Programming Assignment 2 CS 12300 Programming I: Java Fall, 2020
This assignment is due Thursday, September 10.
This assignment makes use of the files contained in this zip file .
In the zip file there is a Java class called Hw2_long.java that prints the following picture. Your assignment is to write a Java program called Hw2.java that prints the exact same picture, but uses "variable abstraction" to make the program much shorter.
++++++++++++++++++++************++++++++++++++++++++ ==================== ==================== ==================== ==================== ==================== ==================== ==================== ==================== ==================== ==================== ==================== ==================== ==================== ==================== ==================== ==================== ==================== ==================== ==================== ==================== ==================== ==================== ==================== ==================== ++++++++++++++++++++____________++++++++++++++++++++ ++++++++++++++++++++************++++++++++++++++++++ ==================== ==================== ==================== ==================== ==================== ==================== ==================== ==================== ==================== ==================== ==================== ==================== ==================== ==================== ==================== ==================== ==================== ==================== ==================== ==================== ==================== ==================== ==================== ==================== ++++++++++++++++++++____________++++++++++++++++++++
Remember that "variable abstraction" is the idea of giving a name to a value that we want to use, and reuse, many times. For example, the string "++++++++++++++++++++" appears several times in the above picture. So we might want to give it a name.
Notice how the single letter s now refers to a twenty letter string. You can get a short program to produce a long output by defining and using String variables that have short names but represent long parts of the desired output.
The file Hw2_long.java in the zip file has 2,581 characters in it. You find that out by right clicking on the file and choosing the "Properties" menu item. For this assignment, write a Java program Hw2.java that outputs the above picture, but your Java program should have no more than 600 characters in it (you check this by right clicking on your file Hw2.java and choosing the "Properties" item from the popup menu).
Your Hw2.java program is supposed to be much shorter than the Hw2_long.java program, but your Hw2.java program should still be a properly formatted and indented Java program with your name and email address at the beginning.
Write a second program, called Hw2_short.java , that also outputs the same picture as Hw2_long.java , but your Hw2_short.java does not need to be nicely formatted. The goal of your Hw2_short.java program is to produce the given output with the shortest program that you can come up with. For example, I have a version of Hw2_short.java that contains 220 characters. Your Hw2_short.java program must compile, run, and produce the correct output, but other than that, you can break any Java rule you want in order to get the program shorter.
Here is an example of something you can do to shorten a program. Suppose you need to declare two string variables.
You can combine these two declarations into one declaration.
Notice that you only need to declare String one time, and you replace one semicolon with a comma.
You can also remove "un-needed" spaces.
In the zip file there is a program called Hw2_short_demo.java that has only 142 characters in it but it produces 1,024 output characters. Look at how it uses variables to amplify its output (and notice that it is kind of unreadable). Here is a puzzle. Can you modify Hw2_short_demo.java so that it is a shorter program but it produces a longer output?
Turn in a zip file called CS123Hw2Surname.zip (where Surname is your last name) containing your versions of Hw2.java and Hw2_short.java .

- $ 0.00 0 items

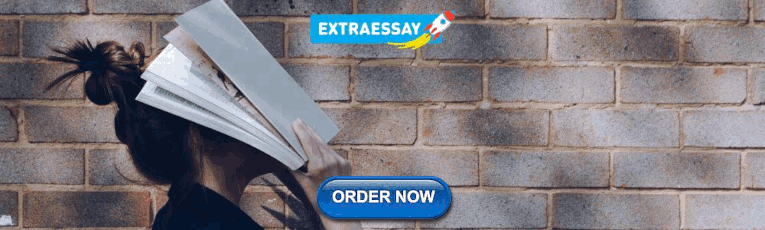
CSCI1100 Homework 2 Strings and Functions solved
$ 29.99
Description
