Next: Conditional Branches , Up: Conditional Expression [ Contents ][ Index ]
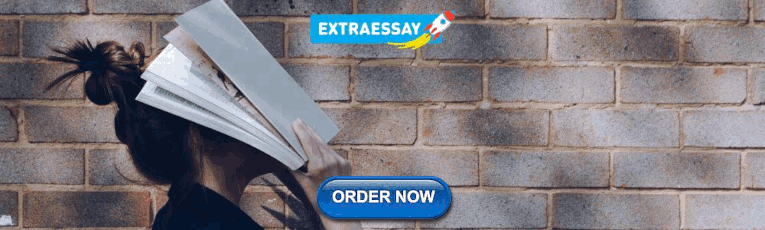
8.4.1 Rules for the Conditional Operator
The first operand, condition , should be a value that can be compared with zero—a number or a pointer. If it is true (nonzero), then the conditional expression computes iftrue and its value becomes the value of the conditional expression. Otherwise the conditional expression computes iffalse and its value becomes the value of the conditional expression. The conditional expression always computes just one of iftrue and iffalse , never both of them.
Here’s an example: the absolute value of a number x can be written as (x >= 0 ? x : -x) .
Warning: The conditional expression operators have rather low syntactic precedence. Except when the conditional expression is used as an argument in a function call, write parentheses around it. For clarity, always write parentheses around it if it extends across more than one line.
Assignment operators and the comma operator (see Comma Operator ) have lower precedence than conditional expression operators, so write parentheses around those when they appear inside a conditional expression. See Order of Execution .
Learn C practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn c interactively, c introduction.
- Keywords & Identifier
- Variables & Constants
- C Data Types
- C Input/Output
- C Operators
- C Introduction Examples
C Flow Control
- C if...else
- C while Loop
- C break and continue
- C switch...case
- C Programming goto
- Control Flow Examples
C Functions
- C Programming Functions
- C User-defined Functions
- C Function Types
- C Recursion
- C Storage Class
- C Function Examples
- C Programming Arrays
- C Multi-dimensional Arrays
- C Arrays & Function
- C Programming Pointers
- C Pointers & Arrays
- C Pointers And Functions
- C Memory Allocation
- Array & Pointer Examples
C Programming Strings
- C Programming String
- C String Functions
- C String Examples
Structure And Union
- C Structure
- C Struct & Pointers
- C Struct & Function
- C struct Examples
C Programming Files
- C Files Input/Output
- C Files Examples
Additional Topics
- C Enumeration
- C Preprocessors
- C Standard Library
- C Programming Examples
Bitwise Operators in C Programming
C Precedence And Associativity Of Operators
- Compute Quotient and Remainder
- Find the Size of int, float, double and char
C if...else Statement
- Demonstrate the Working of Keyword long
C Programming Operators
An operator is a symbol that operates on a value or a variable. For example: + is an operator to perform addition.
C has a wide range of operators to perform various operations.
C Arithmetic Operators
An arithmetic operator performs mathematical operations such as addition, subtraction, multiplication, division etc on numerical values (constants and variables).
Example 1: Arithmetic Operators
The operators + , - and * computes addition, subtraction, and multiplication respectively as you might have expected.
In normal calculation, 9/4 = 2.25 . However, the output is 2 in the program.
It is because both the variables a and b are integers. Hence, the output is also an integer. The compiler neglects the term after the decimal point and shows answer 2 instead of 2.25 .
The modulo operator % computes the remainder. When a=9 is divided by b=4 , the remainder is 1 . The % operator can only be used with integers.
Suppose a = 5.0 , b = 2.0 , c = 5 and d = 2 . Then in C programming,
C Increment and Decrement Operators
C programming has two operators increment ++ and decrement -- to change the value of an operand (constant or variable) by 1.
Increment ++ increases the value by 1 whereas decrement -- decreases the value by 1. These two operators are unary operators, meaning they only operate on a single operand.
Example 2: Increment and Decrement Operators
Here, the operators ++ and -- are used as prefixes. These two operators can also be used as postfixes like a++ and a-- . Visit this page to learn more about how increment and decrement operators work when used as postfix .
C Assignment Operators
An assignment operator is used for assigning a value to a variable. The most common assignment operator is =
Example 3: Assignment Operators
C relational operators.
A relational operator checks the relationship between two operands. If the relation is true, it returns 1; if the relation is false, it returns value 0.
Relational operators are used in decision making and loops .
Example 4: Relational Operators
C logical operators.
An expression containing logical operator returns either 0 or 1 depending upon whether expression results true or false. Logical operators are commonly used in decision making in C programming .
Example 5: Logical Operators
Explanation of logical operator program
- (a == b) && (c > 5) evaluates to 1 because both operands (a == b) and (c > b) is 1 (true).
- (a == b) && (c < b) evaluates to 0 because operand (c < b) is 0 (false).
- (a == b) || (c < b) evaluates to 1 because (a = b) is 1 (true).
