C Programming Tutorial
- Variables in C
Last updated on July 27, 2020
Variables are used to store data, they are named so because their contents can change. C is a strongly typed language, it simply means that once you declare a variable of certain data type then you can’t change the type of the variable later in the program. Recall that C provides 4 fundamental types:
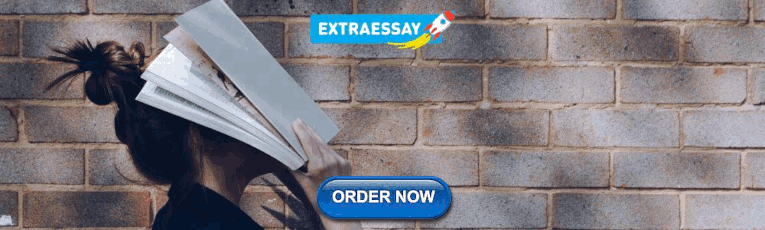
Declaring Variables #
Before you can use a variable you must first declare it. Declaring a variable involves specifying type and name of the variable. Always remember the rules of naming a variable is same as that for naming identifiers. The type and range of values variable can take depends on the type of the variable. Here is the syntax of variable declaration.
Syntax: datatype variablename;
Let's create declare a variable i .
Here i is declared as a variable of type int , so it can take only integral values, you can't use i to store a string constant. On a 16-bit system variable i can take values from -32768 to 32767 , while on a 32-bit system i can take values from -2147483648 to 2147483647 .
If you want you can declare multiple variables of the same type like this:
Here x , y , and z are of type int .
Initializing a variable #
When a variable is declared it contains an undefined value also known as Garbage value. If you want you can assign some initial value to the variable using assignment operator i.e ( = ). Assigning a value to the variable is called initialization of the variable. Here are some examples of variable initialization:
Note: In the last statement, only the d3 variable is initialized, d1 and d2 variables contain a garbage value.
Load Comments
- Intro to C Programming
- Installing Code Blocks
- Creating and Running The First C Program
- Basic Elements of a C Program
- Keywords and Identifiers
- Data Types in C
- Constants in C
- Input and Output in C
- Formatted Input and Output in C
- Arithmetic Operators in C
- Operator Precedence and Associativity in C
- Assignment Operator in C
- Increment and Decrement Operators in C
- Relational Operators in C
- Logical Operators in C
- Conditional Operator, Comma operator and sizeof() operator in C
- Implicit Type Conversion in C
- Explicit Type Conversion in C
- if-else statements in C
- The while loop in C
- The do while loop in C
- The for loop in C
- The Infinite Loop in C
- The break and continue statement in C
- The Switch statement in C
- Function basics in C
- The return statement in C
- Actual and Formal arguments in C
- Local, Global and Static variables in C
- Recursive Function in C
- One dimensional Array in C
- One Dimensional Array and Function in C
- Two Dimensional Array in C
- Pointer Basics in C
- Pointer Arithmetic in C
- Pointers and 1-D arrays
- Pointers and 2-D arrays
- Call by Value and Call by Reference in C
- Returning more than one value from function in C
- Returning a Pointer from a Function in C
- Passing 1-D Array to a Function in C
- Passing 2-D Array to a Function in C
- Array of Pointers in C
- Void Pointers in C
- The malloc() Function in C
- The calloc() Function in C
- The realloc() Function in C
- String Basics in C
- The strlen() Function in C
- The strcmp() Function in C
- The strcpy() Function in C
- The strcat() Function in C
- Character Array and Character Pointer in C
- Array of Strings in C
- Array of Pointers to Strings in C
- The sprintf() Function in C
- The sscanf() Function in C
- Structure Basics in C
- Array of Structures in C
- Array as Member of Structure in C
- Nested Structures in C
- Pointer to a Structure in C
- Pointers as Structure Member in C
- Structures and Functions in C
- Union Basics in C
- typedef statement in C
- Basics of File Handling in C
- fputc() Function in C
- fgetc() Function in C
- fputs() Function in C
- fgets() Function in C
- fprintf() Function in C
- fscanf() Function in C
- fwrite() Function in C
- fread() Function in C
Recent Posts
- Machine Learning Experts You Should Be Following Online
- 4 Ways to Prepare for the AP Computer Science A Exam
- Finance Assignment Online Help for the Busy and Tired Students: Get Help from Experts
- Top 9 Machine Learning Algorithms for Data Scientists
- Data Science Learning Path or Steps to become a data scientist Final
- Enable Edit Button in Shutter In Linux Mint 19 and Ubuntu 18.04
- Python 3 time module
- Pygments Tutorial
- How to use Virtualenv?
- Installing MySQL (Windows, Linux and Mac)
- What is if __name__ == '__main__' in Python ?
- Installing GoAccess (A Real-time web log analyzer)
- Installing Isso
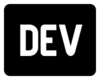
DEV Community

Posted on Jul 24, 2023 • Updated on Jul 29, 2023
A Comprehensive Introduction to Variables in C Programming
All C programs, whatever their size, consist of functions and variables. A function contains statements that specify the computing operations to be done, and variables store values used during the computation.
Variables are one of the basic data objects manipulated in a program. They make it easy for programmers to build programs that can store and retrieve data for different kinds of task with a minimal amount of code. Without Variables, it is difficult to get much done with your program.
In this article, you will learn the meaning of a variable in C programming, how to declare a variable and assign values to it. You will learn about the different types of variables and the range of values they can store. You will learn about the ASCII character set. And you will learn how to print the values stored in the different types of variables with the C standard library functions printf and putchar .
Table Of Content
Prerequisites.
- What is a variable in C
What is an Identifier
- Fundamental Types in C
How to declare a Variable in C
- What happens in your computer when you declare a Variable in C
- How to print the value in an integer type variable
Signed vs Unsigned Integer type
How to print the value in a character type variable, similarities between character constants and integer types, floating types.
To enjoy the full experience of this article, you should have the following:
- A basic understanding of how to compile a C source file using a compiler and how to run the output file of compiled C source file in a terminal.
- A basic understanding of how to use the printf function to print text
- A text editor
- A cup of water or coffee
Every code in this article is written to adhere to betty coding style and compiled on Ubuntu 20.04 LTS using gcc , using the options -wall -Werror -Wextra -pedantic -std=gnu89
What is a Variable in C?
In C, a Variable is a symbolic name that represents a particular storage location on the hard drive, or any memory device connected to the machine executing your program.
"In C, all variables must be declared before they are used, usually at the beginning of the Function before any executable statements." - The C programming language book (Brian W. Kernighan & Dennis M. Ritchie)"
To declare a Variable in C programming language, we first specify the Type of the Variable, and then its Identifier.
An identifier is any name you provide for Variables, Functions, Macros, and other data Objects in your C program. You're required to provide a valid Identifier.
The following list indicates the rules you must follow to construct a valid Identifier in C:
- It must start with an alphabet (the underscore _ counts as an alphabet).
- It must not have any spaces in between.
- It must be unique and must not be a Keyword in C.
- It can be made up of uppercase or lowercase alphabetic characters, the ten digits (0 - 9) and the underscore _
Uppercase and lowercase letters are distinct in C. Therefore sum , Sum , and SUM are different identifiers.
Programming best practices recommend that you construct identifiers that reflect their intended use. Also, a recommended naming convention amongst C programmers is to use only UPPERCASE letters to construct identifiers that represent Macros
What is a Type
A Type is a specification that describes the kind of values an identifier can store or manipulate.
C is a statically typed language. This means all identifiers must be associated with a Type.
The Type specified for an Identifier indicates the following to the compiler:
- The kind of values that are acceptable to be stored through the Identifier
- The acceptable range of values that can be stored through the Identifier.
- The amount of storage space that should be allocated for the Identifier.
- The arithmetic computation that should be allowed to be performed with the Identifier.
If any of the above conditions cannot be satisfied for the Type you have specified for an Identifier, the compiler generates an error message.
Fundamental Types In C
C supports three (3) fundamental Types:
- Integer types
Character types
To specify any of the fundamental types in a variable declaration, we use the dedicated keywords provided for each Type in the C standard:
- int is the keyword used to specify the Integer type.
- float is the keyword used to specify the Floating type.
- char is the keyword used to specify the Character type.
You might have heard or seen people use the term Datatype and wonder what the difference is between Datatype and Type. Although both terms can be used interchangeably, they have slightly different meanings; Type refers to a specification while Datatype refers to data that fits a Type specification.
So when we specify a Type in a variable declaration, we're instructing the compiler to make a variable that can store Datatypes that fits the specifications of the Type specified for the Variable.
To declare a variable in C programming, we first specify the Type of the variable, and then its Identifier. For example, we might declare variables "height" and "profit" as:
The first declaration states that height is a variable of Type int , which means that height can store whole numbers. The second declaration states that profit is a variable of Type float , which means that profit can store numbers with digits after the decimal point.
After a variable has been declared, it can be initialized (given a value) by means of assignment:
What happens in your computer when you declare a variable in C
When you declare a variable in C programming, you essentially introduce the variable to the program. This declaration informs the compiler to allocate storage space for the variable on the hard drive of the computer executing the program.
While compiling your program, the compiler generates machine code that will instruct your computer to allocate a designated portion of memory on its hard drive for every declared variable in your program. The compiler then subsequently assigns corresponding Identifiers to the allocated memory locations. This allocated memory serves as storage for the values assigned to the variables.
The CPU architecture of your computer determines the precise amount of memory that will be allocated for each variable's Type. Different CPU architectures have distinct memory allocation specifications for the fundamental C Types.
Memory allocation specifications are generally defined in Bytes but machines translate memory allocation in Bits .
The following table shows the memory that will be allocated for variables declared with the fundamental C types on most 64-bit Linux machines:
To obtain the memory allocation specifications for each of the fundamental C Types on your computer, pass the Type keyword as an argument to sizeof (a C built-in operator which measures the amount of storage required for a Type) and print its return value with the printf function.
Let's create a program that prints out the memory allocation specification for each of the fundamental C types on our computer:

