
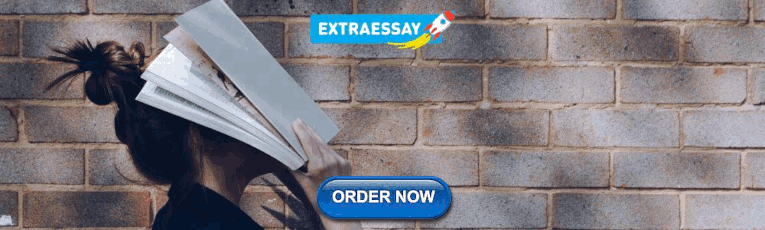
21.12 — Overloading the assignment operator
The copy assignment operator (operator=) is used to copy values from one object to another already existing object .
Related content
As of C++11, C++ also supports “Move assignment”. We discuss move assignment in lesson 22.3 -- Move constructors and move assignment .
Copy assignment vs Copy constructor
The purpose of the copy constructor and the copy assignment operator are almost equivalent -- both copy one object to another. However, the copy constructor initializes new objects, whereas the assignment operator replaces the contents of existing objects.
The difference between the copy constructor and the copy assignment operator causes a lot of confusion for new programmers, but it’s really not all that difficult. Summarizing:
- If a new object has to be created before the copying can occur, the copy constructor is used (note: this includes passing or returning objects by value).
- If a new object does not have to be created before the copying can occur, the assignment operator is used.
Overloading the assignment operator
Overloading the copy assignment operator (operator=) is fairly straightforward, with one specific caveat that we’ll get to. The copy assignment operator must be overloaded as a member function.
This prints:
This should all be pretty straightforward by now. Our overloaded operator= returns *this, so that we can chain multiple assignments together:
Issues due to self-assignment
Here’s where things start to get a little more interesting. C++ allows self-assignment:
This will call f1.operator=(f1), and under the simplistic implementation above, all of the members will be assigned to themselves. In this particular example, the self-assignment causes each member to be assigned to itself, which has no overall impact, other than wasting time. In most cases, a self-assignment doesn’t need to do anything at all!
However, in cases where an assignment operator needs to dynamically assign memory, self-assignment can actually be dangerous:
First, run the program as it is. You’ll see that the program prints “Alex” as it should.
Now run the following program:
You’ll probably get garbage output. What happened?
Consider what happens in the overloaded operator= when the implicit object AND the passed in parameter (str) are both variable alex. In this case, m_data is the same as str.m_data. The first thing that happens is that the function checks to see if the implicit object already has a string. If so, it needs to delete it, so we don’t end up with a memory leak. In this case, m_data is allocated, so the function deletes m_data. But because str is the same as *this, the string that we wanted to copy has been deleted and m_data (and str.m_data) are dangling.
Later on, we allocate new memory to m_data (and str.m_data). So when we subsequently copy the data from str.m_data into m_data, we’re copying garbage, because str.m_data was never initialized.
Detecting and handling self-assignment
Fortunately, we can detect when self-assignment occurs. Here’s an updated implementation of our overloaded operator= for the MyString class:
By checking if the address of our implicit object is the same as the address of the object being passed in as a parameter, we can have our assignment operator just return immediately without doing any other work.
Because this is just a pointer comparison, it should be fast, and does not require operator== to be overloaded.
When not to handle self-assignment
Typically the self-assignment check is skipped for copy constructors. Because the object being copy constructed is newly created, the only case where the newly created object can be equal to the object being copied is when you try to initialize a newly defined object with itself:
In such cases, your compiler should warn you that c is an uninitialized variable.
Second, the self-assignment check may be omitted in classes that can naturally handle self-assignment. Consider this Fraction class assignment operator that has a self-assignment guard:
If the self-assignment guard did not exist, this function would still operate correctly during a self-assignment (because all of the operations done by the function can handle self-assignment properly).
Because self-assignment is a rare event, some prominent C++ gurus recommend omitting the self-assignment guard even in classes that would benefit from it. We do not recommend this, as we believe it’s a better practice to code defensively and then selectively optimize later.
