How-To Geek
How to work with variables in bash.
Want to take your Linux command-line skills to the next level? Here's everything you need to know to start working with variables.
Hannah Stryker / How-To Geek
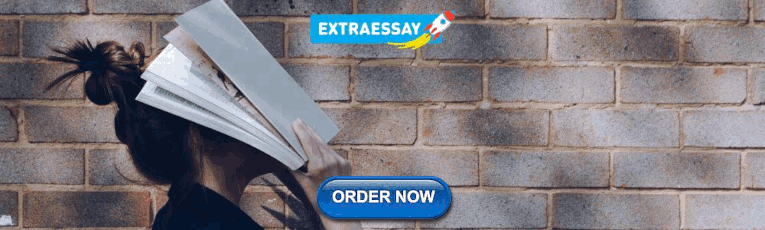
Quick Links
Variables 101, examples of bash variables, how to use bash variables in scripts, how to use command line parameters in scripts, working with special variables, environment variables, how to export variables, how to quote variables, echo is your friend, key takeaways.
- Variables are named symbols representing strings or numeric values. They are treated as their value when used in commands and expressions.
- Variable names should be descriptive and cannot start with a number or contain spaces. They can start with an underscore and can have alphanumeric characters.
- Variables can be used to store and reference values. The value of a variable can be changed, and it can be referenced by using the dollar sign $ before the variable name.
Variables are vital if you want to write scripts and understand what that code you're about to cut and paste from the web will do to your Linux computer. We'll get you started!
Variables are named symbols that represent either a string or numeric value. When you use them in commands and expressions, they are treated as if you had typed the value they hold instead of the name of the variable.
To create a variable, you just provide a name and value for it. Your variable names should be descriptive and remind you of the value they hold. A variable name cannot start with a number, nor can it contain spaces. It can, however, start with an underscore. Apart from that, you can use any mix of upper- and lowercase alphanumeric characters.
Here, we'll create five variables. The format is to type the name, the equals sign = , and the value. Note there isn't a space before or after the equals sign. Giving a variable a value is often referred to as assigning a value to the variable.
We'll create four string variables and one numeric variable,
my_name=Dave
my_boost=Linux
his_boost=Spinach
this_year=2019
To see the value held in a variable, use the echo command. You must precede the variable name with a dollar sign $ whenever you reference the value it contains, as shown below:
echo $my_name
echo $my_boost
echo $this_year
Let's use all of our variables at once:
echo "$my_boost is to $me as $his_boost is to $him (c) $this_year"
The values of the variables replace their names. You can also change the values of variables. To assign a new value to the variable, my_boost , you just repeat what you did when you assigned its first value, like so:
my_boost=Tequila
If you re-run the previous command, you now get a different result:
So, you can use the same command that references the same variables and get different results if you change the values held in the variables.
We'll talk about quoting variables later. For now, here are some things to remember:
- A variable in single quotes ' is treated as a literal string, and not as a variable.
- Variables in quotation marks " are treated as variables.
- To get the value held in a variable, you have to provide the dollar sign $ .
- A variable without the dollar sign $ only provides the name of the variable.
You can also create a variable that takes its value from an existing variable or number of variables. The following command defines a new variable called drink_of_the_Year, and assigns it the combined values of the my_boost and this_year variables:
drink_of-the_Year="$my_boost $this_year"
echo drink_of_the-Year
Scripts would be completely hamstrung without variables. Variables provide the flexibility that makes a script a general, rather than a specific, solution. To illustrate the difference, here's a script that counts the files in the /dev directory.
Type this into a text file, and then save it as fcnt.sh (for "file count"):
#!/bin/bashfolder_to_count=/devfile_count=$(ls $folder_to_count | wc -l)echo $file_count files in $folder_to_count
Before you can run the script, you have to make it executable, as shown below:
chmod +x fcnt.sh
Type the following to run the script:
This prints the number of files in the /dev directory. Here's how it works:
- A variable called folder_to_count is defined, and it's set to hold the string "/dev."
- Another variable, called file_count , is defined. This variable takes its value from a command substitution. This is the command phrase between the parentheses $( ) . Note there's a dollar sign $ before the first parenthesis. This construct $( ) evaluates the commands within the parentheses, and then returns their final value. In this example, that value is assigned to the file_count variable. As far as the file_count variable is concerned, it's passed a value to hold; it isn't concerned with how the value was obtained.
- The command evaluated in the command substitution performs an ls file listing on the directory in the folder_to_count variable, which has been set to "/dev." So, the script executes the command "ls /dev."
- The output from this command is piped into the wc command. The -l (line count) option causes wc to count the number of lines in the output from the ls command. As each file is listed on a separate line, this is the count of files and subdirectories in the "/dev" directory. This value is assigned to the file_count variable.
- The final line uses echo to output the result.
But this only works for the "/dev" directory. How can we make the script work with any directory? All it takes is one small change.
Many commands, such as ls and wc , take command line parameters. These provide information to the command, so it knows what you want it to do. If you want ls to work on your home directory and also to show hidden files , you can use the following command, where the tilde ~ and the -a (all) option are command line parameters:
Our scripts can accept command line parameters. They're referenced as $1 for the first parameter, $2 as the second, and so on, up to $9 for the ninth parameter. (Actually, there's a $0 , as well, but that's reserved to always hold the script.)
You can reference command line parameters in a script just as you would regular variables. Let's modify our script, as shown below, and save it with the new name fcnt2.sh :
#!/bin/bashfolder_to_count=$1file_count=$(ls $folder_to_count | wc -l)echo $file_count files in $folder_to_count
This time, the folder_to_count variable is assigned the value of the first command line parameter, $1 .
The rest of the script works exactly as it did before. Rather than a specific solution, your script is now a general one. You can use it on any directory because it's not hardcoded to work only with "/dev."
Here's how you make the script executable:
chmod +x fcnt2.sh
Now, try it with a few directories. You can do "/dev" first to make sure you get the same result as before. Type the following:
./fnct2.sh /dev
./fnct2.sh /etc
./fnct2.sh /bin
You get the same result (207 files) as before for the "/dev" directory. This is encouraging, and you get directory-specific results for each of the other command line parameters.
