C Functions
C structures, c declare multiple variables, declare multiple variables.
To declare more than one variable of the same type, use a comma-separated list:
You can also assign the same value to multiple variables of the same type:
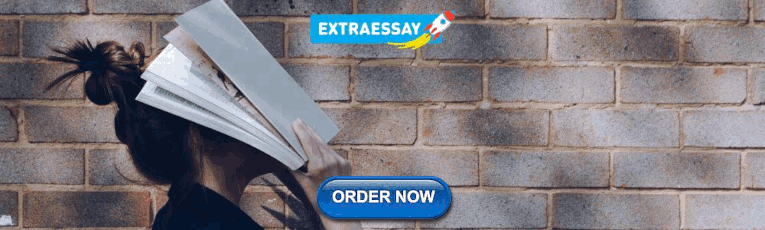
C Exercises
Test yourself with exercises.
Fill in the missing parts to create three variables of the same type, using a comma-separated list :
Start the Exercise

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.

- Data Structure
- Coding Problems
- C Interview Programs
- C++ Aptitude
- Java Aptitude
- C# Aptitude
- PHP Aptitude
- Linux Aptitude
- DBMS Aptitude
- Networking Aptitude
- AI Aptitude
- MIS Executive
- Web Technologie MCQs
- CS Subjects MCQs
- Databases MCQs
- Programming MCQs
- Testing Software MCQs
- Digital Mktg Subjects MCQs
- Cloud Computing S/W MCQs
- Engineering Subjects MCQs
- Commerce MCQs
- More MCQs...
- Machine Learning/AI
- Operating System
- Computer Network
- Software Engineering
- Discrete Mathematics
- Digital Electronics
- Data Mining
- Embedded Systems
- Cryptography
- CS Fundamental
- More Tutorials...
- Tech Articles
- Code Examples
- Programmer's Calculator
- XML Sitemap Generator
- Tools & Generators
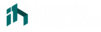
Home » C programming language
How expression a=b=c (Multiple Assignment) evaluates in C programming?
Since C language does not support chaining assignment like a=b=c ; each assignment operator ( = ) operates on two operands only. Then how expression a=b=c evaluates?
According to operators associativity assignment operator ( = ) operates from right to left, that means associativity of assignment operator ( = ) is right to left.
Expression a=b=c is actually a=(b=c) , see how expression a=(b=c) evaluates?
- Value of variable c will be assigned into variable b first.
- Then value of variable b will be assigned into variable a .
Finally value of variables a and b will be same as the value of variable c .
Consider the following program
Assigning a value to multiple variables of same type
By using such kind of expression we can easily assign a value to multiple variables of same data type, for example - if we want to assign 0 to integer variables a , b , c and d ; we can do it by following expression:
Related Tutorials
- Precedence and associativity of Arithmetic Operators
- Difference b/w operators and operands in C
- Unary Operators in C with Examples
- Equality Operators in C,C++
- Logical AND (&&) operator with example
- Logical OR (||) operator with example
- Logical NOT (!) operator with example
- Modulus on negative numbers in C language
- How expression a==b==c (Multiple Comparison) evaluates in C programming?
- Complex return statement using comma operator in c programming language
- Explain comma operator with an example
- Bitwise Operators and their working
- Bitwise One's Compliment (Bitwise NOT Operator) in C
- Modulus of two float or double numbers in C language
- Switch Case Tutorial, Syntax, Examples and Rules in C language
- Switch Statements (features, disadvantages and difference with if else)
- Using range with switch case statement
- 'goto' Statement in C language
- Use of break and continue within the loop in c
- Print numbers from 1 to N using goto statement
- Looping Tutorial in C programming
- Nested Loops in C programming language
- How to use for loop as infinite loop in C?
Comments and Discussions!
Load comments ↻
- Marketing MCQs
- Blockchain MCQs
- Artificial Intelligence MCQs
- Data Analytics & Visualization MCQs
- Python MCQs
- C++ Programs
- Python Programs
- Java Programs
- D.S. Programs
- Golang Programs
- C# Programs
- JavaScript Examples
- jQuery Examples
- CSS Examples
- C++ Tutorial
- Python Tutorial
- ML/AI Tutorial
- MIS Tutorial
- Software Engineering Tutorial
- Scala Tutorial
- Privacy policy
- Certificates
- Content Writers of the Month
Copyright © 2024 www.includehelp.com. All rights reserved.
This browser is no longer supported.
Upgrade to Microsoft Edge to take advantage of the latest features, security updates, and technical support.
C Compound Assignment
- 6 contributors
The compound-assignment operators combine the simple-assignment operator with another binary operator. Compound-assignment operators perform the operation specified by the additional operator, then assign the result to the left operand. For example, a compound-assignment expression such as
expression1 += expression2
can be understood as
expression1 = expression1 + expression2
However, the compound-assignment expression is not equivalent to the expanded version because the compound-assignment expression evaluates expression1 only once, while the expanded version evaluates expression1 twice: in the addition operation and in the assignment operation.
The operands of a compound-assignment operator must be of integral or floating type. Each compound-assignment operator performs the conversions that the corresponding binary operator performs and restricts the types of its operands accordingly. The addition-assignment ( += ) and subtraction-assignment ( -= ) operators can also have a left operand of pointer type, in which case the right-hand operand must be of integral type. The result of a compound-assignment operation has the value and type of the left operand.
In this example, a bitwise-inclusive-AND operation is performed on n and MASK , and the result is assigned to n . The manifest constant MASK is defined with a #define preprocessor directive.
C Assignment Operators
Was this page helpful?
Coming soon: Throughout 2024 we will be phasing out GitHub Issues as the feedback mechanism for content and replacing it with a new feedback system. For more information see: https://aka.ms/ContentUserFeedback .
Submit and view feedback for
Additional resources
Next: Conditional Expression , Previous: Logicals and Comparison , Up: Execution Control Expressions [ Contents ][ Index ]
8.3 Logical Operators and Assignments
There are cases where assignments nested inside the condition can actually make a program easier to read. Here is an example using a hypothetical type list which represents a list; it tests whether the list has at least two links, using hypothetical functions, nonempty which is true if the argument is a nonempty list, and list_next which advances from one list link to the next. We assume that a list is never a null pointer, so that the assignment expressions are always “true.”
Here we take advantage of the ‘ && ’ operator to avoid executing the rest of the code if a call to nonempty returns “false.” The only natural place to put the assignments is among those calls.
It would be possible to rewrite this as several statements, but that could make it much more cumbersome. On the other hand, when the test is even more complex than this one, splitting it into multiple statements might be necessary for clarity.
If an empty list is a null pointer, we can dispense with calling nonempty :
cppreference.com
Assignment operators.
Assignment and compound assignment operators are binary operators that modify the variable to their left using the value to their right.
[ edit ] Simple assignment
The simple assignment operator expressions have the form
Assignment performs implicit conversion from the value of rhs to the type of lhs and then replaces the value in the object designated by lhs with the converted value of rhs .
