std::vector operator=
- since C++20
- since C++17
- since C++11
- until C++11
Replaces the contents of the container with the contents of another.
(1) Copy assignment operator. Replaces the contents with a copy of the contents of other .
If std::allocator_traits<Alloc>::propagate_on_container_copy_assignment::value is true , the allocator of *this is replaced by a copy of that of other.
If the allocator of *this after assignment would compare unequal to its old value, the old allocator is used to deallocate the memory, then the new allocator is used to allocate it before copying the elements.
Otherwise, the memory owned by *this may be reused when possible.
In any case, the elements originally belong to *this may be either destroyed or replaced by element-wise copy-assignment.
(2) Move assignment operator. Replaces the contents with those of other using move semantics (i.e. the data in other is moved from other into this container).
other is in a valid but unspecified state afterwards.
If std::allocator_traits<Alloc>::propagate_on_container_move_assignment::value is true , the allocator of *this is replaced by a copy of that of other.
If it is false and the allocators of *this and other do not compare equal, *this cannot take ownership of the memory owned by other and must move-assign each element individually, allocating additional memory using its own allocator as needed.
In any case, all elements originally belong to *this are either destroyed or replaced by element-wise move-assignment.
(3) Replaces the contents with those identified by initializer list ilist .
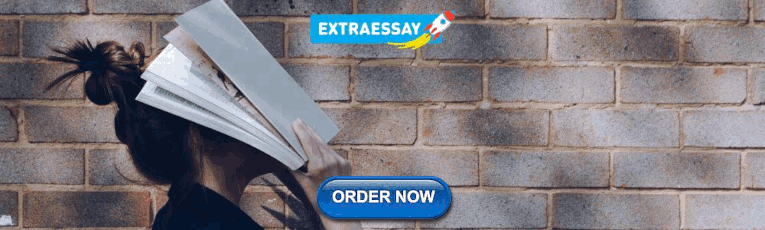
Parameters
- other - another container to use as data source
- ilist - initializer list to use as data source
Return value
Complexity .
(1) Linear in the size of *this and other - O(size() + other.size(()) .
(2) Linear in the size of *this - O(size()) . If allocators do not compare equal and do not propagate, linear in the size of *this and other - O(size() + other.size()) .
(3) Linear in the size of *this and ilist - O(size() + ilist.size()) .
Exceptions
- until C++17
- (1, 2) May throw implementation-defined exceptions.
- (3) Noexcept specification:
After container move assignment (overload (2) ), unless element-wise move assignment is forced by incompatible allocators, references, pointers, and iterators (other than the end iterator) to other remain valid, but refer to elements that are now in *this . The current standard makes this guarantee via the blanket statement in [container.requirements.general]/12 , and a more direct guarantee is under consideration via LWG 2321 .
- Return value
std::vector:: operator=
Replaces the contents of the container.
[ edit ] Parameters
[ edit ] return value, [ edit ] complexity, [ edit ] example.
