and in doing so, passes all contents of the POST request to the constructor. PHP can “smartly” figure out which parameters go to which attributes of the class; however, this isn’t so smart when those attributes are certain things that shouldn’t be assignable! Even if the form only accepts inputs with username , password and email , a malicious actor can guess the other forms and simply add those fields to the JSON manually. As PHP has no way of discerning what it receives from “good” and “bad”, it simply updates them all. If only there were a way to tell PHP exactly which fields we don’t want to be assignable like that!
Impacts of mass assignment
By exploiting mass assignment vulnerabilities, a malicious actor could create multiple security problems including
- Data tampering : Attackers can modify sensitive information in the database, such as password or account balance
- Data theft : Attackers can gain access to confidential information stored in the database
- Elevation of privilege : Attackers can manipulate the properties of an object to gain additional privileges, such as administrator access
- Unauthorized access : Attackers can manipulate the properties of an object to gain unauthorized access to sensitive resources
Scan your code & stay secure with Snyk - for FREE!
Did you know you can use Snyk for free to verify that your code doesn't include this or other vulnerabilities?
Mass assignment mitigation
Use an allowlist of fields that can be assigned to.
Most mass assignment libraries or helper libraries should provide the ability to restrict the fields that will be read from a request and assigned to the data model. By restricting the assignment of user-supplied fields to only fields in the schema that are known safe ones, the values of security-sensitive fields will be prevented from tampering.
Using this strategy, the code for the application would be changed to add an allowlist using the pick() method of the underscore package and listing the allowed fields in the userCreateSafeFields array:
Laravel provides a library, eloquent , which, among other things, introduces object injection protection features! It gives you the ability to indicate variables that can be assigned, or otherwise, you can indicate variables that you don’t want assignable. This means that when you use mass assignment to populate a class with request data, the Laravel backend can (with your guiding hand) separate POST request input data from fields that should be populated and those that should not!
Using this strategy, the code for the application can be changed to add an allow-list of class attributes, enforcing that only these will be updated:
Use a Data Transfer Object (DTO)
Another option is to create an intermediary object (the DTO) that only has safe, assignable properties, which would be a subset of the target object that has those same fields plus any sensitive fields. Using our User example, the DTO would be:
The mass assignment operation can assign any user-supplied data to the DTO without the risk of inadvertently assigning any sensitive fields. The DTO can be copied to the final object, and during this process, any sensitive fields can be set to secure default values.
This method might require much more coding though. DTOs need to be created for all classes with sensitive fields. If there are many schemas with sensitive fields that require corresponding DTOs, then this becomes nearly as much work as not using mass assignment.
Use a denylist to declare fields that can’t be assigned to
The opposite of using an allowlist to define fields that are allowed to be assigned is to use a denylist of fields that shouldn’t be assigned. Security wisdom says to use allowlisting over denylisting because it’s safer to accidentally not include a safe field than to accidentally omit a dangerous field. So, following this advice, a denylist would be the less preferred option of the two. If there are 50 fields in a schema and only one is security-sensitive, then it is obviously much quicker to just denylist the one sensitive field. The danger here though would be if additional sensitive fields were added to the schema later and the developer forgot to add them to the denylist, then you would have a mass assignment vulnerability.
To use denylists, the code for the application would be changed in a similar manner to the code shown in the allow-list strategy shown earlier, except it would use the omit() method of the underscore package and listing the disallowed fields in the userCreateDisallowedFields array:
To use deny-lists, the code for the application would be changed in a similar manner to the code shown in the allow-list strategy shown earlier, the only difference being the User class is changed to have a “hidden” array:
Utilize a static analysis tool
Adding a static application security testing ( SAST ) tool to your devops pipeline as an additional line of defense is an excellent way to catch vulnerabilities before they make it to production. There are many, but Snyk Code is our personal favorite, as it scans in real-time, provides actionable remediation advice, and is available from your favorite IDE.
Keep learning
To learn more about mass assignment vulnerabilities, check out some other great content:
- OWASP guide to mass assignment vulnerabilties
- Find mass assignment in our top 10 list
Congratulations
You have taken your first step into learning what mass assignment is, how it works, what the impacts are, and how to protect your own applications. We hope that you will apply this knowledge to make your applications safer.
We'd really appreciate it if you could take a minute to rate how valuable this lesson was for you and provide feedback to help us improve! Also, make sure to check out our lessons on other common vulnerabilities.
What is mass assignment?
