Compound-Assignment Operators
- Java Programming
- PHP Programming
- Javascript Programming
- Delphi Programming
- C & C++ Programming
- Ruby Programming
- Visual Basic
- M.A., Advanced Information Systems, University of Glasgow
Compound-assignment operators provide a shorter syntax for assigning the result of an arithmetic or bitwise operator. They perform the operation on the two operands before assigning the result to the first operand.
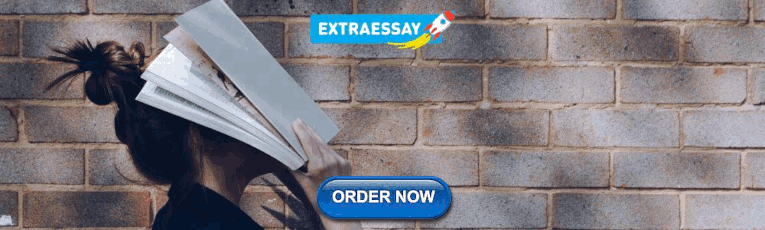
Compound-Assignment Operators in Java
Java supports 11 compound-assignment operators:
Example Usage
To assign the result of an addition operation to a variable using the standard syntax:
But use a compound-assignment operator to effect the same outcome with the simpler syntax:
- Conditional Operators
- Java Expressions Introduced
- Understanding the Concatenation of Strings in Java
- The 7 Best Programming Languages to Learn for Beginners
- Math Glossary: Mathematics Terms and Definitions
- The Associative and Commutative Properties
- The JavaScript Ternary Operator as a Shortcut for If/Else Statements
- Parentheses, Braces, and Brackets in Math
- Basic Guide to Creating Arrays in Ruby
- Dividing Monomials in Basic Algebra
- C++ Handling Ints and Floats
- An Abbreviated JavaScript If Statement
- Using the Switch Statement for Multiple Choices in Java
- How to Make Deep Copies in Ruby
- Definition of Variable
- The History of Algebra
- C++ Language
- Ascii Codes
- Boolean Operations
- Numerical Bases
Introduction
Basics of c++.
- Structure of a program
- Variables and types
- Basic Input/Output
Program structure
- Statements and flow control
- Overloads and templates
- Name visibility
Compound data types
- Character sequences
- Dynamic memory
- Data structures
- Other data types
- Classes (I)
- Classes (II)
- Special members
- Friendship and inheritance
- Polymorphism
Other language features
- Type conversions
- Preprocessor directives
Standard library
- Input/output with files
Assignment operator (=)
Arithmetic operators ( +, -, *, /, % ), compound assignment (+=, -=, *=, /=, %=, >>=, <<=, &=, ^=, |=), increment and decrement (++, --), relational and comparison operators ( ==, =, >, <, >=, <= ), logical operators ( , &&, || ), conditional ternary operator ( ), comma operator ( , ), bitwise operators ( &, |, ^, ~, <<, >> ), explicit type casting operator, other operators, precedence of operators.
- Java Arrays
- Java Strings
- Java Collection
- Java 8 Tutorial
- Java Multithreading
- Java Exception Handling
- Java Programs
- Java Project
- Java Collections Interview
- Java Interview Questions
- Spring Boot
Compound assignment operators in Java
- Java Assignment Operators with Examples
- Basic Operators in Java
- Bitwise Operators in Java
- Difference between Simple and Compound Assignment in Java
- Java Arithmetic Operators with Examples
- Interesting facts about Increment and Decrement operators in Java
- Java Logical Operators with Examples
- Java | Operators | Question 8
- Java | Operators | Question 1
- Java | Operators | Question 3
- Java | Operators | Question 7
- Java | Operators | Question 4
- Assignment Operators in C
- Assignment Operators In C++
- Augmented Assignment Operators in Python
- Assignment Operators in Programming
- Operators in Java
- Solidity - Assignment Operators
- Arithmetic Operators in Solidity
- Arrays in Java
- Spring Boot - Start/Stop a Kafka Listener Dynamically
- Parse Nested User-Defined Functions using Spring Expression Language (SpEL)
- Split() String method in Java with examples
- Arrays.sort() in Java with examples
- For-each loop in Java
- Object Oriented Programming (OOPs) Concept in Java
- Reverse a string in Java
- HashMap in Java
- How to iterate any Map in Java
Compound-assignment operators provide a shorter syntax for assigning the result of an arithmetic or bitwise operator. They perform the operation on the two operands before assigning the result to the first operand. The following are all possible assignment operator in java:
Implementation of all compound assignment operator
Rules for resolving the Compound assignment operators
At run time, the expression is evaluated in one of two ways.Depending upon the programming conditions:
- First, the left-hand operand is evaluated to produce a variable. If this evaluation completes abruptly, then the assignment expression completes abruptly for the same reason; the right-hand operand is not evaluated and no assignment occurs.
- Otherwise, the value of the left-hand operand is saved and then the right-hand operand is evaluated. If this evaluation completes abruptly, then the assignment expression completes abruptly for the same reason and no assignment occurs.
- Otherwise, the saved value of the left-hand variable and the value of the right-hand operand are used to perform the binary operation indicated by the compound assignment operator. If this operation completes abruptly, then the assignment expression completes abruptly for the same reason and no assignment occurs.
- Otherwise, the result of the binary operation is converted to the type of the left-hand variable, subjected to value set conversion to the appropriate standard value set, and the result of the conversion is stored into the variable.
- First, the array reference sub-expression of the left-hand operand array access expression is evaluated. If this evaluation completes abruptly, then the assignment expression completes abruptly for the same reason; the index sub-expression (of the left-hand operand array access expression) and the right-hand operand are not evaluated and no assignment occurs.
- Otherwise, the index sub-expression of the left-hand operand array access expression is evaluated. If this evaluation completes abruptly, then the assignment expression completes abruptly for the same reason and the right-hand operand is not evaluated and no assignment occurs.