To shorten the script, you could dispense with the variable, folder_to_count , altogether, and just reference $1 throughout, as follows:
#!/bin/bash file_count=$(ls $1 wc -l) echo $file_count files in $1
We mentioned $0 , which is always set to the filename of the script. This allows you to use the script to do things like print its name out correctly, even if it's renamed. This is useful in logging situations, in which you want to know the name of the process that added an entry.
The following are the other special preset variables:
- $# : How many command line parameters were passed to the script.
- $@ : All the command line parameters passed to the script.
- $? : The exit status of the last process to run.
- $$ : The Process ID (PID) of the current script.
- $USER : The username of the user executing the script.
- $HOSTNAME : The hostname of the computer running the script.
- $SECONDS : The number of seconds the script has been running for.
- $RANDOM : Returns a random number.
- $LINENO : Returns the current line number of the script.
You want to see all of them in one script, don't you? You can! Save the following as a text file called, special.sh :
#!/bin/bashecho "There were $# command line parameters"echo "They are: $@"echo "Parameter 1 is: $1"echo "The script is called: $0"# any old process so that we can report on the exit statuspwdecho "pwd returned $?"echo "This script has Process ID $$"echo "The script was started by $USER"echo "It is running on $HOSTNAME"sleep 3echo "It has been running for $SECONDS seconds"echo "Random number: $RANDOM"echo "This is line number $LINENO of the script"
Type the following to make it executable:
chmod +x special.sh
Now, you can run it with a bunch of different command line parameters, as shown below.
Bash uses environment variables to define and record the properties of the environment it creates when it launches. These hold information Bash can readily access, such as your username, locale, the number of commands your history file can hold, your default editor, and lots more.
To see the active environment variables in your Bash session, use this command:
If you scroll through the list, you might find some that would be useful to reference in your scripts.
When a script runs, it's in its own process, and the variables it uses cannot be seen outside of that process. If you want to share a variable with another script that your script launches, you have to export that variable. We'll show you how to this with two scripts.
First, save the following with the filename script_one.sh :
#!/bin/bashfirst_var=alphasecond_var=bravo# check their valuesecho "$0: first_var=$first_var, second_var=$second_var"export first_varexport second_var./script_two.sh# check their values againecho "$0: first_var=$first_var, second_var=$second_var"
This creates two variables, first_var and second_var , and it assigns some values. It prints these to the terminal window, exports the variables, and calls script_two.sh . When script_two.sh terminates, and process flow returns to this script, it again prints the variables to the terminal window. Then, you can see if they changed.
The second script we'll use is script_two.sh . This is the script that script_one.sh calls. Type the following:
#!/bin/bash# check their valuesecho "$0: first_var=$first_var, second_var=$second_var"# set new valuesfirst_var=charliesecond_var=delta# check their values againecho "$0: first_var=$first_var, second_var=$second_var"
This second script prints the values of the two variables, assigns new values to them, and then prints them again.
To run these scripts, you have to type the following to make them executable:
chmod +x script_one.shchmod +x script_two.sh
And now, type the following to launch script_one.sh :
./script_one.sh
This is what the output tells us:
- script_one.sh prints the values of the variables, which are alpha and bravo.
- script_two.sh prints the values of the variables (alpha and bravo) as it received them.
- script_two.sh changes them to charlie and delta.
- script_one.sh prints the values of the variables, which are still alpha and bravo.
What happens in the second script, stays in the second script. It's like copies of the variables are sent to the second script, but they're discarded when that script exits. The original variables in the first script aren't altered by anything that happens to the copies of them in the second.
You might have noticed that when scripts reference variables, they're in quotation marks " . This allows variables to be referenced correctly, so their values are used when the line is executed in the script.
If the value you assign to a variable includes spaces, they must be in quotation marks when you assign them to the variable. This is because, by default, Bash uses a space as a delimiter.
Here's an example:
site_name=How-To Geek
Bash sees the space before "Geek" as an indication that a new command is starting. It reports that there is no such command, and abandons the line. echo shows us that the site_name variable holds nothing — not even the "How-To" text.
Try that again with quotation marks around the value, as shown below:
site_name="How-To Geek"
This time, it's recognized as a single value and assigned correctly to the site_name variable.
It can take some time to get used to command substitution, quoting variables, and remembering when to include the dollar sign.
Before you hit Enter and execute a line of Bash commands, try it with echo in front of it. This way, you can make sure what's going to happen is what you want. You can also catch any mistakes you might have made in the syntax.
404 Not found
Bash Variables Explained: A Simple Guide With Examples
Master Bash variables with the help of these explanations and examples.
Variables are used for storing values of different types during program execution. There are two types of variables in Bash scripting: global and local.
Global variables can be used by all Bash scripts on your system, while local variables can only be used within the script (or shell) in which they're defined.
Global variables are generally provided on the system by default and are mainly environment and configuration variables. Local variables, on the other hand, are user-defined and have arbitrary uses.
Bash Local Variables
To create a variable, you need to assign a value to your variable name. Bash is an untyped language, so you don't have to indicate a data type when defining your variables.
Bash also allows multiple assignments on a single line:
Just like many other programming languages, Bash uses the assignment operator = to assign values to variables. It's important to note that there shouldn't be any spaces on either side of the assignment operator. Otherwise, you'll get a compilation error.
Related: What Does "Bash" Mean in Linux?
Another key point to note: Bash doesn't allow you to define a variable first and then assign a value to it later. You must assign a value to the variable at creation.
Sometimes, you may need to assign a string that has a space in it to your variable. In such a case, enclose the string in quotes.
Notice the use of single quotes. These quotes are also called "strong quotes" because they assign the value precisely as it's written without regard to any special characters.
In the example above, you could have also used double quotes ("weak quotes"), though this doesn't mean they can always be used interchangeably. This is because double quotes will substitute special characters (such as those with $ ), instead of interpreting them literally.
See the example below:
If you want to assign a command-line output to your variable, use backquotes ( `` ). They'll treat the string enclosed in them as a terminal command and return its result.
Parameter Expansion in Bash
Parameter Expansion simply refers to accessing the value of a variable. In its simplest form, it uses the special character $ followed by the variable name (with no spaces in between):
You can also use the syntax ${variableName} to access a variable's value. This form is more suitable when confusion surrounding the variable name may arise.