The copy and swap idiom
A better way to handle self-assignment issues is via what’s called the copy and swap idiom. There’s a great writeup of how this idiom works on Stack Overflow .
The implicit copy assignment operator
Unlike other operators, the compiler will provide an implicit public copy assignment operator for your class if you do not provide a user-defined one. This assignment operator does memberwise assignment (which is essentially the same as the memberwise initialization that default copy constructors do).
Just like other constructors and operators, you can prevent assignments from being made by making your copy assignment operator private or using the delete keyword:
Note that if your class has const members, the compiler will instead define the implicit operator= as deleted. This is because const members can’t be assigned, so the compiler will assume your class should not be assignable.
If you want a class with const members to be assignable (for all members that aren’t const), you will need to explicitly overload operator= and manually assign each non-const member.
C++ Tutorial
C++ functions, c++ classes, c++ reference, c++ examples, c++ assignment operators, assignment operators.
Assignment operators are used to assign values to variables.
In the example below, we use the assignment operator ( = ) to assign the value 10 to a variable called x :
The addition assignment operator ( += ) adds a value to a variable:
A list of all assignment operators:

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
This browser is no longer supported.
Upgrade to Microsoft Edge to take advantage of the latest features, security updates, and technical support.
Copy constructors and copy assignment operators (C++)
- 8 contributors
Starting in C++11, two kinds of assignment are supported in the language: copy assignment and move assignment . In this article "assignment" means copy assignment unless explicitly stated otherwise. For information about move assignment, see Move Constructors and Move Assignment Operators (C++) .
Both the assignment operation and the initialization operation cause objects to be copied.
Assignment : When one object's value is assigned to another object, the first object is copied to the second object. So, this code copies the value of b into a :
Initialization : Initialization occurs when you declare a new object, when you pass function arguments by value, or when you return by value from a function.
You can define the semantics of "copy" for objects of class type. For example, consider this code:
The preceding code could mean "copy the contents of FILE1.DAT to FILE2.DAT" or it could mean "ignore FILE2.DAT and make b a second handle to FILE1.DAT." You must attach appropriate copying semantics to each class, as follows:
Use an assignment operator operator= that returns a reference to the class type and takes one parameter that's passed by const reference—for example ClassName& operator=(const ClassName& x); .
Use the copy constructor.
If you don't declare a copy constructor, the compiler generates a member-wise copy constructor for you. Similarly, if you don't declare a copy assignment operator, the compiler generates a member-wise copy assignment operator for you. Declaring a copy constructor doesn't suppress the compiler-generated copy assignment operator, and vice-versa. If you implement either one, we recommend that you implement the other one, too. When you implement both, the meaning of the code is clear.
The copy constructor takes an argument of type ClassName& , where ClassName is the name of the class. For example:
Make the type of the copy constructor's argument const ClassName& whenever possible. This prevents the copy constructor from accidentally changing the copied object. It also lets you copy from const objects.
Compiler generated copy constructors
Compiler-generated copy constructors, like user-defined copy constructors, have a single argument of type "reference to class-name ." An exception is when all base classes and member classes have copy constructors declared as taking a single argument of type const class-name & . In such a case, the compiler-generated copy constructor's argument is also const .
When the argument type to the copy constructor isn't const , initialization by copying a const object generates an error. The reverse isn't true: If the argument is const , you can initialize by copying an object that's not const .
Compiler-generated assignment operators follow the same pattern for const . They take a single argument of type ClassName& unless the assignment operators in all base and member classes take arguments of type const ClassName& . In this case, the generated assignment operator for the class takes a const argument.
When virtual base classes are initialized by copy constructors, whether compiler-generated or user-defined, they're initialized only once: at the point when they are constructed.
The implications are similar to the copy constructor. When the argument type isn't const , assignment from a const object generates an error. The reverse isn't true: If a const value is assigned to a value that's not const , the assignment succeeds.
For more information about overloaded assignment operators, see Assignment .
Was this page helpful?
Coming soon: Throughout 2024 we will be phasing out GitHub Issues as the feedback mechanism for content and replacing it with a new feedback system. For more information see: https://aka.ms/ContentUserFeedback .
