If...Else Statement in C Explained
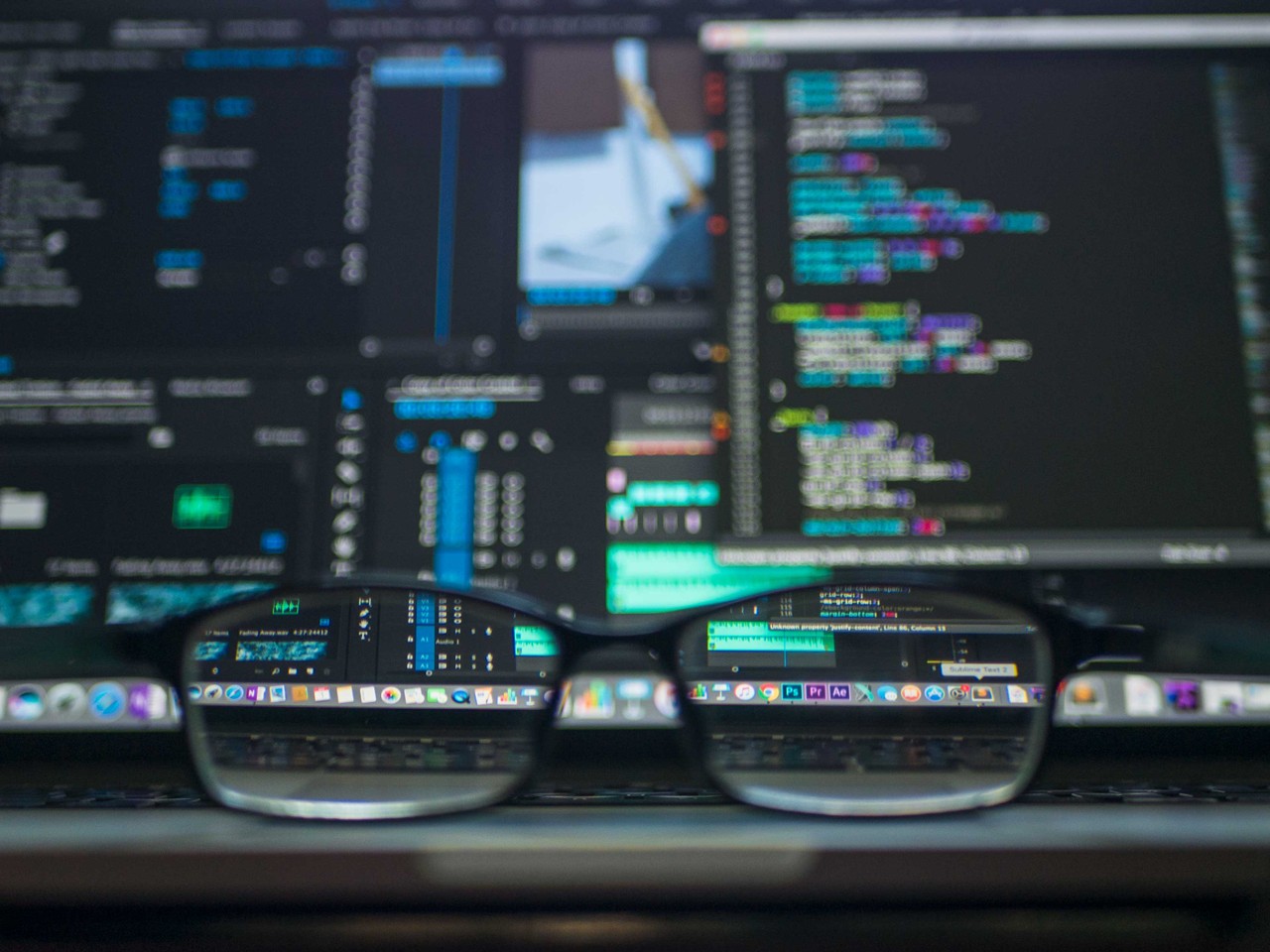
Conditional code flow is the ability to change the way a piece of code behaves based on certain conditions. In such situations you can use if statements.
The if statement is also known as a decision making statement, as it makes a decision on the basis of a given condition or expression. The block of code inside the if statement is executed is the condition evaluates to true. However, the code inside the curly braces is skipped if the condition evaluates to false, and the code after the if statement is executed.
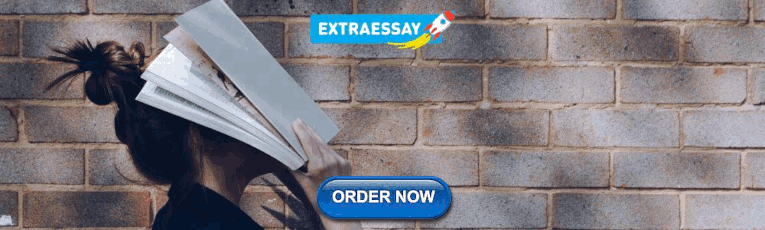
Syntax of an if statement
A simple example.
Let’s look at an example of this in action:
If the code inside parenthesis of the if statement is true, everything within the curly braces is executed. In this case, true evaluates to true, so the code runs the printf function.
if..else statements
In an if...else statement, if the code in the parenthesis of the if statement is true, the code inside its brackets is executed. But if the statement inside the parenthesis is false, all the code within the else statement's brackets is executed instead.
Of course, the example above isn't very useful in this case because true always evaluates to true. Here's another that's a bit more practical:
There are a few important differences here. First, stdbool.h hasn’t been included. That's okay because true and false aren't being used like in the first example. In C, like in other programming languages, you can use statements that evaluate to true or false rather than using the boolean values true or false directly.
Also notice the condition in the parenthesis of the if statement: n == 3 . This condition compares n and the number 3. == is the comparison operator, and is one of several comparison operations in C.
Nested if...else
The if...else statement allows a choice to be made between two possibilities. But sometimes you need to choose between three or more possibilities.
For example the sign function in mathematics returns -1 if the argument is less than zero, +1 if the argument is greater than zero, and returns zero if the argument is zero.
The following code implements this function:
As you can see, a second if...else statement is nested within else statement of the first if..else .
If x is less than 0, then sign is set to -1. However, if x is not less than 0, the second if...else statement is executed. There, if x is equal to 0, sign is also set to 0. But if x is greater than 0, sign is instead set to 1.
Rather than a nested if...else statement, beginners often use a string of if statements:
While this works, it's not recommended since it's unclear that only one of the assignment statements ( sign = ... ) is meant to be executed depending on the value of x . It's also inefficient – every time the code runs, all three conditions are tested, even if one or two don't have to be.
else...if statements
if...else statements are an alternative to a string of if statements. Consider the following:
If the condition for the if statement evaluates to false, the condition for the else...if statement is checked. If that condition evaluates to true, the code inside the else...if statement's curly braces is run.
Comparison Operators
Logical operators.