If you leave out the curly brackets, ${m}ical will be interpreted as a compound variable (that doesn't exist). This use of curly brackets with variables is known as "substitution".
Global Variables
As mentioned earlier, your Linux system has some built-in variables that can be accessed across all of your scripts (or shells). These variables are accessed using the same syntax as local variables.
Related: How to Create and Execute Bash Scripts in Linux
Most of these variables are in BLOCK letters. However, some are single characters that aren't even alphanumeric characters.
Here are some common useful global variables:
HOME : Provides the user's home directory
SHELL : Provides the type of shell you're using (e.g Bash, csh..etc)
? : Provides the exit status of the previous command
To get a list of global variables on your system, run the printenv (or env) command:
Loops in Bash Scripting
Now you know what variables are, how to assign them, and how to perform basic Bash logic using them.
Loops enable you to iterate through multiple statements. Bash accommodates for loops and while loops with a simple syntax for all of your looping needs.
If you're mastering the art of Bash development, for loops ought to be next up on your list.

Home > Bash Scripting Tutorial > Bash Variables > Variable Declaration and Assignment
Variable Declaration and Assignment

In programming, a variable serves as a placeholder for an unknown value, enabling the user to store the different data and access them whenever needed. During compilation or interpretation , the symbolic names of variables are replaced with their corresponding data locations . This article will mainly focus on the variable declaration and assignment in a Bash Script . So, let’s start.
Key Takeaways
- Getting familiar with Bash Variables.
- Learning to declare and assign variables.
Free Downloads
Factors of variable declaration and assignment.
There are multiple factors to be followed to declare a variable and assign data to it. Here I have listed the factors below to understand the process. Check it out.
1. Choosing Appropriate Variable Name
When choosing appropriate variable names for declaration and assignment, it’s essential to follow some guidelines to ensure clarity and readability and to avoid conflicts with other system variables. Here some common guidelines to be followed are listed below:
- Using descriptive names: Using descriptive names in variable naming conventions involves choosing meaningful and expressive names for variables in your code. This practice improves code readability and makes it easier to grasp the purpose of the code at a glance.
- Using lowercase letters: It is a good practice to begin variable names with a lowercase letter . This convention is not enforced by the bash language itself, but it is a widely followed practice to improve code readability and maintain consistency . For example name , age , count , etc.
- Separating words with underscores: Using underscores to separate words in variable names enhances code readability. For example first_name , num_students , etc.
- Avoid starting with numbers: Variable names should not begin with a number. For instance, 1var is not a valid variable
- Avoiding special characters : Avoid using special characters , whitespace , or punctuation marks, as they can cause syntax errors or introduce unexpected behavior. For instance, using any special character such as @, $, or # anywhere while declaring a variable is not legal.
2. Declaring the Variable in the Bash Script
In Bash , declaring variables and assigning values to them can be done for different data types by using different options along with the declare command . However, you can also declare and assign the variable value without explicitly specifying the type .
3. Assigning the Value to the Variable
When declaring variables for numeric values , simply assign the desired number directly, as in age=30 for an integer or price=12.99 for a floating – point value . For strings , enclose the text in single or double quotes , such as name =’ John ‘ or city =” New York “. Booleans can be represented using 0 and 1 , where 0 indicates false and 1 represents true , like is_valid = 1 .
Arrays are declared by assigning a sequence of values enclosed in parentheses to a variable, e.g., fruits=(“apple” “banana” “orange”) . To create associative arrays ( key-value pairs ), use the declare -A command followed by assigning the elements, like declare -A ages=([“John”]=30 [“Jane”]=25) .
4 Practical Cases of Declaring and Assigning Bash Variable
In this section, I have demonstrated some sample Bash scripts that will help you understand the basic concept of variable declaration and assignment in Bash script . So let’s get into it.
Case 01: Basic Variable Assignment in Bash Script
In my first case , I have shown a basic variable assignment with its output. Here, I have taken a string and an integer variable to show the basic variable assignment mechanism. Follow the given steps to accomplish the task.
Steps to Follow >
❶ At first, launch an Ubuntu terminal .
❷ Write the following command to open a file in Nano :
- nano : Opens a file in the Nano text editor.
- basic_var.sh : Name of the file.
❸ Copy the script mentioned below:
The script starts with the shebang line ( #!/bin/bash ), specifying that the script should be executed using the Bash shell . Next, two variables are declared and assigned values using the format variable_name = value . The first variable is name , and it is assigned the string value “ John .” The second variable is age , assigned the numeric value 30 . The script then uses the echo command to print the values of the variables.
❹ Press CTRL+O and ENTER to save the file; CTRL+X to exit.
❺ Use the following command to make the file executable:
- chmod : is used to change the permissions of files and directories.
- u+x : Here, u refers to the “ user ” or the owner of the file and +x specifies the permission being added, in this case, the “ execute ” permission. When u+x is added to the file permissions, it grants the user ( owner ) permission to execute ( run ) the file.
- basic_var.sh : File name to which the permissions are being applied.
❻ Run the script by the following command:
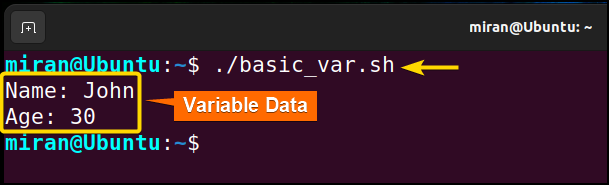
Case 02: Input From User and Variable Assignment
In Bash , you can take input from the user and store it in variables using the read command . To do so use the following script:
You can follow the steps of Case 01 , to create, save and make the script executable.
Script (user_var.sh) >
The script starts with the shebang line ( #!/bin/bash ) to specify that the Bash shell should be used for executing the script. The read command is then used twice, each with the -p option, to prompt the user for input. Both the name and age variables take the name and age of the user and store them in the name and age variable with the help of the read command . Then the script uses the echo command to print the values of the variable’s name and age , respectively. The $ name and $age syntax are used to access the values stored in the variables and display them in the output.