Submit and view feedback for
Additional resources
Copy assignment operator
A copy assignment operator of class T is a non-template non-static member function with the name operator = that takes exactly one parameter of type T , T & , const T & , volatile T & , or const volatile T & . A type with a public copy assignment operator is CopyAssignable .
[ edit ] Syntax
[ edit ] explanation.
- Typical declaration of a copy assignment operator when copy-and-swap idiom can be used
- Typical declaration of a copy assignment operator when copy-and-swap idiom cannot be used
- Forcing a copy assignment operator to be generated by the compiler
- Avoiding implicit copy assignment
The copy assignment operator is called whenever selected by overload resolution , e.g. when an object appears on the left side of an assignment expression.
[ edit ] Implicitly-declared copy assignment operator
If no user-defined copy assignment operators are provided for a class type ( struct , class , or union ), the compiler will always declare one as an inline public member of the class. This implicitly-declared copy assignment operator has the form T & T :: operator = ( const T & ) if all of the following is true:
- each direct base B of T has a copy assignment operator whose parameters are B or const B& or const volatile B &
- each non-static data member M of T of class type or array of class type has a copy assignment operator whose parameters are M or const M& or const volatile M &
Otherwise the implicitly-declared copy assignment operator is declared as T & T :: operator = ( T & ) . (Note that due to these rules, the implicitly-declared copy assignment operator cannot bind to a volatile lvalue argument)
A class can have multiple copy assignment operators, e.g. both T & T :: operator = ( const T & ) and T & T :: operator = ( T ) . If some user-defined copy assignment operators are present, the user may still force the generation of the implicitly declared copy assignment operator with the keyword default .
Because the copy assignment operator is always declared for any class, the base class assignment operator is always hidden. If a using-declaration is used to bring in the assignment operator from the base class, and its argument type could be the same as the argument type of the implicit assignment operator of the derived class, the using-declaration is also hidden by the implicit declaration.
[ edit ] Deleted implicitly-declared copy assignment operator
The implicitly-declared or defaulted copy assignment operator for class T is defined as deleted in any of the following is true:
- T has a non-static data member that is const
- T has a non-static data member of a reference type.
- T has a non-static data member that cannot be copy-assigned (has deleted, inaccessible, or ambiguous copy assignment operator)
- T has direct or virtual base class that cannot be copy-assigned (has deleted, inaccessible, or ambiguous move assignment operator)
- T has a user-declared move constructor
- T has a user-declared move assignment operator
[ edit ] Trivial copy assignment operator
The implicitly-declared copy assignment operator for class T is trivial if all of the following is true:
- T has no virtual member functions
- T has no virtual base classes
- The copy assignment operator selected for every direct base of T is trivial
- The copy assignment operator selected for every non-static class type (or array of class type) memeber of T is trivial
A trivial copy assignment operator makes a copy of the object representation as if by std:: memmove . All data types compatible with the C language (POD types) are trivially copy-assignable.
[ edit ] Implicitly-defined copy assignment operator
If the implicitly-declared copy assignment operator is not deleted or trivial, it is defined (that is, a function body is generated and compiled) by the compiler. For union types, the implicitly-defined copy assignment copies the object representation (as by std:: memmove ). For non-union class types ( class and struct ), the operator performs member-wise copy assignment of the object's bases and non-static members, in their initialization order, using, using built-in assignment for the scalars and copy assignment operator for class types.
The generation of the implicitly-defined copy assignment operator is deprecated (since C++11) if T has a user-declared destructor or user-declared copy constructor.
[ edit ] Notes
If both copy and move assignment operators are provided, overload resolution selects the move assignment if the argument is an rvalue (either prvalue such as a nameless temporary or xvalue such as the result of std:: move ), and selects the copy assignment if the argument is lvalue (named object or a function/operator returning lvalue reference). If only the copy assignment is provided, all argument categories select it (as long as it takes its argument by value or as reference to const, since rvalues can bind to const references), which makes copy assignment the fallback for move assignment, when move is unavailable.