Assignment also returns the same value as what was stored in lhs (so that expressions such as a = b = c are possible). The value category of the assignment operator is non-lvalue (so that expressions such as ( a = b ) = c are invalid).
rhs and lhs must satisfy one of the following:
- both lhs and rhs have compatible struct or union type, or..
- rhs must be implicitly convertible to lhs , which implies
- both lhs and rhs have arithmetic types , in which case lhs may be volatile -qualified or atomic (since C11)
- both lhs and rhs have pointer to compatible (ignoring qualifiers) types, or one of the pointers is a pointer to void, and the conversion would not add qualifiers to the pointed-to type. lhs may be volatile or restrict (since C99) -qualified or atomic (since C11) .
- lhs is a (possibly qualified or atomic (since C11) ) pointer and rhs is a null pointer constant such as NULL or a nullptr_t value (since C23)
[ edit ] Notes
If rhs and lhs overlap in memory (e.g. they are members of the same union), the behavior is undefined unless the overlap is exact and the types are compatible .
Although arrays are not assignable, an array wrapped in a struct is assignable to another object of the same (or compatible) struct type.
The side effect of updating lhs is sequenced after the value computations, but not the side effects of lhs and rhs themselves and the evaluations of the operands are, as usual, unsequenced relative to each other (so the expressions such as i = ++ i ; are undefined)
Assignment strips extra range and precision from floating-point expressions (see FLT_EVAL_METHOD ).
In C++, assignment operators are lvalue expressions, not so in C.
[ edit ] Compound assignment
The compound assignment operator expressions have the form
The expression lhs @= rhs is exactly the same as lhs = lhs @ ( rhs ) , except that lhs is evaluated only once.
[ edit ] References
- C17 standard (ISO/IEC 9899:2018):
- 6.5.16 Assignment operators (p: 72-73)
- C11 standard (ISO/IEC 9899:2011):
- 6.5.16 Assignment operators (p: 101-104)
- C99 standard (ISO/IEC 9899:1999):
- 6.5.16 Assignment operators (p: 91-93)
- C89/C90 standard (ISO/IEC 9899:1990):
- 3.3.16 Assignment operators
[ edit ] See Also
Operator precedence
[ edit ] See also
- Recent changes
- Offline version
- What links here
- Related changes
- Upload file
- Special pages
- Printable version
- Permanent link
- Page information
- In other languages
- This page was last modified on 19 August 2022, at 08:36.
- This page has been accessed 54,567 times.
- Privacy policy
- About cppreference.com
- Disclaimers

Assignment Statement (=) in C Language
Assignment Statement in C language is a statement that assigns or set a value to a variable during program execution. Assignement statements in programming allows the programmer to change or set the value stored in variable using Assignment(=) Operator. The process of assigning the value to a variable using the assignment(=) operator is known as an assignment statement in C. Assignment(=) Operator Assigns The value or value in a variable on right hand side to the variable on the left hand side. The data type of the variable on right hand side should match to the data type of variable or constant or expression on right hand side. C Language has different (types) ways to assigns values to variable, we will learn from the diagram given below.
Syntax :1.Basic Assignment statement
Data Type Variable_name = variable/ constant /expression; The Variable_name is assigned the values in variable or constants or expression. The data type of the variable/ constant/expression on right hand side should match to the left hand side variable Variable_name with a few exceptions where automatic type conversions are possible.
Naming Rules or conventions for Assignment Statement
Programmer need to follow some Rules while writing the Assignment Statements in C program: 1. Variable names should not begin or start with number. Variable name can a letter, underscore, non-number any character like alphabet,underscore. 2. A new value assigned to an existing variable will overwrite the previous value and assign the new value to the variable. 3. The Data type defined and the variable value must match. 4.All the statements declaration must end with a semi-colon. (;) 5. The name of variable must be meaningful and clearly describe the purpose of variable name. 6.Duplicate name of variable is not allowed i.e the name once defined can only be used once in the program. programmer cannot redefine it to store other types of value.

Example 1: C program to illustrates the use of Simple Assignment statement .
/* e.g. C program to illustrate the use of simple Assignment statement or basic of assignment statements */ #include<stdio.h> int main() { int a,b,c; float avg; a = 9 ; c = 10 ; b = c ; printf("\n a=%d",a); printf("\n b=%d",b); printf("\n c=%d",c); b = c+3; printf("\n Value of b after b=c+3-->%d",b); avg = (b+c) / 2.0; printf("\n Value of avg=%.2f",avg); a = b && c; printf("\n a=b&&c--->%d",a); a = (b+c) && (b <c); printf("\n a=(b+c) && (b <c)--->%d",a); return(0); } Output: a=9 b=10 c=10 Value of b after b=c+3-->13 Value of avg=11.50 a=b&&c--->1 a=(b+c) && (b <c)--->0
Program Explanation: 1. In the above program a,b,c is declared as integer variable to store the numbers where as avg is declared as float. int a,b,c; float avg; 2. a = 9 ; c = 10 ; b = c ; variable a is assigned value 9. variable c is assigned value 10. and b is assigned the value of c. Here value of b is 10 .i.e. b=10 3. printf("\n a=%d",a); printf("\n b=%d",b); printf("\n c=%d",c); The above statements displays the integer values of variable a,b,c a=9 b=10 c=10 4. b = c+3; printf("\n Value of b after b=c+3-->%d",b); here 3 is added in c(value of vaiable c is 10) it becomes 13 and value 13 is assigned to left hand side variable b , here b is 13 i.e. b=13. the printf() displays output " Value of b after b=c+3--->13". 5. avg = (b+c) / 2.0; printf("\n Value of avg=%.2f",avg); After execution of a=(b+c)/2.0 the value of a is 11.50 so the output shown by printf() is "Value of avg=11.50". 6. a = b && c; printf("\n a=b&&c--->%d",a); Logical anding operation is performed on the values in the variables a and b. The value of c=10 and the value of b=13. After succesful execution of this statement value of variable a is 1 or 'true'. Any value in a variable except 'zero' (0 i.e false) is considered 'true' or 1. The variable a is assigned to the integer value 1 which is true. so a=1 && 1 is a=1 or a=true && true is a=true or a=1. printf("\n a=b&&c--->%d",a); displays output a=b&&c---->1 7. a = (b+c) && (b <c); printf("\n a=(b+c) && (b <c)--->%d",a); here expression (b+c) is true(1) and (b <c) is false(0). so the entire expression evaluates to false(0) and the value is assigned to the variable a i.e a=0. the printf() display the message " a=(b+c) && (b <c)--->0 "
2.Compound Assignment: As we learn in above section the simple assignment statement is used to assign values in right hand side variable to left hand side variable using = operator. A compound assignment operator has a shorter syntax to assign the result. A compound assignment operator or statements are used to do mathematical operation in shortcut. Two operands needs to perform the compound assignment operations. The operation is performed on the two operands before the result is assigned to the first operand. compound assignment operator are binary operators that modify the variable to their left hand side using the value or variable to their right.