Now, run the following command into your terminal to execute the bash file.
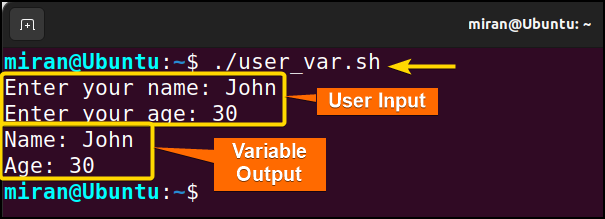
Case 03: Variable Assignment Using Positional Parameters
In Bash , you can assign values to variables using positional parameters . Positional parameters are special variables that hold arguments passed to the script when it is executed. They are referenced by their position, starting from $0 for the script name, $1 for the first argument , $2 for the second argument , and so on. To do the same use the below script.
Script (var_command_line.sh) >
The script starts with the shebang line ( #!/bin/bash ) to specify that the Bash shell should be used for executing the script. The script uses the special variables $1 and $2, which represent the first and second command – line arguments , respectively, and assigns their values to the name and age variables . The $ name and $age syntax are used to access the values stored in the variables and display them using the echo command .
Now, run the following command into your terminal to execute the script.
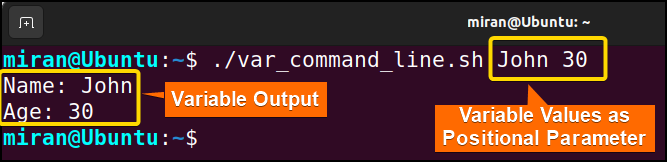
Upon execution of the Bash file , the script takes two command-line arguments, John and 30 , and returns the output Name : John and Age : 30 in the command line.
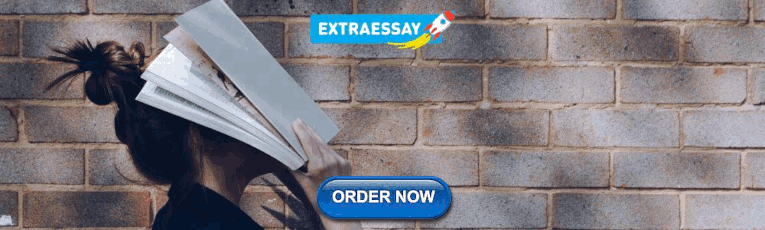
Case 04: Environment Variables and Variable Scope
In Bash script , Environment Variables are another type of variable. Those variables are available in the current session and its child processes once you export your own variable in the environment. However, you can access those and scope it to a function. Here’s a sample Bash script code of environment variables and variable scope describing the concept.
Script (var_scope.sh) >
The script starts with the shebang line ( #!/bin/bash ) to specify that the Bash shell should be used for executing the script. Then, an environment variable named MY_VARIABLE is assigned the value “ Hello, World! ” using the export command . The script also defines a Bash function called my_function . Inside this function, a local variable named local_var is declared using the local keyword . After defining the function, the script proceeds to print the value of the environment variable MY_VARIABLE , which is accessible throughout the script. Upon calling the my_function , it prints the value of the local variable local_var , demonstrating its local scope .
Finally, run the following command into your terminal to execute the bash file.
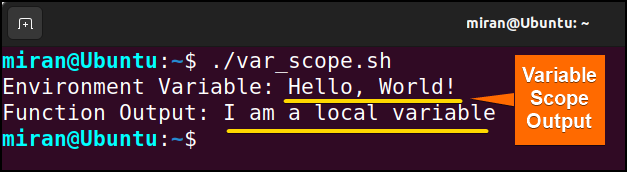
Upon printing the My_VARIABLE and calling the my_function, the code returns “ Environment Variable: Hello, World ” and “ I am a local variable ” respectively.
In conclusion, variable declaration and assignment is a very straightforward process and does not take any extra effort to declare the variable first, just like the Python programming language . In this article, I have tried to give you a guideline on how to declare and assign variables in a Bash Scripts . I have also provided some practical cases related to this topic. However, if you have any questions or queries regarding this article, feel free to comment below. I will get back to you soon. Thank You!
People Also Ask
Related Articles
- How to Declare Variable in Bash Scripts? [5 Practical Cases]
- Bash Variable Naming Conventions in Shell Script [6 Rules]
- How to Assign Variables in Bash Script? [8 Practical Cases]
- How to Check Variable Value Using Bash Scripts? [5 Cases]
- How to Use Default Value in Bash Scripts? [2 Methods]
- How to Use Set – $Variable in Bash Scripts? [2 Examples]
- How to Read Environment Variables in Bash Script? [2 Methods]
- How to Export Environment Variables with Bash? [4 Examples]
<< Go Back to Bash Variables | Bash Scripting Tutorial
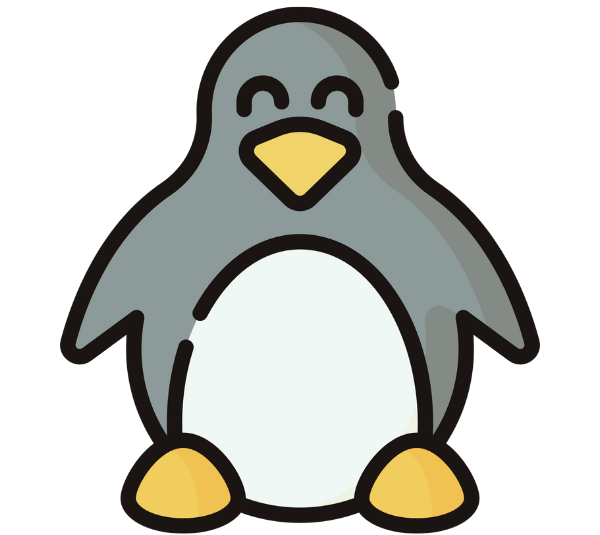
Mohammad Shah Miran
Hey, I'm Mohammad Shah Miran, previously worked as a VBA and Excel Content Developer at SOFTEKO, and for now working as a Linux Content Developer Executive in LinuxSimply Project. I completed my graduation from Bangladesh University of Engineering and Technology (BUET). As a part of my job, i communicate with Linux operating system, without letting the GUI to intervene and try to pass it to our audience.
