How to Learn the Fundamentals of Software Engineering – in a More Interesting and Less Painful Way
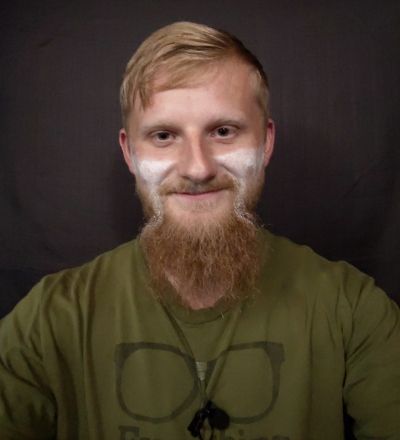
This article is intended to be an introductory guide to the fundamentals of software engineering.
I have written it with the assumption that you, dear reader, may not know much about the basics of the field, why they are important, and when you should bother to learn them.
We shall go into each of these questions and finish by discussing some ways in which I recommend you learn and approach them.
For those who do happen to be familiar with this subject, there may still be some interesting new perspectives, and particularly in the last section, useful ways to speed up your learning process.
In this article we will discuss:
- What made software engineering spooky and intimidating for me, and how that changed
- The reason for, and metrics by which we look at some code and conclude that it is less efficient than another approach (computational complexity)
- A simple but hopefully useful introduction to Data Structures and Algorithms
- The things I personally do to learn topics in software engineering for maximum efficiency and understanding
- A way to motivate your efforts by adding in some basic tests to measure correctness and efficiency of your algorithms
Please be aware that I have tried to structure this article in a logical progression where each section (apart from the next which is more about getting over the fear of diving into this subject) builds upon or motivates the next.
I am condensing over a thousand hours of practice and study into one article, and have done my best to explain things clearly and simply as well.
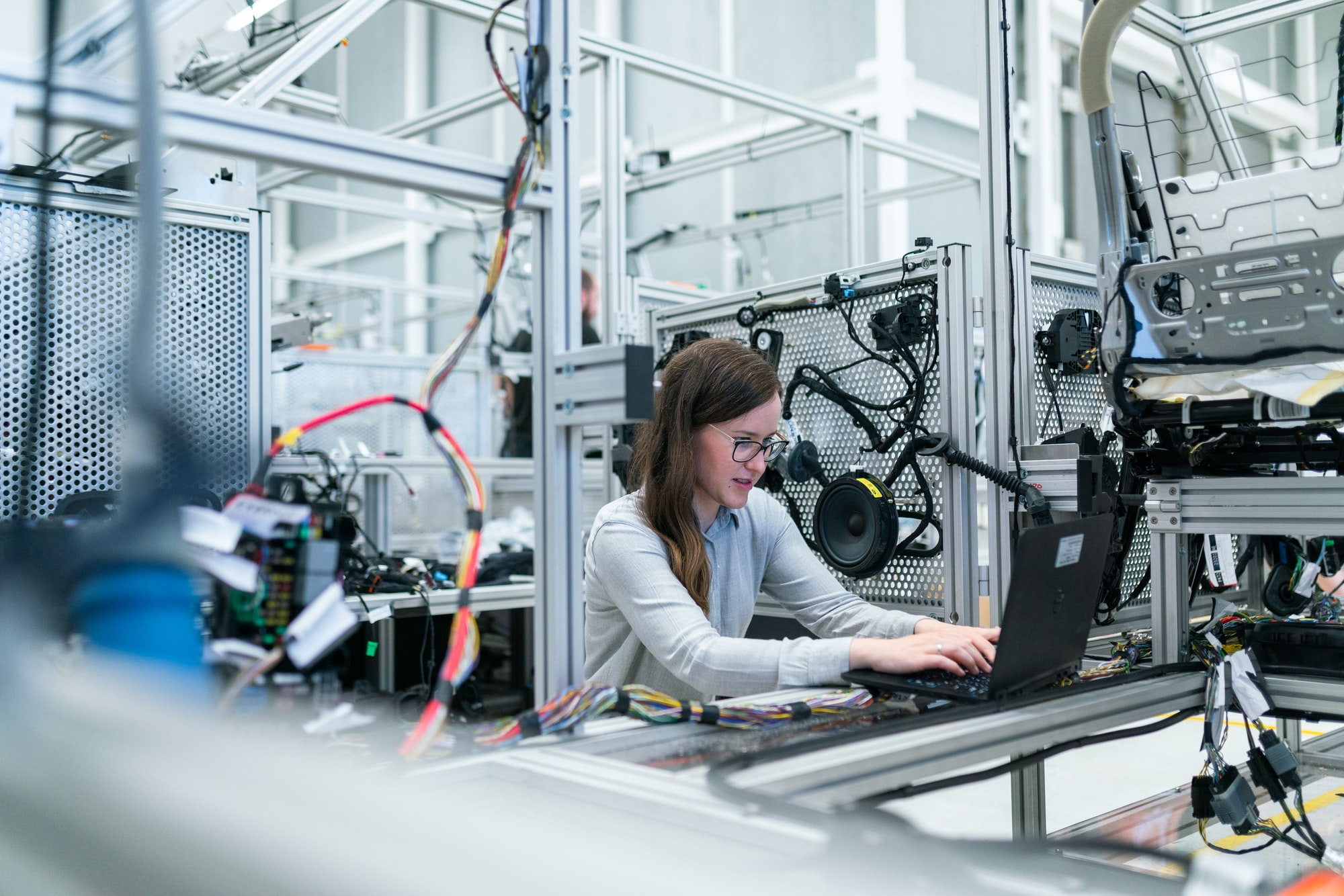
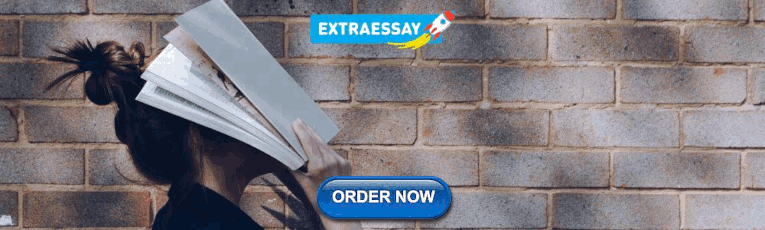
Where The Problems Began
Throughout my limited formal education, I did not have the best relationship with the field of mathematics. And by extension, this impacted my relationship with a great deal of computer science (CS) and software engineering (SEng).
To be specific, it is not that I am bad at math, but I am poor at arithmetic and bad at remembering formulae.
I also found that the way in which mathematics, CS, and SEng are usually taught in schools does not tend to work for me either.
My own learning process to this day is largely driven by pragmatism (emphasis on practical over theoretical knowledge), curiosity about nature, and how this information can help me earn a living – three things I saw rarely emphasized in my western education.
Apart from my uneasy relationship with dry and boring presentations of things which are predominantly of a mathematical nature, I am a self-taught programmer.
To be clear, I took a single programming class at a community college (circa 2013), and the rest of my knowledge comes from self-directed studies. During my early years of that process, I also had to work various day jobs in order to pay the rent, which left me very little spare time and energy to learn my craft.
The end result was that I chose to spend most of my time building personal projects and learning topics specifically for those projects.
This led to me being really quite good at the skill of writing code, learning new technologies, and solving problems. However, if I did apply any concepts in CS and SEng to the code I was writing, it was largely by accident. To summarize this introduction, I am trying to say that the biggest obstacle in my study of SEng was that I just was not very interested in learning it.
I did not know the sense of accomplishment you can get from making a small change to an algorithm which reduces its runtime to completion by a factor of tens or hundreds of times.
I did not know how important it was to pick a data structure based on the nature of the problem I was trying to solve, let alone how to make that decision.
And I had no idea how it was relevant to me slinging mobile apps for a living.
So just in case you are in the same boat, before we get to the technical details, I would like to attempt to answer some of those questions for you. To make things less boring, I will motivate this topic by sharing a true story about what changed my attitude towards this subject.
Swimming With the Big Fish
Towards the end of 2019, I had taken a break from studying Android Development in order to take a deep dive into UNIX Operating Systems and C/C++ programming.
I felt very comfortable with the Android SDK, but many years of JVM programming had left me with a strong sense of having no idea how computers actually worked under the hood. Which bothered me quite a bit.
I was not really looking for work, but at the time a recruiter from a big tech company had reached out to me about my interest in an Android Software Engineer position.
Despite being many months out of practice with Android, I did well in the first interview (it was all core Android concepts that I was familiar with) and was sent an email detailing the topics to be covered in future interviews.
The first section of this email, which detailed Android specific knowledge, was extensive, but I was at least somewhat familiar which most topics and not intimidated. However, when I scrolled down to the section on Data Structures and Algorithms, I suddenly felt like I did when I first started writing code: Like a fish out of water. It is not that I had never applied any of these concepts in my code, but I certainly had not formally studied any of them.
Although I will do my best to give you a soft and clear introduction to these topics, SEng will immediately hit you with a wall of jargon terms, and my face was figuratively very sore and bruised after reading the entire list of DS and Algos I had to learn in that email.
I was very up front about that with my recruiter, who kindly gave me four weeks to prepare before the next interview.
I knew I could not cover every topic in four weeks, but I did hope that learning a year or two of SEng in a few weeks would show some talent and initiative.
I would love to tell you a juicy story about how I epically failed, or completely dazzled in the next interview, but the reality is that things fell apart before I even got the chance.
I am a Canadian citizen, and the position required relocation to one of many campuses in the United States, in either California or Washington State.
Two weeks into my first deep dive into SEng, I received an email from my recruiter stating that their immigration department did not want to sponsor me. I suspect it had to do with some difficulties around sponsoring a worker who had no degree, but the brewing global pandemic may also have been a factor.
In the end, even though I wanted the chance to succeed or fail in real time, I was happy knowing that I had a very clear idea of the knowledge I was lacking to be a software engineer in a big tech company.
With a clear but difficult path in front of me, I resolved to no longer let the field of SEng intimidate me. I wanted to know what it truly means to be a software developer versus a software engineer.
With that in mind, we shall go into the core ideas in SEng, and how to make the learning of them easier. Not easy – just easier.
The "Big Three" Topics in Software Engineering –And Why They Matter
The main topics in software engineering can be summarized using a bunch of big scary words and phrases – as is the tradition in anything related to computer science and mathematics. To avoid confusion, I will instead explain them using the English language and examples which prioritize clarity above all else.
I suggest you follow this section in the order I have laid out, as I have deliberately structured it in a logical progression.
First - What Are Runtime And Memory Space?
I want to start off by explaining the reason why we study these topics to begin with.
Being a fan of physics, I was happy to learn that the interplay of time and space which we see in nature is also directly observed in any kind of computer.
However, in this field, we refer to these qualities as runtime and memory space .
To better understand what runtime is, I suggest you pull up your Task Manager, Activity Monitor, or whatever program you have that tells you about your system’s active “processes.”
A process is just a “running program,” and through the magic of having multiple “processors”, CPU virtualization, and time slicing, it can appear that we have tens or hundreds of processes running at the same time.
I threw those jargon terms in so that you can look them up if you are curious about how operating systems work, but doing so it is not necessary to proceed with this article. In any case, a process’s runtime can generally be thought of as any point in time during which it can be viewed in your system’s process tracking tool.
I use this definition to point out that an active process does not need to have a user interface or even do anything useful even though it may still be taking up runtime in the CPU and memory space.
Speaking of memory space, in order for something to have run time, it has to be somewhere too. That somewhere is the physical memory space of the computer, which is virtualized (again, look up virtualization on your own time but it is not necessary for this article) in order to make it more secure and easier to use.
Every process is allocated its own distinct and protected virtual memory space, which can grow or shrink up to certain boundaries and depending on various factors. Let us take a break from theory to talk about why we should care about it. Since runtime and memory space can be accurately measured but are also limited , humans like you and I can really screw things up if we do not pay attention to these limitations!
To be clear, here are two very important things we want to care about as programmers and engineers:
- Will our program or even the whole system crash because we have mismanaged the finite resource of memory space ?
- Will our programs solve problems for our users in a timely and efficient manner , or will they hang so long that our users decide to force quit, demand a refund, and leave a nasty review?
These question largely dictate the success of our programs whether you formally study them or not. With any luck I have motivated you to learn what I call the big three topics in software engineering, which we shall go into now.
How We Measure Runtime And Memory Space
The first of the big three topics is described using a big scary term: Asymptotic Runtime & Space Complexity .
Having already described runtime and memory space, I think a serviceable replacement for the word complexity here is “efficiency." And asymptotic is related to the fact that we can represent this efficiency (or lack thereof) on a two dimensional Cartesian Graph. You know, x and y , rise over run, and all that stuff.
Do not worry if you are unfamiliar with this stuff. You only need a very basic understanding of these things to apply it in your code.
Also, note that there is such a thing as a Graph data structure , but that concept is far removed from a Cartesian Graph and not what I am referring to.
Since we can represent our code and how it behaves with respect to either runtime or memory space on a Cartesian Graph, it follows that there must be functions which describe how to draw such a graph .
The way in which we describe how efficient our code in this way is to use “Big O” notation.
Here is the simplest introduction I can give you in order to understand this topic. I will use the modern programming language Kotlin for my code samples which will hopefully provide a happy middle ground for you web and native developers.
Suppose three functions (also sometimes known as methods, algorithms, commands, or procedures):
Function printStatement :
Function printArray :
Function printArraySums :
For ease of understanding, suppose that every time println(...) is called, it takes 100ms, or 1/10th of a second on average to complete (in reality 100ms for a single print command is terribly slow but it's easier to imagine than a microsecond or picosecond).
With that in mind, let us think critically about how these functions can be expected to behave differently , based on what inputs they are given .
printStatement , barring anything other than a catastrophic failure of the system itself, will always take an average time of 100ms to complete.
In fact, while Big O notation is very concerned about the size of the arguments given to a function (that will make more sense shortly), this function does not even have any arguments to change its behaviour.
Therefore, we can say that the runtime complexity (time taken until completion), is constant , which can be represented by the following mathematical function and graph:

In the above graphic, T represents the runtime for println(...) which we established to be an average of 100 milliseconds. I will explain what n refers to momentarily.
printArray presents a new problem. It stands to reason that the time it takes for printArray to complete will be directly proportionate to the size of the Array, arr , which is passed into it.
If the Array has four elements, that would result in println(...) being called four times, for a total average runtime of 400ms for printArray itself. To be more mathematically precise, we would say that the runtime complexity of printArray is linear :
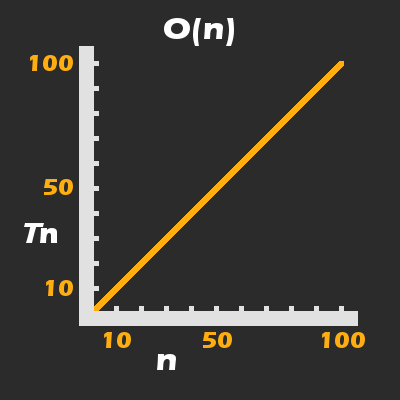
printArraySums takes things a step further into something which you should be concerned about even as a junior or intermediate level developer. The number of arguments/inputs to any given function is referred to with a small n, when using Big O notation.
In our second function, this refers exclusively to the size of the Array (that is, arr.size ), but in the third function it refers to the collective size of multiple arguments (that is, arrOne and arrTwo ).
In Big O notation, there are actually three different qualities of a given piece of code that we can pay attention to:
- How efficient is the code if n is small (best-case performance)?
- How efficient is the code if n is of an average expected size (average performance)?
- How efficient is the code if n is near or at the its maximum allowable value for the system (worst-case performance)?
Generally speaking, in the same sense that a civil engineer is most concerned about the maximum number of vehicles a bridge can support, a software engineer is usually most concerned about worst-case performance.
By looking at printArraySums , you should be able to reason that we can represent its worst-case runtime complexity (the number of times println(...) will be called) as n * n; where n is at or near to the maximum allowable size of an Array in the system.
In case it is not clear, we are not just pairing and summing the elements of arrOne and arrTwo at the same indexes, we are literally summing every value of them together in a nested loop .
From here you can start to truly understand the importance of asymptotic runtime and space complexity. In a worst-case scenario, the runtime grows exponentially in a quadratic curve:

Two final notes on this topic: Firstly, if I suddenly made you a bit fearful of nested loops (and yes, each nested loop potentially adds another factor of n ), then I have done a good job.
Even so , understand that if you are certain that n will not exceed a reasonable size even in a function which has exponential growth, then there is not actually a problem .
If you would like to know how to determine if n will have a negative impact on performance, stick around for the last section of this article.
Secondly, you may have noticed that all of my examples were about runtime complexity, not memory space complexity.
The reason for this is simple: We represent space complexity in exactly the same manner and notation. Since we do not actually allocate any new memory apart from a temporary reference or two within each frame of the forEach{...} loops, asymptotically speaking , the second and third functions are still linear, O( n ), with respect to memory allocation.
Data Structures
The term Data Structure, despite what any single teacher may tell you, does not have a singular definition.
Some teachers will emphasize their abstract nature and how we can represent them in mathematics, some teachers will emphasize how they are physically arranged in memory space, and some will emphasize how they are implemented in a particular language specification.
I hate to tell you this, but this is actually a very common problem in computer science and engineering: One word meaning many things and many words meaning one thing, all at the same time.
Therefore, rather than trying to make every kind of expert from every kind of academic or professional background happy by using a plethora of technical definitions, let me upset everyone equally by explaining things as clearly as I can in plain English .
For the purposes of this article, Data Structures (DS) refer to the ways in which we represent and group together our application’s data in our programs. Things like user profiles, friends lists, social networks, game states, high scores and so on.
When considering DS from the physical perspective of the hardware and operating system, there are two main ways to build a DS. Both ways take advantage of the fact that physical memory is discrete (a fancy word for countable), and therefore addressable.
An easy way to imagine this is to think about street addresses and how, depending on which direction you are physically moving (and depending on how your country organizes street addresses), the address increases or decreases in value.
Physical Array
The first way takes advantage of the fact that we can group pieces of data (for example a list of friends in a social media application) into a chunk of contiguous (physically next to each other) memory space.
This turns out to be a very fast and efficient way for a computer to traverse memory space. Instead of giving the computer an n sized list of addresses for each piece of data, we give the computer a single address denoting the start of this DS in physical memory, and the size of (that is, n ) the DS as a single value.
The instruction set for doing this could be as simple as telling the machine to move from left to right (or whatever direction), decrement the value of n by 1 each move, and to stop/return when that value hits 0.
Lists Of Links (Addresses)
The second way requires each piece of data in the structure itself to contain the address(es) of the next or previous (maybe both?) item within itself.
One of the big problems with contiguous memory spaces is that they present problems when it comes to growing (adding more elements) or shrinking (this can fragment the memory space, which I will not explain but suggest a quick google search).
By having each piece of data Link to the other pieces (usually just the previous or next one), it becomes largely irrelevant where each piece sits in physical memory space. Therefore, we can grow or shrink the data structure with relative ease. You should be able to reason that since each part of the structure stores not just its own data, but the address of the next (or more than that) element, then each piece would necessarily require more memory space than with the contiguous Array approach.
However, whether it is ultimately more efficient depends on what kind of problem you are trying to solve.
The two approaches I have discussed are generally known as an Array and a Linked List . With very little exception, most of what we care about in the study of DS is how to group collections of data which have some kind of reason to be grouped together, and how best to do that.
As I tried to point out, what makes one structure better in a certain situation can make it worse in another.
You should be able to reason from the previous few paragraphs that a Linked List is typically more suitable for a dynamic (changing) collection, whereas an Array is typically more suitable for a fixed collection – at least with respect to runtime and space efficiency .
Do not be misled, however! It is not always the case that our primary concern is to pick the most efficient DS (or algorithm) with respect to runtime and memory space. Remember, if n is very small then worrying about a nanosecond or a few bits of memory here and there are not necessarily as important as ease of use and legibility.
The last thing I would like to say about DS is that I have observed a profound lack of consensus about the difference between a DS and a Data Type (DT).
Again, I think this is largely due to different experts approaching this from different backgrounds (mathematics, digital circuits, low level programming, high level programming) and the fact that it is really quite hard to make a verbal definition of one that does not at least partially (or entirely) describe the other.
At the risk of making the situation even more confusing, on a purely practical level , I think of data structures as things which are independent of a high level programming language’s Type System (assuming it has one). On the other hand, a data type is defined by and within such a Type system.
But I know that Type Theory itself is independent of any particular Type system, so you can hopefully see how tricky it is to say anything concrete about these two terms.
I took quite a long time to explain the previous two topics because they allow me to introduce and motivate this topic rather easily.
Before we continue, I must very briefly try to untangle another mess of jargon. To explain the term “algorithm” in my own way, it is actually very simple: An algorithm is a set of instructions (commands) which can be understood and executed (acted upon) by an Information Processing System (IPS).
For example, if you were to follow a recipe to cook something, then you would be the IPS, the algorithm would be the recipe, and the ingredients and cookware would be the data inputs (arguments).
Now, by that definition the words function, method, procedure, operation, program, script, subroutine and algorithm all point to the same underlying concept.
This is not by accident – these words all fundamentally mean the same thing. The confusion is that different computer scientists and language designers will implement (build) the same idea in a slightly different way. Or even more depressingly, they will build them the same way but give a different name. I wish this was not the case, but the best I can do is to warn you.
That is all you need to know about algorithms in general, so let us be more specific about how they can help us to write better code.
Recall that our primary concern as software engineers is to write code which is guaranteed to be efficient (at least such that it keeps our users happy) and safe with respect to limited system resources.
Also recall that I previously stated that some DS perform better than others with respect to runtime and memory space, particularly as n gets large.
The same is true of algorithms. Depending on what you are trying to do, different algorithms will perform better than others.
It is also worth noting that the DS will tend to shape which algorithms can be applied to the problem, so selecting the right DS and the right algorithm is the true art of software engineering.
To finish off this third main topic, we will look at two common but very different ways to solve the one problem: Searching an ordered Array . By ordered, I mean to say that it is ordered something like least to greatest, greatest to least, or even alphabetically.
Also, assume that the algorithm is given some kind of target value as an argument, which is what we use to locate a particular element. This should become clear in the example in case there is any confusion.
The example problem is as follows: We have a collection of Users (perhaps loaded from a database or server), which is sorted from least to greatest by a field called userId, which is an Integer value.
Suppose that this userId comes from taking the system time (look up Unix Time for more info) just prior to creating the new User. Rounded to the smallest value that still guarantees no repeated values.
If that previous sentence did not make sense, all you need to know is that this is a sorted collection with no repeats.
A simple way to write this algorithm would be to write what we will call a Naive Search (NS). Naive, in this context, means simple, but in a bad way , which refers to the fact that we just tell the computer to start from one end of the collection and move to the other until it finds a match to the target index.
This is generally achieved by using some kind of loop:
Function naiveSearch :
If we happen to only have a few hundred, or even a few thousand users in this collection, then we can expect this function to return quite quickly all the same.
But let us assume we are working in a successful social media tech start up, and we have just hit one million users.
You should be able to reason that naiveSearch has O( n ) asymptotic complexity as its worst case runtime complexity. The reason in short is that if the target User happens to be located at n , then we must invariably traverse the entire collection to get there.
If you are not already familiar with the Binary Search (BS) algorithm, then you should prepare to have your mind blown.
What if I told you that by using a BS algorithm to search our collection with one million elements, you will only ever make, at most, 20 comparisons? That is right; 20 comparisons (as opposed to 1 million with NS) is the worst case scenario .
Now, I will explain how BS works in principle, but my one piece of homework for you to do is to implement it in your preferred programming language. It may be that the language you choose already has a BS implementation from its standard library, but this is an important learning exercise!
In principle, rather than searching an ordered collection one by one, unidirectionally, we start by looking at the value at index n /2. So in a collection with 10 elements, we would check the fifth element. The ordering is important, because we can then compare the element at n /2 with our target:
- If the value of that element is greater than the target, we know that the element we want must be located earlier in the collection
- If the value of that element is less than the target, then we know that the element we want must be located further ahead in the collection
- You should be able to guess what happens if we have a match
Now, the idea is that we are cutting the dataset in half every iteration. Suppose the value at element n /2 was less than our target value. We would next select the middle index between n /2 and n .
From there, our algorithm keeps slicing back or forth using the same logic over a smaller and smaller range of indexes in our collection.
This brings us to the beauty of the BS algorithm applied to a sorted collection: Rather than the time it takes to complete growing linearly, or exponentially with respect to n , it grows logarithmically:
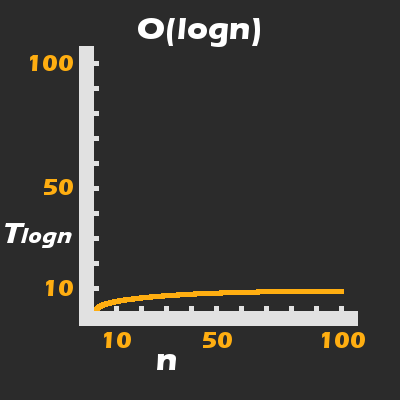
If this article really is your first introduction to the main ideas in software engineering, then please do not expect everything to make sense immediately.
While I hope that some of my explanations helped, my primary goal was to give you a basic list of ideas to study yourself, and what I believe to be a good order in which to study those ideas.
The next step for you is to make a plan for learning this field, and to take action on it. The following section is all about how to do that.
How to Learn Software Engineering – Some Practical Advice
I will now discuss some ideas and approaches which I have personally used to make the process of learning various DS and algorithms easier (but not easy!), both practically, and from motivational perspective.
I am confident that there will at least be one or two points here which will be useful (assuming you have not already arrived at them yourself), but I also want to emphasize that our brains might work slightly differently. Take what is useful and throw away the rest.
As a learning aid, you might also want to watch my live lesson on youtube where I cover this topic. Do not skip the rest of this article though; I go into much more detail here!
Follow A Project-Based Learning Approach
This is actually the first thing I tell any new programmer who asks me the “best way” to learn how to code. I have given a longer version of this explanation many times, but I will summarize the general idea.
In any field of programming, you will notice that there is an incredibly large number of topics to study, and the field itself is constantly evolving both for academics and industry professionals.
As I mentioned in the introduction, not only did I have no curriculum to guide my studies, but I also had limited time to study because I had rent due at the end of each month. This led me out of sheer necessity to develop and follow my project based learning approach.
In essence, what I suggest you do is to avoid learning DS & Algorithms by simply studying things topic by topic and just taking notes about each one.
Instead, you will start by picking a basic topic (like the ones I covered before) and immediately writing a code snippet or small application which uses it.
I created a repository which had a package for every family of DS & algorithm that I wanted to learn. For general algorithms it was mostly the sorts and searches (Bubble Sort, Merge Sort, Quick Sort, Binary Search, and so on). For more specific DS like Linked Lists, Trees, Heap, Stack, and others, I wrote both the DS itself and a few algorithms specific to that particular DS.
Now, I found that some kinds of DS were difficult to understand and implement at first.
One family of DS which gave me trouble for quite some time were called “Graphs.” The field in general is full of some particularly awful and overloaded jargon, but this particular topic even has a misleading name (hint: a better name would be “Networks”).
After spinning my wheels for several weeks (although in fairness I was learning this on the side), I finally admitted to myself that I needed a clear reason to use this DS in some application code. Something to justify and motivate the many hours I was going to spend learning this topic.
Having previously built a Sudoku game using algorithms that worked with two and one dimensional Arrays, I recalled reading somewhere that it was possible to represent and solve a Sudoku game using an Undirected Colored Graph .
This was incredibly useful, as I was already familiar with the problem domain of Sudoku, so I could focus intensively on DS and algorithms.
While there is plenty more that I have to learn, I cannot describe how satisfying it was when I wrote an algorithm that generated and solved 102 Sudoku puzzles in 450 milliseconds.
Speaking of, let me talk about another way to write better algorithms which can also be a great source of motivation and goal setting.
Test Your Code
Look, I know many people make the subject of testing a complete nightmare for beginners. This happens because they confuse the very simple idea of how to test code with some very elaborate and confusing tools one can optionally use to test their code. But this one is important so please stay with me.
To go back to basics, without even talking about Big O notation, how do we know if one algorithm is more efficient than another? Of course, we have to test them both.
Now, it is important to mention that benchmark tests can give you a good (or even great) general idea, but they are also strongly influenced by the system in which they are tested.
The more precise your tests need to be, the more concerned you will need to be about your test environment, set up, and accuracy. However, for the kind of code I typically write, a good general idea is all I need.
There are two kinds of tests which I find most useful for when I am writing my algorithms, both for practice and production code. The first kind of test answers a very simple question: Does it work?
To take an example from my Graph Sudoku application, one of the first hurdles for me was to build what is called an Adjacency List for Sudokus of varying sizes (I tested 4, 9, 16, and 25, which are, not by accident, perfect squares (mathematically speaking).
I cannot explain what an Adjacency List is in detail, but think of it conceptually as a Network of nodes and lines (called edges for some reason). In practice, it forms the virtual structure of the “Graph.”
In the rules for Sudoku, every column, row, or subgrid of numbers may not contain any repeats. From these rules, we can deduce that in a 9x9 Sudoku, there must be 81 nodes (one for each number), and each node ought to possess 21 edges (one for every other node in a given column, row, or subgrid).
The first step was to simply check to make sure that I was building the correct number of nodes:
The algorithm for that was really quite easy to write, but things were slightly more difficult for the next one.
Now I needed to, as they say in Graph jargon, build the edges . This was a bit trickier as I had to write some algorithms to select for rows, columns, and subgrids of dynamic size. Again, to confirm that I was on the right track, I wrote another test:
Sometimes I followed the Test Driven Development (TDD) approach and wrote the tests before the algorithms, and sometimes I wrote the tests after the algorithms.
In any case, once I was able to verify the correctness of each algorithm to the point that I was generating a solved, variably sized Sudoku puzzle, it was time to write a different set of tests: Benchmarks!
This particular kind of benchmark testing is quite blunt, but that is all I needed. To test the efficiency of my algorithms, which at this stage could build and solve a randomly generated Sudoku, I wrote a test which generated 101 Sudoku puzzles:
Initially I had two calls to System.nanoTime() immediately before and after generating 100 puzzles, and subtracted the difference to get an unintelligible number.
However, my IDE also kept track of how long a test would take to complete in minutes, seconds, and milliseconds, so I eventually just went with that. The first set of benchmarks (for 9x9 puzzles) went as follows:
Although I did not have much of a reference point, I knew that it was taking longer than a second to generate a 9x9 Sudoku, which was a very bad sign .
I was not happy about how I was preloading the Graph with some valid numbers ahead of time, so I decided to refactor my approach there.
Naturally, the result after thinking up a new way to do that was worse:
After quite a few more benchmarks which seemed to get slightly worse over time, I was pretty demoralized and wondering what to do.
I had what I thought was a very ingenious way to make my algorithm more or less picky based on how certain it was about placing a number into the puzzle. It was not working out so well in practice, though.
As is often the case, on roughly the 400th pass of my code via a code stepper (part of a debugging tool), I noticed that I had a slight mistake which had to do with how I was adjusting the value which dictated how picky my algorithm was.
What happened next blew my mind.
I ran another benchmark test, and got a weird result:
I was in complete disbelief, so the first thing I did was to undo the change I just made and rerun the test. After 5 minutes I stopped the test as this was clearly the game changing difference, and proceeded to run five more benchmarks:
Just for the hell of it, I decided to try building 101 16x16 puzzles. Previously I couldn't even build one of these (at least I stopped trying after the test ran for 10 minutes):
The point I am trying to communicate is this: It is not just that writing tests allowed me to verify that my algorithms were working . They allowed me to have an objective way to establish their efficiency.
By extension, this gave me a very clear way to know which of the 50 different tweaks to the algorithm I made actually had a positive or negative effect on the outcome.
This is important for the success of the application, but it was also incredibly positive for my own motivation and psychological health.
What I did not yet mention is that the time it took me to get from the first benchmark to the fifth (the fast one), was approximately 40 hours (four days at 10 hours per day).
I really was quite demoralized by the fourth day, but when I finally tweaked things the right way, it was the first time I ever felt like a real software engineer instead of just someone studying it for fun.
To leave you with a great image, after I ran the 16x16 tests and saw they were promising, I took a full 15 minutes to run around my rural property hollering like an excited chimpanzee that just got dosed with adrenaline.
My Final Suggestion
I will keep this one short and sweet. The worst thing you can do as a student, upon not being able to understand something difficult and complicated, is to blame yourself.
Good teachers and explanations are rare, and this is particularly true of topics which most of us find relatively dry and boring.
I had to watch approximately four videos, read approximately five articles/textbook chapters, and blindly dive into writing some code which did not initially make sense to me, just to even get started with Graphs .
This may be good or bad news for you, but I work very hard at what I do, and I very rarely find things in my field which are natural or easy for me.
My goal with this article has never been to imply that learning software engineering was easy for me, nor that it will be easy for you.
The difference is that I am told from time to time that I explain things well, and that unlike people who just regurgitate what other teachers say, I put the time in to find out what works or does not work for me, and try to share that with you. I truly hope that something in this article was useful for you. Good luck with your learning goals, and happy coding!
Before you go...
If you like my writing, you will probably like my video content. I create everything from dedicated tutorials on specific topics, to weekly live Q&A sessions, to 10+ hour coding marathons where I build an entire application in one sitting .
Self taught programmer specializing in Object Oriented Design and Clean Software Architecture. Currently Professional Android Engineer.
If you read this far, thank the author to show them you care. Say Thanks
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
Academia.edu no longer supports Internet Explorer.
To browse Academia.edu and the wider internet faster and more securely, please take a few seconds to upgrade your browser .
Enter the email address you signed up with and we'll email you a reset link.
- We're Hiring!
- Help Center
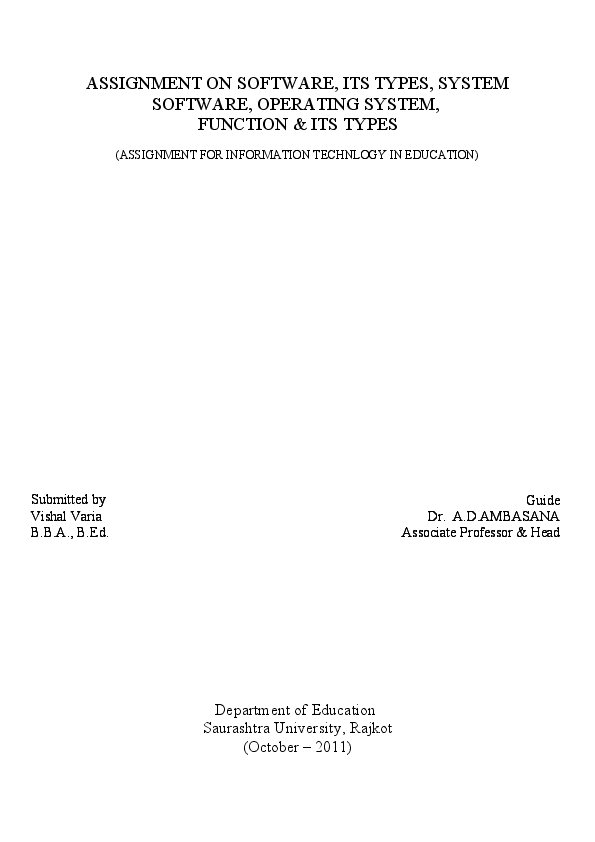
ASSIGNMENT ON SOFTWARE, ITS TYPES, SYSTEM SOFTWARE, OPERATING SYSTEM, FUNCTION & ITS TYPES