We use a format specifier with the printf function to print the value stored in a variable. In the above code snippet, the format specifier %lu is used in the printf function to print the value returned by the sizeof function because its return value is an unsigned Integer type (more details about this Integer type will be discussed soon).
Importance of specifying a Type for a variable declaration
The Type specified for a variable determines the range of values that can be stored through the variable.
C takes portability (the ability of your program to compile on different kinds of machines) seriously and bothers to tell you what ranges of values are guaranteed to be safe for each Type. In the following sections, you will learn more about each Type and its guaranteed range of values.
Integer type
An Integer type variable will store integer constants. int is the keyword that is used to specify the Integer type.
An integer constant consists of a sequence of one or more digits. A minus sign before the sequence indicates that the value is negative. No embedded spaces are permitted between the digits, and values larger than 999 cannot be expressed using commas. C allows integer constants to be written in decimal notation (base 10), octal notation (base 8), or hexadecimal notation (base 16).
Decimal notation of integer constants contains numbers between 0 and 9 but they must not start with a zero:
Octal notation of integer constants contains only numbers between 0 and 7, and must start with a zero:
Hexadecimal notation of integer constants contains numbers between 0 and 9 and letters between a and f, and must start with 0x:
Integers are always stored in binary regardless of what notation we have used to express them in our codes. These notations are nothing more than an alternative way to write numbers.
An Integer type variable can be declared as one of two forms; signed or unsigned . By default, a variable declared with the int keyword is a signed Integer type variable. This means such a variable can store either negative or positive integer constants.
Let's create a program that assigns a random number to a variable. The randomly assigned number could be negative or positive. The program will be able to store a different value every time we will run it:
The focus of the above code snippet is to show you that the variable n declared with the int keyword is a signed integer type variable. You don't have to understand what rand , srand , and RAND_MAX do.

From the output, you can see every time the program (output file of the compiled source code) is executed, it prints a different random value (either positive or negative). The random value printed is first stored in the variable n . Then n is used in the if/elif/else conditional block to determine which printf statement should be executed.
To declare an unsigned Integer type variable, we use the unsigned int keyword. An unsigned Integer type variable can only store positive integer constants. (You can test this by changing the Type keyword in the declaration of the variable n from int to unsigned int . Then recompile the program and run it).
For both signed and unsigned integer types, C provides 3 subtypes to allow us to construct an Integer type variable that meets our needs. The following keywords are used to specify each of the subtypes:
- unsigned short int
- unsigned int
- unsigned long int
C allows us to abbreviate these Integer subtypes keywords in our codes by dropping the word int . For example, unsigned short int can be abbreviated to unsigned short and long int can be shortened to just long . Omitting int this way is a widespread practice among C programmers.
The integer Type you specify in a variable's declaration determines the memory allocation and the range of values that can be stored through the variable. The range of values represented by each integer Type and their memory allocation varies from machine to machine. The following table shows the memory allocation for each integer Type and the range of values that they represent on most 64-bit Linux machines:
How to print the value in an Integer type Variable
The following table shows each Integer subtype and its printf format specifier:
One way to determine the value ranges of the integer types on our computer's implementation is to check the limits.h header, which is part of the standard library. The limits.h header defines macros (Identifiers that represent statements or expressions) that represent the smallest and largest values of each Integer type.
Let's create a program that assigns to variables, macros defined for each integer type in the limits.h header. And then prints out the values stored in each of the variables using the format specifier for their type:

In C programming, unsigned Integer type variables are preferred over signed Integer type variables because unsigned integers are more efficient . Also, unsigned Integers produce defined results for modulo-arithmetic overflow .
A Character type variable will store character constants. char is the keyword that is used to specify the Character type.
In C programming, a single character in a single quote '' forms a character constant.
A character constant is a character that is represented in a character set. A character set is an encoding scheme used to represent text characters with numeric codes that computers understand.
The American Standard Code for Information Interchange(ASCII) is a popular character set used as a standard for character encoding in computers and communication systems. It uses integer constants to encode text characters. ASCII is the underlying character set on most Linux/Unix-like machines.
The following characters are represented in ASCII :
- Character constants of uppercase and lowercase letters of the alphabet.
- Character constants of digits 0 to 9
- Character constants of C special characters.
ASCII is capable of representing 128 different characters:
It is important to note that in C programming, character constants are single characters enclosed in single quotes, not double quotes: 'b' is not the same as "b"
C also considers its special characters as single characters, which is why '\n' (a special character used to inform printf to print a new line) can be a character constant.
%c is the printf format specifier used to print any character constant stored in a char type Variable.
Let's create a program that assigns a character constant to a character type variable and prints the value stored in a Character type variable:

Another way to print character constants is to use the putchar function instead of printf . putchar is a standard library function that can take a single character constant as an argument and print it.
Let's create a program that uses putchar to print all letters of the alphabet in lowercase and then a new line:

putchar can also take as an argument, the integer value that represents a character constant in ASCII and then print the character constant.
Let's create a program that uses putchar to print all single-digit numbers of base 10 starting from 0 and then a new line:

C treats character constants as small Integers, which means character constants can be used in arithmetic computation. When a character constant appears in an arithmetic computation, C simply uses the Integer value that represents the character constant in the underlying character set (which is most likely ASCII ) on the machine executing the program. This opens up the possibility to convert digits represented as strings to integers and vice versa.
Let's create a program that does a simple arithmetic computation with character constants and prints the result of the computations:

In the above code snippet, the subtraction in the line num = '3' - '0'; , is performed to convert the character constant representation of the digit 3 to its decimal integer constant representation. In ASCII encoding, the character constants '0' to '9' are represented by consecutive integer values. The character constant '0' has an ASCII value of 48 , '1' has a value of 49 , '2' has a value of 50 , and so on. Subtracting the ASCII value of '0' (48) from the ASCII value of '3' (51) gives us 51 - 48 = 3 , which is the decimal integer constant representation of the digit 3 .
In the line ch = 9 + '0' the same principle applies but in reverse. here the addition is performed to convert the decimal integer constant representation of the digit 9 to its corresponding character constant representation. Adding the ASCII integer value of '0' (48) to the integer 9 gives us 48 + 9 = 57 , which is the ASCII encoding of the digit 9 .
The difference between a char type variable and any int type variable is their respective storage allocation specifications and range of values. char type variables use a lesser storage allocation and accept a smaller range of values.
Also since C allows character constants to be used as integers in arithmetic computation, a char type variable can exist in both signed and unsigned forms.
The following table shows the storage allocation specification and range of values for the different forms of char type variables:
The C standard doesn't define whether just specifying char in a variable declaration indicates a signed type or an unsigned type. Some compilers treat ordinary char as a signed type while others treat it as an unsigned type... Most of the time it doesn't matter whether char is signed or unsigned.
Do not assume that char is either signed or unsigned by default. If it matters, use the signed char keyword or unsigned char keyword in your variable declaration instead of just char .
A floating type variable will store floating-point constants. float is the keyword that is used to specify the floating type.
Floating point constants are values that have digits after the decimal point. You can omit digits before or after the decimal point, but you can't omit both. Floating point constants can also be expressed in scientific notation.
C provides three (3) Floating types keywords, corresponding to different floating point formats:
- float for single-precision floating-point formats
- double for double-precision floating-point formats
- long double for extended-precision floating-point formats
The C standard doesn't state how much precision the float , double , and long double types provide since different computers may store floating-point numbers in different ways. Most modern computers follow the specification in IEEE Standard 754 (also known as IEC 60559).
By default, all floating-point constants are stored as double-precision values by the C compiler. This means for example when a C compiler finds a floating-point constant of 12.34 in your program, it arranges for the number to be stored in memory in the same format as a value stored through a double variable.
If you want a floating-point constant to be stored in memory as a float add f to the end of the constant as you assign it to a float type variable:
How to print the value in a Floating type Variable
%f , %e , or %g is the format specifier used to print floating-point constants with printf . The format specifier %g produces the most aesthetically pleasing output because it lets printf decide whether to display the floating-point constant as normal floating-point notation or scientific notation. To print the value in a long double type variable add L (ex: %Lf ) to any of the above-mentioned format specifiers.
Let's create a program that store values in floating type variables and then print the values:

