- Java Arrays
- Java Strings
- Java Collection
- Java 8 Tutorial
- Java Multithreading
- Java Exception Handling
- Java Programs
- Java Project
- Java Collections Interview
- Java Interview Questions
- Spring Boot
- Java Tutorial
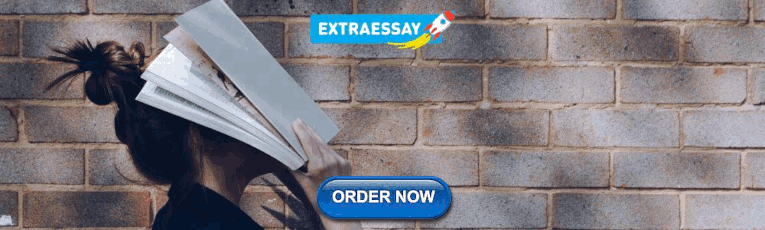
Overview of Java
- Introduction to Java
- The Complete History of Java Programming Language
- C++ vs Java vs Python
- How to Download and Install Java for 64 bit machine?
- Setting up the environment in Java
- How to Download and Install Eclipse on Windows?
- JDK in Java
- How JVM Works - JVM Architecture?
- Differences between JDK, JRE and JVM
- Just In Time Compiler
- Difference between JIT and JVM in Java
- Difference between Byte Code and Machine Code
- How is Java platform independent?
Basics of Java
- Java Basic Syntax
- Java Hello World Program
- Java Data Types
- Primitive data type vs. Object data type in Java with Examples
- Java Identifiers
Operators in Java
- Java Variables
- Scope of Variables In Java
Wrapper Classes in Java
Input/output in java.
- How to Take Input From User in Java?
- Scanner Class in Java
- Java.io.BufferedReader Class in Java
- Difference Between Scanner and BufferedReader Class in Java
- Ways to read input from console in Java
- System.out.println in Java
- Difference between print() and println() in Java
- Formatted Output in Java using printf()
- Fast I/O in Java in Competitive Programming
Flow Control in Java
- Decision Making in Java (if, if-else, switch, break, continue, jump)
- Java if statement with Examples
- Java if-else
- Java if-else-if ladder with Examples
- Loops in Java
- For Loop in Java
- Java while loop with Examples
- Java do-while loop with Examples
- For-each loop in Java
- Continue Statement in Java
- Break statement in Java
- Usage of Break keyword in Java
- return keyword in Java
- Java Arithmetic Operators with Examples
- Java Unary Operator with Examples
Java Assignment Operators with Examples
- Java Relational Operators with Examples
- Java Logical Operators with Examples
- Java Ternary Operator with Examples
- Bitwise Operators in Java
- Strings in Java
- String class in Java
- Java.lang.String class in Java | Set 2
- Why Java Strings are Immutable?
- StringBuffer class in Java
- StringBuilder Class in Java with Examples
- String vs StringBuilder vs StringBuffer in Java
- StringTokenizer Class in Java
- StringTokenizer Methods in Java with Examples | Set 2
- StringJoiner Class in Java
- Arrays in Java
- Arrays class in Java
- Multidimensional Arrays in Java
- Different Ways To Declare And Initialize 2-D Array in Java
- Jagged Array in Java
- Final Arrays in Java
- Reflection Array Class in Java
- util.Arrays vs reflect.Array in Java with Examples
OOPS in Java
- Object Oriented Programming (OOPs) Concept in Java
- Why Java is not a purely Object-Oriented Language?
- Classes and Objects in Java
- Naming Conventions in Java
- Java Methods
Access Modifiers in Java
- Java Constructors
- Four Main Object Oriented Programming Concepts of Java
Inheritance in Java
Abstraction in java, encapsulation in java, polymorphism in java, interfaces in java.
- 'this' reference in Java
- Inheritance and Constructors in Java
- Java and Multiple Inheritance
- Interfaces and Inheritance in Java
- Association, Composition and Aggregation in Java
- Comparison of Inheritance in C++ and Java
- abstract keyword in java
- Abstract Class in Java
- Difference between Abstract Class and Interface in Java
- Control Abstraction in Java with Examples
- Difference Between Data Hiding and Abstraction in Java
- Difference between Abstraction and Encapsulation in Java with Examples
- Difference between Inheritance and Polymorphism
- Dynamic Method Dispatch or Runtime Polymorphism in Java
- Difference between Compile-time and Run-time Polymorphism in Java
Constructors in Java
- Copy Constructor in Java
- Constructor Overloading in Java
- Constructor Chaining In Java with Examples
- Private Constructors and Singleton Classes in Java
Methods in Java
- Static methods vs Instance methods in Java
- Abstract Method in Java with Examples
- Overriding in Java
- Method Overloading in Java
- Difference Between Method Overloading and Method Overriding in Java
- Differences between Interface and Class in Java
- Functional Interfaces in Java
- Nested Interface in Java
- Marker interface in Java
- Comparator Interface in Java with Examples
- Need of Wrapper Classes in Java
- Different Ways to Create the Instances of Wrapper Classes in Java
- Character Class in Java
- Java.Lang.Byte class in Java
- Java.Lang.Short class in Java
- Java.lang.Integer class in Java
- Java.Lang.Long class in Java
- Java.Lang.Float class in Java
- Java.Lang.Double Class in Java
- Java.lang.Boolean Class in Java
- Autoboxing and Unboxing in Java
- Type conversion in Java with Examples
Keywords in Java
- Java Keywords
- Important Keywords in Java
- Super Keyword in Java
- final Keyword in Java
- static Keyword in Java
- enum in Java
- transient keyword in Java
- volatile Keyword in Java
- final, finally and finalize in Java
- Public vs Protected vs Package vs Private Access Modifier in Java
- Access and Non Access Modifiers in Java
Memory Allocation in Java
- Java Memory Management
- How are Java objects stored in memory?
- Stack vs Heap Memory Allocation
- How many types of memory areas are allocated by JVM?
- Garbage Collection in Java
- Types of JVM Garbage Collectors in Java with implementation details
- Memory leaks in Java
- Java Virtual Machine (JVM) Stack Area
Classes of Java
- Understanding Classes and Objects in Java
- Singleton Method Design Pattern in Java
- Object Class in Java
- Inner Class in Java
- Throwable Class in Java with Examples
Packages in Java
- Packages In Java
- How to Create a Package in Java?
- Java.util Package in Java
- Java.lang package in Java
- Java.io Package in Java
- Java Collection Tutorial
Exception Handling in Java
- Exceptions in Java
- Types of Exception in Java with Examples
- Checked vs Unchecked Exceptions in Java
- Java Try Catch Block
- Flow control in try catch finally in Java
- throw and throws in Java
- User-defined Custom Exception in Java
- Chained Exceptions in Java
- Null Pointer Exception In Java
- Exception Handling with Method Overriding in Java
- Multithreading in Java
- Lifecycle and States of a Thread in Java
- Java Thread Priority in Multithreading
- Main thread in Java
- Java.lang.Thread Class in Java
- Runnable interface in Java
- Naming a thread and fetching name of current thread in Java
- What does start() function do in multithreading in Java?
- Difference between Thread.start() and Thread.run() in Java
- Thread.sleep() Method in Java With Examples
- Synchronization in Java
- Importance of Thread Synchronization in Java
- Method and Block Synchronization in Java
- Lock framework vs Thread synchronization in Java
- Difference Between Atomic, Volatile and Synchronized in Java
- Deadlock in Java Multithreading
- Deadlock Prevention And Avoidance
- Difference Between Lock and Monitor in Java Concurrency
- Reentrant Lock in Java
File Handling in Java
- Java.io.File Class in Java
- Java Program to Create a New File
- Different ways of Reading a text file in Java
- Java Program to Write into a File
- Delete a File Using Java
- File Permissions in Java
- FileWriter Class in Java
- Java.io.FileDescriptor in Java
- Java.io.RandomAccessFile Class Method | Set 1
- Regular Expressions in Java
- Regex Tutorial - How to write Regular Expressions?
- Matcher pattern() method in Java with Examples
- Pattern pattern() method in Java with Examples
- Quantifiers in Java
- java.lang.Character class methods | Set 1
- Java IO : Input-output in Java with Examples
- Java.io.Reader class in Java
- Java.io.Writer Class in Java
- Java.io.FileInputStream Class in Java
- FileOutputStream in Java
- Java.io.BufferedOutputStream class in Java
- Java Networking
- TCP/IP Model
- User Datagram Protocol (UDP)
- Differences between IPv4 and IPv6
- Difference between Connection-oriented and Connection-less Services
- Socket Programming in Java
- java.net.ServerSocket Class in Java
- URL Class in Java with Examples
JDBC - Java Database Connectivity
- Introduction to JDBC (Java Database Connectivity)
- JDBC Drivers
- Establishing JDBC Connection in Java
- Types of Statements in JDBC
- JDBC Tutorial
- Java 8 Features - Complete Tutorial
Operators constitute the basic building block of any programming language. Java too provides many types of operators which can be used according to the need to perform various calculations and functions, be it logical, arithmetic, relational, etc. They are classified based on the functionality they provide.
Types of Operators:
- Arithmetic Operators
- Unary Operators
- Assignment Operator
- Relational Operators
- Logical Operators
- Ternary Operator
- Bitwise Operators
- Shift Operators
This article explains all that one needs to know regarding Assignment Operators.
Assignment Operators
These operators are used to assign values to a variable. The left side operand of the assignment operator is a variable, and the right side operand of the assignment operator is a value. The value on the right side must be of the same data type of the operand on the left side. Otherwise, the compiler will raise an error. This means that the assignment operators have right to left associativity, i.e., the value given on the right-hand side of the operator is assigned to the variable on the left. Therefore, the right-hand side value must be declared before using it or should be a constant. The general format of the assignment operator is,
Types of Assignment Operators in Java
The Assignment Operator is generally of two types. They are:
1. Simple Assignment Operator: The Simple Assignment Operator is used with the “=” sign where the left side consists of the operand and the right side consists of a value. The value of the right side must be of the same data type that has been defined on the left side.
2. Compound Assignment Operator: The Compound Operator is used where +,-,*, and / is used along with the = operator.
Let’s look at each of the assignment operators and how they operate:
1. (=) operator:
This is the most straightforward assignment operator, which is used to assign the value on the right to the variable on the left. This is the basic definition of an assignment operator and how it functions.
Syntax:
Example:
2. (+=) operator:
This operator is a compound of ‘+’ and ‘=’ operators. It operates by adding the current value of the variable on the left to the value on the right and then assigning the result to the operand on the left.
Note: The compound assignment operator in Java performs implicit type casting. Let’s consider a scenario where x is an int variable with a value of 5. int x = 5; If you want to add the double value 4.5 to the integer variable x and print its value, there are two methods to achieve this: Method 1: x = x + 4.5 Method 2: x += 4.5 As per the previous example, you might think both of them are equal. But in reality, Method 1 will throw a runtime error stating the “i ncompatible types: possible lossy conversion from double to int “, Method 2 will run without any error and prints 9 as output.
Reason for the Above Calculation
Method 1 will result in a runtime error stating “incompatible types: possible lossy conversion from double to int.” The reason is that the addition of an int and a double results in a double value. Assigning this double value back to the int variable x requires an explicit type casting because it may result in a loss of precision. Without the explicit cast, the compiler throws an error. Method 2 will run without any error and print the value 9 as output. The compound assignment operator += performs an implicit type conversion, also known as an automatic narrowing primitive conversion from double to int . It is equivalent to x = (int) (x + 4.5) , where the result of the addition is explicitly cast to an int . The fractional part of the double value is truncated, and the resulting int value is assigned back to x . It is advisable to use Method 2 ( x += 4.5 ) to avoid runtime errors and to obtain the desired output.
Same automatic narrowing primitive conversion is applicable for other compound assignment operators as well, including -= , *= , /= , and %= .
3. (-=) operator:
This operator is a compound of ‘-‘ and ‘=’ operators. It operates by subtracting the variable’s value on the right from the current value of the variable on the left and then assigning the result to the operand on the left.
4. (*=) operator:
This operator is a compound of ‘*’ and ‘=’ operators. It operates by multiplying the current value of the variable on the left to the value on the right and then assigning the result to the operand on the left.
5. (/=) operator:
This operator is a compound of ‘/’ and ‘=’ operators. It operates by dividing the current value of the variable on the left by the value on the right and then assigning the quotient to the operand on the left.
6. (%=) operator:
This operator is a compound of ‘%’ and ‘=’ operators. It operates by dividing the current value of the variable on the left by the value on the right and then assigning the remainder to the operand on the left.
Please Login to comment...
Similar reads.
- Java-Operators
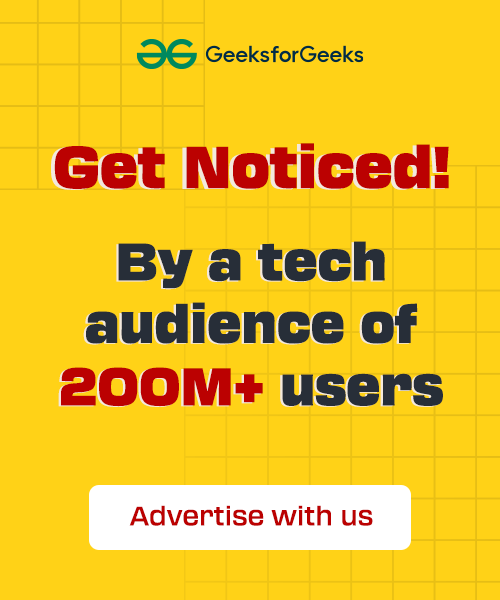
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
The Java Tutorials have been written for JDK 8. Examples and practices described in this page don't take advantage of improvements introduced in later releases and might use technology no longer available. See Java Language Changes for a summary of updated language features in Java SE 9 and subsequent releases. See JDK Release Notes for information about new features, enhancements, and removed or deprecated options for all JDK releases.
Assignment, Arithmetic, and Unary Operators
The simple assignment operator.
One of the most common operators that you'll encounter is the simple assignment operator " = ". You saw this operator in the Bicycle class; it assigns the value on its right to the operand on its left:
This operator can also be used on objects to assign object references , as discussed in Creating Objects .
The Arithmetic Operators
The Java programming language provides operators that perform addition, subtraction, multiplication, and division. There's a good chance you'll recognize them by their counterparts in basic mathematics. The only symbol that might look new to you is " % ", which divides one operand by another and returns the remainder as its result.
The following program, ArithmeticDemo , tests the arithmetic operators.
This program prints the following:
You can also combine the arithmetic operators with the simple assignment operator to create compound assignments . For example, x+=1; and x=x+1; both increment the value of x by 1.
The + operator can also be used for concatenating (joining) two strings together, as shown in the following ConcatDemo program:
By the end of this program, the variable thirdString contains "This is a concatenated string.", which gets printed to standard output.
The Unary Operators
The unary operators require only one operand; they perform various operations such as incrementing/decrementing a value by one, negating an expression, or inverting the value of a boolean.
The following program, UnaryDemo , tests the unary operators:
The increment/decrement operators can be applied before (prefix) or after (postfix) the operand. The code result++; and ++result; will both end in result being incremented by one. The only difference is that the prefix version ( ++result ) evaluates to the incremented value, whereas the postfix version ( result++ ) evaluates to the original value. If you are just performing a simple increment/decrement, it doesn't really matter which version you choose. But if you use this operator in part of a larger expression, the one that you choose may make a significant difference.
The following program, PrePostDemo , illustrates the prefix/postfix unary increment operator:
About Oracle | Contact Us | Legal Notices | Terms of Use | Your Privacy Rights
Copyright © 1995, 2022 Oracle and/or its affiliates. All rights reserved.