Related Papers
Kunwar Nakul Rajput

Rania Talaat
Abasido Archibong
introduction to operating system
karan singh
Seda Marshal
learn operating system
fredy kalonzo
Eidy Estupiñan Varona
Aditya Garg
ratnesh pandey
RELATED PAPERS
Mussa Msengi Gunda
Mussa M S E N G I Gunda
Ayshwarya Baburan
Luis Daniel Martinez
Aj guruvayur
ratan yadav
lIlIlIl lIlIl
1-5 D3 KBN 2017
Kajian Informasi
RELATED TOPICS
- We're Hiring!
- Help Center
- Find new research papers in:
- Health Sciences
- Earth Sciences
- Cognitive Science
- Mathematics
- Computer Science
- Academia ©2024
CS 5150 Software Engineering Fall 2013
Assignments
William Y. Arms Last changed: August 2013
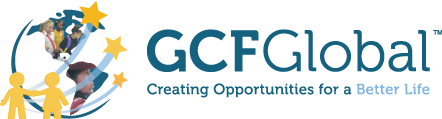
- Get started with computers
- Learn Microsoft Office
- Apply for a job
- Improve my work skills
- Design nice-looking docs
- Getting Started
- Smartphones & Tablets
- Typing Tutorial
- Online Learning
- Basic Internet Skills
- Online Safety
- Social Media
- Zoom Basics
- Google Docs
- Google Sheets
- Career Planning
- Resume Writing
- Cover Letters
- Job Search and Networking
- Business Communication
- Entrepreneurship 101
- Careers without College
- Job Hunt for Today
- 3D Printing
- Freelancing 101
- Personal Finance
- Sharing Economy
- Decision-Making
- Graphic Design
- Photography
- Image Editing
- Learning WordPress
- Language Learning
- Critical Thinking
- For Educators
- Translations
- Staff Picks
- English expand_more expand_less
Computer Science - Hardware and Software
Computer science -, hardware and software, computer science hardware and software.

Computer Science: Hardware and Software
Lesson 2: hardware and software.
/en/computer-science/algorithms/content/
Hardware and software
Hardware and software are two terms you've probably heard of at some point or another. The odds are high that you use both on a daily basis, whether it's with your smartphone or personal computer. Let's take a deeper look at what these two things are and why they're important.
Watch the video below to learn more about hardware and software.
Hardware is any element of a computer that's physical . This includes things like monitors , keyboards , and also the insides of devices, like microchips and hard drives .
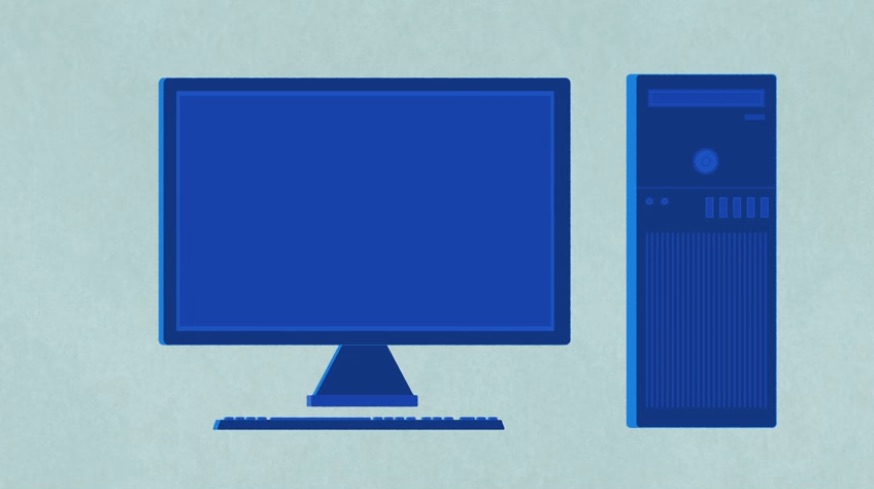
Software is anything that tells hardware what to do and how to do it , including computer programs and apps on your phone. Video games, photo editors, and web browsers are just a few examples.
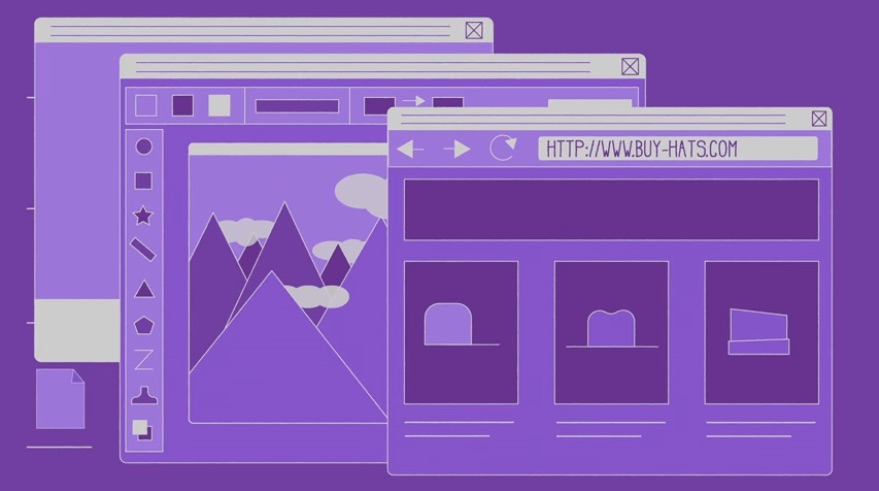
Hardware and software are different from each other, but they also need one another in order to function . Let's look at an example of this using a smartphone. In this case, the hardware would be the physical phone itself, and the software would be its operating system and apps.
If we were to take away the software, we would just have a dead phone in our hands. It wouldn't be able to make calls, text, or go online because it's not receiving any instructions .
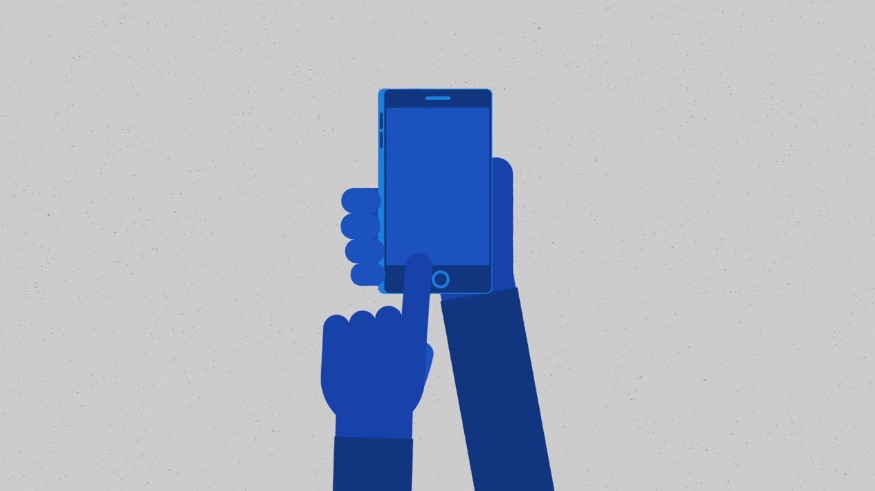
On the other hand, let's say we have no hardware , meaning there's no actual phone. If all we have are the programs, we just have a bunch of instructions but nothing to give these instructions to .
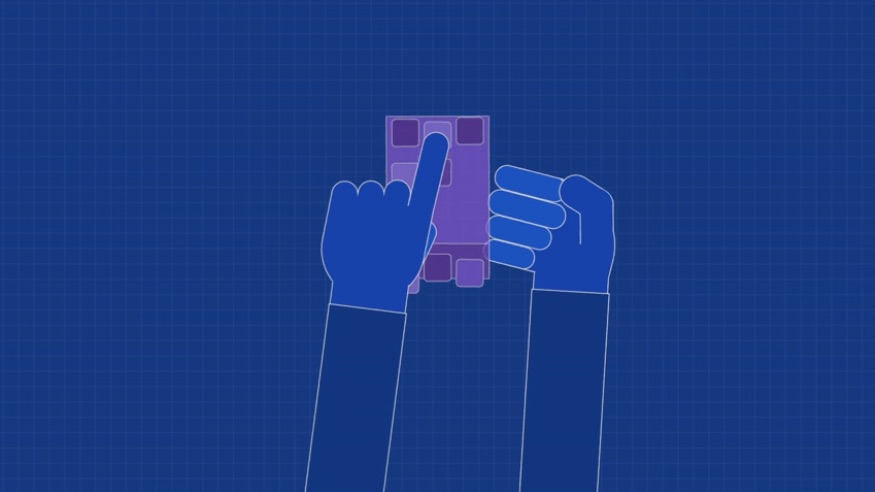
The big picture here is that hardware needs software to tell it what to do, but software also needs hardware in order to act out its directions. When you combine the two, you can do all sorts of things, whether you're using a smartphone, computer, or any other type of device.
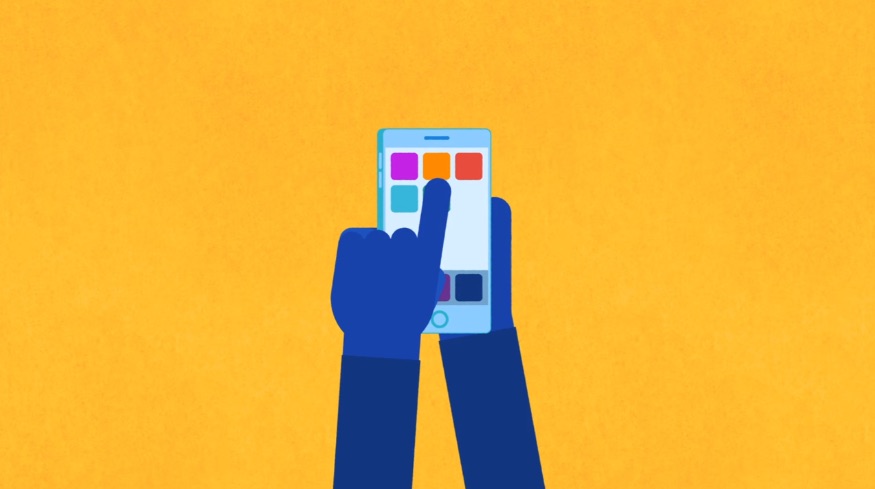
Technology will continue to evolve. We may not be sure how it will look in 100 years, but hardware and software will be there serving as its foundation.

/en/computer-science/binary/content/
- Software Engineering Tutorial
- Software Development Life Cycle
- Waterfall Model
- Software Requirements
- Software Measurement and Metrics
- Software Design Process
- System configuration management
- Software Maintenance
- Software Development Tutorial
- Software Testing Tutorial
- Product Management Tutorial
- Project Management Tutorial
- Agile Methodology
- Selenium Basics
- Pilot Testing in Software Testing
- Scalability Testing - Software Testing
- Taute Software Maintenance Model
- Site Reliability Engineering
- Defect Triage- a simple process
- Recovery Testing in Software Testing
- Scenario Testing - Software Testing
- Difference between SDLC and STLC
- Usability Testing
- Roles and Responsibilities of Participants of Defect Triage Process
- Sandwich Testing - Software Testing
- Advantages of Prototyping approach in Software Process
- Ethical Responsibilities of Reviewer
- Reliability Testing - Software Testing
- Configuration Testing -Software Testing
- Software Engineering | Program Testing
- Graphical User Interface Testing (GUI) Testing
- How to create Pareto Chart
- Different RCM Maintenance Strategies
Principles of Software Design
Prerequisites –
- Software design process
- Software design process | Set 2
- Modern principles of software development
Design means to draw or plan something to show the look, functions and working of it.
Software Design is also a process to plan or convert the software requirements into a step that are needed to be carried out to develop a software system. There are several principles that are used to organize and arrange the structural components of Software design. Software Designs in which these principles are applied affect the content and the working process of the software from the beginning.
These principles are stated below :
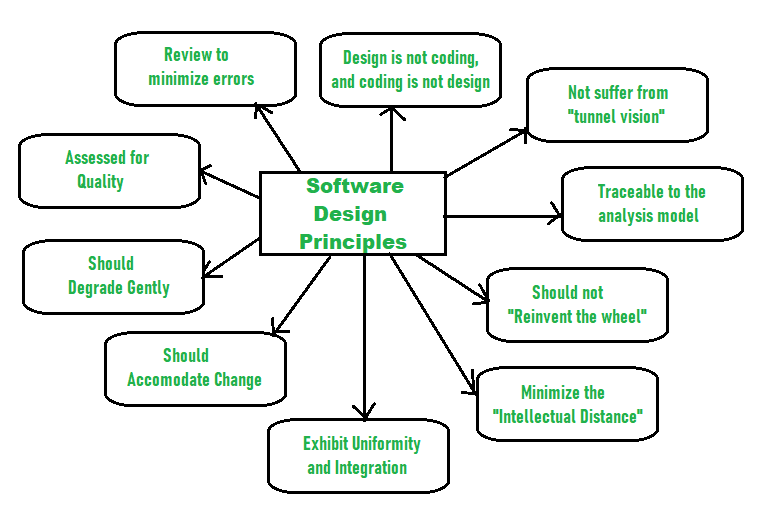
Principles Of Software Design :
- Should not suffer from “Tunnel Vision” – While designing the process, it should not suffer from “tunnel vision” which means that is should not only focus on completing or achieving the aim but on other effects also.
- Traceable to analysis model – The design process should be traceable to the analysis model which means it should satisfy all the requirements that software requires to develop a high-quality product.
- Should not “Reinvent The Wheel” – The design process should not reinvent the wheel that means it should not waste time or effort in creating things that already exist. Due to this, the overall development will get increased.
- Minimize Intellectual distance – The design process should reduce the gap between real-world problems and software solutions for that problem meaning it should simply minimize intellectual distance.
- Exhibit uniformity and integration – The design should display uniformity which means it should be uniform throughout the process without any change. Integration means it should mix or combine all parts of software i.e. subsystems into one system.
- Accommodate change – The software should be designed in such a way that it accommodates the change implying that the software should adjust to the change that is required to be done as per the user’s need.
- Degrade gently – The software should be designed in such a way that it degrades gracefully which means it should work properly even if an error occurs during the execution.
- Assessed or quality – The design should be assessed or evaluated for the quality meaning that during the evaluation, the quality of the design needs to be checked and focused on.
- Review to discover errors – The design should be reviewed which means that the overall evaluation should be done to check if there is any error present or if it can be minimized.
- Design is not coding and coding is not design – Design means describing the logic of the program to solve any problem and coding is a type of language that is used for the implementation of a design.
Please Login to comment...
Similar reads.
- Software Testing
- Software Engineering
- System Design
- CBSE Exam Format Changed for Class 11-12: Focus On Concept Application Questions
- 10 Best Waze Alternatives in 2024 (Free)
- 10 Best Squarespace Alternatives in 2024 (Free)
- Top 10 Owler Alternatives & Competitors in 2024
- 30 OOPs Interview Questions and Answers (2024)
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Ask a question from expert
Software Development Life Cycle (SDLC) Assignment