Syntax: expression1+= expression2; These type of expression can also be written in expanded form, that is expression1=expression1+expression2;
+= Operator is called compound assignment operator. C Language provides the following list of compound Assignment Operators. 1. += plus equal to += is Addition and Assignment operators. It add the value of the variable1 and variable2 and assigns the result to variable1. e.g. X+=Y In the above expression the addition of values in X and Y is performd and assigns result to X. The expression X+=Y is same as X=X+Y 2. -= minus equal to -= is Subtraction and Assignment operators. It Subtract the value of the variable2 from variable1 and assigns the result to variable1. e.g. X-=Y In the above expression the subtraction is performd,the value of X is subtracted from the value in X and assigns result to X. The expression X-=Y is same as X=X-Y 3. *= Multiplication equal to *= is Multiplication and Assignment operators. It Multiply the value of the variable1 and variable2 and assigns the result to variable1. e.g. X*=Y In the above expression the Multiplication is performd,the value of X is Multiplyed to the value in Y and assigns result to X. The expression X*=Y is same as X=X*Y 4. /= Division equal to *= is Division and Assignment operators. It Divides the value of the variable1 byvariable2 and assigns the result to variable1. e.g. X/=Y In the above expression the Division is performd,the value of X is Divided by the value in Y and assigns result to X. The expression X/=Y is same as X=X/Y 5. %= Modulus equal to %= is Modulus and Assignment operators. It Divides the value of the variable1 byvariable2 and assigns the remainder to variable1. e.g. X%=Y In the above expression the Division is performd,the value of X is Divided by the value in Y and assigns remainder to X. The expression X%=Y is same as X=X%Y 6. &= Bitwise and equal to &= is Bitwise AND and Assignment operators. It performs the bitwise AND with variable1 and variable2 and assigns the result to variable1. e.g. X&=Y In the above expression the bitwise anding operation is performd, after executing the X&=Y expression the result is assigned to X. The expression X&=Y is same as X=X&Y 7. |= Bitwise OR equal to != is Bitwise OR Assignment operators. It performs the bitwise OR with variable1 and variable2 and assigns the result to variable1. e.g. X|=Y In the above expression the bitwise OR operation is performd, after executing the X|=Y expression the result is assigned to X. The expression X|=Y is same as X=X|Y 8. ^= Bitwise XOR equal to ^= is Bitwise XOR Assignment operators. It performs the bitwise XOR with variable1 and variable2 and assigns the result to variable1. e.g. X^=Y In the above expression the bitwise XOR operation is performd, after executing the X^=Y expression the result is assigned to X. The expression X^=Y is same as X=X^Y. 9. Bitwise left shift and equal to e.g. X In the above expression the bitwise left shift operation is performd, after executing the X The expression X 10. >>= Bitwise right shift and equal to >>= is Bitwise Right Shift and Assignment operators. It performs the bitwise Right shift with variable1 and assigns the result to variable1. e.g. X>>=Y In the above expression the bitwise right shift operation is performd, after executing the X>>=Y expression the result is assigned to X. The expression X>>=Y is same as X=X>>Y Lets Learn and practice The Assigment operator in detail using the following C program.
2. Assignment Operator complete C Program.
#include <stdio.h> int main() { /* Simple Assignment*/ int x,i; int y,j; float c=30.0; float d=5.0; /*Nested or Multiple Assignment */ x = i = 5; y = j = 3; /*Compound Assignment*/ x += y; printf("After Add and Assign :%d \n",x); i -= j; printf("After Subtract and Assign :%d \n",i); x *= y; printf("After Multiple and Assign :%d \n",x); c /= d; printf("After Divide and Assign :%f \n",c); j %= i; printf("After Modulo and Assign :%d \n",j); j &= i; printf("After Bitwise And and Assign :%d \n",j); j |= i; printf("After Bitwise OR and Assign :%d \n",j); x ^= y; printf("After Bitwise XOR and Assign :%d \n",a); x printf ("After Bitwise Left Shift and Assign :%d \n",a); x >>= 3; printf ("After Bitwise Right Shift and Assign :%d \n",a); return(0); }
Output: After Add and Assign :8 After Subtract and Assign :2 After Multiple and Assign :24 After Divide and Assign :6.000000 After Modulo and Assign :1 After Bitwise And and Assign :0 After Bitwise OR and Assign :2 After Bitwise XOR and Assign :27 After Bitwise Left Shift and Assign :108 After Bitwise Right Shift and Assign :13
/** * C program to check leap year using conditional operator ? */ #include <stdio.h> int main() { int year; /* * Input the year from user */ printf("Enter any year: "); scanf("%d", &year); /* * If year%4==0 and year%100==0 then * print leap year * else if year%400==0 then * print leap year * else * print common year */ (year%4==0 && year%100!=0) ? printf("LEAP YEAR") : (year%400 ==0 ) ? printf("LEAP YEAR") : printf("COMMON YEAR"); return 0; } Output: Enter any year 2004 LEAP YEAR
/** * C program to check leap year using conditional operator ? */ #include <stdio.h> int main() { int year; /* * Input the year from user */ printf("Enter any year: "); scanf("%d", &year); /* If year%4==0 and year%100==0 then print leap year else if year%400==0 then print leap year else print common year */ (year%4==0 && year%100!=0) ? printf("LEAP YEAR") : (year%400 ==0 ) ? printf("LEAP YEAR") : printf("COMMON YEAR"); return 0; } Output: Enter any year 2004 LEAP YEAR
Previous Topic:-->> Constant and Literals in C || Next topic:-->> Input/Output in C.
Get in touch
Assignment Statement in C
How to assign values to the variables? C provides an assignment operator for this purpose, assigning the value to a variable using assignment operator is known as an assignment statement in C.
The function of this operator is to assign the values or values in variables on right hand side of an expression to variables on the left hand side.
The syntax of the assignment expression
Variable = constant / variable/ expression;
The data type of the variable on left hand side should match the data type of constant/variable/expression on right hand side with a few exceptions where automatic type conversions are possible.
Examples of assignment statements,
b = c ; /* b is assigned the value of c */ a = 9 ; /* a is assigned the value 9*/ b = c+5; /* b is assigned the value of expr c+5 */
The expression on the right hand side of the assignment statement can be:
An arithmetic expression; A relational expression; A logical expression; A mixed expression.
The above mentioned expressions are different in terms of the type of operators connecting the variables and constants on the right hand side of the variable. Arithmetic operators, relational
Arithmetic operators, relational operators and logical operators are discussed in the following sections.
For example, int a; float b,c ,avg, t; avg = (b+c) / 2; /*arithmetic expression */ a = b && c; /*logical expression*/ a = (b+c) && (b<c); /* mixed expression*/
Share this:
- Click to share on Twitter (Opens in new window)
- Click to share on Facebook (Opens in new window)
Related Posts
- #define to implement constants
- Preprocessor in C Language
- Pointers and Strings
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
Notify me of follow-up comments by email.