[ edit ] Copy and swap
Copy assignment operator can be expressed in terms of copy constructor, destructor, and the swap() member function, if one is provided:
T & T :: operator = ( T arg ) { // copy/move constructor is called to construct arg swap ( arg ) ; // resources exchanged between *this and arg return * this ; } // destructor is called to release the resources formerly held by *this
For non-throwing swap(), this form provides strong exception guarantee . For rvalue arguments, this form automatically invokes the move constructor, and is sometimes referred to as "unifying assignment operator" (as in, both copy and move).
[ edit ] Example
- Windows Programming
- UNIX/Linux Programming
- General C++ Programming
- copy assignment operator - arrays
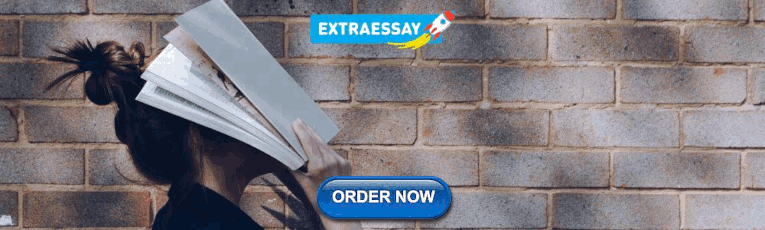
copy assignment operator - arrays

cppreference.com
Std:: array.
std::array is a container that encapsulates fixed size arrays.
This container is an aggregate type with the same semantics as a struct holding a C-style array T [ N ] as its only non-static data member. Unlike a C-style array, it doesn't decay to T * automatically. As an aggregate type, it can be initialized with aggregate-initialization given at most N initializers that are convertible to T : std :: array < int , 3 > a = { 1 , 2 , 3 } ; .
The struct combines the performance and accessibility of a C-style array with the benefits of a standard container, such as knowing its own size, supporting assignment, random access iterators, etc.
std::array satisfies the requirements of Container and ReversibleContainer except that default-constructed array is not empty and that the complexity of swapping is linear, satisfies the requirements of ContiguousContainer , (since C++17) and partially satisfies the requirements of SequenceContainer .
There is a special case for a zero-length array ( N == 0 ). In that case, array. begin ( ) == array. end ( ) , which is some unique value. The effect of calling front ( ) or back ( ) on a zero-sized array is undefined.
An array can also be used as a tuple of N elements of the same type.
[ edit ] Iterator invalidation
As a rule, iterators to an array are never invalidated throughout the lifetime of the array. One should take note, however, that during swap , the iterator will continue to point to the same array element, and will thus change its value.
[ edit ] Template parameters
[ edit ] member types, [ edit ] member functions, [ edit ] non-member functions, [ edit ] helper classes, [ edit ] example, [ edit ] see also.
- Todo with reason
- Recent changes
- Offline version
- What links here
- Related changes
- Upload file
- Special pages
- Printable version
- Permanent link
- Page information
- In other languages
- This page was last modified on 22 December 2023, at 23:28.
- This page has been accessed 4,686,769 times.
- Privacy policy
- About cppreference.com
- Disclaimers

Stay up-to-date with Modern C++
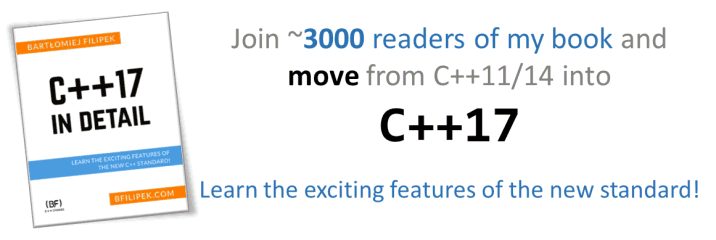
Last Update: 30 April 2024
Understand internals of std::expected
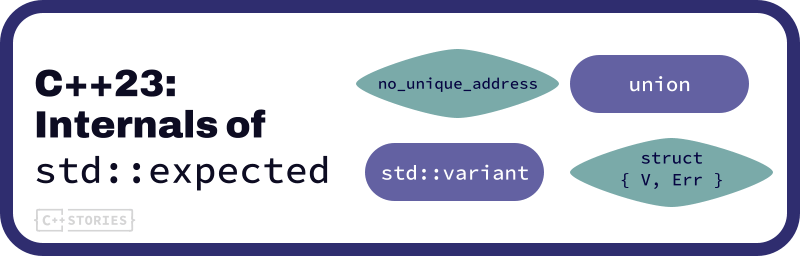
Table of Contents
In the article about std::expected, I introduced the type and showed some basic examples, and in this text, you’ll learn how it is implemented.