Mass assignment is a type of security vulnerability that occurs when an application code allows user-provided data to be used to set properties on an object without verifying that the user has the right to do so.
What to learn next?
Excessive agency, prompt injection, runc process.cwd container breakout vulnerability.
Help | Advanced Search
Computer Science > Cryptography and Security
Title: automated black-box testing of mass assignment vulnerabilities in restful apis.
Abstract: Mass assignment is one of the most prominent vulnerabilities in RESTful APIs. This vulnerability originates from a misconfiguration in common web frameworks, such that naming convention and automatic binding can be exploited by an attacker to craft malicious requests writing confidential resources and (massively) overriding data, that should be read-only and/or confidential. In this paper, we adopt a black-box testing perspective to automatically detect mass assignment vulnerabilities in RESTful APIs. Execution scenarios are generated purely based on the OpenAPI specification, that lists the available operations and their message format. Clustering is used to group similar operations and reveal read-only fields, the latter are candidate for mass assignment. Then, interaction sequences are automatically generated by instantiating abstract testing templates, trying to exploit the potential vulnerabilities. Finally, test cases are run, and their execution is assessed by a specific oracle, in order to reveal whether the vulnerability could be successfully exploited. The proposed novel approach has been implemented and evaluated on a set of case studies written in different programming languages. The evaluation highlights that the approach is quite effective in detecting seeded vulnerabilities, with a remarkably high accuracy.
Submission history
Access paper:.
- Download PDF
- Other Formats

References & Citations
- Google Scholar
- Semantic Scholar
BibTeX formatted citation

Bibliographic and Citation Tools
Code, data and media associated with this article, recommenders and search tools.
- Institution
arXivLabs: experimental projects with community collaborators
arXivLabs is a framework that allows collaborators to develop and share new arXiv features directly on our website.
Both individuals and organizations that work with arXivLabs have embraced and accepted our values of openness, community, excellence, and user data privacy. arXiv is committed to these values and only works with partners that adhere to them.
Have an idea for a project that will add value for arXiv's community? Learn more about arXivLabs .
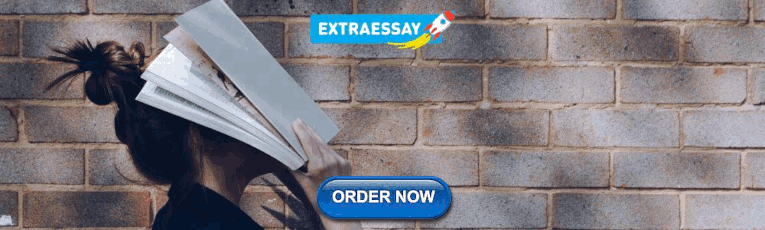
IMAGES
VIDEO
COMMENTS
The attacker also found the endpoint POST /api/v1/videos/new is vulnerable to mass assignment and allows the client to set any property of the video object. The attacker sets a malicious value as follows: "mp4_conversion_params":"-v codec h264 && format C:/". This value will cause a shell command injection once the attacker downloads the video ...
This is called a Mass Assignment vulnerability. Alternative Names¶ Depending on the language/framework in question, this vulnerability can have several alternative names: Mass Assignment: Ruby on Rails, NodeJS. Autobinding: Spring MVC, ASP NET MVC. Object injection: PHP. Example¶ Suppose there is a form for editing a user's account information:
A brief guide on API Mass Assignment Vulnerability. API vulnerabilities are a common thing that can break down your whole system if not patched. Hackers can leverage them to add additional code to your app or get access to your database. This can turn into a huge fiasco real quick. So it is always a good idea to put additional measures.
Mass Assignment & APIs - Exploitation in the Wild. APIs have become an integral part of many applications, with REST APIs being a popular choice for implementation. However, this popularity has led to security risks, with OWASP API Top 10 identifying vulnerabilities commonly found in APIs, including mass assignment.
The "Mass Assignment" vulnerability is a security flaw that occurs when an application assigns user input directly to model attributes without proper validation or sanitization. This can lead to unauthorized access and modification of sensitive data, potentially compromising the security of the application and its users.
Mass Assignment is a common vulnerability that occurs when a web application assigns user-supplied data to properties of an object without proper validation. This cheat sheet provides guidance on how to prevent and mitigate mass assignment attacks in different frameworks and languages. Learn how to use whitelists, blacklists, annotations, and other techniques to protect your web application ...