Java Tutorial
Control statements, java object class, java inheritance, java polymorphism, java abstraction, java encapsulation, java oops misc.
- Send your Feedback to [email protected]
Help Others, Please Share

Learn Latest Tutorials

Transact-SQL

Reinforcement Learning

R Programming

React Native

Python Design Patterns

Python Pillow

Python Turtle

Preparation

Verbal Ability

Interview Questions

Company Questions
Trending Technologies

Artificial Intelligence

Cloud Computing

Data Science

Machine Learning

B.Tech / MCA

Data Structures

Operating System

Computer Network

Compiler Design

Computer Organization

Discrete Mathematics

Ethical Hacking

Computer Graphics

Software Engineering

Web Technology

Cyber Security

C Programming

Control System

Data Mining

Data Warehouse

Java Tutorial
Java methods, java classes, java file handling, java how to, java reference, java examples, java operators.
Operators are used to perform operations on variables and values.
In the example below, we use the + operator to add together two values:
Try it Yourself »
Although the + operator is often used to add together two values, like in the example above, it can also be used to add together a variable and a value, or a variable and another variable:
Java divides the operators into the following groups:
- Arithmetic operators
- Assignment operators
- Comparison operators
- Logical operators
- Bitwise operators
Arithmetic Operators
Arithmetic operators are used to perform common mathematical operations.
Advertisement
Java Assignment Operators
Assignment operators are used to assign values to variables.
In the example below, we use the assignment operator ( = ) to assign the value 10 to a variable called x :
The addition assignment operator ( += ) adds a value to a variable:
A list of all assignment operators:
Java Comparison Operators
Comparison operators are used to compare two values (or variables). This is important in programming, because it helps us to find answers and make decisions.
The return value of a comparison is either true or false . These values are known as Boolean values , and you will learn more about them in the Booleans and If..Else chapter.
In the following example, we use the greater than operator ( > ) to find out if 5 is greater than 3:
Java Logical Operators
You can also test for true or false values with logical operators.
Logical operators are used to determine the logic between variables or values:
Java Bitwise Operators
Bitwise operators are used to perform binary logic with the bits of an integer or long integer.
Note: The Bitwise examples above use 4-bit unsigned examples, but Java uses 32-bit signed integers and 64-bit signed long integers. Because of this, in Java, ~5 will not return 10. It will return -6. ~00000000000000000000000000000101 will return 11111111111111111111111111111010
In Java, 9 >> 1 will not return 12. It will return 4. 00000000000000000000000000001001 >> 1 will return 00000000000000000000000000000100
Test Yourself With Exercises
Multiply 10 with 5 , and print the result.
Start the Exercise

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
Java Assignment Operators
Java programming tutorial index.
The Java Assignment Operators are used when you want to assign a value to the expression. The assignment operator denoted by the single equal sign = .
In a Java assignment statement, any expression can be on the right side and the left side must be a variable name. For example, this does not mean that "a" is equal to "b", instead, it means assigning the value of 'b' to 'a'. It is as follows:
Java also has the facility of chain assignment operators, where we can specify a single value for multiple variables.
- Enterprise Java
- Web-based Java
- Data & Java
- Project Management
- Visual Basic
- Ruby / Rails
- Java Mobile
- Architecture & Design
- Open Source
- Web Services

Developer.com content and product recommendations are editorially independent. We may make money when you click on links to our partners. Learn More .