We might want a bit of code to run if something is not true, or if two things are true. For that we have logical operators:
For example:
An important note about C comparisons
While we mentioned earlier that each comparison is checking if something is true or false, but that's only half true. C is very light and close to the hardware it's running on. With hardware it's easy to check if something is 0 or false, but anything else is much more difficult.
Instead it's much more accurate to say that the comparisons are really checking if something is 0 / false, or if it is any other value.
For example, his if statement is true and valid:
By design, 0 is false, and by convention, 1 is true. In fact, here’s a look at the stdbool.h library:
While there's a bit more to it, this is the core of how booleans work and how the library operates. These two lines instruct the compiler to replace the word false with 0, and true with 1.
If this article was helpful, share it .
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
C Data Types
C operators.
- C Input and Output
- C Control Flow
- C Functions
- C Preprocessors
C File Handling
- C Cheatsheet
C Interview Questions
- C Programming Language Tutorial
- C Language Introduction
- Features of C Programming Language
- C Programming Language Standard
- C Hello World Program
- Compiling a C Program: Behind the Scenes
- Tokens in C
- Keywords in C
C Variables and Constants
- C Variables
- Constants in C
- Const Qualifier in C
- Different ways to declare variable as constant in C
- Scope rules in C
- Internal Linkage and External Linkage in C
- Global Variables in C
- Data Types in C
- Literals in C
- Escape Sequence in C
- Integer Promotions in C
- Character Arithmetic in C
- Type Conversion in C
C Input/Output
- Basic Input and Output in C
- Format Specifiers in C
- printf in C
- Scansets in C
- Formatted and Unformatted Input/Output functions in C with Examples
- Operators in C
- Arithmetic Operators in C
- Unary operators in C
- Relational Operators in C
- Bitwise Operators in C
- C Logical Operators
- Assignment Operators in C
- Increment and Decrement Operators in C
- Conditional or Ternary Operator (?:) in C
- sizeof operator in C
- Operator Precedence and Associativity in C
C Control Statements Decision-Making
- Decision Making in C (if , if..else, Nested if, if-else-if )
- C - if Statement
- C if...else Statement
- C if else if ladder
- Switch Statement in C
- Using Range in switch Case in C
- while loop in C
- do...while Loop in C
- For Versus While
- Continue Statement in C
- Break Statement in C
- goto Statement in C
- User-Defined Function in C
- Parameter Passing Techniques in C
- Function Prototype in C
- How can I return multiple values from a function?
- main Function in C
- Implicit return type int in C
- Callbacks in C
- Nested functions in C
- Variadic functions in C
- _Noreturn function specifier in C
- Predefined Identifier __func__ in C
- C Library math.h Functions
C Arrays & Strings
- Properties of Array in C
- Multidimensional Arrays in C
- Initialization of Multidimensional Array in C
- Pass Array to Functions in C
- How to pass a 2D array as a parameter in C?
- What are the data types for which it is not possible to create an array?
- How to pass an array by value in C ?
- Strings in C
- Array of Strings in C
- What is the difference between single quoted and double quoted declaration of char array?
- C String Functions
- Pointer Arithmetics in C with Examples
- C - Pointer to Pointer (Double Pointer)
- Function Pointer in C
- How to declare a pointer to a function?
- Pointer to an Array | Array Pointer
- Difference between constant pointer, pointers to constant, and constant pointers to constants
- Pointer vs Array in C
- Dangling, Void , Null and Wild Pointers in C
- Near, Far and Huge Pointers in C
- restrict keyword in C
C User-Defined Data Types
- C Structures
- dot (.) Operator in C
- Structure Member Alignment, Padding and Data Packing
- Flexible Array Members in a structure in C
- Bit Fields in C
- Difference Between Structure and Union in C
- Anonymous Union and Structure in C
- Enumeration (or enum) in C
C Storage Classes
- Storage Classes in C
- extern Keyword in C
- Static Variables in C
- Initialization of static variables in C
- Static functions in C
- Understanding "volatile" qualifier in C | Set 2 (Examples)
- Understanding "register" keyword in C
C Memory Management
- Memory Layout of C Programs
- Dynamic Memory Allocation in C using malloc(), calloc(), free() and realloc()
- Difference Between malloc() and calloc() with Examples
- What is Memory Leak? How can we avoid?
- Dynamic Array in C
- How to dynamically allocate a 2D array in C?
- Dynamically Growing Array in C
C Preprocessor
- C Preprocessor Directives
- How a Preprocessor works in C?
- Header Files in C
- What’s difference between header files "stdio.h" and "stdlib.h" ?
- How to write your own header file in C?
- Macros and its types in C
- Interesting Facts about Macros and Preprocessors in C
- # and ## Operators in C
- How to print a variable name in C?
- Multiline macros in C
- Variable length arguments for Macros
- Branch prediction macros in GCC
- typedef versus #define in C
- Difference between #define and const in C?
- Basics of File Handling in C
- C fopen() function with Examples
- EOF, getc() and feof() in C
- fgets() and gets() in C language
- fseek() vs rewind() in C
- What is return type of getchar(), fgetc() and getc() ?
- Read/Write Structure From/to a File in C
- C Program to print contents of file
- C program to delete a file
- C Program to merge contents of two files into a third file
- What is the difference between printf, sprintf and fprintf?
- Difference between getc(), getchar(), getch() and getche()
Miscellaneous
- time.h header file in C with Examples
- Input-output system calls in C | Create, Open, Close, Read, Write
- Signals in C language
- Program error signals
- Socket Programming in C
- _Generics Keyword in C
- Multithreading in C
- C Programming Interview Questions (2024)
- Commonly Asked C Programming Interview Questions | Set 1
- Commonly Asked C Programming Interview Questions | Set 2
- Commonly Asked C Programming Interview Questions | Set 3
C – if Statement
The if in C is the most simple decision-making statement. It consists of the test condition and if block or body. If the given condition is true only then the if block will be executed.
What is if in C?
The if in C is a decision-making statement that is used to execute a block of code based on the value of the given expression. It is one of the core concepts of C programming and is used to include conditional code in our program.
Syntax of if Statement in C
How to use if statement in c.
The following examples demonstrate how to use the if statement in C:
How if in C works?
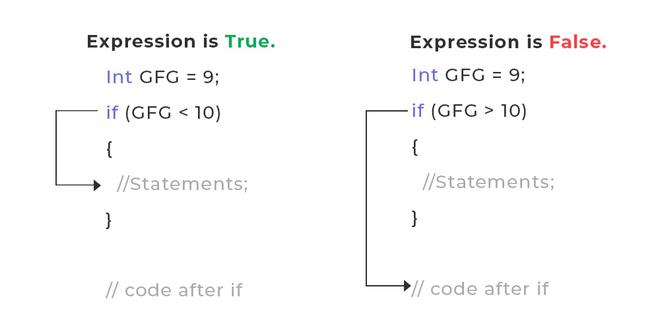
Working of if Statement in C
The working of the if statement in C is as follows:
- STEP 1: When the program control comes to the if statement, the test expression is evaluated.
- STEP 2A: If the condition is true, the statements inside the if block are executed.
- STEP 2B: If the expression is false, the statements inside the if body are not executed.