Here is the Full Assignment Report on Software Development Life Cycles
Introduction: .
The large insurance company predominantly operating in the United Kingdom is now offering its products and services to many countries in its drive to grow and become a large international company. The system that this company is using to keep track of its customer inquiries about information and purchase of its products and services needs to be updated to reflect the changes in the way it operates. So, to go towards the international market this company needs to update its old system and replace it with the new system, which provides other various services. As customers in other countries will be using a wide range of currencies to purchase the products and the insurance company needs to allow for the fluctuating currency exchange rates in its new system. For this software development task, our business consultancy company won the contract and I was hired as a systems analyst to develop a new system for the insurance company.
My Role as System Analyst
For the development of this project, I have to work with my colleagues as part of a development team. In past, the insurance company has a small development team, which solely developed the old system so while developing this new system in-house team will also work alongside us for a better understanding of their requirements. I am going to manage the project analysis and design stage of the new system. Firstly, I am going to update the in-house team on new methodologies of system development used to analyze systems.
1. Description of predictive and adaptive software development models considering at least two iterative and two sequential models.
Software Development Life Cycle:
The software development life cycle (SDLC) is an outline identifying tasks executed at each stage in the software development procedure. SDLC is a design tracked by a development team within the software organization.
It consists of a comprehensive plan describing how to develop, maintain and replace specific software. The life cycle defines a methodology for improving the quality of software and the overall development process.
The software development life cycle is also known as the software development process. (Techopedia, 2019)
Stages of Software Development Life Cycle (SDLC):
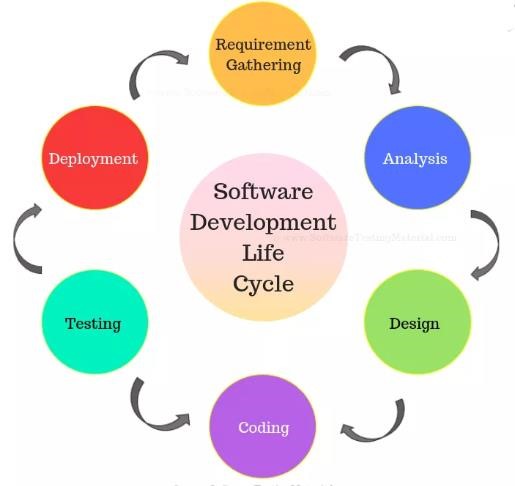
Figure 1: SDLC Phase
- Requirement Phase:
Requirement gathering and analysis is the most important phase in the software development lifecycle. Business Analyst collects the requirement from the
Customer/Client as per the client’s business needs and documents the requirements in the Business Requirement Specification (document name varies depending upon the Organization. Some examples are Customer Requirement Specification (CRS), Business Specification (BS), etc., and provides the same to Development Team.
- Analysis Phase:
Once the requirement gathering and analysis are done the next step is to define and document the product requirements and get them approved by the customer. This is done through the SRS (Software Requirement Specification) document. SRS consists of all the product requirements to be designed and developed during the project life cycle. Key people involved in this phase are the Project Manager, Business Analyst, and Senior members of the Team. The outcome of this phase is the Software Requirement Specification.
- Design Phase:
It has two steps:
HLD – High-Level Design – It gives the architecture of the software product to be developed and is done by architects and senior developers
LLD – Low-Level Design – It is done by senior developers. It describes how each and every feature in the product should work and how every component should work. Here, only the design will be there and not the code
The outcome from this phase is a High-Level Document and Low-Level Document which works as an input to the next phase
- Development Phase:
Developers of all levels (seniors, juniors, freshers) are involved in this phase. This is the phase where we start building the software and start writing the code for the product. The outcome from this phase is Source Code Document (SCD) and the developed product.
- Testing and Integration:
When the software is ready, it is sent to the testing department where the Test team tests it thoroughly for different defects. They either test the software manually or use automated testing tools depending on the process defined in STLC (Software Testing Life Cycle) and ensure that each and every component of the software works fine. Once the QA makes sure that the software is error-free, it goes to the next stage, which is
Implementation. The outcome of this phase is the Quality Product and the Testing Artifacts. After the successful test of the application, we need to integrate the various modules like login, signup, upload, claim, and services.
- Deployment & Maintenance Phase:
After successful testing, the product is delivered/deployed to the customer for their use. Deployment is done by the Deployment/Implementation engineers. Once when the customers start using the developed system then the actual problems will come up and needs to be solved from time to time. Fixing the issues found by the customer comes in the maintenance phase. 100% testing is not possible – because, the way testers test the product is different from the way customers use the product. Maintenance should be done as per SLA (Service Level Agreement)
P1 Describe two iterative and two sequential software lifecycle models
Iterative Models:
The iterative models are particular implementations of a software development lifecycle that focuses on an initial, simplified implementation, which then progressively gains more complexity and a broader feature set until the final system is complete. In this type of model, enhancements can be recognized quickly with implementation throughout each iteration. The two iterative models which I am going to describe are the prototype and agile models.
Agile model, Please add sprint and scum. Standup meeting, client’s feedback, backlog, sprint backlog. Please include the manifesto of agile.
“ Agile SDLC model is a combination of iterative and incremental process models with a focus on process adaptability and customer satisfaction by rapid delivery of working software product. Agile Methods break the product into small incremental builds. These builds are provided in iterations.” (n.d, 2018) In this model, every project is handled differently and existing methods are tailored to best suit the requirements of the project. All tasks are divided into small time frames for delivering specific features in the release. It gives priority to working software and customer collaboration over comprehensive documentation and contract negotiation. This model also allows proper response to change than following the project plan and every iteration includes a cross-functional team working simultaneously.
The agile model is an iterative and team-based way to develop. This model gives importance to the rapid delivery of the system in complete functional components. All time is time boxed in phases known as sprints rather than creating tasks and schedules for system development. While starting each sprint has a defined duration although time may vary according to the project and also the running list of deliveries. Sometimes if planned work for a sprint cannot be completed then work is reprioritized again and information is used for further sprint planning. When the work is completed then it is reviewed and evaluated by the team and customers. This model relies upon a high level of customer involvement throughout the development process and it is more especially during reviewing the system.
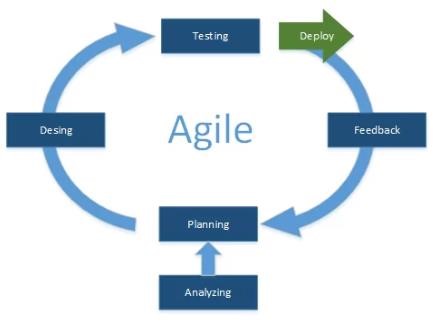
There are various phases in the agile model which are illustrated below:
- Requirements: The initial step in this model is to gather the system requirements. As this model doesn’t need full documentation to gather software requirements meeting is held and all decisions are implemented.
- Design: In this stage with the help of various software designing tools the design of the software is prepared. It can be prepared as the demo version and other important features can be added further while continuing the project. Here also the clients provide their feedback to the system and the system is altered according to their needs.
- Development: Here the designed demo version is brought into implementation for user feedback and in the end of this phase system will almost be ready. Customer collaboration and feedback plays important role in this stage.
- Testing and feedback: In this ending phase the overall system wrapping is done with all the testing required. After the customer is satisfied with the developed system it is handed to the client.
Sequential models:
In this type of model, developers have to follow some rules, regulations, and defined orders for completing the project. Here I am going to describe two sequential models of software development which are the waterfall model and the V model.
Waterfall Model:
The waterfall model is one of the most traditional and commonly used software development methodologies for software development. This life cycle model is often considered the classic style of software development. This model clarifies the software development process in a linear sequential flow which means that any phase in the development process begins only if the earlier phase is completed. This development approach does not define the process to go back to the previous phase to handle changes in requirements.
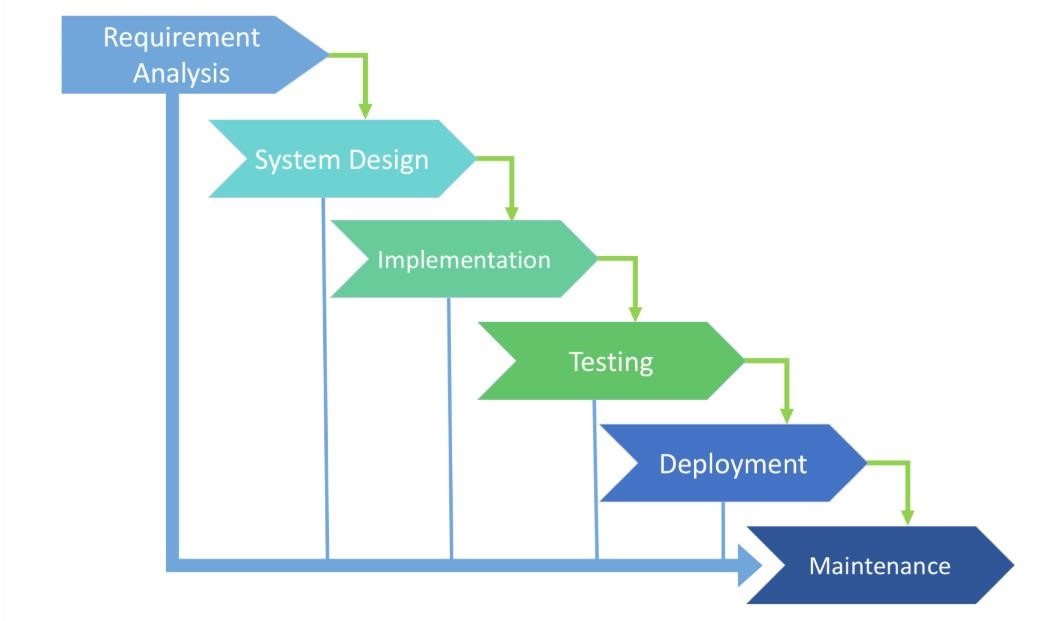
As shown in the above figure there are various sequential phases involved in the waterfall model which are explained below: -
- Requirement Gathering and analysis : All the requirements of the system which need to be developed are collected and then documented in a specification document. Specifications of the final product are marked and studied here.
- System Design: Here requirement specifications gathered from the first phase are studied and then system design is prepared. Thus prepared system design helps the developer while specifying the hardware and system requirements and also helps while defining the architecture of the overall system.
- Implementation: With the input from the system design system is developed in small units that are summed up in the next phase. Each developed unit is tested for functionality which is also known as unit testing.
- Integration and Testing: The units developed in the previous phase are integrated into the system after each unit testing. The entire system is tested for any faults and failures so that client does not face an error while installing and running the software further.
- Deployment of System: After completion of functional and non-functional testing product is deployed in the customer environment or market release as required.
- Maintenance: Even after the system deployment there may come issues in the client environment so to fix such issues patches are released. For further enhancement of the product, further versions are released in the maintenance phase. This phase is done for the entire life of the software till its death.
Application of Waterfall Model
This is the earliest model of software development and is only applicable when the requirements are very well known, clear and fixed. If there is no proper documentation for user requirements then it can’t be used. This model further can be used when the product definition is stable and technology is understood. Only after proper evaluation and reviewing each stage developer should move to the next step so proper documentation of user requirement should be done. It is also applicable where there is no chance of customer collaboration in the project and whole task is done in contract negotiation. This model can be used in projects where there are no ambiguous requirements. Waterfall model is also suitable for projects that transfer from one platform to another platform i.e. all the requirements remain the same and there is only change in system environment or the programming language. This is best suited for the small projects rather than complex large projects which take more time.
The risks involved in each of the models and how the risk can be mitigated /managed in each model by taking a reference of the spiral model.
Risks in Software Development Life Cycle
“Risk is an expectation of loss, a potential problem that may or may not occur in the future. It is generally caused due to lack of information, control or time. A possibility of suffering from loss in software development process is called a software risk. Loss can be anything, increase in production cost, development of poor-quality software, not being able to complete the project on time.” (Hilson, 2011) Those types of risks exist on our project or developing system as the future is uncertain and there are many already unknown and known facts which we cannot incorporate in project plan. While developing system those risks can be of two types first one is internal risks which can be controlled by the project manager and second one is external risks which are beyond the control of project manager. While we use various models for developing software there are also different risks attached with those specific models. Below I have provided those various models with risks involved in them while developing our software: -
- Risks involved in Prototype Model
Although using prototyping model decreases the probability of software development project failure apart from rewards this model has its own risks. The biggest risk is that anyone who is interested in the project after facing a working prototype will decide that the final product is almost ready or not. Another risk involved using prototype model is that after seeing the early prototype end users demand delivery of actual system and even if he is unsatisfied with the initial built prototype, he may lose interest in the project. While using this model in software development process, without proper management iterative process of prototype refinement can take long durations and while developer hurry to build prototype it may end up to sub-optimal solutions. Practically, using this model might increase the complexity of the system as scope of the system may expand beyond the initial plans on software development. Various risks can be encountered as this model leads to implementing and then repairing the way of creating software.
- Risks involved in Agile Model
There are various risks involved in the agile model and while developing the software we have to be aware about those risks before starting our project. Among those various risks the very first common risk is lacking details in task descriptions. We have to make sure that all details are present and clear for the team so they know exactly what they are creating and the best way to write these out are in the form of user stories or technical requirements. Another risk usually encountered while going through agile model is priorities or directions change. Sometimes the priorities of the project changes and thus features that were not originally planned take top priority over the others.
When this happens, it’s important to make sure the clients know about the effect of those changes on the developing system and even also the timeline and budget of project as mentioned in earlier meetings. Another risk which many companies faces while adapting agile model in their software development process is lack of documentation which leads to misunderstanding among the developers. Because of poor documentation in this model when the current programmer or any other member of development team leaves then it will be very difficult for the new recruiters to get adapted with development scenario as there will be less documentation and he won’t be able to grab the speed with other members. If the customer representative isn’t clear about the outcome of project then team can easily get off the track so this risk should not be underestimated while developing team and client representative should be well known about the features that clients wants to get in system. So, there would be both Advantage and Disadvantage of The Agile Method .
- Risks involved in Waterfall model
As there is very less customer interactions involved in software development and product can only be demoed once it is ready therefore once the software is developed and if any failure once then cost of fixing such issues are very high and we have to update everywhere from document till the logic. Another risk is that if the documentation of software development isn’t done well then there is high chance of getting off the track while developing software. There is always lack of project suitability using this model where requirements are at a moderate to high risk of changing. There is high chance of project failure if we use this model for complex and object-oriented projects.
- Risks involved in V-model
As, it is too simple to accurately reflect the software development process, and can lead managers into a false sense of security. The V-Model reflects a project management view of software development and fits the needs of project managers, accountants and lawyers rather than software developers or users. Although it is easily understood by novices, that early understanding is useful only if the novice goes on to acquire a
deeper understanding of the development process and how the V-Model must be adapted and extended in practice. If practitioners persist with their naive view of the VModel they will have great difficulty applying it successfully. It is inflexible and encourages a rigid and linear view of software development and has no inherent ability to respond to change.
(Anon., n.d.)
Explain how risk is managed in the Spiral lifecycle model.
Spiral model was proposed as a risk driven software development process model by Boehm in 1988 wherein the development process is guided by involved risks. This model aims to identify and evaluate the software project risks and also helps to reduce those risks. In this model the major sources of risk despite of its risk driven nature is due to high reliance on human factor and detailed risk management process. According to Boehm, almost the 25% time in this model is taken in risk analysis and it is mostly designed to known risk in the project. It doesn’t only provide the flexibility in project but also helps us to know about the upcoming risks.
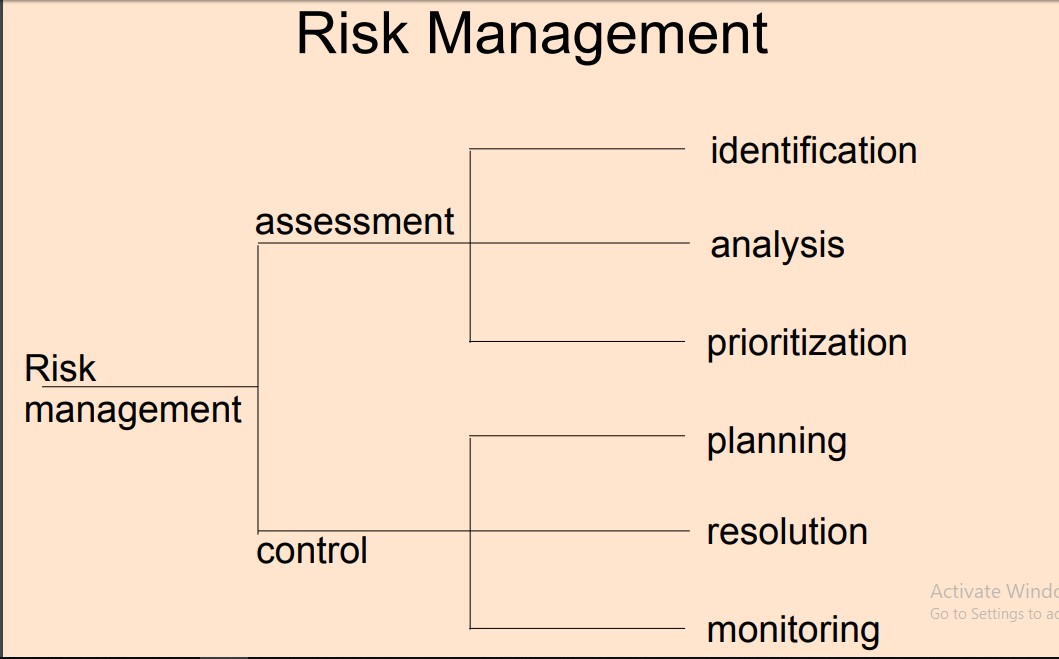
Spiral development supports risk management in software projects in several ways summarized in the following:
- Identifying most risks threaten the project.
- Classifying risks into user interface risks and development risks
- Evaluate these risks to decide upon the risks to handle through each cycle. Moreover, this classification helps developers in implementing risk resolution techniques such as prototyping and benchmarking.
- The evolutionary prototyping spirals that aim at resolving performance and user interface related risks. These spirals help in reducing major risks before proceeding into the development process.
- Resolving program development and interface control risks inherent from the start of the project.
- Evaluating and resolving the new risks that might arise after changing any of the objectives, alternatives, or constraints at the beginning of the cycle.
- Objectives, alternatives and constraints to change as more attractive alternatives exist.
- New technology to be incorporated easily during the development process.
- The maximum optimization of project resources usage.
- To deal with poorly done activities in the earlier phases.
- The review conducted at the end of each cycle with main stakeholders as a decision point to avoid the lack of commitment risks during the next cycle.
- Time and cost overrun risks are best managed using spiral development due to the risk analysis stage conducted at each cycle. In this stage, the cost and time required for each cycle are analyzed in advance to give a clear picture about the critical state of the project.
This helps the project manager and the developers get more control over these risks.
- Risks related to the increased complexity of the project are also managed using spiral.
This is achieved by the partitioning activity conducted at the planning phase.
- Decomposing the project into portions to be developed in parallel spirals obviously reduces time contention related risks, since more work could be achieved during the same interval.
Major Sources of Risk in the Spiral Model Despite its risk driven nature, spiral has its own sources of risks which are summarized in the following:
- All the activities related to identifying, analyzing, and resolving risks rely on the experience of developers and their abilities in identifying and managing risks. If these abilities are unavailable, major risks might remain hidden for several lifecycles and discovered late when it matured into real problems. At that time, the cost of rework to recover from these risks becomes very high.
- Cost and schedule risks might increase using spiral due to its iterative feature, especially for low risk projects wherein risk assessment is not required to be at this level of granularity.
Once you have prepared the report you are required to produce documentation that
M1 Describe with an example why a particular lifecycle model is selected for a development environment. You may consider a different development environment with different business logics, platform, etc., and the applicability of the particular software development model over the development environment .
Copy from above
Adaptive model
Client’s continuous feedback
Iterative model
Continuous testing
Less documentation
Manifesto of Agile
Daily Standup meeting, Sprint board.
It is obvious that the task of choosing the best Software Development Life Cycle (SDLC) methodology is very challenging task to every organizations and project managers. Each model isn’t universal and based on the scenario requirement we have to choose the best suitable model. Every project manager has to be aware about their technical capability, technology constraints, scenario requirements and features, pros and cons of chosen model for project. We can use different model relating different scenario and criteria like; RAD model for fast and rapid software development, we can choose waterfall model based on the requirements, being based on the risk and mitigating them we can choose spiral model and so on. In the scenario I was given the task of creating an application for a large insurance company where with various features in it. First it was very difficult for me to choose best model as every model has their pros and cons but after analyzing the given scenario and client requirement, I preferred agile model above others.
“Agile SDLC model is a combination of iterative and incremental process models with focus on process adaptability and customer satisfaction by rapid delivery of working software product. Agile Methods break the product into small incremental builds. These builds are provided in iterations.” (n.d, 2018) In this model every project is handled differently and existing methods are tailored to best suit requirements of project. All tasks are divided in small time frames for delivering specific features in release. It gives priorities to working software and customer collaboration over comprehensive documentation and contract negotiation. This model also allows proper response to change than following the project plan and every iteration includes cross functional team working simultaneously. Each of the iteration need cross functional team which works simultaneously on areas like: -
- Planning
- Requirement Analysis
- Design
- Coding
- Unit testing
- Acceptance testing
Justification for Selection:
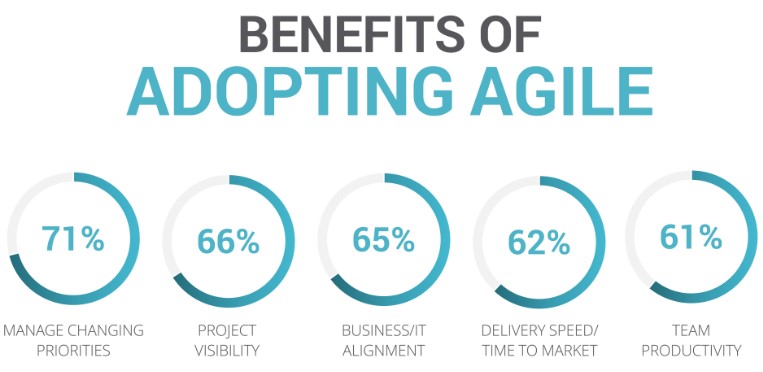
company members when required, generating documentation, guidance and counselling from expert agent and so on. Therefore, being based on such criteria I selected agile model for software development. There are various reasons for choosing this model rather than other models like RAD, Spiral, Waterfall, Prototype and so on. Company management gave me the clarity on the features that they needed in application therefore there was no detail planning required. Our developer team were in same geographical location usually we used to work in our office so it was also very easy for me to monitor the work. Software can be developed faster using this model as we were also bounded by time given by company.
Using agile model helped us to get feedback from real customers. There was never chance of such situation that we could take all our time to develop such application which client didn’t wanted so with this model administration could give us a feedback on the work which help us to be on track. This model provides us the feature of incremental delivery which helped us to build software in the way client wanted. Whenever the company wanted to know about the ongoing project then we could deliver them in smaller increments which gave them the opportunity to see emerging product and they could even respond to it. So, this model could help us and the client to converge on best possible outcome. Another reason of choosing agile model was because of early risk reduction, by delivering software early to company and getting feedback we could reduce the risk of building wrong software. By continuously integrating and developing bug free software we could reduce risk of building wrong software before delivering final product to client. This model also helped us to fix cost, time and quality while giving us the tools for varying business and technical scope. So, as we developed our software and handed it to company at last, we were sure that we delivered what they needed. This way also agile helped us for delivering better quality software. Along with those reasons another reason for selecting agile model was its principle which focuses on the customer satisfaction with the product we delivered. Implementing this model will help us to build better relationship with customers by updating the software in regular time intervals and adding new features based on their feedback. Creating better relationship with such big company administration would help us in various ways. In this today’s competitive world winning their trust by satisfying them could help us to get our future projects from them. Last reason for choosing this model was also because it is trending in today’s software development time. Mostly of the organizations use this model nowadays because of its suitable features to all the scenarios.
D1 Assess the merits of applying the waterfall model to a large software development project with appropriate evidenced researched work with reference to the current context.
Using Waterfall Model in Large Projects This is the first SDLC approach that was used for software development. It is very simple and easy to use and understand. This works in the basis that each phase must be entirely completed before next phase of development begin and also there is no overlapping in phases. In this approach the entire software development process is divided into separate phases from top-bottom approach and output of one phase acts as input for next phase sequentially. Any phase in development process begins only if it is known that previous phase is completed. Although this model is not used nowadays in large software development project in this task, I am going to describe the merits of applying waterfall lifecycle model to such large projects.
Waterfall model probably was the most popular SDLC methodology. All the teams and company’s all over world used this model to manage their projects. After the introduction of new models like agile, prototype, RAD etc. it was strongly criticized and slowly agile model replaced this model because of its various drawbacks. Though it has drawbacks and is replaced still it can be used in software organizations for large software development because of its peculiar features.
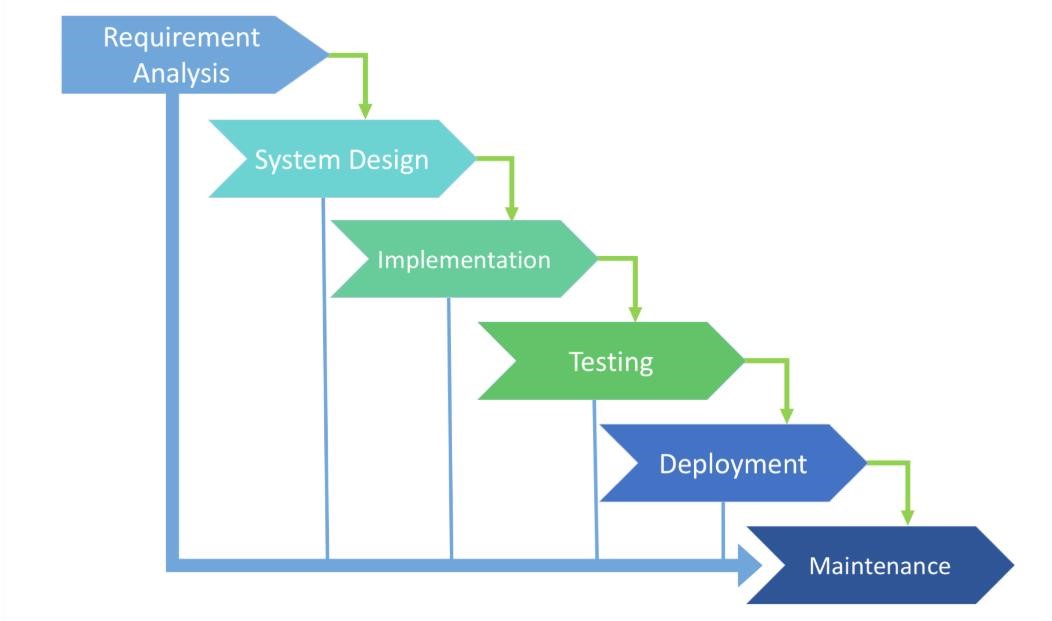
1.Predictive model and already fixed the requirement.
2.Budget has been fixed already.
3.Output of one module will be the input for next module.
Before the development of other models there were always large projects which the water fall model managed. This model provides developer the various advantages in development process. In development phase it prioritizes the task in well-defined and clear steps which makes the task easier to do. This model follows the simple step of development like requirement gathering with proper documentation, system design, implementation, testing, deployment and maintenance. In large projects there are involved various steps and using this model help us to complete each step of development effectively. As this model follows linear sequential way in development we
don’t have to look back after we complete previous stage. This will remove the tension of coming back to the same previous stage and altering the code and design.
Another merits waterfall model provides us is that the requirement is already documented on the project beginning. Therefore, we don’t have to adjust with the changing environment as we can work on specific environment during development. In the beginning of the project all the requirement of customer is gathered and proper documentation of project is prepared. Thus, in such long running projects documentation provides us the better reporting and clear goal can be set rather than changing mind in midway of project. This prepared documentation will also be useful for the future use.
This model prefers initial documentation rather than customer collaboration which also provides benefits to organization. If there was any customer involvement in large project then a lot of time can be spent for understanding between developer and clients. This waste of time would be not once but in regular time intervals so low customer involvement in large projects provides developer their own environment without any scheduled disturbance from customer. In large projects of software development where the time is 1 to 2 years if any of the developer gets transfer this will not disturb the development process. With the proper documentation any of the recruiter can use it for ongoing project works and can cover up the development process quickly.
In large software projects there are many features to be built in software which creates various errors. In such stage developer can use the verification and this way no feature will be missed from software. Moreover, in this model team works together in every step which makes it easier for them to find bugs and errors in system and resolve them. All those efforts from team and developer will lastly help to create a standard flawless software. It is very applicable in large project in term of cost efficient and convenient.
This model allows the project manager and team leader to monitor and handle project easily. After the well understanding of project between team members it can be divided reasonably among the team.
As this model follows linear sequential structure while waiting for their turn they can do their own tasks. For example, in development process coding comes in the last so while waiting for his time programmer can engage in his own work and after his turn comes in he can complete his work. This will also help the team to get their result in structured time and also huge development project can be completed in set out time. In this way using this model various features we can still use it in large projects.
Conclusion:
The SDLC process provides us the purpose to adhere client order in effective and structured manner while ensuring the better time of delivery and efficiency. It also includes plan for how to produce, modify, maintain and replace software system. Spiral model provides us the various steps through which we can eliminate the present and possible risks and complete development process successfully. It provides us the feature like risk assessment which helps in risk identification, analyzing the consequences and effects and prioritizing risk to eliminate them. Another feature was risk control with which we can plan and develop various strategies to resolve the risk and gain our complete control over system. Although agile model has some drawbacks, I preferred to choose this model above all others model. I was given some criteria to develop the software so being based on different criteria like technical capability, technology constraints, and scenario requirements I selected this model. Agile model helped us in various ways like building software faster, getting feedback from customers, better quality, providing satisfaction to customer and so on. Therefore, it is essential to choose model wisely knowing their features, along with its pros and cons so that we don’t get stocked into problem later. Though waterfall model is old model and is replaced by new popular models but still it has some peculiar features which other model don’t have. This model helps to develop a complete client required software in simple and easy way. This model has some very beneficial features for both developer and clients like; preparing the proper documentation and addressing user requirement in initial stage, reducing cost and effort by making change in design phase which overall helps to develop a standard and functional software. So, we have concluded how Systems Analysis and Design are important to analyze.
- You are required to produce a documentation that explains the purpose of the feasibility report and describe how technical solutions can be compared.
- Prepare a brief report discussing the components of the feasibility report.
- Carry out the feasibility study as per the best of your previous research work against the solution to be developed for the given problem and assess the impact of different feasibility criteria on the software investigation.
Report on Feasibility Study
Introduction:
“A feasibility study is an analysis used in measuring the ability and likelihood to complete a project successfully including all relevant factors. It must account for factors that affect it such as economic, technological, legal and scheduling factors. The feasibility study helps to “frame” and “flesh-out” specific business scenarios so they can be studied in-depth. Project managers use feasibility studies to determine potential positive and negative outcomes of a project before investing a considerable amount of time and money into it.” (Don Hofstrand, October, 2009) It tests the viability of opinion, a project or even new business. It is the preliminary study undertaken before the real work of project starts to ascertain the likelihood of the project’s success. The main purpose of feasibility study is to emphasize the problems which could occur if one pursues project and determines if, after considering all significant factors, the project is a good idea. For example, if we are going to develop a web application for our business then we might be looking to perform feasibility study to determine if it should follow through, considering material and budget, opinion from stakeholders and laws that might get affected from it.
A feasibility report is a document that assesses potential solutions to business problem or opportunity and determines which of these are viable for further analysis. The main benefits it provides is that it helps to present the project parameters and define the potential outcomes to the defined problem, need or opportunity. The report is an analysis of possible solutions to a problem and a recommendation on the best solution to use and it contains extensive data related to financial and operational impact including advantages and disadvantages of both the current situation and the proposed plan. The report generated after doing the feasibility study outlines and analyzes several alternatives or ways of achieving success in that project. It helps to narrow the scope of project to identify best scenario while completing it. We have to perform the feasibility study in the planning phase of any project and after the report is generated then it helps to know if the project is worth doing or not. It helps for our confident buildup and it can also be costly sometimes but it is better to know if project will be successful or not by doing feasibility study before. Feasibility report allows companies to determine and organize all the details to make business work. It helps in identifying logistical and business problems and also their solutions. It can also lead to the development of various strategies so that we can invest our time and money in right project.
Purpose of Feasibility Study:
Please write these things in bullets.
The significance of feasibility study is based on organizational desire to “get it right” before implementing resources, budget and time in project. It might uncover new ideas that could completely change a project’s scope. It is always beneficial to make those determinations before rather to jump in and starting the project that won’t work. The outcome of feasibility study gives us and our stakeholders a clear picture of the proposed project and is always beneficial. While doing some project or creating software feasibility study is the second document that is created following business case and this report helps us to determine the factors that will make the business opportunity which was presented in project success.
Another purpose for feasibility report is to explore the different markets where a target audience might be located. For example, if we want to create the online payment system then doing feasibility study helps us to find out where this system can be implemented. It provides us guidance to launch our system in such places where number of our audience is high such as big cities. We can analyze existing competitors to see if our new product will be able to break into the market. If our feasibility study reveal that our company doesn’t have resources to compete then we can focus our mind on other products. In this case feasibility report stops us from making costly mistake and cripple our product before it become establish. It provides us the best response so that we can create the right product for the right customers. Along with the target audience other purpose of feasibility report is to investigate how acceptable the product is to the target audience. While doing the feasibility study we have to be based on certain criteria such as technical, social, law, economical etc. So, the main purpose of feasibility report is to find if our product fits in those criteria. If the outcome of our research doesn’t fit in any of those criteria then there is high chance of encountering problems in future so feasibility report provides us the valuable information for go/no-go decision. It helps us to find out the success or failure chance of any idea. If the feasibility report shows a likely failure then we can save our budget and time for another better opportunity instead of wasting it in developing projects that never had chance of success. A feasibility study is designed to provide an overview of the primary issues related to business idea. The purpose is to identify any "make or break" issues that would prevent your business from being successful in the marketplace. In other words, a feasibility study determines whether the idea makes sense. A thorough feasibility analysis provides a lot of information necessary for project plan.
Recommendation:
In this way doing feasibility study always helps us while doing the project. The main purpose of feasibility report is to find our if it is worth doing and if the report shows negative result then it offers one chance to get it right before we invest our time and money in project. It provides us the various potential risks in that project so that we can mitigate them in initial before they turn into huge problem. Not only it tells us to remove the project ideas but also identifies the reasons not to proceed project so that in future we can be aware about those reasons before. While creating insurance company software it helped our group decide to expand existing services, build or remodel facilities, change methods of operation, add new products etc. In this way using the feasibility study while doing our project for insurance company we are able to understand about our project so I also recommend others to use this while starting their project.
P4 Comparison of Technical Solutions
Technical solutions assess the current resources such as efficiency, performance, legacy system and technology which are required to accomplish user requirements in the system within the allocated time and budget. Similar to the feasibility study of the project the implementation of the technical solution in the project can be wither before the project is started or after the project is been started. Technical solution can be compared with the feasibility study because feasibility study is done for the better decision and technical solution is the solution with the good decision that can make the business/project profitable. We can compare the technical solutions based on such criteria like elimination of human errors, performance, budget, legacy system, performance, efficiency etc. Designing and implementing the solution of the technical problems like financial problem, machinery problems etc. during the lifecycle of the project means Technical solution.
I am going to show the technical solution of cell phone and landlines and compared with the following key drives:
- Performance and efficiency
- Legacy systems upgrade
- Automation
- Elimination of human errors
- Budget/ economic
Performance and efficiency:
Cell phones are mobile which means portable and can be taken from one place to another and are operational anywhere the user can get a signal from a wireless network whereas landline phones are not mobile and customers can only use it in a single location where there is a presence of wired connection to telephone network. A cell phone can be very useful during the emergency that arise when you’re away from home. Cell phones have the capacity and advanced technology. Cell phones can also give you a chance to take live pictures or videos camera whereas landline cannot. Cell phones are more fashionable and comfortable than landline.
Legacy system upgrade
One of the clearest benefits you will see is an immediate drop in both service charges as well as call costs. It helps in the business continuity and employees still have access to core communication systems like phones, sending and receiving messages, video-clips conversation etc. it also make able to add features to your lines for easier conferencing and communication.
Automation
The both system typically play a message, then ask the caller to either press a button or speak a response. Depending on the caller’s input the automated phone system may play some information, route the caller to another prompt or connect the caller with a human operator.
Elimination of human errors
Human error is an imbalance between what the situations requires, what the person interacts and what he/she does. It happens when people plan to do the right thing but with the wrong outcome.
Cell phone have GPS technology that can find your exact location or where you trying to go.
Budget / economic
The similarity of landline and cell phone is the fact that they are both used for communication. The most common reason to choose a cell phone is that in most areas, the cost of a cell phone plan is lower than the cost of a landline, especially when you count the cost of a long distance calling plan. Nowadays, we see many people on the streets with their cell phones, as many people know that it’s easier and cheaper.
Components of Feasibility Report:
A feasibility report is a document that assesses potential solutions to business problem or opportunity and determines which of these are viable for further analysis. The main benefits it provides is that it helps to present the project parameters and define the potential outcomes to the defined problem, need or opportunity. The report generated after doing the feasibility study outlines and analyzes several alternatives or ways of achieving success in that project. It helps to narrow the scope of project to identify best scenario while completing it. It is the preliminary study undertaken before the real work of project starts to ascertain the likelihood of the project’s success. The report is an analysis of possible solutions to a problem and a recommendation on the best solution to use and it contains extensive data related to financial and operational impact including advantages and disadvantages of both the current situation and the proposed plan. We have to perform the feasibility study in the planning phase of any project and after the report is generated then it helps to know if the project is worth doing or not.
A feasibility study evaluates the potential success of project so perceived objectives are important factors in credibility of study for potential investors or other clients. While doing the feasibility study there are various criteria based on which we have to perform the task. Below I have described those components of feasibility study:
- Introduction
Background information of the proposed business or proposed project will be described such as the proposed name and purpose of the business. It also includes the skills and experience of the business investors. When we thought of doing feasibility study we have to whom the results of the analysis, research and evaluation of a proposed project and determine if this project is technically feasible, cost effective and profitable. In the introduction part of the feasibility study we have to show the general overview of the report which we are going to publish. When I performed feasibility study for insurance company in its report, we have to show the background information company, what are its features, in which country it operates etc.
- Business or Project Description
Information about the business or project as well as the nature of industry or nature of the project is discussed in this section. In this part we have to give the overall overview of project and how we are going to undertake the feasibility study for certain project.
- Purpose
It is the main component of feasibility study, first of all the researcher should understand the purpose of doing feasibility study to achieve the desired result. The main purpose for the feasibility study can be based on organizational desire to “get it right” before any organization implements resources, budget and time in project. In the purpose of feasibility report it can uncover new ideas which could completely change a project’s scope.
- Methodologies
Methodologies give the way of performing or conducting feasibility study in a proper manner. It helps to select appropriate method for the data collection, organizing data and way of doing the project. For doing the feasibility study we have to be based on various methods to finds data and facts about the project. The various methodologies for feasibility study can be questionnaire, interview, surveys, meetings with people. By interacting with the people, we can know more about the scope of project in certain location and for certain audience.
- Background History
Background history is also considered as the component of feasibility study. Gathering background information of any project will leads the project in the successful way. These components of feasibility study show us the background of project what was the need for performing the feasibility study in this project.
- Intended Audience
These components refer to the audience which will be using the software once it is developed. For that we have to perform feasibility study on them and find out whether the audience needs such type of software or not. If the developed software helps the intended audience to make their task easy then the success rate of software launch will be high.
- Feasibility Criteria
The feasibility criteria are the points based upon which we have to perform feasibility study. The feasibility criteria include technical, social, legal, operational, economic, schedule etc. While we perform the feasibility study in targeted audience, we have to do research based on those criteria. For example, in schedule criteria we have to find out whether the proposed project will be completed in time or not, and in economic criteria we have to find out whether the allocated budget will be enough for software completion.
- Outcome
This component is also called the final result of the feasibility study. After we perform the feasibility study in the targeted audience then the report published needs to be analyzed. After the report is analyzed by the system analyst, we can finally come to the conclusion of whether the project should be started or not. These components provide us the final output of feasibility and evaluating this we can decide whether to start project or not.
- Conclusion and Recommendation
After the outcome of feasibility study is published then system analyst and other project stakeholders have to interact with each other and come to the final conclusion. These components provide overview to the other individuals about the project and feasibility study. After the conclusion is drawn from the report then we also have to recommend it to other projects. We have to recommend it to project managers so that again in another project we get chance to get it right before we start any project.
- Evaluation Criteria
We must remember that a feasibility study is a way of thinking rather than a bureaucratic process . For example, what I just described is basically the same process that we all use when buying a car or a house. As the scope of the project grows, it becomes important to document the feasibility study, especially if it involves large sums of money and/or how important delivery is.
The feasibility study should contain not only sufficient details to continue the next phase of the project, but should also be used to conduct a comparative analysis when preparing a final project review that analyzes what has been delivered against what was proposed in a feasibility study.
Feasibility studies represent a general approach to planning. Frankly, it's just a good job to implement. However, I have read that some people in the field of information technology, such as proponents of the "agile" methodology, consider that feasibility studies are a huge waste of time. If that's true, got to have a good used car I want to sell.
About feasibility study in Insurance Company
“A feasibility study is an analysis used in measuring the ability and likelihood to complete a project successfully including all relevant factors. It must account for factors that affect it such as economic, technological, legal and scheduling factors. The feasibility study helps to “frame” and “flesh-out” specific business scenarios so they can be studied in-depth. Project managers use feasibility studies to determine potential positive and negative outcomes of a project before investing a considerable amount of time and money into it.” (Don Hofstrand, October, 2009)It tests the viability of opinion, a project or even new system which insurance company is planning to do. It is the preliminary study undertaken before the real work of project starts to ascertain the likelihood of the project’s success. The main purpose of doing feasibility study in insurance company new system is to emphasize the problems which could occur if we pursue project and determines if, after considering all significant factors, the project is a good idea. A feasibility study evaluates the potential success of our new system so perceived objectives are important factors in credibility of study for insurance company potential investors or other clients of company. There is special purpose of doing feasibility study in insurance company new system as we have to know if the new proposed system will be worth doing or we have to reconsider some of its functions which might affect us further.
Problem Statement of Old System
From the very few years the insurance company was running a system which has less features as compared to the today’s technology so to go through latest system functions company has decided to update it to latest versions. In the previous version there was the system which was only used to keep track of customer enquiries about information and purchase of its products and services and making claims. Previously the system was only being used in United Kingdom thus there weren’t used the range of currencies to purchase the products so there was no need to allow for fluctuating currency exchange rates. In the previous versions customer were only able to read the facts and not allowed to make desired change in the system. Customers weren’t able to communicate to company through online customer care service through email or any other messages. Previously, there was only the documentation of customer information and proper security wasn’t provided to the system as related to today’s technology.
My Feasibility Study’s report for given project
After the project was given to our company and before signing the final agreement document my company told me to do feasibility study in this project. So, based on the various criteria of feasibility study I began my research and reached to final conclusion of project work. While doing the work my feasibility research was based on different criteria which were related to company’s environment such as technical, economic, social, legal, operational, schedule etc. Below I have provided the documentation of my research work on the given scenario project.
Technical Criteria
The technical criteria of feasibility study focus on the available technical resources in the organization. It helps the company to determine whether the technical resources available in organization meet capacity and whether their technical team will be able to convert ideas into the working system. It also involves the evaluation of software, hardware and other various technological requirements for proposed system. This criterion explores if the project feasibility is within the limits of current technology and if it is available with given resource constraints.
While conducting feasibility study here I find out what type of hardware and software’s we will need for making such large software. We have to use high performance server and another device for managing network traffic. If all those features can be provided by us then we can move forward otherwise we have created some solutions to these criteria for moving forward.
Impact on Software Investigation
The above given facts are only planned while doing the feasibility study and for such large projects if somewhere there is need of extra technical requirements like extra computers, extra features and need of other manpower then such requirements can be added as required. All the above given technical requirements can be fulfilled by our company current manpower and requirements so we can use and manage those technologies which is positive impact for system development.
Alternative Solutions for Problems
While developing the system we can encounter problems anywhere like in the designing phase, development, testing etc. therefore we need to have some alternative solutions if the problems arise during development. If the problem arise for the manpower of the development team then quickly new members will be recruited and development process will go on. If we encounter the problem related to hardware and software then for the hardware backup files will be sent to another computer and if software crashed or any other problem arise then we will renew those software’s.
Legal Criteria
The legal criteria investigate whether any aspect of proposed system conflicts with the legal requirements like zoning laws, data protection acts or social media laws. We have to check our system comply with all the legal requirements like data processing system must comply with local data protection acts etc. Our project might face legal issues after completion if these criteria isn’t considered at this stage and performing these criteria, we can save considerable time and effort by learning that project wasn’t feasible right from the beginning. While doing the research on our insurance company system feasibility we find out if the planned project will affect with some country’s legal act or not. If our project affects any country laws and acts then we have to find out some alternate solutions for those laws. We find out that our proposed system doesn’t affect any country cyber laws or Data Protection Acts so we can move to next step and we are going to follow rules and regulations for each country where company has branch and the proposed system will be implemented which is positive impact on the system investigation.
Economic Criteria
The bottom line for many projects is economic criteria. It involves cost/benefit analysis of project and helps us to determine viability, cost and benefits analysis of project before the financial resources are allocated. In this criteria cost of hardware, software and user training are considered and we also need to determine the budget of the project in advance after analyzing the requirements. We can also use economic analysis for evaluating the effectiveness of the proposed system. As soon as the specific requirements and solutions are identified then the project analyst can find the costs and benefits of each alternative by performing cost-benefit analysis. While conducting feasibility study I figured out if the given budget will be sufficient for the system development or not. The given budget for the system development is 30 lacs so for each phase of software development we have to divide budget as required. Below I have divided the total budget provided by company for various stages of system development.
Impact on System Investigation
In the above table I have provided the budget separation for the system development. I have separated the budget for each phase of system development life cycle with feasibility study, hardware and software. If we make software for budget of 30 lacs the in return the insurance company can get benefits from Nepal, United Kingdom and from other international market then it will provide us the positive impact. In the above table I have only provided the budget figure of 26 lacs so that in case of emergency we can use another 4 lacs.
Alternative Solutions for Problems
While developing the system we can encounter problems anywhere like in the designing phase, development, testing etc. therefore we need to have some alternative solutions for budget if the problems arise during development or any other stages. While doing the feasibility study I have figured the total budget of 26 lacs while the given budget is 30 lacs so that we can use another four lacs in emergency case. Also, for the remaining 4 lacs we can use it for adding programmers or backup hardware and software if we have to finish work quickly or if we encounter problems for hardware and software.
Social Criteria
This criterion determines whether the proposed project will be satisfactory for the people or not. In this stage we have to examine the probability that the system would be accepted by the group of people that are directly affected by the proposed system. If the proposed system affects the people then either we have to solve those issues or if we start project without solving those issues it might create huge problems in future. In this criterion we also determined what skills the target population need to learn on the job and how to learn them if the jobs will meet their needs. It incorporates a participation strategy for involving a wide range of stakeholders and accesses whether the organization is likely to succeed in making the social changes.
We found out that the proposed insurance company system doesn’t affect the people and there was high probability of system being accepted by the society as they are the one directly affected by the proposed system which is positive impact. If somehow, we encountered the problems in society regarding the system then first, we will find out the reasons which are affecting then we will try our best to mitigate those problems.
Schedule Criteria
This criterion is the most important for project success after all a project will fail if not completed on time and organization estimates how much time the project will take to complete. We also have to determine if the project deadlines are reasonable whether constraints place on the project schedule can be reasonably met. Some projects are initiated with specific deadlines and we have to determine whether the deadline of project development are mandatory or desirable and if it is preferable to deliver a properly functioning information system two months late than to deliver error prone useless information system on time. Although the missed schedules are bad but inadequate system are much worse in today’s competitive world. The given time for creating system is five months so we checked through schedule criteria of feasibility study if the given time will be sufficient for or not.
In the above table
I have provided the time schedule for the system development. I have separated the time for each phase of system development life cycle with feasibility study, design and development etc. In the above table I have only provided the time figure of four months and twenty days so that in case of emergency we can use another ten days fixing those errors and complete project within time. As we create this table in feasibility study we can properly use and manage the scheduled time while developing our system which is positive impact on system investigation.
While developing the system we can encounter some problems anywhere like in designing phase, development, testing etc. therefore we need to have some alternative solutions for schedule if problems arise during development or any other stages. While doing the feasibility study I have only provided the time figure of four months and twenty days so that in case of emergency we can use another ten days fixing those errors and complete project within time.
Also, we are going to negotiate to the company if in given time our task isn’t completed as we believe in delivering quality product to our clients and for this time shouldn’t create any problems.
Operational Criteria
This criterion involves undertaking a study to analyses and determine how well the company need can be met by project completion. It also defines the urgency of problem and acceptability of any solution and also shows if the system is made then how it will be used. This criterion also analyses how system plan satisfy the user requirements identified in the early stage of requirement analysis of system development. The operational criteria include the people oriented and internal issues such as manpower problems. We checked how the designed product i.e. system of insurance company performs in real operational environment and how operation should provide the adequate throughput and response time. In this criterion we can check if there will be resistance from uses that affects the possible application benefits or not and it also provide us the cost-effective information services to the business which is the positive impact for the system investigation.
In this way I have provided the documentation of my feasibility study as per my research work when we were developing system for insurance company. In the above documentation I have also assessed the impact of different feasibility criteria on the software investigation.