Java provides many types of operators to perform a variety of calculations and functions, such as logical , arithmetic , relational , and others. With so many operators to choose from, it helps to group them based on the type of functionality they provide. This programming tutorial will focus on Java’s numerous a ssignment operators.
Before we begin, however, you may want to bookmark our other tutorials on Java operators, which include:
- Arithmetic Operators
- Comparison Operators
- Conditional Operators
- Logical Operators
- Bitwise and Shift Operators
Assignment Operators in Java
As the name conveys, assignment operators are used to assign values to a variable using the following syntax:
The left side operand of the assignment operator must be a variable, whereas the right side operand of the assignment operator may be a literal value or another variable. Moreover, the value or variable on the right side must be of the same data type of the operand on the left side. Otherwise, the compiler will raise an error. Assignment operators have a right to left associativity in that the value given on the right-hand side of the operator is assigned to the variable on the left. Therefore, the right-hand side variable must be declared before assignment.
You can learn more about variables in our programming tutorial: Working with Java Variables .
Types of Assignment Operators in Java
Java assignment operators are classified into two types: simple and compound .
The Simple assignment operator is the equals ( = ) sign, which is the most straightforward of the bunch. It simply assigns the value or variable on the right to the variable on the left.
Compound operators are comprised of both an arithmetic, bitwise, or shift operator in addition to the equals ( = ) sign.
Equals Operator (=) Java Example
First, let’s learn to use the one-and-only simple assignment operator – the Equals ( = ) operator – with the help of a Java program. It includes two assignments: a literal value to num1 and the num1 variable to num2 , after which both are printed to the console to show that the values have been assigned to the numbers:
The += Operator Java Example
A compound of the + and = operators, the += adds the current value of the variable on the left to the value on the right before assigning the result to the operand on the left. Here is some sample code to demonstrate how to use the += operator in Java:
The -= Operator Java Example
Made up of the – and = operators, the -= first subtracts the variable’s value on the right from the current value of the variable on the left before assigning the result to the operand on the left. We can see it at work below in the following code example showing how to decrement in Java using the -= operator:
The *= Operator Java Example
This Java operator is comprised of the * and = operators. It operates by multiplying the current value of the variable on the left to the value on the right and then assigning the result to the operand on the left. Here’s a program that shows the *= operator in action:
The /= Operator Java Example
A combination of the / and = operators, the /= Operator divides the current value of the variable on the left by the value on the right and then assigns the quotient to the operand on the left. Here is some example code showing how to use the /= operator in Java:
%= Operator Java Example
The %= operator includes both the % and = operators. As seen in the program below, it divides the current value of the variable on the left by the value on the right and then assigns the remainder to the operand on the left:
Compound Bitwise and Shift Operators in Java
The Bitwise and Shift Operators that we just recently covered can also be utilized in compound form as seen in the list below:
- &= – Compound bitwise Assignment operator.
- ^= – Compound bitwise ^ assignment operator.
- >>= – Compound right shift assignment operator.
- >>>= – Compound right shift filled 0 assignment operator.
- <<= – Compound left shift assignment operator.
The following program demonstrates the working of all the Compound Bitwise and Shift Operators :
Final Thoughts on Java Assignment Operators
This programming tutorial presented an overview of Java’s simple and compound assignment Operators. An essential building block to any programming language, developers would be unable to store any data in their programs without them. Though not quite as indispensable as the equals operator, compound operators are great time savers, allowing you to perform arithmetic and bitwise operations and assignment in a single line of code.
Read more Java programming tutorials and guides to software development .
Get the Free Newsletter!
Subscribe to Developer Insider for top news, trends & analysis
Latest Posts
What is the role of a project manager in software development, how to use optional in java, overview of the jad methodology, microsoft project tips and tricks, how to become a project manager in 2023, related stories, understanding types of thread synchronization errors in java, understanding memory consistency in java threads.
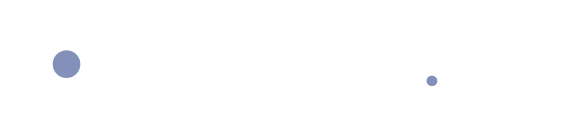
- Java - Home
- Java - Overview
- Java - History
- Java - Features
- Java 8 - New Features
- Java 9 - New Features
- Java vs C++
- Java Virtual Machine(JVM)
- Java - JDK vs JRE vs JVM
- Java - Hello World Program
- Java - Environment Setup
- Java - Basic Syntax
- Java - Variable Types
- Java - Data Types
- Java - Type Casting
- Java - Unicode System
- Java - Basic Operators
- Java - Comments
- Java - Streams
- Java - Datetime Api
- Java - User Input
- Java - Loop Control
- Java - Decision Making
- Java - If-else
- Java - Switch
- Java - For Loops
- Java - For-Each Loops
- Java - While Loops
- Java - do-while Loops
- Java - Break
- Java - Continue
- Java - OOPs Concepts
- Java - Object & Classes
- Java - Class Attributes
- Java - Class Methods
- Java - Methods
- Java - Variables Scope
- Java - Constructors
- Java - Access Modifiers
- Java - Inheritance
- Java - Aggregation
- Java - Polymorphism
- Java - Overriding
- Java - Method Overloading
- Java - Dynamic Binding
- Java - Static Binding
- Java - Instance Initializer Block
- Java - Abstraction
- Java - Encapsulation
- Java - Interfaces
- Java - Packages
- Java - Inner Classes
- Java - Static Class
- Java - Anonymous Class
- Java - Singleton Class
- Java - Wrapper Classes
- Java - Enums
- Java - Enum Constructor
- Java - Enum Strings
- Java - Reflection
- Java - Number
- Java - Boolean
- Java - Characters
- Java - Strings
- Java - Arrays
- Java - Date & Time
- Java - Math Class
- Java - Files
- Java - Create a File
- Java - Write to File
- Java - Read Files
- Java - Delete Files
- Java - Directories
- Java - I/O Streams
- Java - Exceptions
- Java - try-catch Block
- Java - try-with-resources
- Java - Multi-catch Block
- Java - Nested try Block
- Java - Finally Block
- Java - throw Exception
- Java - Exception Propagation
- Java - Built-in Exceptions
- Java - Custom Exception
- Java - Annotations
- Java - Logging
- Java - Assertions
- Java - Multithreading
- Java - Thread Life Cycle
- Java - Creating a Thread
- Java - Starting a Thread
- Java - Joining Threads
- Java - Naming Thread
- Java - Thread Scheduler
- Java - Thread Pools
- Java - Main Thread
- Java - Thread Priority
- Java - Daemon Threads
- Java - Thread Group
- Java - Shutdown Hook
- Java - Synchronization
- Java - Block Synchronization
- Java - Static Synchronization
- Java - Inter-thread Communication
- Java - Thread Deadlock
- Java - Interrupting a Thread
- Java - Thread Control
- Java - Reentrant Monitor
- Java - Networking
- Java - Socket Programming
- Java - URL Processing
- Java - URL Class
- Java - URLConnection Class
- Java - HttpURLConnection Class
- Java - Socket Class
- Java - ServerSocket Class
- Java - InetAddress Class
- Java - Generics
- Java - Collections
- Java - Collection Interface
- Java - List Interface
- Java - ArrayList
- Java - Vector Class
- Java - Stack Class
- Java - Queue Interface
- Java - PriorityQueue
- Java - Deque Interface
- Java - LinkedList
- Java - ArrayDeque
- Java - Map Interface
- Java - HashMap
- Java - LinkedHashMap
- Java - WeakHashMap
- Java - EnumMap
- Java - SortedMap Interface
- Java - TreeMap
- Java - The IdentityHashMap Class
- Java - Set Interface
- Java - HashSet
- Java - EnumSet
- Java - LinkedHashSet
- Java - SortedSet Interface
- Java - TreeSet
- Java - Data Structures
- Java - Enumeration
- Java - BitSet Class
- Java - Dictionary
- Java - Hashtable
- Java - Properties
- Java - Collections Algorithms
- Java - Iterators
- Java - Comparators
- Java - Comparable Interface in Java
- Java - Recursion
- Java - Regular Expressions
- Java - Serialization
- Java - Process API Improvements
- Java - Stream API Improvements
- Java - Enhanced @Deprecated Annotation
- Java - CompletableFuture API Improvements
- Java - Maths Methods
- Java - Array Methods
- Java - Keywords Reference
- Java - Command-Line Arguments
- Java - Lambda Expressions
- Java - Sending Email
- Java - Applet Basics
- Java - Javadoc Comments
- Java - Autoboxing and Unboxing
- Java - File Mismatch Method
- Java - REPL (JShell)
- Java - Multi-Release Jar Files
- Java - Private Interface Methods
- Java - Inner Class Diamond Operator
- Java - Multiresolution Image API
- Java - Collection Factory Methods
- Java - Module System
