Two linters I'll always add to new Go projects: errcheck and ineffassign
Jul 17, 2020
The reason for that is that they are the best bang for your buck by far. They are easy to appease, I have yet to see them produce false positives, they very frequently catch outright buggy code and retrofitting them is a pain.
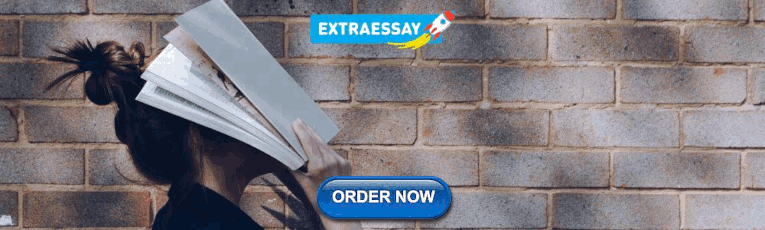
ineffassign
This is the sort of thing that ineffassign prevents:
This is perfectly valid code, and will write garbage sometimes, without any indication whatsoever. Yes, Go does refuse to compile code like this
because data is not used, but declaring a variable and then immediately overwriting its value is perfectly legal and almost never what you want. At best the variable was never needed, in which case a _ is a good way to signal it, or it was meant to be used and ineffassign found a bug.
I’ve seen this pattern frequently enough in tests, where part of the test code accidentally doesn’t check intermediate errors or return values, leading to parts of the test silently breaking over time.
To its credit, gopls does check for this now .
In a similar vein, errcheck enforces checking error return values. In Go, errors are values, and unlike other 1 languages 2 , nothing enforces they are checked.
This is valid:
And so are all these:
The only difference is that the first example carries no indication where this was intentional or not. errcheck enforces that error values are at least assigned to _ , therefore being explicit that a decision was made to ignore the error 3 .
One case where this matters is when writing new code. It’s easy enough to forget about checking all error returns of all functions called. Again, tests passing by accident is a very frequent occasion, and so is production code.
Another also interesting case is functions changing signatures. When adding an error return to a function that previously returned nothing or updating a dependency that does so, you probably want to verify all the call sites, at the very least making the executive choice to explicitly ignore the errors.
Retrofitting using golangci-lint
golangci-lint has positioned itself as the tool everyone uses on CI, and I’d say with good reason. It supports many linters, has improved massively over the past couple of years and has facilities for wiring up into existing codebases by only checking code that changes 4 , allowing for incremental cleanup.
For example:
No one has to fix the unchecked error, until they touch the call in bar() . This works well, until you realise there are transformations where this heuristic falls flat. This is still true according to the latest golangci-lint, 1.28.3.
Here is an example of this in action:
Since the call to foo() is not touched, golangci-lint considers the unchecked error pre-existing and does not report it! The check is completely elided on changes that simply go from zero returns to a single error return. This simply makes the check not as useful as it could be, allowing regressions to merge over time.
The other problem with retrofitting is that the cleanup can be boring and take a long time. Clearing hundreds for errors in bulk is mind-numbing. Merely shifting around existing code might require fixing existing issues, unrelated to the change at hand.
Why go through that, when simply adding these linters from the start does the trick and saves you from bugs?
Addendum - 18th July
I got a bit curious about k8s’ code, and ran ineffassign against it. There is one case where ineffassign could be considered noisy, and that is using foo := true instead of var foo bool :
The code in question:
This nudges towards var exist bool or bool := false . Clearly there is no bug here, the result is the same either way, so it boils down to the style used when declaring variables.
good ↩
Rust ↩
Not necessarily a good decision, you can always find yourself staring at git blame wondering why. ↩
according to git and revgrep, using the new- settings in the config . Nowadays it works, a long time ago I found out the hard way it didn’t ↩
Trail of Bits Blog
Security assessment techniques for go projects.
- November 7, 2019
The Trail of Bits Assurance practice has received an influx of Go projects, following the success of our Kubernetes assessment this summer. As a result, we’ve been adapting for Go projects some of the security assessment techniques and tactics we’ve used with other compiled languages.
We started by understanding the design of the language, identifying areas where developers may not fully understand the functionality of a language semantic. Many of these misunderstood semantics originated from findings we reported to our clients and independent research into the language itself. While not exhaustive, some of these problem areas include scoping, coroutines, error handling, and dependency management. Notably, many of theses are not directly related to the runtime. The Go runtime itself is designed to be safe by default, preventing many C-like vulnerabilities.