In this part of my assignment I have provided the documentation that explains the purpose of feasibility report and describes how technical solutions can be compared. I have explained how the main purpose of feasibility study is to emphasize the problems which could occur if one pursues project and determines if, after considering all significant factors, the project is a good idea. The feasibility report is an analysis of possible solutions to a problem and a recommendation on the best solution to use and it contains extensive data related to financial and operational impact including advantages and disadvantages of both the current situation and the proposed plan. I have also explained how the technical solutions can be compared based on different criteria such as efficiency, performance, legacy system, elimination of human errors. A feasibility study evaluates the potential success of project so perceived objectives are important factors in credibility of study for potential investors or other clients. While doing the feasibility study there are various criteria based on which we have to perform the task such as economic, technical, schedule, legal, social etc. After the project was given to our company and before signing the final agreement document my company told me to do feasibility study in this project. So, based on the various criteria of feasibility study I began my research and reached to final conclusion of project work. I have also provided the documentation of my feasibility research which was based on different criteria which were related to company’s environment such as technical, economic, social, legal, operational, schedule etc.
1. Undertake the software investigation to meet the business need using appropriate software analysis tools/techniques to carry out a software investigation and create a supporting documentation. You may submit this task in the form a report structured with background information, problem statements, data collection process and summary etc.
In order to carry out the systems investigation you are required to identify the stakeholders, identify the requirements of the client, specify the scopes like inputs, outputs, processes and the process descriptors, consideration of alternative solutions and security considerations and the quality assurance applied.
You are also required to identity the constraints like costs, organizational policies, legacy systems, hardware requirements etc.
For software analysis you may use the following tools:
- Data Flow Diagram up to second level
- Entity Relationship Diagram
While I am working as the system analyst in the ongoing project identifying the stakeholder and to know the client requirements from the project is important task for me. In this part of my assignment I am going to undertake software investigation to meet the business need using appropriate software analysis tools to carry out a software investigation and create supporting documentation. I am also going to identify the stakeholders, identify the requirements of clients, specify the scopes like inputs, outputs, processes and the process descriptors, consideration of alternative solutions and quality assurance applied.
Identify Stakeholders:
A stakeholder is either an individual, group or organization who is impacted by the outcome of a project. They have an interest in the success of the project and can be within or outside the organization that is sponsoring the project. A stakeholder is a person, like any other member of the project, and some will be easier to manage than others. There are a lot of people involved in getting a project from inception to successful completion. We are going to have to know how to manage each and everyone one of them, even those who don’t work directly under us. One such person is the project stakeholder. Stakeholder and Community Engagement can have a positive or negative influence on the project.
It is very important to identify the stakeholders of our project as the success of project depends upon it. We have to meet up with the requirements of project stakeholders and we have to figure this out as soon as our project starts. We can identify our stakeholders by reviewing our project charter which are the reason for the project and appoints the project manager. We can also discern the stakeholders among the information about objects, budget, assumptions and constraints, project sponsor and top management schedule. In the table below I have provided the stakeholders of our project.
Identify Client Requirements:
Clients have certain needs and requirements for the system and they need to establish the relationship between the organization and the clients. We can’t forward the project without knowing the requirements of clients and project success depends only when the requirements of clients are met so it is very important to know the client’s requirements and fulfill them. Each of the clients might have different requirements for the software they want and identifying the requirements of the client helps us to accomplish the development of the software.
Software Scope:
“Software scope is a well-defined boundary, which encompasses all the activities that are done to develop and deliver the software product. The software scope clearly defines all functionalities and artifacts to be delivered as a part of the software. The scope identifies what the product will do and what it will not do, what the end product will contain and what it will not contain.” (Bhatia, 2017) It describes the functions and features which are meant to be delivered to end users and this is where we have to list out the tasks which needs to be done given the project goals and estimate how much time will be required to do them. The software which we develop will be used by the insurance company to save data about their clients, stakeholders, employees and it will make their work easier. We have defined the input, process and output of the software which is given in table below: -
Process Descriptors:
Our system has various functionality and activities which are performed in the software and it works on many processes. The system which we developed for the company has many features which was developed according to our client’s requirements. When we instruct some input to the system then it shows some specific outcomes of the activity as input are processed and then output is shown. While the user tries to login to the system then first the login panel will appear where user have to provide username and password then system will verify those login credentials and only then user get access to the main page of system. Also, when the user tries to search the client details in the search box then system looks for the searched name in database then only it lists out the client details. Whenever the new user tries to save its details in system then first it will get connected to the database then only the data are stored. When the user tries to generate report of his details the first system has to verify if user’s transaction is recorded in system then only the report is shown to user. We have also provided the feature of customer care and contacting directly to company and for this process first system will look if company’s customer care is currently available and if yes then user can contact to the customer care services.
Consideration of Alternative Solution:
Due to competitiveness in the software industry and also because of today’s online market it is always better to have alternative solution for any software. Creating software for such huge insurance company with all required features isn’t an easy task and it is necessary that it is computationally supported since everyday projects are larger and these should be developed in shortest time and within given budget. Although the insurance company told us to create software but while considering the alternative solution, we can also use web application. Web application also helps the company to work securely and prevents from uncertain and sudden problems for such large company such as system failure, crash of software, system hang due to load; so, in such case web application can be alternative solution. Also, In the current scenario many companies prefer to build web application then software as it is little cheap then creating software, easy and nowadays most of those large company clients are moving to online market.
Software Security:
“Software security is about building secure software: designing software to be secure, making sure that software is secure and educating software developers, architects and users about how to build secure things. On the other hand, application security is about protecting software and the systems that software runs in a post facto way, after development is complete. Issues critical to this subfield include sandboxing code (as the Java virtual machine does), protecting against malicious code, obfuscating code, locking down executables, monitoring programs as they run (especially their input), enforcing the software use policy with technology and dealing with extensible systems.” (gary, 2011) We have to provide integrity, authentication, availability and accountability to the system to protect it fromattacks like buffer overflow, command injection and SQL injections. We can prevent our software from these type of attacks by following good programming techniques while creating the software and then after we can provide the system level security using firewalls, intrusion detection and prevention which might help us in stopping attackers from easy access to our software. Moreover we have also tried to make user password strong, unique and hard to guess by using password policy.
System Quality Assurance
“Software quality assurance (SQA) systematically finds patterns and the actions needed to improve development cycles. Finding and fixing coding errors can carry unintended consequences; it is possible to fix one thing, yet break other features and functionality at the same time. SQA has become important for developers as a means of avoiding errors before they occur, saving development time and expenses. Even with SQA processes in place, an update to software can break other features and cause defects commonly known as bugs.” (Rouse, 2015) For implementing the quality assurance system first we have to set goals for the standard and then we have to consider the advantages and trade-offs of each approach. We can do this by maximizing efficiency, reducing cost or minimizing errors and for this management must be willing to implement process changes and work together to support quality assurance and establish standards for quality. System quality assurance is organized into goals, commitments, measurements, verifications and abilities. For example for the quality assurance of our software we hired highly skilled developers, and skilled testing team so that we can find out bugs in testing phase and it might not create problems further. Testing was done in each phase to ensure that nothing is missed in the development of the software. Proper documentation was done before the developing process where we enlisted everything like things needed to be done in each phase, requirements, etc. We have made sure that the quality of our developed software will meet the requirement of the clients.
Constraints
The constraints in system development are anything that restricts the action of development team which can cover a lot of territory. The main constraints for project or software development are time, resources and quality and most of the projects in IT industry are driven by those triple constraints i.e. time, resources and quality. All the projects are carried out under the certain constraints and it is the budget or cost available to be used toward the development of system. Constraints have direct impact on how the system development is planned and tracked and what can be developed by a specific point in time. Below I have identified the constraints for our project like costs, organizational policies, legacy systems, hardware requirements etc.
Cost: This is the estimation of the amount of money that will be required to complete the project. Cost itself encompasses various things, such as: resources, labor rates for contractors, risk estimates, bills of materials etc. All aspects of the project that have a monetary component are made part of the overall cost structure. We were given the total budget of 30 lacs while developing system and below I have provided my feasibility report which shows total of 26 lacs and remaining 4 lacs we are going to use in case of emergency.
Organizational Policy:
“An organizational policy is a set of guidelines and best practices put in place to protect the company, employees, and customers. Organizational policies may cover employment practices, employee conduct, disciplinary procedures, Internet and e-mail use. Customer policies provide guidelines on working with customers and outline what customers can expect from the organization. In general, organizational policies define what is or is not permitted within the organization. By doing this, they establish expectations and limitations related to behavior.” (Dawson, 2013) The main purpose for our organizational policy is to provide clear definition or boundaries within which to work, define what is acceptable and unacceptable behavior and provide guidelines and set customer expectations. There shouldn’t be inclusion of any other external policy while developing system. Also, while developing the system we are going to pay attention to different countries rules and regulations.
Legacy System:
A legacy system, in the context of computing, refers to outdated computer systems, programming languages or application software that are used instead of available upgraded versions. There might an outdated system which could still be working well. So, the developed software will be made compatible with old system. Older system may require added compatibility layers to facilitate device functionality in incompatible environments. In our development team we have also hired the developers of insurance company development team so that they can tell us about old system and we can make new system compatible with old system.
Hardware Requirements
The system was developed for the hardware like Personal Computers, Laptop, MacBook and Mobile. It can be used in mobile like android, IOS and windows phone. Software can be used in computers. Various hardware was required in building the system Personal computers, Laptop, MacBook, storage disks, etc. Below I have provided the hardware requirements for software.
Background Information:
A large insurance company which is predominantly operating in United Kingdom is going to be offering its products and services to many international countries in its drive to grow and become a large international company. The company has been using a system to keep track of customer enquiries about information and purchase of its products and services and making claims etc. which needs to be updated. As company will be going globally, customers from various countries will be purchasing the products with much wider range of currencies. So, the new system that insurance company needed is according to the currency exchange rate. There should be this system in the newly developed system for the insurance company.
Problem Statement:
From the past few years the system of insurance company was providing user the application facility through general features only like Creating account, Updating the details and Deleting the details. As the technology is slowly directing towards the online the company wants to upgrade old system into new version with all the required functions. Previously, the company was using system only to track its customer enquiries, purchase of its products, services and claims etc. As now the company is going to extend its branch globally and become a leading insurance company it needs to upgrade its old system into new with today’s required features. There will be customers from different countries, different currencies will be given by the customer in order to purchase the products so there should be feature of fluctuating currency exchange rates in system and also Speed, Accuracy and Flexibility should be provided to the application using right technologies which wasn’t available in old system. Therefore to overcome those previous problems company hired us to develop new system which will function properly in all countries with new popular features in online market.
Data Collection Process:
It is the way of gathering and measuring information on variables of interest, in an established systematic process that enables one to answer stated research questions and evaluate outcomes. The data collection component of research is common to all fields of study including physical and social sciences, humanities, business, etc. Before starting to develop a new system for company there were other important things to do like collecting the data about company and its facilities. For the data collection process, we took an interview from the IT team of company and what they want from new system. We also provided the questionnaire to clients and read about the previous system review so that we can find out what they were expecting from the new system. We also identified the stakeholders for the system and distributed questionnaires to know their expectations from the system and organized workshop to provide certain information to group members on the working phases and techniques to be used while developing a new system for insurance company. By this process we were able to know various things about the client’s and user expectations from us and what additional features we can add to system so that it will be easy for new users.
Software Analysis Tools:
For software analysis, we used various tools such as Data flow diagram (DFD) and entity relationship diagram (ERD) . The software analysis tools are discussed briefly below:
Data Flow Diagram (DFD)
“A data flow diagram (DFD) maps out the flow of information for any process or system. It uses defined symbols like rectangles, circles and arrows, plus short text labels, to show data inputs, outputs, storage points and the routes between each destination. Data flowcharts can range from simple, even hand-drawn process overviews, to in-depth, multi-level DFDs that dig progressively deeper into how the data is handled. They can be used to analyze an existing system or model a new one.” (Bhagnani, 2017) This diagram shows how the data is processed by a system in terms of inputs and outputs and as its name illustrates DFD mainly focus on the information flow in system, where data comes from, where it goes and how it gets stored in the database. Apart from the data flow in system there is also shown the information flow which is represented by various symbols like rectangle, arrow sign and circle. Although there are various levels in Data Flow Diagram below, I have provided the diagram of level second. In the second level data flow diagram information is extracted and figured out than the previous levels one and zero. Sometimes it becomes complex to design the clear second level data flow diagram but when designed by the skilled person it provides us the clear picture of system data flow and provides a lot of information.
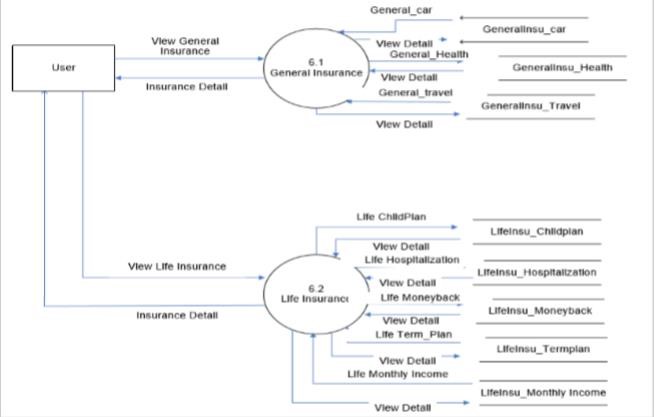
Entity relationship diagram:
“An entity relationship diagram (ERD), also known as an entity relationship model, is a graphical representation of an information system that depicts the relationships among people, objects, places, concepts or events within that system. An ERD is a data modeling technique that can help define business processes and be used as the foundation for a relational database.” (Rouse, 2017) This diagram helps us in the representation of the relationship among the entity and the attributes and helps us in representing how the entity is directly related to the attributes and how they relate with the system. This process is used for the system and project development as it helps us in the identification of the elements and relationship among them. The elements that are used to draw the ER diagram are attribute, entity and relationship among them.
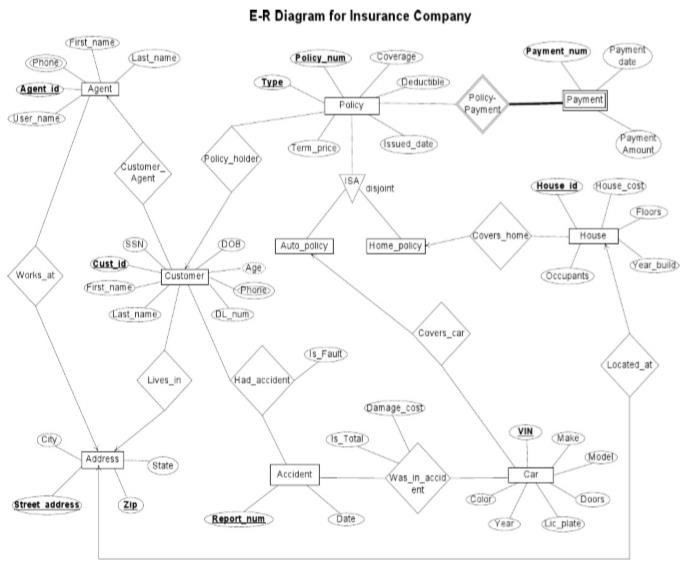
Developing the new system for such large company with inclusion of all its features is very difficult project to do. When the project was first given to our company then first of all we decided to perform the feasibility study on this new system development and when the report of study was good then we dived into the software development process. To develop any system first we need to choose the best system development model and be clear about the reasons for choosing that specific model in this project. We analyzed the various system development models like Waterfall, Agile, Prototype, Spiral etc. After knowing the pros and cons of each model we gathered the overview from all the project members and decided to go with Agile model in this project. Then for the initial planning phase we went through the feasibility study report and hired the new required developers, technologies, software etc. As in the agile model we don’t have to do documentation and we can add new requirements from clients whenever we have to so after the proper planning of project, we moved toward the next step which is designing phase. We designed the Data Flow Diagram and ER-Diagram for the system which helped the developer and any other project member to get overview of whole system and how it will work once it is completed. We hired the top designer from our country to design the system layout using various technologies and after the completion of this step we moved toward the development phase. In the development phase we used top technologies and popular language for proper coding to make the software fast, reliable and accurate. We also used various technologies to provide security for the software using firewall and popular web hosting company. Different constraints were listed out such as costs and organizational policy. We also find out the reason behind developing a new system and problem that insurance company had in their old system. We went through a data collection process where we held workshop, formed groups, took an interview and asked questions to the head of the insurance company. We tried our best for the quality assurance of system and for this we used highly skilled developer, testing in each phase, proper documentation although it is not highly required in agile model. We also arranged face to face client interaction so that we can show them the ongoing process in system development and get quick feedback which helped us to increase the software quality.
Software Requirements:
The software requirements are description of features and functionalities of the target system. Requirements convey the expectations of users from the software product. The requirements can be obvious or hidden, known or unknown, expected or unexpected from client’s point of view. They are descriptions of the services that a software system must provide and the constraints under which it must operate. Requirements can range from high-level abstract statements of services or system constraints to detailed mathematical functional specifications. We should try to understand what sort of requirements may arise in the requirement phase and what kinds of requirements are expected from the software system.
Although every development model says that we have to state the software requirements in the initial stage of development stage but we can also trace the software requirements throughout the software lifecycle. In software development models like Agile, Prototype there is no proper client requirements documentation required so we can easily add new requirements whenever we want. After finding out the software requirements we can prioritize those requirements based upon their needs and making work easier by implementing them on system. High prioritized requirements can be done first and then according to the priority of requirements we can implement them on system. After doing this user can easily surf on the system and find out the features which he needed to perform in system. We can also develop the prototype to meet the system requirements. Prototyping is building the user interface without the detail functionality for user to interpret the functions of intended software products. It will help us giving better idea of requirements and if user isn’t aware of its own requirements then we can create the prototype based on the mentioned requirements. Then prototype can be shown to client and feedback can be noted and software requirements can be found out using prototype.
The model used in the software development process also helps us to find requirements. As we were using agile software development model it made us easy to get requirements in each phase of the development process. The lifecycle of software starts from planning phase and ends in maintenance after it no longer requires update and new version replaces it. Therefore, we can trace the software requirements throughout the software lifecycle from the initial planning phase to the last maintenance phase when the system is replaced by new software. The first step of software lifecycle produces high level overview of project relating requirements and scope of software. It is very important for software requirements in software development process. In the next phase of software lifecycle i.e. analysis the development team visits the user and studies their system. They investigate the need for development in the given system. By the end of the feasibility study, the team furnishes a document that holds the different specific recommendations for the candidate system. It also includes the personnel assignments, costs, project schedule and target dates. The requirements-gathering process is intensified and focuses specifically on software. To understand the nature of the programs to be built, the system developer must understand the information area for the software, as well as required function, its behavior, performance and other systems it interfaces with.
The next step in software lifecycle is design phase where we can figure out how the application will function to meet both the needs of the business and the needs of its users. Software design can take on many forms. There may be wireframes that sketch out the web or mobile app screens. There may be a higher fidelity design or prototype where you get to see or use a mockup of the system complete with great graphics, fonts, and images. There may even be very technical specifications that detail logic, workflow, or the data of the system. All of these documents help describe on paper how the system should look and work. We can add new software requirements for the design of software in this phase. In the implementation part of software development requirements can be prioritized. According to level of prioritization, software developments things will be done. High priorities things will be done in the first place of development phase of software. While implementing, we can add more requirements if needed for the software. Used models can also help us to find out the more requirements in this phase.
In the testing phase of software lifecycle requirements testing is done to clarify whether the software requirements are feasible or not in terms of time, resources and budget. This procedure helps improve requirements quality and reduce the number of tests necessary to meet these requirements. When testing the system, we can also find out the new additional requirements which we can add to the system. The maintenance phase is the longest period of software lifecycle and majority of the software requirements are added in this phase. After the new system is launched in the market then after certain period of time, we have to add new features to the system by updating it and we this way we can trace those new software requirements. The used model for system development also helps us to find out the requirements.
In this way we can trace software requirements throughout the software lifecycle and in each phase. We can prioritize our requirements then high prioritized task can be performed first and also we can develop prototype to meet requirements. The used model for development process can also help us to find requirements as agile model helped us to add requirements later after completing one step.
Software Quality
Software quality is the method of assuring if the requirement of the business context and the customers are meet or not. It is the totality of functionality and features of a software product that bear on its ability to satisfy stated or implied needs. The software quality maintenance at the organization is the process of checking if the software is produced in the correct format or not and if it can compete with the similar software in the market or not. Maintaining the quality of
the software is a necessary factor because with this the actual work of the system and the facility to the customers will be provided. Measuring the quality of the software checks the risk, cost and all the factors that can affect on the development of the project. The quality assurance process of the software is the continuous and the ongoing process that is still not completed until the end of the software. Assuring the quality of the software is the set of the things that is necessary to be done for the quality of the products. The software quality approach helps in checking the weaknesses of the project and then with the identification of the weaknesses correcting those weaknesses continually to improve the quality of the software.
The way to provide high-quality software is to implement effective QA management that provides tools and methodologies for building bug-free products. Software quality management is an umbrella term covering three core aspects: quality assurance, quality control, and testing.
Software underpins nearly every business process. Therefore, it’s essential to lower the cost of testing and improve the quality of your software.
Below I have provided some approaches which helps us to improve software quality and improve testing efficiency.
1. Communication
To improve software quality, it is important that all parties to the project have full information through fluid communication channels. The important aspect of fluid communication is that all parties have the opportunity to provide feedback to the team to ensure that all expectations are being met. It is also important to keep all stakeholders in the loop and not isolate team members from the vendors or end user of the software. Isolation can mean that the project is delayed or may not deliver on the goals expected by senior management.
2. Review, revise, and remember
Producing software quality is not a coincidence. This is why we must always do the following three things which are review – Testing often is a pillar of ensuring software quality. It ensures that standards are continuously met and bugs, errors and distractions can be fixed before they spiral out of control. Revise shows what has worked throughout the software process. Utilize what is working and see if innovation can transcend our software quality even further. Remember When we deliver quality remember what worked well and did not work well. We have to keep an updated record of both the positives and negatives of any given project and turn to it frequently when we start the next project from scratch.
3. Extensive Unit Testing
Unit testing is the closest we can come to having peace of mind after deploying new code into our production environment. Unit tests should be written first and then used to ensure that our code satisfies the insurance company requirements, rather than vice versa. This is sometimes difficult for teams that have not worked with unit tests before to grasp, but it is a very important step.
Below I have discussed the two approaches that can be applied to our scenario to improve software quality.
1. Know the requirements
While developing the system for insurance company our software development team needs to be provided with a definition of what a quality outcome looks like and how it fits into the time, resource and budget constraints. If our development knows the requirements then carefully defining each phase of software development will lead to an overall improvement in the ability of the team by not over taxing the various resources with unrealistic expectations. As the system analyst I have to understand the importance and need of providing clear and concise business requirements so that our development team can execute those goals. Although it is difficult for us to go into too much detail here but giving developers poor outline of what’s required and expecting them to fill those gaps will push software development over the deadline and this might result in unhappy users and poor-quality software. As we are using Agile model for software development, we don’t have to do the proper documentation for knowing client’s requirements. We can always have the face to face interaction with clients and show them the ongoing work in development and take the quick feedback from them and change the features according to their requirements.
2. Implement quality controls & encourage innovations from beginning
If we wish to deliver the quality software then we have to start implementing the quality controls from the beginning which is an ongoing process throughout the deliver. Our team testers can monitor quality controls and create awareness in partnership with developers to ensure standards are continuously being met. If there is a good relationship between testers and developers then it can help the project software strategy develop effectively. Also, while developing software a systematic methodology in quality control can ensure that the coding errors and bugs are dealt with effectively, following a structured process. Although it is important in our software development to place testing structures and quality measures in their place, however we should also provide room for innovation. We can place innovation by automating testing where possible to minimize time spent on controls. Innovations are important because they can lead to improvements in software quality that have the capability to transform how projects are delivered also while developing software for insurance company research and development should be encouraged for such large projects. We can also empower teams to explore, experiment and investigate continuously and we can also ensure that advancements in innovation are duly rewarded. The team member have capacity to transcend ongoing software quality and deliver projects with a competitive advantage over today’s IT industry competition.
In this way we can improve the software quality by using above mentioned various approaches. If we are able to follow those approach effectively then surely we can deliver quality system to our clients. Software underpins nearly every business process. Therefore, it’s essential to lower the cost of testing and improve the quality of your software.
Function Design Paradigm
“Functional Design paradigm is a paradigm used to simplify the design of hardware and software devices such as computer software and increasingly, 3D models. A functional design assures that each modular part of a device has only one responsibility and performs that responsibility with the minimum of side effects on other parts. Functionally designed modules tend to have low coupling.” (Smith, 2018) Systems with functionally designed parts are easier to modify because each part does only what it claims to do. Since maintenance is more than 3/4 of a successful system's life, this feature is a crucial advantage. It also makes the system easier to understand and document, which simplifies training. The result is that the practical lifetime of a functional system is longer. In a system of programs, a functional module will be easier to reuse because it
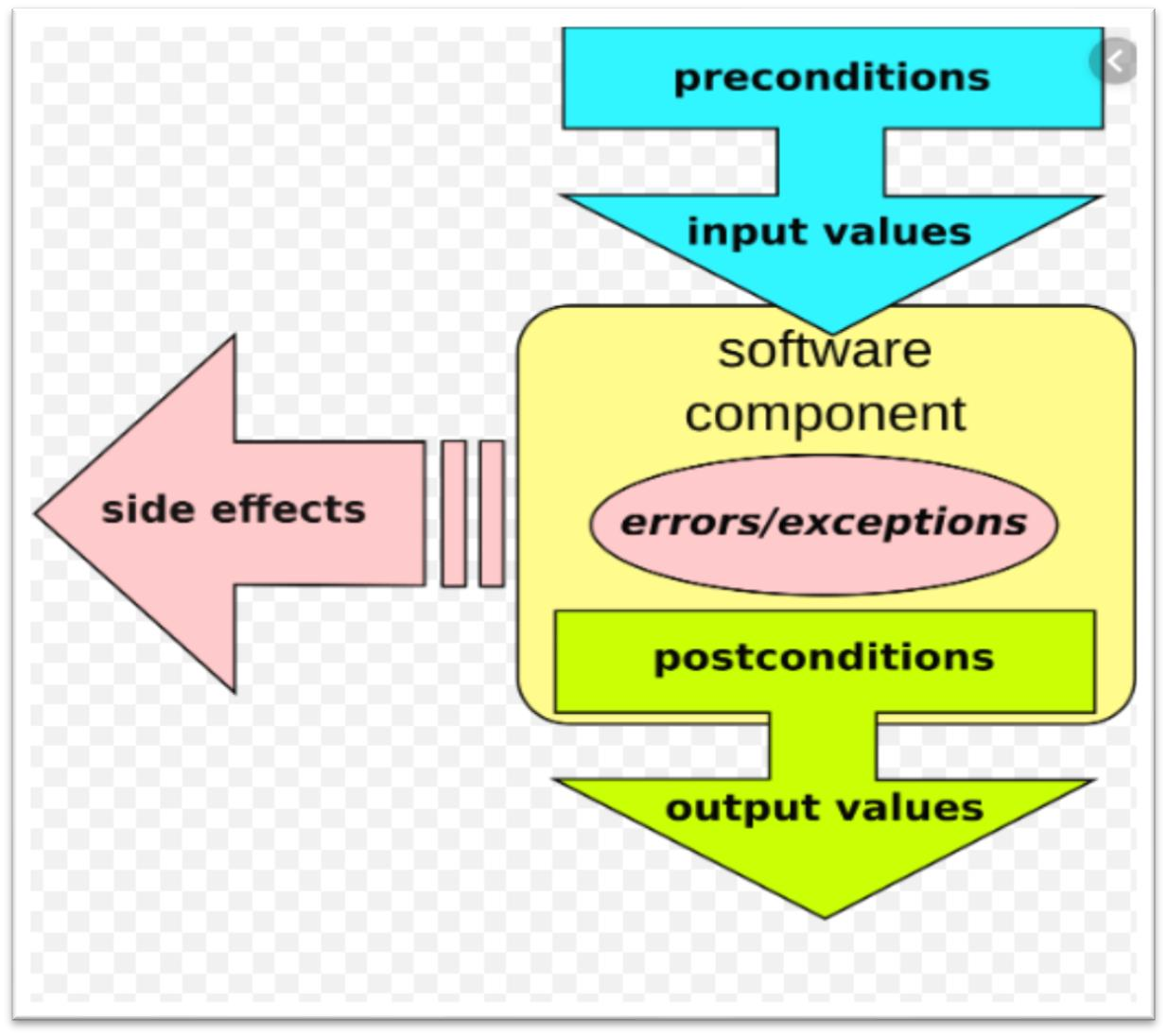
is less likely to have side effects that appear in other parts of the system.
It is the method that is been used to simplify the design of hardware and software devices such as computer software and graphic designing. It is also known as the formal documentation that is been created so as to describe in details about the software developers connecting the developers and the user interactions. Proper environment of the project along with the setup goals of the organization in an analytic phase of the each iteration, it helps in redefining and improves the characteristic that helps in the development of the quality software.
Previously I have stated how the software quality depends on various factors like reliability, usability, efficiency, security etc. Likewise those different factors software quality can also be increased by the functional design paradigms as it apply to the quality improvement process in the iterative manners. By using the functional design paradigm we were able to use effective methods, process and techniques to develop a quality software which is best suited for the current working environment of insurance company. It helped us to implement the best methodologies from the early stages to prevent the development from bugs and defects in system providing framework to the software. It also helped us to clearly understand the nature of company by examining the previous working system. For example while we were creating the new software for company the working environment of company’s previous system was examined and requirements of company were gathered which helped us in designing new software while maintaining quality and standard. While using functional design paradigm it was also easier to acknowledge the goals of the insurance company which helped us to associate with the reasons for creating quality software which fulfills objectives of the company. The function design paradigm also makes the sound execution of design, methods and techniques possible. It simplify the design of software and assures that each modular part perform their respective task and have low coupling.
The function design paradigm helps us to analyze the result generated from the execution of the designed modules and frameworks. We were able to examine whether the outcomes generated satisfy the needs of insurance company or not and we also evaluated the performance, stability, robustness and some other features of software that maintain the standard of software developed which resulted in development of quality software which our client required. Then after the evaluation of factors which determine quality of software we were also able to generate feedback where modification and improvements were needed. For the improvements in the software we removed all the defects, bugs and weakness to improve the software quality and make it robust.
Recommendation:
In this way we used the function design paradigm while developing the system for insurance company and the outcome was good. Using the function design paradigm in software development lifecycle we were able to improve the software quality. I even also recommend other software development companies and individuals to use this paradigm while developing system. It provides us various benefits like robustness, stability and overall it improves software quality which is ultimate goal of every software company.
Conclusion:
In this way to complete this part of assignment I undertake out the software investigation to meet the business need using appropriate software analysis techniques to carry out a software investigation and created a supporting documentation for this. I identified the stakeholders involved in the development of software and client’s requirements that needed to be addressed in the software. Also, described about the scope of the software and process descriptors on how software will work in output, input and process. Consideration of alternative solutions were developed in case of any emergency. Security and quality of the software defines the success of the software. So, I have described about the security that how we planned the security in the software and quality assurance that are made in the software for the betterment of the software. For the software analysis I developed the data flow diagram upto second level and also created entity relationship diagram which helped us for the clear understanding of the software. Different constraints were listed out such as costs and organizational policy. I also find out the reason behind developing a new system and problem that insurance company had in their old system. I went through a data collection process where me and my team members held workshop, formed groups, took an interview and asked questions to the head of the insurance company. Then I have discussed various approaches to improve the software quality and briefly discussed two approaches which can be applied to the given scenario.
- Reference to your task above that required some level of intensive research work analyze how software requirements can be traced throughout the software lifecycle.
- Discuss different approaches to improve the software quality and considering the above context discusses the two approaches that can be applied at this context to improve the software quality.
- Critically evaluate how the use of the function design paradigm in the software development lifecycle can improve the software quality. Support your ideas with reference to the tasks you have done.
- Prepare a documentation that explains how user and software requirements have been addressed. You may tabulate this task with the columns that has the expected client requirements and the actual output of the product to be developed after the appropriate analysis.
- Discuss about the different software specification methods and suggest two software behavioral specification methods and illustrate their use with an example relevant to the project that needs to be constructed for the given context. Some of the software specification techniques include flowcharts, pseudo code and formal specification methods and so on.
“User requirements, often referred to as user needs, describe what the user does with the system, such as what activities that users must be able to perform. User requirements are generally documented in a User Requirements Document (URD) using narrative text. User requirements are generally signed off by the user and used as the primary input for creating system requirements. System requirements are the building blocks developers use to build the system. These are the traditional “shall” statements that describe what the system “shall do.” System requirements are classified as either functional or supplemental requirements. A functional requirement specifies something that a user needs to perform their work.” (Parker, 2012) In our project of developing software for insurance company it was very important for us to address user and software requirements. Without the user and software requirements it was impossible for us to develop the required software for insurance company. The best way to know the user requirements was documentation. As we were using the agile model for software development rather than documentation at first, we arranged face to face interaction and meeting with clients to know their requirements which helped us to include their all requirements and develop quality software.
Developing the new system for such large company with inclusion of all its features and user requirements is very difficult project to do. When the project was first given to our company then first of all we decided to perform the feasibility study on this new system development and when the report of study was good then we dived into the software development process. To develop any system first we need to choose the best system development model and be clear about the reasons for choosing that specific model in this project. We analyzed the various system development models like Waterfall, Agile, Prototype, Spiral etc. After knowing the pros and cons of each model we gathered the overview from all the project members and decided to go with Agile model in this project. Then for the initial planning phase we went through the feasibility study report and hired the new required developers, technologies, software etc. As in the agile model we don’t have to do documentation and we can add new requirements from clients whenever we have to so after the proper planning of project, we moved toward the next step which is designing phase.
We designed the Data Flow Diagram and ER-Diagram for the system which helped the developer and any other project member to get overview of whole system and how it will work once it is completed and what are the user requirements from this system. We hired the top designer from our country to design the system layout using various technologies and after the completion of this step we moved toward the development phase. In the development phase we used top technologies and popular language for proper coding to make the software fast, reliable and accurate. We also used various technologies to provide security for the software using firewall and popular web hosting company. Different constraints were listed out such as costs and organizational policy. We also find out the reason behind developing a new system and problem that insurance company had in their old system. We went through a data collection process where we held workshop, formed groups, took an interview and asked questions to the head of the insurance company. We tried our best for the quality assurance of system and for this we used highly skilled developer, testing in each phase, proper documentation although it is not highly required in agile model. We also arranged face to face client interaction so that we can show them the ongoing process in system development and get quick feedback which helped us to increase the software quality.
After that for knowing the software requirements we prioritize the tasks and according to priority high prioritize tasks were done first and low prioritize later which helped us to trace software requirements. Although every development model says that we have to state the software requirements in the initial stage of development stage but we can also trace the software requirements throughout the software lifecycle. In software development models like Agile there is no proper client requirements documentation required so we can easily add new requirements whenever we want. Used model also helped us to find requirements as in agile model we can add requirements whenever clients ask for new requirements in software. Moreover, below I have tabulated this task with columns with the expected client requirement and actual output of the product: -
Software Behavior Specification:
“A technique for software system behavior specification appropriate for use in designing systems with concurrency is presented. The technique is based upon a generalized ability to define events, or significant occurrences in a software system, and then indicate whatever constraints the designer might wish to see imposed upon the ordering or simultaneity of those events. The relationship of this technique to other behavior specification techniques is also discussed in software behavior specification. “Any piece of computer software, coded in some programming language, implicitly specifies the behavior which it will produce when run on a given computing system with a given set of input data. Such a program defined behavior specification.” (Wileden, 2015) It is the comprehensive description of the purpose and the environment of the software under the development phases. The software specification helps in meeting the requirement of the clients and makes the project better. The main purpose of software specification is to minimize the time and effort that is required by the developers to achieve the desired goals and also minimizes the cost of the development.
Importance of Software Behavior Specification In SDLC:
A software behavior specification is literally the conversation of a specific point. It's difficult in this instance to avoid the circular reference. A project's specifications consist of the body of information that should guide the project developers, engineers, and designers through the work of creating the software. A software behavior specification document describes how something is supposed to be done and describes the step by step working process of the software. A specifications document may list out all of the possible error states for a certain form, along with all of the error messages that should be displayed. The specifications may also describe the steps of any functional interaction, and the order in which they should be followed by the user. A requirements document, on the other hand, would state that the software must handle error states reasonably and effectively, and provide explicit feedback to the users. The specifications show how to meet this requirement. Specifications may take several forms. They can be a straightforward listing of functional attributes, they can be diagrams or schematics of functional relationships or flow logic, or they can occupy some middle ground. Software requirements specifications can also be in the form of prototypes, mockups, and models. Project specifications are much more important for determining the quality of the product. Every rule and functional relationship provide a test point. A disparity between the program and its specification is an error in the program if and only if the software requirement specification exists and is correct. A program that follows a terrible specification perfectly is terrible, not perfect. This is how software behavior specification is important and plays a vital role in the development of the software. Below I have provided two different software specifications methods: -
Flowchart
“A flowchart is a formalized graphic representation of a logic sequence, work or manufacturing process, organization chart, or similar formalized structure. The purpose of a flow chart is to provide people with a common language or reference point when dealing with a project or process. . Each step in the process is represented by a different symbol and contains a short description of the process step.” (nicholas, 2015) They are the methodology used to analyze, improve, document and manage a process or program. Flowcharts are helpful for collecting data about particular process, helping with decision making, measuring the performance of a process, tracking the process flow, highlighting important steps and elimination the unnecessary steps etc.
Flowcharts are commonly used in developing business plans, designing algorithms and determining troubleshooting steps. Many software programs are available to design flowcharts. There are various benefits of using flowcharts in software development. While developing our software for insurance company also we created flowcharts for the better way of communicating the logic of system to all concerned or involved. For example flowchart made the communication easier to all the people involved in system development as compared to actual program code because all of them like project managers, other staff weren’t aware of all programming techniques and languages. It helped us in effective analysis, with the help of flowchart problem can be analyse in more effective way therefore it reduces cost and wastage of time. It helped us in proper documentation as the program flowcharts serve as good program documentation which is needed for various purposes, making things more efficient. It also helped our development team for efficient coding & debugging. The flowchart acted as a guide while we were doing system analysis and program development and also in debugging process. While we have to do the maintenance in future it becomes easy with the help of flowchart and helps programmer to put efforts more efficiently on that part.
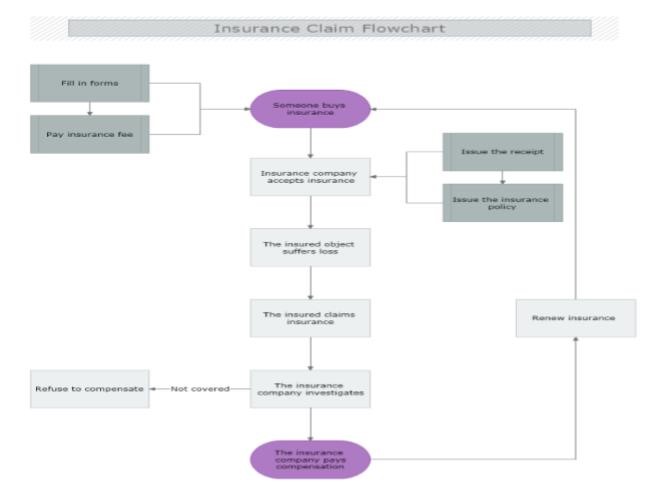
Pseudocode:
Pseudocode is an informal way of programming description that does not require any strict programming language syntax or underlying technology considerations. It is used for creating an outline or a rough draft of a program. Pseudocode summarizes a program’s flow, but excludes underlying details. System designers write pseudocode to ensure that programmers understand a software project's requirements and align code accordingly. Pseudocode makes creating programs easier. Programs can be complex and long; preparation is the key. For years, flowcharts were used to map out programs before writing one line of code in a language. However, they were difficult to modify and with the advancement of programming languages, it was difficult to display all parts of a program with a flowchart. It is challenging to find a mistake without understanding the complete flow of a program. That is where pseudocode becomes more appealing.
Pseudocode is not an actual programming language. So it cannot be compiled into an executable program. It uses short terms or simple English language syntaxes to write code for programs before it is actually converted into a specific programming language. This is done to identify top level flow errors, and understand the programming data flows that the final program is going to use. This definitely helps save time during actual programming as conceptual errors have been already corrected. Firstly, program description and functionality is gathered and then pseudocode is used to create statements to achieve the required results for a program. Detailed pseudocode is inspected and verified by the designer’s team or programmers to match design specifications. Catching errors or wrong program flow at the pseudocode stage is beneficial for development as it is less costly than catching them later. Once the pseudocode is accepted by the team, it is rewritten using the vocabulary and syntax of a programming language. The purpose of using pseudocode is an efficient key principle of an algorithm. It is used in planning an algorithm with sketching out the structure of the program before the actual coding takes place.
Advantages of Pseudocode
- Pseudocode is understood by the programmers of all types.
- It enables the programmer to concentrate only on the algorithm part of the code development.
- It cannot be compiled into an executable program. Example, Java code: if (i < 10) { i
++; } pseudocode :if i is less than 10, increment i by 1.
Below I have provided the pseudocode for the Insurance Company Software: -
Finite State Machine:
“In general, a state machine is any device that stores the status of something at a given time and can operate on input to change the status and/or cause an action or output to take place for any given change. A computer is basically a state machine and each machine instruction is input that changes one or more states and may cause other actions to take place. Each computer's data register stores a state. The read-only memory from which a boot program is loaded stores a state (the boot program itself is an initial state).” (Rouse, 2018) The operating system is itself a state and each application that runs begins with some initial state that may change as it begins to handle input. Thus, at any moment in time, a computer system can be seen as a very complex set of states and each program in it as a state machine. In practice, however, state machines are used to develop and describe specific device or program interactions.
A deterministic finite automaton (DFA) is described by a five-element tuple Q = a finite set of states
∑= a finite, nonempty input alphabet ∂ = a series of transition functions q₀ = the starting state
F= the set of accepting states or final states
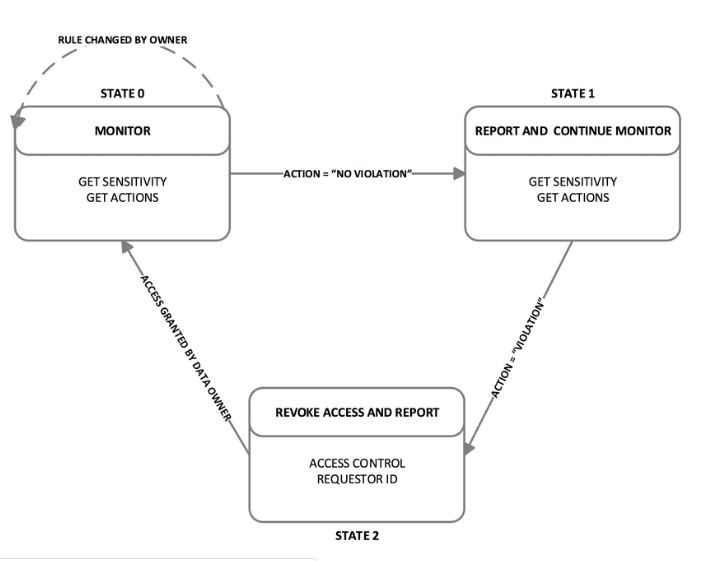
Advantages of Finite State Machine
The advantages of Finite State Machine include the following.
- Finite state machines are flexible
- Easy to move from a significant abstract to a code execution
- Low processor overhead
- Easy determination of reachability of a state
Disadvantages of Finite State Machine
The disadvantages of the finite state machine include the following
- The expected character of deterministic finite state machines can be not needed in some areas like computer games
- The implementation of huge systems using FSM is hard for managing without any idea of design.
- Not applicable for all domains
- The orders of state conversions are inflexible.
(Anon., n.d.)
Extended Finite State Machine:
“In an extended finite state machine (EFSM) model, the transition can be expressed by an “if statement” consisting of a set of trigger conditions. If trigger conditions are all satisfied, the transition is fired, bringing the machine from the current state to the next state and performing the specified data operations.” (Vyatkin, 2015) Extended Finite State Machines provide a powerful model for the derivation of functional tests for software systems and protocols. Many EFSM based testing problems, such as mutation testing, fault diagnosis, and test derivation involve the derivation of input sequences that distinguish configurations of a given EFSM specification. a method is proposed for the derivation of a distinguishing sequence for two explicitly given or symbolically represented, possibly infinite, sets of EFSM configurations using a corresponding FSM abstraction that is derived based on finite sets of predicates and parameterized inputs. An abstraction function that maps configurations and transitions of the EFSM specification to corresponding abstract states and transitions of the abstraction FSM is proposed. Properties of the abstraction are established along with a discussion on a proper selection of the sets of predicates and parameterized inputs used while deriving an FSM abstraction. If no distinguishing sequence is found for the current FSM abstraction, then a refined abstraction is constructed by extending the sets of predicates and parameterized inputs.
The cycle behavior of an EFSM can be divided into three steps:
- In E-block, evaluate all trigger conditions.
- In FSM-block, compute the next state & the signals controlling A-block.
- In A-block, perform the necessary data operations and data movements.
An EFSM is defined as a 7-tuple where:
- S is a set of symbolic states
- I is a set of input symbols
- O is a set of output symbols
- D is an n-dimensional linear space D1 *…. * Dn
- F is a set of enabling functions Fi: D = {0, 1}
- U is a set of update functions ui: D=D
- T is a transition relation, T: S * F * I =S * U * O
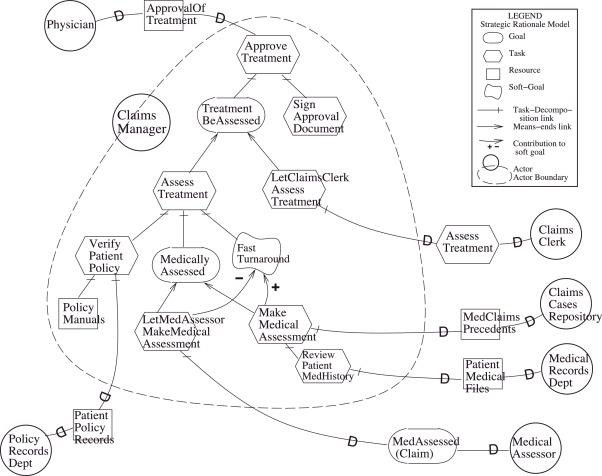
Differentiate between a finite state machine (FSM) and an extended Finite State providing an application for both.
Data Driven Software
In computer programming, data-driven programming is a programming paradigm in which the program statements describe the data to be matched and the processing required rather than defining a sequence of steps to be taken. Data driven software is one that automates process, problems and opportunities. In data driven software, data is the centric part of the architecture and all the remaining parts are designed around this (Norm, 2012) The application that is been created by the data driven approach are more user friendly, communicative and allows users to perform various of the tasks. The main application of the data driven software is it helps in the exploring of the data relationships, exporting or importing of the files, browsing and filtering large sets of data and interacting with other systems. The data driven software helps to establish patterns between operational and business activities. The decisions made by the data driven software is suitable and productive. Data driven application are the applications where application flow is governed by data it processes. Input data set can change the behavior of your application. Programming logic will remain same but it will be coded in such a way that input changes the way application behaves. These applications require lots of testing as it produces error when data issues are encountered.
Nowadays the use of data driven software is increasing as this type of software provides us the result based upon the provided facts. Along with the objectoriented design the data driven design has argued to lead to bad object-oriented design especially when dealing with the more abstract data. It is quite easy because the functionality in the data driven simply required to know the abstract data type of the variables it is working with. The traditional software of the insurance company is going to be replaced by the new system that contains a lot of the facilities along with the capturing With the use of data driven software all the difficulties and issues present due to the old traditional system can be eliminated completely and help to make reliable and rational decisions. Similarly, the availability of data driven testing help to enhance the reliability and effectiveness of the software. The data driven testing test methods, describes increased capability in identifying bugs, and can effectively ensure product quality with legitimate inputs that further assures thriving deployment. The data driven software automates processes, problems and opportunities and allows end to end optimization for the software where in necessary steps and process can be eliminated to optimize the performance and efficiency in any process. It also helps in the logistics storage and distribution that excel the performance of the software and ultimately enhance its effectiveness. It operates on diverse data from many multichannel data sources and seamlessly combines operational and analytic capabilities.
We can find out the statistics using the data driven software. For example, if we want to know about the IT industry scope in our country then we can use data driven software for finding results in this. This software relies upon the data and finds out the desired results for us based upon the available data. Another example of data driven software is telecom. When we call someone and talk to him for 1 hour then based upon the talk time data the result i.e. the bill is published. The data driven software only works when there is proper data available if not then this software doesn’t function properly. For the software to be reliable and effective it should always provide us the right decision if any software displays the wrong decisions or wrong message then that software can’t be called reliable. The data driven software relies upon the data and provides us the right decision only so in this way data driven software can improve the reliability and effectiveness of the software.
Conclusion
In this way I have completed the final part of my assignment by preparing the documentation which explains how user and software requirements have been addressed. In our project of developing software for insurance company it was very important for us to address user and software requirements. Without the user and software requirements it was impossible for us to develop the required software for insurance company. The best way to know the user requirements was documentation. Then I discussed about the software specification methods and recommended two software specification methods. A software behavior specification document describes how something is supposed to be done and describes the step by step working process of the software. A specifications document may list out all of the possible error states for a certain form, along with all of the error messages that should be displayed. Project Management Information System ,then I differentiated about finite state machine and extended finite state machine and at last I provided justifications with example on how data driven software can improve the reliability and effectiveness of the software.
There are no sources in the current document.