- Java - Nashorn JavaScript
- Java - Optional Class
- Java - Method References
- Java - Functional Interfaces
- Java - Default Methods
- Java - Base64 Encode Decode
- Java - Switch Expressions
- Java - Teeing Collectors
- Java - Microbenchmark
- Java - Text Blocks
- Java - Dynamic CDS archive
- Java - Z Garbage Collector (ZGC)
- Java - Null Pointer Exception
- Java - Packaging Tools
- Java - NUMA Aware G1
- Java - Sealed Classes
- Java - Record Classes
- Java - Hidden Classes
- Java - Pattern Matching
- Java - Compact Number Formatting
- Java - Programming Examples
- JDBC Tutorial
- SWING Tutorial
- AWT Tutorial
- Servlets Tutorial
- JSP Tutorial
- Java Compiler
- Java - Questions and Answers
- Java 8 - Questions and Answers
- Java - Quick Guide
- Java - Useful Resources
- Java - Discussion
- Java - Examples
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
Java - Assignment Operators with Examples
Java assignment operators.
Following are the assignment operators supported by Java language −
The following programs are simple examples which demonstrate the assignment operators. Copy and paste the following Java programs as Test.java file, and compile and run the programs −
In this example, we're creating three variables a,b and c and using assignment operators . We've performed simple assignment, addition AND assignment, subtraction AND assignment and multiplication AND assignment operations and printed the results.
In this example, we're creating two variables a and c and using assignment operators . We've performed Divide AND assignment, Multiply AND assignment, Modulus AND assignment, bitwise exclusive OR AND assignment, OR AND assignment operations and printed the results.
In this example, we're creating two variables a and c and using assignment operators . We've performed Left shift AND assignment, Right shift AND assignment, operations and printed the results.
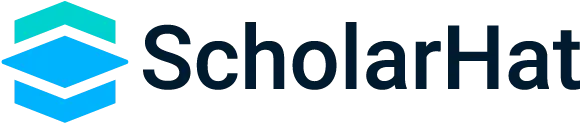
01 Career Opportunities
- Top 50 Java Interview Questions and Answers
- Java Developer Salary Guide in India – For Freshers & Experienced
02 Beginner
- Hierarchical Inheritance in Java
- Arithmetic operators in Java
- Unary operator in Java
- Relational operators in Java
Assignment operator in Java
- Logical operators in Java
- Primitive Data Types in Java
- Multiple Inheritance in Java
- Parameterized Constructor in Java
- Constructor Chaining in Java
- What is a Bitwise Operator in Java? Type, Example and More
- Constructor Overloading in Java
- Ternary Operator in Java
- For Loop in Java: Its Types and Examples
- Best Java Developer Roadmap 2024
- While Loop in Java
- What are Copy Constructors In Java? Explore Types,Examples & Use
- Do-While Loop in Java
- Hybrid Inheritance in Java
- Single Inheritance in Java
- Top 10 Reasons to know why Java is Important?
- What is Java? A Beginners Guide to Java
- Differences between JDK, JRE, and JVM: Java Toolkit
- Variables in Java: Local, Instance and Static Variables
- Conditional Statements in Java: If, If-Else and Switch Statement
- Data Types in Java - Primitive and Non-Primitive Data Types
- What are Operators in Java - Types of Operators in Java ( With Examples )
- Java VS Python
- Looping Statements in Java - For, While, Do-While Loop in Java
- Jump Statements in JAVA - Types of Statements in JAVA (With Examples)
- Java Arrays: Single Dimensional and Multi-Dimensional Arrays
- What is String in Java - Java String Types and Methods (With Examples)
03 Intermediate
- OOPs Concepts in Java: Encapsulation, Abstraction, Inheritance, Polymorphism
- What is Class in Java? - Objects and Classes in Java {Explained}
- Access Modifiers in Java: Default, Private, Public, Protected
- Constructors in Java: Types of Constructors with Examples
- Polymorphism in Java: Compile time and Runtime Polymorphism
- Abstract Class in Java: Concepts, Examples, and Usage
- What is Inheritance in Java: Types of Inheritance in Java
- Exception handling in Java: Try, Catch, Finally, Throw and Throws
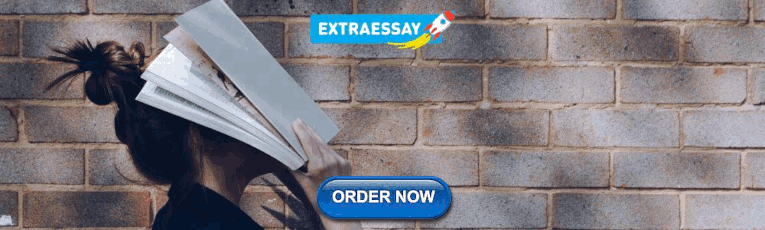
04 Training Programs
- Java Programming Course
- C++ Programming Course
- MERN: Full-Stack Web Developer Certification Training
- Data Structures and Algorithms Training
- Assignment Operator In Ja..
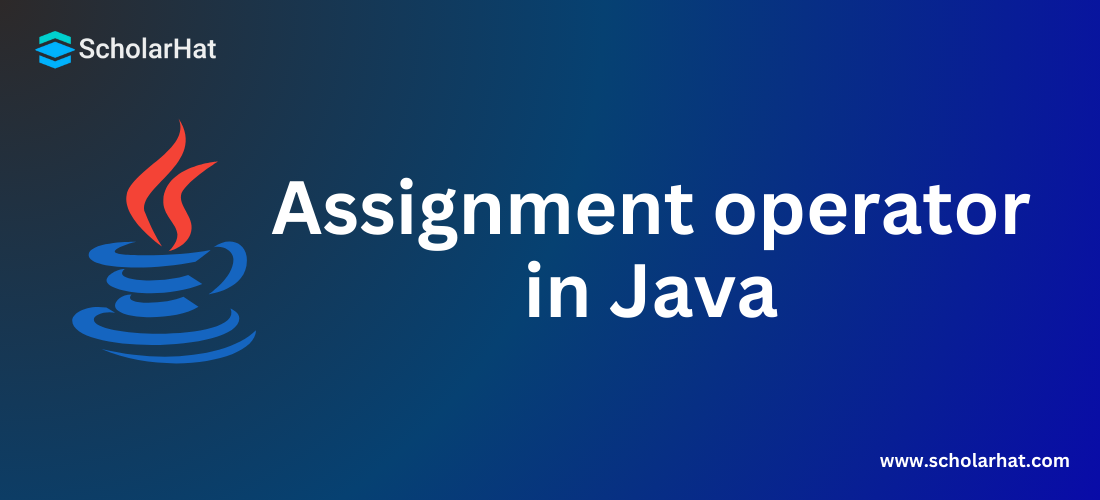
Java Programming For Beginners Free Course
Assignment operators in java: an overview.
We already discussed the Types of Operators in the previous tutorial Java. In this Java tutorial , we will delve into the different types of assignment operators in Java, and their syntax, and provide examples for better understanding. Because Java is a flexible and widely used programming language. Assignment operators play a crucial role in manipulating and assigning values to variables. To further enhance your understanding and application of Java assignment operator's concepts, consider enrolling in the best Java Certification Course .
What are the Assignment Operators in Java?
Assignment operators in Java are used to assign values to variables . They are classified into two main types: simple assignment operator and compound assignment operator.
The general syntax for a simple assignment statement is:
And for a compound assignment statement:
Read More - Advanced Java Interview Questions
Types of Assignment Operators in Java
- Simple Assignment Operator: The Simple Assignment Operator is used with the "=" sign, where the operand is on the left side and the value is on the right. The right-side value must be of the same data type as that defined on the left side.
- Compound Assignment Operator: Compound assignment operators combine arithmetic operations with assignments. They provide a concise way to perform an operation and assign the result to the variable in one step. The Compound Operator is utilized when +,-,*, and / are used in conjunction with the = operator.
1. Simple Assignment Operator (=):
The equal sign (=) is the basic assignment operator in Java. It is used to assign the value on the right-hand side to the variable on the left-hand side.
Explanation
2. addition assignment operator (+=) :, 3. subtraction operator (-=):, 4. multiplication operator (*=):.
Read More - Java Developer Salary
5. Division Operator (/=):
6. modulus assignment operator (%=):, example of assignment operator in java.
Let's look at a few examples in our Java Playground to illustrate the usage of assignment operators in Java:
- Unary Operator in Java
- Arithmetic Operators in Java
- Relational Operators in Java
- Logical Operators in Java
Q1. Can I use multiple assignment operators in a single statement?
Q2. are there any other compound assignment operators in java, q3. how many types of assignment operators.
- 1. (=) operator
- 1. (+=) operator
- 2. (-=) operator
- 3. (*=) operator
- 4. (/=) operator
- 5. (%=) operator
About Author
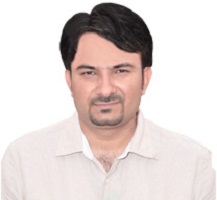
- 22+ Video Courses
- 750+ Hands-On Labs
- 300+ Quick Notes
- 55+ Skill Tests
- 45+ Interview Q&A Courses
- 10+ Real-world Projects
- Career Coaching Sessions
- Email Support
Upcoming Master Classes
We use cookies to make interactions with our websites and services easy and meaningful. Please read our Privacy Policy for more details.
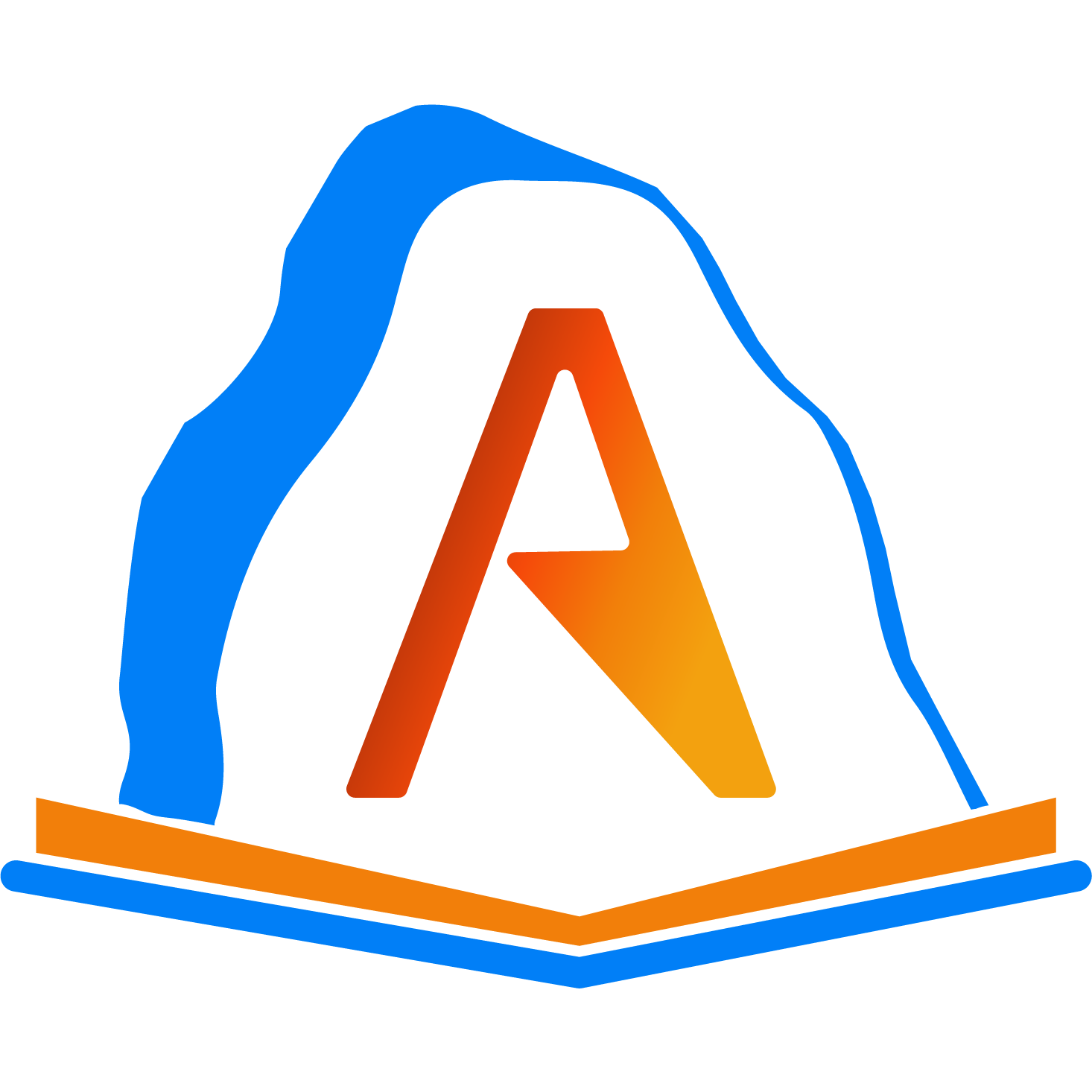
- Table of Contents
- Course Home
- Assignments
- Peer Instruction (Instructor)
- Peer Instruction (Student)
- Change Course
- Instructor's Page
- Progress Page
- Edit Profile
- Change Password
- Scratch ActiveCode
- Scratch Activecode
- Instructors Guide
- About Runestone
- Report A Problem
- 1.1 Preface
- 1.2 Why Programming? Why Java?
- 1.3 Variables and Data Types
- 1.4 Expressions and Assignment Statements
- 1.5 Compound Assignment Operators
- 1.6 Casting and Ranges of Variables
- 1.7 Java Development Environments (optional)
- 1.8 Unit 1 Summary
- 1.9 Unit 1 Mixed Up Code Practice
- 1.10 Unit 1 Coding Practice
- 1.11 Multiple Choice Exercises
- 1.12 Lesson Workspace
- 1.3. Variables and Data Types" data-toggle="tooltip">
- 1.5. Compound Assignment Operators' data-toggle="tooltip" >
1.4. Expressions and Assignment Statements ¶
In this lesson, you will learn about assignment statements and expressions that contain math operators and variables.
1.4.1. Assignment Statements ¶
Remember that a variable holds a value that can change or vary. Assignment statements initialize or change the value stored in a variable using the assignment operator = . An assignment statement always has a single variable on the left hand side of the = sign. The value of the expression on the right hand side of the = sign (which can contain math operators and other variables) is copied into the memory location of the variable on the left hand side.
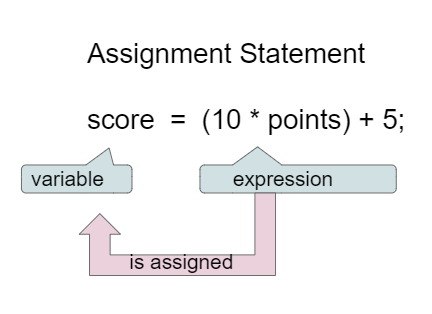
Figure 1: Assignment Statement (variable = expression) ¶
Instead of saying equals for the = operator in an assignment statement, say “gets” or “is assigned” to remember that the variable on the left hand side gets or is assigned the value on the right. In the figure above, score is assigned the value of 10 times points (which is another variable) plus 5.
The following video by Dr. Colleen Lewis shows how variables can change values in memory using assignment statements.
As we saw in the video, we can set one variable to a copy of the value of another variable like y = x;. This won’t change the value of the variable that you are copying from.