The following code uses to assign one std:: vector to another:
[ edit ] See also
- conditionally noexcept
- <cassert> (assert.h)
- <cctype> (ctype.h)
- <cerrno> (errno.h)
- C++11 <cfenv> (fenv.h)
- <cfloat> (float.h)
- C++11 <cinttypes> (inttypes.h)
- <ciso646> (iso646.h)
- <climits> (limits.h)
- <clocale> (locale.h)
- <cmath> (math.h)
- <csetjmp> (setjmp.h)
- <csignal> (signal.h)
- <cstdarg> (stdarg.h)
- C++11 <cstdbool> (stdbool.h)
- <cstddef> (stddef.h)
- C++11 <cstdint> (stdint.h)
- <cstdio> (stdio.h)
- <cstdlib> (stdlib.h)
- <cstring> (string.h)
- C++11 <ctgmath> (tgmath.h)
- <ctime> (time.h)
- C++11 <cuchar> (uchar.h)
- <cwchar> (wchar.h)
- <cwctype> (wctype.h)
Containers:
- C++11 <array>
- <deque>
- C++11 <forward_list>
- <list>
- <map>
- <queue>
- <set>
- <stack>
- C++11 <unordered_map>
- C++11 <unordered_set>
- <vector>
Input/Output:
- <fstream>
- <iomanip>
- <ios>
- <iosfwd>
- <iostream>
- <istream>
- <ostream>
- <sstream>
- <streambuf>
Multi-threading:
- C++11 <atomic>
- C++11 <condition_variable>
- C++11 <future>
- C++11 <mutex>
- C++11 <thread>
- <algorithm>
- <bitset>
- C++11 <chrono>
- C++11 <codecvt>
- <complex>
- <exception>
- <functional>
- C++11 <initializer_list>
- <iterator>
- <limits>
- <locale>
- <memory>
- <new>
- <numeric>
- C++11 <random>
- C++11 <ratio>
- C++11 <regex>
- <stdexcept>
- <string>
- C++11 <system_error>
- C++11 <tuple>
- C++11 <type_traits>
- C++11 <typeindex>
- <typeinfo>
- <utility>
- <valarray>
- vector<bool>
- vector::~vector
- vector::vector
member functions
- vector::assign
- vector::back
- vector::begin
- vector::capacity
- C++11 vector::cbegin
- C++11 vector::cend
- vector::clear
- C++11 vector::crbegin
- C++11 vector::crend
- C++11 vector::data
- C++11 vector::emplace
- C++11 vector::emplace_back
- vector::empty
- vector::end
- vector::erase
- vector::front
- vector::get_allocator
- vector::insert
- vector::max_size
- vector::operator[]
- vector::operator=
- vector::pop_back
- vector::push_back
- vector::rbegin
- vector::rend
- vector::reserve
- vector::resize
- C++11 vector::shrink_to_fit
- vector::size
- vector::swap
non-member overloads
- relational operators (vector)
- swap (vector)
std:: vector ::operator[]
Return value, iterator validity, exception safety.

21.12 — Overloading the assignment operator
The copy assignment operator (operator=) is used to copy values from one object to another already existing object .
Related content
As of C++11, C++ also supports “Move assignment”. We discuss move assignment in lesson 22.3 -- Move constructors and move assignment .
Copy assignment vs Copy constructor
The purpose of the copy constructor and the copy assignment operator are almost equivalent -- both copy one object to another. However, the copy constructor initializes new objects, whereas the assignment operator replaces the contents of existing objects.
The difference between the copy constructor and the copy assignment operator causes a lot of confusion for new programmers, but it’s really not all that difficult. Summarizing:
- If a new object has to be created before the copying can occur, the copy constructor is used (note: this includes passing or returning objects by value).
- If a new object does not have to be created before the copying can occur, the assignment operator is used.
Overloading the assignment operator
Overloading the copy assignment operator (operator=) is fairly straightforward, with one specific caveat that we’ll get to. The copy assignment operator must be overloaded as a member function.
This prints:
This should all be pretty straightforward by now. Our overloaded operator= returns *this, so that we can chain multiple assignments together:
Issues due to self-assignment
Here’s where things start to get a little more interesting. C++ allows self-assignment:
This will call f1.operator=(f1), and under the simplistic implementation above, all of the members will be assigned to themselves. In this particular example, the self-assignment causes each member to be assigned to itself, which has no overall impact, other than wasting time. In most cases, a self-assignment doesn’t need to do anything at all!
However, in cases where an assignment operator needs to dynamically assign memory, self-assignment can actually be dangerous:
First, run the program as it is. You’ll see that the program prints “Alex” as it should.
Now run the following program:
You’ll probably get garbage output. What happened?
Consider what happens in the overloaded operator= when the implicit object AND the passed in parameter (str) are both variable alex. In this case, m_data is the same as str.m_data. The first thing that happens is that the function checks to see if the implicit object already has a string. If so, it needs to delete it, so we don’t end up with a memory leak. In this case, m_data is allocated, so the function deletes m_data. But because str is the same as *this, the string that we wanted to copy has been deleted and m_data (and str.m_data) are dangling.
Later on, we allocate new memory to m_data (and str.m_data). So when we subsequently copy the data from str.m_data into m_data, we’re copying garbage, because str.m_data was never initialized.