- STEP 3: Program control moves out of the if block and the code after the if block is executed.
Flowchart of if in C
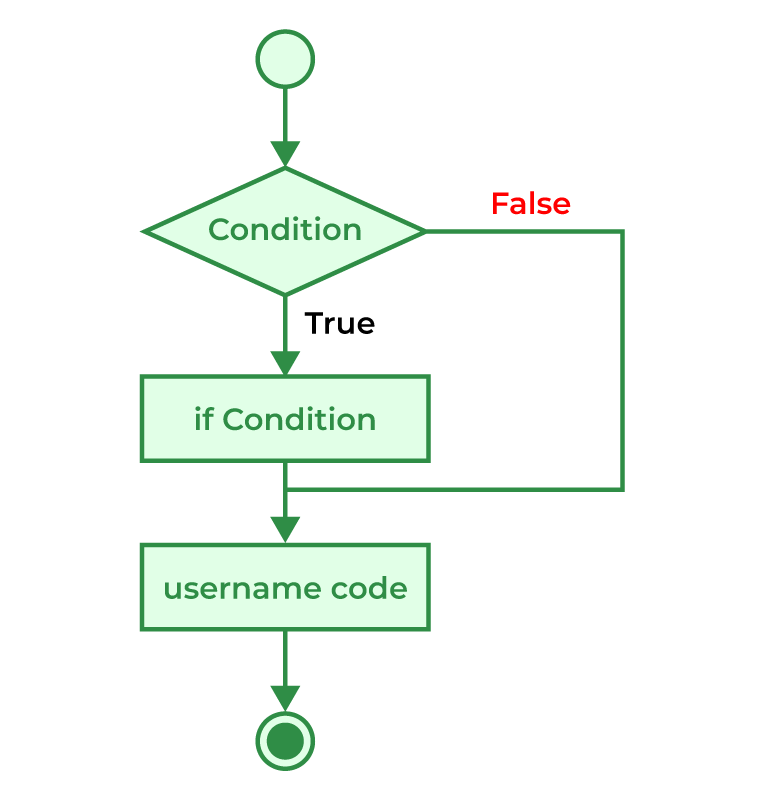
Flow Diagram of if Statement in C
Examples of if Statements in C
Example 1: c program to check whether the number is even or odd..
In this program, we will make use of the logic that if the number is divisible by 2, then it is even else odd except one.
Example 2: C Program to check whether a number is prime or not.
In this program, we will check for the smallest factor of the given number N starting from 2 to sqrt (N) using a loop. Whenever we find the factor, we will set the flag and exit the loop. The code to be executed will be contained inside the if statement.
Advantages of if Statement
Following are the main advantages of the if statement in C:
- It is the simplest decision-making statement.
- It is easy to use and understand.
- It can evaluate expressions of all types such as int, char, bool, etc.
Disadvantages of if Statement
The main limitations of if block is listed below:
- It contains only a single block. In case when there are multiply related if blocks, all the blocks will be tested even when the matching if block is found at the start
- When there are a large number of expressions, the code of the if block gets complex and unreadable.
- It is slower for a large number of conditions.
The if statement is the simplest decision-making statement due to which it is easy to use and understand. But being simple, it also has many limitations. We can use if-else, if-else-if ladder, or switch statements to overcome these limitations. Still, the if statement is widely used in C programming to add some conditional code to the program.
FAQs on if in C
1. define c if staement..
The if statement is a program control statement in C language that is used to execute a part of code based on some condition.
2. How many types of decision-making statements are there in the C language?
There are 5 types of conditional statements or decision-making statements in C language:
- if Statement
- if-else Statement
- if-else-if Ladder
- switch Statement
- Conditional Operator
3. Can we specify multiple conditions in if statement?
We can specify multiple conditions in the if statement but not separately. We have to join these multiple conditions using logical operators making them into a single expression. We can then use this expression in the if statement.
Valid Expressions
Invalid expressions.
In the above expression, the rightmost expression in the parenthesis will be considered.
Please Login to comment...
Similar reads.
- 10 Best Slack Integrations to Enhance Your Team's Productivity
- 10 Best Zendesk Alternatives and Competitors
- 10 Best Trello Power-Ups for Maximizing Project Management
- Google Rolls Out Gemini In Android Studio For Coding Assistance
- 30 OOPs Interview Questions and Answers (2024)
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Next: Execution Control Expressions , Previous: Arithmetic , Up: Top [ Contents ][ Index ]
7 Assignment Expressions
As a general concept in programming, an assignment is a construct that stores a new value into a place where values can be stored—for instance, in a variable. Such places are called lvalues (see Lvalues ) because they are locations that hold a value.
An assignment in C is an expression because it has a value; we call it an assignment expression . A simple assignment looks like
We say it assigns the value of the expression value-to-store to the location lvalue , or that it stores value-to-store there. You can think of the “l” in “lvalue” as standing for “left,” since that’s what you put on the left side of the assignment operator.
However, that’s not the only way to use an lvalue, and not all lvalues can be assigned to. To use the lvalue in the left side of an assignment, it has to be modifiable . In C, that means it was not declared with the type qualifier const (see const ).
The value of the assignment expression is that of lvalue after the new value is stored in it. This means you can use an assignment inside other expressions. Assignment operators are right-associative so that
is equivalent to
This is the only useful way for them to associate; the other way,
would be invalid since an assignment expression such as x = y is not valid as an lvalue.
Warning: Write parentheses around an assignment if you nest it inside another expression, unless that is a conditional expression, or comma-separated series, or another assignment.
cppreference.com
Assignment operators.
Assignment operators modify the value of the object.
[ edit ] Definitions
Copy assignment replaces the contents of the object a with a copy of the contents of b ( b is not modified). For class types, this is performed in a special member function, described in copy assignment operator .
For non-class types, copy and move assignment are indistinguishable and are referred to as direct assignment .
Compound assignment replace the contents of the object a with the result of a binary operation between the previous value of a and the value of b .
[ edit ] Assignment operator syntax
The assignment expressions have the form
- ↑ target-expr must have higher precedence than an assignment expression.
- ↑ new-value cannot be a comma expression, because its precedence is lower.
[ edit ] Built-in simple assignment operator
For the built-in simple assignment, the object referred to by target-expr is modified by replacing its value with the result of new-value . target-expr must be a modifiable lvalue.
The result of a built-in simple assignment is an lvalue of the type of target-expr , referring to target-expr . If target-expr is a bit-field , the result is also a bit-field.
[ edit ] Assignment from an expression
If new-value is an expression, it is implicitly converted to the cv-unqualified type of target-expr . When target-expr is a bit-field that cannot represent the value of the expression, the resulting value of the bit-field is implementation-defined.
If target-expr and new-value identify overlapping objects, the behavior is undefined (unless the overlap is exact and the type is the same).
