- Java for Android
- Android Studio
- Android Kotlin
- Android Project
- Android Interview
- How to Build a Sudoku Solver Android App?
- How to Build a Simple Torch App in Android using Kotlin?
- Alternatives for the Deprecated AsyncTask in Android
- How to Fetch Currently Running Tasks in Android Programmatically?
- How to Change the ListView Text Color in Android?
- Overflow Items in Android
- How to Take Sub-View Screenshot in Android?
- Android Floating Action Button in Kotlin
- How to Remove ListView Item Divider in Android?
- Android - Deep Linking with Kotlin
- Android - Implement Preference Setting Screen with Kotlin
- How to Send Data Back to MainActivity in Android using Kotlin?
- Exit From App on Double Click of Back Button in Android using Kotlin
- Android - RecyclerView using GridLayoutManager with Kotlin
- Serialize and Deserialize JSON using GSON Library in Android
- Android - Point Graph Series with Kotlin
- How to Draw Different Types of Circles in Android?
- Android - Create a Pie Chart with Kotlin
- How to Create Splash Screen Without an Extra Activity in Android?
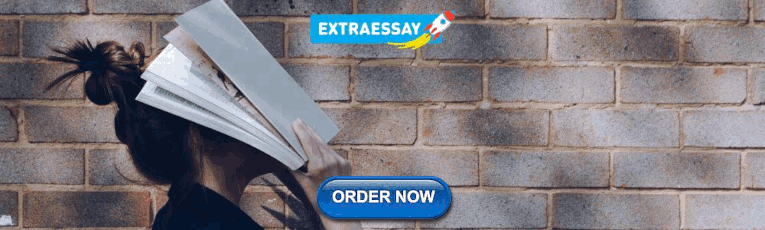
Speech to Text Application in Android with Kotlin
Speech to Text is seen in many applications such as Google search. With the help of this feature, the user can simply speak the query he wants to search. The text format of that speech will be automatically generated in the search bar. In this article, we will be taking a look at How to implement Speech Text in Android applications using Kotlin.
Note : If you want to implement speech to text in android application using java, Check out the following article: Speech to Text in Android using Java
Step by Step Implementation
Step 1: Create a New Project in Android Studio
To create a new project in Android Studio please refer to How to Create/Start a New Project in Android Studio . Note that select Kotlin as the programming language.
Step 2: Add mic image in drawable folder
Navigate to the app > res > drawable > Right-click on it. Then click on New > Vector Asset. After that click on the clip art icon. Inside that simply search for the mic icon and we will get to see the icon. After that, we will have to rename the icon as ic_mic. After adding this simply click on Finish to add this icon to the drawable folder.
Step 3: Working with the activity_main.xml file
Navigate to the app > res > layout > activity_main.xml and add the below code to that file. Below is the code for the activity_main.xml file. Comments are added inside the code to understand the code in more detail.
Step 4: Working with the MainActivity.kt file
Go to the MainActivity.kt file and refer to the following code. Below is the code for the MainActivity.kt file. Comments are added inside the code to understand the code in more detail.
Now run your application to see the output of it.
Output:
Please Login to comment...
- Android-projects
- 10 Best Tools to Convert DOC to DOCX
- How To Summarize PDF Documents Using Google Bard for Free
- Best free Android apps for Meditation and Mindfulness
- TikTok Is Paying Creators To Up Its Search Game
- 30 OOPs Interview Questions and Answers (2024)
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
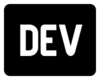
DEV Community

Posted on May 30, 2020
Speech-to-text and Text-to-speech with Android
Have you ever wondered how does Google's speech search work, or ever thought of building an ebook narration app? At the first glance it might seem some complex piece of technology. While it is complicated to implement it on your own, thankfully Android (via Google Services) has built in speech-to-text and text-to-speech APIs which make it extremely easy to setup these features.
See it in action
How does this work?
For Speech-to-text, Android provides an Intent based API which launches Google's Speech Recognition service and returns back the text result to you. There is a catch though - the device will require Google Search app for the service to work.
The Text-to-speech API, unlike Speech Recognition, is available without Google Services, and can be found in android.speech.tts package.
Source code
You can find the source of this tutorial on GitHub .
Let's develop!
Fire up Android Studio and create a project with a Blank Activity.
User interface
The user interface is going to be simple - a LinearLayout as the root view group, inside wich there will be a Button which launches the Speech Recognition API, an EditText that shows the Speech Recognition output as well as serves as input to Text-to-speech functionality, and another Button to trigger Text-to-speech output.
The resultant XML file is as follows:

Setting up speech recognition
The Speech Recognition API comes bundled with the Google Search app, and can be launched using an Intent. The result of this Intent holds the recognized text, which can be extracted from the result intent in onActivityResult .
All the code beyond here is in Kotlin.
Firstly, let's define our request code constant.
Then, we'll attach an onClickListener to our button, in which we will construct and launch the Speech Recognition Intent .
The above code will launch the Speech Recognition API. But how do we get the result? We'll override the activity's onActivityResult and get the recognized text.
At this point, if your run your code, you will be able to use the Speech Recognition.
Setting up Text-to-speech
Unlike Speech Recognition API, Text-to-speech has it own class and doesn't run on Intents. We'll start off by creating a TextToSpeech object. The TextToSpeech class constructor expects a Context and an OnInitListener .
Then, we'll set an OnClickListener to our TTS button and call the text-to-speech API on our input text.
As a safety measure and to prevent memory leaks, we must override onPause and onDestroy methods and appropriately stop or shutdown the TextToSpeech object.
And that's it. Give it a try!
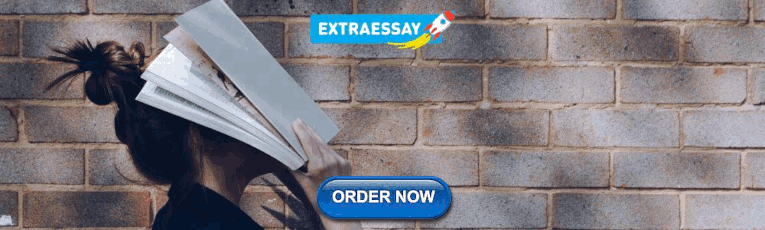
Closing Thoughts
With the standard APIs, Speech Recognition (or Speech-to-text) and Text-to-speech in Android is extremely easy to implement. While this might suffice most use cases, some advanced use cases would require more sophisticated third-party APIs or a custom implementation in your backend. We'll probably cover that sometime later.
Until then, keep coding, and as always do let me know if you have any questions in the comments section!
Top comments (5)
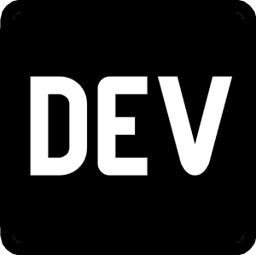
Templates let you quickly answer FAQs or store snippets for re-use.

- Joined Jan 16, 2021
Thx for article, but I still have some questions: How make speech recognition, which would be return recognized words in Set? How to make permanent voice recognition until the user turns it off? And how to remove google activity when it recognize speech, I want to show all words in text inside my app

- Email [email protected]
- Location Pune, India
- Education Bachelor of Computer Engineering
- Work Software Engineer
- Joined May 5, 2020
Hey @samartinell
By default, speech recognition returns a string, you could do a String.split() call on on with a regex that identifies words as per your preference. This will give you a list of words.
For permanent voice recognition, you will have to play with Recognition. The trick is to listen to when the speech recognition ends and then restart it. If you don't want the Google Dialog while recognizing speech, and also keep an always on speech recognition feature, check out this answer on StackOverflow, might help: stackoverflow.com/a/45833487
Do let the community here know if it worked for you!
Thank you very much! I'm already found how recognize speech without google dialog and how split string to set. Now i'm gonna try your way to permanent recognition

- Work Android developer
- Joined Jan 20, 2022
Thank you for the details, is it possible to use it while recording videos with audio ? With Android cameraX?