- Otherwise, if the value of the array reference sub-expression is null, then no assignment occurs and a NullPointerException is thrown.
- Otherwise, the value of the array reference sub-expression indeed refers to an array. If the value of the index sub-expression is less than zero, or greater than or equal to the length of the array, then no assignment occurs and an ArrayIndexOutOfBoundsException is thrown.
- Otherwise, the value of the index sub-expression is used to select a component of the array referred to by the value of the array reference sub-expression. The value of this component is saved and then the right-hand operand is evaluated. If this evaluation completes abruptly, then the assignment expression completes abruptly for the same reason and no assignment occurs.
Examples : Resolving the statements with Compound assignment operators
We all know that whenever we are assigning a bigger value to a smaller data type variable then we have to perform explicit type casting to get the result without any compile-time error. If we did not perform explicit type-casting then we will get compile time error. But in the case of compound assignment operators internally type-casting will be performed automatically, even we are assigning a bigger value to a smaller data-type variable but there may be a chance of loss of data information. The programmer will not responsible to perform explicit type-casting. Let’s see the below example to find the difference between normal assignment operator and compound assignment operator. A compound assignment expression of the form E1 op= E2 is equivalent to E1 = (T) ((E1) op (E2)), where T is the type of E1, except that E1 is evaluated only once.
For example, the following code is correct:
and results in x having the value 7 because it is equivalent to:
Because here 6.6 which is double is automatically converted to short type without explicit type-casting.
Refer: When is the Type-conversion required?
Explanation: In the above example, we are using normal assignment operator. Here we are assigning an int (b+1=20) value to byte variable (i.e. b) that’s results in compile time error. Here we have to do type-casting to get the result.
Explanation: In the above example, we are using compound assignment operator. Here we are assigning an int (b+1=20) value to byte variable (i.e. b) apart from that we get the result as 20 because In compound assignment operator type-casting is automatically done by compile. Here we don’t have to do type-casting to get the result.
Reference: http://docs.oracle.com/javase/specs/jls/se7/html/jls-15.html#jls-15.26.2
Please Login to comment...
Similar reads.
- Java-Operators
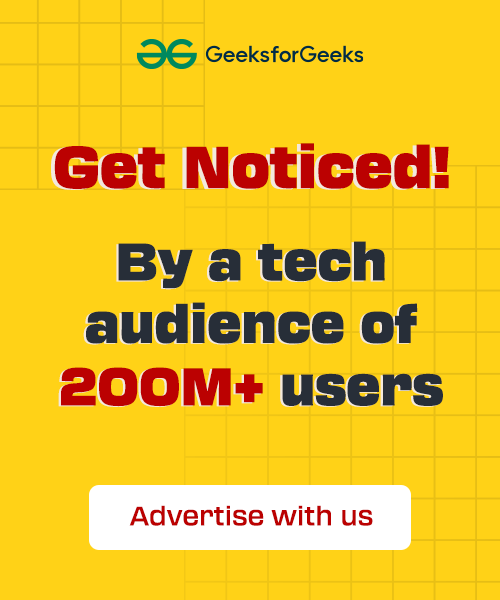
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
cppreference.com
Assignment operators.
Assignment and compound assignment operators are binary operators that modify the variable to their left using the value to their right.
[ edit ] Simple assignment
The simple assignment operator expressions have the form
Assignment performs implicit conversion from the value of rhs to the type of lhs and then replaces the value in the object designated by lhs with the converted value of rhs .
Assignment also returns the same value as what was stored in lhs (so that expressions such as a = b = c are possible). The value category of the assignment operator is non-lvalue (so that expressions such as ( a = b ) = c are invalid).
rhs and lhs must satisfy one of the following:
- both lhs and rhs have compatible struct or union type, or..
- rhs must be implicitly convertible to lhs , which implies
- both lhs and rhs have arithmetic types , in which case lhs may be volatile -qualified or atomic (since C11)
- both lhs and rhs have pointer to compatible (ignoring qualifiers) types, or one of the pointers is a pointer to void, and the conversion would not add qualifiers to the pointed-to type. lhs may be volatile or restrict (since C99) -qualified or atomic (since C11) .
- lhs is a (possibly qualified or atomic (since C11) ) pointer and rhs is a null pointer constant such as NULL or a nullptr_t value (since C23)
[ edit ] Notes
If rhs and lhs overlap in memory (e.g. they are members of the same union), the behavior is undefined unless the overlap is exact and the types are compatible .
Although arrays are not assignable, an array wrapped in a struct is assignable to another object of the same (or compatible) struct type.
The side effect of updating lhs is sequenced after the value computations, but not the side effects of lhs and rhs themselves and the evaluations of the operands are, as usual, unsequenced relative to each other (so the expressions such as i = ++ i ; are undefined)
Assignment strips extra range and precision from floating-point expressions (see FLT_EVAL_METHOD ).
In C++, assignment operators are lvalue expressions, not so in C.
[ edit ] Compound assignment
The compound assignment operator expressions have the form
The expression lhs @= rhs is exactly the same as lhs = lhs @ ( rhs ) , except that lhs is evaluated only once.
[ edit ] References
- C17 standard (ISO/IEC 9899:2018):
- 6.5.16 Assignment operators (p: 72-73)
- C11 standard (ISO/IEC 9899:2011):
- 6.5.16 Assignment operators (p: 101-104)
- C99 standard (ISO/IEC 9899:1999):
- 6.5.16 Assignment operators (p: 91-93)
- C89/C90 standard (ISO/IEC 9899:1990):
- 3.3.16 Assignment operators
[ edit ] See Also
Operator precedence
[ edit ] See also
- Recent changes
- Offline version
- What links here
- Related changes
- Upload file
- Special pages
- Printable version
- Permanent link
- Page information
- In other languages
- This page was last modified on 19 August 2022, at 08:36.
- This page has been accessed 54,567 times.
- Privacy policy
- About cppreference.com
- Disclaimers