In overload resolution against user-defined operators , for every type T , the following function signatures participate in overload resolution:
For every enumeration or pointer to member type T , optionally volatile-qualified, the following function signature participates in overload resolution:
For every pair A1 and A2 , where A1 is an arithmetic type (optionally volatile-qualified) and A2 is a promoted arithmetic type, the following function signature participates in overload resolution:
[ edit ] Built-in compound assignment operator
The behavior of every built-in compound-assignment expression target-expr op = new-value is exactly the same as the behavior of the expression target-expr = target-expr op new-value , except that target-expr is evaluated only once.
The requirements on target-expr and new-value of built-in simple assignment operators also apply. Furthermore:
- For + = and - = , the type of target-expr must be an arithmetic type or a pointer to a (possibly cv-qualified) completely-defined object type .
- For all other compound assignment operators, the type of target-expr must be an arithmetic type.
In overload resolution against user-defined operators , for every pair A1 and A2 , where A1 is an arithmetic type (optionally volatile-qualified) and A2 is a promoted arithmetic type, the following function signatures participate in overload resolution:
For every pair I1 and I2 , where I1 is an integral type (optionally volatile-qualified) and I2 is a promoted integral type, the following function signatures participate in overload resolution:
For every optionally cv-qualified object type T , the following function signatures participate in overload resolution:
[ edit ] Example
Possible output:
[ edit ] Defect reports
The following behavior-changing defect reports were applied retroactively to previously published C++ standards.
[ edit ] See also
Operator precedence
Operator overloading
- Recent changes
- Offline version
- What links here
- Related changes
- Upload file
- Special pages
- Printable version
- Permanent link
- Page information
- In other languages
- This page was last modified on 25 January 2024, at 22:41.
- This page has been accessed 410,142 times.
- Privacy policy
- About cppreference.com
- Disclaimers

Code With C
The Way to Programming
- C Tutorials
- Java Tutorials
- Python Tutorials
- PHP Tutorials
- Java Projects
C++ And in If Statement: Writing Conditional Logic
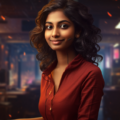
C++ and the “in” in if Statement: Mastering Conditional Logic Like a Pro! 💻
Hey there, fellow coders! Today, we’re going to unravel the magic behind the “if” statement in C++. Buckle up as we dive into the nitty-gritty of conditional logic and get cozy with those logical operators and nested if statements! Let’s get this programming party started 🚀
Basics of the “if” Statement in C++
So, you’ve got your hands on C++ and you’re ready to rock the world of conditional logic. The “if” statement is where it all begins. It’s like the superhero of decision-making in programming. You throw it a condition, and it makes things happen – just like that!
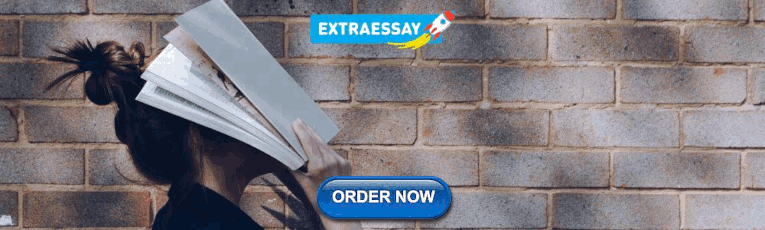
Syntax of the “if” Statement
In C++, the syntax for the “if” statement is as easy as pie:
Execution Flow of the “if” Statement
Now, let’s talk about the flow of execution. When the condition inside the “if” statement evaluates to true, the code block inside the curly braces gets executed. Simple, right? But wait, there’s more! If the condition is false, the code inside the block is totally ignored. It’s like having a secret passage in a game that opens only when you have the right key. 🗝️
Using Logical Operators in the “if” Statement
Alright, now it’s time to sprinkle some magic with logical operators in our “if” statements. We’re talking about the mighty && and the glorious ||!
&& Operator in the “if” Statement
The && operator is like the gatekeeper of our conditions. It ensures that all conditions are met before allowing the code block to be executed.
|| Operator in the “if” Statement
On the other hand, the || operator is more like a flexible friend. It lets the code block run if any of the conditions are true.
Nested “if” Statements in C++
Now, let’s take things up a notch with nested “if” statements. It’s like opening a treasure chest within a treasure chest – double the excitement, right?
Syntax of Nested “if” Statements
In C++, nesting “if” statements is as easy as stacking your favorite flavored Oreos. Check this out:
Benefits of Using Nested “if” Statements
Nested “if” statements give you the power to set up intricate conditions. It’s like creating a chain of events where each step depends on the previous one. Super cool, I must say!
Conditional Operators in the “if” Statement
Just when you thought things couldn’t get any cooler, here come the conditional operators! Say hello to the ternary operator – the ‘?:’ operator!
Ternary Operator in the “if” Statement
The ternary operator is a compact little fella that helps you take quick decisions within a single line of code. It’s like having a mini superhero that swoops in for quick saves.
Use of Conditional (or Ternary) Operator in C++
Here’s how you’d use the ternary operator in C++ to keep your code snazzy and concise:
In this example, if the condition (a > b) is true, ‘a’ is assigned to the variable ‘result’; otherwise, ‘b’ steps in and takes over. It’s like a swift game of passing the baton in a relay race!
Common Mistakes to Avoid in “if” Statements
Let’s admit it, we’ve all been there – the land of oopsie daisies! Here are a few goof-ups you might want to steer clear of when working with “if” statements.
Forgetting to Use Curly Brackets
You know, those little curly braces are like the safety belts of your code. Forgetting to use them can lead to some serious chaos. Always remember to snug your code within those braces!
Not Using Relational Operators Correctly in “if” Statements
Mixing up your relational operators? Oh boy, that can lead to some head-scratching bugs. Make sure you’re using the right operators to compare your values and sail smoothly through your conditions!
Phew, we’ve covered quite a bit of ground, haven’t we?! From basic “if” statements to nesting and the magic of logical and conditional operators, it’s been a rollercoaster ride of conditional logic. I’d say it’s time for a congratulatory pat on the back for coming this far, wouldn’t you? 🌟
Important note: patience is the key to mastering “if” statements. Take your time, experiment, make those errors, and remember to enjoy the journey! Now go out there and conquer the world of conditional logic with your newfound coding superpowers! 🦸♂️
Overall, diving deep into the intricacies of “if” statements has been an exhilarating journey! Remember, in the world of coding, it’s not about being error-free, it’s about learning and experimenting fearlessly. So, arm yourself with the knowledge and go write some impeccable, rock-solid “if” statements!
Keep coding, keep creating, and most importantly, keep being the amazing coder that you are. Until next time, happy coding and may your “if” statements always evaluate to true! 🎉
Program Code – C++ And in If Statement: Writing Conditional Logic
Code output:, code explanation:.
The program begins by including the iostream header, which is used to input and output data. After that, the using namespace std line allows us to avoid writing std:: before every standard function and object.
The main function is declared next, which is the entry point of the program. The function begins by declaring two variables: ‘score’ as an integer and ‘grade’ as a character data type. The score is then set to 85 to represent a student’s score.