Click on the Show CodeLens button to step through the code and see how the values of the variables change.
The program is supposed to figure out the total money value given the number of dimes, quarters and nickels. There is an error in the calculation of the total. Fix the error to compute the correct amount.
Calculate and print the total pay given the weekly salary and the number of weeks worked. Use string concatenation with the totalPay variable to produce the output Total Pay = $3000 . Don’t hardcode the number 3000 in your print statement.

Assume you have a package with a given height 3 inches and width 5 inches. If the package is rotated 90 degrees, you should swap the values for the height and width. The code below makes an attempt to swap the values stored in two variables h and w, which represent height and width. Variable h should end up with w’s initial value of 5 and w should get h’s initial value of 3. Unfortunately this code has an error and does not work. Use the CodeLens to step through the code to understand why it fails to swap the values in h and w.
1-4-7: Explain in your own words why the ErrorSwap program code does not swap the values stored in h and w.
Swapping two variables requires a third variable. Before assigning h = w , you need to store the original value of h in the temporary variable. In the mixed up programs below, drag the blocks to the right to put them in the right order.
The following has the correct code that uses a third variable named “temp” to swap the values in h and w.
The code is mixed up and contains one extra block which is not needed in a correct solution. Drag the needed blocks from the left into the correct order on the right, then check your solution. You will be told if any of the blocks are in the wrong order or if you need to remove one or more blocks.
After three incorrect attempts you will be able to use the Help Me button to make the problem easier.
Fix the code below to perform a correct swap of h and w. You need to add a new variable named temp to use for the swap.
1.4.2. Incrementing the value of a variable ¶
If you use a variable to keep score you would probably increment it (add one to the current value) whenever score should go up. You can do this by setting the variable to the current value of the variable plus one (score = score + 1) as shown below. The formula looks a little crazy in math class, but it makes sense in coding because the variable on the left is set to the value of the arithmetic expression on the right. So, the score variable is set to the previous value of score + 1.
Click on the Show CodeLens button to step through the code and see how the score value changes.
1-4-11: What is the value of b after the following code executes?
- It sets the value for the variable on the left to the value from evaluating the right side. What is 5 * 2?
- Correct. 5 * 2 is 10.
1-4-12: What are the values of x, y, and z after the following code executes?
- x = 0, y = 1, z = 2
- These are the initial values in the variable, but the values are changed.
- x = 1, y = 2, z = 3
- x changes to y's initial value, y's value is doubled, and z is set to 3
- x = 2, y = 2, z = 3
- Remember that the equal sign doesn't mean that the two sides are equal. It sets the value for the variable on the left to the value from evaluating the right side.
- x = 1, y = 0, z = 3
1.4.3. Operators ¶
Java uses the standard mathematical operators for addition ( + ), subtraction ( - ), multiplication ( * ), and division ( / ). Arithmetic expressions can be of type int or double. An arithmetic operation that uses two int values will evaluate to an int value. An arithmetic operation that uses at least one double value will evaluate to a double value. (You may have noticed that + was also used to put text together in the input program above – more on this when we talk about strings.)
Java uses the operator == to test if the value on the left is equal to the value on the right and != to test if two items are not equal. Don’t get one equal sign = confused with two equal signs == ! They mean different things in Java. One equal sign is used to assign a value to a variable. Two equal signs are used to test a variable to see if it is a certain value and that returns true or false as you’ll see below. Use == and != only with int values and not doubles because double values are an approximation and 3.3333 will not equal 3.3334 even though they are very close.
Run the code below to see all the operators in action. Do all of those operators do what you expected? What about 2 / 3 ? Isn’t surprising that it prints 0 ? See the note below.
When Java sees you doing integer division (or any operation with integers) it assumes you want an integer result so it throws away anything after the decimal point in the answer, essentially rounding down the answer to a whole number. If you need a double answer, you should make at least one of the values in the expression a double like 2.0.
With division, another thing to watch out for is dividing by 0. An attempt to divide an integer by zero will result in an ArithmeticException error message. Try it in one of the active code windows above.
Operators can be used to create compound expressions with more than one operator. You can either use a literal value which is a fixed value like 2, or variables in them. When compound expressions are evaluated, operator precedence rules are used, so that *, /, and % are done before + and -. However, anything in parentheses is done first. It doesn’t hurt to put in extra parentheses if you are unsure as to what will be done first.
In the example below, try to guess what it will print out and then run it to see if you are right. Remember to consider operator precedence .
1-4-15: Consider the following code segment. Be careful about integer division.
What is printed when the code segment is executed?
- 0.666666666666667
- Don't forget that division and multiplication will be done first due to operator precedence.
- Yes, this is equivalent to (5 + ((a/b)*c) - 1).
- Don't forget that division and multiplication will be done first due to operator precedence, and that an int/int gives an int result where it is rounded down to the nearest int.
1-4-16: Consider the following code segment.
What is the value of the expression?
- Dividing an integer by an integer results in an integer
- Correct. Dividing an integer by an integer results in an integer
- The value 5.5 will be rounded down to 5
1-4-17: Consider the following code segment.
- Correct. Dividing a double by an integer results in a double
- Dividing a double by an integer results in a double
1-4-18: Consider the following code segment.
- Correct. Dividing an integer by an double results in a double
- Dividing an integer by an double results in a double
1.4.4. The Modulo Operator ¶
The percent sign operator ( % ) is the mod (modulo) or remainder operator. The mod operator ( x % y ) returns the remainder after you divide x (first number) by y (second number) so 5 % 2 will return 1 since 2 goes into 5 two times with a remainder of 1. Remember long division when you had to specify how many times one number went into another evenly and the remainder? That remainder is what is returned by the modulo operator.
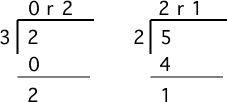
Figure 2: Long division showing the whole number result and the remainder ¶
In the example below, try to guess what it will print out and then run it to see if you are right.
The result of x % y when x is smaller than y is always x . The value y can’t go into x at all (goes in 0 times), since x is smaller than y , so the result is just x . So if you see 2 % 3 the result is 2 .
1-4-21: What is the result of 158 % 10?
- This would be the result of 158 divided by 10. modulo gives you the remainder.
- modulo gives you the remainder after the division.
- When you divide 158 by 10 you get a remainder of 8.
1-4-22: What is the result of 3 % 8?
- 8 goes into 3 no times so the remainder is 3. The remainder of a smaller number divided by a larger number is always the smaller number!
- This would be the remainder if the question was 8 % 3 but here we are asking for the reminder after we divide 3 by 8.
- What is the remainder after you divide 3 by 8?
1.4.5. FlowCharting ¶
Assume you have 16 pieces of pizza and 5 people. If everyone gets the same number of slices, how many slices does each person get? Are there any leftover pieces?
In industry, a flowchart is used to describe a process through symbols and text. A flowchart usually does not show variable declarations, but it can show assignment statements (drawn as rectangle) and output statements (drawn as rhomboid).
The flowchart in figure 3 shows a process to compute the fair distribution of pizza slices among a number of people. The process relies on integer division to determine slices per person, and the mod operator to determine remaining slices.
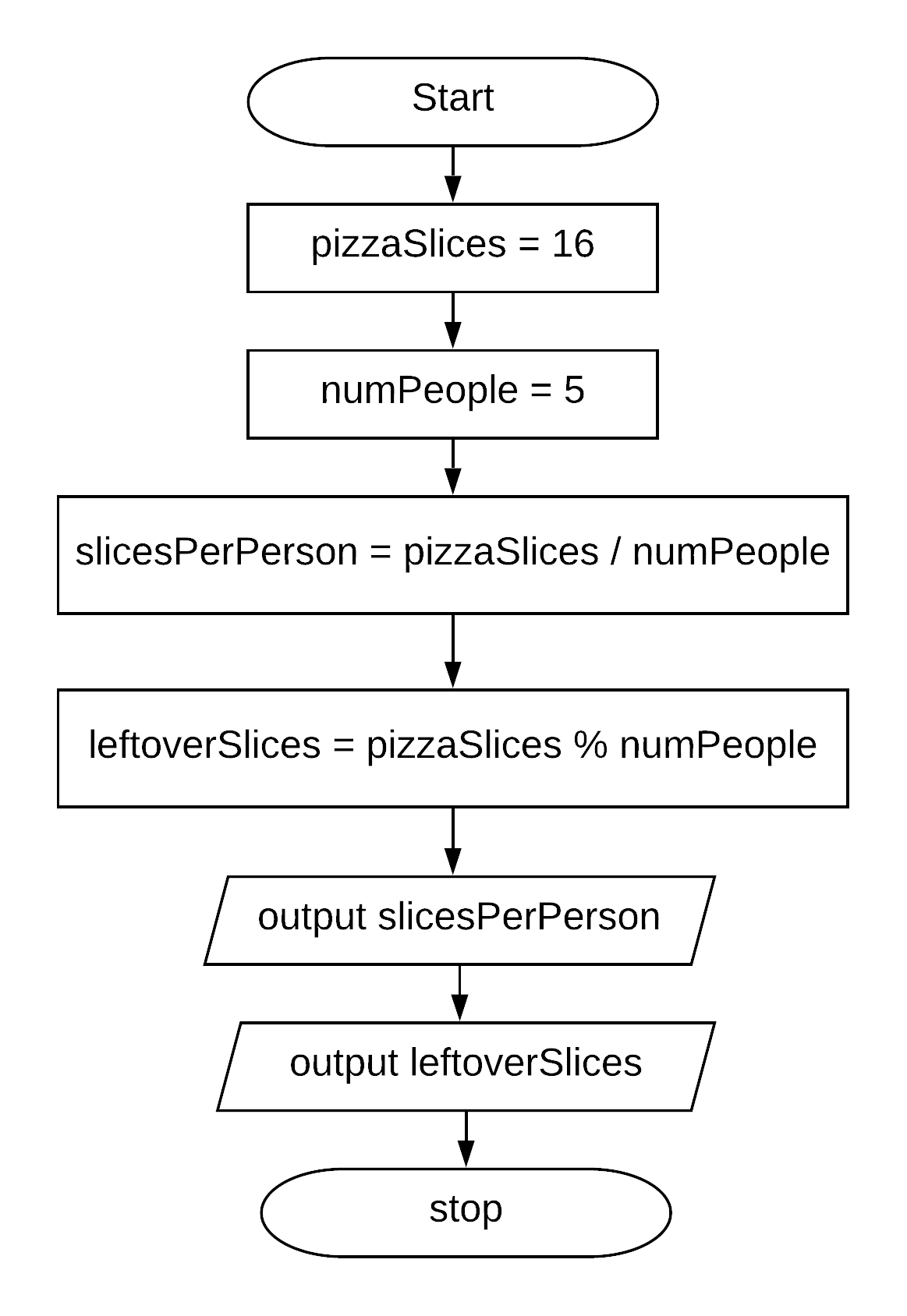
Figure 3: Example Flow Chart ¶
A flowchart shows pseudo-code, which is like Java but not exactly the same. Syntactic details like semi-colons are omitted, and input and output is described in abstract terms.
Complete the program based on the process shown in the Figure 3 flowchart. Note the first line of code declares all 4 variables as type int. Add assignment statements and print statements to compute and print the slices per person and leftover slices. Use System.out.println for output.
1.4.6. Storing User Input in Variables ¶
Variables are a powerful abstraction in programming because the same algorithm can be used with different input values saved in variables.