With a better understanding of the root causes, we searched for existing tooling to help us quickly and effectively instrument client codebases. The result was a sample of static and dynamic open-source tools, including several that were Go-agnostic. To complement these tools, we also identified several compiler configurations that help with instrumentation.
Static analysis
Because Go is a compiled language, the compiler detects and prevents many potentially erroneous patterns before the binary executable is even produced. While this is a major annoyance for newer Go developers, these warnings are extremely important in preventing unexpected behavior and keeping code clean and readable.
Static analysis tends to catch a lot of very low hanging fruit not included in compiler errors and warnings. Within the Go ecosystem, there are many disparate tools such as go-vet , staticcheck , and those within the analysis package. These tools typically identify problems like variable shadowing, unsafe pointer use, and unused function return values. Investigating the areas of a project where these tools display warnings typically leads to exploitable functionality.
These tools are by no means perfect. For example, go-vet can miss very common accidents like the one below, where the A function’s err return value is unused, and immediately reassigned during the assignment of bSuccess on the left-hand side of the expression. The compiler will not provide a warning, and go-vet does not detect this; nor does errcheck . In fact, the tools that successfully identify this case (non-exhaustive) are the aforementioned staticcheck and ineffassign , which identify the err return value of A as unused or ineffectual.
Figure 1: An example program showing an ineffectual assignment of err tricking go-vet and errcheck into considering err as checked.
Figure 2: The output of the example program, along with errcheck, go-vet, staticcheck, and ineffassign.
When you look deeper into this example, you may wonder why the compiler does not warn on this problem. The Go compiler will error when variables are not used within a program, but this example successfully compiles. This is caused by the semantics of the “short variable declaration.”
Figure 3: The grammar specification of the “short variable declaration.”
According to the specification, the short variable declaration has the special ability to redeclare variables as long as:
- The redeclaration is in a multi-variable short declaration.
- The redeclared variable is declared earlier in the same block or function’s parameter list.
- The redeclared variable is of the same type as the previous declaration.
- At least one non-blank variable in the declaration is new.
All of these constraints hold in the previous example, preventing the compiler from producing errors for this problem.
Many tools have edge cases like this where they are unsuccessful in identifying related issues, or identify an issue but describe it differently. Compounding the problem, these tools often require building the Go source code before analysis can be performed. This makes third-party security assessments complicated if the analysts cannot easily build the codebase or its dependencies.
Despite these pitfalls, when put together, the available tools can provide good hints as to where to look for problems within a given project, with just a little bit of effort. We recommend using gosec , go-vet , and staticcheck , at a minimum. These have the best documentation and ergonomics for most codebases. They also provide a wide variety of checks (such as ineffassign or errcheck ) for common issues, without getting too specific. For more in-depth analysis of a particular type of issue, however, one might have to use the more specific analyzers, develop custom tooling directly against the SSA , or use $emmle .
Dynamic analysis
Once static analysis has been performed and the results have been reviewed, dynamic analysis techniques are typically the next step for deeper results. Due to Go’s memory safety, the problems normally found with dynamic analysis are those that result in a hard crash or an invalidation of program state. Various tools and approaches have been built to help identify these types of issues within the Go ecosystem. Additionally, it’s possible to retrofit existing language-agnostic tooling for the dynamic testing of Go software, which we show next.
The best-known dynamic testing tool in the Go space is likely Dimitry Vyukov’s implementation of dvyukov/go-fuzz . This tool allows you to quickly and effectively implement mutational fuzzing. It even has an extensive wall of trophies . More advanced users may also find the distributed fuzzing and libFuzzer support useful when hunting for bugs.
Google also produced a more primitive fuzzer with a confusingly similar name, google/gofuzz , that assists users by initializing structures with random values. Unlike Dimitry’s go-fuzz , Google’s gofuzz does not generate a harness or assist with storing crash output, fuzzed input, or any other type of information. While this can be a downside for testing some targets, it makes for a lightweight and extensible framework.
For the sake of brevity, we refer you to examples of both tools in their respective READMEs.