- Joined Sep 1, 2021
Can we add custom words to help better recognise words
Are you sure you want to hide this comment? It will become hidden in your post, but will still be visible via the comment's permalink .
Hide child comments as well
For further actions, you may consider blocking this person and/or reporting abuse
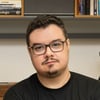
Kotlin Design Patterns: Simplifying the Proxy Pattern
Lucas Fugisawa - Feb 20
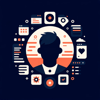
Gradle Essentials für React Native Entwickler
Pascal C - Jan 14
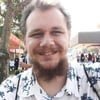
Running Capacitor in a VS Code DevContainer
Michael Rademeyer - Jan 17
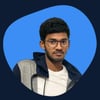
Power your Flutter App with Gemini AI ✨
Dhanush Vardhan - Feb 16
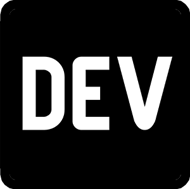
We're a place where coders share, stay up-to-date and grow their careers.
Search code, repositories, users, issues, pull requests...
Provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
speech-to-text-android
Here are 5 public repositories matching this topic..., elishaaz / sayboard.
An open-source on-device voice IME (keyboard) for Android using the Vosk library.
- Updated Mar 20, 2024
akash251 / Speech_To_Text_In_Compose
Speech to text using jetpack compose
- Updated Jul 6, 2022
tiro-is / heyra
- Updated Sep 26, 2022
orbitalsonic / SpeechToText-Converter-using-Google-Android
Speech to text converter using Google Android
- Updated Dec 5, 2023
orbitalsonic / Speech-Recognition-SpeechToTextConverter
The Speech Recognition or Speech-to-Text Converter module in Android, implemented using Kotlin, facilitates the conversion of spoken language into written text
- Updated Jul 18, 2023
Improve this page
Add a description, image, and links to the speech-to-text-android topic page so that developers can more easily learn about it.
Curate this topic
Add this topic to your repo
To associate your repository with the speech-to-text-android topic, visit your repo's landing page and select "manage topics."
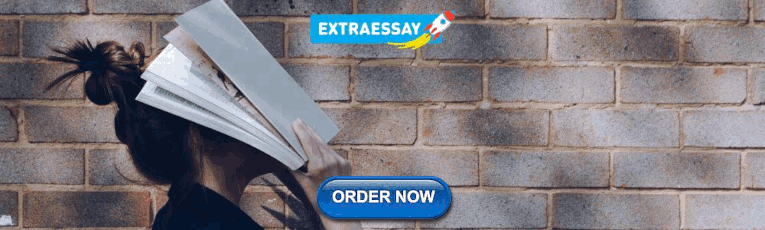
IMAGES
VIDEO
COMMENTS
Note that select Kotlin as the programming language. Step 2: Add mic image in drawable folder. Navigate to the app > res > drawable > Right-click on it. Then click on New > Vector Asset. After that click on the clip art icon. Inside that simply search for the mic icon and we will get to see the icon.
In the onBeginningOfSpeeh() method we will add the following code to tell the user that his voice is being recognized. @Override public void onBeginningOfSpeech() {editText.setText("Listening ...
The Text-to-speech API, unlike Speech Recognition, is available without Google Services, and can be found in android.speech.tts package. Source code You can find the source of this tutorial on GitHub. Let's develop! Fire up Android Studio and create a project with a Blank Activity. User interface
Pull requests. Speech To Text Recognition App converts spoken words to written text in real-time using the browser's speech recognition API. The app is built on React and provides users with easy control of speech recognition, manipulation of text, and copying to the clipboard. It is an accessible way to input text for users with disabilities.
Here’s an example of how to implement speech recognition using RecognizerIntent in Kotlin: class SpeechToTextManager(private val context: Context) {. private val recognizer = SpeechRecognizer ...
1. Create a new project. Start by creating a new Android project in Android Studio or your preferred development environment. 2. Setup a layout. Create a Button to trigger the speech recognition ...
Modern Android. Quickly bring your app to life with less code, using a modern declarative approach to UI, and the simplicity of Kotlin. Explore Modern Android. Adopt Compose for teams. Get started. Start by creating your first app. Go deeper with our training courses or explore app development on your own.
Android has a builtin feature i.e Speech To Text through which we can provide Speech input to your app. In this video we will use this feature to take Voice ...
Add this topic to your repo. To associate your repository with the speech-to-text-android topic, visit your repo's landing page and select "manage topics." GitHub is where people build software. More than 100 million people use GitHub to discover, fork, and contribute to over 420 million projects.