Overview This homework is worth 75 points total toward your overall homework grade (each part is 25 points), and is due Thursday. There are three parts to the homework, each to be submitted separately. All parts should be submitted by the deadline or your program will be considered late. Note on grading. Make sure you read the comments from Homework # 1. They apply to this and all future homeworks and will be of increasing importance. In all parts of the homework, we will specify which functions you must provide. Make sure you write these functions, even if they can be very simple. Otherwise, you will lose points. We will write more complex functions as the semester goes on. In addition, we will also look at program structure (see Lecture 4), and at the names of variables and functions in grading this homework. Overall, autograding will account for 60 out of the 75 available points (20 points/part). The remaining 15 points (5 points / part) will be assigned by your TA based on program structure and understandability. The homework submission server URL is below for your convenience: https://submit.cs.rpi.edu/index.php?course=csci1100 Part 1: Geometry and breathing! This problem is a warm up of the use of functions. You will be asked to write a few simple functions. A number of countries and companies are planning manned trips to Mars in the next few decades. Let’s think ahead to one of the more important considerations for the trip – breathable air. Now, the space station gets most of its oxygen from the electrolysis of water and I expect that a trip to Mars will use a similar method, but what if we needed to carry all the oxygen needed along with the astronauts? How much oxygen would we need to carry? We will make some simplifying assumptions: the capsule is a sphere, 41% of the oxygen in the capsule is used each day, and oxygen is stored in a set of cylindrical tanks (essentially SCUBA tanks). These tanks are pressurized to 3000 psi, which means that each reservoir tank holds 210 times its internal volume of gas. Your problem is to determine the total volume of oxygen needed for the trip, the amount of oxygen per tank, and therefore the number of oxygen tanks. Your program will ask the user for the dimensions of the spherical capsule (radius) and the dimensions of the reservoir cylinder, again (radius, height), reading these through input. The program will then produce the results as follows Calculate and output the total amount of oxygen needed during the journey to Mars. This is the volume in the spherical capsule times the amount of oxygen in the air (21%) times the percent of the oxygen used each day (41%) times 300 days for the one way trip. Remember the volume of a sphere is given by pi* radius**3 * 4/3. You must write a function volume_sphere(radius) to compute this and use it in your computation. You must use the math module for the value of pi. Calculate and output the amount of oxygen held in each cylindrical tank with the given dimensions radius, height. Remember, the volume of a cylinder is given by pi* radius**2 * height. Write a function volume_cylinder(radius, height) that finds and returns the volume of a cylinder with the given measurements. (Here you are welcome to make use of the code from the lecture notes!) Output the amount of oxygen the cylinder holds at 3000 psi (210 times the volume of the cylindrical tank). You must use the math module for the value of pi. Calculate and output the number of oxygen tanks the capsule will need to carry with it on the journey. Always choose the next largest whole number, so 0.2 tanks becomes 1 tank, while 1 tank remains 1 tank. Look at the ceil function in the math module to help you do this. As a final note, assume that all of the oxygen needed is contained in the reservoirs. In other words, you do not need to account for the oxygen already in the capsule at the start of the journey, nor do you need to be concerned with the amount of oxygen left in the tank at the end. Here is example output of your program (how it will look on Wing IDE): Radius of capsule (m) == 2.5 2.5 Radius of oxygen reservoir (m) == 0.093 0.093 Height of oxygen reservoir (m) == 0.633 0.633 Oxygen needed for the trip is 1690.570m^3. Each cylinder holds 3.612m^3 at 3000 psi. The trip will require 469 reservoir tanks. Remember, you will need to use formating strings to generate the output in exactly the form we have shown it above. This is necessary to match our output. When you have tested your code, please submit it as Part 1 of HW 2. Part 2: A simple trick (numerical functions)! The original of this simple parlor trick is attributed to Albert Einstein, but it probably dates to an earlier date! We’ve modified it a little to test a few more math functions and give a little more challenge. The trick: First, write the number 1089 on a piece of paper, fold it, and put it away. Next, ask your friend to write down a five-digit number, emphasizing that the first and third digits must differ by at least two. Don’t watch your friend doing the arithmetic. After your friend has written down the five-digit number, ask