- google/gofuzz#gofuzz
- dvyukov/go-fuzz#usage
Property testing
Diverging from more traditional fuzzing approaches, Go’s testing package (typically used for unit and integration testing) provides the testing/quick sub-package for “black box testing” of Go functions. In other terms, it is a basic primitive for property testing. Given a function and generator, the package can be used to build a harness to test for potential property violations given the range of the input generator. The following example is pulled directly from the documentation .
Figure 4: The OddMultipleOfThree function is being tested, where its return value should always be an odd multiple of three. If it’s not, the f function will return false and the property will be violated. This is detected by the quick. Check function.
While the functionality provided by this package is acceptable for simple applications of property testing, important properties do not often fit well into such a basic interface. To address these shortcomings, the leanovate/gopter framework was born. Gopter provides a wide variety of generators for the common Go types, and has helpers to assist you in creating your own generators compatible with Gopter. Stateful tests are also supported through the gopter/commands sub-package, which is useful for testing that properties hold across sequences of actions. Compounding this, when a property is violated, Gopter shrinks the generated inputs. See a brief example of property tests with input shrinking in the output below.
Figure 5: The testing harness for the Compute structure.
Figure 6: Executing the test harness and observing the output of the property tests, where Divide fails.
Fault injection
Fault injection has been surprisingly effective when attacking Go systems. The most common mistakes we found using this method involve the handling of the error type. Since error is only a type in Go, when it is returned it does not change a program’s execution flow on it’s own like a panic statement would. We identify such bugs by enforcing errors from the lowest level: the kernel. Because Go produces static binaries, faults must be injected without LD_PRELOAD . One of our tools, KRF , allows us to do exactly this.
During our recent assessment of the Kubernetes codebase, the use of KRF provided a finding deep inside a vendored dependency, simply by randomly faulting read and write system calls spawned by a process and its children. This technique was effective against the Kubelet, which commonly interfaces with the underlying system. The bug was triggered when the ionice command was faulted, producing no output to STDOUT and sending an error to STDERR . After the error was logged, execution continued instead of returning the error in STDERR to the caller. This results in STDOUT later being indexed, causing an index out of range runtime panic.
Figure 7: The shortened callstack of the resulting Kubelet panic.
Figure 8: The logging of STDERR without returning the error to the caller.
Figure 9: The attempted indexing of STDOUT, even though it is empty. This is the cause of the runtime panic.
For a more complete walkthrough containing reproduction steps, our Kubernetes Final Report details the use of KRF against the Kubelet in Appendix G (pg. 109).
Go’s compiler also allows instrumentation to be included in a binary, which permits detection of race conditions at runtime. This is extremely useful for identifying potentially exploitable races as an attacker, but it can also be leveraged to identify incorrect handling of defer, panic, and recover . We built trailofbits/on-edge to do exactly this: Identify global state changes between a function entrypoint and the point at which a function panics, and exfiltrate this information through the Go race detector. More in-depth use of OnEdge can be found in our previous blog post, “Panicking the Right Way in Go.”
In practice, we recommend using:
- dvyukov/go-fuzz to build harnesses for components parsing input,
- google/gofuzz for testing structure validations,
- leanovate/gopter for augmenting existing unit and integration tests and testing specification correctness, and
- trailofbits/krf and trailofbits/on-edge for testing error handling.
All of these tools, with the exception of KRF, require a bit of effort to use in practice.
Using the compiler to our advantage
The Go compiler has many built-in features and directives that aid in finding bugs. These features are hidden in and throughout various switches, and require a bit of configuration for our purposes.
Subverting the type system
Sometimes when attempting to test the functionality of a system, the exported functions aren’t what we want to test. Getting testable access to the desired functions may require renaming a lot of them so they can be exported, which can be burdensome. To help address this problem, the build directives of the compiler can be used to perform name linking, accessing controls provided by the export system. As an example of this functionality, the program below (graciously extracted from a Stack Overflow answer ) accesses the unexported reflect.typelinks function and subsequently iterates the type link table to identify types present in the compiled program.
Figure 10: A generalized version of the Stack Overflow answer, using the link name build directive.
Figure 11: The output of the typelinks table.
In situations where you need even more granular control at runtime (i.e., more than just the link name directive), you can write in Go’s intermediate assembly and include it during compilation. While it may be incomplete and slightly out of date in some places, the teh-cmc/go-internals repository provides a great introduction to how Go assembles functions.