Detecting and handling self-assignment
Fortunately, we can detect when self-assignment occurs. Here’s an updated implementation of our overloaded operator= for the MyString class:
By checking if the address of our implicit object is the same as the address of the object being passed in as a parameter, we can have our assignment operator just return immediately without doing any other work.
Because this is just a pointer comparison, it should be fast, and does not require operator== to be overloaded.
When not to handle self-assignment
Typically the self-assignment check is skipped for copy constructors. Because the object being copy constructed is newly created, the only case where the newly created object can be equal to the object being copied is when you try to initialize a newly defined object with itself:
In such cases, your compiler should warn you that c is an uninitialized variable.
Second, the self-assignment check may be omitted in classes that can naturally handle self-assignment. Consider this Fraction class assignment operator that has a self-assignment guard:
If the self-assignment guard did not exist, this function would still operate correctly during a self-assignment (because all of the operations done by the function can handle self-assignment properly).
Because self-assignment is a rare event, some prominent C++ gurus recommend omitting the self-assignment guard even in classes that would benefit from it. We do not recommend this, as we believe it’s a better practice to code defensively and then selectively optimize later.
The copy and swap idiom
A better way to handle self-assignment issues is via what’s called the copy and swap idiom. There’s a great writeup of how this idiom works on Stack Overflow .
The implicit copy assignment operator
Unlike other operators, the compiler will provide an implicit public copy assignment operator for your class if you do not provide a user-defined one. This assignment operator does memberwise assignment (which is essentially the same as the memberwise initialization that default copy constructors do).
Just like other constructors and operators, you can prevent assignments from being made by making your copy assignment operator private or using the delete keyword:
Note that if your class has const members, the compiler will instead define the implicit operator= as deleted. This is because const members can’t be assigned, so the compiler will assume your class should not be assignable.
If you want a class with const members to be assignable (for all members that aren’t const), you will need to explicitly overload operator= and manually assign each non-const member.
- Standard Template Library
- STL Priority Queue
- STL Interview Questions
- STL Cheatsheet
- C++ Templates
- C++ Functors
- C++ Iterators
- Vector in C++ STL
- Initialize a vector in C++ (7 different ways)
Commonly Used Methods
- vector::begin() and vector::end() in C++ STL
- vector::empty() and vector::size() in C++ STL
- vector::operator= and vector::operator[ ] in C++ STL
- vector::front() and vector::back() in C++ STL
- vector::push_back() and vector::pop_back() in C++ STL
- vector insert() Function in C++ STL
- vector emplace() function in C++ STL
vector :: assign() in C++ STL
- vector erase() and clear() in C++
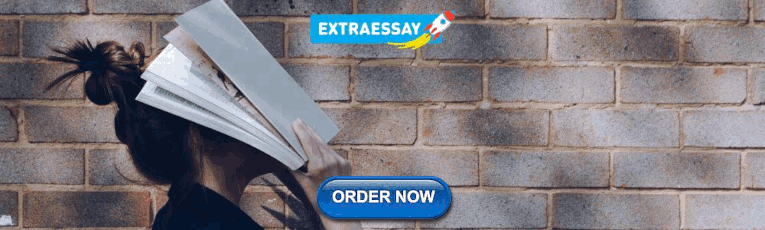
Other Member Methods
- vector max_size() function in C++ STL
- vector capacity() function in C++ STL
- vector rbegin() and rend() function in C++ STL
- vector :: cbegin() and vector :: cend() in C++ STL
- vector::crend() & vector::crbegin() with example
- vector : : resize() in C++ STL
- vector shrink_to_fit() function in C++ STL
- Using std::vector::reserve whenever possible
- vector data() function in C++ STL
- 2D Vector In C++ With User Defined Size
- Passing Vector to a Function in C++
- How does a vector work in C++?
- How to implement our own Vector Class in C++?
- Advantages of vector over array in C++
Common Vector Programs
- Sorting a vector in C++
- How to reverse a Vector using STL in C++?
- How to find the minimum and maximum element of a Vector using STL in C++?
- How to find index of a given element in a Vector in C++
vector:: assign() is an STL in C++ which assigns new values to the vector elements by replacing old ones. It can also modify the size of the vector if necessary.