them to reverse it, take the first three digits of the original number and the last three digits of the reversed number, then subtract the smaller from the larger. Example: 32156 reversed is 65123 321 – 123 = 198. Tell your friend to reverse the new number. Example: 198 becomes 891. Next ask your friend to add the new number and its reverse together. Example: 198 + 891 = 1089. If all goes as planned, your friend will be amazed. The number you wrote down at the start –1089– will always be the same as the end result of this mathematical trick. Your job is to write a program that mimics this parlor trick by first predicting the outcome, then requesting a five-digit number from the user, applying the steps above, outputing the steps as it goes, and checking the result to make sure it is indeed 1089. When looking at a problem like this, it is important to try to think about the central issue — the most challenging question — and try to solve it first and check your solution. Here, the most difficult question for us is reversing the digits in an integer. There are a number of ways to do this, but we want you to think about it by making use of integer division and integer remainders (the // and % operators). Here is code that will help you get started and help you understand as well how to grab the first three digits: x = 14926 x // 100 # Gets the first three digits 149 x % 100 # Gets the last two digits 26 y = 75 # We are going to reverse a two digit number tens = y//10 # Gets the tens digit tens 7 ones = y%10 # Gets the ones digit ones 5 ones*10 + tens # Gives us the reversed number 57 Start your programming effort by writing and testing a function called reverse3 that calculates and returns the reverse of a three digit number. Test this function carefully. For example reverse3(123) 321 Next, write and test a function called reverse5 that calculates and returns the reverse of a five digit number. Test this function carefully. For example, reverse5(26417) 71462 With these two functions done, write the complete program to mimic the parlor trick. The program should the produce output given below by asking the user for a five-digit integer, and then carrying out the computation outlined above, using the two reverse functions as needed. The program should output the steps of the computation. Then, add a print statement at the end of your program to say “You see, I told you”. Your output must match the following (this is how it will look on Wing): Enter a 5 digit number whose first and third digits must differ by at least 2. The answer will be 1089, if your number is valid Enter a value == 29467 29467 Here is the computation: 29467 reversed is 76492 492 – 294 = 198 198 + 891 = 1089 You see, I told you. When you have tested your code, please submit it as Part 2 of HW 2. Part 3: Find the hidden message! (string functions and an if) Write a program that either encrypts or decrypts a string, depending on the choice that the user makes. First, the program should ask the user whether they want to encrypt (’E’) or decrypt (’D’), then it should ask the user for a sentence using (input) written in a cipher to decrypt, or in clear text to either encrypt (if ’E’ was input) or decrypt. The program should then carry out the encrypt or decrypt operation using the rules described below and print the resulting sentence. For both encrypt and decrypt, the program should print the difference in length between the cipher and clear text versions. The difference should always be printed as a positive number. The ’E’ for encrypt, or ’D’ for decrypt should be case insensitive, which means that ’e’ means encrypt, just like ’E’ does. If something other than ’E’ or ’D’ is entered in respone to the first query, just print “I didn’t understand … exiting” and exit the program. Processing the input will require an if/elif/else block. We will cover if/elif/else on Monday. Until then, you can write the encrypt and decrypt functions and apply both to any string that is given. Do not let the fact that we have not covered if keep you from getting a jump on this homework. All of parts 1 and 2 can be completed as well as most of part 3. Here is a series of examples running of the program (what you will see on Wing): Run 1: Enter ‘E’ for encrypt or ‘D’ for decrypt == d d Enter cipher text == wh*%$ s7654 s2(*2(ri7654@@@s wh*%$ s7654 s2(*2(ri7654@@@s Deciphered as == why so serious Difference in length == 14 Run 2: Enter ‘E’ for encrypt or ‘D’ for decrypt == e e Enter regular text == back off man I am a scientist back off man I am a scientist Encrypted as == back 7654ff m-? I%4%m%4% sci2(*2(ntist Difference in length == 9 Run 3: Enter ‘E’ for encrypt or ‘D’ for decrypt == D D Enter cipher text == wh*%$ s7654 s2(*2(ri7654@@@s wh*%$ s7654 s2(*2(ri7654@@@s Deciphered as == why so serious Difference in length == 14 Run 4: Enter ‘E’ for encrypt or ‘D’ for decrypt == E E Enter regular text == back off man I am a scientist back off man I am a scientist Encrypted as == back 7654ff m-? I%4%m%4% sci2(*2(ntist Difference in length == 9 Run 5: Enter ‘E’ for encrypt or ‘D’ for decrypt == a a I didn’t understand … exiting The encryption rules are based on a set of string replacements, they should be applied in this order exactly: ‘ a’ = ‘%4%’ replace any a after a space with %4%. ‘he’ = ‘7!’ replace all occurrences of string he with 7! ‘e’ = ‘9(*9(‘ replace any remaining e with 9(*9( ‘y’ = ‘*%$’ replace all occurrences of string y with *%$ ‘u’ = ‘@@@’ replace all occurrences of string u with @@@ ‘an’ = ‘-?’ replace all occurrences of string an with -? ‘th’ = ‘!@+3’ replace all occurrences of string th with !@+3 ‘o’ = ‘7654’ replace all occurrences of string o with 7654 ‘9’ = ‘2’ replace all occurrences of string 9 with 2 For example the cipher for methane is m2(*2(!@3-?2(*2(+. Here is how we get this: ‘methane’.replace(‘e’,’9(*9(‘) ‘m9(*9(than9(*9(‘ ‘m9(*9(than9(*9(‘.replace(‘an’,’-?’) ‘m9(*9(th-?9(*9(‘ ‘m9(*9(th-?9(*9(‘.replace(‘th’,’!@+3′) ‘m9(*9(!@+3-?9(*9(‘ ‘m9(*9(!@+3-?9(*9(‘.replace(‘9’, ‘2’) ‘m2(*2(!@+3-?2(*2(‘ Decrypting will involve using the rules in reverse order. Your program must use two functions: Write one function encrypt(word) that takes as an argument a string in plain English, and returns a ciphered version of it as a string. Write a second function decrypt(word) that does the reverse: takes a string in cipher and returns the plain English version of it. Both functions will be very similar in structure, but they will use the string replacement rules in different order. You can now test whether your functions are correct by first encrypting a string, and then decrypting. The result should be identical to the original string (assuming the replacement rules are not ambiguous). Use these functions to implement the above program. When you have tested your code, please submit it as Part 2 of HW 2. Finished and still want extra challenges to sharpen your programming skills? These are not extra credit but for you to do additional practice. Revise part 2 so that if the person enters a number that is not valid (i.e. first and third digit do not differ by two), you immediately print a statement informing the user and do not compute anything. Otherwise, you carry out the above computation. Revise part 3 so that it takes an input sentence, encrypts it and then decrypts it, and then checks whether the result is the same. It should print “same” if they are the same and “different” otherwise.
Related products

CSCI1100 Lab 4 — Images and Modules solved
Csci1100 lab 6 — sudoku solved.

CSCI1100 Homework 1 Calculations and Strings solution

CS 240: Software Engineering • Fall 2014 • University of Puget Sound
Quick Links: Home • Calendar • Homeworks • Project • Resources
CS 240 Homework 2 - Ruby
Due fri sep 19 sun sep 21 at 11:59pm.
In this assignment you will do some simple programming exercises to get familiar and comfortable with the Ruby language. You will also practice using the testing tool RSpec to automatically test and grade your code.
- Practice writing Ruby code, methods, and classes.
- Practice running RSpec tests on your code
Necessary Files
You will want to get the starter code and instructions from the GitHub homework repository. Be sure and pull the latest version of the homework upstream repo, per the instructions in Hwk1 . The starter code you're looking for is in the hwk2 folder.
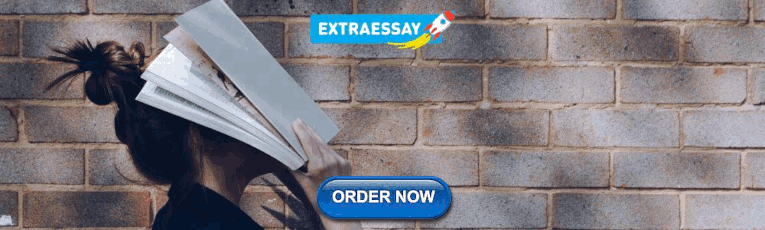
Assignment Details
If you have time, it may be helpful to go through a tutorial on using Ruby. I recommend either Ruby in Twenty Minutes or the Codecademy Ruby course .
The goal of this multi-part assignment is to get you accustomed to basic Ruby coding and introduce you to RSpec, the unit testing tool we will be using heavily.
RSpec is a tool testing Ruby code (see Sections 8.1 and 8.2 of the text). It lets you define a series of tests (a "spec") which can be run to tell you if your code does what it is supposed to. While I will provide explanations of what your code should do, you should get accustomed to the idea that with Test-Driven Development, the true specification for code is in these test files!
I have provided test files for all of the code you need to write in this assignment: you can thus check your work by running the appopriate spec file.
- You need to have rspec 2.14 installed for these spec files to work properly.