Inscreva-se
Game dev series vol.01, o primeiro livro didático sobre programação para jogos digitais..
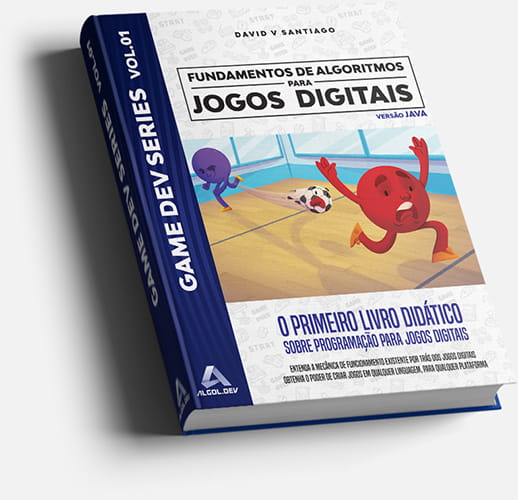
Comece com os conceitos básicos de programação.
Conheça o nosso curso gratuito para você que quer começar a aprender programação., conheça as nossas publicações na página de artigos..
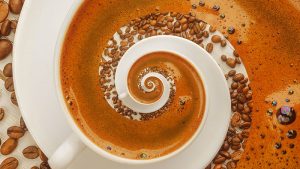
Termos de uso
Política de Privacidade