In C programming, a Variable is first declared before it is used. Declarations list the variables to be used and state what type they have.
In this article, you have been introduced to the fundamentals of how to declare variables that suit specific needs in your program, how to assign values to variables, and how to print the values stored in different types of variables using C standard library functions and format specifiers.
Thank you for taking the time to read my article. Please click reaction icons and share the article to your social network, the information in this article might help someone in your network.❤
The C Book — Keywords and identifiers
C Identifiers
The C Book — Integral types
Top comments (3)
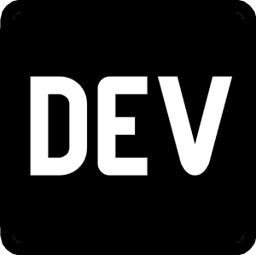
Templates let you quickly answer FAQs or store snippets for re-use.

- Email [email protected]
- Location San Francisco Bay Area
- Education M.S., University of Illinois at Urbana-Champaign
- Work Retired Principal Software Engineer
- Joined Jan 21, 2017
- There's no reason to require gcc vs, say, clang.
- There's no reason to require Ubuntu or any version of Linux.
- There's no reason to suggest vim.
- Your variable declaration syntax is wrong. See here .
- Your multiple variable declaration syntax is also wrong: you are missing the commas.
- You don't mention typical conventions for identifier use, e.g., ALL CAPS are generally used for macros in C.
- sizeof is not a standard library function: it's built-in to the C language.
- The printf type specifier to be used with sizeof is %zu since sizeof returns size_t , not unsigned long . Your code as written is not portable.
- You never actually say that signed integers produce undefined behavior for overflow. You also don't explain what undefined behavior is.
- Things like 'v' are character literals , not constants. Things like char const c are character constants.
- Your use of () in things like return (0) uses unnecessary parentheses. The return statement is a statement — no reason to make it look like a function call
- You mention signed char and unsigned char but never actually say why you'd want to use either.
- Your introduction is not comprehensive since you don't mention storage classes static and extern , pointers, arrays, declarations in for loops, or const . "Comprehensive" by definition means "complete."

- Education ALX software engineering
- Joined May 20, 2023
I appreciate that you took the time to read my article and share these feedbacks. Thank you.
But in response to this point you made
Things like 'v' are character literals, not constants. Things like char const c are character constants.
This is an excerpt from The C programming Language (Second edition) (Section 2.3, Page 37)
"A character constant is an integer, written as one character within single quotes, such as 'x' "
The terms are often used interchangeably, but "literal" is more precise. See here .
Are you sure you want to hide this comment? It will become hidden in your post, but will still be visible via the comment's permalink .
Hide child comments as well
For further actions, you may consider blocking this person and/or reporting abuse
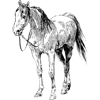
Quick introduction to the Prisma ORM
Awais - Apr 13
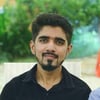
What is Python? It's History, Applications and Future
Shaheryar - Apr 3
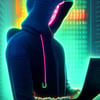
Quantum AI —Introduction - A better future?
Urooj Ahmad - Mar 30
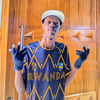
Postman 101 Workshop
NTWALI - Mar 29
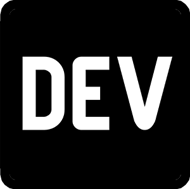
We're a place where coders share, stay up-to-date and grow their careers.