Business Management
Writing & Compare Documents
Computer Science
Teaching and Learning
Personal Development
Research Topics
Recruitment
NVQ Level 3 Diploma in health & social care
Digital Marketing
Assignment Writing
International
Speech Topics
Travel And Lifestyle
Project Proposals
Your Feedback matters
- Mindset Network
- Introduction to Software
- Information Technology
- System Software
- Basic concepts of computing
- Data and Information Management
- Introduction to Algorithms
- Introduction to Using a Graphical Programming Tool
- Software Engineering Principles
- Communications Technology - Electronic Communications
- Communications Technology - Networks
- Computer Management
- Internet Technologies - Internet and WWW
- Social Implications
- Electronic Communications
- Software Engineering Principles and Problem solving
- Application Development using a high-level programming language
- Database Management
- Internet and the WWW
- Internet Services Technologies
- Database design concepts
Disabled Feature
This functionality is only active if you sign-in with your Google account.
Related Resources

Connect with social media
Sign in with your email address.
- Create new account
- Request new password
Comeau Software Solutions
Making sense of technology since 2000.
Software Development: A First Assignment
Passion vs. profit.
My first programming experience was in 1985 on an old TRS-80 color computer that was sitting unused in one of my high school classrooms. I had a lot of free time in that class so I decided to pick up the manual and start learning.
I knew right away that I’d found something special because my brain wouldn’t let go of it. Even away from the computer and after school, I kept thinking of different things I could try with the BASIC language and its commands. When I’d see other subjects in some of my classes or in real life, I thought about how I could turn them into code with the very limited programming knowledge I had at the time. This happy little obsession continued in college where I spent hours after school on my own independent projects.

It was several years before I actually did any programming for money but that early enthusiasm guaranteed that I would eventually find a way. I still believe that lasting software careers start with a love of software creation and a desire to explore the possibilities rather than a desire for a paycheck. If you’re going to spend your days hacking away at programming languages, trying to coax the necessary behavior from the CPU and accepting all that comes with it, including ridiculous project timelines, inadequate specifications and all the politics and nonsense that go with any career, there better be something about it that you truly love or else you will be miserable.
No salary or benefits package on its own will be worth it otherwise; trust me. I’ve had people tell me that I’m not really working because programming is something I do for fun but I remind them that companies and clients are very adept at turning that activity into work.
Beyond enduring the inevitable downsides of a career, a love for the work affects the level of craftsmanship and that word applies as much to software as it does to furniture or cars. People will naturally tend to excel at work that brings them happiness; the innovation and attention to detail will be there. Some of the best programmers I’ve worked with and learned from did not have college degrees or at least did not go to school for programming. When one of my mentors was asked how he learned so much about programming, he said “I just f*** around with things.”
While companies like to list degree requirements in their job listings, often for salaries that don’t justify those qualifications, programmers are still fortunate to be able to find work without a degree if they have the initiative to self-train and promote their skills. Bootcamps are also a well-known option but they tend to be very expensive and are not a substitute for the enthusiasm that will enable someone to use and maintain those new programming skills after the class time is over. Ultimately, it’s up to the individual to build their own portfolio of skills that will sustain them through technology changes and job transitions. That’s another aspect of the career that’s more likely to happen if there’s a passion for the work.

Unfortunately, as programming jobs have become associated with six-figure salaries in the U.S., that idea can get lost in the gold rush of students wanting a quick path to those salaries. The idea of developing personal projects, even small ones, is sometimes dismissed by new programmers who would rather just do interview prep and grind Leetcode to help them pass the job interview and get on board.
I even fell into a similar trap when I was teaching a college class in programming and promoting it to future students. At that time, the easiest 10-minute message to present to a group of bored high schoolers was about the earning potential of a career in software development, so as to at least hook their interest. I’m happy to say that the class itself was an intense workshop experience with multiple projects that the students had to complete to develop their skills. Unfortunately, that also contributed to a high washout rate which didn’t please the vocational career school whose business model depended on maintaining admission, graduation and placement numbers.
I don’t know that there’s a solution to the commoditization of programming jobs and the focus on profit before professional excellence. If such classic professions as medicine and law couldn’t escape that trend, then such a young profession as software development in which so much money is involved can’t be immune to it. I can only trust that the sheer availability of free programming knowledge and materials will continue to draw in new enthusiasts who pursue it for the excitement of seeing the logic come together and that some of them will realize, as one of my students did, that code can be beautiful.
I do know that if I was trying to recruit more students and help them appreciate the profession, I wouldn’t even mention the salaries involved. Instead, I would help them experience the same shift in perspective that I did when I first learned to code.
The Assignment
Computer programming could be viewed as a form of education as well as engineering; the programmer is teaching the computer to perform specific tasks. The computer has no actual intelligence, only the ability to instantly respond to any programming language that can be installed on it. It’s up to the programmer to learn and use a language well enough to provide the best instructions to the computer. The more talent and experience the programmer has in communicating with the machine, the better results they will get.
The obstacle that comes before that is the developer’s own understanding of the process and their ability to break that process down into steps and terms that can be communicated. It’s really the same basic challenge that anyone faces when they try to instruct someone else.
For a first programming challenge, I ask you to consider how you would teach the computer to simulate a tree.
Imagine that you are responsible for creating a hyper-realistic simulation of this tree that duplicates its behavior and lifespan as closely as possible.

Programming is actually a misleading term here because I’m going to tell you to forget about the code for now. Don’t worry about what language you will use; we’re not anywhere near there yet.
Forget about the way the tree looks, too; the computer can’t see it and wouldn’t know what to do with the image if it could. When I say simulation, I’m not talking about graphics or animation on a screen because that’s only an outward perception, anyway. This simulation will focus on the tree’s function and measurable effects on its surroundings. Once that’s in place, any type of graphics can be used to represent it.
What the computer needs is your understanding of the tree itself. In reality, the tree is an immensely complex system with processes that happen down to the microscopic level. The more of these fine details you are able to understand and communicate to the computer, the more accurate the simulation will be.
Writing the code is actually one of the last steps in creating software. The first is to gather the requirements for the software and the rules of the system in which it will operate.
Just like software might need user input or a connection to the Internet, this tree has inputs and dependencies including sunlight and soil nutrients. It has resulting outputs such as oxygen and the leaves that it might return to the environment every year, depending on its type.

Just like software, the tree has users that depend on its presence and those users have specific requirements of the tree just as business users require specific operations from a software system. Users from birds to moss might depend on the tree for food or shelter. Humans and animals might harvest its fruit. In this case, if the tree is unable to meet those requirements, the users will move on like clients who are no longer satisfied with a product.
A computer program is often made up of specific functions and routines that run in sequence, on schedule or in response to user actions. The tree has functions like photosynthesis where it continuously processes the CO2, water and sunlight to sustain itself and release oxygen. Again, these functions depend on chemical reactions that happen on the microscopic level. In your simulation, you could choose to approximate the rates of input and output based on observation of actual trees and the known science but the more fine details you give the program, the more accurate the simulation will be. The more information it has on the biology of the tree and its chemical interactions, the more likely it will be to go beyond the current understanding and actually suggest new possibilities about the nature of the tree itself.

As one more example, software often has events in which it responds to certain conditions by notifying other parts of the program or even outside programs and possibly making information available. The code that receives these notifications is sometimes called a subscriber . The lifecycle of the tree also has a number of events that could be coded from the germination of a seed to the sprouting of leaves and production of fruit or flowers that give off scent which then notifies other life within the greater system that the tree inhabits. Even the death of the tree is an event that has effects on the surrounding environment and this would need to be taken into account within the simulation.
Software has requirements that must be established before programming can begin and that means asking the right questions, sometimes repeatedly. For the tree and its simulation, there’s also a list of requirements.
- How much sunlight and water does the tree require? What the best climate for its growth?
- Should the leaves change color with the seasons?
- Does the tree produce any chemicals or other defenses against pests?
- How resilient should the tree be to a change in climate or to pollutants within the soil?
Trees, like software programs, often have to interact with each other and other parts of the larger system. This means more questions.
- Can the tree compensate for lower light levels caused by crowding by other trees?
- Does the tree spread its seeds on the wind or are they activated by a forest fire or spread by animals?
- If a tree succumbs to disease, is that a design issue or a necessary interaction? How likely are different specimens of the tree to resist the disease and reproduce?

Software development requires understanding systems both large and small. Whether you’re working for a company or a client, you need to understand the customer’s requirements and needs and account for the realities of the environment where the software will run. Successful software adapts to the needs of the users and environment, not the other way around.
To understand a process well enough to explain it to another is to educate yourself. Once you ask enough questions to gain that understanding, you’ll never see that process the same again. Your perspective is forever changed because you have learned to understand it well enough to recreate some part of it, if only in someone else’s mind or the computer’s memory. You take that new perspective with you from every project.
I guarantee, the next time you look at a tree, you’ll see something new.
If you’re still wondering about the code itself, I’ll give you a hint. Trees, like humans, have chromosomes and genes that determine traits. This can be considered an example of design architecture , the structure that determines how information and instructions are stored and made available. Of course, with architecture, opinions differ as to what type works best and software developers are as opinionated as anyone else.
Find related products on Amazon.com ...

