30 ReactJS Coding Interview Questions for Beginner, Mid-Level and Expert Developers
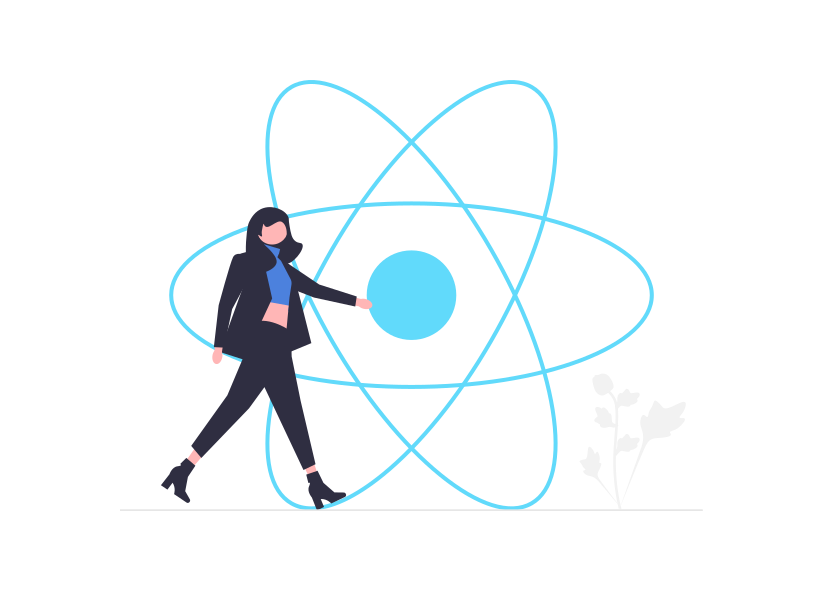
ReactJS is one of the leading front-end development frameworks in the IT industry. It is part of the open-source development ecosystem and is widely used by many companies.
Today we will discuss how to design technical coding challenges for ReactJS developers. Some ReactJS concepts that should be evaluated include React Component Lifecycle, State management, Virtual DOM, Router, JSX and hooks.
We have divided the coding challenges into three sections based on the experience level of developers; beginner, mid-level and expert. Let’s start with coding challenges for beginner-level developers.
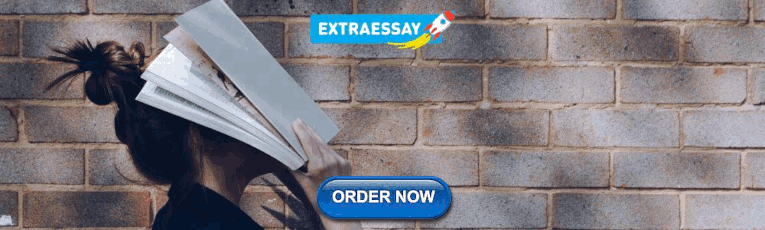
Interview Questions for Beginner ReactJS Developers