- Initially, are the tests are marked as "pending" (that is, they shouldn't be run repeatedly because there isn't code yet!), as indicated by the argument :pending => true in each describe block. To start testing a question, remove this option: e.g., in warmup_spec.rb , change line 7: describe " #hello " , :pending => true do to describe " #hello " do
- You can use autotest to automate the process of running these spec files. Simply run the command autotest from the command line (in the top level of the hwk2 directory), and it will re-run all the RSpec tests each time you make changes to your code.
- Note that all the spec files contains a number of test cases. At a minimum, all should pass when you submit your code. I may run additional cases as well.
Appetizer: Warmup
Specs: spec/warmup_spec.rb
In the first part of the assignment you'll write some simple Ruby methods. Check the documentation for Array , Hash , Enumerable , and String , as they could help tremendously with these exercises.
- Define a method hello(name) that takes a string representing a name and returns the string "Hello, " concatenated with the name.
- Define a method sum which takes an array of integers as an argument and returns the sum of its elements. For an empty array it should return zero.
- Define a method max_2_sum which takes an array of integers as an argument and returns the sum of its two largest elements. For an empty array it should return zero. For an array with just one element, it should return that element.
- Define a method sum_to_n? which takes an array of integers and an additional integer, n , as arguments and returns true if any two distinct elements in the array of integers sum to n . An empty array or single element array should both return false.
Entree: Fun With Strings
Specs: spec/fun_with_strings_spec.rb
In the second part of the assignment, you'll implement some functions that perform basic string processing. You should start from the provided fun_with_strings.rb template. This arranges to mix your methods into the String class, so that methods can be called like
A palindrome is a word or phrase that reads the same forwards as backwards, ignoring case, punctuation, and nonword characters. (A "nonword character" is defined for our purposes as "a character that Ruby regular expressions would treat as a nonword character".)
Write a method palindrome? that returns true if and only if its receiver is a palindrome.
Your solution shouldn't use loops or iteration of any kind. Instead, you will find regular-expression syntax very useful; it's reviewed briefly in the textbook, and the website rubular.com lets you try out Ruby regular expressions "live". Some methods that you might find useful (which you'll have to look up in Ruby documentation at ruby-doc.org ) include: String#downcase , String#gsub , and String#reverse .
Define a method count_words that, given an input string, return a hash whose keys are words in the string and whose values are the number of times each word appears:
Your solution shouldn't use for-loops, but iterators like each are permitted. As before, nonwords and case should be ignored. A word is defined as a string of characters between word boundaries.
An anagram group is a group of words such that any one can be converted into any other just by rearranging the letters. For example, "rats", "tars" and "star" are an anagram group.
Given a String that is a space separated list of words, write a method that groups them into anagram groups and returns the array of groups. Case doesn't matter in classifying string as anagrams (but case should be preserved in the output), and the order of the anagrams in the groups doesn't matter.
Dessert: Basic Object-Oriented Programming
Specs: spec/dessert_spec.rb
In the third part of this assignment, you'll develop a simple class and do some basic object-oriented programming.
- Create a class Dessert with getters and setters for name and calories. The constructor should accept arguments for name and calories.
- Define instance methods healthy? , which returns true if and only if a dessert has less than 200 calories, and delicious? , which returns true for all desserts.
- Create a class JellyBean that inherits from Dessert . The constructor should accept a single argument giving the jelly bean's flavor; a newly-created jelly bean should have 5 calories and its name should be the flavor plus "jelly bean", for example, "strawberry jelly bean".
- Add a getter and setter for the flavor.
- Modify delicious? to return false if the flavor is licorice , but true for all other flavors. The behavior of delicious? for non-jelly-bean desserts should be unchanged.
Coffee: Ruby Metaprogramming
Specs: spec/attr_accessor_with_history_spec.rb
In the last part of the assignment you will perform some in-depth metaprogramming to extend Ruby classes. Note that this part is conceptually challenging but not programmatically complex.
The textbook discusses how attr_accessor uses metaprogramming to create getters and setters for object attributes on the fly. Define a method attr_accessor_with_history that provides the same functionality as attr_accessor but also tracks every value the attribute has ever had:
Calling bar_history before bar 's setter is ever called should return nil .
History of instance variables should be maintained separately for each object instance. that is:
then the last line should just return [nil,3] , rather than [nil,1,3] .