Python Programming for Beginners Made Easy: Learn the Essentials in 7 Days and Fast-Track Your Path to a Coding Job with Easy Tutorials and Hands-On Projects

Art of Computer Programming, The, Volumes 1-4B, Boxed Set (Art of Computer Programming, 1-4)
Assignment on Computer Software
> A program is a sequence of instructions written in a language, which can be understood by a computer.
> A software package is a group of programs, which solve a specific problem, or perform a specific type of job.
Glocal24.com
Assignment on strategies for building voip networks, apple branding strategy, splashdown following a historic moon mission, nasa’s orion returns to earth, ex-apple employee takes face id privacy complaint to europe, islam: historical overview, bitcoin briefly breaks the $50,000 barrier as coinbase’s direct listing looms, days of music: johannes brahms and peter ilyich tchaikovsky, molecular engineering, latest post, spark-gap transmitter, how an ignition coil works, new detecting system tries to warn about landslides and tsunamis, root microorganisms might be the key to a better tasting cup of tea, urban climatology.
for Education
- Google Classroom
- Google Workspace Admin
- Google Cloud
Easily distribute, analyze, and grade student work with Assignments for your LMS
Assignments is an application for your learning management system (LMS). It helps educators save time grading and guides students to turn in their best work with originality reports — all through the collaborative power of Google Workspace for Education.
- Get started
- Explore originality reports
Bring your favorite tools together within your LMS
Make Google Docs and Google Drive compatible with your LMS
Simplify assignment management with user-friendly Google Workspace productivity tools
Built with the latest Learning Tools Interoperability (LTI) standards for robust security and easy installation in your LMS
Save time distributing and grading classwork
Distribute personalized copies of Google Drive templates and worksheets to students
Grade consistently and transparently with rubrics integrated into student work
Add rich feedback faster using the customizable comment bank
Examine student work to ensure authenticity
Compare student work against hundreds of billions of web pages and over 40 million books with originality reports
Make student-to-student comparisons on your domain-owned repository of past submissions when you sign up for the Teaching and Learning Upgrade or Google Workspace for Education Plus
Allow students to scan their own work for recommended citations up to three times
Trust in high security standards
Protect student privacy — data is owned and managed solely by you and your students
Provide an ad-free experience for all your users
Compatible with LTI version 1.1 or higher and meets rigorous compliance standards
Product demos
Experience google workspace for education in action. explore premium features in detail via step-by-step demos to get a feel for how they work in the classroom..
“Assignments enable faculty to save time on the mundane parts of grading and...spend more time on providing more personalized and relevant feedback to students.” Benjamin Hommerding , Technology Innovationist, St. Norbert College
Classroom users get the best of Assignments built-in
Find all of the same features of Assignments in your existing Classroom environment
- Learn more about Classroom
Explore resources to get up and running
Discover helpful resources to get up to speed on using Assignments and find answers to commonly asked questions.
- Visit Help Center
Get a quick overview of Assignments to help Educators learn how they can use it in their classrooms.
- Download overview
Get started guide
Start using Assignments in your courses with this step-by-step guide for instructors.
- Download guide
Teacher Center Assignments resources
Find educator tools and resources to get started with Assignments.
- Visit Teacher Center
How to use Assignments within your LMS
Watch this brief video on how Educators can use Assignments.
- Watch video
Turn on Assignments in your LMS
Contact your institution’s administrator to turn on Assignments within your LMS.
- Admin setup
Explore a suite of tools for your classroom with Google Workspace for Education
You're now viewing content for a different region..
For content more relevant to your region, we suggest:
Sign up here for updates, insights, resources, and more.
Never forget a class or assignment again.
Unlock your potential and manage your classes, tasks and exams with mystudylife- the world's #1 student planner and school organizer app..
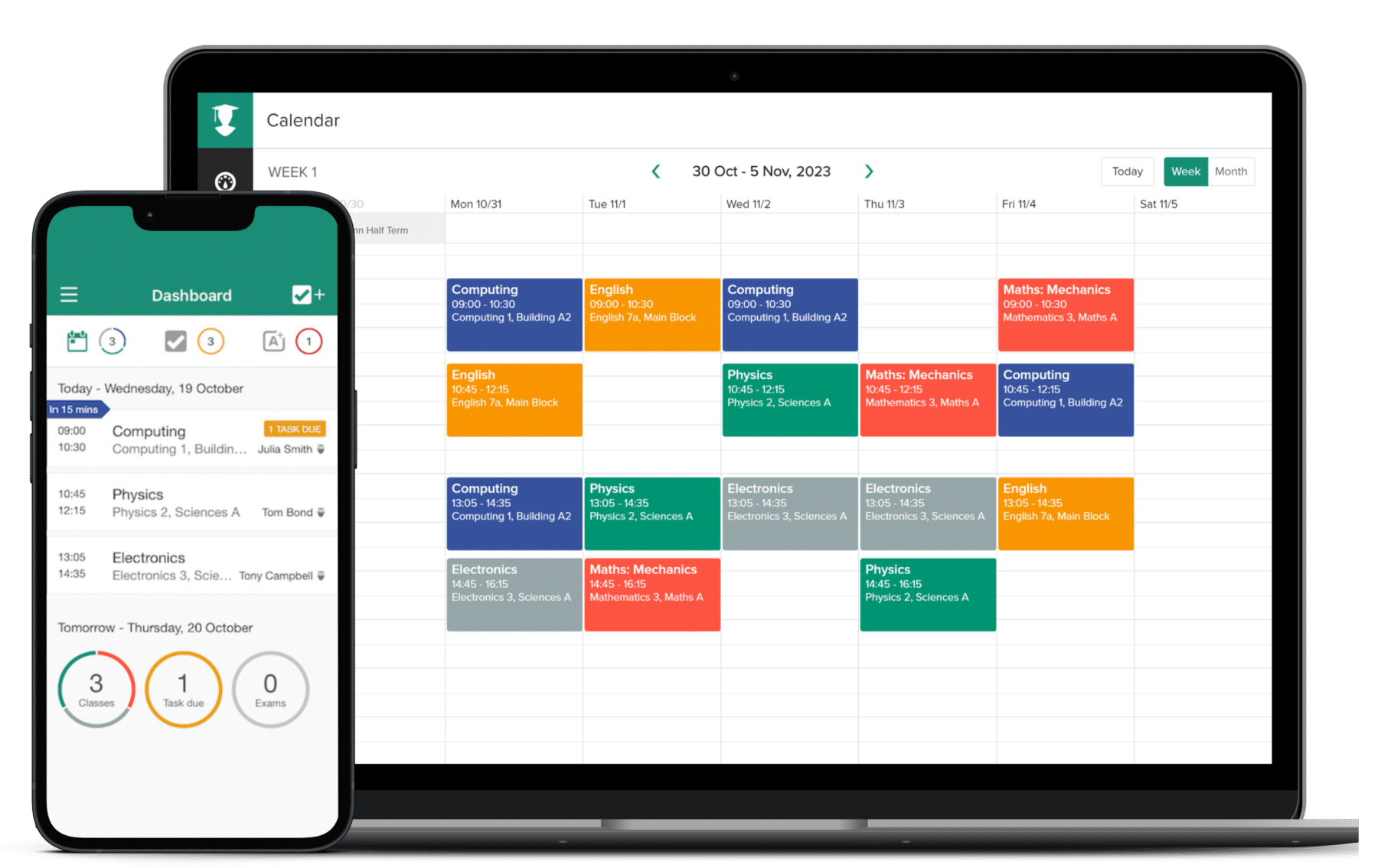
School planner and organizer
The MyStudyLife planner app supports rotation schedules, as well as traditional weekly schedules. MSL allows you to enter your school subjects, organize your workload, and enter information about your classes – all so you can effortlessly keep on track of your school calendar.
Homework planner and task tracker
Become a master of task management by tracking every single task with our online planner – no matter how big or small.
Stay on top of your workload by receiving notifications of upcoming classes, assignments or exams, as well as incomplete tasks, on all your devices.

“Featuring a clean interface, MyStudyLife offers a comprehensive palette of schedules, timetables and personalized notifications that sync across multiple devices.”
” My Study Life is a calendar app designed specifically for students. As well as showing you your weekly timetable– with support for rotations – you can add exams, essay deadlines and reminders, and keep a list of all the tasks you need to complete. It also works on the web, so you can log in and check your schedule from any device.”
“MyStudyLife is a great study planner app that makes it simple for students to add assignments, classes, and tests to a standard weekly schedule.”
“I cannot recommend this platform enough. My Study Life is the perfect online planner to keep track of your classes and assignments. I like to use both the website and the mobile app so I can use it on my phone and computer! I do not go a single day without using this platform–go check it out!!”
“Staying organized is a critical part of being a disciplined student, and the MyStudyLife app is an excellent organizer.”

The ultimate study app
The MyStudyLife student planner helps you keep track of all your classes, tasks, assignments and exams – anywhere, on any device.
Whether you’re in middle school, high school or college MyStudyLife’s online school agenda will organize your school life for you for less stress, more productivity, and ultimately, better grades.

Take control of your day with MyStudyLife

Stay on top of your studies. Organize tasks, set reminders, and get better grades, one day at a time.

We get it- student life can be busy. Start each day with the confidence that nothing important will be forgotten, so that you can stay focused and get more done.

Track your class schedule on your phone or computer, online or offline, so that you always know where you’re meant to be.

Shift your focus back to your goals, knowing that MyStudyLife has your back with timely reminders that make success the main event of your day

Say goodbye to last minute stress with MyStudyLife’s homework planner to make procrastination a thing of the past.

Coming soon!
MyStudyLife has lots of exciting changes and features in the works. Stay tuned!
Stay on track on all of your devices.
All your tasks are automatically synced across all your devices, instantly.
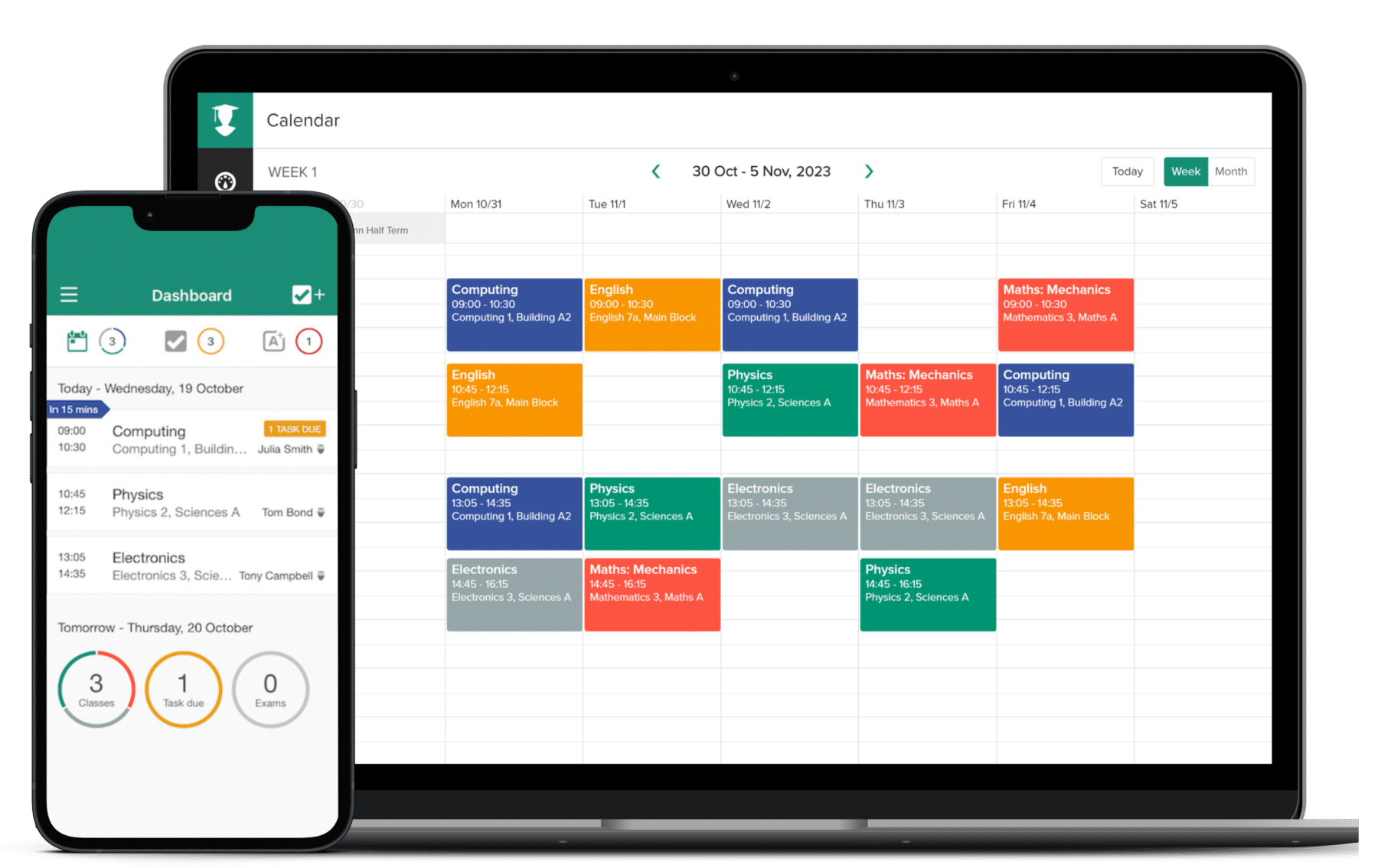
Trusted by millions of students around the world.

School can be hard. MyStudyLife makes it easier.
Our easy-to-use online study planner app is available on the App Store, the Google Play Store and can be used on desktop. This means that you can use MyStudyLife anywhere and on any device.
Discover more on the MyStudyLife blog
See how MyStudyLife can help organize your life.

JEE Main 2024: Best Tips, Study Plan & Timetable
Las 10 mejores apps gratis para estudiar mejor en 2024 , filter by category.
- Career Planning
- High School Tips and Tricks
- Productivity
- Spanish/Español
- Student News
- University Advice
- Using MyStudyLife
Hit enter to search or ESC to close

- App Templates
- Assignment Management
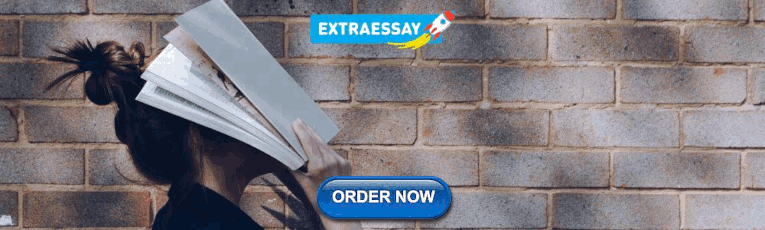
Make assignment management simple using our assignment tracker software
Assignments are one of the best ways to track the progress of both students and teachers. Students can put their understanding to the test, while teachers can assess how well their lessons are being received. This assignment tracker management software eliminates the drudgery that comes with grading, and makes creating assignments a breeze.
Start your 15-day free trial
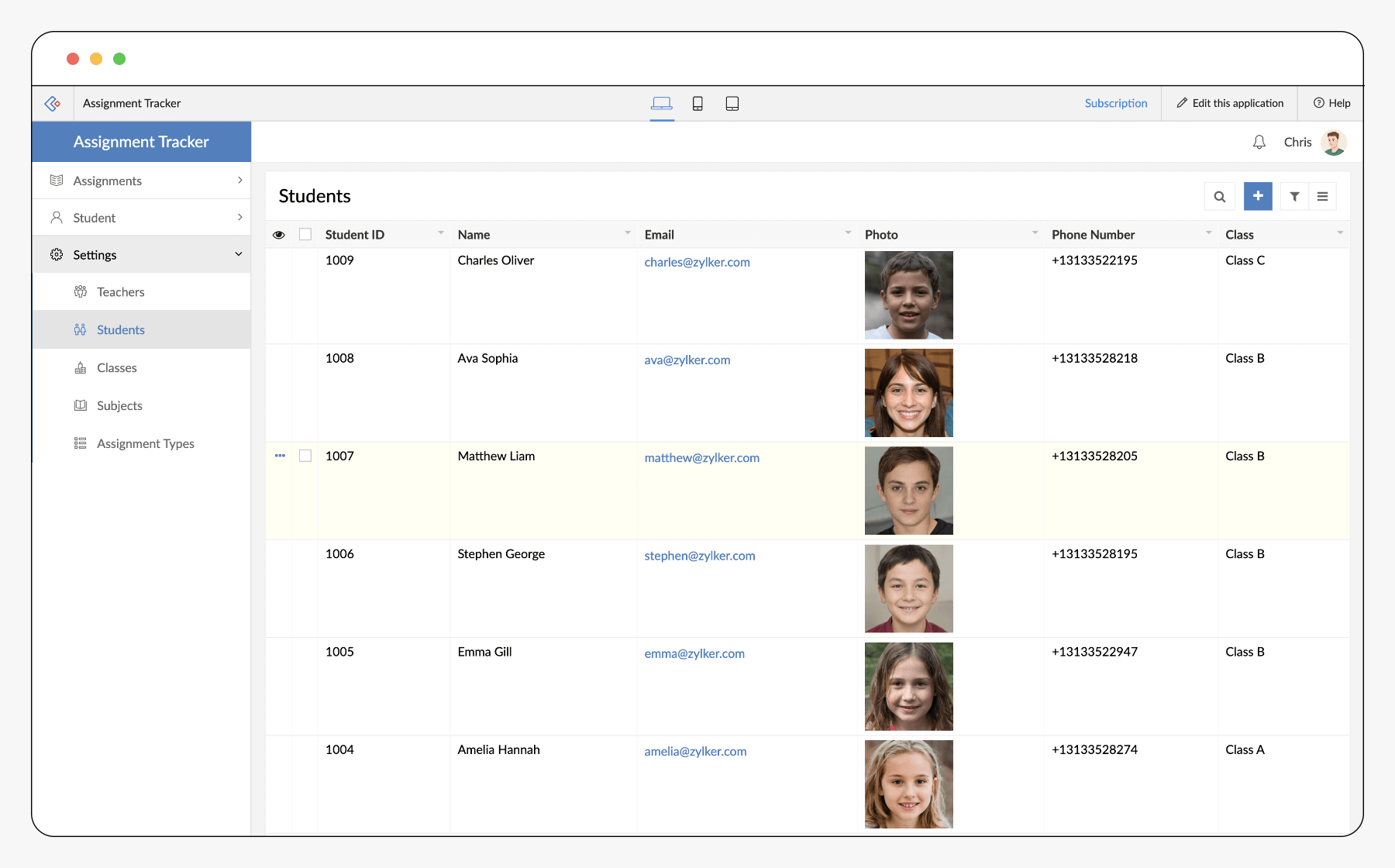
Take the drudgery out of your day
Instantly access relevant data.
With student, teacher, and course details stored right in the assignment tracker app, you never have to go anywhere else to obtain data. Cloud storage allows you to access it anytime, across web and mobile platforms.
Share assignments with ease
Teachers can group students together and distribute assignments, or send them out individually. They can also keep all students on the same page by setting due dates and priority levels.
Review assignments with interactive reports
Assignments can be made active or inactive at the click of a button; corresponding reports automatically get generated, once the assignments have been made inactive. Teachers can review the submitted assignments and include grades, right in the report.
Encourage students to submit assignments online
Students just need to type their answers and upload them into this assignment management app—it's as easy as that. Through detailed reports, they can view pending and submitted assignments, submission details, and marks awarded.
More flexibility. More power.
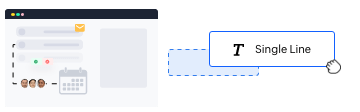
Our assignment tracker management software is fully customizable, right out of the box. The drag-and-drop form builder, with guided scripting feature, allows you to add new functionality to your apps quickly. You can add modules to suit business requirements and implement changes driven by user feedback.
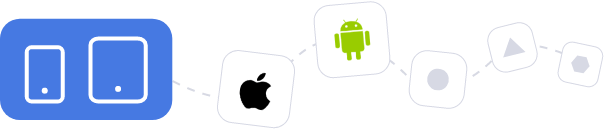
To accompany every app you build on a computer, there's a fully optimized native one waiting for you on your smartphone. Enjoy all of your assignment tracker app's features, from any location, on any device. You get limitless business functionality at your fingertips, all on the go.
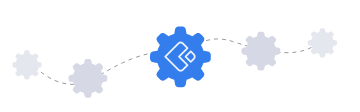
Zoho Creator offers a wide range of prebuilt integrations, such as Workspace, Zapier, and Salesforce, making it easy for you to integrate your assignment management app with them. You can also integrate with online payment systems like PayPal, or inventory management software like SalesBinder, using APIs.
Related Apps
Install, customize, and make this assignment tracker management software yours.
Help | Advanced Search
Computer Science > Software Engineering
Title: peer-aided repairer: empowering large language models to repair advanced student assignments.
Abstract: Automated generation of feedback on programming assignments holds significant benefits for programming education, especially when it comes to advanced assignments. Automated Program Repair techniques, especially Large Language Model based approaches, have gained notable recognition for their potential to fix introductory assignments. However, the programs used for evaluation are relatively simple. It remains unclear how existing approaches perform in repairing programs from higher-level programming courses. To address these limitations, we curate a new advanced student assignment dataset named Defects4DS from a higher-level programming course. Subsequently, we identify the challenges related to fixing bugs in advanced assignments. Based on the analysis, we develop a framework called PaR that is powered by the LLM. PaR works in three phases: Peer Solution Selection, Multi-Source Prompt Generation, and Program Repair. Peer Solution Selection identifies the closely related peer programs based on lexical, semantic, and syntactic criteria. Then Multi-Source Prompt Generation adeptly combines multiple sources of information to create a comprehensive and informative prompt for the last Program Repair stage. The evaluation on Defects4DS and another well-investigated ITSP dataset reveals that PaR achieves a new state-of-the-art performance, demonstrating impressive improvements of 19.94% and 15.2% in repair rate compared to prior state-of-the-art LLM- and symbolic-based approaches, respectively
Submission history
Access paper:.
- HTML (experimental)
- Other Formats
References & Citations
- Google Scholar
- Semantic Scholar
BibTeX formatted citation

Bibliographic and Citation Tools
Code, data and media associated with this article, recommenders and search tools.
- Institution
arXivLabs: experimental projects with community collaborators
arXivLabs is a framework that allows collaborators to develop and share new arXiv features directly on our website.
Both individuals and organizations that work with arXivLabs have embraced and accepted our values of openness, community, excellence, and user data privacy. arXiv is committed to these values and only works with partners that adhere to them.
Have an idea for a project that will add value for arXiv's community? Learn more about arXivLabs .
Filter by Keywords
20 Best Task Management Software Tools to Elevate Your Workflows [2024 Edition]
Sarah Burner
ClickUp Contributor
March 30, 2024
Is your personal or team workload spiraling out of control? We’ve all been there! What’s worse is the anxiety-inducing feeling of dread that follows when you have a bunch of unattended tasks, gradually leading to a lack of motivation . In fact, recent research reveals that 41% of workers find heavy workloads to be the main cause of workplace stress. 😖
However, more often than not, it’s not the amount of work that makes you or your employees feel overwhelmed—it’s poor task management. For instance, setting unreasonable deadlines or delegating tasks without considering team availability may lead people to burnout faster than you can blink.
Luckily, using the right task management software can help you prevent this chaos! We’re here to introduce you to the 20 best task management software that can assist you in assigning tasks and optimizing priorities effectively in 2024. Learn about their key features, limitations, and pricing before you pick your ideal task manager tool. 🌻
What Should You Look for in a Task Management Tool?
- 1. ClickUp—best overall project & task management software
2. Asana—best for remote teams
3. jira—best for experienced professionals, 4. monday.com—best for marketing and pmo teams, 5. trello—best for small teams, 6. hive—best task management software for team collaboration, 7. meistertask—best for kanban task management, 8. ntask—best task management software for scheduling, 9. zenhub—best for software teams, 10. zoho projects—best for complex project management, 11. activecollab—best for personalized task management experience, 12. wrike—best for workflow management, 13. todoist—best for teams on a budget, 14. proofhub—best for task tracking, 15. smarttask—best for managing multiple projects, 16. hubspot (hubspot task management)—best for crm tasks, 17. paymo—best for client tasks and bookings, 18. timecamp—best for time tracking and billing tasks, 19. any.do—best for creating to-do lists, 20. smartsheet—best for spreadsheet-style task management.