Figure 4: Program input and output ¶
A Java program can ask the user to type in one or more values. The Java class Scanner is used to read from the keyboard input stream, which is referenced by System.in . Normally the keyboard input is typed into a console window, but since this is running in a browser you will type in a small textbox window displayed below the code. The code below shows an example of prompting the user to enter a name and then printing a greeting. The code String name = scan.nextLine() gets the string value you enter as program input and then stores the value in a variable.
Run the program a few times, typing in a different name. The code works for any name: behold, the power of variables!
Run this program to read in a name from the input stream. You can type a different name in the input window shown below the code.
Try stepping through the code with the CodeLens tool to see how the name variable is assigned to the value read by the scanner. You will have to click “Hide CodeLens” and then “Show in CodeLens” to enter a different name for input.
The Scanner class has several useful methods for reading user input. A token is a sequence of characters separated by white space.
Run this program to read in an integer from the input stream. You can type a different integer value in the input window shown below the code.
A rhomboid (slanted rectangle) is used in a flowchart to depict data flowing into and out of a program. The previous flowchart in Figure 3 used a rhomboid to indicate program output. A rhomboid is also used to denote reading a value from the input stream.
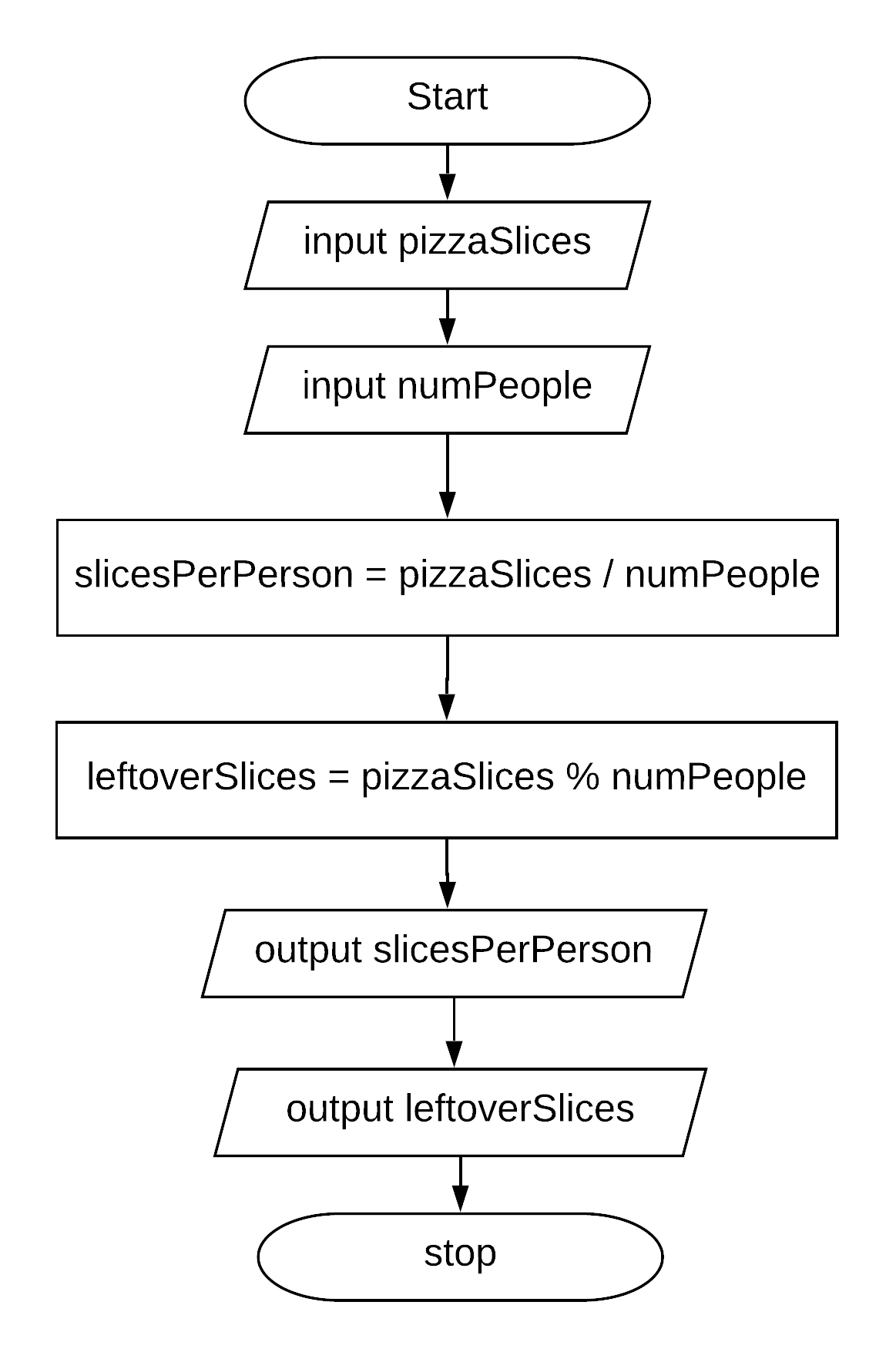
Figure 5: Flow Chart Reading User Input ¶
Figure 5 contains an updated version of the pizza calculator process. The first two steps have been altered to initialize the pizzaSlices and numPeople variables by reading two values from the input stream. In Java this will be done using a Scanner object and reading from System.in.
Complete the program based on the process shown in the Figure 5 flowchart. The program should scan two integer values to initialize pizzaSlices and numPeople. Run the program a few times to experiment with different values for input. What happens if you enter 0 for the number of people? The program will bomb due to division by zero! We will see how to prevent this in a later lesson.
The program below reads two integer values from the input stream and attempts to print the sum. Unfortunately there is a problem with the last line of code that prints the sum.
Run the program and look at the result. When the input is 5 and 7 , the output is Sum is 57 . Both of the + operators in the print statement are performing string concatenation. While the first + operator should perform string concatenation, the second + operator should perform addition. You can force the second + operator to perform addition by putting the arithmetic expression in parentheses ( num1 + num2 ) .
More information on using the Scanner class can be found here https://www.w3schools.com/java/java_user_input.asp
1.4.7. Programming Challenge : Dog Years ¶
In this programming challenge, you will calculate your age, and your pet’s age from your birthdates, and your pet’s age in dog years. In the code below, type in the current year, the year you were born, the year your dog or cat was born (if you don’t have one, make one up!) in the variables below. Then write formulas in assignment statements to calculate how old you are, how old your dog or cat is, and how old they are in dog years which is 7 times a human year. Finally, print it all out.
Calculate your age and your pet’s age from the birthdates, and then your pet’s age in dog years. If you want an extra challenge, try reading the values using a Scanner.
1.4.8. Summary ¶
Arithmetic expressions include expressions of type int and double.
The arithmetic operators consist of +, -, * , /, and % (modulo for the remainder in division).
An arithmetic operation that uses two int values will evaluate to an int value. With integer division, any decimal part in the result will be thrown away, essentially rounding down the answer to a whole number.
An arithmetic operation that uses at least one double value will evaluate to a double value.
Operators can be used to construct compound expressions.
During evaluation, operands are associated with operators according to operator precedence to determine how they are grouped. (*, /, % have precedence over + and -, unless parentheses are used to group those.)
An attempt to divide an integer by zero will result in an ArithmeticException to occur.
The assignment operator (=) allows a program to initialize or change the value stored in a variable. The value of the expression on the right is stored in the variable on the left.
During execution, expressions are evaluated to produce a single value.
The value of an expression has a type based on the evaluation of the expression.
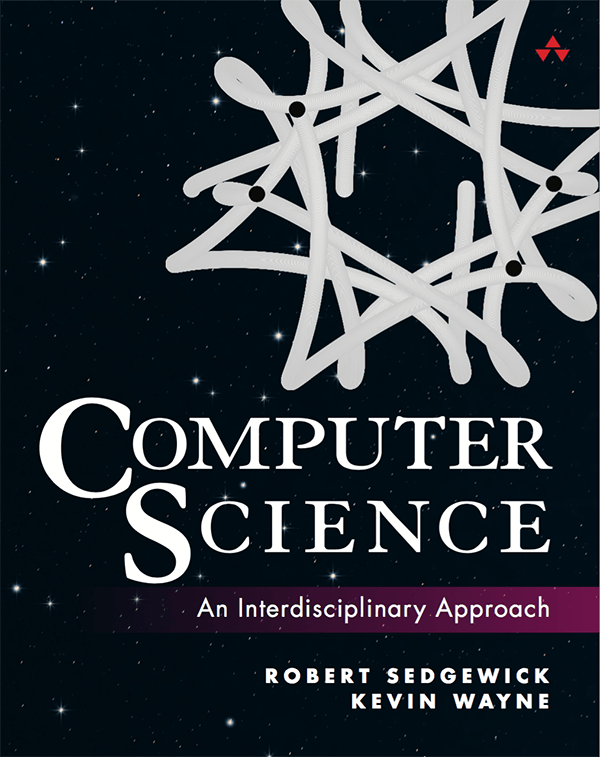
Programming in Java · Computer Science · An Interdisciplinary Approach
Online content. , introduction to programming in java., computer science., for teachers:, for students:.
Browse Course Material
Course info, instructors.
- Adam Marcus
Departments
- Electrical Engineering and Computer Science
As Taught In
- Programming Languages
- Software Design and Engineering
Learning Resource Types
Introduction to programming in java, assignments.