- (a != b) || (c < b) evaluates to 0 because both operand (a != b) and (c < b) are 0 (false).
- !(a != b) evaluates to 1 because operand (a != b) is 0 (false). Hence, !(a != b) is 1 (true).
- !(a == b) evaluates to 0 because (a == b) is 1 (true). Hence, !(a == b) is 0 (false).
C Bitwise Operators
During computation, mathematical operations like: addition, subtraction, multiplication, division, etc are converted to bit-level which makes processing faster and saves power.
Bitwise operators are used in C programming to perform bit-level operations.
Visit bitwise operator in C to learn more.
Other Operators
Comma operator.
Comma operators are used to link related expressions together. For example:
The sizeof operator
The sizeof is a unary operator that returns the size of data (constants, variables, array, structure, etc).
Example 6: sizeof Operator
Other operators such as ternary operator ?: , reference operator & , dereference operator * and member selection operator -> will be discussed in later tutorials.
Table of Contents
- Arithmetic Operators
- Increment and Decrement Operators
- Assignment Operators
- Relational Operators
- Logical Operators
- sizeof Operator
Video: Arithmetic Operators in C
Sorry about that.
Related Tutorials
PrepBytes Blog
ONE-STOP RESOURCE FOR EVERYTHING RELATED TO CODING
Sign in to your account
Forgot your password?
Login via OTP
We will send you an one time password on your mobile number
An OTP has been sent to your mobile number please verify it below
Register with PrepBytes
Conditional operator in c.
Last Updated on May 16, 2023 by Prepbytes
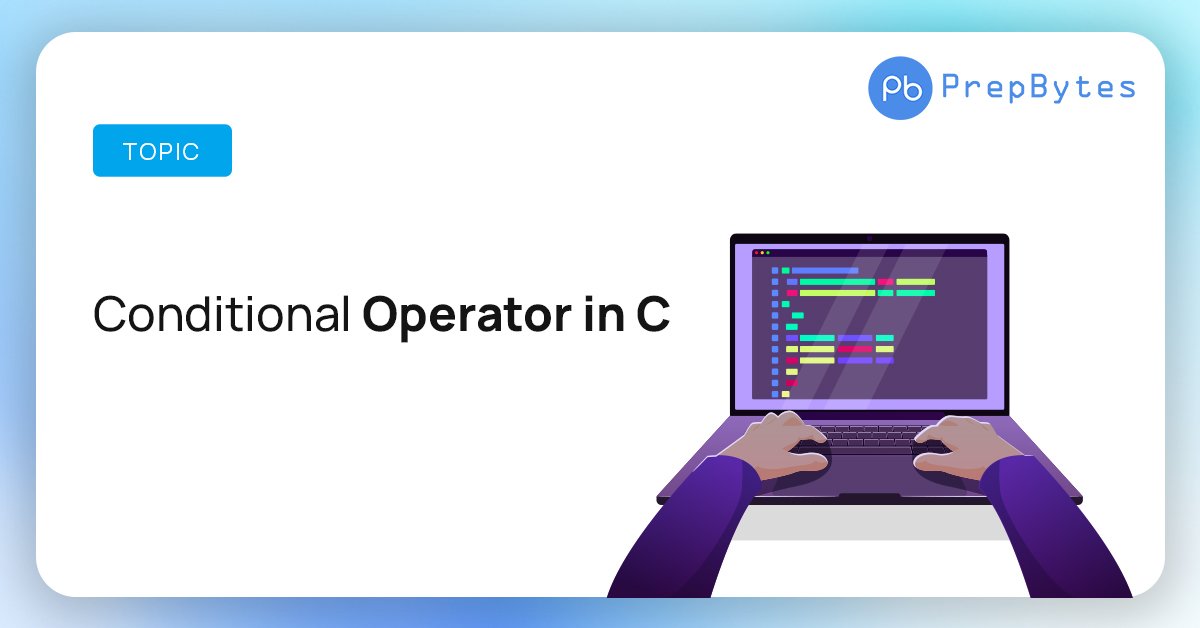
The conditional operator in C programming language, as well as its syntax and operation, will be covered in this article. We’ll also see when the if-else condition should be used instead of the conditional operator. The only ternary operator in the C programming language is the conditional operator. If the "if else" has only one statement each, it can be used as an alternative to an if-else condition. The conditional operator takes an expression and, depending on whether it evaluates to true or false, either the first or second statement is executed.
What is a Conditional Operator In C?
You probably encountered this case once or more when you had to construct an if-else code in order to carry out a single statement. Yes, that is a bit of a hassle. For this issue, the conditional operator was created specifically. When compared to an if-else statement, which would require more than one line, the conditional operator allows you to express decision-making statements in just one line.
The conditional operator is a ternary operator since it requires three operands. Since the only ternary operator is the conditional operator in C programming language, the terms ternary operator and conditional operator are interchangeable when referring to this operator. Expressions, statements, constants, and variables can all be used as operands. They are appropriately called conditional operators because they always begin with a condition as the first operand.