1.4 — Variable assignment and initialization
In the previous lesson ( 1.3 -- Introduction to objects and variables ), we covered how to define a variable that we can use to store values. In this lesson, we’ll explore how to actually put values into variables and use those values.
As a reminder, here’s a short snippet that first allocates a single integer variable named x , then allocates two more integer variables named y and z :
Variable assignment
After a variable has been defined, you can give it a value (in a separate statement) using the = operator . This process is called assignment , and the = operator is called the assignment operator .
By default, assignment copies the value on the right-hand side of the = operator to the variable on the left-hand side of the operator. This is called copy assignment .
Here’s an example where we use assignment twice:
This prints:
When we assign value 7 to variable width , the value 5 that was there previously is overwritten. Normal variables can only hold one value at a time.
One of the most common mistakes that new programmers make is to confuse the assignment operator ( = ) with the equality operator ( == ). Assignment ( = ) is used to assign a value to a variable. Equality ( == ) is used to test whether two operands are equal in value.
Initialization
One downside of assignment is that it requires at least two statements: one to define the variable, and another to assign the value.
These two steps can be combined. When an object is defined, you can optionally give it an initial value. The process of specifying an initial value for an object is called initialization , and the syntax used to initialize an object is called an initializer .
In the above initialization of variable width , { 5 } is the initializer, and 5 is the initial value.
Different forms of initialization
Initialization in C++ is surprisingly complex, so we’ll present a simplified view here.
There are 6 basic ways to initialize variables in C++:
You may see the above forms written with different spacing (e.g. int d{7}; ). Whether you use extra spaces for readability or not is a matter of personal preference.
Default initialization
When no initializer is provided (such as for variable a above), this is called default initialization . In most cases, default initialization performs no initialization, and leaves a variable with an indeterminate value.
We’ll discuss this case further in lesson ( 1.6 -- Uninitialized variables and undefined behavior ).
Copy initialization
When an initial value is provided after an equals sign, this is called copy initialization . This form of initialization was inherited from C.
Much like copy assignment, this copies the value on the right-hand side of the equals into the variable being created on the left-hand side. In the above snippet, variable width will be initialized with value 5 .
Copy initialization had fallen out of favor in modern C++ due to being less efficient than other forms of initialization for some complex types. However, C++17 remedied the bulk of these issues, and copy initialization is now finding new advocates. You will also find it used in older code (especially code ported from C), or by developers who simply think it looks more natural and is easier to read.
For advanced readers
Copy initialization is also used whenever values are implicitly copied or converted, such as when passing arguments to a function by value, returning from a function by value, or catching exceptions by value.
Direct initialization
When an initial value is provided inside parenthesis, this is called direct initialization .
Direct initialization was initially introduced to allow for more efficient initialization of complex objects (those with class types, which we’ll cover in a future chapter). Just like copy initialization, direct initialization had fallen out of favor in modern C++, largely due to being superseded by list initialization. However, we now know that list initialization has a few quirks of its own, and so direct initialization is once again finding use in certain cases.
Direct initialization is also used when values are explicitly cast to another type.
One of the reasons direct initialization had fallen out of favor is because it makes it hard to differentiate variables from functions. For example:
List initialization
The modern way to initialize objects in C++ is to use a form of initialization that makes use of curly braces. This is called list initialization (or uniform initialization or brace initialization ).
List initialization comes in three forms:
As an aside…
Prior to the introduction of list initialization, some types of initialization required using copy initialization, and other types of initialization required using direct initialization. List initialization was introduced to provide a more consistent initialization syntax (which is why it is sometimes called “uniform initialization”) that works in most cases.
Additionally, list initialization provides a way to initialize objects with a list of values (which is why it is called “list initialization”). We show an example of this in lesson 16.2 -- Introduction to std::vector and list constructors .
List initialization has an added benefit: “narrowing conversions” in list initialization are ill-formed. This means that if you try to brace initialize a variable using a value that the variable can not safely hold, the compiler is required to produce a diagnostic (usually an error). For example:
In the above snippet, we’re trying to assign a number (4.5) that has a fractional part (the .5 part) to an integer variable (which can only hold numbers without fractional parts).
Copy and direct initialization would simply drop the fractional part, resulting in the initialization of value 4 into variable width . Your compiler may optionally warn you about this, since losing data is rarely desired. However, with list initialization, your compiler is required to generate a diagnostic in such cases.
Conversions that can be done without potential data loss are allowed.
To summarize, list initialization is generally preferred over the other initialization forms because it works in most cases (and is therefore most consistent), it disallows narrowing conversions, and it supports initialization with lists of values (something we’ll cover in a future lesson). While you are learning, we recommend sticking with list initialization (or value initialization).
Best practice
Prefer direct list initialization (or value initialization) for initializing your variables.
Author’s note
Bjarne Stroustrup (creator of C++) and Herb Sutter (C++ expert) also recommend using list initialization to initialize your variables.
In modern C++, there are some cases where list initialization does not work as expected. We cover one such case in lesson 16.2 -- Introduction to std::vector and list constructors .
Because of such quirks, some experienced developers now advocate for using a mix of copy, direct, and list initialization, depending on the circumstance. Once you are familiar enough with the language to understand the nuances of each initialization type and the reasoning behind such recommendations, you can evaluate on your own whether you find these arguments persuasive.
Value initialization and zero initialization
When a variable is initialized using empty braces, value initialization takes place. In most cases, value initialization will initialize the variable to zero (or empty, if that’s more appropriate for a given type). In such cases where zeroing occurs, this is called zero initialization .
Q: When should I initialize with { 0 } vs {}?
Use an explicit initialization value if you’re actually using that value.
Use value initialization if the value is temporary and will be replaced.
Initialize your variables
Initialize your variables upon creation. You may eventually find cases where you want to ignore this advice for a specific reason (e.g. a performance critical section of code that uses a lot of variables), and that’s okay, as long the choice is made deliberately.
Related content
For more discussion on this topic, Bjarne Stroustrup (creator of C++) and Herb Sutter (C++ expert) make this recommendation themselves here .
We explore what happens if you try to use a variable that doesn’t have a well-defined value in lesson 1.6 -- Uninitialized variables and undefined behavior .
Initialize your variables upon creation.
Initializing multiple variables
In the last section, we noted that it is possible to define multiple variables of the same type in a single statement by separating the names with a comma:
We also noted that best practice is to avoid this syntax altogether. However, since you may encounter other code that uses this style, it’s still useful to talk a little bit more about it, if for no other reason than to reinforce some of the reasons you should be avoiding it.
You can initialize multiple variables defined on the same line:
Unfortunately, there’s a common pitfall here that can occur when the programmer mistakenly tries to initialize both variables by using one initialization statement:
In the top statement, variable “a” will be left uninitialized, and the compiler may or may not complain. If it doesn’t, this is a great way to have your program intermittently crash or produce sporadic results. We’ll talk more about what happens if you use uninitialized variables shortly.
The best way to remember that this is wrong is to consider the case of direct initialization or brace initialization:
Because the parenthesis or braces are typically placed right next to the variable name, this makes it seem a little more clear that the value 5 is only being used to initialize variable b and d , not a or c .
Unused initialized variables warnings
Modern compilers will typically generate warnings if a variable is initialized but not used (since this is rarely desirable). And if “treat warnings as errors” is enabled, these warnings will be promoted to errors and cause the compilation to fail.
Consider the following innocent looking program:
When compiling this with the g++ compiler, the following error is generated:
and the program fails to compile.
There are a few easy ways to fix this.
- If the variable really is unused, then the easiest option is to remove the defintion of x (or comment it out). After all, if it’s not used, then removing it won’t affect anything.
- Another option is to simply use the variable somewhere:
But this requires some effort to write code that uses it, and has the downside of potentially changing your program’s behavior.
The [[maybe_unused]] attribute C++17
In some cases, neither of the above options are desirable. Consider the case where we have a bunch of math/physics values that we use in many different programs:
If we use these a lot, we probably have these saved somewhere and copy/paste/import them all together.
However, in any program where we don’t use all of these values, the compiler will complain about each variable that isn’t actually used. While we could go through and remove/comment out the unused ones for each program, this takes time and energy. And later if we need one that we’ve previously removed, we’ll have to go back and re-add it.
To address such cases, C++17 introduced the [[maybe_unused]] attribute, which allows us to tell the compiler that we’re okay with a variable being unused. The compiler will not generate unused variable warnings for such variables.
The following program should generate no warnings/errors:
Additionally, the compiler will likely optimize these variables out of the program, so they have no performance impact.
In future lessons, we’ll often define variables we don’t use again, in order to demonstrate certain concepts. Making use of [[maybe_unused]] allows us to do so without compilation warnings/errors.
Question #1
What is the difference between initialization and assignment?
Show Solution
Initialization gives a variable an initial value at the point when it is created. Assignment gives a variable a value at some point after the variable is created.
Question #2
What form of initialization should you prefer when you want to initialize a variable with a specific value?
Direct list initialization (aka. direct brace initialization).
Question #3
What are default initialization and value initialization? What is the behavior of each? Which should you prefer?