When preparing interview questions for beginner-level ReactJS developers, your questions should test basic javascript, props, state, JSX, life cycle methods, routing, and styling. Here are some sample questions in this regard:
What will be the output of the below code if the button is clicked:
Find the issue in the below code snippet after rendering the list of names.
Develop a currency converter application that allows users to input an amount in one currency and convert it to another. For the sake of this challenge, you can use a hard-coded exchange rate. Take advantage of React state and event handlers to manage the input and conversion calculations.
What will be the output when the user types in the input field:
What is missing in the below code snippet:
Create a stopwatch application through which users can start, pause and reset the timer. Use React state, event handlers and the setTimeout or setInterval functions to manage the timer’s state and actions.
Analyze the below code snippet and advise what will be shown on the screen when the App component is rendered with <App name=”Claire” />?
Find the issue with the form’s input field in the below code snippet:
Question 10
What issue exists in the below code regarding state variable:
Interview Questions for Mid-level ReactJS Developers
When designing interview questions for intermediate-level ReactJS developers, you should design challenges that test a deeper understanding of the framework and advanced features. Some of the areas that should be evaluated include advanced react concepts, Redux, Server-Side Rendering, API Integration, Advanced CSS and CI/CD. Find below some of the challenges that test these concepts:
Find the issue in the below code when using the index as a key for list items:
Analyze the below code and advise what will be the value of “Count” when the button is clicked:
Develop a messaging application that allows users to send and receive messages in real time. The application should display a list of conversations and allow the user to select a specific conversation to view its messages. The messages should be displayed in a chat interface with the most recent message at the top. Users should be able to send new messages and receive push notifications.
Build a weather application that retrieves real-time weather data from any weather API and displays it on the screen. The app should provide a search feature through which users can search for a location by city name or zip code and display the current temperature, humidity, wind speed and other relevant weather data.
What is the issue in the below code regarding useMemo hook:
What will be logged to the console after the button is clicked:
Will the below code throw any error when adding a new item to the list? If yes, what error will that be?
Build a simple drag-and-drop interface that allows users to reorder a list of items through drag-and-drop. Implement a visual cue to indicate the new position of the dragged item. Use React state, components and hooks to manage the list’s data and user interactions.
What is wrong with the below code:
Develop a paginated list view that shows items fetched from an API or a mock dataset. Implement a pagination system through which users can navigate between pages. Also, display the number of items per page. Use React state, components and hooks to manage the data and user interactions.
Interview Questions for Experts
When designing coding challenges for expert-level ReactJS developers, you should evaluate the advanced features of the language and the ability to develop applications in a distributed system. Some of the areas to evaluate include architecture and design patterns, performance optimization, testing, advanced react concepts, security and integration with other technologies. Here are some coding challenges which are suitable for expert ReactJS developers:
Find the issue in the below code:
What is the output of the Toolbar component in the below code snippet?
See the below code snippet and advise, will there be any issue making a REST API call in a component’s useEffect hook?
What will be the behavior of useEffect hook in the below code:
Develop a web application of your choice that makes frequent requests to an external REST API. To improve latency and reduce the load on the API, implement a client-side caching strategy that caches responses to API requests and serves them from the client-side cache when the same request is made again.
What is wrong with using async/await in a useEffect hook in reference to the below code snippet?
What will be the behavior of the useRef and useCallback hooks in the below code snippet?
Develop a file upload component to upload multiple files simultaneously. It should display progress indicators for each file and should display a success or error message after the upload is complete.
How can you optimize the handling of async data promises in the below code?
Develop a dashboard that shows real-time data from multiple sources, including REST APIs and websockets. The data should update in real time without needing to reload. The dashboard should display gracefully on mobile devices and should have a user-friendly UI/UX.
Today we went through 30 coding challenges for ReactJS developers. These coding challenges test the knowledge and skills of ReactJS engineers in various areas of ReactJS, ranging from basic ReacJS concepts to advanced concepts like architecture and design patterns.
By categorizing the coding challenges separately for beginner level to advanced level, we have made it easy for hiring managers to evaluate candidates based on their experience. By completing these challenges, developers can enhance their knowledge of ReactJS and gain valuable experience in solving relevant problems.

Further reading:
- Java Coding Interview Questions
- Python Coding Interview Questions
- JavaScript Coding Interview Questions
- C# Coding Interview Questions
- C++ Coding Interview Questions
- PHP Coding Interview Questions
- 73 Advanced Coding Interview Questions
- Share on Facebook
- Email this Page
- Share on LinkedIn
Contact Mentor
Projects + Tutorials on React JS & JavaScript
10 React JS Practice Exercises with solution
1. build search filter in react.
React code to build a simple search filter functionality to display a filtered list based on the search query entered by the user.
The following are the steps to create a Search filter using React JS:
- Declare React states for search input values.
- Create HTML input text for entering search term and update state in onChange function.
- Add Array.filter() on list of items with search term value.

2. Simple counter exercise
Creating a simple counter using React which increments or decrements count dynamically on-screen as the user clicks on the button. This exercise requires knowledge of fundamental React concepts such as State, Component, etc.
We can complete the simple counter exercise with the following steps:
- Create React state to store the count value.
- Declare JS functions to incement or decrement the value through setState() .
- Add HTML buttons with onClick to JSX code.

3. Display a list in React
React code to print each item from the list on the page using Array.map() function to display each item on the page.
The list of items is displayed using React JS through the following steps:
- Declare list of items as JS Array.
- Access each item using Array.map()
- Return JSX code in callback function for every item.