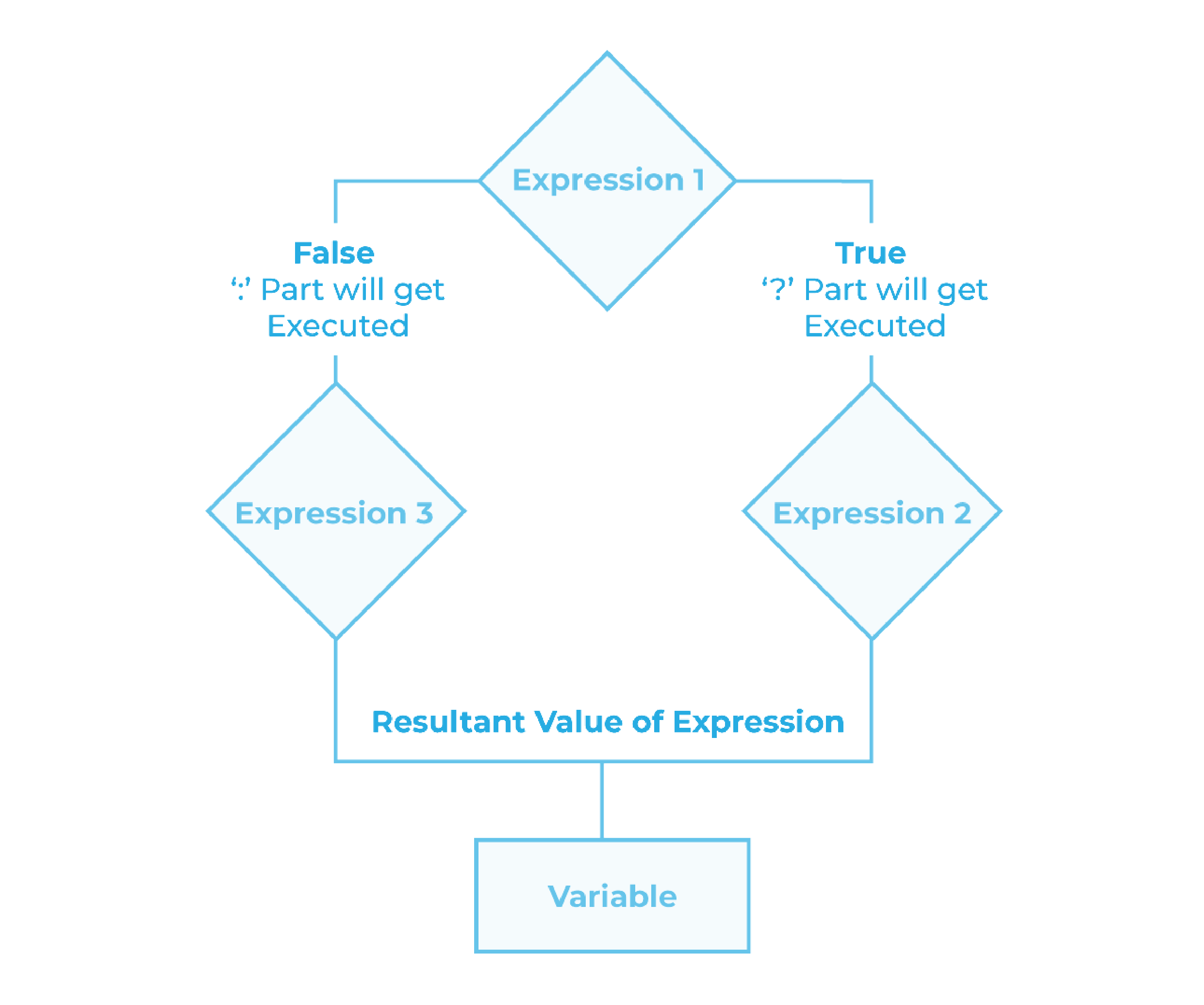
Expression Syntax of Conditional Operator in C
Any value other than 0 will be regarded as true, and 0 will be seen as false, as the expression is being evaluated as a logical condition. Statements 1 and 2 may take the form of statements, expressions, variables, or constants. Depending on the outcome of the evaluation of the provided expression, one of the statements will be put into action.
Working for the Expression of Conditional Operator in C
The conditional operator of the C programming language works as follows:
- The result of the condition evaluation is implicitly changed to a bool once the condition is first evaluated.
- The first statement—the one following the question mark—will be executed if the condition evaluates to true.
- The second statement—the one that comes after the colon—is performed if the condition evaluates to be false.
- One of the two phrases included in the conditional operator’s result is the outcome of the conditional operator.
- The last two operands or expressions are simply ignored, with only one of them being evaluated. The diagram below will make it much easier for you to recall how the conditional operator in C operates.