Task management software helps identify, monitor, and manage tasks efficiently . It goes beyond a simple to-do list and offers features to let you collaborate on elaborate workflows for effective goal completion .
Naturally, the best task management software allows you to set deadlines, prioritize tasks , track progress, and adjust schedules with ease. Other useful functionalities include:
- Flexibility: A good task management software has a user-friendly interface and runs smoothly on all major operating systems across devices, allowing you more control over your work
- Time tracking: Choose a task management solution that lets you improve your time management through seamless time tracking
- Task automation: The best task management software lets you automate recurring admin tasks, leaving everyone more time to focus on cognitively demanding, high-level assignments
- Communication support: If you’re running a team, look for a tool that supports productive task-related discussions, file sharing, and instant announcements
- Reporting and analytics: Quality task management software provides reporting features to record and analyze information (like clock-in/clock-out data, absence, and overtime hours) for productivity analysis, invoicing, and payroll management
- Integration with other tools: Pick a task management system that integrates with other software you use, like messaging apps , project management software , and writing assistants
20 Best Task Management Software for Balanced Task Planning and Execution
There are tons of task management apps available on the market, but they’re not all cut from the same cloth—for instance, some are versatile tools, while others focus on specific functions like task dependencies.
We’ve chosen the 20 best task management software to help you find the perfect app for your business needs. These are expert-vetted options, cherry-picked after considering criteria like feature set and affordability.
Let’s dive in! 🧐
1. ClickUp —best overall project & task management software
Why end with a bang when we can start with one? 💥
Introducing ClickUp —the best task management solution for individuals, professionals, and teams. It’s not just us; even G2 considers it to be the best-rated task management and team task management software , ranking it #1 in 15+ competitive categories in 2024!
What makes ClickUp click is its AI-enabled, end-to-end support for managing tasks and priorities, monitoring progress, and staying productive.
Leverage ClickUp Tasks for task planning, scheduling, and tracking within a centralized hub. Easily categorize team tasks by type, set Custom Task Status from To Do to Done , and use Custom Fields to set deadlines, add assignees, and track details. For each task assignment, you can:
- Add links and comments to centralize contextual knowledge and discussions
- Specify task dependencies, subtasks, and checklists
- Use @mentions and action items to delegate tasks and share updates
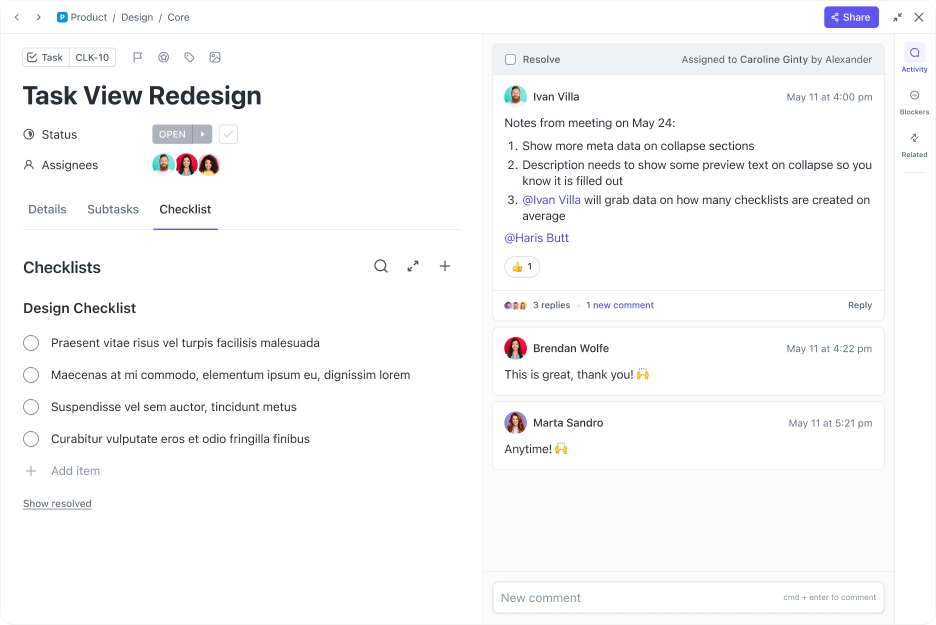
ClickUp’s Calendar View comes with drag-and-drop capabilities for granular task planning. You can also ClickUp’s Recurring Tasks to schedule routine stuff, like meetings and sprint retrospectives, and visualize or adjust your day-to-day on the Calendar.
Stay on top of task lists with ClickUp Reminders that you can create from any task comments or notifications and manage seamlessly from any device.
Prefer a visual task manager? ClickUp’s got you! This adaptable task management software offers 15+ Views for managing tasks on Kanban boards, sorting tasks in the List view, or visualizing timelines with colorful Gantt charts. 🌈
And, if you’re dealing with an overwhelming workload, identify what to prioritize and deprioritize using ClickUp Task Priorities and its color-coded tags. You can also lighten the workload for your employees with ClickUp Automatons , which lets you automate time-consuming tasks like checking emails or organizing documents.
Embrace stress-free productivity with ClickUp Brain and pre-made templates
Beyond tracking and prioritizing tasks, ClickUp also lends a hand with Project Management . For instance, you can employ ClickUp Brain , the platform’s AI assistant, to automate project summaries, task planning, and task standups and updates.
ClickUp Brain is a neural network connecting your tasks, documents, and discussions on the platform—it helps you extract and summarize information and action items through simple prompts and questions.
Take your productivity to new heights with numerous customizable ClickUp templates to help you standardize task assignments immediately! The ClickUp Task Management Template is a great starting point. With this template, you can organize your tasks into Lists like Action Items , Ideas , and Backlog for easier navigation and open separate views to track assignments by department or priority label. 🚩
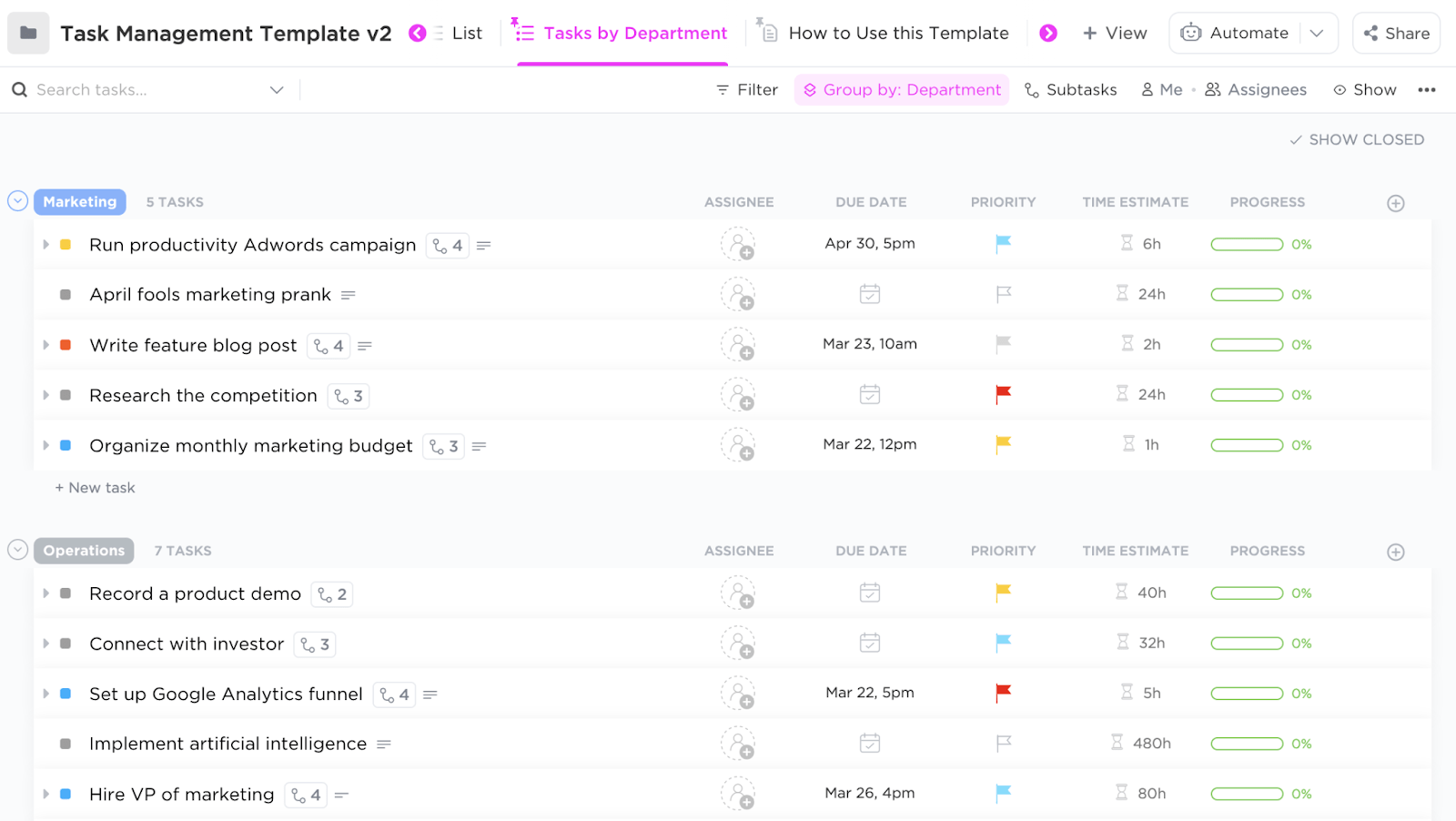
ClickUp best features
- 1,000+ templates to kickstart skillful task management
- Supports time tracking and time blocking
- ClickUp Dashboards for real-time task tracking and productivity metrics
- ClickUp Goals to set measurable task KPIs
- Task Checklist feature for easy to-do list creation
- Multiple project views like List, Table, and Timeline
- Custom task statuses to complement your workflow
- A Bulk Action Toolbar to edit multiple tasks at once
- Automates recurring, error-prone tasks
- Built-in task prioritization levels
- Universal Search and Tags to access tasks quickly
- Supports task generation directly from ClickUp Docs and Whiteboards
- Integrates with 1,000+ apps like Slack, Gmail, Zoom, Outlook, and HubSpot
- Dedicated mobile app for iOS and Android
- Compatible with Mac, Windows, and Linux
- Built-in chat and file-sharing options for task collaboration
- Easy-to-use, no-code interface
ClickUp limitations
- It takes time to explore all its task management features
- A dedicated subtask reporting feature would be a great add-on
ClickUp pricing
- Free Forever
- Unlimited: $7/month per user
- Business: $12/month per user
- Enterprise: Contact for pricing
- ClickUp AI: Add to any paid plan for $5 per member per month
*All listed prices refer to the yearly billing model
ClickUp ratings and reviews
- G2: 4.7/5 (9,000+ reviews)
- Capterra: 4.7/5 (4,000+ reviews)
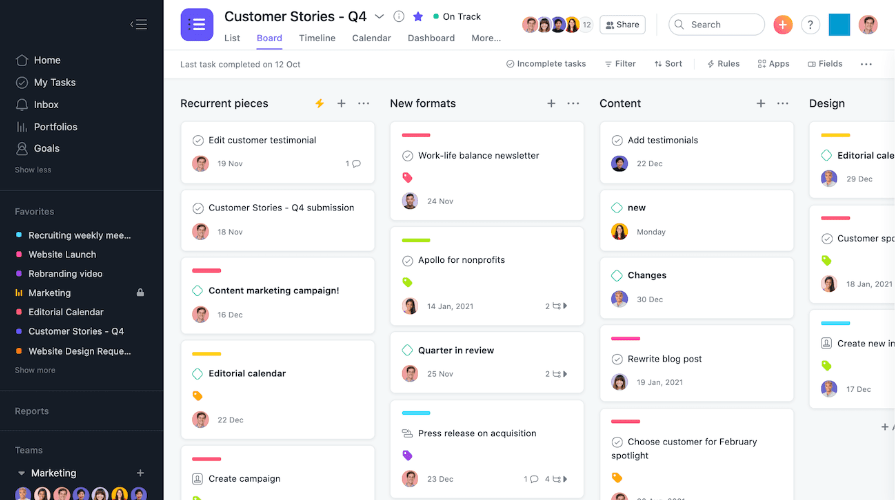
Asana is an online task management software that lets you document all assignments in one location. Like ClickUp, Asana makes creating tasks a breeze. Add task descriptions in the form of bulleted lists, embed files to add context, set due dates, and add assignees in a few clicks. You can also create subtasks, identify blockers, and quickly streamline approvals on task changes.
This quality task management software features a My Tasks list , which helps you prioritize work by auto-promoting tasks based on due dates.
What makes Asana stand out among its alternatives is the Workflow Builder feature , which allows you to create workflows without coding, helping you automate routine tasks and expedite projects. You can even track the same task across departments to avoid work duplication.⚡
Asana is a collaborative task management tool —it lets you communicate with your team directly within the app, encouraging cross-functional collaboration . For added transparency, add relevant collaborators to your team’s tasks to keep them updated on progress.
Asana best features
- Templates for task management
- Remote-friendly collaboration tools
- Zero-code workflow builder
- Search and filtering options for simpler navigation
- Integrates with apps like Slack and Dropbox
- Mobile app for Android and iOS
Asana limitations
- May be challenging to implement for large teams
- Storage and collaboration features could use improvement
Asana pricing
- Personal: Free forever
- Starter: $10.99/month per user
- Advanced: $24.99/month per user
Asana ratings and reviews
- G2: 4.3/5 (9,000+ reviews)
- Capterra: 4.5/5 (12,000+ reviews)
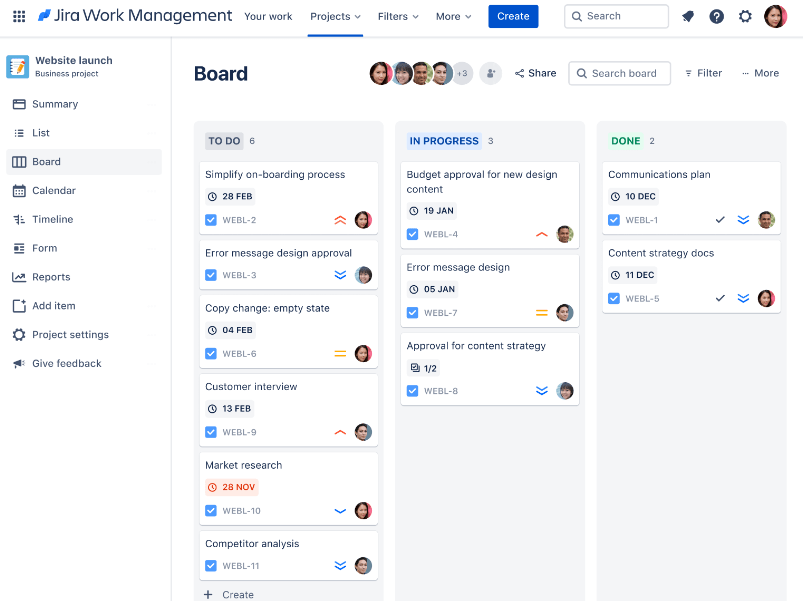
While not as beginner-friendly as most alternatives due to its complex features, Jira is a popular task management software option among seasoned professionals in industries like IT, finance, marketing, and HR. It was first launched by Atlassian as an issue-tracking tool for software developers but has branched out to become a useful tool for project managers and task managers alike.
Jira is a handy tool for agile project management , allowing teams to break a complex project into manageable sprints . Its multiple views, like boards and timelines, help track sprint tasks and identify roadblocks to ensure continuous improvement .
Jira also supports team collaboration by allowing members to brainstorm and exchange task information across departments through shared workflows.
Jira, like ClickUp , lets you update task statuses in real time, while its automaton feature helps you run manual actions in the background. Plus, project managers can automate work using its numerous task management templates . 😀
Jira best features
- Workflow automation support
- Multiple task views for agile teams
- Templates for niches like human resources , marketing, and IT
- Integrates with Atlassian Work Management and other popular apps
- Task management apps for Android and iOS
Jira limitations
- The system can be slow at times
- The user interface and dashboard design could be improved
Jira pricing
- Free: Up to 10 users
- Standard: $8.15/month per user
- Premium: $16/month per user
- Enterprise: Contact sales for pricing
Jira ratings and reviews
- G2: 4.3/5 (5,000+ reviews)
- Capterra: 4.5/5 (13,000+ reviews)
Check out these Jira alternatives !
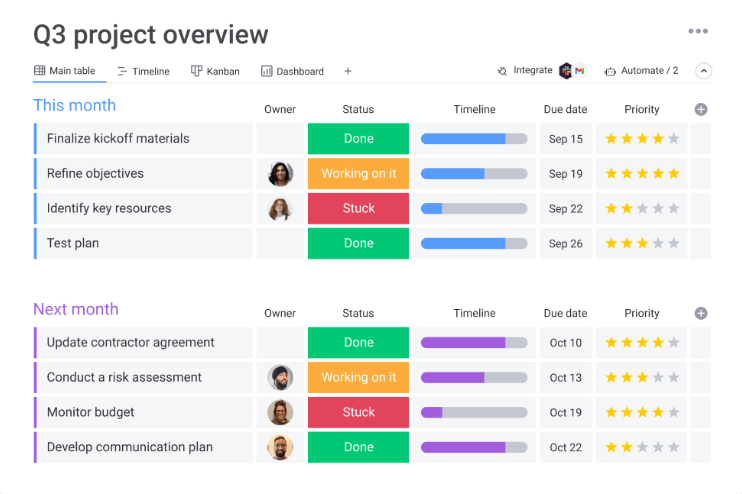
Using Monday.com to manage tasks can make Mondays less dreadful! This simple task management software provides all key features, from multiple task views to task templates, that you’d find in most Monday alternatives .
Still, Monday.com stands out with its smooth interface . Its Work Management feature lets you create no-code workflows. Even project managers enjoy an intuitive layout that allows them to make informed decisions by accessing quick overviews of task progress on a dashboard. 📈
Monday.com allows you to organize workspaces into projects with individual tasks and subtasks, complete with color-coding options to help you track task priorities and statuses easily.
The software offers specific task management features for marketing and PMO teams . For example, marketing teams can benefit from key features like brand asset management , robust Gantt charts, and campaign tracking . Meanwhile, PMO teams rely on features for monitoring OKRs , identifying dependencies, and tracking time for future task planning.
Overall, it’s a well-rounded task management tool—quite similar to ClickUp and Plaky in terms of design and feature set.
Monday.com best features
- Includes 200+ automation options
- Color-coded task statuses and priority levels
- User-friendly
- No-code workflows setup
- Offers a mobile app for iOS and Android
- Integrates with over 200 apps
Monday.com limitations
- The initial setup tends to be overwhelming
- Some users struggle with custom reminders and experience delays in notifications
Monday.com pricing
- Free: Up to two users
- Basic: $9/month per user
- Standard: $12/month per user
- Pro: $19/month per user
Monday.com ratings and reviews
- G2: 4.7/5 (10,000+ reviews)
- Capterra: 4.6/5 (4,000+ reviews)
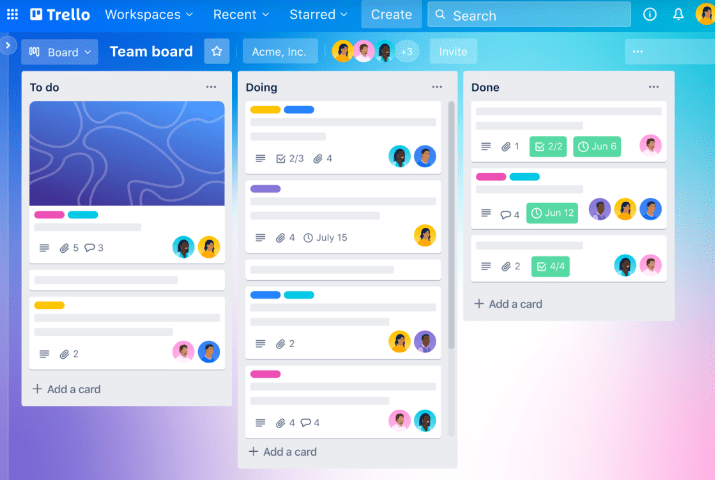
If you’re a fan of Kanban boards, you’ll have a blast using Trello. This task management software is all about moving tasks like sticky notes on a drag-and-drop Kanban board for easier status tracking.
Individuals can use it to create personalized task lists, while team members can rely on advanced checklists to add context to tasks by adding due dates and assignees.
This software supports task prioritization through labels like high, medium, or low priority—filter the labels to access a specific batch of tasks . It also has a no-code automation tool called Butler, which lets you create rules, buttons, and commands to automate select recurring tasks.
Trello lets you view your tasks on calendars, timelines, and neatly organized tables, which is often more than enough to manage workloads in a smaller team. However, if you’re running a larger team that may prefer more flexibility and a wider variety of project views, Trello alternatives with enterprise features may be a better option.
Trello best features
- Butler—a no-code automation tool
- Kanban-based task tracking software
- Includes checklists, calendars, and timelines
- Color coding helps prioritize tasks
- Android and iOS mobile apps
Trello limitations
- Gets costly as users increase in number
- May not support complex project management
Trello pricing
- Standard: $5/month per user
- Premium: $10/month per user
- Enterprise: $17.5/month per user
Trello ratings and reviews
- G2: 4.4/5 (13,000+ reviews)
- Capterra: 4.6/5 (23,000+ reviews)
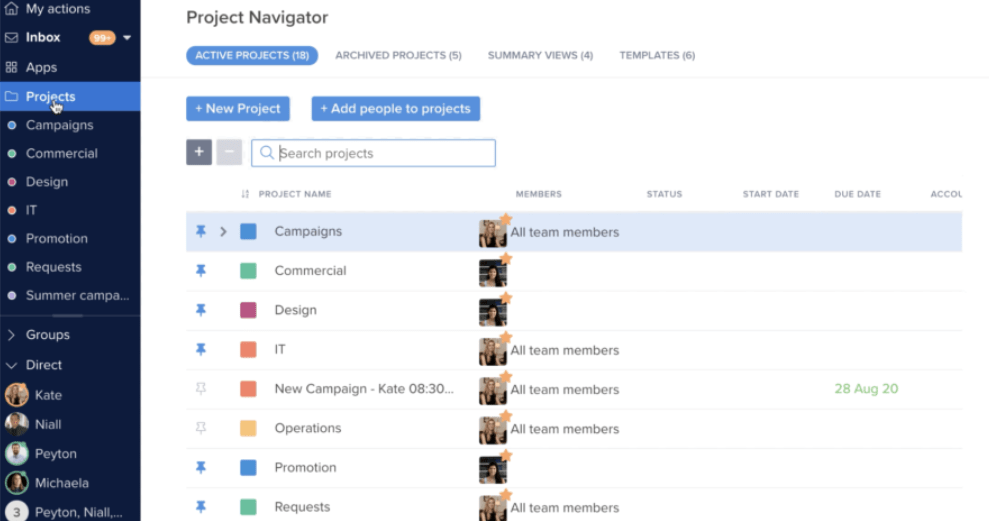
Hive is an all-encompassing project management software that provides any hybrid team with a task management platform to:
- Assign, track, and execute tasks together, regardless of location
- Monitor upcoming tasks across departments
- Collaborate async or live with Hive Notes and Text and Voice Chat
This software lets you observe your tasks from multiple angles . For example, use the Portfolio view to get a detailed overview of tasks across projects or the Label view to categorize subtasks. Focus better by activating the My Actions view to display your task assignments in a concentrated way.
Rely on key features like task status to easily track progress, templates to customize project layouts, and notifications to never miss a beat.
Plus, Hive offers Workflows App —a no-code task automation tool to help you cut down on manual labor and design personalized automated workflows.
Like ClickUp, Hive supports project collaboration by letting teammates communicate across projects by leaving comments on action cards or tagging team members.
Hive best features
- Collaborative task management tool
- Supports individual, group, or project discussions
- AI assistant ( HiveMind ) and templates to support standard tasks
- My Actions view tracks all the tasks assigned to you
- Includes a no-code task automation tool
- Integrates with Dropbox and Google Drive
Hive limitations
- The mobile app has limited functionality
- UX may get compromised with many collaborators
Hive pricing
- Starter: $5/month per user
- Teams: $12/month per user
Hive ratings and reviews
- G2: 4.6/5 (500+ reviews)
- Capterra: 4.5/5 (100+ reviews)
Check out these Hive alternatives !
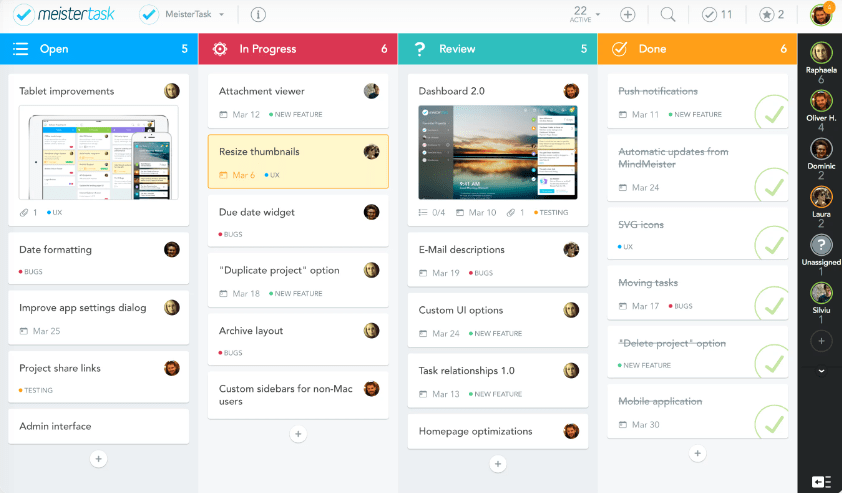
Most alternatives to MeisterTask include Kanban boards as one of their key features. However, MeisterTask excels as Kanban-based task management software because of how user-friendly and intuitive it is. 😻
It provides customizable Kanban-style boards that let you organize and monitor task cards to mark if they’re In Progress , Done , or on the To Do list. These boards keep your work centralized and let you communicate effectively through task comments.
You can mention teammates in the comments, add them as project watchers, and assign them tasks directly from the board. Activate real-time notifications to keep everyone in the loop about the latest project updates.
Besides collaborative boards, the software offers personal pinboards called Agendas, where you can organize all the tasks assigned to you, as well as access additional details like due dates and important files faster.
MeisterTask best features
- Easy-to-use Kanban boards for organizing tasks
- Can serve as a personal task management software
- Task-related collaboration through comments
- Dashboards to track upcoming tasks, time-tracking data, and notifications
- Lets you automate recurring tasks
- iOS and Android mobile apps
MeisterTask limitations
- The software could benefit from adding a workflow calendar to its features
- Notifications may be delayed on mobile devices
MeisterTask pricing
- Basic: Free
- Pro: $6.50/month per user
MeisterTask ratings and reviews
- G2: 4.6/5 (100+ reviews)
- Capterra: 4.7/5 (1,000+ reviews)
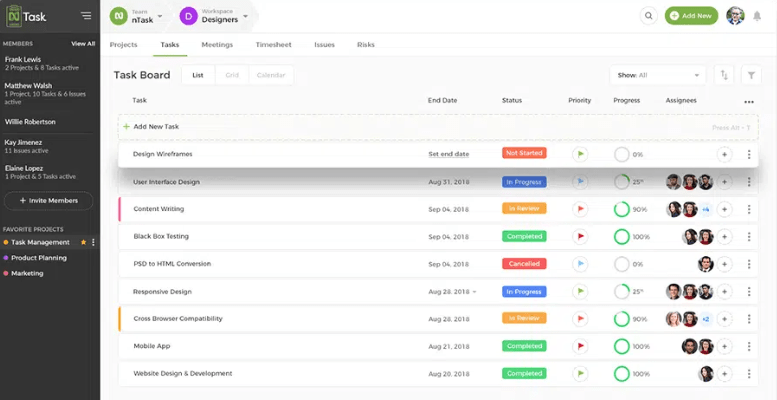
nTask serves as a central hub for tracking and scheduling tasks . Use it’s task scheduling software to assign project tasks or entire task lists to your teammates and set real-time notifications to ensure no action remains overlooked.
Its robust time-tracking capabilities can help you monitor time spent on tasks and estimate the amount of time you’ll need for future tasks and projects.
nTask is a visual task management tool —it lets you plan and prioritize tasks, manage interconnected delivery timelines, and visualize projects with color-coded Gantt charts. Plan your projects easily by creating tasks and adding descriptions and assignees.
Schedule tasks by setting planned and actual due dates and creating task dependencies and milestones to ensure timely completion. Then, set priorities and track their progress using custom statuses.
nTask best features
- Offers multiple project views (board, grid, list, and calendar)
- Comprehensive task tracking
- Visual task management and task scheduling through Gantt charts
- Recurring tasks feature with daily, weekly, or monthly repeats
- Integrates with 1,000+ tools like Apple Calendar, Zoom, and Microsoft Teams
- Android and iOS support
nTask limitations
- Attaching documents and photos may be challenging
- User interface could be more intuitive
nTask pricing
- Premium: $3/month per user
- Business: $8/month per user
nTask ratings and reviews
- G2: 4.4/5 (10+ reviews)
- Capterra: 4.2/5 (100+ reviews)
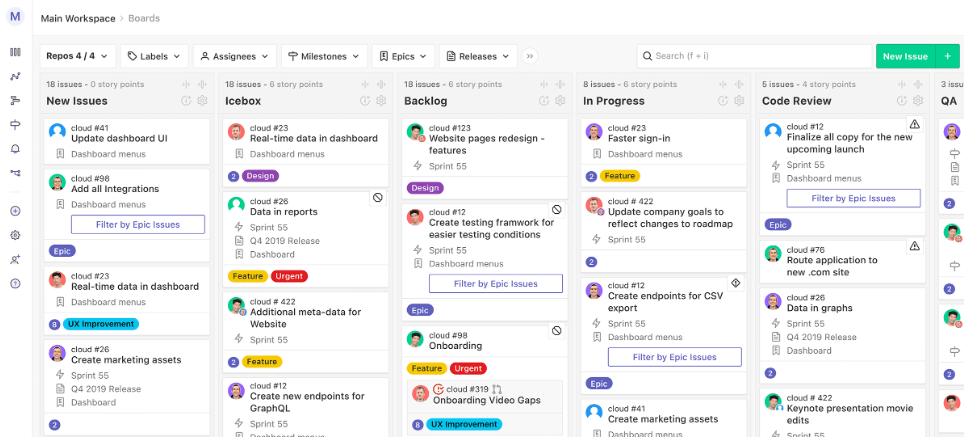
ZenHub is a project management solution that primarily caters to software development teams. It provides a native GitHub integration via a browser extension for Google Chrome or Mozilla Firefox. This helps reduce context switching by keeping project tasks connected with GitHub code.
Plus, ZenHub offers automatic task updates as users complete issues in GitHub, streamlining task completion by eliminating manual status check-ins from your team’s to-do list. There are also automated hand-offs to update all connected workspaces.
This online task management and software development tool brings tasks together on intuitive, drag-and-drop Kanban boards , allowing you to view all current tasks and their status.
Use these boards to prioritize tasks, track projects, and attach pull requests to corresponding issues. Group related issues into Epics or connect various private and public GitHub repos to a single board. 👨🏫
Additionally, ZenHub offers sprint planning features, making it a handy tool for agile teams . It lets you set automated sprint cycles, auto-generate new sprints with prioritized and unfinished tasks, and receive periodical reports on progress.
ZenHub best features
- Various key features for agile teams
- Native GitHub integration
- Provides spring planning and tracking
- Boards for easy task management
- Automate repetitive tasks and hand-offs
- Acts as a visual task management tool with roadmaps and timelines
- Integrates with Figma, Miro, and Loom
ZenHub limitations
- Steep learning curve
- The interface may be challenging to navigate
ZenHub pricing
- Free: 14-day free trial
- For Teams: $8.33/month per user (annual billing)
- For Enterprises: Contact sales for pricing
ZenHub ratings and reviews
- G2: 4.3/5 (30+ reviews)
- Capterra: 4.4/5 (30+ reviews)
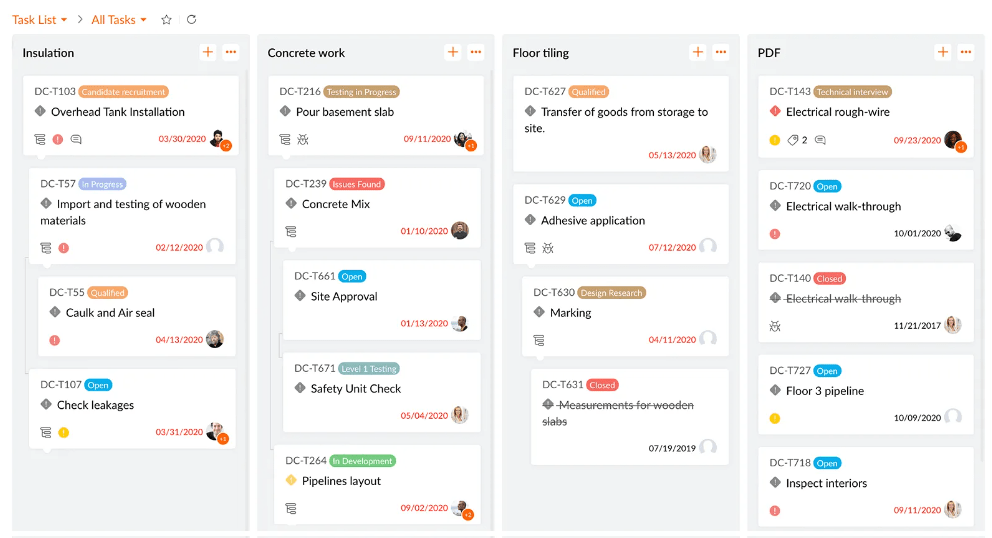
Zoho Projects is a project management software with features such as issue tracking, team monitoring , project timesheets, and resource utilization charts. It helps you manage projects in industries like marketing, construction, IT, and consulting.
It also offers a comprehensive toolset for task management that assists you in breaking projects down into milestones, task lists, and subtasks for simpler tracking.
This task management platform is all about details—each task can have attributes like assignees, work hours, priorities, and reminders. Use timesheets and timers to record time spent on each task.
Like many Zoho alternatives , this tool simplifies task organization by providing Kanban-style boards with drag-and-drop task cards and flexible statuses. As for planning and tracking, use Gantt charts to identify your team’s tasks, set dependencies, and monitor deadlines to ensure everything is done on time. ⌛
The software lets you schedule tasks on a calendar, which provides an overview of team members’ capacity. That way, you enjoy balanced task planning and avoid the risk of team burnout.
Zoho Projects best features
- Quick-access Gantt charts
- Supports versatile task management use cases
- Highly customizable workflows
- Easy task scheduling on calendars
- Integrates with various Zoho, Google, and Microsoft apps
- Task management apps (iOS 9.0+ and Android 4.1+)
Zoho Projects limitations
- Initial setup may be challenging
- Software customization can be time-consuming
Zoho Projects pricing
- Free: Up to three users
- Premium: $4/month per user
- Enterprise: $9/month per user
Zoho Projects ratings and reviews
- G2: 4.3/5 (300+ reviews)
- Capterra: 4.4/5 (400+ reviews)
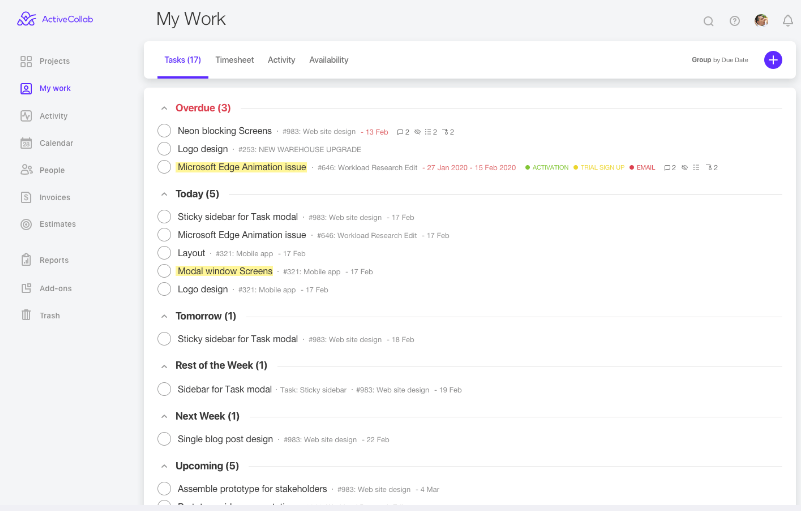
ActiveCollab is all about increasing productivity and meeting deadlines. The tool lets you add due dates, assignees, and detailed task descriptions. Break down complex actions into subtasks, ensuring all assigned tasks get done as planned. You can also:
- Group tasks based on different criteria
- Duplicate repetitive tasks
This online task management software offers three project views to choose from, which is less than what ActiveCollab alternatives typically offer. Still, it includes frequently used views—the Kanban board, Gantt chart, and List view. 📝
ActiveCollab helps you reduce manual labor by automating recurring tasks. And, if you need to ensure actions are completed in a fixed order, just create dependencies between multiple tasks.
ActiveCollab stresses the importance of a personal task manager —it provides a My Work page where you can find and organize your task assignments by project or due date.
This page also serves as your personal timesheet, offering an overview of all your time records. You can revisit your activity in terms of the comments posted, tasks created or closed, or due dates modified.
ActiveCollab best features
- Excellent personal task management software
- Automation for repetitive tasks
- Dependencies for order-specific actions
- Android and iOS smartphone apps
- Integrates with other task management tools like Asana and Trello
ActiveCollab limitations
- The file-sharing feature of this task management software could be simpler to use
- Adding more customization options could be beneficial
ActiveCollab pricing
- Plus: $9.5/month 3 users/month
- Pro: $8/month per user/month
- Pro+Get Paid: $11.75/month per user
ActiveCollab ratings and reviews
- G2: 4.2/5 (90+ reviews)
- Capterra: 4.5/5 (300+ reviews)
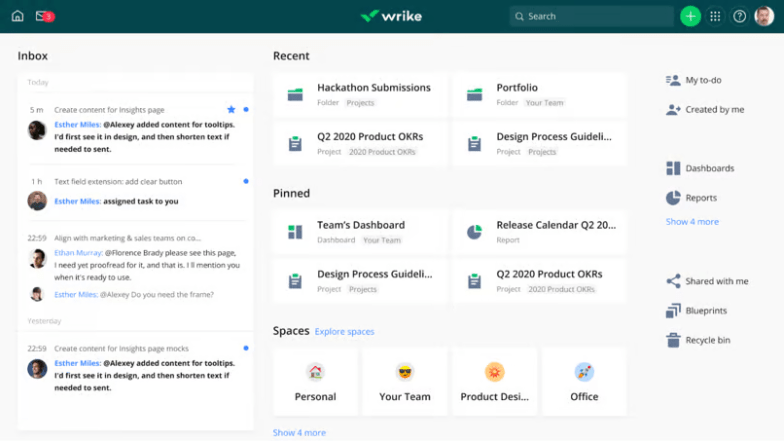
Wrike is well-known for its robust task and workflow management features. It allows you to prioritize work on personalized dashboards by pinning important tasks from your to-do list and automatically sorting them by priority and due date.
Like most of Wrike’s competitors , Wrike supports strong teamwork —it lets teams collaborate on real-time project plans, task assignments, and decision-making within the platform.
Wrike’s workflows adapt to your needs, so regardless of your project management methodology, you can visualize your tasks in views like Gantt Chart, Kanban, and Calendar.
Plus, the software includes built-in proofing tools for quick yet detailed task feedback, task automation to save time, and collaboration tools to increase work efficiency.
Additionally, Wrike allows project managers to build customizable reports on task and team performance. They can further optimize workflows by analyzing relevant key performance indicators (KPIs) on dashboards.
Wrike best features
- Comprehensive project dashboards with personalized reports
- Supports feedback-friendly team collaboration
- Multiple project views
- 400+ integrations
- Task automation
Wrike limitations
- Could use more custom display options
- Some users experience task syncing issues
Wrike pricing
- Team: $9.80/month per user
- Business: $24.80/month per user
- Enterprise and Pinnacle: Contact sales
Wrike ratings and reviews
- G2: 4.2/5 (3,000+ reviews)
- Capterra: 4.3/5 (2,000+ reviews)
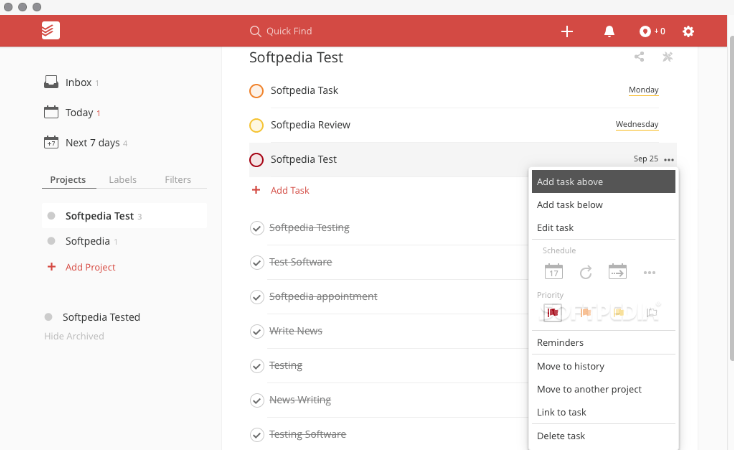
While many Todoist alternatives on this list offer additional project management features, Todoist is purely focused on task management. It assists you in creating detailed daily to-do lists to ensure optimal day-to-day organization.
The tool is simple to use—just create tasks and subtasks, determine priorities, add due dates, and set reminders to ensure nothing goes overlooked. 👀
Although it’s primarily a task management app, Todoist can help you manage projects as it includes goal-setting features , labels to categorize tasks, and task descriptions to keep information centralized.
You can use its Upcoming calendar view to see which tasks are due urgently and use a drag-and-drop function to reschedule assignments. If you need to focus on tasks that are currently due, simply go to the Today view .
While Todoist’s simple navigation and low price make it a handy tool for personal task management, it’s also a good to-do list app for teams on a tight budget . Its project management features aren’t too comprehensive, but you can still assign tasks and leverage comments for better teamwork and transparency.
Todoist best features
- Efficient daily task management
- Multiple task views (including Kanban boards and lists)
- Basic team collaboration support
- Easy navigation
- Integrates with various productivity and time-tracking apps
- Has a to-do list app for Android and iOS
Todoist limitations
- Integrating the software with some apps may be challenging
- It would be beneficial if the app displayed tasks when you’re offline
Todoist pricing
- Beginner: Free
- Pro: $4/month per user
- Business: $6/month per user
Todoist ratings and reviews
- G2: 4.4/5 (700+ reviews)
- Capterra: 4.6/5 (2,000+ reviews)
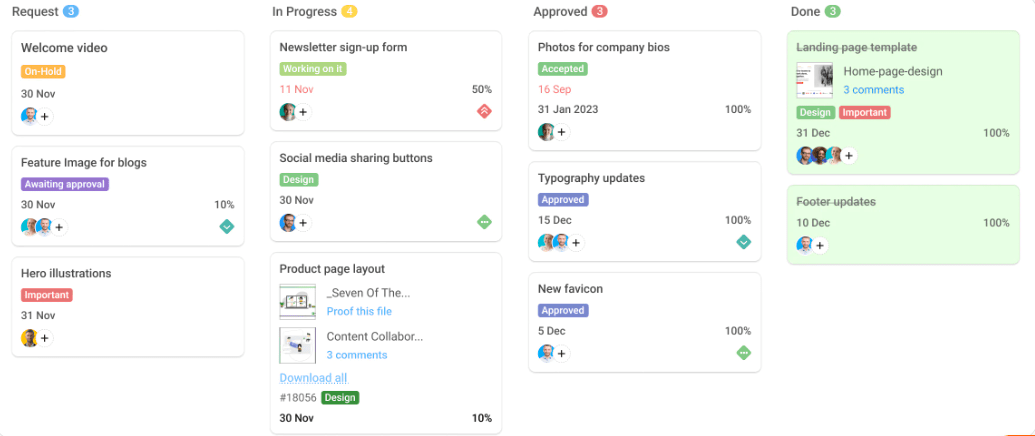
Besides being a task management tool, ProofHub is a task tracking software that lets you easily create task lists and individual tasks, assign them to team members, and closely follow due dates and priorities across task categories . Got complex tasks? Divide them into subtasks for simpler tracking.
Similar to ProofHub alternatives , the software is fully customizable —it lets you build personalized workflows and use custom fields to capture any task-related data you deem important. Custom fields enhance task tracking —because you can customize them to track virtually anything, be it deadlines or output.
Speaking of tracking, ProofHub has a feature for creating task dependencies to help you ensure tasks are completed in a predetermined order. If you want to offer more clarity to assignees, leave comments on tasks or use mentions to guide them.
Additionally, ProofHub offers various reporting options to help you gain insight into your project’s progress. You can enhance resource allocation by analyzing resource utilization reports or tracking project-specific progress with task completion reports.
ProofHub best features
- Multi-faceted task tracking software
- Customizable workflows
- Supports task-related discussions through comments and mentions
- Reports to gauge task progress
- Various project views like Kanbans and tables
- Mobile-friendly
ProofHub limitations
- Notifications can get difficult to manage
- Some users find its customization features complex
ProofHub pricing
- Essential: $45/month
- Ultimate Control: $150/month (or $89/month for a limited time offer)
ProofHub ratings and reviews:
- G2: 4.5/5 (80+ reviews)
- Capterra: 4.6/5 (90+ reviews)
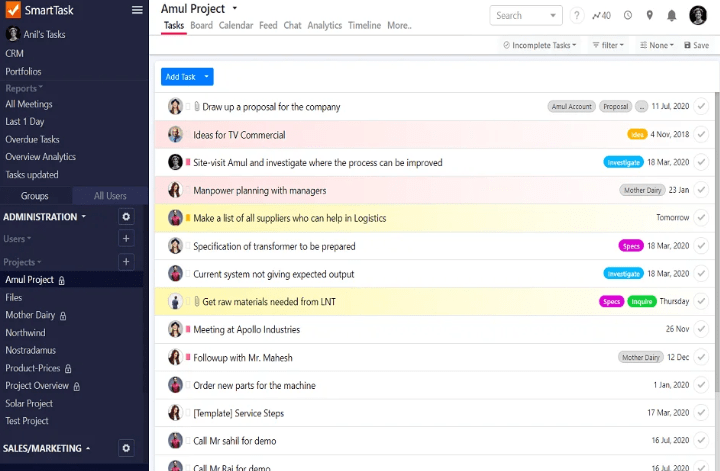
SmartTask includes all key features you’d typically find in a task management tool—it lets you create subtasks, set deadlines, add dependencies, and manage tasks in views like calendar, list, and board.
However, this software especially stands out with its tools for managing multiple projects simultaneously in a cross-functional setup. Its Project Portfolios feature allows you to create multiple boards to track separate goals, aligning your marketing, sales, and design teams. To play it safer, you can even share task lists across teams to prevent orphaned actions.
SmartTask supports team collaboration by letting you comment on tasks and generate real-time notifications for updates. You can also invite clients, vendors, and external stakeholders to collaborate on any task or project by sending them an invitation.
Plus, it provides reports on both project tasks and sales that you can easily find through its advanced search bar. 🔎
SmartTask best features
- Internal and external team communication
- Set recurring tasks (daily, weekly, or monthly)
- Task and sales reports
- Supports managing tasks across projects
- Time tracking for individual tasks
- Task management tools for iOS and Android
SmartTask limitations
- The UI and UX could use some improvement
- Adding more integrations would be useful
SmartTask pricing
- Premium: $7.99/month per user
- Business: $10.99/month per user
SmartTask ratings and reviews
- G2: 4.5/5 (60+ reviews)
- Capterra: 4.6/5 (30+ reviews)
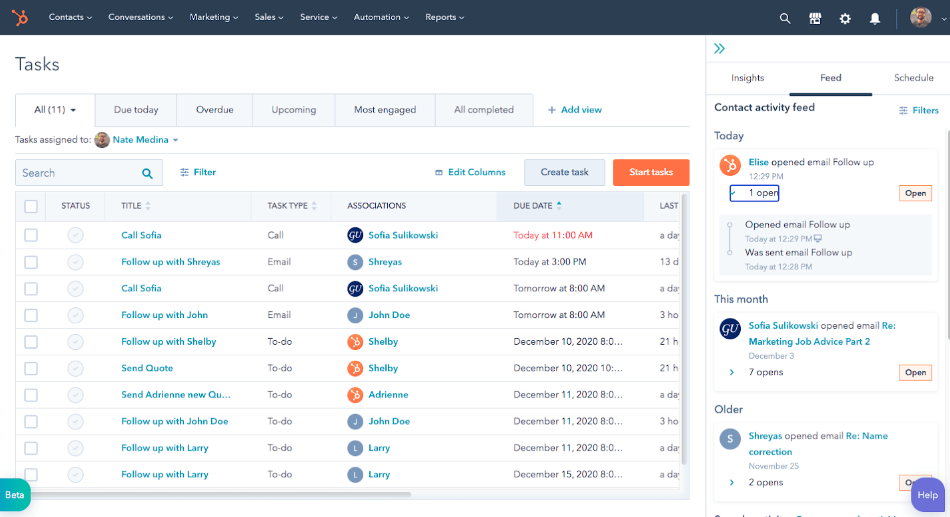
HubSpot Task Management is far from a traditional task manager—it’s a suite designed to help marketers streamline Customer Relationship Management (CRM) processes. Effective CRM depends on properly storing, tracking, and managing lead- and customer-based activities. HubSpot helps you streamline these scattered tasks to boost revenue.
The software reduces the need to switch between multiple tabs when talking to prospects by providing dashboards that centralize all your work. Use them to track your to-do list items, view prospect details, and stay on top of meetings.
Need to create new tasks? Do that directly from your workflows, email inbox, or to-do list. If you need insights from past task performances, like deals made and activities completed, leverage HubSpot’s reporting tools.
This platform simplifies communication with email templates (for sending messages in bulk) and real-time team chat.
While sales and marketing teams may enjoy HubSpot’s task management style, some of HubSpot’s alternatives could be a better choice for companies in IT or finance.
HubSpot best features
- A wide array of CRM functionalities
- Live team chats
- Dashboards to centralize prospect-related work
- Integrates with apps like WordPress, Gmail, and Zapier
- iOS and Android mobile app for HubSpot CRM
HubSpot limitations
- Limited option to prioritize tasks
- Workflow automation is limited on the free plan
HubSpot pricing
For individuals and small teams:
- Starter: $15/month
- Professional: $800/month
HubSpot (CRM) ratings and reviews
- G2: 4.4/5 (11,000+ reviews)
- Capterra: 4.5/5 (4,000+ reviews)
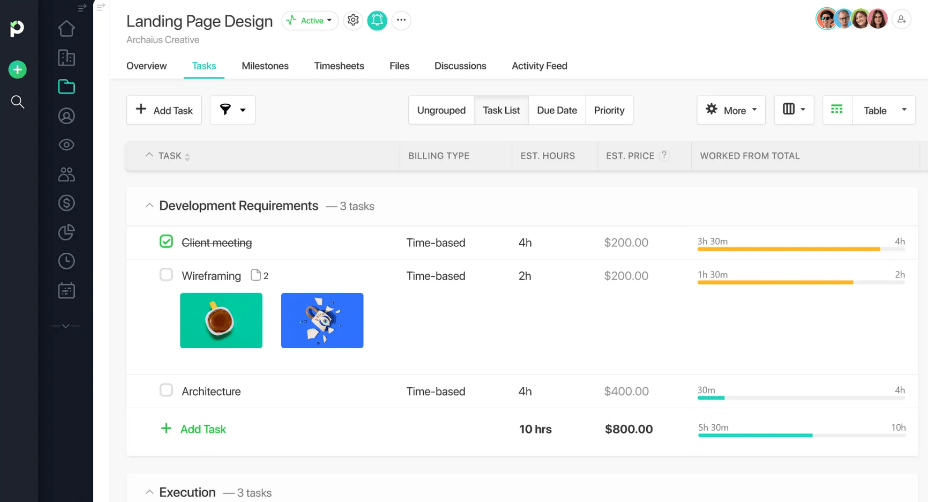
Paymo is a simple task management software designed for professionals who want to manage client work and resources within one platform . It lets you automatically set up ghost bookings based on the task details you’ve entered in the past and receive a visual overview of all bookings for the entire team on a single screen. 🖥️
Paymo also simplifies managing resources by allowing multiple managers to work on schedule to avoid overbooking or underbooking. You can:
- Book time for time-sensitive tasks by the hour
- Accommodate unscheduled tasks with a simple drag-and-drop function
- Visualize your team’s availability on timelines
- Send booking notifications only to relevant team members
The platform lets employees view their tasks in a single view, while task managers monitor both their personal tasks and team’s work in separate views. Like with most Paymo alternatives , you get project views like Gantt charts, Tables, and Calendars to visualize tasks.
It also encourages team collaboration, offering a detailed Task View to chat in real-time about the latest task updates. Finally, Paymo provides task priority levels like critical, high, normal, or low across teams.
Paymo best features
- Timelines to assess employee availability
- Allows booking time for client tasks by the hour
- Real-time chat
- Project views like Gantt chart and Kanban board
- Integrates with apps like Slack, Google Calendar, and JotForm
- Mobile app support for iOS and Android
Paymo limitations
- The mobile app may be tricky to navigate
- Takes time to train employees to use the platform
Paymo pricing
- Starter: $5.9/month per user
- Small Office: $10.9/month per user
- Business: $16.9/month per user
Paymo ratings and reviews
- Capterra: 4.8/5 (400+ reviews)
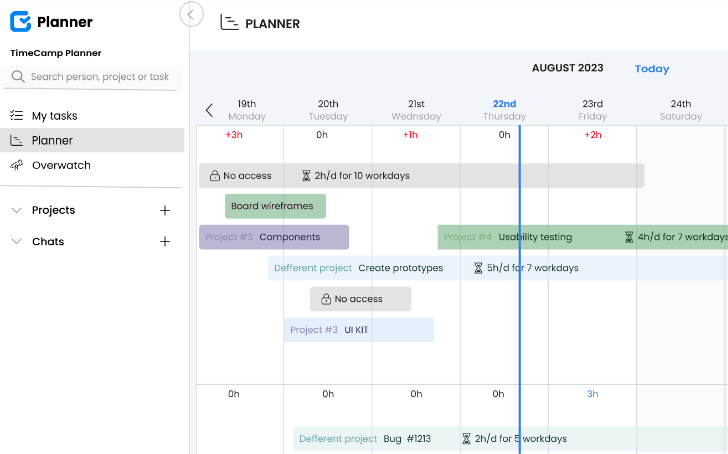
While most TimeCamp alternatives on this list include typical task and project management features, TimeCamp shifts its focus to time tracking instead.
With its robust time-tracking capabilities , you can track your team’s working hours, standardize times for specific jobs, plan task assignments, and monitor each team member’s productivity.
TimeCamp also provides features for measuring the profitability of your projects and tracking billable hours, streamlining invoicing and resource management. Basically, the tasks you had to do manually, like creating invoices and approving timesheets, can be automated with TimeCamp.
Although this software highlights time tracking, it’s still a task management tool. Its TimeCamp Planner product helps you organize tasks and monitor their progress on Kanban-style boards.
If you’re dealing with repeatable tasks, automate them by specifying information like due dates, assignees, and progress statuses. Plus, you can switch from team task overview to your own using the My Tasks feature , which displays your assigned tasks on a list, calendar, or timeline view.
Note that TimeCamp’s time tracking and task management features are available on separate pricing plans .
TimeCamp best features
- Comprehensive time-tracking tools
- Kanban board for easy task tracking
- Personal task lists
- Can automate repeatable tasks
- Includes apps for Android and iOS
- TimeCamp (not the TimeCamp Planner) integrates with tools like ClickUp, Google Calendar, and Slack
TimeCamp limitations
- The user interface and server load time could be better
- The tool may be buggy at times
TimeCamp pricing (for TimeCamp Planner)
- Pro: $2.99/month per user
TimeCamp ratings and reviews
- G2: 4.7/5 (100+ reviews)
- Capterra: 4.7/5 (500+ reviews)
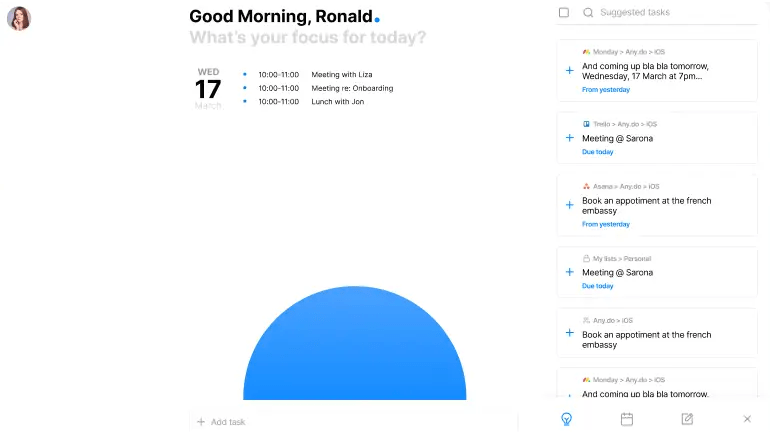
Any.do specializes in providing easy-to-use to-do lists to help you achieve personal or professional goals efficiently. You can organize your to-dos in separate lists and projects, all color-coded to set clear priorities and determine task categories. Enhance your task lists with:
- Subtasks and attachments for added task awareness
- Deadlines and reminders
Sharing responsibilities is also simple—just share a task list with your teammates or family to collaborate on task completion . For example, you can assign different items on a grocery list to family members and track the list to ensure they complete the task successfully. If there’s an urgent matter you wish to discuss, use the chat function to communicate in real time.
For companies looking for a task management tool with project management capabilities, Any.do is a solid choice as it also offers features like customizable workflows, pre-made templates, and powerful integrations with other work tools.
If you want more comprehensive workflow features, though, explore some more advanced Any.do alternatives .
Any.do best features
- Provides simple to-do lists
- Great as a daily task manager
- Lets you share lists with teams, family, and friends
- Reminders for timely task completion
- Has apps for iOS and Android
- Integrates with other task management tools like ClickUp and Asana
Any.do limitations
- Some users desire more integrations (like with Microsoft Calendar)
- Limited advanced features for this price range
Any.do pricing
- Premium: $5/month per user
- Family: $8/month per four users
- Teams: $5/month per user
*All listed prices refer to approx values billed annually
Any.do ratings and reviews
- G2: 4.2/5 (100+ reviews)
- Capterra: 4.4/5 (100+ reviews)
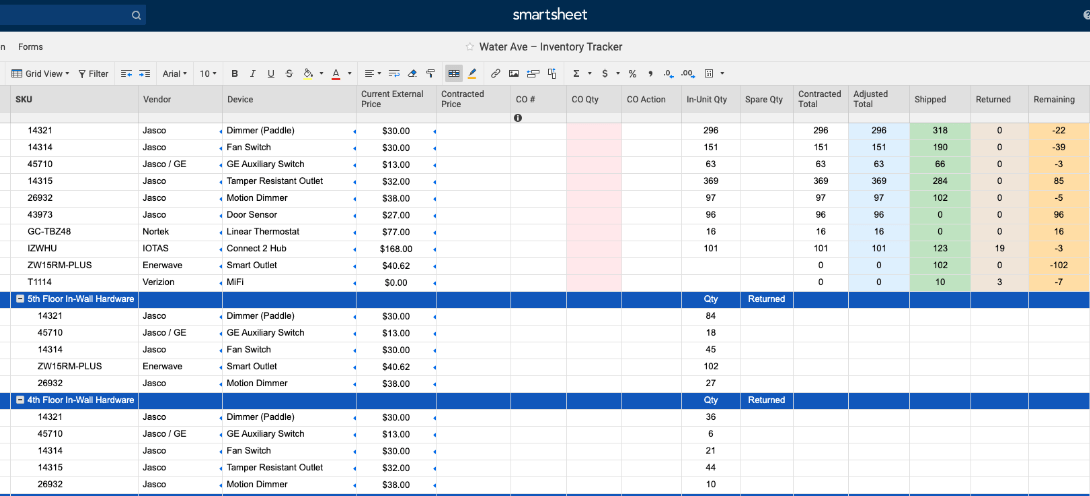
Smartsheet is a spreadsheet-like task management tool . It’s great for those who are comfortable tracking tasks in Excel but are open to switching to a tool with less complicated calculation work.
Like ClickUp, Smartsheet offers comprehensive project and task management tools for handling different workflows. For example, it lets you schedule tasks, create subtasks, allocate resources, and track assignments efficiently from one platform. ✅
Here, tasks are represented on spreadsheets for individual projects. Like most Smartsheet alternatives , you can add basic task information, like due dates and assignees, in clear fields or columns.
However, these aren’t traditional spreadsheets—they also provide the advanced features of a modern task management tool. For instance, you can use checkboxes, dropdown lists, and comments to interact with tasks on Smartsheet.
This is a great task management software for teams working on extensive projects as it includes templates for agile project management, OKR tracking, budgeting, and resource management.
It also supports task automation, real time collaboration , and advanced analytics. Plus, it integrates with services you may already use, like Google Workspace and Microsoft Teams.
Smartsheet best features
- Spreadsheet-based project management tool
- Advanced functionalities for monitoring complex projects
- Supports real-time team communication
- Templates for agile project management
- Integrates with numerous communication, data visualization, and project delivery apps
- Available as an iOS or Android mobile app
Smartsheet limitations
- There may be a steep learning curve for users figuring out advanced features
- It could benefit from adding more template options
Smartsheet pricing
- Pro: $7/month per user
- Business: $25/month per user
Smartsheet ratings and reviews
- G2: 4.4/5 (14,000+ reviews)
- Capterra: 4.5/5 (3,000+ reviews)
Leveraging the Best Task Management Software: Which Tool to Pick
Since all the products on our list are rated well by users, it’s natural to be overwhelmed by the choices. So, what do you really prioritize in a task management platform?
The answer is straightforward— great task management software provides features that help you plan, organize, track, and assign tasks, but you shouldn’t have to pay a fortune for these functions. Don’t settle for a tool that doesn’t include the bare minimum, i.e., reminders, automations, task views, and collaboration features.
Lastly, task management tools are supposed to make your life easier, not vice versa, so ensure your chosen tool is easy to implement and fun to work with! 🥳
If you can’t make a choice, try ClickUp ! It’s got all the features you’ll need to streamline task management with minimal effort. Whether you want to set goals, track and assign tasks, manage time, reduce manual labor, or communicate with your team, the possibilities are endless.
Sign up to ClickUp today and enjoy the best toolkit a free task management software has to offer! 💖
Questions? Comments? Visit our Help Center for support.
Receive the latest WriteClick Newsletter updates.
Thanks for subscribing to our blog!
Please enter a valid email
- Free training & 24-hour support
- Serious about security & privacy
- 99.99% uptime the last 12 months
Assignments
Create an assignment in Microsoft Teams
Assign quizzes in Microsoft Teams
Turn in an assignment in Microsoft Teams
View and navigate your assignments (student)
View and navigate your assignments (educator)
Manage assignments on a mobile device
Grade, return, and reassign assignments
Delete an assignment in Microsoft Teams
Add a tag to your assignment
Adjust assignment settings in your class team
Assign work to multiple classes at once
Create group assignments or assign to individual students
Create and manage grading rubrics in Microsoft Teams
Collaborate with other educators on a form or quiz
Edit an assignment in Microsoft Teams
Schedule work to assign later
Save an assignment as a draft in Microsoft Teams
Repost an assignment in Microsoft Teams
Add MakeCode activities to assignments in Microsoft Teams
Send weekly assignment summaries to parents and guardians
Turn-in celebrations in Microsoft Teams assignments
Use Turnitin with Microsoft Teams
Edit Word documents in Teams for Education
Use OneNote Class Notebook in Teams
Review student work in Class Notebook
Provide written, audio, or video feedback in Class Notebook
Deleting and restoring a OneNote Class Notebook that's linked to a Microsoft Teams assignment
Assign quizzes from Microsoft Teams without affecting individual Forms limits