4. Build Accordion in React
Creating an accordion that toggles text content on click of the accordion header using React State and conditional rendering.
The following are the steps to create an accordion in React JS:
- Display every title of accordion with body.
- Hide every accordion body using element.display = none;
- Toggle visibility of accordion body on click of title.

5. Image Slider using React JS
React exercise to create an image slide, where users can view multiple images with next/previous buttons. Additionally, there is also an option to select an image from any index of the list through a click-on option circle.
The following are the steps to create an image slider in React JS:
- Declare array of objects with id and image url.
- For pre/next functionality, we decrement/increment count of the index of active image.
- Update active image index with clicked option.

6. Create a Checklist in React
React code to display a checklist with multiple options that can select and the selected options are dynamically displayed on the screen. React State is used to keep track of checked options and onChange() Event handler is triggered to alter the state whenever an option is checked or unchecked.
The following are the steps to create a Checklist in React JS:
- Create React state to track checkbox value.
- Function to update checkbox value based on event.target.checked
- Add checkbox input element to JSX code with onChange function.

7. Simple Login form in React
React code for simple login form where the user login by entering their username and password. The form inputs are validated to check if correct information is entered and the error messages are the validation fails. The login form is hidden and the “Welcome, ${name}” message is shown when the user login is successful.
The following are the steps to create a simple login form using React JS:
- Create name, email and password input form elements.
- React States to store user input values.
- Add form validation for compare name, email and password with correct values.
- Display “Welcome, ${name}” if login is successful, else display the error message.

8. Print data from REST API
React code to collect data from rest API using fetch() in JavaScript combined with useEffect() to load the content on page render.
The following are the steps to print data from REST API in React JS:
- Create React state to store the API response.
- Declare State to Component JSX code to display the API response.
- JS function where API call is through axios.get() or fetch() .
- Add callback function to update the React state with API response.
- Add HTML button with JS function with onChange attribute.

9. Multi-Page navigation using React Router
React code to develop a multipage application with navigation for Home, About and Blog pages. The route-based component rendering is implemented using the “ react-dom ” npm package to allow users to navigate to different pages and render the component with respect to the route.
The following are the steps to Navigation with React Router in React JS:
- Add “ react-router-dom ” npm package to package.json .
- Specify the path for every component.
- Enclose the routes with <Router> and <Switch> in App.js

10. Context API in React Components
Context allows values to be passed from multiple levels of child components without using props. Thus context can be used as an alternative to Redux in some of the cases. Learn more about the context in React from reactjs.org/docs/context.html .
The following are the steps to use Context API using React JS:
- Create React context using React.createContext() .
- Declare context object with properties and values.
- Wrap App.js with <AppContext.Provider> </AppContext.Provider> with values as context object.
- Access context object through components using React.useContext() .