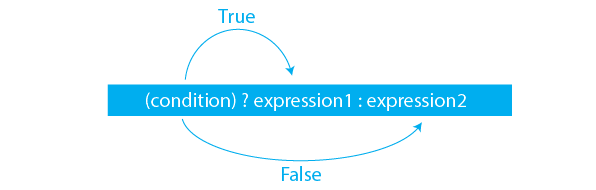
Another Version of the Conditional Operator in C
For situations where the if statement would only contain one statement, the conditional operator is a condensed form of the if-else statement. Additionally, the conditional operator has a condensed form of itself. One of the most frequently used use cases in programming is the process of checking a condition and putting a value on a variable. This version of the conditional operator was created to assist with this process.
Syntax of the conditional operator in C
Working of the Conditional Operator in C
This conditional operator’s functionality is more similar to that of the original conditional operator. If the condition is found to be true, the compiler will first evaluate it and then assign value1 to the variable; if the condition is found to be false, value2 will be allocated to the variable.
The diagram below will make it much easier for you to recall how the conditional operator in C operates.
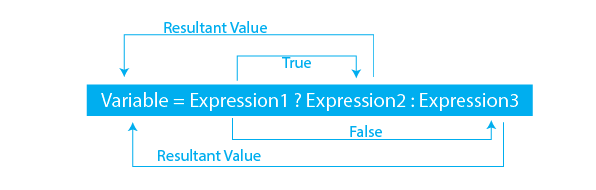
Some important remarks about the Conditional Operator
The conditional operator’s first operand should be an integral or a pointer type. The C compiler will implicitly convert the second and third operands to the same data type if they are not of the same type.
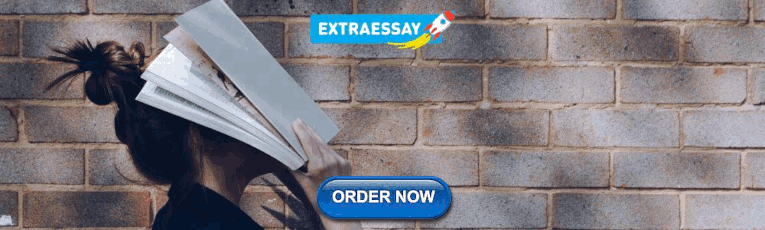
Associativity of the Conditional Operator in C
The operator’s operands are performed in the order specified by the Associativity property. When an expression contains several operators, and multiple operators share the same precedence, the execution order of the operators is determined by associativity. The conditional operator is associative from right to left.
Difference between Conditional Operator in C and If-else Statement in C
The if-else statement and conditional operator in C are more comparable to one another. They virtually always carry out the same action: they check a condition and then make a statement based on the outcome of the condition’s evaluation. The conditional operator was created to make it easier for users to write code. If an if-else expression only has one statement to execute, the conditional operator can be used in that situation. The conditional operator helps programs write fewer lines of code.
The table below contains a list of some key distinctions between the conditional operator in C and the if-else expression in C
Examples of the Conditional operator in C
Let’s get started with some coding to illustrate some scenarios where the conditional operator may be quite useful because reading through all these theories can be tedious.
Print Whether the Given Number is Odd or Even
Working The first three lines of code are ones you are already used to. They read an integer input and initialize the variable num. The second half of the sentence is executed because the condition 13% 2 == 1 evaluates to false in the first input, 13, which the conditional operator checks for first. The result then displays the string "The given number is odd."
Conclusion We learned about the conditional operator in C, as well as its syntax, visual representation, and semantics, in this article. You can use assignment statements in a different way by using the conditional operator, which we also looked at. We looked at an example of how the conditional operator can be used to determine whether a given integer is odd or even.
Frequently Asked Questions (FAQs)
Q1. Is ++ a ternary operator? Ans. No, ++ is not a ternary operator
Q2. Which is a logical operator? Ans. A logical operator is a symbol or word used to connect two or more expressions such that the value of the compound expression produced depends only on that of the original expressions and on the meaning of the operator. Common logical operators include AND, OR, and NOT.
Q3. What is ternary vs binary operator? Ans. The three categories of operators based on the number of operands they require are: Unary operators: which require one operand (Un) Binary operators: which require two operands (Bi) Ternary operators: which require three operands (Ter)
Q4. Why is it called a ternary operator? Ans. The name ternary refers to the fact that the operator takes three operands. The condition is a boolean expression that evaluates to either true or false
Q5. What are the 5 logical operators? Ans. Five logical operators are:
- Logical Negation.
- Logical Conjunction (AND)
- Logical Disjunction (Inclusive OR)
- Logical Implication (Conditional)
- Logical Biconditional (Double Implication)
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
- Linked List
- Segment Tree
- Backtracking
- Dynamic Programming
- Greedy Algorithm
- Operating System
- Company Placement
- Interview Tips
- General Interview Questions
- Data Structure
- Other Topics
- Computational Geometry
- Game Theory
Related Post
Null character in c, assignment operator in c, ackermann function in c, median of two sorted arrays of different size in c, number is palindrome or not in c, implementation of queue using linked list in c.