Este site usa cookies para garantir que você obtenha a melhor experiência.
Compound assignment operators
The compound assignment operators consist of a binary operator and the simple assignment operator. They perform the operation of the binary operator on both operands and store the result of that operation into the left operand, which must be a modifiable lvalue.
The following table shows the operand types of compound assignment expressions:
Note that the expression
is equivalent to
The following table lists the compound assignment operators and shows an expression using each operator:
Although the equivalent expression column shows the left operands (from the example column) twice, it is in effect evaluated only once.
This browser is no longer supported.
Upgrade to Microsoft Edge to take advantage of the latest features, security updates, and technical support.
Assignment operators
- 8 contributors
expression assignment-operator expression
assignment-operator : one of = *= /= %= += -= <<= >>= &= ^= |=
Assignment operators store a value in the object specified by the left operand. There are two kinds of assignment operations:
simple assignment , in which the value of the second operand is stored in the object specified by the first operand.
compound assignment , in which an arithmetic, shift, or bitwise operation is performed before storing the result.
All assignment operators in the following table except the = operator are compound assignment operators.
Assignment operators table
Operator keywords.
Three of the compound assignment operators have keyword equivalents. They are:
C++ specifies these operator keywords as alternative spellings for the compound assignment operators. In C, the alternative spellings are provided as macros in the <iso646.h> header. In C++, the alternative spellings are keywords; use of <iso646.h> or the C++ equivalent <ciso646> is deprecated. In Microsoft C++, the /permissive- or /Za compiler option is required to enable the alternative spelling.
Simple assignment
The simple assignment operator ( = ) causes the value of the second operand to be stored in the object specified by the first operand. If both objects are of arithmetic types, the right operand is converted to the type of the left, before storing the value.
Objects of const and volatile types can be assigned to l-values of types that are only volatile , or that aren't const or volatile .
Assignment to objects of class type ( struct , union , and class types) is performed by a function named operator= . The default behavior of this operator function is to perform a member-wise copy assignment of the object's non-static data members and direct base classes; however, this behavior can be modified using overloaded operators. For more information, see Operator overloading . Class types can also have copy assignment and move assignment operators. For more information, see Copy constructors and copy assignment operators and Move constructors and move assignment operators .
An object of any unambiguously derived class from a given base class can be assigned to an object of the base class. The reverse isn't true because there's an implicit conversion from derived class to base class, but not from base class to derived class. For example:
Assignments to reference types behave as if the assignment were being made to the object to which the reference points.
For class-type objects, assignment is different from initialization. To illustrate how different assignment and initialization can be, consider the code
The preceding code shows an initializer; it calls the constructor for UserType2 that takes an argument of type UserType1 . Given the code
the assignment statement
can have one of the following effects:
Call the function operator= for UserType2 , provided operator= is provided with a UserType1 argument.
Call the explicit conversion function UserType1::operator UserType2 , if such a function exists.
Call a constructor UserType2::UserType2 , provided such a constructor exists, that takes a UserType1 argument and copies the result.
Compound assignment
The compound assignment operators are shown in the Assignment operators table . These operators have the form e1 op = e2 , where e1 is a non- const modifiable l-value and e2 is:
an arithmetic type
a pointer, if op is + or -
a type for which there exists a matching operator *op*= overload for the type of e1
The built-in e1 op = e2 form behaves as e1 = e1 op e2 , but e1 is evaluated only once.
Compound assignment to an enumerated type generates an error message. If the left operand is of a pointer type, the right operand must be of a pointer type, or it must be a constant expression that evaluates to 0. When the left operand is of an integral type, the right operand must not be of a pointer type.
Result of built-in assignment operators