Notify me of new posts by email.
This site uses Akismet to reduce spam. Learn how your comment data is processed .
How to structure a multi-file C program: Part 1
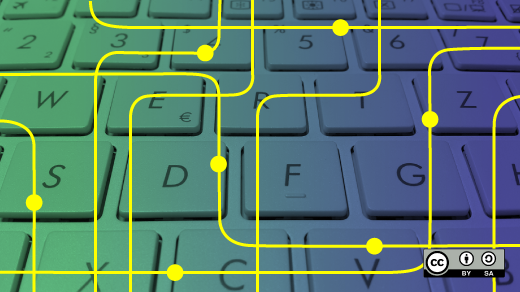
Opensource.com
It has often been said that the art of computer programming is part managing complexity and part naming things. I contend that this is largely true with the addition of "and sometimes it requires drawing boxes."
In this article, I'll name some things and manage some complexity while writing a small C program that is loosely based on the program structure I discussed in " How to write a good C main function "—but different. This one will do something. Grab your favorite beverage, editor, and compiler, crank up some tunes, and let's write a mildly interesting C program together.
Philosophy of a good Unix program
The first thing to know about this C program is that it's a Unix command-line tool. This means that it runs on (or can be ported to) operating systems that provide a Unix C runtime environment. When Unix was invented at Bell Labs, it was imbued from the beginning with a design philosophy . In my own words: programs do one thing, do it well, and act on files . While it makes sense to do one thing and do it well, the part about "acting on files" seems a little out of place.
It turns out that the Unix abstraction of a "file" is very powerful. A Unix file is a stream of bytes that ends with an end-of-file (EOF) marker. That's it. Any other structure in a file is imposed by the application and not the operating system. The operating system provides system calls that allow a program to perform a set of standard operations on files: open, read, write, seek, and close (there are others, but those are the biggies). Standardizing access to files allows different programs to share a common abstraction and work together even when different people implement them in different programming languages.
Having a shared file interface makes it possible to build programs that are composable . The output of one program can be the input of another program. The Unix family of operating systems provides three files by default whenever a program is executed: standard in ( stdin ), standard out ( stdout ), and standard error ( stderr ). Two of these files are opened in write-only mode: stdout and stderr , while stdin is opened read-only. We see this in action whenever we use file redirection in a command shell like Bash:
This construction can be described briefly as: the output of ls is written to stdout, which is redirected to the stdin of grep , whose stdout is redirected to sed , whose stdout is redirected to write to a file called ack in the current directory.
We want our program to play well in this ecosystem of equally flexible and awesome programs, so let's write a program that reads and writes files.
MeowMeow: A stream encoder/decoder concept
When I was a dewy-eyed kid studying computer science in the <mumbles>s, there were a plethora of encoding schemes. Some of them were for compressing files, some were for packaging files together, and others had no purpose but to be excruciatingly silly. An example of the last is the MooMoo encoding scheme .
To give our program a purpose, I'll update this concept for the 2000s and implement a concept called MeowMeow encoding (since the internet loves cats). The basic idea here is to take files and encode each nibble (half of a byte) with the text "meow." A lower-case letter indicates a zero, and an upper-case indicates a one. Yes, it will balloon the size of a file since we are trading 4 bits for 32 bits. Yes, it's pointless. But imagine the surprise on someone's face when this happens:
This is going to be awesome.
Implementation, finally
The full source for this can be found on GitHub , but I'll talk through my thought process while writing it. The object is to illustrate how to structure a C program composed of multiple files.
Having already established that I want to write a program that encodes and decodes files in MeowMeow format, I fired up a shell and issued the following commands:
In short, I created a directory full of empty files and committed them to git.
Even though the files are empty, you can infer the purpose of each from its name. Just in case you can't, I annotated each touch with a brief description.
Usually, a program starts as a single, simple main.c file, with only two or three functions that solve the problem. And then the programmer rashly shows that program to a friend or her boss, and suddenly the number of functions in the file balloons to support all the new "features" and "requirements" that pop up. The first rule of "Program Club" is don't talk about "Program Club." The second rule is to minimize the number of functions in one file.
To be honest, the C compiler does not care one little bit if every function in your program is in one file. But we don't write programs for computers or compilers; we write them for other people (who are sometimes us). I know that is probably a surprise, but it's true. A program embodies a set of algorithms that solve a problem with a computer, and it's important that people understand it when the parameters of the problem change in unanticipated ways. People will have to modify the program, and they will curse your name if you have all 2,049 functions in one file.
So we good and true programmers break functions out, grouping similar functions into separate files. Here I've got files main.c , mmencode.c , and mmdecode.c . For small programs like this, it may seem like overkill. But small programs rarely stay small, so planning for expansion is a "Good Idea."
But what about those .h files? I'll explain them in general terms later, but in brief, those are called header files, and they can contain C language type definitions and C preprocessor directives. Header files should not have any functions in them. You can think of headers as a definition of the application programming interface (API) offered by the .c flavored file that is used by other .c files.
But what the heck is a Makefile?
I know all you cool kids are using the "Ultra CodeShredder 3000" integrated development environment to write the next blockbuster app, and building your project consists of mashing on Ctrl-Meta-Shift-Alt-Super-B. But back in my day (and also today), lots of useful work got done by C programs built with Makefiles. A Makefile is a text file that contains recipes for working with files, and programmers use it to automate building their program binaries from source (and other stuff too!).
Take, for instance, this little gem:
Text after an octothorpe/pound/hash is a comment, like in line 00.
Line 01 is a variable assignment where the variable TARGET takes on the string value my_sweet_program . By convention, OK, my preference, all Makefile variables are capitalized and use underscores to separate words.
Line 02 consists of the name of the file that the recipe creates and the files it depends on. In this case, the target is my_sweet_program , and the dependency is main.c .
The final line, 03, is indented with a tab and not four spaces. This is the command that will be executed to create the target. In this case, we call cc the C compiler frontend to compile and link my_sweet_program .
Using a Makefile is simple:
The Makefile that will build our MeowMeow encoder/decoder is considerably more sophisticated than this example, but the basic structure is the same. I'll break it down Barney-style in another article.
Form follows function
My idea here is to write a program that reads a file, transforms it, and writes the transformed data to another file. The following fabricated command-line interaction is how I imagine using the program:
We need to write code to handle command-line parsing and managing the input and output streams. We need a function to encode a stream and write it to another stream. And finally, we need a function to decode a stream and write it to another stream. Wait a second, I've only been talking about writing one program, but in the example above, I invoke two commands: meow and unmeow ? I know you are probably thinking that this is getting complex as heck.
Minor sidetrack: argv[0] and the ln command
If you recall, the signature of a C main function is:
where argc is the number of command-line arguments, and argv is a list of character pointers (strings). The value of argv[0] is the path of the file containing the program being executed. Many Unix utility programs with complementary functions (e.g., compress and uncompress) look like two programs, but in fact, they are one program with two names in the filesystem. The two-name trick is accomplished by creating a filesystem "link" using the ln command.