This browser is no longer supported.
Upgrade to Microsoft Edge to take advantage of the latest features, security updates, and technical support.
?? and ??= operators - the null-coalescing operators
- 9 contributors
The null-coalescing operator ?? returns the value of its left-hand operand if it isn't null ; otherwise, it evaluates the right-hand operand and returns its result. The ?? operator doesn't evaluate its right-hand operand if the left-hand operand evaluates to non-null. The null-coalescing assignment operator ??= assigns the value of its right-hand operand to its left-hand operand only if the left-hand operand evaluates to null . The ??= operator doesn't evaluate its right-hand operand if the left-hand operand evaluates to non-null.
The left-hand operand of the ??= operator must be a variable, a property , or an indexer element.
The type of the left-hand operand of the ?? and ??= operators can't be a non-nullable value type. In particular, you can use the null-coalescing operators with unconstrained type parameters:
The null-coalescing operators are right-associative. That is, expressions of the form
are evaluated as
The ?? and ??= operators can be useful in the following scenarios:
In expressions with the null-conditional operators ?. and ?[] , you can use the ?? operator to provide an alternative expression to evaluate in case the result of the expression with null-conditional operations is null :
When you work with nullable value types and need to provide a value of an underlying value type, use the ?? operator to specify the value to provide in case a nullable type value is null :
Use the Nullable<T>.GetValueOrDefault() method if the value to be used when a nullable type value is null should be the default value of the underlying value type.
You can use a throw expression as the right-hand operand of the ?? operator to make the argument-checking code more concise:
The preceding example also demonstrates how to use expression-bodied members to define a property.
You can use the ??= operator to replace the code of the form
with the following code:
Operator overloadability
The operators ?? and ??= can't be overloaded.
C# language specification
For more information about the ?? operator, see The null coalescing operator section of the C# language specification .
For more information about the ??= operator, see the feature proposal note .
- Null check can be simplified (IDE0029, IDE0030, and IDE0270)
- C# reference
- C# operators and expressions
- ?. and ?[] operators
- ?: operator
Coming soon: Throughout 2024 we will be phasing out GitHub Issues as the feedback mechanism for content and replacing it with a new feedback system. For more information see: https://aka.ms/ContentUserFeedback .
Submit and view feedback for
Additional resources
- Graphics and multimedia
- Language Features
- Unix/Linux programming
- Source Code
- Standard Library
- Tips and Tricks
- Tools and Libraries
- Windows API
- The Conditional (or Ternary) Operator (?
The Conditional (or Ternary) Operator (?:)

cppreference.com
C operator precedence.
The following table lists the precedence and associativity of C operators. Operators are listed top to bottom, in descending precedence.
- ↑ The operand of prefix ++ and -- can't be a type cast. This rule grammatically forbids some expressions that would be semantically invalid anyway. Some compilers ignore this rule and detect the invalidity semantically.
- ↑ The operand of sizeof can't be a type cast: the expression sizeof ( int ) * p is unambiguously interpreted as ( sizeof ( int ) ) * p , but not sizeof ( ( int ) * p ) .
- ↑ The expression in the middle of the conditional operator (between ? and : ) is parsed as if parenthesized: its precedence relative to ?: is ignored.
- ↑ Assignment operators' left operands must be unary (level-2 non-cast) expressions. This rule grammatically forbids some expressions that would be semantically invalid anyway. Many compilers ignore this rule and detect the invalidity semantically. For example, e = a < d ? a ++ : a = d is an expression that cannot be parsed because of this rule. However, many compilers ignore this rule and parse it as e = ( ( ( a < d ) ? ( a ++ ) : a ) = d ) , and then give an error because it is semantically invalid.
When parsing an expression, an operator which is listed on some row will be bound tighter (as if by parentheses) to its arguments than any operator that is listed on a row further below it. For example, the expression * p ++ is parsed as * ( p ++ ) , and not as ( * p ) ++ .
Operators that are in the same cell (there may be several rows of operators listed in a cell) are evaluated with the same precedence, in the given direction. For example, the expression a = b = c is parsed as a = ( b = c ) , and not as ( a = b ) = c because of right-to-left associativity.
[ edit ] Notes
Precedence and associativity are independent from order of evaluation .
The standard itself doesn't specify precedence levels. They are derived from the grammar.
In C++, the conditional operator has the same precedence as assignment operators, and prefix ++ and -- and assignment operators don't have the restrictions about their operands.
Associativity specification is redundant for unary operators and is only shown for completeness: unary prefix operators always associate right-to-left ( sizeof ++* p is sizeof ( ++ ( * p ) ) ) and unary postfix operators always associate left-to-right ( a [ 1 ] [ 2 ] ++ is ( ( a [ 1 ] ) [ 2 ] ) ++ ). Note that the associativity is meaningful for member access operators, even though they are grouped with unary postfix operators: a. b ++ is parsed ( a. b ) ++ and not a. ( b ++ ) .