The conditional logic starts with an if-else chain that assigns a grade based on the value of ‘score.’ This structure allows for execution of a distinct block of code depending on the score range. The largest score range is checked first, then the next largest, and so on until the smallest range. This ensures that the score is matched against the proper condition.
If none of the if or else if conditions are met (meaning the score is below 60), the else block is executed, assigning an ‘F’ to ‘grade.’
After determining the grade, the program prints the score and the corresponding grade to the console with an informative message.
Next, nested if statements are used to give additional feedback based on the score. If the score is above 50, a nested if checks whether the score is above 75 to print a congratulatory message. If it’s not, but still above 50, another message is printed, suggesting room for improvement. For scores below 50, an outer else block handles the response, indicating that the student did not pass and encouraging them to try again.
Finally, the main function returns zero, signaling the successful completion of the program. The conditional logic demonstrated here is fundamental to programming in C++ and essential in making decisions within the code based on different conditions.
You Might Also Like
The significance of ‘c’ in c programming language, c programming languages: understanding the basics and beyond, exploring the c programming language: from basics to advanced concepts, object-oriented programming: the pillar of modern software development, object-oriented coding: best practices and techniques.
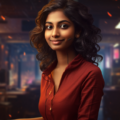
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Latest Posts
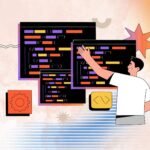
Ultimate Bug Tracker Project: A Python Programmer’s Guide
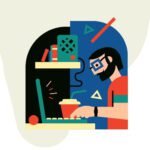
AI Shopping System: Advanced Python Project Ideas
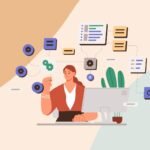
Detecting Credit Card Fraud Using Python – A Must-Try Project
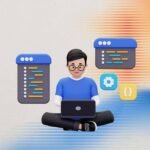
Cutting-Edge Python Data Leakage Detection System Project
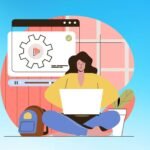
Python Projects: Building an Automated Payroll System with GPS Tracking and Image Capture Project
Privacy overview.
Sign in to your account
Username or Email Address
Remember Me
PrepBytes Blog
ONE-STOP RESOURCE FOR EVERYTHING RELATED TO CODING
Sign in to your account
Forgot your password?
Login via OTP
We will send you an one time password on your mobile number
An OTP has been sent to your mobile number please verify it below
Register with PrepBytes
Assignment operator in c.
Last Updated on June 23, 2023 by Prepbytes
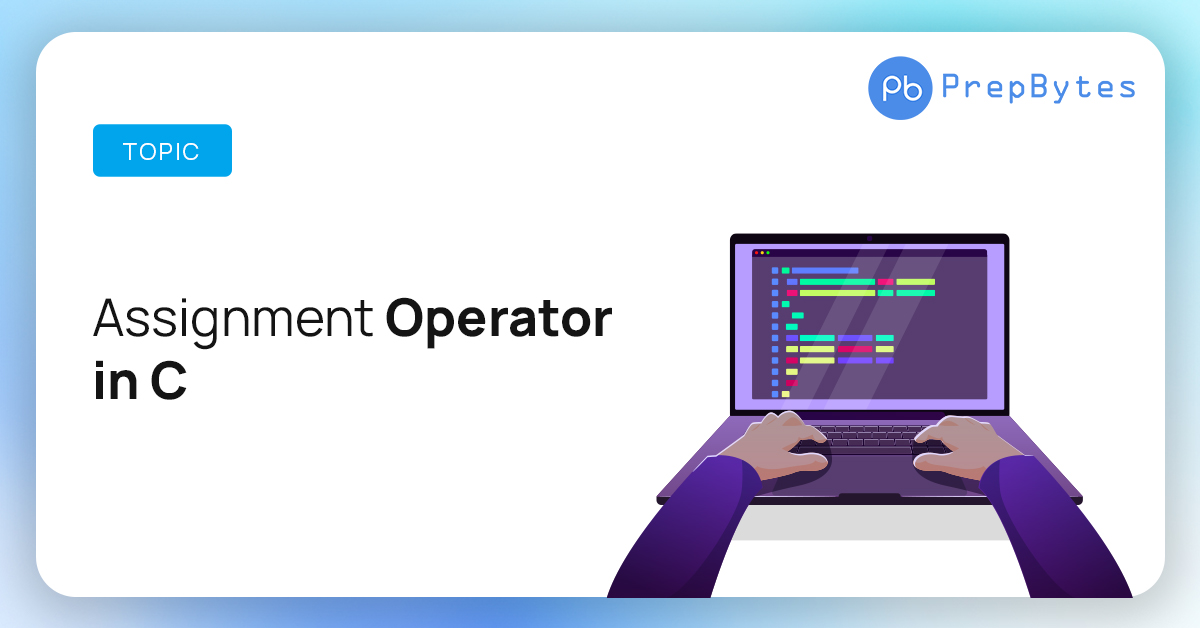
This type of operator is employed for transforming and assigning values to variables within an operation. In an assignment operation, the right side represents a value, while the left side corresponds to a variable. It is essential that the value on the right side has the same data type as the variable on the left side. If this requirement is not fulfilled, the compiler will issue an error.
What is Assignment Operator in C language?
In C, the assignment operator serves the purpose of assigning a value to a variable. It is denoted by the equals sign (=) and plays a vital role in storing data within variables for further utilization in code. When using the assignment operator, the value present on the right-hand side is assigned to the variable on the left-hand side. This fundamental operation allows developers to store and manipulate data effectively throughout their programs.
Example of Assignment Operator in C
For example, consider the following line of code:
Types of Assignment Operators in C
Here is a list of the assignment operators that you can find in the C language:
Simple assignment operator (=): This is the basic assignment operator, which assigns the value on the right-hand side to the variable on the left-hand side.
Addition assignment operator (+=): This operator adds the value on the right-hand side to the variable on the left-hand side and assigns the result back to the variable.
x += 3; // Equivalent to x = x + 3; (adds 3 to the current value of "x" and assigns the result back to "x")
Subtraction assignment operator (-=): This operator subtracts the value on the right-hand side from the variable on the left-hand side and assigns the result back to the variable.
x -= 4; // Equivalent to x = x – 4; (subtracts 4 from the current value of "x" and assigns the result back to "x")
* Multiplication assignment operator ( =):** This operator multiplies the value on the right-hand side with the variable on the left-hand side and assigns the result back to the variable.
x = 2; // Equivalent to x = x 2; (multiplies the current value of "x" by 2 and assigns the result back to "x")
Division assignment operator (/=): This operator divides the variable on the left-hand side by the value on the right-hand side and assigns the result back to the variable.
x /= 2; // Equivalent to x = x / 2; (divides the current value of "x" by 2 and assigns the result back to "x")
Bitwise AND assignment (&=): The bitwise AND assignment operator "&=" performs a bitwise AND operation between the value on the left-hand side and the value on the right-hand side. It then assigns the result back to the left-hand side variable.