Need more help?
Want more options.
Explore subscription benefits, browse training courses, learn how to secure your device, and more.
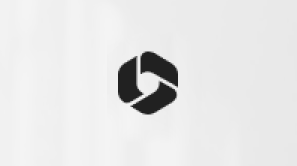
Microsoft 365 subscription benefits
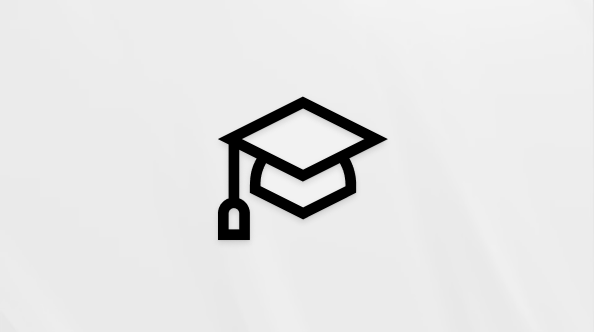
Microsoft 365 training
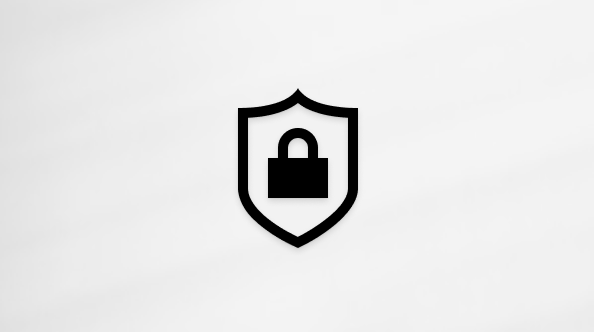
Microsoft security
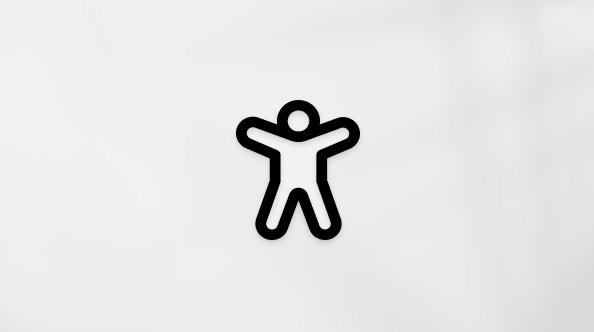
Accessibility center
Communities help you ask and answer questions, give feedback, and hear from experts with rich knowledge.

Ask the Microsoft Community
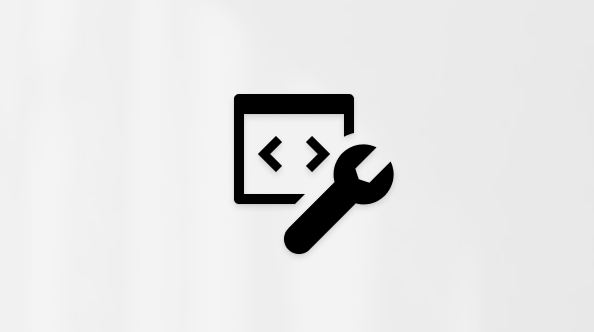
Microsoft Tech Community
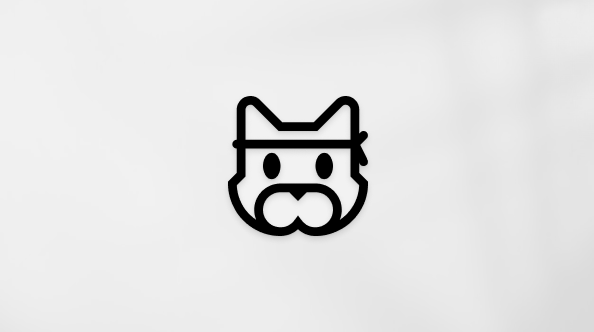
Windows Insiders
Microsoft 365 Insiders
Was this information helpful?
Thank you for your feedback.
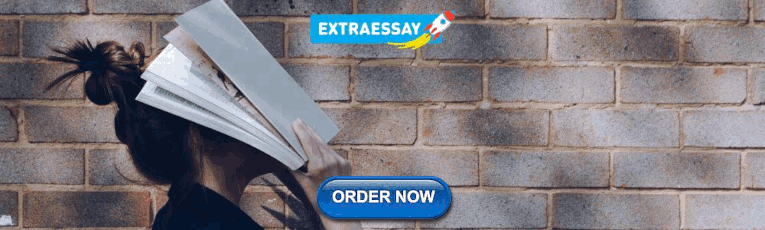
IMAGES
VIDEO
COMMENTS
Operating System: It is the main program of a computer system. When the computer system ON it is the first software that loads into the computer's memory. Basically, it manages all the resources such as computer memory, CPU, printer, hard disk, etc., and provides an interface to the user, which helps the user to interact with the computer system.It also provides various services to other ...
The main topics in software engineering can be summarized using a bunch of big scary words and phrases - as is the tradition in anything related to computer science and mathematics. ... In Kotlin, brackets following a class name denotes //an assignment statement; it does not use a "new" keyword val fourGraph = SudokuPuzzle(4, Difficulty ...
ASSIGNMENT ON SOFTWARE, ITS TYPES, SYSTEM SOFTWARE, OPERATING SYSTEM, FUNCTION & ITS TYPES (ASSIGNMENT FOR INFORMATION TECHNLOGY IN EDUCATION) Department of Education Saurashtra University, Rajkot (October - 2011) HARDWARE Vs SOFTWARE The electrical, electronic, mechanical and magnetic components that make up the computer system are together ...
Module 1 • 2 hours to complete. This module provides you with an overview to the field of software engineering. In the first lesson of this module, you will be introduced to the field of software engineering, and learn about the software development lifecycle (SDLC), elements of building high-quality software, and writing requirements.
The primary objectives of this course are to introduce software engineering techniques and their application to real-world business problems. You will be equipped with practical design and programming techniques for the purpose of modeling significant business applications quickly. In a step-by-step manner, the instructor will take you through ...
Assignment Layout Assignment 2 Assessment. Assignment is neatly assembled on 8 1/2 by 11 paper. Cover page with your name (last name first followed by a comma then first name), username and section number with a signed statement of independent effort is included. Problem and system analysis documentation is correct.
Assignment 2. The purpose of Assignment 2 is to describe to your client and the course team the progress up to Milestone 2. The deliverables for Assignment 2 are: Presentation on Milestone 2 (group assignment) Report on Milestone 2 (group assignment) Survey 2 (individual assignment) If the team is following an iterative process Milestone 2 is ...
Introduction to Software Development 1.1 Basic Software Design \I conclude that there are two ways of constructing a software design: One way is to make it so simple that there are obviously no de ciencies and the other way is to make it so complicated that there are no obvious de ciencies." { C. A. R. Hoare
Watch on. Hardware is any element of a computer that's physical. This includes things like monitors, keyboards, and also the insides of devices, like microchips and hard drives. Software is anything that tells hardware what to do and how to do it, including computer programs and apps on your phone. Video games, photo editors, and web browsers ...
There are 4 modules in this course. After completing this course, you will have an understanding of the fundamental principles and processes of software testing. You will have actively created test cases and run them using an automated testing tool. You will being writing and recognizing good test cases, including input data and expected outcomes.
Unmute. Principles Of Software Design : Should not suffer from "Tunnel Vision" -. While designing the process, it should not suffer from "tunnel vision" which means that is should not only focus on completing or achieving the aim but on other effects also. Traceable to analysis model -. The design process should be traceable to the ...
Here is the difference between software engineering and coding: Software engineering focuses on creating business value by addressing the correct problems. Failing to understand the needs of the business is the most common reason why software projects fail. Therefore, understanding the goal is undoubtedly the most crucial part of any take-home ...
Here is the Full Assignment Report on Software Development Life Cycles Part: 1 Introduction: The large insurance company predominantly operating in the United Kingdom is now offering its products and services to many countries in its drive to grow and become a large international company.
Dislike. In this lesson we explore how computer software and hardware work together for processing to take place. Software programs are either application software or system. This lesson is aligned with NCS for grade 10. Learner Video. Information Technology / Grade 10 / System Software. Information Technology / Grade 11 / Software.
The Assignment. Computer programming could be viewed as a form of education as well as engineering; the programmer is teaching the computer to perform specific tasks. The computer has no actual intelligence, only the ability to instantly respond to any programming language that can be installed on it. It's up to the programmer to learn and ...
Assignment. What is software ? > The term software refers to a collection of programs. > A program is a sequence of instructions written in a language, which can be understood by a computer. > A software package is a group of programs, which solve a specific problem, or perform a specific type of job. > Consists of computer programs and data ...
Easily distribute, analyze, and grade student work with Assignments for your LMS. Assignments is an application for your learning management system (LMS). It helps educators save time grading and guides students to turn in their best work with originality reports — all through the collaborative power of Google Workspace for Education. Get ...
Assignment 01 - Software Development Lifecycles Plagiarism Plagiarism is a particular form of cheating. Plagiarism must be avoided at all costs and students who break the rules, however innocently, may be penalised. It is your responsibility to ensure that you understand correct referencing practices. As a
The ultimate study app. The MyStudyLife student planner helps you keep track of all your classes, tasks, assignments and exams - anywhere, on any device. Whether you're in middle school, high school or college MyStudyLife's online school agenda will organize your school life for you for less stress, more productivity, and ultimately ...
Make assignment management simple using our assignment tracker software. Assignments are one of the best ways to track the progress of both students and teachers. Students can put their understanding to the test, while teachers can assess how well their lessons are being received. This assignment tracker management software eliminates the ...
Automated generation of feedback on programming assignments holds significant benefits for programming education, especially when it comes to advanced assignments. Automated Program Repair techniques, especially Large Language Model based approaches, have gained notable recognition for their potential to fix introductory assignments. However, the programs used for evaluation are relatively ...
Capterra: 4.6/5 (2,000+ reviews) 14. ProofHub—best for task tracking. Via: ProofHub. Besides being a task management tool, ProofHub is a task tracking software that lets you easily create task lists and individual tasks, assign them to team members, and closely follow due dates and priorities across task categories.
View and navigate your assignments (educator) Manage assignments on a mobile device. Grade, return, and reassign assignments. Delete an assignment in Microsoft Teams. Learn how to create, edit, and turn in assignments using the Assignments app in Microsoft Teams for Education. Tips for due dates, grading rubrics, and adding files, too.