An example from /usr/bin on my laptop is:
Here git and git-receive-pack are the same file with different names. We can tell it's the same file because they have the same inode number (the first column). An inode is a feature of the Unix filesystem and is super outside the scope of this article.
Good and/or lazy programmers can use this feature of the Unix filesystem to write less code but double the number of programs they deliver. First, we write a program that changes its behavior based on the value of argv[0] , then we make sure to create links with the names that cause the behavior.
In our Makefile, the unmeow link is created using this recipe:
I tend to parameterize everything in my Makefiles, rarely using a "bare" string. I group all the definitions at the top of the Makefile, which makes it easy to find and change them. This makes a big difference when you are trying to port software to a new platform and you need to change all your rules to use xcc instead of cc .
The recipe should appear relatively straightforward except for the two built-in variables $@ and $< . The first is a shortcut for the target of the recipe; in this case, $(DECODER) . (I remember this because the at-sign looks like a target to me.) The second, $< is the rule dependency; in this case, it resolves to $(ENCODER) .
Things are getting complex for sure, but it's managed.
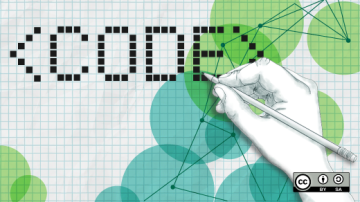
How to write a good C main function
Learn how to structure a C file and write a C main function that handles command line arguments like a champ.
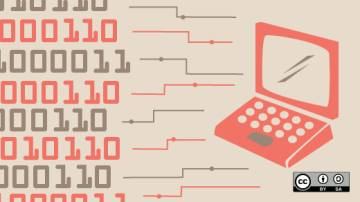
What is open source programming?
Open source is more than just chucking some code up on GitHub. Learn what it is—and what it's not.
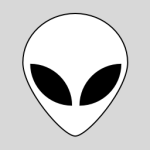
Related Content
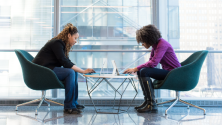
PrepBytes Blog
ONE-STOP RESOURCE FOR EVERYTHING RELATED TO CODING
Sign in to your account
Forgot your password?
Login via OTP
We will send you an one time password on your mobile number
An OTP has been sent to your mobile number please verify it below
Register with PrepBytes
Assignment operator in c.
Last Updated on June 23, 2023 by Prepbytes
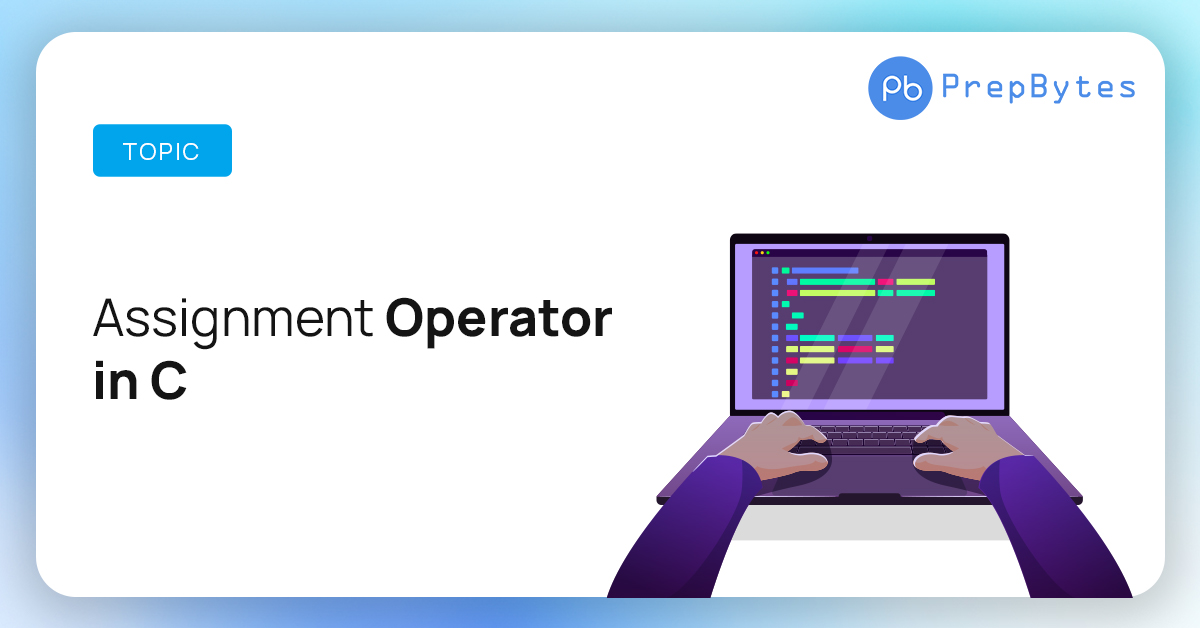
This type of operator is employed for transforming and assigning values to variables within an operation. In an assignment operation, the right side represents a value, while the left side corresponds to a variable. It is essential that the value on the right side has the same data type as the variable on the left side. If this requirement is not fulfilled, the compiler will issue an error.
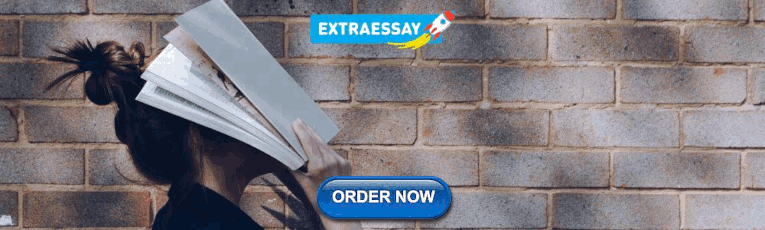
What is Assignment Operator in C language?
In C, the assignment operator serves the purpose of assigning a value to a variable. It is denoted by the equals sign (=) and plays a vital role in storing data within variables for further utilization in code. When using the assignment operator, the value present on the right-hand side is assigned to the variable on the left-hand side. This fundamental operation allows developers to store and manipulate data effectively throughout their programs.
Example of Assignment Operator in C
For example, consider the following line of code:
Types of Assignment Operators in C
Here is a list of the assignment operators that you can find in the C language:
Simple assignment operator (=): This is the basic assignment operator, which assigns the value on the right-hand side to the variable on the left-hand side.