If you're interested in how the template works, the first thing to notice is that if we define attr_accessor_with_history in class Class , we can use it as in the snippet above. This is because a Ruby class like Foo or String is actually just an object of class Class . (If that makes your brain hurt, just don't worry about it for now. It'll come.)
The second thing to notice is that Ruby provides a method class_eval that takes a string and evaluates it in the context of the current class, that is, the class from which you're calling attr_accessor_with_history . This string will need to contain a method definition that implements a setter-with-history for the desired attribute attr_name . In short: this method will contain inside it the code to define an accessor for the arbitrary variable with attr_name , where the name of the variable is itself a variable. Wow. Much meta.
If you think of Generics in Java, where the "type" of the generic is itself a variable, you can get a feel for what is going on here.
- Note that one powerful metaprogramming feature in Ruby is class_eval that can be called in the meta-class Class. class_eval can interpret a string on the fly to create some new code. In the example below, we define add_method() to the meta-class (and, through inheritance, to any class). When called, this method defines a new method that returns a string saying hello to some name (notice how #{name} gets replaced with the parameter passed to add_method, so that calling add_method(hello_joel) causes class_eval to execute the code def hello_joel() ... ). class Class def add_method (name) class_eval %Q{ def hello_ #{ name } () 'hello #{ name } ' end } end end class MyClass add_method :hello_joel end mc = MyClass .new puts mc.hello_joel # => 'hello joel'
- Don't forget that the very first time the attribute receives a value, its history array will have to be initialized.
- An attribute's initial value is always nil by default, so if foo_history is referenced before foo has ever been assigned, the correct answer is nil (no history), but after the first assignment to foo , the correct value for foo_history would be [nil] (it has history, but that history was nothing).
- Don't forget that instance variables are referred to as @bar within getters and setters, as Section 3.4 of the ESaaS textbook explains.
- Although the existing attr_accessor can handle multiple arguments (e.g. attr_accessor :foo, :bar ), your version just needs to handle a single argument.
- Your implementation should be general enough to work in the context of any class and for attributes of any (legal) variable name. This is easier than it may seem, and comes for free with good metaprogramming practice.
Make sure that your code passes all tests, and then push your code out to your personal homework repo (which you set you in Homework 1).
Each part of this assignment will be graded out of 10 points, based on the number of tests that pass.
Joel Ross • [email protected]
Assignment by Patterson and Fox
Page Last Modified: 2014-09-21 07:47:15 -0700
Mathematics and Computer Science Department • University of Puget Sound
Search code, repositories, users, issues, pull requests...
Provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
Scupus/homework_strings
Folders and files.
- Java 100.0%
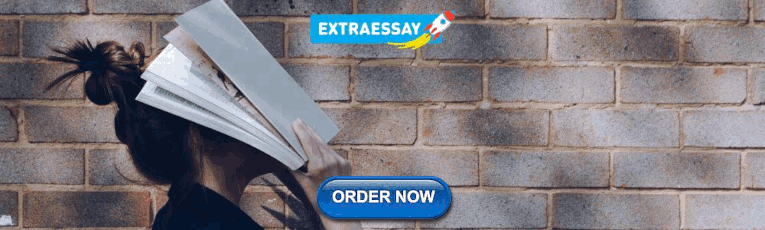
IMAGES
VIDEO
COMMENTS
Introduction to Java & Object Oriented Programming Homework 2: Custom String This assignment is designed to give you practice writing code and applying lessons and topics for the current module. This homework deals with the following topics: The Java Language Strings Unit Testing The Assignment In this assignment, you will implement a simple class called CustomString.
Step 2:This is a Java class named CustomString that represents a custom string data type. The class has two instance variables: myString: This is a String variable that holds the value of the current string. isSet: This is a boolean variable that indicates whether the value of myString has been set or not. The class has the following methods:
This class represents a more customizable version * of a String, with additional attributes and methods. * For example, the CustomString class has a "reverse" method which returns a new string version of the current string where the * capitalization is reversed (i.e., lowercase to uppercase and uppercase to lowercase) for the alphabetical ...
Answered by premhessa on coursehero.com. for the solution. below are 2 files. CustomString.java. CustomStringTest.java. also i have added 3 extra test cases to each unit test. also i have attached the screenshot to successfull passing of every unit tests. Note : the methods in CustomString have been designed in a manner that they don't affect ...