Leave a Comment Cancel reply
Save my name, email, and website in this browser for the next time I comment.
- bash / FAQ / Shell Scripting
Bash variable assignment examples
by Ramakanta · Published January 19, 2013 · Updated February 11, 2015

This section we will describe the following: 1. Variable assignment 2. Variable substitution 3. Built-in shell variables 4. Other shell variables 5. Variable Assignment
Variable names consist of any number of letters, digits, or underscores. Upper- and lowercase letters are distinct, and names may not start with a digit.
Variables are assigned values using the = operator. There may not be any whitespace between the variable name and the value. You can make multiple assignments on the same line by separating each one with whitespace:
By convention, names for variables used or set by the shell have all uppercase letters; however, you can use uppercase names in your scripts if you use a name that isn’t special to the shell. By default, the shell treats variable values as strings, even if the value of the string is all digits. However, when a value is assigned to an integer variable (created via declare -i), Bash evaluates the righthand side of the assignment as an expression.
For example:
The += operator allows you to add or append the righthand side of the assignment to an existing value. Integer variables treat the righthand side as an expression, which is evaluated and added to the value. Arrays add the new elements to the array.
Variable Substitution
No spaces should be used in the following expressions. The colon (:) is optional; if it’s included, var must be nonnull as well as set.
var=value … Set each variable var to a value.
${var} Use value of var; braces are optional if var is separated from the following text. They are required for array variables.
${var:-value} Use var if set; otherwise, use value.
${var:=value} Use var if set; otherwise, use value and assign value to var.
${var:?value} Use var if set; otherwise, print value and exit (if not interactive). If value isn’t supplied, print the phrase parameter null or not set.
${var:+value} Use value if var is set; otherwise, use nothing.
${#var} Use the length of var.
${#*} Use the number of positional parameters.
${#@} Same as previous.
${var#pattern} Use value of var after removing text matching pattern from the left. Remove the shortest matching piece.
${var##pattern} Same as #pattern, but remove the longest matching piece.
${var%pattern} Use value of var after removing text matching pattern from the right. Remove the shortest matching piece.
${var%%pattern} Same as %pattern, but remove the longest matching piece.
${var^pattern} Convert the case of var to uppercase. The pattern is evaluated as for filename matching. If the first letter of var matches the pattern, it is converted to uppercase. var can be * or @, in which case the positional parameters are modified. var can also be an array subscripted by * or @, in which case the substitution is applied to all the elements of the array.
${var^^pattern} Same as ^pattern, but apply the match to every letter in the string.
${var,pattern} Same as ^pattern, but convert matching characters to lower case. Applies only to the first character in the string.
${var,,pattern} Same as ,pattern, but apply the match to every letter in the string.
${!prefix*},${!prefix@} List of variables whose names begin with prefix.
${var:pos},${var:pos:len} Starting at position pos (0-based) in variable var, extract len characters, or extract rest of string if no len. pos and len may be arithmetic expressions.When var is * or @, the expansion is performed upon the positional parameters. If pos is zero, then $0 is included in the resulting list. Similarly, var can be an array indexed by * or @.
${var/pat/repl} Use value of var, with first match of pat replaced with repl.
${var/pat} Use value of var, with first match of pat deleted.
${var//pat/repl} Use value of var, with every match of pat replaced with repl.
${var/#pat/repl} Use value of var, with match of pat replaced with repl. Match must occur at beginning of the value.
${var/%pat/repl} Use value of var, with match of pat replaced with repl. Match must occur at end of the value.
${!var} Use value of var as name of variable whose value should be used (indirect reference).
Bash provides a special syntax that lets one variable indirectly reference another:
$ greet=”hello, world” Create initial variable
$ friendly_message=greet Aliasing variable
$ echo ${!friendly_message} Use the alias
hello, world Example:
Built-in Shell Variables
Built-in variables are automatically set by the shell and are typically used inside shell scripts. Built-in variables can make use of the variable substitution patterns shown previously. Note that the $ is not actually part of the variable name, although the variable is always referenced this way. The following are
available in any Bourne-compatible shell:
$# Number of command-line arguments.
$- Options currently in effect (supplied on command line or to set). The shell sets some options automatically.
$? Exit value of last executed command.
$$ Process number of the shell.
$! Process number of last background command.
$0 First word; that is, the command name. This will have the full pathname if it was found via a PATH search.
$n Individual arguments on command line (positional parameters).
The Bourne shell allows only nine parameters to be referenced directly (n = 1–9); Bash allows n to be greater than 9 if specified as ${n}.
$*, $@ All arguments on command line ($1 $2 …).
“$*” All arguments on command line as one string (“$1 $2…”). The values are separated by the first character in $IFS.
“$@” All arguments on command line, individually quoted (“$1” “$2” …). Bash automatically sets the following additional variables: $_ Temporary variable; initialized to pathname of script or program being executed. Later, stores the last argument of previous command. Also stores name of matching MAIL file during mail checks.
BASH The full pathname used to invoke this instance of Bash.
BASHOPTS A read-only, colon-separated list of shell options that are currently enabled. Each item in the list is a valid option for shopt -s. If this variable exists in the environment when Bash starts up, it sets the indicated options before executing any startup files.
BASHPID The process ID of the current Bash process. In some cases, this can differ from $$.
BASH_ALIASES Associative array variable. Each element holds an alias defined with the alias command. Adding an element to this array creates a new alias; removing an element removes the corresponding alias.
BASH_ARGC Array variable. Each element holds the number of arguments for the corresponding function or dot-script invocation. Set only in extended debug mode, with shopt –s extdebug. Cannot be unset.
BASH_ARGV An array variable similar to BASH_ARGC. Each element is one of the arguments passed to a function or dot-script. It functions as a stack, with values being pushed on at each call. Thus, the last element is the last argument to the most recent function or script invocation. Set only in extended debug
mode, with shopt -s extdebug. Cannot be unset.
BASH_CMDS Associative array variable. Each element refers to a command in the internal hash table maintained by the hash command. The index is the command name and the value is the full path to the command. Adding an element to this array adds a command to the hash table; removing an element removes the corresponding entry.