Addition assignment operator (+=): This operator adds the value on the right-hand side to the variable on the left-hand side and assigns the result back to the variable.
x += 3; // Equivalent to x = x + 3; (adds 3 to the current value of "x" and assigns the result back to "x")
Subtraction assignment operator (-=): This operator subtracts the value on the right-hand side from the variable on the left-hand side and assigns the result back to the variable.
x -= 4; // Equivalent to x = x – 4; (subtracts 4 from the current value of "x" and assigns the result back to "x")
* Multiplication assignment operator ( =):** This operator multiplies the value on the right-hand side with the variable on the left-hand side and assigns the result back to the variable.
x = 2; // Equivalent to x = x 2; (multiplies the current value of "x" by 2 and assigns the result back to "x")
Division assignment operator (/=): This operator divides the variable on the left-hand side by the value on the right-hand side and assigns the result back to the variable.
x /= 2; // Equivalent to x = x / 2; (divides the current value of "x" by 2 and assigns the result back to "x")
Bitwise AND assignment (&=): The bitwise AND assignment operator "&=" performs a bitwise AND operation between the value on the left-hand side and the value on the right-hand side. It then assigns the result back to the left-hand side variable.
x &= 3; // Binary: 0011 // After bitwise AND assignment: x = 1 (Binary: 0001)
Bitwise OR assignment (|=): The bitwise OR assignment operator "|=" performs a bitwise OR operation between the value on the left-hand side and the value on the right-hand side. It then assigns the result back to the left-hand side variable.
x |= 3; // Binary: 0011 // After bitwise OR assignment: x = 7 (Binary: 0111)
Bitwise XOR assignment (^=): The bitwise XOR assignment operator "^=" performs a bitwise XOR operation between the value on the left-hand side and the value on the right-hand side. It then assigns the result back to the left-hand side variable.
x ^= 3; // Binary: 0011 // After bitwise XOR assignment: x = 6 (Binary: 0110)
Left shift assignment (<<=): The left shift assignment operator "<<=" shifts the bits of the value on the left-hand side to the left by the number of positions specified by the value on the right-hand side. It then assigns the result back to the left-hand side variable.
x <<= 2; // Binary: 010100 (Shifted left by 2 positions) // After left shift assignment: x = 20 (Binary: 10100)
Right shift assignment (>>=): The right shift assignment operator ">>=" shifts the bits of the value on the left-hand side to the right by the number of positions specified by the value on the right-hand side. It then assigns the result back to the left-hand side variable.
x >>= 2; // Binary: 101 (Shifted right by 2 positions) // After right shift assignment: x = 5 (Binary: 101)
Conclusion The assignment operator in C, denoted by the equals sign (=), is used to assign a value to a variable. It is a fundamental operation that allows programmers to store data in variables for further use in their code. In addition to the simple assignment operator, C provides compound assignment operators that combine arithmetic or bitwise operations with assignment, allowing for concise and efficient code.
FAQs related to Assignment Operator in C
Q1. Can I assign a value of one data type to a variable of another data type? In most cases, assigning a value of one data type to a variable of another data type will result in a warning or error from the compiler. It is generally recommended to assign values of compatible data types to variables.
Q2. What is the difference between the assignment operator (=) and the comparison operator (==)? The assignment operator (=) is used to assign a value to a variable, while the comparison operator (==) is used to check if two values are equal. It is important not to confuse these two operators.
Q3. Can I use multiple assignment operators in a single statement? No, it is not possible to use multiple assignment operators in a single statement. Each assignment operator should be used separately for assigning values to different variables.
Q4. Are there any limitations on the right-hand side value of the assignment operator? The right-hand side value of the assignment operator should be compatible with the data type of the left-hand side variable. If the data types are not compatible, it may lead to unexpected behavior or compiler errors.
Q5. Can I assign the result of an expression to a variable using the assignment operator? Yes, it is possible to assign the result of an expression to a variable using the assignment operator. For example, x = y + z; assigns the sum of y and z to the variable x.
Q6. What happens if I assign a value to an uninitialized variable? Assigning a value to an uninitialized variable will initialize it with the assigned value. However, it is considered good practice to explicitly initialize variables before using them to avoid potential bugs or unintended behavior.
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
- Linked List
- Segment Tree
- Backtracking
- Dynamic Programming
- Greedy Algorithm
- Operating System
- Company Placement
- Interview Tips
- General Interview Questions
- Data Structure
- Other Topics
- Computational Geometry
- Game Theory
Related Post
Null character in c, ackermann function in c, median of two sorted arrays of different size in c, number is palindrome or not in c, implementation of queue using linked list in c, c program to replace a substring in a string.
C Data Types
C operators.
- C Input and Output
- C Control Flow
- C Functions
- C Preprocessors
C File Handling
- C Cheatsheet
C Interview Questions
- C Programming Language Tutorial
- C Language Introduction
- Features of C Programming Language
- C Programming Language Standard
- C Hello World Program
- Compiling a C Program: Behind the Scenes
- Tokens in C
- Keywords in C
C Variables and Constants
- C Variables
- Constants in C
- Const Qualifier in C
- Different ways to declare variable as constant in C
- Scope rules in C
- Internal Linkage and External Linkage in C
- Global Variables in C
- Data Types in C
- Literals in C
- Escape Sequence in C
- Integer Promotions in C
- Character Arithmetic in C
- Type Conversion in C
C Input/Output
- Basic Input and Output in C
- Format Specifiers in C
- printf in C
- Scansets in C
- Formatted and Unformatted Input/Output functions in C with Examples
- Operators in C
- Arithmetic Operators in C
- Unary operators in C
- Relational Operators in C
- Bitwise Operators in C
- C Logical Operators
- Assignment Operators in C
- Increment and Decrement Operators in C
- Conditional or Ternary Operator (?:) in C
- sizeof operator in C
- Operator Precedence and Associativity in C
C Control Statements Decision-Making
- Decision Making in C (if , if..else, Nested if, if-else-if )
- C - if Statement
- C if...else Statement
- C if else if ladder
- Switch Statement in C
- Using Range in switch Case in C
- while loop in C
- do...while Loop in C
- For Versus While
- Continue Statement in C
- Break Statement in C
- goto Statement in C
- User-Defined Function in C
- Parameter Passing Techniques in C
- Function Prototype in C
- How can I return multiple values from a function?
- main Function in C
- Implicit return type int in C
- Callbacks in C
- Nested functions in C
- Variadic functions in C
- _Noreturn function specifier in C
- Predefined Identifier __func__ in C
- C Library math.h Functions
C Arrays & Strings
- Properties of Array in C
- Multidimensional Arrays in C
- Initialization of Multidimensional Array in C
- Pass Array to Functions in C
- How to pass a 2D array as a parameter in C?
- What are the data types for which it is not possible to create an array?
- How to pass an array by value in C ?
- Strings in C
- Array of Strings in C
- What is the difference between single quoted and double quoted declaration of char array?
- C String Functions
- Pointer Arithmetics in C with Examples
- C - Pointer to Pointer (Double Pointer)
- Function Pointer in C
- How to declare a pointer to a function?
- Pointer to an Array | Array Pointer
- Difference between constant pointer, pointers to constant, and constant pointers to constants
- Pointer vs Array in C
- Dangling, Void , Null and Wild Pointers in C
- Near, Far and Huge Pointers in C
- restrict keyword in C
C User-Defined Data Types
C structures.