You are leaving MIT OpenCourseWare
Learn Java practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn java interactively, java introduction.
- Get Started With Java
- Your First Java Program
- Java Comments
Java Fundamentals
- Java Variables and Literals
- Java Data Types (Primitive)
Java Operators
- Java Basic Input and Output
- Java Expressions, Statements and Blocks
Java Flow Control
- Java if...else Statement
Java Ternary Operator
- Java for Loop
- Java for-each Loop
- Java while and do...while Loop
- Java break Statement
- Java continue Statement
- Java switch Statement
- Java Arrays
- Java Multidimensional Arrays
- Java Copy Arrays
Java OOP(I)
- Java Class and Objects
- Java Methods
- Java Method Overloading
- Java Constructors
- Java Static Keyword
- Java Strings
- Java Access Modifiers
- Java this Keyword
- Java final keyword
- Java Recursion
Java instanceof Operator
Java OOP(II)
- Java Inheritance
- Java Method Overriding
- Java Abstract Class and Abstract Methods
- Java Interface
- Java Polymorphism
- Java Encapsulation
Java OOP(III)
- Java Nested and Inner Class
- Java Nested Static Class
- Java Anonymous Class
- Java Singleton Class
- Java enum Constructor
- Java enum Strings
- Java Reflection
- Java Package
- Java Exception Handling
- Java Exceptions
- Java try...catch
- Java throw and throws
- Java catch Multiple Exceptions
- Java try-with-resources
- Java Annotations
- Java Annotation Types
- Java Logging
- Java Assertions
- Java Collections Framework
- Java Collection Interface
- Java ArrayList
- Java Vector
- Java Stack Class
- Java Queue Interface
- Java PriorityQueue
- Java Deque Interface
- Java LinkedList
- Java ArrayDeque
- Java BlockingQueue
- Java ArrayBlockingQueue
- Java LinkedBlockingQueue
- Java Map Interface
- Java HashMap
- Java LinkedHashMap
- Java WeakHashMap
- Java EnumMap
- Java SortedMap Interface
- Java NavigableMap Interface
- Java TreeMap
- Java ConcurrentMap Interface
- Java ConcurrentHashMap
- Java Set Interface
- Java HashSet Class
- Java EnumSet
- Java LinkedHashSet
- Java SortedSet Interface
- Java NavigableSet Interface
- Java TreeSet
- Java Algorithms
- Java Iterator Interface
- Java ListIterator Interface
Java I/o Streams
- Java I/O Streams
- Java InputStream Class
- Java OutputStream Class
- Java FileInputStream Class
- Java FileOutputStream Class
- Java ByteArrayInputStream Class
- Java ByteArrayOutputStream Class
- Java ObjectInputStream Class
- Java ObjectOutputStream Class
- Java BufferedInputStream Class
- Java BufferedOutputStream Class
- Java PrintStream Class
Java Reader/Writer
- Java File Class
- Java Reader Class
- Java Writer Class
- Java InputStreamReader Class
- Java OutputStreamWriter Class
- Java FileReader Class
- Java FileWriter Class
- Java BufferedReader
- Java BufferedWriter Class
- Java StringReader Class
- Java StringWriter Class
- Java PrintWriter Class
Additional Topics
- Java Keywords and Identifiers
Java Operator Precedence
Java Bitwise and Shift Operators
- Java Scanner Class
- Java Type Casting
- Java Wrapper Class
- Java autoboxing and unboxing
- Java Lambda Expressions
- Java Generics
- Nested Loop in Java
- Java Command-Line Arguments
Java Tutorials
- Java Math IEEEremainder()
Operators are symbols that perform operations on variables and values. For example, + is an operator used for addition, while * is also an operator used for multiplication.
Operators in Java can be classified into 5 types:
- Arithmetic Operators
- Assignment Operators
- Relational Operators
- Logical Operators
- Unary Operators
- Bitwise Operators
1. Java Arithmetic Operators
Arithmetic operators are used to perform arithmetic operations on variables and data. For example,
Here, the + operator is used to add two variables a and b . Similarly, there are various other arithmetic operators in Java.
Example 1: Arithmetic Operators
In the above example, we have used + , - , and * operators to compute addition, subtraction, and multiplication operations.
/ Division Operator
Note the operation, a / b in our program. The / operator is the division operator.
If we use the division operator with two integers, then the resulting quotient will also be an integer. And, if one of the operands is a floating-point number, we will get the result will also be in floating-point.
% Modulo Operator
The modulo operator % computes the remainder. When a = 7 is divided by b = 4 , the remainder is 3 .
Note : The % operator is mainly used with integers.
2. Java Assignment Operators
Assignment operators are used in Java to assign values to variables. For example,
Here, = is the assignment operator. It assigns the value on its right to the variable on its left. That is, 5 is assigned to the variable age .
Let's see some more assignment operators available in Java.
Example 2: Assignment Operators
3. java relational operators.
Relational operators are used to check the relationship between two operands. For example,
Here, < operator is the relational operator. It checks if a is less than b or not.
It returns either true or false .
Example 3: Relational Operators
Note : Relational operators are used in decision making and loops.
4. Java Logical Operators
Logical operators are used to check whether an expression is true or false . They are used in decision making.
Example 4: Logical Operators
Working of Program
- (5 > 3) && (8 > 5) returns true because both (5 > 3) and (8 > 5) are true .
- (5 > 3) && (8 < 5) returns false because the expression (8 < 5) is false .
- (5 < 3) || (8 > 5) returns true because the expression (8 > 5) is true .
- (5 > 3) || (8 < 5) returns true because the expression (5 > 3) is true .
- (5 < 3) || (8 < 5) returns false because both (5 < 3) and (8 < 5) are false .
- !(5 == 3) returns true because 5 == 3 is false .
- !(5 > 3) returns false because 5 > 3 is true .
5. Java Unary Operators
Unary operators are used with only one operand. For example, ++ is a unary operator that increases the value of a variable by 1 . That is, ++5 will return 6 .
Different types of unary operators are:
- Increment and Decrement Operators
Java also provides increment and decrement operators: ++ and -- respectively. ++ increases the value of the operand by 1 , while -- decrease it by 1 . For example,
Here, the value of num gets increased to 6 from its initial value of 5 .
Example 5: Increment and Decrement Operators
In the above program, we have used the ++ and -- operator as prefixes (++a, --b) . We can also use these operators as postfix (a++, b++) .
There is a slight difference when these operators are used as prefix versus when they are used as a postfix.
To learn more about these operators, visit increment and decrement operators .
6. Java Bitwise Operators
Bitwise operators in Java are used to perform operations on individual bits. For example,
Here, ~ is a bitwise operator. It inverts the value of each bit ( 0 to 1 and 1 to 0 ).
The various bitwise operators present in Java are:
These operators are not generally used in Java. To learn more, visit Java Bitwise and Bit Shift Operators .
Other operators
Besides these operators, there are other additional operators in Java.
The instanceof operator checks whether an object is an instanceof a particular class. For example,
Here, str is an instance of the String class. Hence, the instanceof operator returns true . To learn more, visit Java instanceof .
The ternary operator (conditional operator) is shorthand for the if-then-else statement. For example,
Here's how it works.
- If the Expression is true , expression1 is assigned to the variable .
- If the Expression is false , expression2 is assigned to the variable .
Let's see an example of a ternary operator.
In the above example, we have used the ternary operator to check if the year is a leap year or not. To learn more, visit the Java ternary operator .
Now that you know about Java operators, it's time to know about the order in which operators are evaluated. To learn more, visit Java Operator Precedence .
Table of Contents
- Introduction
- Java Arithmetic Operators
- Java Assignment Operators
- Java Relational Operators
- Java Logical Operators
- Java Unary Operators
- Java Bitwise Operators
Sorry about that.
Related Tutorials
Java Tutorial
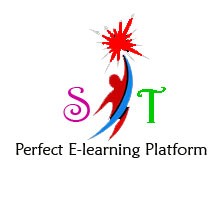
Java Script
- Python Programs
- Java Script Program
- Java Program
- Ask Your Question
- Java Script FAQ
- CBSE Comp Science Question Paper
- MDU python question Papers
- Java Assignment
- C Assignment
- Python assignment
- Java Script Assignment
- Python Interview Question
- Video Tutorials
- 11th,12th Class Python Syllabus CBSE Board
- ICSE Board 11-12 Computer Syllabus
- Top 10 Programming Language 2020
7 Best Java Homework Help Websites: How to Choose Your Perfect Match?
J ava programming is not a field that could be comprehended that easily; thus, it is no surprise that young learners are in search of programming experts to get help with Java homework and handle their assignments. But how to choose the best alternative when the number of proposals is enormous?
In this article, we are going to talk about the top ‘do my Java assignment’ services that offer Java assignment assistance and dwell upon their features. In the end, based on the results, you will be able to choose the one that meets your demands to the fullest and answer your needs. Here is the list of services that are available today, as well as those that are on everyone's lips:
TOP Java Assignment Help Services: What Makes Them Special?
No need to say that every person is an individual and the thing that suits a particular person could not meet the requirements of another. So, how can we name the best Java assignment help services on the web? - We have collected the top issues students face when searching for Java homework help and found the companies that promise to meet these requirements.
What are these issues, though?
- Pricing . Students are always pressed for budget, and finding services that suit their pockets is vital. Thus, we tried to provide services that are relatively affordable on the market. Of course, professional services can’t be offered too cheaply, so we have chosen the ones that balance professionalism and affordability.
- Programming languages . Not all companies have experts in all possible programming languages. Thus, we tried to choose the ones that offer as many different languages as possible.
- Expert staff . In most cases, students come to a company when they need to place their ‘do my Java homework’ orders ASAP. Thus, a large expert staff is a real benefit for young learners. They want to come to a service, place their order and get a professional to start working on their project in no time.
- Reviews . Of course, everyone wants to get professional help with Java homework from a reputable company that has already completed hundreds of Java assignments for their clients. Thus, we have mentioned only those companies that have earned enough positive feedback from their clients.
- Deadline options. Flexible deadline options are also a benefit for those who are placing their last-minute Java homework help assignments. Well, we also provide services with the most extended deadlines for those who want to save some money and place their projects beforehand.
- Guarantees . This is the must-feature if you want to get quality assistance and stay assured you are totally safe with the company you have chosen. In our list, we have only named companies that provide client-oriented guarantees and always keep their word, as well as offer only professional Java assignment experts.
- Customization . Every service from the list offers Java assistance tailored to clients’ personal needs. There, you won’t find companies that offer pre-completed projects and sell them at half-price.
So, let’s have a closer look at each option so you can choose the one that totally meets your needs.
DoMyAssignments.com
At company service, you can get assistance with academic writing as well as STEM projects. The languages you can get help with are C#, C++, Computer science, Java, Javascript, HTML, PHP, Python, Ruby, and SQL.
The company’s prices start at $30/page for a project that needs to be done in 14+ days.
Guarantees and extra services
The company offers a list of guarantees to make your cooperation as comfortable as possible. So, what can you expect from the service?
- Free revisions . When you get your order, you can ask your expert for revisions if needed. It means that if you see that any of your demands were missed, you can get revisions absolutely for free.
- Money-back guarantee. The company offers professional help, and they are sure about their experts and the quality of their assistance. Still, if you receive a project that does not meet your needs, you can ask for a full refund.
- Confidentiality guarantee . Stay assured that all your personal information is safe and secure, as the company scripts all the information you share with them.
- 100% customized assistance . At this service, you won’t find pre-written codes, all the projects are completed from scratch.
Expert staff
If you want to hire one of the top Java homework experts at DoMyAssignments , you can have a look at their profile, see the latest orders they have completed, and make sure they are the best match for your needs. Also, you can have a look at the samples presented on their website and see how professional their experts are. If you want to hire a professional who completed a particular sample project, you can also turn to a support team and ask if you can fire this expert.
CodingHomeworkHelp.org
CodingHomeworkHelp is rated at 9.61/10 and has 10+ years of experience in the programming assisting field. Here, you can get help with the following coding assignments: MatLab, Computer Science, Java, HTML, C++, Python, R Studio, PHP, JavaScript, and C#.
Free options all clients get
Ordering your project with CodingHomeworkHelp.org, you are to enjoy some other options that will definitely satisfy you.
- Partial payments . If you order a large project, you can pay for it in two parts. Order the first one, get it done, and only then pay for the second one.
- Revisions . As soon as you get your order, you can ask for endless revisions unless your project meets your initial requirements.
- Chat with your expert . When you place your order, you get an opportunity to chat directly with your coding helper. If you have any questions or demands, there is no need to first contact the support team and ask them to contact you to your assistant.
- Code comments . If you have questions concerning your code, you can ask your helper to provide you with the comments that will help you better understand it and be ready to discuss your project with your professor.
The prices start at $20/page if you set a 10+ days deadline. But, with CodingHomeworkHelp.org, you can get a special discount; you can take 20% off your project when registering on the website. That is a really beneficial option that everyone can use.
CWAssignments.com
CWAssignments.com is an assignment helper where you can get professional help with programming and calculations starting at $30/page. Moreover, you can get 20% off your first order.
Working with the company, you are in the right hands and can stay assured that the final draft will definitely be tailored to your needs. How do CWAssignments guarantee their proficiency?