BASH_COMMAND The command currently executing or about to be executed. Inside a trap handler, it is the command running when the trap was invoked.
BASH_EXECUTION_STRING The string argument passed to the –c option.
BASH_LINENO Array variable, corresponding to BASH_SOURCE and FUNCNAME. For any given function number i (starting at zero), ${FUNCNAME[i]} was invoked in file ${BASH_SOURCE[i]} on line ${BASH_LINENO[i]}. The information is stored with the most recent function invocation first. Cannot be unset.
BASH_REMATCH Array variable, assigned by the =~ operator of the [[ ]] construct. Index zero is the text that matched the entire pattern. The other
indices are the text matched by parenthesized subexpressions. This variable is read-only.
BASH_SOURCE Array variable, containing source filenames. Each element corresponds to those in FUNCNAME and BASH_LINENO. Cannot be unset.
BASH_SUBSHELL This variable is incremented by one each time a subshell or subshell environment is created.
BASH_VERSINFO[0] The major version number, or release, of Bash.
BASH_VERSINFO[1] The minor version number, or version, of Bash.
BASH_VERSINFO[2] The patch level.
BASH_VERSINFO[3] The build version.
BASH_VERSINFO[4] The release status.
BASH_VERSINFO[5] The machine type; same value as in $MACHTYPE.
BASH_VERSION A string describing the version of Bash.
COMP_CWORD For programmable completion. Index into COMP_WORDS, indicating the current cursor position.
COMP_KEY For programmable completion. The key, or final key in a sequence, that caused the invocation of the current completion function.
COMP_LINE For programmable completion. The current command line.
COMP_POINT For programmable completion. The position of the cursor as a character index in $COMP_LINE.
COMP_TYPE For programmable completion. A character describing the type of programmable completion. The character is one of Tab for normal completion, ? for a completions list after two Tabs, ! for the list of alternatives on partial word completion, @ for completions if the word is modified, or % for menu completion.
COMP_WORDBREAKS For programmable completion. The characters that the readline library treats as word separators when doing word completion.
COMP_WORDS For programmable completion. Array variable containing the individual words on the command line.
COPROC Array variable that holds the file descriptors used for communicating with an unnamed coprocess.
DIRSTACK Array variable, containing the contents of the directory stack as displayed by dirs. Changing existing elements modifies the stack, but only pushd and popd can add or remove elements from the stack.
EUID Read-only variable with the numeric effective UID of the current user.
FUNCNAME Array variable, containing function names. Each element corresponds to those in BASH_SOURCE and BASH_LINENO.
GROUPS Array variable, containing the list of numeric group IDs in which the current user is a member.
HISTCMD The history number of the current command.
HOSTNAME The name of the current host.
HOSTTYPE A string that describes the host system.
LINENO Current line number within the script or function.
MACHTYPE A string that describes the host system in the GNU cpu-company-system format.
MAPFILE Default array for the mapfile and readarray commands.
OLDPWD Previous working directory (set by cd).
OPTARG Value of argument to last option processed by getopts.
OPTIND Numerical index of OPTARG.
OSTYPE A string that describes the operating system.
PIPESTATUS Array variable, containing the exit statuses of the commands in the most recent foreground pipeline.
PPID Process number of this shell’s parent.
PWD Current working directory (set by cd).
RANDOM[=n] Generate a new random number with each reference; start with integer n, if given.
READLINE_LINE For use with bind -x. The contents of the editing buffer are available in this variable.
READLINE_POINT For use with bind -x. The index in $READLINE_LINE of the insertion point.
REPLY Default reply; used by select and read.
SECONDS[=n] Number of seconds since the shell was started, or, if n is given, number of seconds since the assignment + n.
SHELLOPTS A read-only, colon-separated list of shell options (for set -o). If set in the environment at startup, Bash enables each option present in the list before reading any startup files.
SHLVL Incremented by one every time a new Bash starts up.
UID Read-only variable with the numeric real UID of the current user.
Other Shell Variables
The following variables are not automatically set by the shell, although many of them can influence the shell’s behavior. You typically use them in your .bash_profile or .profile file, where you can define them to suit your needs. Variables can be assigned values by issuing commands of the form:
This list includes the type of value expected when defining these variables:
BASH_ENV If set at startup, names a file to be processed for initialization commands. The value undergoes parameter expansion, command substitution, and arithmetic expansion before being interpreted as a filename.
BASH_XTRACEFD=n File descriptor to which Bash writes trace output (from set -x).
CDPATH=dirs Directories searched by cd; allows shortcuts in changing directories; unset by default.
COLUMNS=n Screen’s column width; used in line edit modes and select lists.
COMPREPLY=(words …) Array variable from which Bash reads the possible completions generated by a completion function.
EMACS If the value starts with t, Bash assumes it’s running in an Emacs buffer and disables line editing.
ENV=file Name of script that is executed at startup in POSIX mode or when Bash is invoked as /bin/sh; useful for storing alias and function definitions. For example, ENV=$HOME/.shellrc.
FCEDIT=file Editor used by fc command. The default is /bin/ed when Bash is in POSIX mode. Otherwise, the default is $EDITOR if set, vi if unset.
FIGNORE=patlist Colon-separated list of patterns describing the set of filenames to ignore when doing filename completion.
GLOBIGNORE=patlist Colon-separated list of patterns describing the set of filenames to ignore during pattern matching.
HISTCONTROL=list Colon-separated list of values controlling how commands are saved in the history file. Recognized values are ignoredups, ignorespace, ignoreboth, and erasedups.
HISTFILE=file File in which to store command history.
HISTFILESIZE=n Number of lines to be kept in the history file. This may be different from the number of commands.
HISTIGNORE=list A colon-separated list of patterns that must match the entire command line. Matching lines are not saved in the history file. An unescaped & in a pattern matches the previous history line.
HISTSIZE=n Number of history commands to be kept in the history file.
HISTTIMEFORMAT=string A format string for strftime(3) to use for printing timestamps along with commands from the history command. If set (even if null), Bash saves timestamps in the history file along with the commands.
HOME=dir Home directory; set by login (from /etc/passwd file).