Compiler-generated coverage maps
To help with testing, the Go compiler can perform preprocessing to generate coverage information . This is intended for identifying unit and integration testing coverage information, but we can also use it to identify coverage generated by our fuzzing and property testing. Filippo Valsorda provides a simple example of this in a blog post .
Type-width safety
Go has support for automatically determining the size of integers and floating-point numbers based on the target platform. However, it also allows for fixed-width definitions, such as int32 and int64 . When mixing both automatic and fixed-width sizes, there are opportunities for incorrect assumptions about behavior across multiple target platforms.
Testing against both 32-bit and 64-bit platform builds of a target will help identify platform-specific problems. These problems tend to be found in areas performing validation, decoding, or type conversion, where improper assumptions about the source and destination type properties are made. Examples of this were identified in the Kubernetes security assessment, specifically TOB-K8S-015: Overflows when using strconv.Atoi and downcasting the result (pg. 42 in the Kubernetes Final Report ), with an example inlined below.
Figure 12: An example of downcasting to a fixed-width integer from an automatic-width integer (returned by Atoi).
Figure 13: The resulting overflow from incorrect type-width assumptions.
In practice, the type system subversion is rarely necessary. The most interesting targets for testing are already exported, available through traditional imports. We recommend using this only when helpers and similar unexported functions are required for testing. As for testing type-width safety, we recommend compiling against all targets when possible, even if it is not directly supported, since problems may be more apparent on different targets. Finally, we recommend generating coverage reports on projects with unit and integration tests, at a minimum. It helps identify areas that are not directly tested, which can be prioritized for review.
A note about dependencies
In languages such as JavaScript and Rust , dependency managers have built-in support for dependency auditing—scanning project dependencies for versions known to have vulnerabilities. In Go, no such tool exists, at least not in a publicly available and non-experimental state.
This lack likely stems from the fact that there are many different methods of dependency management: go-mod , go-get , vendored , etc. These various methods use radically different approaches, resulting in no straightforward way to universally identify dependencies and their versions. Furthermore, in some cases it is common for developers to subsequently modify their vendored dependency source code.
The problem of dependency management has progressed over the years of Go’s development, and most developers are moving towards the use of go mod . This allows dependencies to be tracked and versioned within a project through the go.mod file , opening the door for future dependency scanning efforts. An example of such an effort can be seen within the OWASP DependencyCheck tool, which has an experimental go mod plugin .
Ultimately, there are quite a few tools available for use within the Go ecosystem. Although mostly disparate, the various static analysis tools help identify “low hanging fruit” within a given project. When looking for deeper concerns, fuzzing, property testing, and fault injection tools are readily available. Compiler configuration subsequently augments the dynamic techniques, making it easier to build harnesses and evaluate their effectiveness.
Interested in seeing these techniques shake out bugs in your Go systems? Trail of Bits can make that happen. Do you want custom analysis built specifically for your organization? We do that too. Contact us !
Share this:
5 thoughts on “ security assessment techniques for go projects ”.
Pingback: Security assessment techniques for Go projects – Hacker News Robot
Excellent post thanks a lot for sharing this, secure development practices are very important in product development. Wanted to give a shout-out to https://github.com/golangci/golangci-lint for bringing some of the linters mentioned in the post into a nice bundle.
Pingback: Security assessment techniques for Go projects - Golang News
Actually, Snyk is free and the CLI is open source tool for security vulnerabilities scanning and it supports Go projects that are managed with dep, govendor or go modules. How to get started here: https://snyk.io/docs/snyk-for-golang/ and there’s a whole vulnerabilities database open to browsing at https://snyk.io/vuln?type=golang for Golang.
Full disclosure: I’m a developer advocate at Snyk
CodeQL is a tool for static analysis that supports Go
https://help.semmle.com/QL/learn-ql/go/ql-for-go.html
It’s free for open-source projects and I think they offer options to use it for proprietary software. In fact, the rules for detecting issues are open-sourced. Anybody can contribute.
Leave a Reply Cancel reply
Discover more from trail of bits blog.
Subscribe now to keep reading and get access to the full archive.
Type your email…
Continue reading
Ineffassign Save
Detect ineffectual assignments in Go code.
ineffassign
Detect ineffectual assignments in Go code. An assignment is ineffectual if the variable assigned is not thereafter used.