React Beginner Tutorial

Related Articles
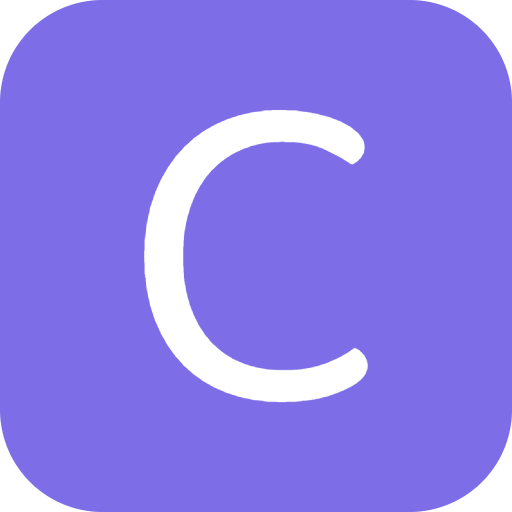

45 Advanced React Interview Questions (With Hooks) Devs Must Clarify and Know
React has been on a roll for a good 5 years now, and currently there is no end in sight. It keeps evolving. The average React Js Developer salary in USA is $125,000 per year or $64.10 per hour. Entry level positions start at $63,050 per year while most experienced workers make up to $195,000 per year. Follow along to learn most advanced React Interview Questions for your next tech interview.
Q1 : What are props in React?
Props are inputs to a React component. They are single values or objects containing a set of values that are passed to React Components on creation using a naming convention similar to HTML-tag attributes. i.e, They are data passed down from a parent component to a child component.
The primary purpose of props in React is to provide following component functionality:
- Pass custom data to your React component.
- Trigger state changes.
- Use via this.props.reactProp inside component's render() method.
For example, let us create an element with reactProp property,
This reactProp (or whatever you came up with) name then becomes a property attached to React's native props object which originally already exists on all components created using React library.
Q2 : How to create refs in React?
Refs are created using React.createRef() method and attached to React elements via the ref attribute. In order to use refs throughout the component, just assign the ref to the instance property with in constructor.
We can also use it in functional components with the help of closures.
Q3 : What are Higher-Order Components (HOC) in React?
A higher-order component (HOC) is a function that takes a component and returns a new component. Basically, it’s a pattern that is derived from React’s compositional nature We call them as “pure’ components” because they can accept any dynamically provided child component but they won’t modify or copy any behavior from their input components.
HOC can be used for many use cases as below,
- Code reuse, logic and bootstrap abstraction
- Render High jacking
- State abstraction and manipulation
- Props manipulation
Q4 : What are refs used for in React?
Refs are an escape hatch which allow you to get direct access to a DOM element or an instance of a component. In order to use them you add a ref attribute to your component whose value is a callback function which will receive the underlying DOM element or the mounted instance of the component as its first argument.
Above notice that our input field has a ref attribute whose value is a function. That function receives the actual DOM element of input which we then put on the instance in order to have access to it inside of the handleSubmit function.
It’s often misconstrued that you need to use a class component in order to use refs, but refs can also be used with functional components by leveraging closures in JavaScript.
Q5 : What are advantages of using React Hooks?
Primarily, hooks in general enable the extraction and reuse of stateful logic that is common across multiple components without the burden of higher order components or render props. Hooks allow to easily manipulate the state of our functional component without needing to convert them into class components.
Hooks don’t work inside classes (because they let you use React without classes). By using them, we can totally avoid using lifecycle methods, such as componentDidMount , componentDidUpdate , componentWillUnmount . Instead, we will use built-in hooks like useEffect .
Q6 : What are the differences between a Class component and Functional component ?
Class Components
- Class-based Components uses ES6 class syntax. It can make use of the lifecycle methods.
- Class components extend from React.Component.
- In here you have to use this keyword to access the props and functions that you declare inside the class components.
Functional Components
- Functional Components are simpler comparing to class-based functions.
- Functional Components mainly focuses on the UI of the application, not on the behavior.
- To be more precise these are basically render function in the class component.
- Functional Components can have state and mimic lifecycle events using Reach Hooks
Q7 : What does it mean for a component to be mounted in React?
It has a corresponding element created in the DOM and is connected to that.
Q8 : What is useState() in React?
Explain what is the use of useState(0) there:
useState is one of build-in react hooks. useState(0) returns a tuple where the first parameter count is the current state of the counter and setCounter is the method that will allow us to update the counter's state.
We can use the setCounter method to update the state of count anywhere - In this case we are using it inside of the setCount function where we can do more things; the idea with hooks is that we are able to keep our code more functional and avoid class based components if not desired/needed.
Q9 : What is the difference between state and props ?
Both props and state are plain JavaScript objects. While both of them hold information that influences the output of render, they are different in their functionality with respect to component. i.e,
- Props get passed to the component similar to function parameters
- State is managed within the component similar to variables declared within a function.
Q10 : What is the purpose of using super constructor with props argument in React?
A child class constructor cannot make use of this reference until super() method has been called. The same applies for ES6 sub-classes as well. The main reason of passing props parameter to super() call is to access this.props in your child constructors.
Passing props:
Not passing props:
The above code snippets reveals that this.props behavior is different only with in the constructor. It would be same outside the constructor.
Q11 : What's the difference between a Controlled component and an Uncontrolled one in React?
This relates to stateful DOM components (form elements) and the React docs explain the difference:
- A Controlled Component is one that takes its current value through props and notifies changes through callbacks like onChange . A parent component "controls" it by handling the callback and managing its own state and passing the new values as props to the controlled component. You could also call this a "dumb component".
- A Uncontrolled Component is one that stores its own state internally, and you query the DOM using a ref to find its current value when you need it. This is a bit more like traditional HTML.
Most native React form components support both controlled and uncontrolled usage:
In most (or all) cases you should use controlled components .
Q12 : Can you initialise state from a function? Provide and example
Yes ! Consider:
Q13 : Does React useState Hook update immediately ?
And how do you perform an action after useState hook has triggered?
React useState and setState don’t make changes directly to the state object; they create queues to optimize performance, which is why the changes don’t update immediately. The process to update React state is asynchronous for performance reasons.
To perform side effects after state has change, you must use the useEffect
Q14 : Given the React code defined above, can you identify two problems?
Take a look at the code below:
Given the code defined above, can you identify two problems?
- The constructor does not pass its props to the super class. It should include the following line:
- The event listener (when assigned via addEventListener() ) is not properly scoped because ES2015 doesn’t provide autobinding . Therefore the developer can re-assign clickHandler in the constructor to include the correct binding to this:
Q15 : How can I make use of Error Boundaries in functional React components?
As of v16.2.0, there's no way to turn a functional component into an error boundary. The componentDidCatch() method works like a JavaScript catch {} block, but for components. Only class components can be error boundaries. In practice, most of the time you’ll want to declare an error boundary component once and use it throughout your application.
Also bear in mind that try/catch blocks won't work on all cases . If a component deep in the hierarchy tries to update and fails, the try/catch block in one of the parents won't work -- because it isn't necessarily updating together with the child.
A few third party packages on npm implement error boundary hooks.
Q16 : What are the lifecycle methods of ReactJS class components?
- componentWillMount : Executed before rendering and is used for App level configuration in your root component.
- componentDidMount : Executed after first rendering and here all AJAX requests, DOM or state updates, and set up eventListeners should occur.
- componentWillReceiveProps : Executed when particular prop updates to trigger state transitions.
- shouldComponentUpdate : Determines if the component will be updated or not. By default it returns true. If you are sure that the component doesn't need to render after state or props are updated, you can return false value. It is a great place to improve performance as it allows you to prevent a rerender if component receives new prop.
- componentWillUpdate : Executed before re-rendering the component when there are pros & state changes confirmed by shouldComponentUpdate which returns true.
- componentDidUpdate : Mostly it is used to update the DOM in response to prop or state changes.
- componentWillUnmount : It will be used to cancel any outgoing network requests, or remove all event listeners associated with the component.
Q17 : What are the different phases of ReactJS component lifecycle ?
There are four different phases of React component’s lifecycle:
- Initialization: In this phase react component prepares setting up the initial state and default props.
- Mounting: The react component is ready to mount in the browser DOM. This phase covers componentWillMount and componentDidMount lifecycle methods.
- Updating: In this phase, the component get updated in two ways, sending the new props and updating the state. This phase covers shouldComponentUpdate, componentWillUpdate and componentDidUpdate lifecycle methods.
- Unmounting: In this last phase, the component is not needed and get unmounted from the browser DOM. This phase include componentWillUnmount lifecycle method.
Q18 : What do these three dots ( ... ) in React do?
What does the ... do in this React (using JSX) code and what is it called?
That's property spread notation. It was added in ES2018 (spread for arrays/iterables was earlier, ES2015).
For instance, if this.props contained a: 1 and b: 2 , then
would be the same as:
Spread notation is handy not only for that use case, but for creating a new object with most (or all) of the properties of an existing object — which comes up a lot when you're updating state, since you can't modify state directly:
Q19 : What is equivalent of the following using React.createElement ?
What is equivalent of the following using React.createElement ?
Q20 : What is prop drilling and how can you avoid it?
When building a React application, there is often the need for a deeply nested component to use data provided by another component that is much higher in the hierarchy. The simplest approach is to simply pass a prop from each component to the next in the hierarchy from the source component to the deeply nested component. This is called prop drilling .
The primary disadvantage of prop drilling is that components that should not otherwise be aware of the data become unnecessarily complicated and are harder to maintain.