A simple idea with struct
In short, std::expected should contain two data members: the actual expected value and the unexpected error object. So, in theory, we could use a simple structure:
However, there are better solutions than this. Here are some obvious issues for our “struct” approach.
- The size of the object is the sum of the Value type and the Error type (plus padding if needed).
- Two data members are “active” and initialized, which might not be possible - for example, what if the Value type has no default constructor? The Standard requires that std::expected" holds either a value of type T or an error of type E` within its storage.
- We’d have to guarantee that _Ty cannot be a reference type or an array type; it must be a Destructible Type.
- Similarly for the _Err type we have to guarantee that it’s also Destructible , and must be a valid template argument for std::unexpected (so not an array, non-object type, nor cv-qualified type).
- Plus, we’d have to write a lot of code that creates an API for the type
How about std::variant ?
Ok, since we want to have a more compact type, why not use std::variant ?
std::variant is a tagged union, so it holds only one of the list of types and has an efficient way to switch between them.
We can try with the following code:
However, std::variant does not provide some specific behaviors that std::expected might need, such as conditional explicit constructors and assignment operators based on the contained types’ properties. So, additional work would be required to implement these properly.
Furthermore, std::variant has to be very generic and offers a way to handle many alternative types in one object, which is “too much” for the expected type, which needs only two alternatives.
Real implementation
Let’s look at some open implementations and see what’s under the hood.
We can go to the Microsoft STL repository:
https://github.com/microsoft/STL/blob/main/stl/inc/expected
The expected class uses a union to store either a value of type _Ty or an error of type _Err :
Here, _Value and _Unexpected are two data members that share the same memory location within an instance of expected . Which member of the union is currently active (i.e., contains valid data) is not tracked by the union itself. Therefore, the expected class maintains an additional boolean member, _Has_value , to track whether the union currently holds a _Ty value ( _Has_value is true) or an _Err error ( _Has_value is false).
As you can see, this approach is much more advanced than our simple structure and uses some ideas from std::variant .
Size of Objects:
The size of an expected object depends on several factors:
- Size of _Ty and _Err : The size of the union will be at least as large as the size of its largest member because the union allocates enough space to hold the largest member.
- Alignment : we have to honour _Ty ’s and _Err ’s alignment requirements.
- Boolean _Has_value : There’s also a boolean member variable _Has_value indicating which member of the union is active. This adds to the total size of the class.
So, the total size of an expected<_Ty, _Err> object will be approximately:
Here are some examples of the sizes on GCC x64:
You can check the code here: @Compiler Explorer .
Going further down
Let’s have a look at some other member functions:
Those are relatively simple: they check the flag and return the value.
On the other hand, one of the overloads for the assignment operators (see at this line ) is more complex:
The _Reinit_expected template function manages the transition of an std::expected object from one state to another. Because the code uses union, it has to manage the lifetime of alternatives.
Other implementations
- In GCC libstdc++ - expected @ Github - it also uses union to hold the alternatives
- In LLVM libc++: expected @Github - in many places it uses no_unique_address attribute and Empty Base Class Optimization so in theory if might use less space than the regular union approach.
Summary
In this text, I covered a rough idea of how to implement the std::expected type. As you can see, it’s not just a simple structure of <ValueT, ErrorT> and contains a lot of tricks to fulfill all of the requirements.
I believe that understanding and appreciating the patterns within the Standard Library can be valuable, even if we don’t need to delve too deeply into its implementation in our everyday C++ tasks.
See the introduction to std::expected in my previous article .
I've prepared a valuable bonus if you're interested in Modern C++! Learn all major features of recent C++ Standards! Check it out here:
Similar Articles:
- Using std::expected from C++23
- Parsing Numbers At Compile Time with C++17, C++23, and C++26
- Six Handy Operations for String Processing in C++20/23
- How to use std::span from C++20
- Spans, string_view, and Ranges - Four View types (C++17 to C++23)
IP address data type
This is an abstract data type and cannot be used directly with standard C unary or binary operators. Only local or global variables of type ip_addr_t are supported. A variable of this type can also be stored in an associate array either as a key or as a value.