This tool misses some cases because it does not consider any type information in its analysis. For example, assignments to struct fields are never marked as ineffectual. It should, however, never give any false positives.
For basic usage, run the following command from the root of your project:
Which will analyze all packages beneath the current directory.
ineffassign returns 1 if any problems were found in the checked files. It returns 3 if there were any invalid arguments.
Open Source Agenda Badge
From the blog.

How to Choose Which Programming Language to Learn First?
Sign in to osa.
Information
Github star history, license type, more resources, you can contribute, latest from our blog, a closer look at bugprove.
Sun Nov 19 2023
I have never been a huge fan of IoT devices. Granted, they make our life easier, but they also open the door to a lot of security issues. Most IoT devices are black boxes. I don't know what's inside and I don't know what they connect to.
Welcoming Bearer as Our Sponsor: Simple Ruby and JavaScript App Security
Thu Apr 06 2023
As a developer, I have faced my fair share of security mishaps. I recall times when I accidentally exposed sensitive data in logs or sent a network request over a non-encrypted HTTP channel when HTTPS was available. I'm sure many of you can relate to these situations. We may not be security experts, but that doesn't mean we shouldn't take measures to protect our applications. This is where Bearer, a new security tool for Ruby and JavaScript apps (Java coming soon), comes into play.
Redesigning Analysis Tools
Wed Mar 29 2023
We are happy to announce that we completely rebuilt analysis-tools.dev from scratch with more features and a new design!This is a major milestone for us, as it marks the first time we sat down to reinvision what the project should become in the next few years.
Picking the Right Static Analysis Tool For Your Use-Case
Tue Jan 26 2021
This project started as a way to scratch my own itch:Years later, many people still seem to have the same problem. There are more than 500 static analysis (SAST) tools out there; how can you possibly find the "best" one?
Static Analysis Is Broken - Let’s Fix It!
Wed Aug 19 2020
Static analysis is great! It helps improve code quality by inspecting source code without even running it. There are hundreds of great tools to choose from — many are free or open-source . Unfortunately, many projects still don’t make use of static analysis tools for various reasons.
Our Mission
Thu Jul 16 2020
We found that static code analysis is a topic that is attracting a lot of engineers, which care about code-quality and solid engineering standards. Our goal is to create an open community for developers that want to take their code and skill set to the next level.
Welcome, DeepCode!
Today we welcome DeepCode as our first sponsor.It makes us incredibly happy to see the backing of our community project from such a forward-thinking company. Just like us, DeepCode thinks that the space of analysis tools could be vastly improved to increase code quality and foster best practices within organizations of any size.
Stay Informed
Sign up to our newsletter and always stay up to date with the latest tools and trends in development
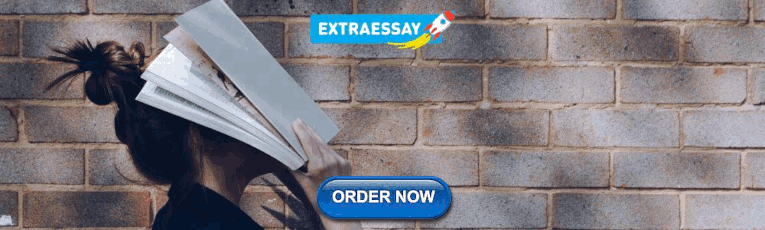
ineffassign
Detect ineffectual assignments in Go code.
Tutorials / Guides
47 Alternatives for ineffassign
Find inefficiently packed structs.
Checks whether HTTP response body is closed.
Finds unused code.
dingo-hunter
Static analyser for finding deadlocks in Go.
Finds assignments/declarations with too many blank identifiers.
Reports potentially duplicated code.
Check that error return values are used.
Wrap and fix Go errors with the new %w verb directive. This tool analyzes fmt.Errorf() calls and reports calls that contain a verb directive that is different than the new %w verb directive introduced in Go v1.13. It's also capable of rewriting calls to use the new %w wrap verb directive.
Get info on length of functions in a Go package.
Package ast declares the types used to represent syntax trees for Go packages.
go-consistent
Analyzer that helps you to make your Go programs more consistent.
Go source code linter that maintains checks which are currently not implemented in other linters.
go tool vet --shadow
Reports variables that may have been unintentionally shadowed.
Examines Go source code and reports suspicious.