Default initialization is when a variable initialization has no initializer (e.g. int x; ). In most cases, the variable is left with an indeterminate value.
Value initialization is when a variable initialization has an empty brace (e.g. int x{}; ). In most cases this will perform zero-initialization.
You should prefer value initialization to default initialization.
C Data Types
C operators.
- C Input and Output
- C Control Flow
- C Functions
- C Preprocessors
C File Handling
- C Cheatsheet
C Interview Questions
- C Programming Language Tutorial
- C Language Introduction
- Features of C Programming Language
- C Programming Language Standard
- C Hello World Program
- Compiling a C Program: Behind the Scenes
- Tokens in C
- Keywords in C
C Variables and Constants
C variables.
- Constants in C
- Const Qualifier in C
- Different ways to declare variable as constant in C
- Scope rules in C
- Internal Linkage and External Linkage in C
- Global Variables in C
- Data Types in C
- Literals in C
- Escape Sequence in C
- Integer Promotions in C
- Character Arithmetic in C
- Type Conversion in C
C Input/Output
- Basic Input and Output in C
- Format Specifiers in C
- printf in C
- Scansets in C
- Formatted and Unformatted Input/Output functions in C with Examples
- Operators in C
- Arithmetic Operators in C
- Unary operators in C
- Relational Operators in C
- Bitwise Operators in C
- C Logical Operators
- Assignment Operators in C
- Increment and Decrement Operators in C
- Conditional or Ternary Operator (?:) in C
- sizeof operator in C
- Operator Precedence and Associativity in C
C Control Statements Decision-Making
- Decision Making in C (if , if..else, Nested if, if-else-if )
- C - if Statement
- C if...else Statement
- C if else if ladder
- Switch Statement in C
- Using Range in switch Case in C
- while loop in C
- do...while Loop in C
- For Versus While
- Continue Statement in C
- Break Statement in C
- goto Statement in C
- User-Defined Function in C
- Parameter Passing Techniques in C
- Function Prototype in C
- How can I return multiple values from a function?
- main Function in C
- Implicit return type int in C
- Callbacks in C
- Nested functions in C
- Variadic functions in C
- _Noreturn function specifier in C
- Predefined Identifier __func__ in C
- C Library math.h Functions
C Arrays & Strings
- Properties of Array in C
- Multidimensional Arrays in C
- Initialization of Multidimensional Array in C
- Pass Array to Functions in C
- How to pass a 2D array as a parameter in C?
- What are the data types for which it is not possible to create an array?
- How to pass an array by value in C ?
- Strings in C
- Array of Strings in C
- What is the difference between single quoted and double quoted declaration of char array?
- C String Functions
- Pointer Arithmetics in C with Examples
- C - Pointer to Pointer (Double Pointer)
- Function Pointer in C
- How to declare a pointer to a function?
- Pointer to an Array | Array Pointer
- Difference between constant pointer, pointers to constant, and constant pointers to constants
- Pointer vs Array in C
- Dangling, Void , Null and Wild Pointers in C
- Near, Far and Huge Pointers in C
- restrict keyword in C
C User-Defined Data Types
- C Structures
- dot (.) Operator in C
- Structure Member Alignment, Padding and Data Packing
- Flexible Array Members in a structure in C
- Bit Fields in C
- Difference Between Structure and Union in C
- Anonymous Union and Structure in C
- Enumeration (or enum) in C
C Storage Classes
- Storage Classes in C
- extern Keyword in C
- Static Variables in C
- Initialization of static variables in C
- Static functions in C
- Understanding "volatile" qualifier in C | Set 2 (Examples)
- Understanding "register" keyword in C
C Memory Management
- Memory Layout of C Programs
- Dynamic Memory Allocation in C using malloc(), calloc(), free() and realloc()
- Difference Between malloc() and calloc() with Examples
- What is Memory Leak? How can we avoid?
- Dynamic Array in C
- How to dynamically allocate a 2D array in C?
- Dynamically Growing Array in C
C Preprocessor
- C Preprocessor Directives
- How a Preprocessor works in C?
- Header Files in C
- What’s difference between header files "stdio.h" and "stdlib.h" ?
- How to write your own header file in C?
- Macros and its types in C
- Interesting Facts about Macros and Preprocessors in C
- # and ## Operators in C
- How to print a variable name in C?
- Multiline macros in C
- Variable length arguments for Macros
- Branch prediction macros in GCC
- typedef versus #define in C
- Difference between #define and const in C?
- Basics of File Handling in C
- C fopen() function with Examples
- EOF, getc() and feof() in C
- fgets() and gets() in C language
- fseek() vs rewind() in C
- What is return type of getchar(), fgetc() and getc() ?
- Read/Write Structure From/to a File in C
- C Program to print contents of file
- C program to delete a file
- C Program to merge contents of two files into a third file
- What is the difference between printf, sprintf and fprintf?
- Difference between getc(), getchar(), getch() and getche()
Miscellaneous
- time.h header file in C with Examples
- Input-output system calls in C | Create, Open, Close, Read, Write
- Signals in C language
- Program error signals
- Socket Programming in C
- _Generics Keyword in C
- Multithreading in C
- C Programming Interview Questions (2024)
- Commonly Asked C Programming Interview Questions | Set 1
- Commonly Asked C Programming Interview Questions | Set 2
- Commonly Asked C Programming Interview Questions | Set 3
A variable in C language is the name associated with some memory location to store data of different types. There are many types of variables in C depending on the scope, storage class, lifetime, type of data they store, etc. A variable is the basic building block of a C program that can be used in expressions as a substitute in place of the value it stores.
What is a variable in C?
A variable in C is a memory location with some name that helps store some form of data and retrieves it when required. We can store different types of data in the variable and reuse the same variable for storing some other data any number of times.
They can be viewed as the names given to the memory location so that we can refer to it without having to memorize the memory address. The size of the variable depends upon the data type it stores.
C Variable Syntax
The syntax to declare a variable in C specifies the name and the type of the variable.
- data_type: Type of data that a variable can store.
- variable_name: Name of the variable given by the user.
- value: value assigned to the variable by the user.
Note: C is a strongly typed language so all the variables types must be specified before using them.
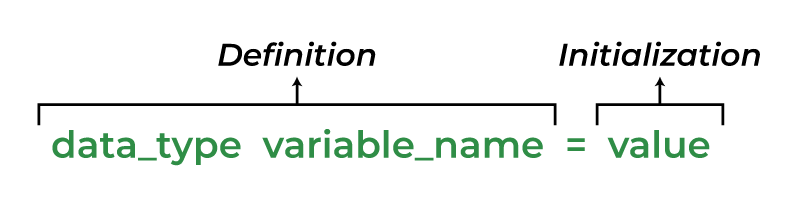
Variable Syntax Breakdown
There are 3 aspects of defining a variable:
- Variable Declaration
- Variable Definition
- Variable Initialization
1. C Variable Declaration
Variable declaration in C tells the compiler about the existence of the variable with the given name and data type.When the variable is declared compiler automatically allocates the memory for it.
2. C Variable Definition
In the definition of a C variable, the compiler allocates some memory and some value to it. A defined variable will contain some random garbage value till it is not initialized.
Note: Most of the modern C compilers declare and define the variable in single step. Although we can declare a variable in C by using extern keyword, it is not required in most of the cases. To know more about variable declaration and definition, click here.
3. C Variable Initialization
Initialization of a variable is the process where the user assigns some meaningful value to the variable.
How to use variables in C?
The below example demonstrates how the use variables in C language.
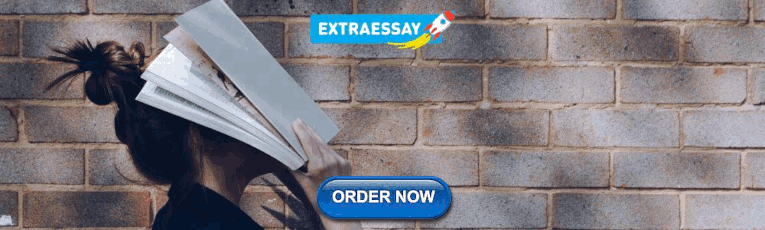
Rules for Naming Variables in C
You can assign any name to the variable as long as it follows the following rules:
- A variable name must only contain alphabets, digits, and underscore.
- A variable name must start with an alphabet or an underscore only. It cannot start with a digit.
- No whitespace is allowed within the variable name.
- A variable name must not be any reserved word or keyword.
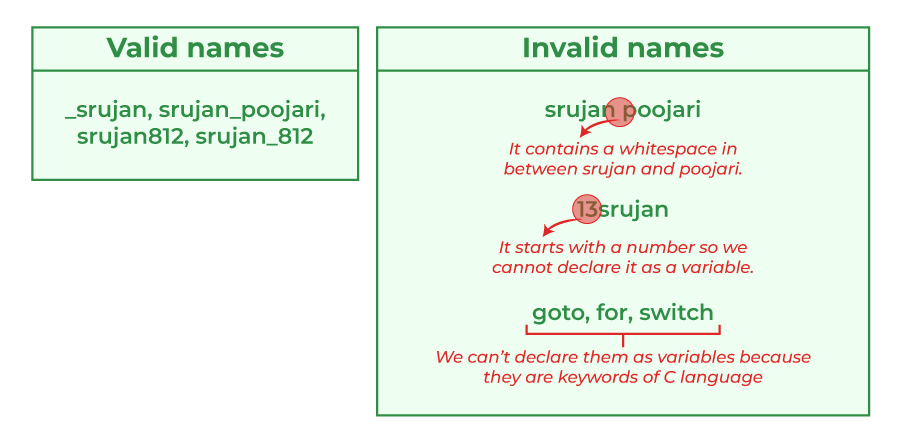
C Variable Types
The C variables can be classified into the following types:
- Local Variables
- Global Variables
- Static Variables
- Automatic Variables
- Extern Variables
- Register Variables
1. Local Variables in C
A Local variable in C is a variable that is declared inside a function or a block of code. Its scope is limited to the block or function in which it is declared.
Example of Local Variable in C
In the above code, x can be used only in the scope of function(). Using it in the main function will give an error.
2. Global Variables in C
A Global variable in C is a variable that is declared outside the function or a block of code. Its scope is the whole program i.e. we can access the global variable anywhere in the C program after it is declared.
Example of Global Variable in C
In the above code, both functions can use the global variable as global variables are accessible by all the functions.
Note: When we have same name for local and global variable, local variable will be given preference over the global variable by the compiler. For accessing global variable in this case, we can use the method mention here.
3. Static Variables in C
A static variable in C is a variable that is defined using the static keyword. It can be defined only once in a C program and its scope depends upon the region where it is declared (can be global or local ).
The default value of static variables is zero.
Syntax of Static Variable in C
As its lifetime is till the end of the program, it can retain its value for multiple function calls as shown in the example.
Example of Static Variable in C
In the above example, we can see that the local variable will always print the same value whenever the function will be called whereas the static variable will print the incremented value in each function call.
Note: Storage Classes in C is the concept that helps us to determine the scope, lifetime, memory location, and default value (initial value) of a variable.
4. Automatic Variable in C
All the local variables are automatic variables by default . They are also known as auto variables.
Their scope is local and their lifetime is till the end of the block. If we need, we can use the auto keyword to define the auto variables.
The default value of the auto variables is a garbage value.
Syntax of Auto Variable in C
Example of auto variable in c.
In the above example, both x and y are automatic variables. The only difference is that variable y is explicitly declared with the auto keyword.
5. External Variables in C
External variables in C can be shared between multiple C files . We can declare an external variable using the extern keyword.
Their scope is global and they exist between multiple C files.
Syntax of Extern Variables in C
Example of extern variable in c.
In the above example, x is an external variable that is used in multiple C files.
6. Register Variables in C
Register variables in C are those variables that are stored in the CPU register instead of the conventional storage place like RAM. Their scope is local and exists till the end of the block or a function.
These variables are declared using the register keyword.
The default value of register variables is a garbage value .
Syntax of Register Variables in C
Example of register variables in c.
NOTE: We cannot get the address of the register variable using addressof (&) operator because they are stored in the CPU register. The compiler will throw an error if we try to get the address of register variable.
Constant Variable in C
Till now we have only seen the variables whose values can be modified any number of times. But C language also provides us a way to make the value of a variable immutable. We can do that by defining the variable as constant.
A constant variable in C is a read-only variable whose value cannot be modified once it is defined. We can declare a constant variable using the const keyword.
Syntax of Const Variable in C
Note: We have to always initialize the const variable at the definition as we cannot modify its value after defining.
Example of Const Variable in C