[ edit ] References
- C17 standard (ISO/IEC 9899:2018):
- A.2.1 Expressions
- C11 standard (ISO/IEC 9899:2011):
- C99 standard (ISO/IEC 9899:1999):
- C89/C90 standard (ISO/IEC 9899:1990):
- A.1.2.1 Expressions
[ edit ] See also
Order of evaluation of operator arguments at run time.
- Recent changes
- Offline version
- What links here
- Related changes
- Upload file
- Special pages
- Printable version
- Permanent link
- Page information
- In other languages
- This page was last modified on 31 July 2023, at 09:28.
- This page has been accessed 2,665,989 times.
- Privacy policy
- About cppreference.com
- Disclaimers

C Data Types
C operators.
- C Input and Output
- C Control Flow
- C Functions
- C Preprocessors
C File Handling
- C Cheatsheet
C Interview Questions
- Solve Coding Problems
- C Programming Language Tutorial
- C Language Introduction
- Features of C Programming Language
- C Programming Language Standard
- C Hello World Program
- Compiling a C Program: Behind the Scenes
- Tokens in C
- Keywords in C
C Variables and Constants
- C Variables
- Constants in C
- Const Qualifier in C
- Different ways to declare variable as constant in C
- Scope rules in C
- Internal Linkage and External Linkage in C
- Global Variables in C
- Data Types in C
- Literals in C
- Escape Sequence in C
- Integer Promotions in C
- Character Arithmetic in C
- Type Conversion in C
C Input/Output
- Basic Input and Output in C
- Format Specifiers in C
- printf in C
- Scansets in C
- Formatted and Unformatted Input/Output functions in C with Examples
- Operators in C
- Arithmetic Operators in C
- Unary operators in C
- Relational Operators in C
- Bitwise Operators in C
- C Logical Operators
Assignment Operators in C
- Increment and Decrement Operators in C
- Conditional or Ternary Operator (?:) in C
- sizeof operator in C
- Operator Precedence and Associativity in C
C Control Statements Decision-Making
- Decision Making in C (if , if..else, Nested if, if-else-if )
- C - if Statement
- C if...else Statement
- C if else if ladder
- Switch Statement in C
- Using Range in switch Case in C
- while loop in C
- do...while Loop in C
- For Versus While
- Continue Statement in C
- Break Statement in C
- goto Statement in C
- User-Defined Function in C
- Parameter Passing Techniques in C
- Function Prototype in C
- How can I return multiple values from a function?
- main Function in C
- Implicit return type int in C
- Callbacks in C
- Nested functions in C
- Variadic functions in C
- _Noreturn function specifier in C
- Predefined Identifier __func__ in C
- C Library math.h Functions
C Arrays & Strings
- Properties of Array in C
- Multidimensional Arrays in C
- Initialization of Multidimensional Array in C
- Pass Array to Functions in C
- How to pass a 2D array as a parameter in C?
- What are the data types for which it is not possible to create an array?
- How to pass an array by value in C ?
- Strings in C
- Array of Strings in C
- What is the difference between single quoted and double quoted declaration of char array?
- C String Functions
- Pointer Arithmetics in C with Examples
- C - Pointer to Pointer (Double Pointer)
- Function Pointer in C
- How to declare a pointer to a function?
- Pointer to an Array | Array Pointer
- Difference between constant pointer, pointers to constant, and constant pointers to constants
- Pointer vs Array in C
- Dangling, Void , Null and Wild Pointers in C
- Near, Far and Huge Pointers in C
- restrict keyword in C
C User-Defined Data Types
- C Structures
- dot (.) Operator in C
- Structure Member Alignment, Padding and Data Packing
- Flexible Array Members in a structure in C
- Bit Fields in C
- Difference Between Structure and Union in C
- Anonymous Union and Structure in C
- Enumeration (or enum) in C
C Storage Classes
- Storage Classes in C
- extern Keyword in C
- Static Variables in C
- Initialization of static variables in C
- Static functions in C
- Understanding "volatile" qualifier in C | Set 2 (Examples)
- Understanding "register" keyword in C
C Memory Management
- Memory Layout of C Programs
- Dynamic Memory Allocation in C using malloc(), calloc(), free() and realloc()
- Difference Between malloc() and calloc() with Examples
- What is Memory Leak? How can we avoid?
- Dynamic Array in C
- How to dynamically allocate a 2D array in C?
- Dynamically Growing Array in C
C Preprocessor
- C Preprocessor Directives
- How a Preprocessor works in C?
- Header Files in C
- What’s difference between header files "stdio.h" and "stdlib.h" ?
- How to write your own header file in C?
- Macros and its types in C
- Interesting Facts about Macros and Preprocessors in C
- # and ## Operators in C
- How to print a variable name in C?
- Multiline macros in C
- Variable length arguments for Macros
- Branch prediction macros in GCC
- typedef versus #define in C
- Difference between #define and const in C?