For example, given constants BASE=2 and LIMIT=100000 and input i= 3 , your function should initially display 2 3= 8. Upon a key pressed, it displays 2 4= 1 6, upon the next key: 2 5= 3 2, then 2 6= 6 4, and so forth. Stop when the term exceeds 100000. Use the expt function to compute the initial World state, i.e. BASE i.
Here's some suggestions: Header. #include <ostream> incurs a lot of overhead. You do not need the whole std::ostream in the header.#include <iosfwd> is enough. It's std::size_t, not size_t.Also, you forgot to #include <cstddef>.. asCString fails to propagate const.. Not being implicitly convertible to const char* is one of the basic benefits of proper containers over raw arrays.
(* if you use this function to compare two strings (returns true if the same string), then you avoid several of the functions in problem 1 having polymorphic types that may be confusing *) fun same_string(s1 : string, s2 : string) = s1 = s2 (* put your solutions for problem 1 here *) (* a. *) fun all_except_option (inputString ...
CS 12300. Programming I: Java. Fall, 2020. This assignment is due Thursday, September 10. This assignment makes use of the files contained in this zip file . In the zip file there is a Java class called Hw2_long.java that prints the following picture. Your assignment is to write a Java program called Hw2.java that prints the exact same picture ...
Homework 2-6 1 Dec, 9 AM Introduction There are two parts to HW2-6. Part One is the continuation of HW2-5, where we'll build on printConcordance(). Part Two will dicuss regexes. ... Form a string that spans the position. For example, it could span 20 characters of either side of where the word begins.
1.Print the strings back out. 2.Print the length of the second string. 3.Print the rst string concatenated with the second. 4.Print true if the rst and second strings are the same, and false otherwise. 5.Print the rst index where the character c appears in the rst string (or -1 if it doesn't).
Question: Question In this exercise, you will be writing a CH program that defines a custom string class. You will write the program into separate files. You are provided with a codebase containing a class named CustomString consisting of an attribute of type string, named data, several methods, and operators.
CS3200: Programming Languages Homework 2 Spring 2020 Homework 2 1. Write regular expressions to capture the following regular languages: (a) The set of binary strings which have an even number of 0's or which contain three consecutive 1's somewhere in the string (or both). Hint: this is an OR, so build the two
This is a homework problem, so I'm not looking for direct answers, but I am quite lost as to where to even begin with this problem. I am tasked with creating my own implementation of the StringBuilder class called MyStringBuilder. ... Custom 'String' Class . 2. Setting a StringBuilder. 0. What are the best practices for building strings in the ...
CS3200: Programming Languages Homework 2 Spring 2018 Homework 2 1. Write regular expressions to capture the following regular languages: (a) The set of binary strings that either contain an even number of 0's, or contain an odd number of 1's (or both). (b) All strings of lower case letters where all ve vowels appear once and are in alphabetical
Description. This homework is worth 75 points total toward your overall homework grade (each part is 25 points), and is due Thursday. There are three parts to the homework, each to be submitted separately. All parts should be submitted by the deadline or your program will be considered late. Note on grading.
The goal of this project is to expand your submission for Homework #1 to use arrays instead of having to use the SubString method for strings. See the guidelines for Homework #1 on the course webpage. In short, this is what I want you to do. I want you to convert the string that the user enters into a character array.
Saved searches Use saved searches to filter your results more quickly
HW2 - Free download as PDF File (.pdf), Text File (.txt) or view presentation slides online. This document contains instructions for two programming exercises. The first exercise prompts the user to enter their name and date of birth, then generates a password from parts of that information. The second exercise takes two integer command line arguments, calculates their sum, difference, product ...
CS 240 Homework 2 - Ruby Due Fri Sep 19 Sun Sep 21 at 11:59pm Overview. ... Given a String that is a space separated list of words, write a method that groups them into anagram groups and returns the array of groups. Case doesn't matter in classifying string as anagrams (but case should be preserved in the output), and the order of the anagrams ...
Scupus/homework_strings. This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository. master. Switch branches/tags. Branches Tags. Could not load branches. Nothing to show {{ refName }} default View all branches. Could not load tags. Nothing to show
Create custom format strings for a new .NET type, for use in String.Format? 1. padding / alignment in StringTemplate. 1. ... Is it ethical to study online solutions to for-credit math master's homework problems? Cruise with single engine on a twin, good idea? ...