The built-in assignment operators return the value of the object specified by the left operand after the assignment (and the arithmetic/logical operation in the case of compound assignment operators). The resultant type is the type of the left operand. The result of an assignment expression is always an l-value. These operators have right-to-left associativity. The left operand must be a modifiable l-value.
In ANSI C, the result of an assignment expression isn't an l-value. That means the legal C++ expression (a += b) += c isn't allowed in C.
Expressions with binary operators C++ built-in operators, precedence, and associativity C assignment operators
Was this page helpful?
Coming soon: Throughout 2024 we will be phasing out GitHub Issues as the feedback mechanism for content and replacing it with a new feedback system. For more information see: https://aka.ms/ContentUserFeedback .
Submit and view feedback for
Additional resources
- Read Tutorial
- Watch Guide Video
- Complete the Exercise
Now that we've talked about operators. Let's talk about something called the compound assignment operator and I'm going make one little change here in case you're wondering if you ever want to have your console take up the entire window you come up to the top right-hand side here you can undock it into a separate window and you can see that it takes up the entire window.
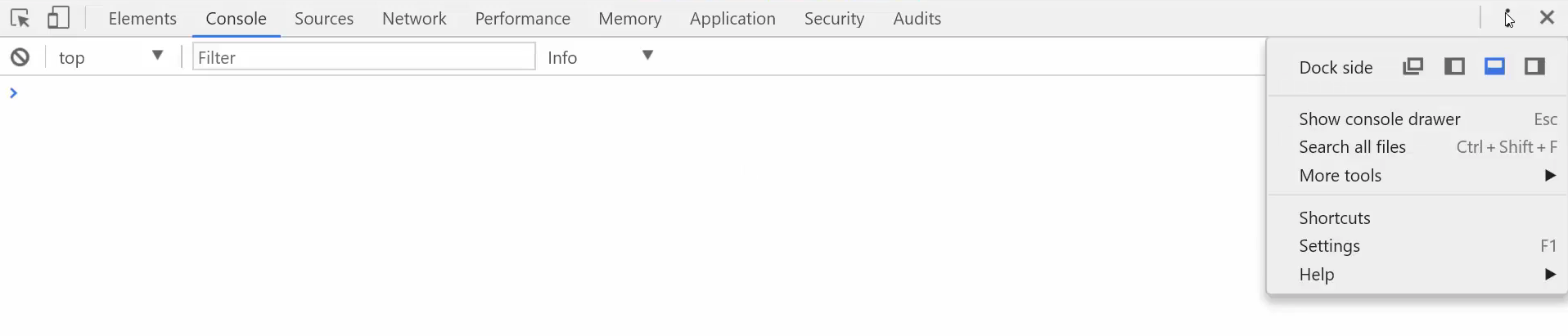
So just a little bit more room now.
Additionally, I have one other thing I'm going to show you in the show notes. I'm going to give you access to this entire set of assignment operators but we'll go through a few examples here. I'm going to use the entire window just to make it a little bit easier to see.
Let's talk about what assignment is. Now we've been using assignment ever since we started writing javascript code. You're probably pretty used to it. Assignment is saying something like var name and then setting up a name
And that is assignment the equals represents assignment.
Now javascript gives us the ability to have the regular assignment but also to have that assignment perform tasks. So for example say that you want to add items up so say that we want to add up a total set of grades to see the total number of scores. I can say var sum and assign it equal to zero.
And now let's create some grades.
I'm going to say var gradeOne = 100.
and then var gradeTwo = 80.
Now with both of these items in place say that we wanted to add these if you wanted to just add both of them together you definitely could do something like sum = (gradeOne + gradeTwo); and that would work.
However, one thing I want to show you is, there are many times where you don't have gradeOne or gradeTwo in a variable. You may have those stored in a database and then you're going to loop through that full set of records. And so you need to be able to add them on the fly. And so that's what a compound assignment operator can do.
Let's use one of the more basic ones which is to have the addition assignment.
Now you can see that sum is equal to 100.
Then if I do
If we had 100 grades we could simply add them just like that.
Essentially what this is equal to is it's a shorthand for saying something like
sum = sum + whatever the next one is say, that we had a gradeThree, it would be the same as doing that. So it's performing assignment, but it also is performing an operation. That's the reason why it's called a compound assignment operator.
Now in addition to having the ability to sum items up, you could also do the same thing with the other operators. In fact literally, every one of the operators that we just went through you can use those in order to do this compound assignment. Say that you wanted to do multiplication you could do sum astrix equals and then gradeTwo and now you can see it equals fourteen thousand four hundred.
This is that was the exact same as doing sum = whatever the value of sum was times gradeTwo. That gives you the exact same type of process so that is how you can use the compound assignment operators. And if you reference the guide that is included in the show notes. You can see that we have them for each one of these from regular equals all the way through using exponents.
Then for right now don't worry about the bottom items. These are getting into much more advanced kinds of fields like bitwise operators and right and left shift assignments. So everything you need to focus on is actually right at the top for how we're going to be doing this. This is something that you will see in a javascript code. I wanted to include it, so when you see it you're not curious about exactly what's happening.
It's a great shorthand syntax for whenever you want to do assignment but also perform an operation at the same time.
- Documentation for Compound Assignment Operators
- Source code
devCamp does not support ancient browsers. Install a modern version for best experience.