x &= 3; // Binary: 0011 // After bitwise AND assignment: x = 1 (Binary: 0001)
Bitwise OR assignment (|=): The bitwise OR assignment operator "|=" performs a bitwise OR operation between the value on the left-hand side and the value on the right-hand side. It then assigns the result back to the left-hand side variable.
x |= 3; // Binary: 0011 // After bitwise OR assignment: x = 7 (Binary: 0111)
Bitwise XOR assignment (^=): The bitwise XOR assignment operator "^=" performs a bitwise XOR operation between the value on the left-hand side and the value on the right-hand side. It then assigns the result back to the left-hand side variable.
x ^= 3; // Binary: 0011 // After bitwise XOR assignment: x = 6 (Binary: 0110)
Left shift assignment (<<=): The left shift assignment operator "<<=" shifts the bits of the value on the left-hand side to the left by the number of positions specified by the value on the right-hand side. It then assigns the result back to the left-hand side variable.
x <<= 2; // Binary: 010100 (Shifted left by 2 positions) // After left shift assignment: x = 20 (Binary: 10100)
Right shift assignment (>>=): The right shift assignment operator ">>=" shifts the bits of the value on the left-hand side to the right by the number of positions specified by the value on the right-hand side. It then assigns the result back to the left-hand side variable.
x >>= 2; // Binary: 101 (Shifted right by 2 positions) // After right shift assignment: x = 5 (Binary: 101)
Conclusion The assignment operator in C, denoted by the equals sign (=), is used to assign a value to a variable. It is a fundamental operation that allows programmers to store data in variables for further use in their code. In addition to the simple assignment operator, C provides compound assignment operators that combine arithmetic or bitwise operations with assignment, allowing for concise and efficient code.
FAQs related to Assignment Operator in C
Q1. Can I assign a value of one data type to a variable of another data type? In most cases, assigning a value of one data type to a variable of another data type will result in a warning or error from the compiler. It is generally recommended to assign values of compatible data types to variables.
Q2. What is the difference between the assignment operator (=) and the comparison operator (==)? The assignment operator (=) is used to assign a value to a variable, while the comparison operator (==) is used to check if two values are equal. It is important not to confuse these two operators.
Q3. Can I use multiple assignment operators in a single statement? No, it is not possible to use multiple assignment operators in a single statement. Each assignment operator should be used separately for assigning values to different variables.
Q4. Are there any limitations on the right-hand side value of the assignment operator? The right-hand side value of the assignment operator should be compatible with the data type of the left-hand side variable. If the data types are not compatible, it may lead to unexpected behavior or compiler errors.
Q5. Can I assign the result of an expression to a variable using the assignment operator? Yes, it is possible to assign the result of an expression to a variable using the assignment operator. For example, x = y + z; assigns the sum of y and z to the variable x.
Q6. What happens if I assign a value to an uninitialized variable? Assigning a value to an uninitialized variable will initialize it with the assigned value. However, it is considered good practice to explicitly initialize variables before using them to avoid potential bugs or unintended behavior.
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
- Linked List
- Segment Tree
- Backtracking
- Dynamic Programming
- Greedy Algorithm
- Operating System
- Company Placement
- Interview Tips
- General Interview Questions
- Data Structure
- Other Topics
- Computational Geometry
- Game Theory
Related Post
Null character in c, ackermann function in c, median of two sorted arrays of different size in c, number is palindrome or not in c, implementation of queue using linked list in c, c program to replace a substring in a string.
Learn C++ practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn c++ interactively, c++ introduction.
- C++ Variables and Literals
- C++ Data Types
- C++ Basic I/O
- C++ Type Conversion
- C++ Operators
- C++ Comments
C++ Flow Control
- C++ if...else
- C++ for Loop
- C++ do...while Loop
- C++ continue
- C++ switch Statement
- C++ goto Statement
- C++ Functions
- C++ Function Types
- C++ Function Overloading
- C++ Default Argument
- C++ Storage Class
- C++ Recursion
- C++ Return Reference
C++ Arrays & String
- Multidimensional Arrays
- C++ Function and Array
- C++ Structures
- Structure and Function
- C++ Pointers to Structure
- C++ Enumeration
C++ Object & Class
- C++ Objects and Class
- C++ Constructors
- C++ Objects & Function
- C++ Operator Overloading
- C++ Pointers
- C++ Pointers and Arrays
- C++ Pointers and Functions
- C++ Memory Management
- C++ Inheritance
- Inheritance Access Control
- C++ Function Overriding
- Inheritance Types
- C++ Friend Function
- C++ Virtual Function
- C++ Templates
C++ Tutorials
C++ break Statement
C++ continue Statement
C++ Ternary Operator
C++ while and do...while Loop
- Display Factors of a Number
- C++ switch..case Statement
C++ if, if...else and Nested if...else
In computer programming, we use the if...else statement to run one block of code under certain conditions and another block of code under different conditions.
For example, assigning grades (A, B, C) based on marks obtained by a student.
- if the percentage is above 90 , assign grade A
- if the percentage is above 75 , assign grade B
- if the percentage is above 65 , assign grade C
There are three forms of if...else statements in C++.
- if statement
- if...else statement
- if...else if...else statement
- C++ if Statement
The syntax of the if statement is:
The if statement evaluates the condition inside the parentheses ( ) .
- If the condition evaluates to true , the code inside the body of if is executed.
- If the condition evaluates to false , the code inside the body of if is skipped.
Note: The code inside { } is the body of the if statement.
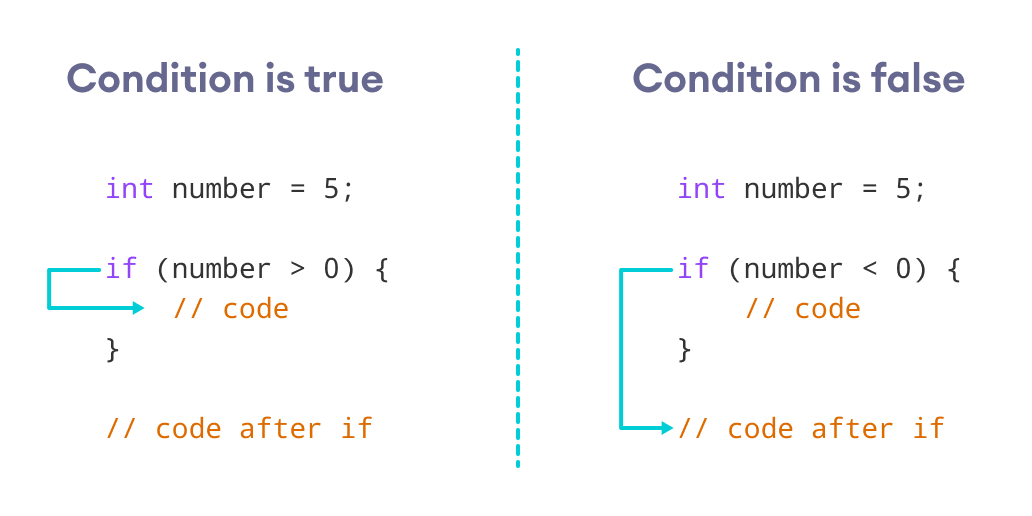
Example 1: C++ if Statement
When the user enters 5 , the condition number > 0 is evaluated to true and the statement inside the body of if is executed.