Go AST (Abstract Syntax Tree) based static analysis tool with Rego.
gochecknoglobals
Checks that no globals are present.
Finds repeated strings that could be replaced by a constant.
Calculate cyclomatic complexities of functions in Go source code.
Checks if the code is properly formatted and could not be further simplified.
Checks missing or unreferenced package imports.
Golang security analysis with a focus on minimizing false positives. It is capable of tracing the source of variables and function arguments to determine whether input sources are safe.
GolangCI-Lint
Alternative to Go Meta Linter : GolangCI-Lint is a linters aggregator.
Prints out coding style mistakes in Go source code.
goroutine-inspect
An interactive tool to analyze Golang goroutine dump.
gosec (gas)
Inspects source code for security problems by scanning the Go AST.
Syntactic and semantic analysis similar to the Go compiler.
govulncheck
Govulncheck reports known vulnerabilities that affect Go code. It uses static analysis of source code or a binary's symbol table to narrow down reports to only those that could affect the application. By default, govulncheck makes requests to the Go vulnerability database at https://vuln.go.dev. Requests to the vulnerability database contain only module paths, not code or other properties of your program.
Suggest narrower interfaces that can be used.
Report long lines.
Detect structs that would take less memory if their fields were sorted.
Finds commonly misspelled English words.
Finds naked returns.
Finds unused arguments in function declarations.
Finds slice declarations that could potentially be preallocated.
A tool for posting review comments from any linter in any code hosting service.
Fast, configurable, extensible, flexible, and beautiful linter for Go. Drop-in replacement of golint.
Static analysis tool for Golang that protects against SQL injections.
A lightweight static code analyzer designed for developers and security teams. It allows you to analyze and transform source code with an intuitive DSL similar to sed, but for code.
staticcheck
Go static analysis that specialises in finding bugs, simplifying code and improving performance.
structcheck
Find unused struct fields.
Static analyzer for Go that recommends struct field rearrangements to provide for maximum space/allocation efficiency
Show location of test failures from the stdlib testing module.
Detect redundant type conversions.
Find unused function parameters.
Find unused global variables and constants.
Enforces empty lines at the right places.
Instant live visualization of your Go application runtime statistics in the browser. It plots heap usage, MSpans/MCaches, Object counts, Goroutines and GC/CPU fraction.
23 Multi-Language Tools
Applicationinspector.
Creates reports of over 400 rule patterns for feature detection (e.g. the use of cryptography or version control in apps).
autocorrect
A linter and formatter to help you to improve copywriting, correct spaces, words, punctuations between CJK (Chinese, Japanese, Korean).
Open-Source static code analysis tool to discover, filter and prioritize security risks and vulnerabilities leading to sensitive data exposures (PII, PHI, PD). Highly configurable and easily extensible, built for security and engineering teams.
Statically generates a call graph image and displays it on screen.
Checkmarx CxSAST
Commercial Static Code Analysis which doesn't require pre-compilation.
Emerge is a source code and dependency visualizer that can be used to gather insights about source code structure, metrics, dependencies and complexity of software projects. After scanning the source code of a project it provides you an interactive web interface to explore and analyze your project by using graph structures.
Finds N+1 queries (SQL calls in a for loop) in go code
Comments on style violations in GitHub pull requests. Supports Coffeescript, Go, HAML, JavaScript, Ruby, SCSS and Swift.
Lizard is an extensible Cyclomatic Complexity Analyzer for many programming languages including C/C++ (doesn't require all the header files or Java imports). It also does copy-paste detection (code clone detection/code duplicate detection) and many other forms of static code analysis. Counts lines of code without comments, CCN (cyclomatic complexity number), token count of functions, parameter count of functions.
Mega-Linter
Mega-Linter can handle any type of project thanks to its 70+ embedded Linters, its advanced reporting, runnable on any CI system or locally, with assisted installation and configuration, able to apply formatting and fixes
A fast, open-source, static analysis tool for finding bugs and enforcing code standards at editor, commit, and CI time. Its rules look like the code you already write; no abstract syntax trees or regex wrestling. Supports 17+ languages.
ShiftLeft Scan
Scan is a free open-source DevSecOps platform for detecting security issues in source code and dependencies. It supports a broad range of languages and CI/CD pipelines.
Sigrid helps you to improve your software by measuring your system's code quality, and then compares the results against a benchmark of thousands of industry systems to give you concrete advice on areas where you can improve.