HOSTFILE=file Name of a file in the same format as /etc/hosts that Bash should use to find hostnames for hostname completion.
IFS=’chars’ Input field separators; default is space, Tab, and newline.
IGNOREEOF=n Numeric value indicating how many successive EOF characters must be typed before Bash exits. If null or nonnumeric value, default is 10.
INPUTRC=file Initialization file for the readline library. This overrides the default value of ~/.inputrc.
LANG=locale Default value for locale; used if no LC_* variables are set.
LC_ALL=locale Current locale; overrides LANG and the other LC_* variables.
LC_COLLATE=locale Locale to use for character collation (sorting order).
LC_CTYPE=locale Locale to use for character class functions.
LC_MESSAGES=locale Locale to use for translating $”…” strings.
LC_NUMERIC=locale Locale to use for the decimal-point character.
LC_TIME=locale Locale to use for date and time formats.
LINES=n Screen’s height; used for select lists.
MAIL=file Default file to check for incoming mail; set by login.
MAILCHECK=n Number of seconds between mail checks; default is 600 (10 minutes).
MAILPATH=files One or more files, delimited by a colon, to check for incoming mail. Along with each file, you may supply an optional message that the shell prints when the file increases in size. Messages are separated from the filename by a ? character, and the default message is You have mail in $_. $_ is replaced with the name of the file. For example, you might have MAIL PATH=”$MAIL?Candygram!:/etc/motd?New Login Message” OPTERR=n When set to 1 (the default value), Bash prints error messages from the built-in getopts command.
PATH=dirlist One or more pathnames, delimited by colons, in which to search for commands to execute. The default for many systems is /bin:/usr/bin. On Solaris, the default is /usr/bin:. However, the standard startup scripts change it to /usr/bin:/usr/ucb:/etc:.
POSIXLY_CORRECT=string When set at startup or while running, Bash enters POSIX mode, disabling behavior and modifying features that conflict with the POSIX standard.
PROMPT_COMMAND=command If set, Bash executes this command each time before printing the primary prompt.
PROMPT_DIRTRIM=n Indicates how many trailing directory components to retain for the \w or \W special prompt strings. Elided components are replaced with an ellipsis.
PS1=string Primary prompt string; default is $.
PS2=string Secondary prompt (used in multiline commands); default is >.
PS3=string Prompt string in select loops; default is #?.
PS4=string Prompt string for execution trace (bash –x or set -x); default is +.
SHELL=file Name of user’s default shell (e.g., /bin/sh). Bash sets this if it’s not in the environment at startup.
TERM=string Terminal type.
TIMEFORMAT=string A format string for the output from the time keyword.
TMOUT=n If no command is typed after n seconds, exit the shell. Also affects the read command and the select loop.
TMPDIR=directory Place temporary files created and used by the shell in directory.
auto_resume=list Enables the use of simple strings for resuming stopped jobs. With a value of exact, the string must match a command name exactly. With a value of substring, it can match a substring of the command name.
histchars=chars Two or three characters that control Bash’s csh-style history expansion. The first character signals a history event, the second is the “quick substitution” character, and the third indicates the start of a comment. The default value is !^#.
In case of any ©Copyright or missing credits issue please check CopyRights page for faster resolutions.
Like the article... Share it.
- Click to print (Opens in new window)
- Click to email a link to a friend (Opens in new window)
- Click to share on Reddit (Opens in new window)
- Click to share on Pinterest (Opens in new window)
- Click to share on LinkedIn (Opens in new window)
- Click to share on WhatsApp (Opens in new window)
- Click to share on Twitter (Opens in new window)
- Click to share on Facebook (Opens in new window)
- Click to share on Tumblr (Opens in new window)
- Click to share on Pocket (Opens in new window)
- Click to share on Telegram (Opens in new window)
CURRENTLY TRENDING...
Tags: bash variable
- Next story Grep Command Tutorial For Unix
- Previous story Bash redirect to file and screen
Leave a Reply Cancel reply
This site uses Akismet to reduce spam. Learn how your comment data is processed .
Sign Up For Our Free Email Newsletter
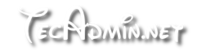
Assignment Operators in Bash
In the Bash shell, assignment operators are used to assign values to variables. They are essential tools in scripting and programming, providing a method to store and manipulate data. This article will take you through the fundamental assignment operators in Bash, along with examples of their usage.
Standard Assignment Operator
In Bash, the standard assignment operator is the `=` symbol. It is used to assign the value on the right-hand side to the variable on the left-hand side. There should not be any spaces around the `=` operator. Here is an example:
In this example, the variable `NAME` is assigned the value “John Doe” . If you use `echo $NAME` , the output will be “John Doe” .
Compound Assignment Operators
Compound assignment operators combine an operation and an assignment into a single operation.
Please note that Bash only supports integer arithmetic natively. If you need to perform operations with floating-point numbers, you will need to use external tools like bc.
Read-only Assignment Operator
The readonly operator is used to make a variable’s value constant, which means the value assigned to the variable cannot be changed later. If you try to change the value of a readonly variable, Bash will give an error.
In the above example, PI is declared as a readonly variable and assigned a value of 3.14 . When we try to reassign the value 3.1415 to PI , Bash will give an error message: bash: PI: readonly variable .
Local Assignment Operator
The local operator is used within functions to create a local variable – a variable that can only be accessed within the function where it was declared.
In the above example, MY_VAR is declared as a local variable in the my_func function. When we call the function, it prints “I am local” . However, when we try to echo MY_VAR outside of the function, it prints nothing because MY_VAR is not accessible outside my_func .
Bash assignment operators are a crucial part of shell scripting, enabling the storage and manipulation of data. By understanding and using these operators effectively, you can enhance the functionality and efficiency of your scripts. This article covered the basic assignment operator, compound assignment operators, and special assignment operators like readonly and local. Understanding how and when to use each operator is a key aspect of mastering Bash scripting.
Related Posts
What is the “/dev/null 2>&1” in bash: a comprehensive guide, using the ‘-ge’ operator in bash: a comprehensive guide.