When the user enters -5 , the condition number > 0 is evaluated to false and the statement inside the body of if is not executed.
The if statement can have an optional else clause. Its syntax is:
The if..else statement evaluates the condition inside the parenthesis.
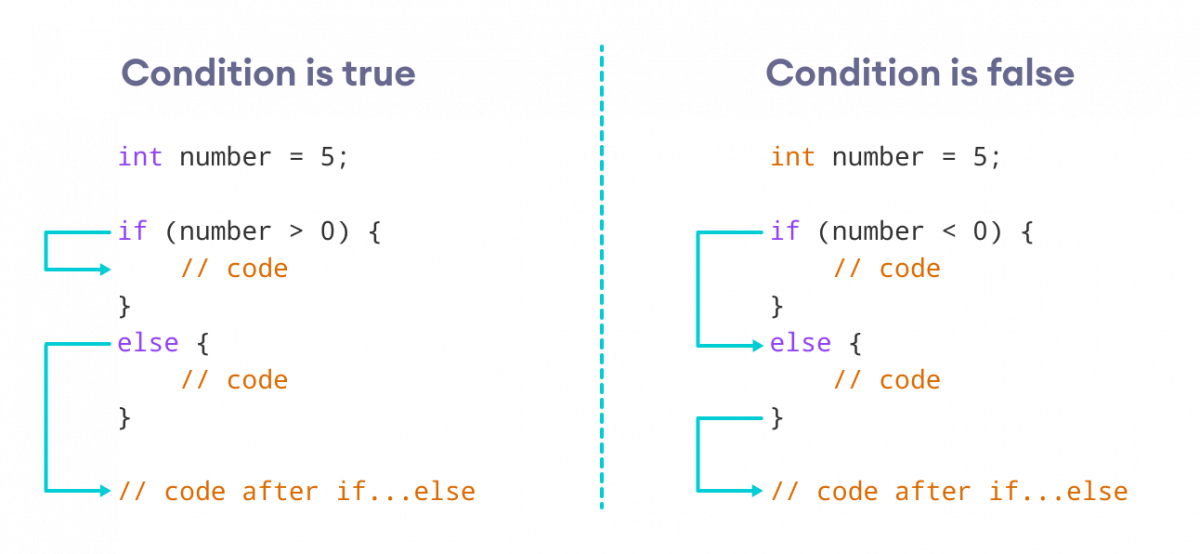
If the condition evaluates true ,
- the code inside the body of if is executed
- the code inside the body of else is skipped from execution
If the condition evaluates false ,
- the code inside the body of else is executed
- the code inside the body of if is skipped from execution
Example 2: C++ if...else Statement
In the above program, we have the condition number >= 0 . If we enter the number greater or equal to 0 , then the condition evaluates true .
Here, we enter 4 . So, the condition is true . Hence, the statement inside the body of if is executed.
Here, we enter -4 . So, the condition is false . Hence, the statement inside the body of else is executed.
- C++ if...else...else if statement
The if...else statement is used to execute a block of code among two alternatives. However, if we need to make a choice between more than two alternatives, we use the if...else if...else statement.
The syntax of the if...else if...else statement is:
- If condition1 evaluates to true , the code block 1 is executed.
- If condition1 evaluates to false , then condition2 is evaluated.
- If condition2 is true , the code block 2 is executed.
- If condition2 is false , the code block 3 is executed.
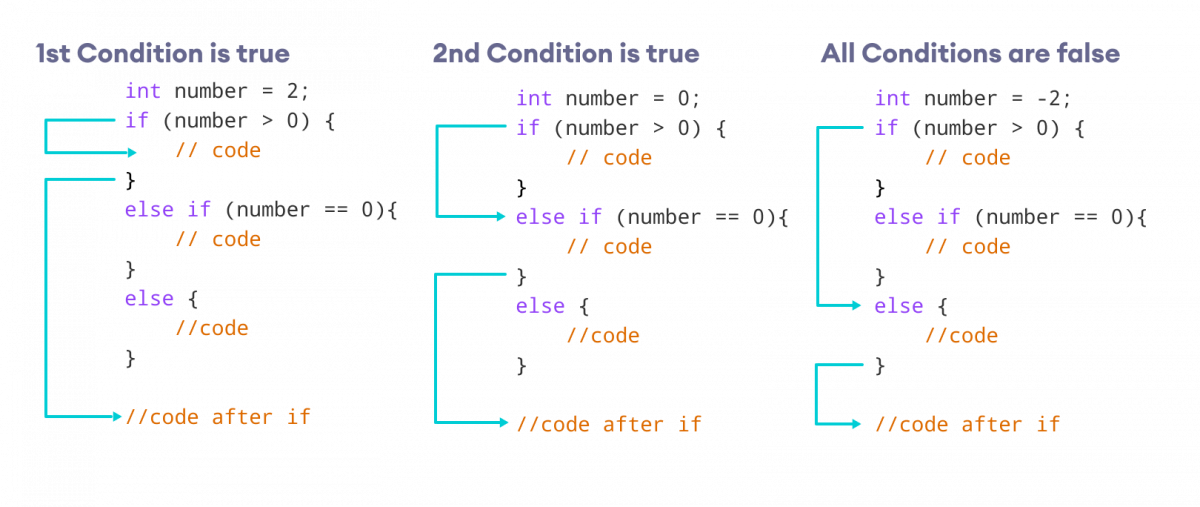
Note: There can be more than one else if statement but only one if and else statements.
Example 3: C++ if...else...else if
In this program, we take a number from the user. We then use the if...else if...else ladder to check whether the number is positive, negative, or zero.
If the number is greater than 0 , the code inside the if block is executed. If the number is less than 0 , the code inside the else if block is executed. Otherwise, the code inside the else block is executed.
- C++ Nested if...else
Sometimes, we need to use an if statement inside another if statement. This is known as nested if statement.
Think of it as multiple layers of if statements. There is a first, outer if statement, and inside it is another, inner if statement. Its syntax is:
- We can add else and else if statements to the inner if statement as required.
- The inner if statement can also be inserted inside the outer else or else if statements (if they exist).
- We can nest multiple layers of if statements.
Example 4: C++ Nested if
In the above example,
- We take an integer as an input from the user and store it in the variable num .
- If true , then the inner if...else statement is executed.
- If false , the code inside the outer else condition is executed, which prints "The number is 0 and it is neither positive nor negative."
- If true , then we print a statement saying that the number is positive.
- If false , we print that the number is negative.
Note: As you can see, nested if...else makes your logic complicated. If possible, you should always try to avoid nested if...else .
Body of if...else With Only One Statement
If the body of if...else has only one statement, you can omit { } in the program. For example, you can replace
The output of both programs will be the same.
Note: Although it's not necessary to use { } if the body of if...else has only one statement, using { } makes your code more readable.