SonarLint is a free IDE extension available for IntelliJ, VS Code, Visual Studio, and Eclipse, to find and fix coding issues in real-time, flagging issues as you code, just like a spell-checker. More than a linter, it also delivers rich contextual guidance to help developers understand why there is an issue, assess the risk, and educate them on how to fix it.
Performs static analysis on raw SQL queries in your Go code base to surface potential runtime errors. It checks for SQL syntax error, identifies unsafe queries that could potentially lead to SQL injections makes sure column count matches value count in INSERT statements and validates table- and column names.
StaticReviewer
Static Reviewer executes code checks according to the most relevant Secure Coding Standards, OWASP, CWE, CVE, CVSS, MISRA, CERT, for 40+ programming languages, using 1000+ built-in validation rules for Security, Deadcode & Best Practices Available a module for Software Composition Analysis (SCA) to find vulnerabilities in open source and third party libraries.
Super-Linter
Combination of multiple linters to install as a GitHub Action.
Static code analysis tool for Java,C,C++,C#,Go.
TencentCodeAnalysis
Tencent Cloud Code Analysis (TCA for short, code-named CodeDog inside the company early) is a comprehensive platform for code analysis and issue tracking. TCA consist of three components, server, web and client. It integrates of a number of self-developed tools, and also supports dynamic integration of code analysis tools in various programming languages.
Linter for integrating annotated TODOs with your issue trackers
Modern repositories include many technologies, each with its own set of linters. With 30+ linters and counting, Trunk makes it dead-simple to identify, install, configure, and run the right linters, static analyzers, and formatters for all your repos.
Crash Analysis and Severity Report.
Continuous Hybrid Fuzzing and Dynamic Analysis for Security Development Lifecycle.
Help make this list better Suggest Tools
Our Sponsors
This website is completely open source. To fund our work, we fully rely on sponsors. Thanks to them, we can keep the site free for everybody. Please check out their offers below.
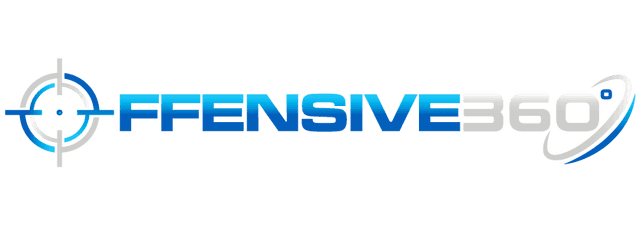
Package Info
Ineffassign, tool to detect ineffectual assignments in go code, unspecified.
This tool misses some cases because does not consider any type information in its analysis. (For example, assignments to struct fields are never marked as ineffectual.) It should, however, never give any false positives.
ineffassign
This package is not in the latest version of its module.
Detect ineffectual assignments in Go code.
This tool misses some cases because does not consider any type information in its analysis. (For example, assignments to struct fields are never marked as ineffectual.) It should, however, never give any false positives.
Documentation ¶
There is no documentation for this package.
Source Files ¶
- ineffassign.go
Keyboard shortcuts
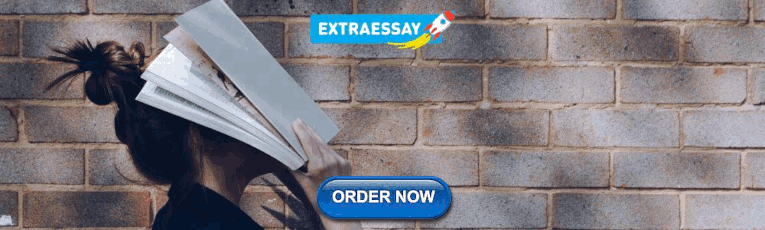
IMAGES
VIDEO
COMMENTS
@Ishmeet, note that the linter wasn't forcing you to use a var declaration; instead it hinted at that you might have a bug in your code—because the first assignment could be way more involved like assigning the return value of some function call which would return a value inintialized in a complicated way, and possibly depending on some input data.
ineffassign. Detect ineffectual assignments in Go code. An assignment is ineffectual if the variable assigned is not thereafter used. This tool misses some cases because it does not consider any type information in its analysis. For example, assignments to struct fields are never marked as ineffectual. It should, however, never give any false ...
go初学踩坑:ineffectual assignment to 变量 (ineffassign)错误 最近在写一些go的代码,在写到一个一行代码的时候,突然出现了这么一个错误:ineffectual assignment to content (ineffassign)其中content是我的一个变量,我的代码如下:func main() { content, code := httpGet("https://www ...