- Introduction to C
- Download MinGW GCC C Compiler
- Configure MinGW GCC C Compiler
- The First C Program
- Data Types in C
- Variables, Keywords, Constants
- If Statement
- If Else Statement
- Else If Statement
- Nested If Statement
- Nested If Else Statement
- Do-While Loop
- Break Statement
- Switch Statement
- Continue Statement
- Goto Statement
- Arithmetic Operator in C
- Increment Operator in C
- Decrement Operator in C
- Compound Assignment Operator
- Relational Operator in C
- Logical Operator in C
- Conditional Operator in C
- 2D array in C
- Functions with arguments
- Function Return Types
- Function Call by Value
- Function Call by Reference
- Recursion in C
- Reading String from console
- C strchr() function
- C strlen() function
- C strupr() function
- C strlwr() function
- C strcat() function
- C strncat() function
- C strcpy() function
- C strncpy() function
- C strcmp() function
- C strncmp() function
- Structure with array element
- Array of structures
- Formatted Console I/O functions
- scanf() and printf() function
- sscanf() and sprintf() function
- Unformatted Console I/O functions
- getch(), getche(), getchar(), gets()
- putch(), putchar(), puts()
- Reading a File
- Writing a File
- Append to a File
- Modify a File
Advertisement
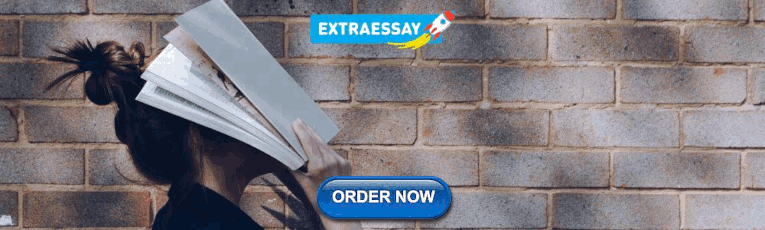
+= operator
- Add operation.
- Assignment of the result of add operation.
- Statement i+=2 is equal to i=i+2 , hence 2 will be added to the value of i, which gives us 4.
- Finally, the result of addition, 4 is assigned back to i, updating its original value from 2 to 4.
Example with += operator
-= operator.
- Subtraction operation.
- Assignment of the result of subtract operation.
- Statement i-=2 is equal to i=i-2 , hence 2 will be subtracted from the value of i, which gives us 0.
- Finally, the result of subtraction i.e. 0 is assigned back to i, updating its value to 0.
Example with -= operator
*= operator.
- Multiplication operation.
- Assignment of the result of multiplication operation.
- Statement i*=2 is equal to i=i*2 , hence 2 will be multiplied with the value of i, which gives us 4.
- Finally, the result of multiplication, 4 is assigned back to i, updating its value to 4.
Example with *= operator
/= operator.
- Division operation.
- Assignment of the result of division operation.
- Statement i/=2 is equal to i=i/2 , hence 4 will be divided by the value of i, which gives us 2.
- Finally, the result of division i.e. 2 is assigned back to i, updating its value from 4 to 2.
Example with /= operator
Please share this article -.