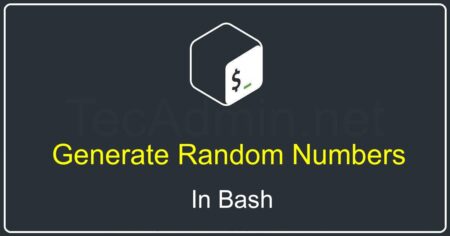
Generate Random Numbers in Bash
Save my name, email, and website in this browser for the next time I comment.
Type above and press Enter to search. Press Esc to cancel.
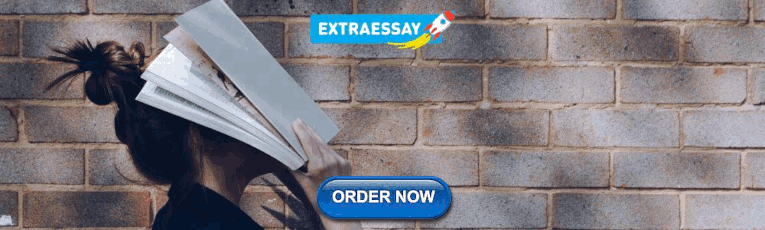
IMAGES
VIDEO
COMMENTS
Assigning multiple variables in a single line of code is a handy feature in some programming languages, such as Python and PHP. In this quick tutorial, we'll take a closer look at how to do multiple variable assignment in Bash scripts. 2. Multiple Variable Assignment
Chapter 5 of the Bash Cookbook by O'Reilly, discusses (at some length) the reasons for the requirement in a variable assignment that there be no spaces around the '=' sign MYVAR="something" The explanation has something to do with distinguishing between the name of a command and a variable (where '=' may be a valid argument).
As the image depicts above, the var_int variables return the assigned value 23 as the Student ID.. Case 02: Multi-Variable Assignment in a Single Line of a Bash Script. Multi-variable assignment in a single line is a concise and efficient way of assigning values to multiple variables simultaneously in Bash scripts.This method helps reduce the number of lines of code and can enhance readability ...
A possible caveat is SC2155 in that you should declare and assign separately. That being said it will work except for using multiple declare options between the parameters. Also note that the declare parameters will apply to all variables (in this case -i). script0: #!/bin/bash declare a b c a=foo b=bar c=baz foo { local a=1 b=2 c=3 echo "From ...
Bash: let Statement vs. Assignment. 1. Overview. The built-in let command is part of the Bash and Korn shell fundamental software. Its main usage focuses on building variable assignments with basic arithmetic operations. In this article, we describe the typical usage syntax of the command. Thereupon, we show all meaningful properties that ...
Here, we'll create five variables. The format is to type the name, the equals sign =, and the value. Note there isn't a space before or after the equals sign. Giving a variable a value is often referred to as assigning a value to the variable. We'll create four string variables and one numeric variable, my_name=Dave.
Assigning multiple variables in a single row the code is a handy feature into some programming languages, create as Python and PHP. In this quick training, we'll take a nearest show at how to do multiple variable assignment includes Rush scripts. 2. Multiple Variable Assignment
Bash Local Variables To create a variable, you need to assign a value to your variable name. Bash is an untyped language, so you don't have to indicate a data type when defining your variables. var1=Hello. Bash also allows multiple assignments on a single line: a=6 b=8 c=9
The script starts with the shebang line (#!/bin/bash), specifying that the script should be executed using the Bash shell.Next, two variables are declared and assigned values using the format variable_name=value.The first variable is name, and it is assigned the string value "John."The second variable is age, assigned the numeric value 30.The script then uses the echo command to print the ...
The solutions given by esuoxu and Mickaël Bucas are the common and more portable ways of doing this.. Here are a few bash solutions (some of which should also work in other shells, like zsh).Firstly with the += append operator (which works in a slightly different way for each of an integer variable, a regular variable and an array).. text="Lorem ipsum dolor sit amet, consectetur adipisicing ...
Now, I'd like to assign the second and fourth word of the output (in this case two and four) to the variables w1 and w2. I thought I could use the read command like so: echo "one two three four" | awk '{print $2 " " $4}' | read w1 w2 but this doesn't work, probably because the read command is executed in a sub-process.
For use in a shell script, files need to be delimited by something useful. You asked, "How can I do such multiline assignment in shell", but the assignment in your example is actually a SINGLE line, with the ^ at the end of each input line negating the following newline ( not escaping it, as another answer suggested).
Bash variable assignment examples. by Ramakanta · Published January 19, 2013 · Updated February 11, 2015. This section we will describe the following: 1. ... You can make multiple assignments on the same line by separating each one with whitespace: firstname=Arnold lastname=Robbins numkids=4 numpets=1
In the Bash shell, assignment operators are used to assign values to variables. They are essential tools in scripting and programming, providing a method to store and manipulate data. This article will take you through the fundamental assignment operators in Bash, along with examples of their usage. Standard Assignment Operator In Bash, the standard assignment
Since the output shows, the assignment works as we expect. Using the multiple variable assignment instrumentation the Bash scripts can make ours code look compact and give us the benefit away better capacity, particularly when we want go assign multiple set by the output to expensive command execution.
You need to carefully type in the Tab character for the lines starting with the here-doc and the lines ending the here-doc. My vim configuration has mapped the tab character to 8 spaces. If you want to make it even neater, modify the spacing rule in vim by setting the spacing for tab to 4 spaces as :set tabstop=4.
Bash multiple variable assignment. Ask Question Asked 6 years, 5 months ago. Modified 6 years, 5 months ago. Viewed 951 times 2 Objective. Assign HTTP status code and date from cURL output to RESP and DATE variables using one-liner command. Expectation [08 Nov 2017 19:28:44 GMT] 301 ...
As WP says, $(()) can only contain arithmetic expressions and therefore, a general ternary assignment doesn't exist in Bash. - zakmck. Apr 10, 2023 at 18:49. @zakmck i think you should check again. try the code in the example. - mikeserv. ... EC261 with multiple connections
Whereas test2.sh is attempting to concatenate strings and assign to a variable using an incorrect concatenation method. To break a string across lines simply leave off the closing quote and continue on the next line, like so: text="some text \. some more text". Share.
From man bash: IFS : The Internal Field Separator that is used for word splitting after expansion and to split lines into words with the read builtin command. Share