- dot (.) Operator in C
- Structure Member Alignment, Padding and Data Packing
- Flexible Array Members in a structure in C
- Bit Fields in C
- Difference Between Structure and Union in C
- Anonymous Union and Structure in C
- Enumeration (or enum) in C
C Storage Classes
- Storage Classes in C
- extern Keyword in C
- Static Variables in C
- Initialization of static variables in C
- Static functions in C
- Understanding "volatile" qualifier in C | Set 2 (Examples)
- Understanding "register" keyword in C
C Memory Management
- Memory Layout of C Programs
- Dynamic Memory Allocation in C using malloc(), calloc(), free() and realloc()
- Difference Between malloc() and calloc() with Examples
- What is Memory Leak? How can we avoid?
- Dynamic Array in C
- How to dynamically allocate a 2D array in C?
- Dynamically Growing Array in C
C Preprocessor
- C Preprocessor Directives
- How a Preprocessor works in C?
- Header Files in C
- What’s difference between header files "stdio.h" and "stdlib.h" ?
- How to write your own header file in C?
- Macros and its types in C
- Interesting Facts about Macros and Preprocessors in C
- # and ## Operators in C
- How to print a variable name in C?
- Multiline macros in C
- Variable length arguments for Macros
- Branch prediction macros in GCC
- typedef versus #define in C
- Difference between #define and const in C?
- Basics of File Handling in C
- C fopen() function with Examples
- EOF, getc() and feof() in C
- fgets() and gets() in C language
- fseek() vs rewind() in C
- What is return type of getchar(), fgetc() and getc() ?
- Read/Write Structure From/to a File in C
- C Program to print contents of file
- C program to delete a file
- C Program to merge contents of two files into a third file
- What is the difference between printf, sprintf and fprintf?
- Difference between getc(), getchar(), getch() and getche()
Miscellaneous
- time.h header file in C with Examples
- Input-output system calls in C | Create, Open, Close, Read, Write
- Signals in C language
- Program error signals
- Socket Programming in C
- _Generics Keyword in C
- Multithreading in C
- C Programming Interview Questions (2024)
- Commonly Asked C Programming Interview Questions | Set 1
- Commonly Asked C Programming Interview Questions | Set 2
- Commonly Asked C Programming Interview Questions | Set 3
The structure in C is a user-defined data type that can be used to group items of possibly different types into a single type. The struct keyword is used to define the structure in the C programming language. The items in the structure are called its member and they can be of any valid data type.
C Structure Declaration
We have to declare structure in C before using it in our program. In structure declaration, we specify its member variables along with their datatype. We can use the struct keyword to declare the structure in C using the following syntax:
The above syntax is also called a structure template or structure prototype and no memory is allocated to the structure in the declaration.
C Structure Definition
To use structure in our program, we have to define its instance. We can do that by creating variables of the structure type. We can define structure variables using two methods:
1. Structure Variable Declaration with Structure Template
2. structure variable declaration after structure template, access structure members.
We can access structure members by using the ( . ) dot operator.
In the case where we have a pointer to the structure, we can also use the arrow operator to access the members.
Initialize Structure Members
Structure members cannot be initialized with the declaration. For example, the following C program fails in the compilation.
The reason for the above error is simple. When a datatype is declared, no memory is allocated for it. Memory is allocated only when variables are created.
We can initialize structure members in 3 ways which are as follows:
- Using Assignment Operator.
- Using Initializer List.
- Using Designated Initializer List.
1. Initialization using Assignment Operator
2. initialization using initializer list.
In this type of initialization, the values are assigned in sequential order as they are declared in the structure template.
3. Initialization using Designated Initializer List
Designated Initialization allows structure members to be initialized in any order. This feature has been added in the C99 standard .
The Designated Initialization is only supported in C but not in C++.
Example of Structure in C
The following C program shows how to use structures
typedef for Structures
The typedef keyword is used to define an alias for the already existing datatype. In structures, we have to use the struct keyword along with the structure name to define the variables. Sometimes, this increases the length and complexity of the code. We can use the typedef to define some new shorter name for the structure.
Nested Structures
C language allows us to insert one structure into another as a member. This process is called nesting and such structures are called nested structures . There are two ways in which we can nest one structure into another:
1. Embedded Structure Nesting
In this method, the structure being nested is also declared inside the parent structure.
2. Separate Structure Nesting
In this method, two structures are declared separately and then the member structure is nested inside the parent structure.
One thing to note here is that the declaration of the structure should always be present before its definition as a structure member. For example, the declaration below is invalid as the struct mem is not defined when it is declared inside the parent structure.
Accessing Nested Members
We can access nested Members by using the same ( . ) dot operator two times as shown:
Example of Structure Nesting
Structure pointer in c.
We can define a pointer that points to the structure like any other variable. Such pointers are generally called Structure Pointers . We can access the members of the structure pointed by the structure pointer using the ( -> ) arrow operator.
Example of Structure Pointer
Self-referential structures.
The self-referential structures in C are those structures that contain references to the same type as themselves i.e. they contain a member of the type pointer pointing to the same structure type.
Example of Self-Referential Structures
Such kinds of structures are used in different data structures such as to define the nodes of linked lists, trees, etc.
C Structure Padding and Packing
Technically, the size of the structure in C should be the sum of the sizes of its members. But it may not be true for most cases. The reason for this is Structure Padding.
Structure padding is the concept of adding multiple empty bytes in the structure to naturally align the data members in the memory. It is done to minimize the CPU read cycles to retrieve different data members in the structure.
There are some situations where we need to pack the structure tightly by removing the empty bytes. In such cases, we use Structure Packing. C language provides two ways for structure packing:
- Using #pragma pack(1)
- Using __attribute((packed))__
Example of Structure Padding and Packing
As we can see, the size of the structure is varied when structure packing is performed.
To know more about structure padding and packing, refer to this article – Structure Member Alignment, Padding and Data Packing .
Bit Fields are used to specify the length of the structure members in bits. When we know the maximum length of the member, we can use bit fields to specify the size and reduce memory consumption.
Syntax of Bit Fields
example of bit fields.
As we can see, the size of the structure is reduced when using the bit field to define the max size of the member ‘a’.
Uses of Structure in C
C structures are used for the following:
- The structure can be used to define the custom data types that can be used to create some complex data types such as dates, time, complex numbers, etc. which are not present in the language.
- It can also be used in data organization where a large amount of data can be stored in different fields.
- Structures are used to create data structures such as trees, linked lists, etc.
- They can also be used for returning multiple values from a function.
Limitations of C Structures
In C language, structures provide a method for packing together data of different types. A Structure is a helpful tool to handle a group of logically related data items. However, C structures also have some limitations.
- Higher Memory Consumption: It is due to structure padding.
- No Data Hiding: C Structures do not permit data hiding. Structure members can be accessed by any function, anywhere in the scope of the structure.
- Functions inside Structure: C structures do not permit functions inside the structure so we cannot provide the associated functions.
- Static Members: C Structure cannot have static members inside its body.
- Construction creation in Structure: Structures in C cannot have a constructor inside Structures.