Vue supports the following characteristics and operations for the IP address type variables:
The qualifiers signed, unsigned, register, static, thread and kernel are not supported for the ip_addr_t type variables.
Assignment operation
The assignment (=) operator allows a ip_addr_t type variable to be assigned to another ip_addr_t type variable and also it allows constant IP address or hostname to be assigned to ip_addr_t type variable. The original values of the variable is overwritten. No type casting is allowed from or to the ip_addr_t variable types.
Comparison operation
Only equality (==) and inequality (! =) operators are allowed for ip_addr_t types variables. The comparison allowed only between two ip_addr_t type variables and with constant string type (IP address or hostnames are provided in double quotes “192.168.1.1” or “example.com").
The result of the equality operator is True (1) if both contains the same IP address type (IPV4 or IPV6) and values. or False (0) otherwise. The inequality operator is the exact compliment of that. No other comparison operators (>=, >, < or =<) are allowed for the ip_addr_t type variables.
A ip_addr_t type variable can be printed with “%I” format specifier to print IP address in dotted decimal or hex format and “%H” format specifier to print hostname in the printf() function of Vue. This printing hostname involves a time consuming dns lookup operation. Hence it should be judiciously used in VUE scripts.
- The array of the ip_addr_t variable cannot be declared.
- Pointer to ip_addr_t variable is not allowed.
- Typecasting of ip_addr_t variable to any other type or typecasting any other type to ip_addr_t type is not allowed.
- No arithmetic operator (+, -, *, /, ++, -- etc) can be used with ip_addr_t type variable.
- DSA with JS - Self Paced
- JS Tutorial
- JS Exercise
- JS Interview Questions
- JS Operator
- JS Projects
- JS Examples
- JS Free JS Course
- JS A to Z Guide
- JS Formatter
- How to use Lodash to find & Return an Object from Array ?
- How to Sort an Array of Object using a Value in TypeScript ?
- How to Swap Two Array of Objects Values using JavaScript ?
- How to Find Property Values in an Array of Object using if/else Condition in JavaScript ?
- How to get distinct values from an array of objects in JavaScript?
- How to use forEach with an Array of Objects in JavaScript ?
- How to search the max value of an attribute in an array object ?
- How to filter an array of objects in ES6 ?
- JSON Modify an Array Value of a JSON Object
- How to use splice on Nested Array of Objects ?
- How to convert Object's array to an array using JavaScript ?
- How to Push an Object into an Array using For Loop in JavaScript ?
- How to find property values from an array containing multiple objects in JavaScript ?
- How Check if object value exists not add a new object to array using JavaScript ?
- How to remove duplicates from an array of objects using JavaScript ?
- How to remove object from array of objects using JavaScript ?
- JavaScript Program to Find Index of an Object by Key and Value in an Array
- How to Access Array of Objects in JavaScript ?
- How to Convert an Object into Array of Objects in JavaScript ?
- How to extract value of a property as array from an array of objects ?
- How to print object by id in an array of objects in JavaScript ?
- How to compare Arrays of Objects in JavaScript ?
- How to modify an object's property in an array of objects in JavaScript ?
- How to Convert Object to Array in JavaScript?
- How to get a key in a JavaScript object by its value ?
- How to check an Array Contains an Object of Attribute with Given Value in JavaScript ?
- How to use 'lodash' Package for Array Manipulation in a JavaScript Project ?
- How to get the same value from another array and assign to object of arrays ?
- How to Flatten Dynamically Nested Objects in Order in TypeScript ?
How to Find & Update Values in an Array of Objects using Lodash ?
To find and update values in an array of objects using Lodash, we employ utility functions like find or findIndex to iterate over the elements. These functions facilitate targeted updates based on specific conditions, enhancing data manipulation capabilities.
Table of Content
Using find and assign Functions
Using findindex function, run the below command to install lodash:.