FAQs on C Variables
Q1. what is the difference between variable declaration and definition in c.
In variable declaration, only the name and type of the variable is specified but no memory is allocated to the variable. In variable definition, the memory is also allocated to the declared variable.
Q2. What is the variable’s scope?
The scope of a variable is the region in which the variable exists and it is valid to perform operations on it. Beyond the scope of the variable, we cannot access it and it is said to be out of scope.
Please Login to comment...
Similar reads.
- C-Variable Declaration and Scope
- 5 Reasons to Start Using Claude 3 Instead of ChatGPT
- 6 Ways to Identify Who an Unknown Caller
- 10 Best Lavender AI Alternatives and Competitors 2024
- The 7 Best AI Tools for Programmers to Streamline Development in 2024
- 30 OOPs Interview Questions and Answers (2024)
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
C++ Tutorial
C++ functions, c++ classes, c++ examples, c++ declare multiple variables, declare many variables.
To declare more than one variable of the same type , use a comma-separated list:
One Value to Multiple Variables
You can also assign the same value to multiple variables in one line:

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
PrepBytes Blog
ONE-STOP RESOURCE FOR EVERYTHING RELATED TO CODING
Sign in to your account
Forgot your password?
Login via OTP
We will send you an one time password on your mobile number
An OTP has been sent to your mobile number please verify it below
Register with PrepBytes
Assignment operator in c.
Last Updated on June 23, 2023 by Prepbytes
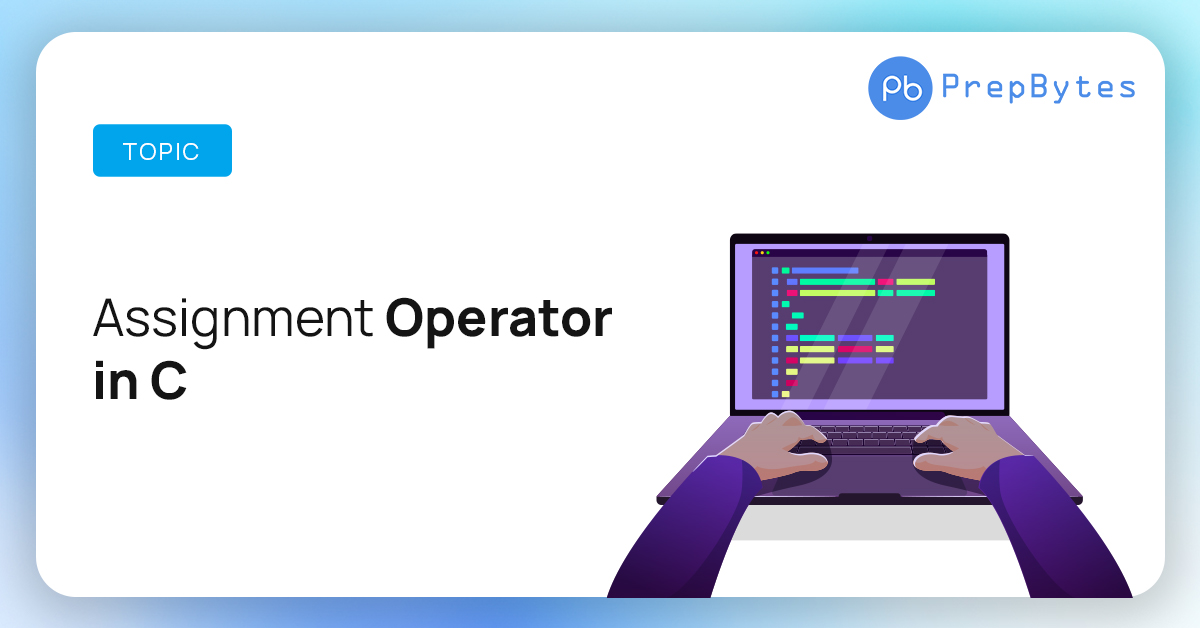
This type of operator is employed for transforming and assigning values to variables within an operation. In an assignment operation, the right side represents a value, while the left side corresponds to a variable. It is essential that the value on the right side has the same data type as the variable on the left side. If this requirement is not fulfilled, the compiler will issue an error.
What is Assignment Operator in C language?
In C, the assignment operator serves the purpose of assigning a value to a variable. It is denoted by the equals sign (=) and plays a vital role in storing data within variables for further utilization in code. When using the assignment operator, the value present on the right-hand side is assigned to the variable on the left-hand side. This fundamental operation allows developers to store and manipulate data effectively throughout their programs.
Example of Assignment Operator in C
For example, consider the following line of code:
Types of Assignment Operators in C
Here is a list of the assignment operators that you can find in the C language:
Simple assignment operator (=): This is the basic assignment operator, which assigns the value on the right-hand side to the variable on the left-hand side.
Addition assignment operator (+=): This operator adds the value on the right-hand side to the variable on the left-hand side and assigns the result back to the variable.
x += 3; // Equivalent to x = x + 3; (adds 3 to the current value of "x" and assigns the result back to "x")
Subtraction assignment operator (-=): This operator subtracts the value on the right-hand side from the variable on the left-hand side and assigns the result back to the variable.
x -= 4; // Equivalent to x = x – 4; (subtracts 4 from the current value of "x" and assigns the result back to "x")
* Multiplication assignment operator ( =):** This operator multiplies the value on the right-hand side with the variable on the left-hand side and assigns the result back to the variable.
x = 2; // Equivalent to x = x 2; (multiplies the current value of "x" by 2 and assigns the result back to "x")
Division assignment operator (/=): This operator divides the variable on the left-hand side by the value on the right-hand side and assigns the result back to the variable.
x /= 2; // Equivalent to x = x / 2; (divides the current value of "x" by 2 and assigns the result back to "x")
Bitwise AND assignment (&=): The bitwise AND assignment operator "&=" performs a bitwise AND operation between the value on the left-hand side and the value on the right-hand side. It then assigns the result back to the left-hand side variable.
x &= 3; // Binary: 0011 // After bitwise AND assignment: x = 1 (Binary: 0001)
Bitwise OR assignment (|=): The bitwise OR assignment operator "|=" performs a bitwise OR operation between the value on the left-hand side and the value on the right-hand side. It then assigns the result back to the left-hand side variable.
x |= 3; // Binary: 0011 // After bitwise OR assignment: x = 7 (Binary: 0111)
Bitwise XOR assignment (^=): The bitwise XOR assignment operator "^=" performs a bitwise XOR operation between the value on the left-hand side and the value on the right-hand side. It then assigns the result back to the left-hand side variable.
x ^= 3; // Binary: 0011 // After bitwise XOR assignment: x = 6 (Binary: 0110)
Left shift assignment (<<=): The left shift assignment operator "<<=" shifts the bits of the value on the left-hand side to the left by the number of positions specified by the value on the right-hand side. It then assigns the result back to the left-hand side variable.
x <<= 2; // Binary: 010100 (Shifted left by 2 positions) // After left shift assignment: x = 20 (Binary: 10100)
Right shift assignment (>>=): The right shift assignment operator ">>=" shifts the bits of the value on the left-hand side to the right by the number of positions specified by the value on the right-hand side. It then assigns the result back to the left-hand side variable.
x >>= 2; // Binary: 101 (Shifted right by 2 positions) // After right shift assignment: x = 5 (Binary: 101)
Conclusion The assignment operator in C, denoted by the equals sign (=), is used to assign a value to a variable. It is a fundamental operation that allows programmers to store data in variables for further use in their code. In addition to the simple assignment operator, C provides compound assignment operators that combine arithmetic or bitwise operations with assignment, allowing for concise and efficient code.
FAQs related to Assignment Operator in C
Q1. Can I assign a value of one data type to a variable of another data type? In most cases, assigning a value of one data type to a variable of another data type will result in a warning or error from the compiler. It is generally recommended to assign values of compatible data types to variables.
Q2. What is the difference between the assignment operator (=) and the comparison operator (==)? The assignment operator (=) is used to assign a value to a variable, while the comparison operator (==) is used to check if two values are equal. It is important not to confuse these two operators.
Q3. Can I use multiple assignment operators in a single statement? No, it is not possible to use multiple assignment operators in a single statement. Each assignment operator should be used separately for assigning values to different variables.
Q4. Are there any limitations on the right-hand side value of the assignment operator? The right-hand side value of the assignment operator should be compatible with the data type of the left-hand side variable. If the data types are not compatible, it may lead to unexpected behavior or compiler errors.
Q5. Can I assign the result of an expression to a variable using the assignment operator? Yes, it is possible to assign the result of an expression to a variable using the assignment operator. For example, x = y + z; assigns the sum of y and z to the variable x.
Q6. What happens if I assign a value to an uninitialized variable? Assigning a value to an uninitialized variable will initialize it with the assigned value. However, it is considered good practice to explicitly initialize variables before using them to avoid potential bugs or unintended behavior.
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
- Linked List
- Segment Tree
- Backtracking
- Dynamic Programming
- Greedy Algorithm
- Operating System
- Company Placement
- Interview Tips
- General Interview Questions
- Data Structure
- Other Topics
- Computational Geometry
- Game Theory
Related Post