Please Subscribe

Notifications
Please check our latest addition C#, PYTHON and DJANGO

Java Tutorial
Control statements, java object class, java inheritance, java polymorphism, java abstraction, java encapsulation, java oops misc.
Using Compound Assignment Operator in a Java Program
CompoundAssignmentOperator.java

- Send your Feedback to [email protected]
Help Others, Please Share

Learn Latest Tutorials

Transact-SQL

Reinforcement Learning

R Programming

React Native

Python Design Patterns

Python Pillow

Python Turtle

Preparation

Verbal Ability

Interview Questions

Company Questions
Trending Technologies

Artificial Intelligence

Cloud Computing

Data Science

Machine Learning

B.Tech / MCA

Data Structures

Operating System

Computer Network

Compiler Design

Computer Organization

Discrete Mathematics

Ethical Hacking

Computer Graphics

Software Engineering

Web Technology

Cyber Security

C Programming

Control System

Data Mining

Data Warehouse


- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
Compound Assignment Operators in C++
The compound assignment operators are specified in the form e1 op= e2, where e1 is a modifiable l-value not of const type and e2 is one of the following −
- An arithmetic type
- A pointer, if op is + or –
The e1 op= e2 form behaves as e1 = e1 op e2, but e1 is evaluated only once.
The following are the compound assignment operators in C++ −
Let's have a look at an example using some of these operators −
This will give the output −
Note that Compound assignment to an enumerated type generates an error message. If the left operand is of a pointer type, the right operand must be of a pointer type or it must be a constant expression that evaluates to 0. If the left operand is of an integral type, the right operand must not be of a pointer type.

Related Articles
- Compound assignment operators in C#
- Compound assignment operators in Java\n
- Assignment Operators in C++
- What are assignment operators in C#?
- Perl Assignment Operators
- Assignment operators in Dart Programming
- What are Assignment Operators in JavaScript?
- Compound operators in Arduino
- What is the difference between = and: = assignment operators?
- Passing the Assignment in C++
- Airplane Seat Assignment Probability in C++
- What is an assignment operator in C#?
- Copy constructor vs assignment operator in C++
- Ternary Operators in C/C++
- Unary operators in C/C++
Kickstart Your Career
Get certified by completing the course
- Skip to main content
- Skip to search
- Skip to select language
- Sign up for free
- English (US)
Addition assignment (+=)
The addition assignment ( += ) operator performs addition (which is either numeric addition or string concatenation) on the two operands and assigns the result to the left operand.
Description
x += y is equivalent to x = x + y , except that the expression x is only evaluated once.
Using addition assignment
Specifications, browser compatibility.
BCD tables only load in the browser with JavaScript enabled. Enable JavaScript to view data.
- Assignment operators in the JS guide
- Addition ( + )
- Share full article
Advertisement
Supported by
Six Things to Know About ‘Forever Chemicals’
The federal government is ordering the removal of PFAS, a class of chemicals that poses serious health risks, from drinking water systems across the country.

By Lisa Friedman
Almost half the tap water in the United States contains PFAS, a class of chemicals linked to serious health problems. On Wednesday, the Environmental Protection Agency announced that, for the first time, municipal utilities will have to detect and remove PFAS from drinking water.
Here’s what you need to know.
What are PFAS?
In 1938 a young chemist working on refrigerants for Dupont accidentally discovered a new compound that was remarkably resistant to water and grease, a finding that would lead to the creation of the Teflon brand of nonstick cookware.
Today there are nearly 15,000 per- and polyfluoroalkyl substances, which collectively go by the acronym PFAS, according to a database maintained by the E.P.A.
The common link is that they have a special bond of carbon and fluorine atoms, making them incredibly strong and resistant to heat, water, oil and dirt. For that reason, PFAS is used for everyday items as varied as microwave popcorn bags, water-repellent clothing and stain-resistant carpets. PFAS are also in firefighting foam, cosmetics, shampoos, toys and even dental floss.
Where are PFAS?
Everywhere, including drinking water. The indestructible nature that makes PFAS useful in some products also makes them harmful to human health. The chemicals are virtually indestructible and do not fully degrade, accumulating in the environment and the human body.
The chemicals are so ubiquitous that they can be found in the blood of almost every person in the country. One recent government study detected PFAS chemicals in nearly half of the nation’s tap water . A global study of more than 45,000 water samples around the world found that about 31 percent of tested groundwater samples that weren’t near any obvious source of contamination had PFAS levels considered harmful to human health.
What does PFAS do to the body?
According to the E.P.A., exposure to PFAS can cause damage to the liver and immune system and also has been linked to low birth weight, birth defects and developmental delays as well as increased risk of some prostate, kidney and testicular cancers. New research published in the past year found links between PFAS exposure and a delay in the onset of puberty in girls, leading to a higher incidence of breast cancer, renal disease and thyroid disease; a decrease in bone density in teenagers, potentially leading to osteoporosis; and an increased risk of Type 2 diabetes in women.
Why didn’t the E.P.A. regulate PFAS in water sooner?
Many environmental advocates argue that PFAS contamination should have been dealt with long ago.
“For generations, PFAS chemicals slid off every federal environmental law like a fried egg off a Teflon pan,” said Ken Cook, president and co-founder of the Environmental Working Group, a nonprofit advocacy group.
Activists blame chemical companies, which for decades hid evidence of the dangers of PFAS, according to lawsuits and a peer-reviewed study , published in the Annals of Global Health, of previously secret industry documents.
The new E.P.A. rule requires utilities to reduce PFAS in drinking water to near-zero levels.
How can I get rid of PFAS?
Not easily. In homes, filters attached to faucets or in pitchers generally do not remove PFAS substances. Under-sink reverse-osmosis systems have been shown to remove most but not all PFAS in studies performed by scientists at Duke University and North Carolina State University.
Municipal water systems can install one of several technologies including carbon filtration or a reverse-osmosis water filtration system that can reduce levels of the chemicals.
Now that limits have been set, when will PFAS disappear from tap water?
It could take years. Under the rule, a water system has three years to monitor and report its PFAS levels. Then, if the levels exceed the E.P.A.’s new standard, the utility will have another two years to purchase and install filtration technology.
But trade groups and local governments are expected to mount legal challenges against the regulation, potentially delaying it even before a court makes a final ruling. And if former President Donald J. Trump were to retake the White House in November, his administration could also reverse or weaken the rule.
An earlier version of this article described incorrectly the molecular structure of PFAS compounds. They have carbon and fluorine atoms, not carbon and fluoride.
How we handle corrections
Lisa Friedman is a Times reporter who writes about how governments are addressing climate change and the effects of those policies on communities. More about Lisa Friedman
The Proliferation of ‘Forever Chemicals’
Pfas, or per- and polyfluoroalkyl substances, are hazardous compounds that pose a global threat to human health..
For the first time, the U.S. government is requiring municipal water systems to detect and remove PFAS from drinking water .
A global study found harmful levels of PFAS in water samples taken far from any obvious source of contamination.
Virtually indestructible, PFAS are used in fast-food packaging and countless household items .
PFAS lurk in much of what we eat, drink and use, but scientists are only beginning to understand how they affect our health .
Though no one can avoid forever chemicals entirely, Wirecutter offers tips on how to limit your exposure .
Scientists have spent years searching for ways to destroy forever chemicals. In 2022, a team of chemists found a cheap, effective method to break them down .