The syntax for assigning constant values:
Program 1: The program below shows how to assign constant values to a vector
The syntax for assigning values from an array or list:
Program 2: The program below shows how to assign values from an array or list
The syntax for modifying values from a vector
Program 3: The program below shows how to modify the vector
Time Complexity – Linear O(N)
Syntax for assigning values with initializer list:
Program 4:The program below shows how to assign a vector with an initializer list.
Please Login to comment...
Similar reads.
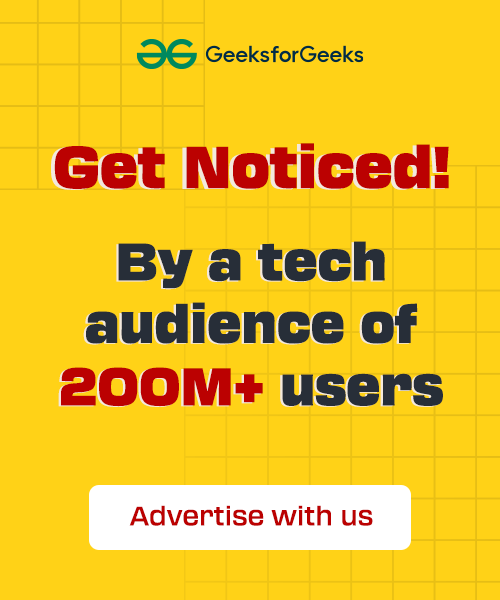
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
cppreference.com
Copy assignment operator.
A copy assignment operator is a non-template non-static member function with the name operator = that can be called with an argument of the same class type and copies the content of the argument without mutating the argument.
[ edit ] Syntax
For the formal copy assignment operator syntax, see function declaration . The syntax list below only demonstrates a subset of all valid copy assignment operator syntaxes.
[ edit ] Explanation
The copy assignment operator is called whenever selected by overload resolution , e.g. when an object appears on the left side of an assignment expression.
[ edit ] Implicitly-declared copy assignment operator
If no user-defined copy assignment operators are provided for a class type, the compiler will always declare one as an inline public member of the class. This implicitly-declared copy assignment operator has the form T & T :: operator = ( const T & ) if all of the following is true:
- each direct base B of T has a copy assignment operator whose parameters are B or const B & or const volatile B & ;
- each non-static data member M of T of class type or array of class type has a copy assignment operator whose parameters are M or const M & or const volatile M & .
Otherwise the implicitly-declared copy assignment operator is declared as T & T :: operator = ( T & ) .
Due to these rules, the implicitly-declared copy assignment operator cannot bind to a volatile lvalue argument.
A class can have multiple copy assignment operators, e.g. both T & T :: operator = ( T & ) and T & T :: operator = ( T ) . If some user-defined copy assignment operators are present, the user may still force the generation of the implicitly declared copy assignment operator with the keyword default . (since C++11)
The implicitly-declared (or defaulted on its first declaration) copy assignment operator has an exception specification as described in dynamic exception specification (until C++17) noexcept specification (since C++17)
Because the copy assignment operator is always declared for any class, the base class assignment operator is always hidden. If a using-declaration is used to bring in the assignment operator from the base class, and its argument type could be the same as the argument type of the implicit assignment operator of the derived class, the using-declaration is also hidden by the implicit declaration.
[ edit ] Implicitly-defined copy assignment operator
If the implicitly-declared copy assignment operator is neither deleted nor trivial, it is defined (that is, a function body is generated and compiled) by the compiler if odr-used or needed for constant evaluation (since C++14) . For union types, the implicitly-defined copy assignment copies the object representation (as by std::memmove ). For non-union class types, the operator performs member-wise copy assignment of the object's direct bases and non-static data members, in their initialization order, using built-in assignment for the scalars, memberwise copy-assignment for arrays, and copy assignment operator for class types (called non-virtually).
[ edit ] Deleted copy assignment operator
An implicitly-declared or explicitly-defaulted (since C++11) copy assignment operator for class T is undefined (until C++11) defined as deleted (since C++11) if any of the following conditions is satisfied:
- T has a non-static data member of a const-qualified non-class type (or possibly multi-dimensional array thereof).