Null character in c, ackermann function in c, median of two sorted arrays of different size in c, number is palindrome or not in c, implementation of queue using linked list in c, c program to replace a substring in a string.
C# Tuples as Syntactic Sugar for Multiple Variable Assignment
Published 12 Jun 2020
- Patterns and Practices
I came across an interesting pattern for using Tuples in an article by Mads Torgersen on C# 9.0 . At first I thought it must be something new in 9.0, but a little digging and actually engaging my brain made me realise it has been possible since C# 7.0 and the introduction of Tuples. It isn't something I've seen anybody else use so I thought it would be worth writing about.
I have to admit that I've not really looked at Tuples that much and learning a bit more about them reveals some features that allow a style of assignment through destructuring that is ubiquitous in modern JavaScript.
Here is the bit of code from Mads' article that triggered my curiosity (expression bodies wrapped by me for legibility online):
At this point in his article he is actually explaining records using the data keyword (very cool). These feature new init-only properties and are intended as value-like immutable data. He offers the above example as a positional approach to record creation and usage. I have to admit I'd not known about the Deconstruct method which assigns values to any number of out variables. We'll get to that below, but the bit that initially caught my eye was the constructor. Both properties are wrapped in parentheses, as are the two constructor parameters, so the assignments are both done in one line. So what's going on here?
The answer, as I've alluded to above, is Tuple deconstruction. The normal way that you consume tuples as return types is to create the variables in the expression:
The bit that I was not aware of is tucked away at the end of the section on deconstruction in the tuple docs , where it says that you can deconstruct tuples with existing declarations as well, which is what Mads has done. So the code creates a tuple of the existing declared variables, and one from the arguments and assigns values from one to the other.
As a side note, it is worth pointing out that you cannot mix existing declarations with declarations inside the parentheses, it is one or the other.
Why is this a useful pattern to consider? The answer is brevity and readability. Let's look at an example with a simple class and constructor. The standard approach would look like this:
with the destructuring syntax this becomes:
and now we have a shorter single line expression we can also use that other bit of syntactic sugar, the expression body, so we can get the whole constructor on one line:
Many of the improvements that have been applied to C# over the years have been about the reduction in the amount of boilerplate code you need to write, what Jon Skeet refers to as "ceremony". It is about getting the compiler to do the work for you so you can get on with the task at hand. Now you always have to be wary of when terseness turns into obscurity. However, in this case I would argue that this approach is actually more easy to read and comprehend than the traditional approach - it is quite expressive of the intent which is why I like it as a pattern.
JavaScript Style Destructuring
I promised to get back to the Deconstruct method, as this can bring a similar reduction in ceremony, that makes some code that looks very JavaScript-like. As a reminder, Mads' example featured this line:
The power of this method is that it can be used to assign variables like this:
This is why I say it is a strong parallel to JavaScript object destructuring where you would see something like this:
Where this gets really interesting is that you can apply a Deconstruct method to types you have not created via extension methods, so you can use this brief destructuring syntax wherever you want like this:
You can create multiple Deconstruct methods with different out parameters, but this could get confusing and create more work than it saves. As an alternative you can have single/fewer methods and use Discards , another C# 7.0 feature that I've not used. This uses the underscore to assign a temporary dummy variable that is never used by your application. You use it like this:
I've never really spent much time exploring tuples because I've preferred to instantiate return values as types. I've not found situations where those types felt like an overhead. I'm not sure I've changed my stance on that after spending a bit more time investigating them, but this ability to add a Deconstruct extension to a class to enable destructuring assignment is interesting and I'm going to be looking out for opportunities to use this approach to see how it works.
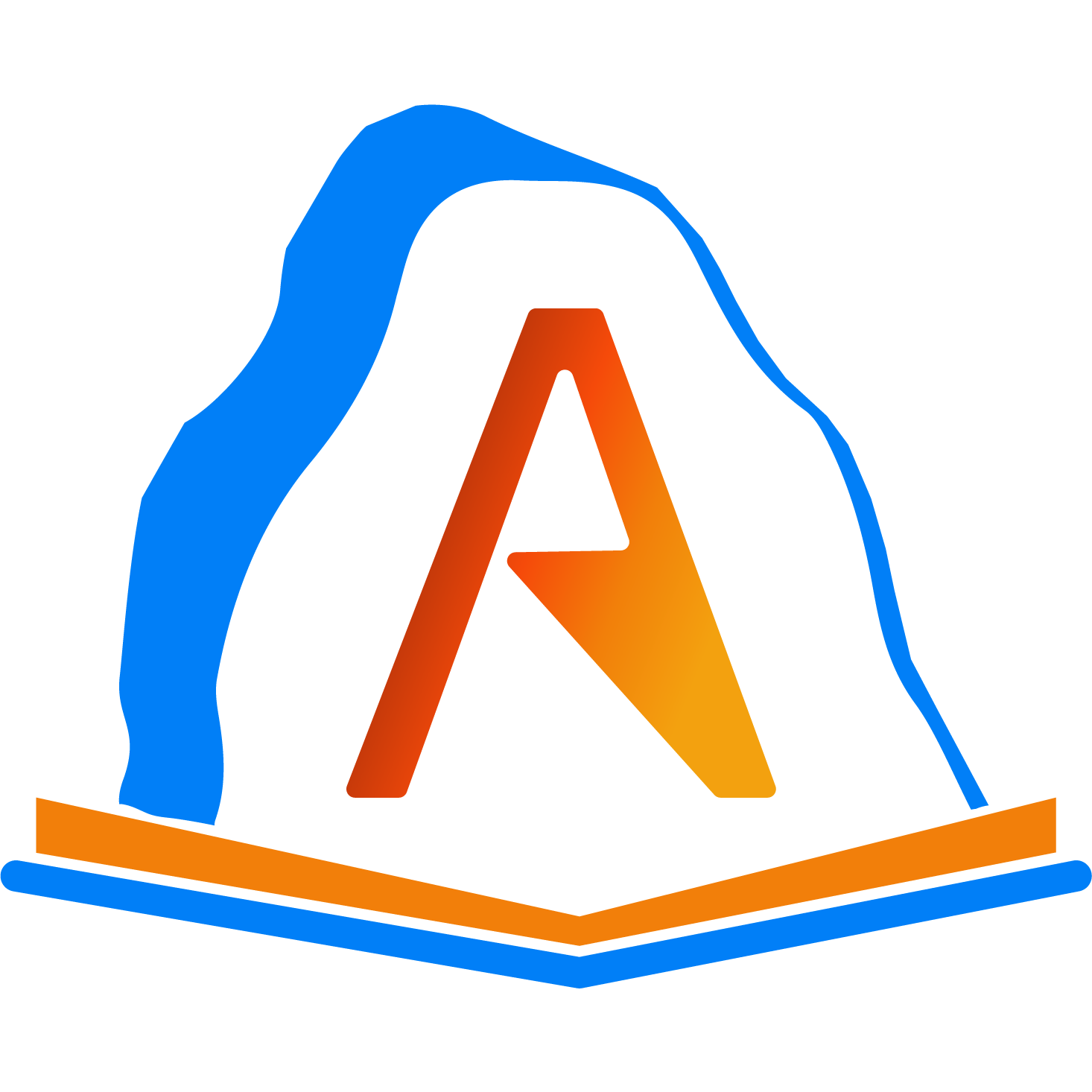
- Table of Contents
- Course Home
- Assignments
- Peer Instruction (Instructor)
- Peer Instruction (Student)
- Change Course
- Instructor's Page
- Progress Page
- Edit Profile
- Change Password
- Scratch ActiveCode
- Scratch Activecode
- Instructors Guide
- About Runestone
- Report A Problem
- Coding Practice
- Activecode Exercises
- Mixed Up Code Practice
- 6.1 Multiple assignment
- 6.2 Iteration
- 6.3 The while statement
- 6.5 Two-dimensional tables
- 6.6 Encapsulation and generalization
- 6.7 Functions
- 6.8 More encapsulation
- 6.9 Local variables
- 6.10 More generalization
- 6.11 Glossary
- 6.12 Multiple Choice Exercises
- 6.13 Mixed-Up Code Exercises
- 6.14 Coding Practice
- 6. Iteration" data-toggle="tooltip">
- 6.2. Iteration' data-toggle="tooltip" >
6.1. Multiple assignment ¶
I haven’t said much about it, but it is legal in C++ to make more than one assignment to the same variable. The effect of the second assignment is to replace the old value of the variable with a new value.
The active code below reassigns fred from 5 to 7 and prints both values out.
The output of this program is 57 , because the first time we print fred his value is 5, and the second time his value is 7.
The active code below reassigns fred from 5 to 7 without printing out the initial value.
However, if we do not print fred the first time, the output is only 7 because the value of fred is just 7 when it is printed.
This kind of multiple assignment is the reason I described variables as a container for values. When you assign a value to a variable, you change the contents of the container, as shown in the figure:
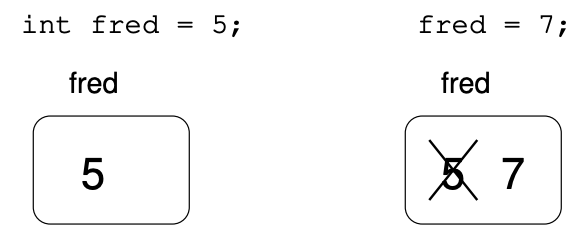
When there are multiple assignments to a variable, it is especially important to distinguish between an assignment statement and a statement of equality. Because C++ uses the = symbol for assignment, it is tempting to interpret a statement like a = b as a statement of equality. It is not!
An assignment statement uses a single = symbol. For example, x = 3 assigns the value of 3 to the variable x . On the other hand, an equality statement uses two = symbols. For example, x == 3 is a boolean that evaluates to true if x is equal to 3 and evaluates to false otherwise.
First of all, equality is commutative, and assignment is not. For example, in mathematics if \(a = 7\) then \(7 = a\) . But in C++ the statement a = 7; is legal, and 7 = a; is not.
Furthermore, in mathematics, a statement of equality is true for all time. If \(a = b\) now, then \(a\) will always equal \(b\) . In C++, an assignment statement can make two variables equal, but they don’t have to stay that way!
The third line changes the value of a but it does not change the value of b , and so they are no longer equal. In many programming languages an alternate symbol is used for assignment, such as <- or := , in order to avoid confusion.
Although multiple assignment is frequently useful, you should use it with caution. If the values of variables are changing constantly in different parts of the program, it can make the code difficult to read and debug.
- Checking if a is equal to b
- Assigning a to the value of b
- Setting the value of a to 4
Q-4: What will print?
- There are no spaces between the numbers.
- Remember, in C++ spaces must be printed.
- Carefully look at the values being assigned.
Q-5: What is the correct output?
- Remember that printing a boolean results in either 0 or 1.
- Is x equal to y?
- x is equal to y, so the output is 1.
- Windows Programming
- UNIX/Linux Programming
- General C++ Programming
- Multiple Assignment
Multiple Assignment