- Basics of File Handling in C
- C fopen() function with Examples
- EOF, getc() and feof() in C
- fgets() and gets() in C language
- fseek() vs rewind() in C
- What is return type of getchar(), fgetc() and getc() ?
- Read/Write Structure From/to a File in C
- C Program to print contents of file
- C program to delete a file
- C Program to merge contents of two files into a third file
- What is the difference between printf, sprintf and fprintf?
- Difference between getc(), getchar(), getch() and getche()
Miscellaneous
- time.h header file in C with Examples
- Input-output system calls in C | Create, Open, Close, Read, Write
- Signals in C language
- Program error signals
- Socket Programming in C
- _Generics Keyword in C
- Multithreading in C
- C Programming Interview Questions (2024)
- Commonly Asked C Programming Interview Questions | Set 1
- Commonly Asked C Programming Interview Questions | Set 2
- Commonly Asked C Programming Interview Questions | Set 3
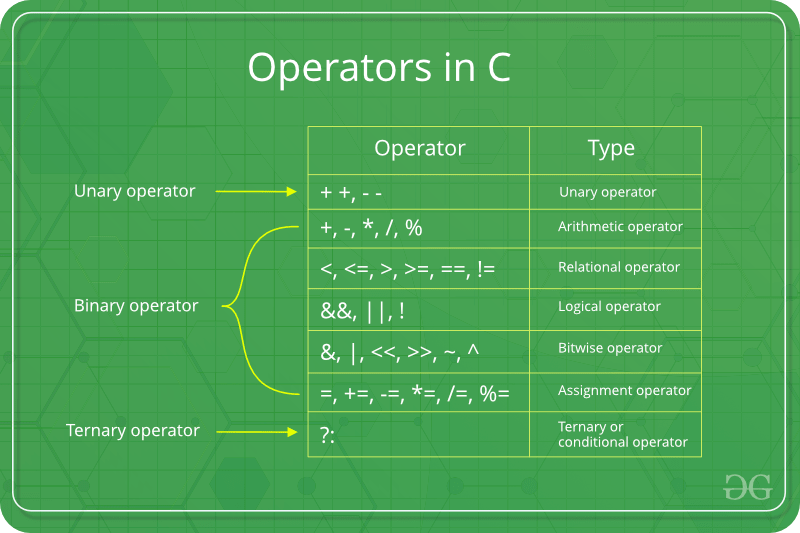
Assignment operators are used for assigning value to a variable. The left side operand of the assignment operator is a variable and right side operand of the assignment operator is a value. The value on the right side must be of the same data-type of the variable on the left side otherwise the compiler will raise an error.
Different types of assignment operators are shown below:
1. “=”: This is the simplest assignment operator. This operator is used to assign the value on the right to the variable on the left. Example:
2. “+=” : This operator is combination of ‘+’ and ‘=’ operators. This operator first adds the current value of the variable on left to the value on the right and then assigns the result to the variable on the left. Example:
If initially value stored in a is 5. Then (a += 6) = 11.
3. “-=” This operator is combination of ‘-‘ and ‘=’ operators. This operator first subtracts the value on the right from the current value of the variable on left and then assigns the result to the variable on the left. Example:
If initially value stored in a is 8. Then (a -= 6) = 2.
4. “*=” This operator is combination of ‘*’ and ‘=’ operators. This operator first multiplies the current value of the variable on left to the value on the right and then assigns the result to the variable on the left. Example:
If initially value stored in a is 5. Then (a *= 6) = 30.
5. “/=” This operator is combination of ‘/’ and ‘=’ operators. This operator first divides the current value of the variable on left by the value on the right and then assigns the result to the variable on the left. Example:
If initially value stored in a is 6. Then (a /= 2) = 3.
Below example illustrates the various Assignment Operators:
Please Login to comment...
- C-Operators
- cpp-operator
- WhatsApp To Launch New App Lock Feature
- Top Design Resources for Icons
- Node.js 21 is here: What’s new
- Zoom: World’s Most Innovative Companies of 2024
- 30 OOPs Interview Questions and Answers (2024)
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
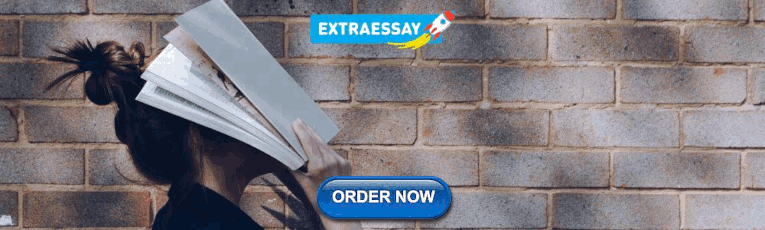
IMAGES
COMMENTS
The conditional operator in C is kind of similar to the if-else statement as it follows the same algorithm as of if-else statement but the conditional operator takes less space and helps to write the if-else statements in the shortest way possible. It is also known as the ternary operator in C as it operates on three operands.. Syntax of Conditional/Ternary Operator in C
8. The fact that the ternary operator is an expression, not a statement, allows it to be used in macro expansions for function-like macros that are used as part of an expression. Const may not have been part of original C, but the macro pre-processor goes way back. One place where I've seen it used is in an array package that used macros for ...