Related Articles
- C Structures vs C++ Structure
Please Login to comment...
Similar reads.
- C-Structure & Union
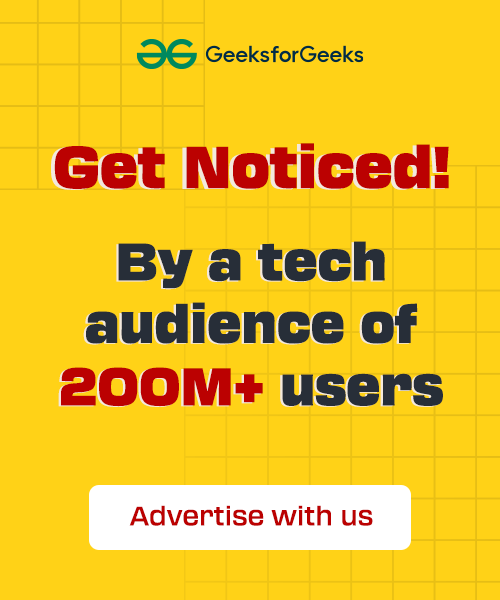
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
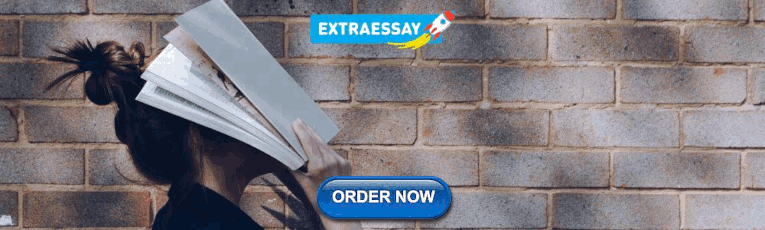
IMAGES
VIDEO
COMMENTS
(Note that this sequencing freedom is specific to C language, in which the result of an assignment in an rvalue. In C++ assignment evaluates to an lvalue, which requires "chained" assignments to be sequenced.) ... Because, the multiple assignment translates to: sample2 = 0; sample1 = sample2; So instead of 2 initializations you do only one and ...
But C language treats the multiple assignments like a chain, like this: In C, "X = Y = Z" means that the value of Z must be first assigned to Y, and then the value of Y must be assigned to X. Here is a sample program to see how the multiple assignments in single line work:
7 Assignment Expressions. As a general concept in programming, an assignment is a construct that stores a new value into a place where values can be stored—for instance, in a variable. Such places are called lvalues (see Lvalues) because they are locations that hold a value. An assignment in C is an expression because it has a value; we call it an assignment expression.
Exercise: Fill in the missing parts to create three variables of the same type, using a comma-separated list: myNum1 = 10 myNum2 = 15 myNum3 = 25; printf("%d", myNum1 + myNum2 + myNum3); Submit Answer ». Start the Exercise. Previous Next .
Before Multiple Assignent a=0,b=0,c=100 After Multiple Assignent a=100,b=100,c=100 Assigning a value to multiple variables of same type By using such kind of expression we can easily assign a value to multiple variables of same data type, for example - if we want to assign 0 to integer variables a , b , c and d ; we can do it by following ...
The C language includes a set of preprocessor directives, which are used for things such as macro text replacement, conditional compilation, and file inclusion. ... When multiple assignment statements appear as subexpressions in a single larger expression, they are evaluated right to left. Comma operator expressions. The above list is somewhat ...
The compound-assignment operators combine the simple-assignment operator with another binary operator. Compound-assignment operators perform the operation specified by the additional operator, then assign the result to the left operand. For example, a compound-assignment expression such as. expression1 += expression2. can be understood as.
8.3 Logical Operators and Assignments. There are cases where assignments nested inside the condition can actually make a program easier to read. Here is an example using a hypothetical type list which represents a list; it tests whether the list has at least two links, using hypothetical functions, nonempty which is true if the argument is a nonempty list, and list_next which advances from one ...
This C Exercise page contains the top 30 C exercise questions with solutions that are designed for both beginners and advanced programmers. It covers all major concepts like arrays, pointers, for-loop, and many more. So, Keep it Up! Solve topic-wise C exercise questions to strengthen your weak topics.
1. "=": This is the simplest assignment operator. This operator is used to assign the value on the right to the variable on the left. Example: a = 10; b = 20; ch = 'y'; 2. "+=": This operator is combination of '+' and '=' operators. This operator first adds the current value of the variable on left to the value on the right and ...
Assignment performs implicit conversion from the value of rhs to the type of lhs and then replaces the value in the object designated by lhs with the converted value of rhs . Assignment also returns the same value as what was stored in lhs (so that expressions such as a = b = c are possible). The value category of the assignment operator is non ...
1.Simple Assignment or Basic Form: Simple Assignment or Basic form is one of the common forms of Assignment Statements in C program. Simple Assignment allow the programmer to declare,define and initialize and assign a value in the same statement to a variable. This form is generally used when the programmer want to use the Variable a few times.
Using , operator you can assign a value to a variable multiple times. e.g. K = 20, K = 30; This will assign 30 to K overwriting the previous value of 20. I thought it's invalid to change a variable more than one time in one statement. Yes it leads to undefined behavior if we try to modify a variable more than once in a same C statement but here ...
The concept of operator precedence and associativity in C helps in determining which operators will be given priority when there are multiple operators in the expression. It is very common to have multiple operators in C language and the compiler first evaluates the operater with higher precedence. It helps to maintain the ambiguity of the ...
Examples of assignment statements, b = c ; /* b is assigned the value of c */. a = 9 ; /* a is assigned the value 9*/. b = c+5; /* b is assigned the value of expr c+5 */. The expression on the right hand side of the assignment statement can be: An arithmetic expression; A relational expression;
The object is to illustrate how to structure a C program composed of multiple files. Having already established that I want to write a program that encodes and decodes files in MeowMeow format, I fired up a shell and issued the following commands: $ mkdir meowmeow. $ cd meowmeow. $ git init.
Types of Assignment Operators in C. Here is a list of the assignment operators that you can find in the C language: Simple assignment operator (=): This is the basic assignment operator, which assigns the value on the right-hand side to the variable on the left-hand side. Example: int x = 10; // Assigns the value 10 to the variable "x"
6.1. Multiple assignment. I haven't said much about it, but it is legal in C++ to make more than one assignment to the same variable. The effect of the second assignment is to replace the old value of the variable with a new value. The active code below reassigns fred from 5 to 7 and prints both values out. The output of this program is 57 ...
C Structures. The structure in C is a user-defined data type that can be used to group items of possibly different types into a single type. The struct keyword is used to define the structure in the C programming language. The items in the structure are called its member and they can be of any valid data type.
Dec 31, 2012 at 21:39. 1. It may be considered bad style because it's a bit more taxing to read (at 2am when you're debugging to a deadline) than three simple assignments. On the other hand, one line is less than three, so it can be argued both ways. - Omri Barel. Dec 31, 2012 at 21:40.