More on Decision Making
In certain situations, a ternary operator can replace an if...else statement. To learn more, visit C++ Ternary Operator .
If we need to make a choice between more than one alternatives based on a given test condition, the switch statement can be used. To learn more, visit C++ switch .
- C++ Program to Check Whether Number is Even or Odd
- C++ Program to Check Whether a character is Vowel or Consonant.
- C++ Program to Find Largest Number Among Three Numbers
Table of Contents
- Introduction
- Example: if Statement
- Example: if...else Statement
- Example: C++ if...else...else if
- Example: Nested if...else
Sorry about that.
Related Tutorials
C++ Tutorial
The Federal Register
The daily journal of the united states government, request access.
Due to aggressive automated scraping of FederalRegister.gov and eCFR.gov, programmatic access to these sites is limited to access to our extensive developer APIs.
If you are human user receiving this message, we can add your IP address to a set of IPs that can access FederalRegister.gov & eCFR.gov; complete the CAPTCHA (bot test) below and click "Request Access". This process will be necessary for each IP address you wish to access the site from, requests are valid for approximately one quarter (three months) after which the process may need to be repeated.
An official website of the United States government.
If you want to request a wider IP range, first request access for your current IP, and then use the "Site Feedback" button found in the lower left-hand side to make the request.
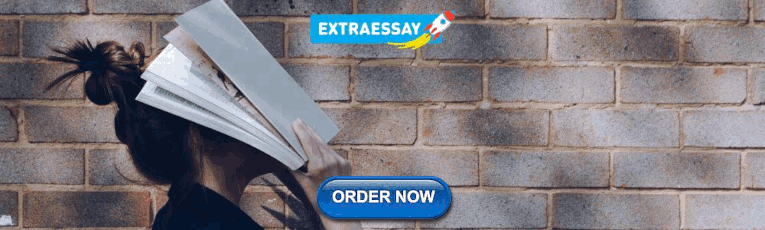
IMAGES
VIDEO
COMMENTS
Basically C evaluates expressions. In. s = data[q] The value of data[q] is the the value of expression here and the condition is evaluated based on that. The assignment. s <- data[q] is just a side-effect.
4. if-else-if Ladder in C. The if else if statements are used when the user has to decide among multiple options. The C if statements are executed from the top down. As soon as one of the conditions controlling the if is true, the statement associated with that if is executed, and the rest of the C else-if ladder is bypassed.
C++ culture is more accepting of assignment-in-condition than the other languages, and implicit boolean conversions are actually used in some idioms, e.g. type queries: ... however the problem is that you can't clearly understand Why there is an assignment within the while statement unless you keep the function name exactly same as ...
The if else statement essentially means that " if this condition is true do the following thing, else do this thing instead". If the condition inside the parentheses evaluates to true, the code inside the if block will execute. However, if that condition evaluates to false, the code inside the else block will execute.
Conditional code flow is the ability to change the way a piece of code behaves based on certain conditions. In such situations you can use if statements.. The if statement is also known as a decision making statement, as it makes a decision on the basis of a given condition or expression. The block of code inside the if statement is executed is the condition evaluates to true.
The working of the if statement in C is as follows: STEP 1: When the program control comes to the if statement, the test expression is evaluated. STEP 2A: If the condition is true, the statements inside the if block are executed. STEP 2B: If the expression is false, the statements inside the if body are not executed. STEP 3: Program control moves out of the if block and the code after the if ...
7 Assignment Expressions. As a general concept in programming, an assignment is a construct that stores a new value into a place where values can be stored—for instance, in a variable. Such places are called lvalues (see Lvalues) because they are locations that hold a value. An assignment in C is an expression because it has a value; we call it an assignment expression.
if Statement. If the condition evaluates to true, the code inside the curly braces {} executes. If the condition evaluates to false, the code doesn't execute. An `if` statement is used to test an expression for truth and execute some code based on it. Here's a simple form of the `if` statement:
This process is called decision making in 'C.'. In 'C' programming conditional statements are possible with the help of the following two constructs: 1. If statement. 2. If-else statement. It is also called as branching as a program decides which statement to execute based on the result of the evaluated condition.
The if statement is easy. When the user enters -2, the test expression number<0 is evaluated to true. Hence, You entered -2 is displayed on the screen. Output 2. Enter an integer: 5 The if statement is easy. When the user enters 5, the test expression number<0 is evaluated to false and the statement inside the body of if is not executed
1. else and else..if are optional statements, a program having only "if" statement would run fine. 2. else and else..if cannot be used without the "if". 3. There can be any number of else..if statement in a if else..if block. 4. If none of the conditions are met then the statements in else block gets executed. 5.
Here is our nested if-else statement, which checks to see if n is divisible by 8. If condition 2 returns true, we proceed to execute line 1. We print here that the integer is even and divisible by 8. If condition 2 is false, we enter the else statement and print that the number is even but not divisible by 8. Similarly, if condition 1 evaluates ...
Explanation. If the condition yields true after conversion to bool, statement-true is executed.. If the else part of the if statement is present and condition yields false after conversion to bool, statement-false is executed.. In the second form of if statement (the one including else), if statement-true is also an if statement then that inner if statement must contain an else part as well ...
for assignments to class type objects, the right operand could be an initializer list only when the assignment is defined by a user-defined assignment operator. removed user-defined assignment constraint. CWG 1538. C++11. E1 ={E2} was equivalent to E1 = T(E2) ( T is the type of E1 ), this introduced a C-style cast. it is equivalent to E1 = T{E2}
Program Code - C++ And in If Statement: Writing Conditional Logic. Copy Code. #include <iostream>. using namespace std; int main () { int score = 85; // Initialize a variable to store the score. char grade; // Variable to store the grade. // The if-else chain begins here to handle different ranges of scores.
In C, the assignment operator serves the purpose of assigning a value to a variable. It is denoted by the equals sign (=) and plays a vital role in storing data within variables for further utilization in code. When using the assignment operator, the value present on the right-hand side is assigned to the variable on the left-hand side.
In all the cases you've shown, it's still true that you've provided an expression that eventually is convertible to bool.If you look at the << operator of std::cout and similar ostreams, you'll see they all return a reference to the same stream. This stream also defines a conversion to bool operator, which is implicitly called in a context where a bool is expected.
In computer programming, we use the if...else statement to run one block of code under certain conditions and another block of code under different conditions.. For example, assigning grades (A, B, C) based on marks obtained by a student. if the percentage is above 90, assign grade A; if the percentage is above 75, assign grade B; if the percentage is above 65, assign grade C
The result of the assignment operation is the value stored in the left operand after the assignment has taken place; the result is an lvalue. The result of the expression a = 5 is 5. [6.4/4] [..] The value of a condition that is an expression is the value of the expression, implicitly converted to bool for statements other than switch.
The text of those statements may be examined at the places specified in Item IV below. The Exchange has prepared summaries, set forth in sections A, B, and C below, of the most significant parts of such statements. A. Self-Regulatory Organization's Statement of the Purpose of, and the Statutory Basis for, the Proposed Rule Change 1. Purpose