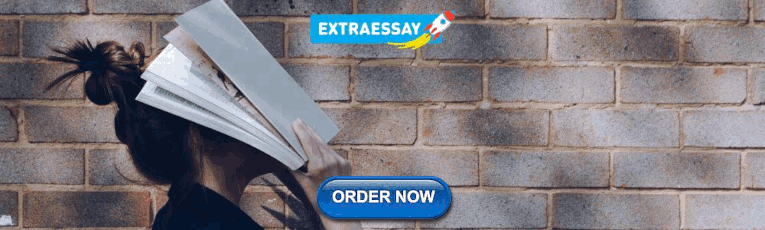
IMAGES
VIDEO
COMMENTS
Y = Z and X = Z (I.E., Assign the value of Z to both X and Y) But C language treats the multiple assignments like a chain, like this: In C, "X = Y = Z" means that the value of Z must be first assigned to Y, and then the value of Y must be assigned to X. Here is a sample program to see how the multiple assignments in single line work:
In C++ assignment evaluates to an lvalue, which requires "chained" assignments to be sequenced.) There's no way to say whether it is a good or bad programming practice without seeing more context. In cases when the two variables are tightly related (like x and y coordinate of a point), setting them to some common value using "chained ...
Multiple assignment of single variable in a single line of code. 1. Value assignment in C while declaring multiple variable in 1 line. Hot Network Questions Would caves maintain a cool temperature on the hot side of a tidally locked planet?
Declare Multiple Variables. To declare more than one variable of the same type, use a comma-separated list: Example. int x = 5, y = 6, z = 50; printf("%d", x + y + z); Try it Yourself » You can also assign the same value to multiple variables of the same type: Example. int x, y, z;
If you want you can declare multiple variables of the same type like this: int x, y, z; // declaring three variables x,y and z of type int. Here x, y, ... If you want you can assign some initial value to the variable using assignment operator i.e (=). Assigning a value to the variable is called initialization of the variable. Here are some ...
As we've left it a little late in the semester to explore functions, we're well-used to declaring/assigning multiple variables of the same type on a single line, as below: int a, b, c = 0; This, however, does not appear to work when integers a, b, and c are the parameters to be passed to a function: int function(int a, int b, int c);
Variables Constants Display Variables Multiple Variables Identifiers. C# Data Types C# Type Casting C# User Input C# Operators. Arithmetic Assignment Comparison Logical. C# Math C# Strings. ... You can also assign the same value to multiple variables in one line: Example int x, y, z; x = y = z = 50; Console.WriteLine(x + y + z); ...
This operator first adds the current value of the variable on left to the value on the right and then assigns the result to the variable on the left. Example: (a += b) can be written as (a = a + b) If initially value stored in a is 5. Then (a += 6) = 11. 3. "-=" This operator is combination of '-' and '=' operators.
To declare a variable in C programming, we first specify the Type of the variable, and then its Identifier. For example, we might declare variables "height" and "profit" as: /* examples of variable declaration */ int height; float profit; The first declaration states that height is a variable of Type int, which means that height can store whole ...
int x; // define an integer variable named x int y, z; // define two integer variables, named y and z. Variable assignment. After a variable has been defined, you can give it a value (in a separate statement) using the = operator. This process is called assignment, and the = operator is called the assignment operator.
A variable in C language is the name associated with some memory location to store data of different types. There are many types of variables in C depending on the scope, storage class, lifetime, type of data they store, etc. A variable is the basic building block of a C program that can be used in expressions as a substitute in place of the ...
W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more.
In C, the assignment operator serves the purpose of assigning a value to a variable. It is denoted by the equals sign (=) and plays a vital role in storing data within variables for further utilization in code. When using the assignment operator, the value present on the right-hand side is assigned to the variable on the left-hand side.
The normal way that you consume tuples as return types is to create the variables in the expression: private static (double, double, int) ComputeSumAndSumOfSquares(IEnumerable<double> sequence) {. // method body omitted. } // you can explicitly declare the types of each field. ( int count, double sum, double sumOfSquares ...
6.1. Multiple assignment. I haven't said much about it, but it is legal in C++ to make more than one assignment to the same variable. The effect of the second assignment is to replace the old value of the variable with a new value. The active code below reassigns fred from 5 to 7 and prints both values out. The output of this program is 57 ...
Solution 3: In C++, it is possible to assign multiple variables at once using a single assignment statement. This is known as multiple assignment or parallel assignment. Here is an example of how to assign multiple variables at once in C++: int x, y, z; x = y = z = 10; In this example, the variables x, y, and z are all assigned the value 10 in ...
Packing multiple operations and/or statements into the same line is straightforward - both you and Xerzi have come up with ways. I think what the OP is looking for is something subtly different - a syntax construct to apply the same operation to multiple variables, concisely in one statement. I don't know of any such syntax in standard C++.
Using , operator you can assign a value to a variable multiple times. e.g. K = 20, K = 30; This will assign 30 to K overwriting the previous value of 20. I thought it's invalid to change a variable more than one time in one statement. Yes it leads to undefined behavior if we try to modify a variable more than once in a same C statement but here ...
Here's how you can assign one value to multiple variables in C++: Step 1: Declare the Variables. Before assigning a value to multiple variables, you need to declare them, either using the multiple declaration approach or by declaring an array or container, as mentioned in the previous sections. Step 2: Assign the Value.