In this approach, we are using Lodash’s find function to locate the object with an id equal to 102 in the courses array. Then, we use the assign method to update its duration property to ‘ 10 weeks ‘, demonstrating a targeted find-and-update operation in an array of objects using Lodash.
Example: The below example uses find and assign functions to find and update values in an array of objects using lodash.
In this approach, we use _.findIndex to locate the index of the object with an id equal to 3 in the student’s array. Then, we update the score property of that object to 80 if the index is found, demonstrating a targeted find-and-update operation using Lodash’s findIndex function.
Example: The below example uses findIndex to find and update values in an array of objects using lodash.
Please Login to comment...
Similar reads.
- JavaScript-Lodash
- Web Technologies
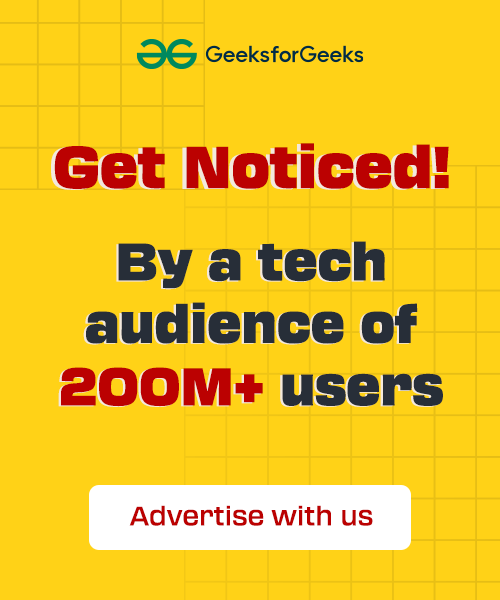
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
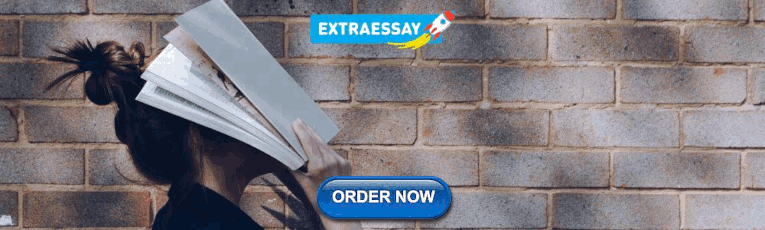
IMAGES
VIDEO
COMMENTS
for assignments to class type objects, the right operand could be an initializer list only when the assignment is defined by a user-defined assignment operator. removed user-defined assignment constraint. CWG 1538. C++11. E1 ={E2} was equivalent to E1 = T(E2) ( T is the type of E1 ), this introduced a C-style cast. it is equivalent to E1 = T{E2}
Mar 11, 2011 at 22:41. By the way, to achieve copy semantics with arrays a possible solution is to wrap them in a struct or in a class: the default copy constructor & assignment operator will do the trick. But in that case you will probably want to use directly the std::array template. - Matteo Italia.
Assignment performs implicit conversion from the value of rhs to the type of lhs and then replaces the value in the object designated by lhs with the converted value of rhs . Assignment also returns the same value as what was stored in lhs (so that expressions such as a = b = c are possible). The value category of the assignment operator is non ...
the copy assignment operator selected for every non-static class type (or array of class type) member of T is trivial. A trivial copy assignment operator makes a copy of the object representation as if by std::memmove. All data types compatible with the C language (POD types) are trivially copy-assignable.
In C++, the addition assignment operator (+=) combines the addition operation with the variable assignment allowing you to increment the value of variable by a specified expression in a concise and efficient way. Syntax. variable += value; This above expression is equivalent to the expression: variable = variable + value; Example.
Assignment operator (C++) In the C++ programming language, the assignment operator, =, is the operator used for assignment. Like most other operators in C++, it can be overloaded . The copy assignment operator, often just called the "assignment operator", is a special case of assignment operator where the source (right-hand side) and ...
21.12 — Overloading the assignment operator. Alex November 27, 2023. The copy assignment operator (operator=) is used to copy values from one object to another already existing object. As of C++11, C++ also supports "Move assignment". We discuss move assignment in lesson 22.3 -- Move constructors and move assignment .