The Assignment with Audie Cornish
Each week on the assignment, host audie cornish pulls listeners out of their digital echo chambers to hear from the people whose lives intersect with the news cycle. from the sex work economy to the battle over what’s taught in classrooms, no topic is off the table. listen to the assignment every monday and thursday..
- Apple Podcasts

Back to episodes list
While the political world fixates on Donald Trump’s Manhattan criminal trial, the Supreme Court is weighing two decisions that could re-define the November election and invalidate charges for hundreds of January 6th defendants. CNN’s Senior Supreme Court Analyst Joan Biskupic is here to explain what’s at stake and read the tea leaves as to which way the justices are leaning. Joan’s book, Nine Black Robes , is now out in paperback.
The Federal Register
The daily journal of the united states government, request access.
Due to aggressive automated scraping of FederalRegister.gov and eCFR.gov, programmatic access to these sites is limited to access to our extensive developer APIs.
If you are human user receiving this message, we can add your IP address to a set of IPs that can access FederalRegister.gov & eCFR.gov; complete the CAPTCHA (bot test) below and click "Request Access". This process will be necessary for each IP address you wish to access the site from, requests are valid for approximately one quarter (three months) after which the process may need to be repeated.
An official website of the United States government.
If you want to request a wider IP range, first request access for your current IP, and then use the "Site Feedback" button found in the lower left-hand side to make the request.
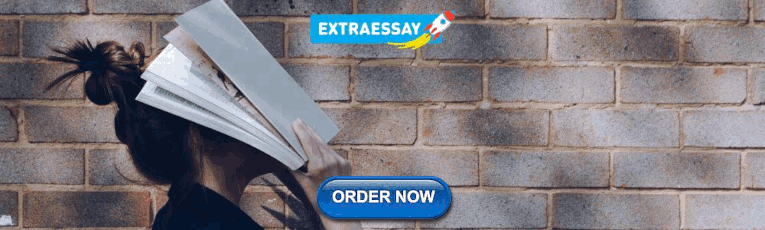
COMMENTS
Compound-Assignment Operators. Compound-assignment operators provide a shorter syntax for assigning the result of an arithmetic or bitwise operator. They perform the operation on the two operands before assigning the result to the first operand.
The result of a compound-assignment operation has the value and type of the left operand. #define MASK 0xff00 n &= MASK; In this example, a bitwise-inclusive-AND operation is performed on n and MASK, and the result is assigned to n. The manifest constant MASK is defined with a #define preprocessor directive. See also. C Assignment Operators
Compound assignment operators modify the current value of a variable by performing an operation on it. They are equivalent to assigning the result of an operation to the first operand: ... Although in simple expressions like x++ or ++x, both have exactly the same meaning; in other expressions in which the result of the increment or decrement ...
for assignments to class type objects, the right operand could be an initializer list only when the assignment is defined by a user-defined assignment operator. removed user-defined assignment constraint. CWG 1538. C++11. E1 ={E2} was equivalent to E1 = T(E2) ( T is the type of E1 ), this introduced a C-style cast. it is equivalent to E1 = T{E2}
Compound-assignment operators provide a shorter syntax for assigning the result of an arithmetic or bitwise operator. They perform the operation on the two operands before assigning the result to the first operand. The following are all possible assignment operator in java: 1.
Assignment performs implicit conversion from the value of rhs to the type of lhs and then replaces the value in the object designated by lhs with the converted value of rhs . Assignment also returns the same value as what was stored in lhs (so that expressions such as a = b = c are possible). The value category of the assignment operator is non ...
The ++ (increment) and −− (decrement) compound assignment operators are treated in a particular way. In fact, they are even simpler to understand. The ++ (increment) operator only adds one unit to the value of the numeric variable.. For example: the expression "x = x + 1" could be rewritten as "x++". The −− (decrement) operator only subtracts one unit from the value of the ...
The compound operators are different in two ways, which we see by looking more precisely at their definition. The Java language specification says that: The compound assignment E1 op= E2 is equivalent to [i.e. is syntactic sugar for] E1 = (T) ((E1) op (E2)) where T is the type of E1, except that E1 is evaluated only once.
The compound assignment operators consist of a binary operator and the simple assignment operator. They perform the operation of the binary operator on both operands and store the result of that operation into the left operand, which must be a modifiable lvalue. The following table shows the operand types of compound assignment expressions:
Compound assignment. The compound assignment operators are shown in the Assignment operators table. These operators have the form e1 op= e2, where e1 is a non-const modifiable l-value and e2 is: an arithmetic type. a pointer, if op is + or -a type for which there exists a matching operator *op*= overload for the type of e1
According to Microsoft, "However, the compound-assignment expression is not equivalent to the expanded version because the compound-assignment expression evaluates expression1 only once, while the expanded version evaluates expression1 twice: in the addition operation and in the assignment operation". Here is what I am expecting some kind of ...
Compound assignment operators in Java are shorthand notations that combine an arithmetic or bitwise operation with an assignment. They allow you to perform an operation on a variable's value and then assign the result back to the same variable in a single step.
Compound Assignment Operators. An assignment operator is a binary operator that assigns the result of the right-hand side to the variable on the left-hand side. The simplest is the "=" assignment operator: int x = 5; This statement declares a new variable x, assigns x the value of 5 and returns 5. Compound Assignment Operators are a shorter ...
An assignment operator assigns a value to its left operand based on the value of its right operand. The simple assignment operator is equal (=), which assigns the value of its right operand to its left operand.That is, x = f() is an assignment expression that assigns the value of f() to x. There are also compound assignment operators that are shorthand for the operations listed in the ...
And so you need to be able to add them on the fly. And so that's what a compound assignment operator can do. Let's use one of the more basic ones which is to have the addition assignment. sum += gradeOne; // 100. Now you can see that sum is equal to 100. Then if I do. sum += gradeTwo; // 180.
A special case scenario for all the compound assigned operators. int i= 2 ; i+= 2 * 2 ; //equals to, i = i+(2*2); In all the compound assignment operators, the expression on the right side of = is always calculated first and then the compound assignment operator will start its functioning. Hence in the last code, statement i+=2*2; is equal to i ...
The compound assignment operator is the combination of more than one operator. It includes an assignment operator and arithmetic operator or bitwise operator. The specified operation is performed between the right operand and the left operand and the resultant assigned to the left operand. Generally, these operators are used to assign results ...
The compound assignment operators are specified in the form e1 op= e2, where e1 is a modifiable l-value not of const type and e2 is one of the following −. The e1 op= e2 form behaves as e1 = e1 op e2, but e1 is evaluated only once. The following are the compound assignment operators in C++ −. Multiply the value of the first operand by the ...
The addition assignment (+=) operator performs addition (which is either numeric addition or string concatenation) on the two operands and assigns the result to the left operand. ... TypeError: can't define property "x": "obj" is not extensible; TypeError: can't delete non-configurable array element; TypeError: can't redefine non-configurable ...
The federal government is ordering the removal of PFAS, a class of chemicals that poses serious health risks, from drinking water systems across the country.
The wave of drones and missiles that flew towards Israel overnight on Sunday brought with it a new phase of tension, uncertainty and confrontation in the Middle East.
6. The compound assignment operators are in the second lowest precedence group of all in C++ (taking priority over only the comma operator). Thus, your a += b % c case would be equivalent to a += ( b % c ), or a = a + ( b % c ). This explains why your two code snippets are different. The second:
The Assignment with Audie Cornish Each week on The Assignment, host Audie Cornish pulls listeners out of their digital echo chambers to hear from the people whose lives intersect with the news cycle.
Hello, Characteristic and Class Assignment tabs are missing from Basic Data Details in MDG-M UI.In order for them to be displayed, the users have to press on the Settings -> Reset to Default every time it is needed to maintain Class or Characteristics values from the mentioned UIBBs.. After this actions is done, both UIBBs are displayed at that time, but when a new session is opened the ...
2,991 3 21 31. From the definition of compound: "a thing that is composed of two or more separate elements; a mixture." From that, I would think that let n = 1 + someval is a compound assignment and let n = 1 is not? Couldn't say with absolute confidence though...
The definition is also revised to provide additional context on construction actions. Wetlands: The final rule revises the definition for "wetlands" by removing the part of the definition that described how wetlands are determined and moves that description to a new § 55.9, "Identifying wetlands." The final rule also removes the non ...