- T has a non-static data member of a reference type.
- T has a potentially constructed subobject of class type M (or possibly multi-dimensional array thereof) such that the overload resolution as applied to find M 's copy assignment operator
- does not result in a usable candidate, or
- in the case of the subobject being a variant member , selects a non-trivial function.
[ edit ] Trivial copy assignment operator
The copy assignment operator for class T is trivial if all of the following is true:
- it is not user-provided (meaning, it is implicitly-defined or defaulted);
- T has no virtual member functions;
- T has no virtual base classes;
- the copy assignment operator selected for every direct base of T is trivial;
- the copy assignment operator selected for every non-static class type (or array of class type) member of T is trivial.
A trivial copy assignment operator makes a copy of the object representation as if by std::memmove . All data types compatible with the C language (POD types) are trivially copy-assignable.
[ edit ] Eligible copy assignment operator
Triviality of eligible copy assignment operators determines whether the class is a trivially copyable type .
[ edit ] Notes
If both copy and move assignment operators are provided, overload resolution selects the move assignment if the argument is an rvalue (either a prvalue such as a nameless temporary or an xvalue such as the result of std::move ), and selects the copy assignment if the argument is an lvalue (named object or a function/operator returning lvalue reference). If only the copy assignment is provided, all argument categories select it (as long as it takes its argument by value or as reference to const, since rvalues can bind to const references), which makes copy assignment the fallback for move assignment, when move is unavailable.
It is unspecified whether virtual base class subobjects that are accessible through more than one path in the inheritance lattice, are assigned more than once by the implicitly-defined copy assignment operator (same applies to move assignment ).
See assignment operator overloading for additional detail on the expected behavior of a user-defined copy-assignment operator.
[ edit ] Example
[ edit ] defect reports.
The following behavior-changing defect reports were applied retroactively to previously published C++ standards.
[ edit ] See also
- converting constructor
- copy constructor
- copy elision
- default constructor
- aggregate initialization
- constant initialization
- copy initialization
- default initialization
- direct initialization
- initializer list
- list initialization
- reference initialization
- value initialization
- zero initialization
- move assignment
- move constructor
- Recent changes
- Offline version
- What links here
- Related changes
- Upload file
- Special pages
- Printable version
- Permanent link
- Page information
- In other languages
- This page was last modified on 2 February 2024, at 15:13.
- This page has been accessed 1,333,785 times.
- Privacy policy
- About cppreference.com
- Disclaimers

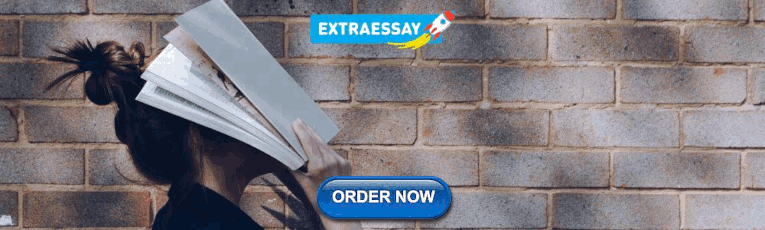
IMAGES
VIDEO
COMMENTS
The assignment operator does this, as does vector::assign: v2.assign(v1.begin(), v1.end()); ... The invocation of std::copy may try to access items beyond the end of the destination vector. Use assignment. It's not your job to micro-optimize: that's the library writer's responsibility, and ultimately the compiler's responsibility. ...
The copy assignment (1) copies all the elements from x into the container (with x preserving its contents). The move assignment (2) moves the elements of x into the container (x is left in an unspecified but valid state). The initializer list assignment (3) copies the elements of il into the container. The container preserves its current allocator, except if the allocator traits indicate that ...
1. It is used to assign new contents to the container and replace its current contents. It is used to assign new contents to the container by replacing its current contents. 2. Its syntax is -: vector& operator= (const vector& x); Its syntax is -: vector& operator= (const vector& x); 3.
Actually there are three assignment operators defined: vector& operator=( const vector& other ); vector& operator=( vector&& other ); vector& operator=( std::initializer_list<T> ilist ); Your suggestion vector& vector::operator=(vector other) uses the copy and swap idiom. That means, that when the operator is called, the original vector will be ...