Arrays Arrays and Loops Omit Array Size Get Array Size Multidimensional Arrays. C++ Structures C++ References. Create References Memory Address. C++ Pointers. ... In the example below, we use the assignment operator (=) to assign the value 10 to a variable called x: Example. int x = 10;
Use an assignment operator operator= that returns a reference to the class type and takes one parameter that's passed by const reference—for example ClassName& operator=(const ClassName& x);. Use the copy constructor. If you don't declare a copy constructor, the compiler generates a member-wise copy constructor for you.
What is an assignment operator? The assignment operator for a class is what allows you to use = to assign one instance to another. For example: 1 2: ... This class wraps an array of some user-specified type. It has two data members: a pointer to the array and a number of
The copy assignment operator selected for every non-static class type (or array of class type) memeber of T is trivial. A trivial copy assignment operator makes a copy of the object representation as if by std::memmove. All data types compatible with the C language (POD types) are trivially copy-assignable.
In those situations where copy assignment cannot benefit from resource reuse (it does not manage a heap-allocated array and does not have a (possibly transitive) member that does, such as a member std::vector or std::string), there is a popular convenient shorthand: the copy-and-swap assignment operator, which takes its parameter by value (thus working as both copy- and move-assignment ...
The assignment operator,"=", is the operator used for Assignment. It copies the right value into the left value. Assignment Operators are predefined to operate only on built-in Data types. Assignment operator overloading is binary operator overloading. Overloading assignment operator in C++ copies all values of one object to another object.
But, there are some basic differences between them: Copy constructor. Assignment operator. It is called when a new object is created from an existing object, as a copy of the existing object. This operator is called when an already initialized object is assigned a new value from another existing object. It creates a separate memory block for ...
Assignment operators are used in programming to assign values to variables. We use an assignment operator to store and update data within a program. They enable programmers to store data in variables and manipulate that data. The most common assignment operator is the equals sign (=), which assigns the value on the right side of the operator to ...
What you have above does not work on C arrays, they can't use the assignment operator. If they could, it would, presumably, work just like integers: int a; int b; a = b; //b still exists, of course, and still has its value! it would be unusual to make a custom operator for any type (arrays or not) that self-destructed the right hand side ...
std::array is a container that encapsulates fixed size arrays.. This container is an aggregate type with the same semantics as a struct holding a C-style array T [N] as its only non-static data member. Unlike a C-style array, it doesn't decay to T * automatically. As an aggregate type, it can be initialized with aggregate-initialization given at most N initializers that are convertible to T ...
The _Reinit_expected template function manages the transition of an std::expected object from one state to another. Because the code uses union, it has to manage the lifetime of alternatives. Other implementations In GCC libstdc++ - expected @ Github - it also uses union to hold the alternatives In LLVM libc++: expected @Github - in many places it uses no_unique_address attribute and Empty ...
@K-ballo: His implementation, perhaps by accident, actually covers the problem of self assignment. It checks if the arrays are the same size. If they're not the same size, they can't be the same object.
Assignment operation. The assignment (=) operator allows a ip_addr_t type variable to be assigned to another ip_addr_t type variable and also it allows constant IP address or hostname to be assigned to ip_addr_t type variable. The original values of the variable is overwritten. No type casting is allowed from or to the ip_addr_t variable types.
Anyway, there is a default assignment operator which does exactly that. You should only implement this operator if your class handles resources internally, like pointers, devices, etc. - mfontanini. Apr 29, 2012 at 16:54. ... Overloaded assignment operator with arrays. 0. Dynamic array implementaion c++ , Overloading assignment operator.
Run the below command to install Lodash: npm i lodash Using find and assign Functions. In this approach, we are using Lodash's find function to locate the object with an id equal to 102 in the courses array. Then, we use the assign method to update its duration property to ' 10 weeks ', demonstrating a targeted find-and-update operation in an array of objects using Lodash.
It is idiomatic to provide couple of overloads of the operator[] function - one for const objects and one for non-const objects. The return type of the const member function can be a const& or just a value depending on the object being returned while the return type of the non-const member function is usually a reference.. struct Heap{ int H[100]; int operator [] (int i) const {return H[i ...