Attack surface visibility Improve security posture, prioritize manual testing, free up time. CI-driven scanning More proactive security - find and fix vulnerabilities earlier. Application security testing See how our software enables the world to secure the web. DevSecOps Catch critical bugs; ship more secure software, more quickly. Penetration testing Accelerate penetration testing - find ...
The mass assignment bug class is #6 on the OWASP API Security Top 10. One of the most notorious vulnerability disclosures, back in 2012, was when researcher Egar Homakov used a mass assignment exploit against GitHub to add his own public key to the Ruby on Rails repository and commit a message directly to the master branch. Why Mass Assignment?
API Mass Assignment Mass assignment vulnerabilites occur when a user is able to initialize or overwrite server-side variables for which are not intended by the application. By manually crafting a request to include additional parameters in a request, a malicious user may adversely affect application functionality. Common root causes of mass assignment vulnerabilities may include […]
API6:2019 — Mass assignment. The API takes data that client provides and stores it without proper filtering for whitelisted properties. Attackers can try to guess object properties or provide additional object properties in their requests, read the documentation, or check out API endpoints for clues where to find the openings to modify properties they are not supposed to on the data objects ...
WSTG - Latest is a comprehensive guide for web application security testing, covering various topics and techniques. One of them is testing for mass assignment, a common vulnerability that allows attackers to modify or create arbitrary objects by manipulating the request parameters. Learn how to identify and prevent this risk with OWASP's best practices and tools.
Steps to find APIs with potential Mass Assignment vulnerability: 1. Turn on the Burp Suite proxy and start visiting every endpoint in a web application. Focus on endpoints that allow a user to create resources into the application such as creating account, updating, creating wallet or inviting a user. 2.
The mass assignment vulnerability is a lack of data input validation that allows an attacker to modify data or elevate privileges by manipulating payload data. In the case of an object database, for example, if the payload maps directly to the stored data and is inserted directly, without input validation compared against authorisation levels, then the attacker can craft a payload that alters ...
Getting the Mass Assignment: Insecure Binder Configuration (API Abuse, ... @ShibinaEC Are there any solutions for insecure binder vulnerability in spring rest api? - fatih yavuz. Sep 25, 2020 at 9:46. Add a comment | ... To fix the warning, you could mark it as a false positive (if this is possible inside your setup). If that is not possible ...
This mass assignment vulnerability allowed any user to associate their public key to a given GitHub public or private repo and take ownership of that repo. The attack made use of one of GitHub's public APIs to find the identifier ID for a given repo. An attacker could then pair this identifier with their own public key and submit the data to ...
Objects in modern applications might contain many properties. Some of these properties should be updated directly by the client (e.g., user.first_name or user.address) and some of them should not (e.g., user.is_vip flag). An API endpoint is vulnerable if it automatically converts client parameters into internal object properties, without considering the sensitivity and the exposure level of ...
Besides introducing data transfer objects / view models layer to our app can help us prevent mass assignment vulnerabilities. This way we have view-models with minimum required properties which are mapped to EntityFramework models afterwards. Providing additional layer has its own drawback which is extra work.
The mass assignment vulnerability. So, how might an attacker exploit this? As may be evident from the code above, the framework is taking all the input fields from the Request variable and updating the model without performing any kind of validation. Therefore, its trusting that all the fields provided are intended to be updateable.
aware of mass assignment vulnerabilities in order to apply this mitigation correctly. As a matter of fact, the mass assignment vulnerability is still widespread and listed among the most common vulnerabilities in REST APIs. III. APPROACH The OWASP Foundation provides some guidelines [7] to detect and mitigate mass assignment vulnerabilities ...
The mass assignment operation can assign any user-supplied data to the DTO without the risk of inadvertently assigning any sensitive fields. The DTO can be copied to the final object, and during this process, any sensitive fields can be set to secure default values. This method might require much more coding though.
Download PDF Abstract: Mass assignment is one of the most prominent vulnerabilities in RESTful APIs. This vulnerability originates from a misconfiguration in common web frameworks, such that naming convention and automatic binding can be exploited by an attacker to craft malicious requests writing confidential resources and (massively) overriding data, that should be read-only and/or confidential.
API9:2019 Improper Assets Management. Old API versions are usually unpatched and are an easy way to compromise systems without having to fight state-of-the-art security mechanisms, which might be in place to protect the most recent API versions. Outdated documentation makes it more difficult to find and/or fix vulnerabilities.