1) Copy assignment operator. Replaces the contents with a copy of the contents of other . If std::allocator_traits<allocator_type>::propagate_on_container_copy_assignment::value is true, the allocator of *this is replaced by a copy of other. If the allocator of *this after assignment would compare unequal to its old value, the old allocator is ...
std::vector<T,Allocator>:: assign. Replaces the contents of the container. 1) Replaces the contents with count copies of value value. 2) Replaces the contents with copies of those in the range [first,last). The behavior is undefined if either argument is an iterator into *this . This overload has the same effect as overload (1) if InputIt is an ...
Complexity Linear on initial and final sizes (destructions, constructions). Additionally, in the range version (1), if InputIterator is not at least of a forward iterator category (i.e., it is just an input iterator) the new capacity cannot be determined beforehand and the operation incurs in additional logarithmic complexity in the new size (reallocations while growing).
for assignments to class type objects, the right operand could be an initializer list only when the assignment is defined by a user-defined assignment operator. removed user-defined assignment constraint. CWG 1538. C++11. E1 ={E2} was equivalent to E1 = T(E2) ( T is the type of E1 ), this introduced a C-style cast. it is equivalent to E1 = T{E2}
std::vector operator=. Replaces the contents of the container with the contents of another. (1) Copy assignment operator. Replaces the contents with a copy of the contents of other. If std::allocator_traits<Alloc>::propagate_on_container_copy_assignment::value is true, the allocator of *this is replaced by a copy of that of other.
2) Move assignment operator. Replaces the contents with those of other using move semantics (i.e. the data in other is moved from other into this container).other is in a valid but unspecified state afterwards. If std::allocator_traits<allocator_type>::propagate_on_container_move_assignment() is true, the target allocator is replaced by a copy of the source allocator.
Returns a reference to the element at position n in the vector container. A similar member function, vector::at, has the same behavior as this operator function, except that vector::at is bound-checked and signals if the requested position is out of range by throwing an out_of_range exception. Portable programs should never call this function with an argument n that is out of range, since this ...
21.12 — Overloading the assignment operator. The copy assignment operator (operator=) is used to copy values from one object to another already existing object. As of C++11, C++ also supports "Move assignment". We discuss move assignment in lesson 22.3 -- Move constructors and move assignment .
vector:: assign () is an STL in C++ which assigns new values to the vector elements by replacing old ones. It can also modify the size of the vector if necessary. The syntax for assigning constant values: vectorname.assign(int size, int value) Parameters: size - number of values to be assigned.
Assignment performs implicit conversion from the value of rhs to the type of lhs and then replaces the value in the object designated by lhs with the converted value of rhs . Assignment also returns the same value as what was stored in lhs (so that expressions such as a = b = c are possible). The value category of the assignment operator is non ...
3. Now you can release the old resources. This is unlikely to go wrong; But even if something goes wrong your object is in a good state. So your Copy assignment should look like this: MyVector& operator=(const MyVector& rhs) {. // Make a copy to temp. std::size_t tSize = rhs.m_Size; int* tInt = new int[tSize];
The move assignment operator is called whenever it is selected by overload resolution, e.g. when an object appears on the left-hand side of an assignment expression, where the right-hand side is an rvalue of the same or implicitly convertible type.. Move assignment operators typically "steal" the resources held by the argument (e.g. pointers to dynamically-allocated objects, file descriptors ...
The Copy Assignment Operator in a class is a non-template non-static member function that is declared with the operator=. When you create a class or a type that is copy assignable (that you can copy with the = operator symbol), it must have a public copy assignment operator. Here is a simple syntax for the typical declaration of a copy ...
The problem is v1 = v2; would work if both are instances of the same std::vector<T> (and T provides an assignment operator) but you left out the T in your question. - Scheff's Cat Sep 21, 2018 at 14:46
the copy assignment operator selected for every non-static class type (or array of class type) member of T is trivial. A trivial copy assignment operator makes a copy of the object representation as if by std::memmove. All data types compatible with the C language (POD types) are trivially copy-assignable.