- Money-back guarantee . If you are not satisfied with the final work, if it does not meet your expectations, you can request a refund.
- Privacy policy . The service collects only the data essential to complete your order to make your cooperation effective and legal.
- Security payment system . All the transactions are safe and encrypted to make your personal information secure.
- No AI-generated content . The company does not use any AI tools to complete their orders. When you get your order, you can even ask for the AI detection report to see that your assignment is pure.
With CWAssignments , you can regulate the final cost of your project. As it was mentioned earlier, the prices start at $30/page, but if you set a long-term deadline or ask for help with a Java assignment or with a part of your task, you can save a tidy sum.
DoMyCoding.com
This company has been offering its services on the market for 18+ years and provides assistance with 30+ programming languages, among which are Python, Java, C / C++ / C#, JavaScript, HTML, SQL, etc. Moreover, here, you can get assistance not only with programming but also with calculations.
Pricing and deadlines
With DoMyCoding , you can get help with Java assignments in 8 hours, and their prices start at $30/page with a 14-day deadline.
Guarantees and extra benefits
The service offers a number of guarantees that protect you from getting assistance that does not meet your requirements. Among the guarantees, you can find:
- The money-back guarantee . If your order does not meet your requirements, you will get a full refund of your order.
- Free edits within 7 days . After you get your project, you can request any changes within the 7-day term.
- Payments in parts . If you have a large order, you can pay for it in installments. In this case, you get a part of your order, check if it suits your needs, and then pay for the other part.
- 24/7 support . The service operates 24/7 to answer your questions as well as start working on your projects. Do not hesitate to use this option if you need to place an ASAP order.
- Confidentiality guarantee . The company uses the most secure means to get your payments and protects the personal information you share on the website to the fullest.
More benefits
Here, we also want to pay your attention to the ‘Samples’ section on the website. If you are wondering if a company can handle your assignment or you simply want to make sure they are professionals, have a look at their samples and get answers to your questions.
AssignCode.com
AssignCode is one of the best Java assignment help services that you can entrust with programming, mathematics, biology, engineering, physics, and chemistry. A large professional staff makes this service available to everyone who needs help with one of these disciplines. As with some of the previous companies, AssignCode.com has reviews on different platforms (Reviews.io and Sitejabber) that can help you make your choice.
As with all the reputed services, AssignCode offers guarantees that make their cooperation with clients trustworthy and comfortable. Thus, the company guarantees your satisfaction, confidentiality, client-oriented attitude, and authenticity.
Special offers
Although the company does not offer special prices on an ongoing basis, regular clients can benefit from coupons the service sends them via email. Thus, if you have already worked with the company, make sure to check your email before placing a new one; maybe you have received a special offer that will help you save some cash.
AssignmentShark.com
Reviews about this company you can see on different platforms. Among them are Reviews.io (4.9 out of 5), Sitejabber (4.5 points), and, of course, their own website (9.6 out of 10). The rate of the website speaks for itself.
Pricing
When you place your ‘do my Java homework’ request with AssignmentShark , you are to pay $20/page for the project that needs to be done in at least ten days. Of course, if the due date is closer, the cost will differ. All the prices are presented on the website so that you can come, input all the needed information, and get an approximate calculation.
Professional staff
On the ‘Our experts’ page, you can see the full list of experts. Or, you can use filters to see the professional in the required field.
The company has a quick form on its website for those who want to join their professional staff, which means that they are always in search of new experts to make sure they can provide clients with assistance as soon as the need arises.
Moreover, if one wants to make sure the company offers professional assistance, one can have a look at the latest orders and see how experts provide solutions to clients’ orders.
What do clients get?
Placing orders with the company, one gets a list of inclusive services:
- Free revisions. You can ask for endless revisions until your order fully meets your demands.
- Code comments . Ask your professional to provide comments on the codes in order to understand your project perfectly.
- Source files . If you need the list of references and source files your helper turned to, just ask them to add these to the project.
- Chat with the professional. All the issues can be solved directly with your coding assistant.
- Payment in parts. Large projects can be paid for in parts. When placing your order, let your manager know that you want to pay in parts.
ProgrammingDoer.com
ProgrammingDoer is one more service that offers Java programming help to young learners and has earned a good reputation among previous clients.
The company cherishes its reputation and does its best to let everyone know about their proficiency. Thus, you, as a client, can read what people think about the company on several platforms - on their website as well as at Reviews.io.
What do you get with the company?
Let’s have a look at the list of services the company offers in order to make your cooperation with them as comfortable as possible.
- Free revisions . If you have any comments concerning the final draft, you can ask your professional to revise it for free as many times as needed unless it meets your requirements to the fullest.
- 24/7 assistance . No matter when you realize that you have a programming assignment that should be done in a few days. With ProgrammingDoer, you can place your order 24/7 and get a professional helper as soon as there is an available one.
- Chat with the experts . When you place your order with the company, you get an opportunity to communicate with your coding helper directly to solve all the problems ASAP.
Extra benefits
If you are not sure if the company can handle your assignment the right way, if they have already worked on similar tasks, or if they have an expert in the needed field, you can check this information on your own. First, you can browse the latest orders and see if there is something close to the issue you have. Then, you can have a look at experts’ profiles and see if there is anyone capable of solving similar issues.
Can I hire someone to do my Java homework?
If you are not sure about your Java programming skills, you can always ask a professional coder to help you out. All you need is to find the service that meets your expectations and place your ‘do my Java assignment’ order with them.
What is the typical turnaround time for completing a Java homework assignment?
It depends on the service that offers such assistance as well as on your requirements. Some companies can deliver your project in a few hours, but some may need more time. But, you should mind that fast delivery is more likely to cost you some extra money.
What is the average pricing structure for Java assignment help?
The cost of the help with Java homework basically depends on the following factors: the deadline you set, the complexity level of the assignment, the expert you choose, and the requirements you provide.
How will we communicate and collaborate on my Java homework?
Nowadays, Java assignment help companies provide several ways of communication. In most cases, you can contact your expert via live chat on a company’s website, via email, or a messenger. To see the options, just visit the chosen company’s website and see what they offer.
Regarding the Author:
Nayeli Ellen, a dynamic editor at AcademicHelp, combines her zeal for writing with keen analytical skills. In her comprehensive review titled " Programming Assignment Help: 41 Coding Homework Help Websites ," Nayeli offers an in-depth analysis of numerous online coding homework assistance platforms.
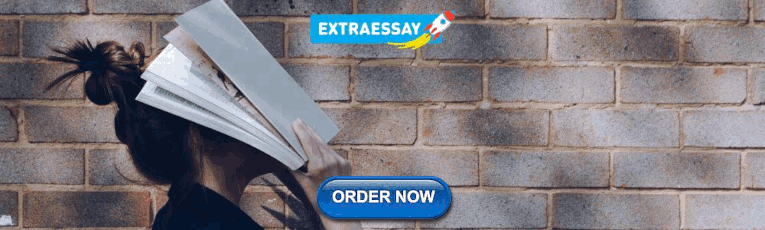
IMAGES
VIDEO
COMMENTS
Note: The compound assignment operator in Java performs implicit type casting. Let's consider a scenario where x is an int variable with a value of 5. int x = 5; If you want to add the double value 4.5 to the integer variable x and print its value, there are two methods to achieve this: Method 1: x = x + 4.5. Method 2: x += 4.5.
This beginner Java tutorial describes fundamentals of programming in the Java programming language ... The Simple Assignment Operator. One of the most common operators that you'll encounter is the simple assignment operator "=". You saw this operator in the Bicycle class; it assigns the value on its right to the operand on its left: ...
There are mainly two types of assignment operators in Java, which are as follows: Simple Assignment Operator ; We use the simple assignment operator with the "=" sign, where the left side consists of an operand and the right side is a value. The value of the operand on the right side must be of the same data type defined on the left side.
To assign a value to a variable, use the basic assignment operator (=). It is the most fundamental assignment operator in Java. It assigns the value on the right side of the operator to the variable on the left side. Example: int x = 10; int x = 10; In the above example, the variable x is assigned the value 10.
Java Assignment Operators. Assignment operators are used to assign values to variables. In the example below, we use the assignment operator (=) to assign the value 10 to a variable called x: Example int x = 10;
Java Assignment Operators. The Java Assignment Operators are used when you want to assign a value to the expression. The assignment operator denoted by the single equal sign =. In a Java assignment statement, any expression can be on the right side and the left side must be a variable name. For example, this does not mean that "a" is equal to ...
Java assignment operators are classified into two types: simple and compound. The Simple assignment operator is the equals ( =) sign, which is the most straightforward of the bunch. It simply assigns the value or variable on the right to the variable on the left. Compound operators are comprised of both an arithmetic, bitwise, or shift operator ...
The following programs are simple examples which demonstrate the assignment operators. Copy and paste the following Java programs as Test.java file, and compile and run the programs −. Example 1. In this example, we're creating three variables a,b and c and using assignment operators. We've performed simple assignment, addition AND assignment ...
As you can see, In the above example, we are using assignment operator in if statement. We did a comparison of value 10 to an assignment operator which resulted in a 'true' output because the return of assignment operator is the value of left operand. Recommended Posts. Arithmetic Operators in Java with Examples; Unary Operators in Java ...
Java is the foundation for virtually every type of networked application and is the global standard for developing and delivering embedded and mobile applications, games, Web-based content, and enterprise software. With more than 9 million developers worldwide, Java enables you to efficiently develop, deploy and use exciting applications and ...
Assignment Operators in Java: An Overview. We already discussed the Types of Operators in the previous tutorial Java. In this Java tutorial, we will delve into the different types of assignment operators in Java, and their syntax, and provide examples for better understanding.Because Java is a flexible and widely used programming language. Assignment operators play a crucial role in ...
Java allows you to combine assignment and addition operators using a shorthand operator. For example, the preceding statement can be written as: i +=8; //This is same as i = i+8; The += is called the addition assignment operator. Other shorthand operators are shown below table. Operator. Name.
In this lesson, you will learn about assignment statements and expressions that contain math operators and variables. 1.4.1. Assignment Statements ¶. Remember that a variable holds a value that can change or vary. Assignment statements initialize or change the value stored in a variable using the assignment operator =.
Programming assignments. Creative programming assignments that we have used at Princeton. You can explore these resources via the sidebar at left. Introduction to Programming in Java. Our textbook Introduction to Programming in Java [ Amazon · Pearson · InformIT] is an interdisciplinary approach to the traditional CS1 curriculum with Java. We ...
This section provides the assignments for the course, supporting files, and a special set of assignment files that can be annotated. Browse Course Material ... GravityCalculator.java 2 FooCorporation 3 Marathon Marathon.java 4 Library Book.java . Library.java . 5 Graphics! initial.png . SimpleDraw.java ...
2. Java Assignment Operators. Assignment operators are used in Java to assign values to variables. For example, int age; age = 5; Here, = is the assignment operator. It assigns the value on its right to the variable on its left. That is, 5 is assigned to the variable age. Let's see some more assignment operators available in Java.
Next, let's see which assignment operators we can use in Java. 9.1. The Simple Assignment Operator. The simple assignment operator (=) is a straightforward but important operator in Java. Actually, we've used it many times in previous examples. It assigns the value on its right to the operand on its left:
I am new to Java and I have some questions in mind regarding object assignment. For instance, Test t1 = new Test(); Test t2 = t1; t1.i=1; Assuming variable i is defined inside Test class, am I right to assume both t1 and t2 point to the same object where the modification t1.i=1 affects both t1 and t2?Actually I tested it out and seems like I was right.
It's the Addition assignment operator. Let's understand the += operator in Java and learn to use it for our day to day programming. x += y in Java is the same as x = x + y. It is a compound assignment operator. Most commonly used for incrementing the value of a variable since x++ only increments the value by one.
Q.1 WAP in java to create Box class with parameterized constructor with an object arguement. to initialize length, breadth and height also create a function volume which returns the volume. of the box and print it in main method. Q.2 Write a program in java with class Employee and do the following operations on it.
Nowadays, Java assignment help companies provide several ways of communication. In most cases, you can contact your expert via live chat on a company's website, via email, or a messenger. To see ...