An assignment is ineffectual if the variable assigned is not thereafter used. This tool misses some cases because it does not consider any type information in its analysis. For example, assignments to struct fields are never marked as ineffectual. ... ineffassign returns 1 if any problems were found in the checked files. It returns 3 if there ...
ineffassign version: last version available go version: go version go1.18.3 Running the linter when interacting with a var after passing it to a deferred function call marks it as ineffectual assignment package main import "errors" func ...
Package ineffassign implements a static analyzer to ensure that there are no ineffectual assignments in source code. Index ¶ Constants; Variables; Constants ¶ View Source const Doc = "Tool to make sure there are no ineffectual assignments in source code" Doc explaining the tool.
package main import "fmt" func main() { var i int i = 3 i = 5 fmt.Println(i) } $ ineffassign main.go does not produce any results. It should catch that the i = 3 assignment was ineffective (its value was never used). I created the issue ...
At best the variable was never needed, in which case a _ is a good way to signal it, or it was meant to be used and ineffassign found a bug. I've seen this pattern frequently enough in tests, where part of the test code accidentally doesn't check intermediate errors or return values, leading to parts of the test silently breaking over time.
<snip>/main.go:10:12: ineffectual assignment to err Figure 2: The output of the example program, along with errcheck, go-vet, staticcheck, and ineffassign. When you look deeper into this example, you may wonder why the compiler does not warn on this problem.
Readme. ineffassign. Detect ineffectual assignments in Go code. An assignment is ineffectual if the variable assigned is not thereafter used. This tool misses some cases because it does not consider any type information in its analysis. For example, assignments to struct fields are never marked as ineffectual.
ineffassign. Detect ineffectual assignments in Go code. This tool misses some cases because it does not consider any type information in its analysis. (For example, assignments to struct fields are never marked as ineffectual.) ... ineffassign returns 1 if any problems were found in the checked files. It returns 3 if there were any invalid ...
This program triggers a false positive reported by ineffassign: main.go:9:3: ineffectual assignment to iv. iv and ivSlice point to the same data so this is not a ineffective assignment. package main. import "fmt" func main () {. var iv [ 16] byte ivSlice := iv [:] for i := range ivSlice {.
ineffassign. Detect ineffectual assignments in Go code. An assignment is ineffectual if the variable assigned is not thereafter used. This tool misses some cases because it does not consider any type information in its analysis. For example, assignments to struct fields are never marked as ineffectual. It should, however, never give any false ...
Performs static analysis on raw SQL queries in your Go code base to surface potential runtime errors. It checks for SQL syntax error, identifies unsafe queries that could potentially lead to SQL injections makes sure column count matches value count in INSERT statements and validates table- and column names. go. sql.
Package ineffassign implements a static analyzer to ensure that there are no ineffectual assignments in source code.
Hi, if it's possible, I would like to work on this. I had run ineffassign on x/text again an found more results. See the the updated list below. Almost all issues can be easily fixed by simply removing or refactoring the ineffectual assignments clauses.
Tool to detect ineffectual assignments in Go code. Unspecified. This tool misses some cases because does not consider any type information in its analysis. (For example, assignments to struct fields are never marked as ineffectual.) ... ineffassign; ..0+git20210225.2e10b26-bp154.1.73 info: GA Release: 2022-05-09: 15 SP4: AArch64; ppc64le ...
ineffassign. Detect ineffectual assignments in Go code. This tool misses some cases because does not consider any type information in its analysis. (For example, assignments to struct fields are never marked as ineffectual.) It should, however, never give any false positives.
ineffassign. Detect ineffectual assignments in Go code. This tool misses some cases because does not consider any type information in its analysis. (For example, assignments to struct fields are never marked as ineffectual.) It should, however, never give any false positives.
http_example_test.go:57:12: ineffectual assignment to `err` (ineffassign) listener, err := net.ListenTCP("tcp", &net.TCPAddr{}) The text was updated successfully, but these errors were encountered: All reactions. Copy link Owner. dgrijalva commented Jun 20, 2019. Let's just track ...