The conditional operator in C is a conditional statement that returns the first value if the condition is true and returns another value if the condition is false. It is similar to the if-else statement. The if-else statement takes more than one line of the statements, but the conditional operator finishes the same task in a single statement.
Assign the ternary operator to a variable. In C programming, we can also assign the expression of the ternary operator to a variable. For example, Here, if the test condition is true, expression1 will be assigned to the variable. Otherwise, expression2 will be assigned. // create variables char operator = '+';
8.4.1 Rules for the Conditional Operator. The first operand, condition, should be a value that can be compared with zero—a number or a pointer. If it is true (nonzero), then the conditional expression computes iftrue and its value becomes the value of the conditional expression. Otherwise the conditional expression computes iffalse and its ...
Like the conditional operator, a conditional ref expression evaluates only one of the two expressions: either consequent or alternative. In a conditional ref expression, the type of consequent and alternative must be the same. Conditional ref expressions aren't target-typed. Conditional operator and an if statement
Syntax. The assignment operators in C can both transform and assign values in a single operation. C provides the following assignment operators: In assignment, the type of the right-hand value is converted to the type of the left-hand value, and the value is stored in the left operand after the assignment has taken place. The left operand must ...
In computer programming, the ternary conditional operator is a ternary operator that is part of the syntax for basic conditional expressions in several programming languages. ... In C++ there are conditional assignment situations where use of the if-else statement is impossible, ...
Assignment performs implicit conversion from the value of rhs to the type of lhs and then replaces the value in the object designated by lhs with the converted value of rhs . Assignment also returns the same value as what was stored in lhs (so that expressions such as a = b = c are possible). The value category of the assignment operator is non ...
An operator is a symbol that operates on a value or a variable. For example: + is an operator to perform addition. In this tutorial, you will learn about different C operators such as arithmetic, increment, assignment, relational, logical, etc. with the help of examples.
Conditional operator in C if-else statement in C; The conditional operator is a single programming statement and can only perform one operation. The if-else statement is a block statement, you can group multiple statements using a parenthesis. The conditional operator can return a value and so can be used for performing assignment operations.
In expressions with the null-conditional operators ?. and ?[], you can use the ?? operator to provide an alternative expression to evaluate in case the result of the expression with null-conditional operations is null:
Conditional operator and assignment operator in C. Ask Question Asked 13 years, 5 months ago. Modified 13 years, 5 months ago. Viewed 789 times 4 On http ... C conditional operator in GCC. 1. How the assignment statement in an if statement serves as a condition? 0.
The precedence of the conditional operator is quite lower than the arithmetic, logical and relational operators. But it is higher than the assignment and compound assignment operator. The associativity of conditional operator is from right to left (see Operator Precedence in C). Consider the following conditional expression:
In this ongoing C programming tutorial series, we have already discussed some of the basic stuff like arithmetic, logical, and relational operators as well as conditional loops like 'if' and 'while'.Adding upon that, this tutorial will focus on assignment operators (other than =) and conditional expressions.
The conditional operator is an operator used in C and C++ (as well as other languages, such as C#). The ?: operator returns one of two values depending on the result of an expression. If expression 1 evaluates to true, then expression 2 is evaluated.
Conditional Operator in Java: Syntax of Ternary Operator in Java: The syntax of the ternary operator in Java is the same as in C and C++: condition ? expression_if_true : expression_if_false; Comparison with Other Languages: Java's ternary operator syntax is similar to C and C++. However, there are a few differences, such as the requirement for compatible types in the expressions on both ...
The operator precedence in the C/C++ language in not defined by a table or numbers, but by a grammar. Here is the grammar for conditional operator from C++0x draft chapter 5.16 Conditional operator [expr.cond]: conditional-expression: logical-or-expression logical-or-expression ? expression : assignment-expression
They are derived from the grammar. In C++, the conditional operator has the same precedence as assignment operators, and prefix ++ and -- and assignment operators don't have the restrictions about their operands. Associativity specification is redundant for unary operators and is only shown for completeness: unary prefix operators always ...
The reason is: Performance improvement (sometimes) Less code (always) Take an example: There is a method someMethod () and in an if condition you want to check whether the return value of the method is null. If not, you are going to use the return value again. If(null != someMethod()){.
This operator first adds the current value of the variable on left to the value on the right and then assigns the result to the variable on the left. Example: (a += b) can be written as (a = a + b) If initially value stored in a is 5. Then (a += 6) = 11. 3. "-=" This operator is combination of '-' and '=' operators.
One of the most elegant applications of these two principles is to declare a variable in a conditional." -- Stroustrup, "The C++ Programming Language." - Andy Thomas. ... assignment operator and conditional statements. 0. setting a variable in an if statement. 1. Make assignment within if-statement. 1.