To avoid prop drilling, a common approach is to use React context. This allows a Provider component that supplies data to be defined, and allows nested components to consume context data via either a Consumer component or a useContext hook.
Q21 : What is StrictMode in React?
React's StrictMode is sort of a helper component that will help you write better react components, you can wrap a set of components with <StrictMode /> and it'll basically:
- Verify that the components inside are following some of the recommended practices and warn you if not in the console.
- Verify the deprecated methods are not being used, and if they're used strict mode will warn you in the console.
- Help you prevent some side effects by identifying potential risks.
Q22 : What is the difference between ShadowDOM and VirtualDOM ?
Virtual DOM
Virtual DOM is about avoiding unnecessary changes to the DOM, which are expensive performance-wise, because changes to the DOM usually cause re-rendering of the page. Virtual DOM also allows to collect several changes to be applied at once, so not every single change causes a re-render, but instead re-rendering only happens once after a set of changes was applied to the DOM.
Shadow dom is mostly about encapsulation of the implementation. A single custom element can implement more-or-less complex logic combined with more-or-less complex DOM. An entire web application of arbitrary complexity can be added to a page by an import and <body><my-app></my-app> but also simpler reusable and composable components can be implemented as custom elements where the internal representation is hidden in the shadow DOM like <date-picker></date-picker> .
Q23 : What's wrong with that code?
What's wrong with that code?
Because this.props and this.state may be updated asynchronously, you should not rely on their values for calculating the next state. To fix it, use a second form of setState() that accepts a function rather than an object. That function will receive the previous state as the first argument, and the props at the time the update is applied as the second argument:
Q24 : Why do class methods need to be bound to a class instance?
In JavaScript, the value of this changes depending on the current context. Within React class component methods, developers normally expect this to refer to the current instance of a component, so it is necessary to bind these methods to the instance. Normally this is done in the constructor—for example:
Q25 : Why we should not update state directly?
If you try to update state directly then it won’t re-render the component.
Instead use setState() method. It schedules an update to a component’s state object. When state changes, the component responds by re-rendering
Note: The only place you can assign the state is constructor.
Q26 : Describe Flux vs MVC ?
Traditional MVC patterns have worked well for separating the concerns of data (Model), UI (View) and logic (Controller) — but MVC architectures frequently encounter two main problems:
- Poorly defined data flow: The cascading updates which occur across views often lead to a tangled web of events which is difficult to debug.
- Lack of data integrity: Model data can be mutated from anywhere, yielding unpredictable results across the UI.
With the Flux pattern complex UIs no longer suffer from cascading updates; any given React component will be able to reconstruct its state based on the data provided by the store. The Flux pattern also enforces data integrity by restricting direct access to the shared data.
Q27 : Describe how events are handled in React
In order to solve cross browser compatibility issues, your event handlers in React will be passed instances of SyntheticEvent , which is React’s cross-browser wrapper around the browser’s native event. These synthetic events have the same interface as native events you’re used to, except they work identically across all browsers.
What’s mildly interesting is that React doesn’t actually attach events to the child nodes themselves. React will listen to all events at the top level using a single event listener. This is good for performance and it also means that React doesn’t need to worry about keeping track of event listeners when updating the DOM.
Q28 : Do Hooks replace render props and higher-order components (HOC)?
Often, render props and higher-order components render only a single child. React team thinks Hooks are a simpler way to serve this use case .
There is still a place for both patterns (for example, a virtual scroller component might have a renderItem prop, or a visual container component might have its own DOM structure). But in most cases, Hooks will be sufficient and can help reduce nesting in your tree.
Q29 : Explain why and when would you use useMemo() ?
In the lifecycle of a component, React re-renders the component when an update is made. When React checks for any changes in a component, it may detect an unintended or unexpected change due to how JavaScript handles equality and shallow comparisons. This change in the React application will cause it to re-render unnecessarily.
Additionally, if that re-rendering is an expensive operation, like a long for loop , it can hurt performance. Expensive operations can be costly in either time, memory, or processing.
Optimal if the wrapped function is large and expensive.
Memoization is an optimization technique which passes a complex function to be memoized. In memoization, the result is “remembered” when the same parameters are passed-in subsequently.
useMemo takes in a function and an array of dependencies. The dependency’s list are the elements useMemo watches: if there are no changes, the function result will stay the same. Otherwise, it will re-run the function. If they don’t change, it doesn’t matter if our entire component re-renders, the function won’t re-run but instead return the stored result.
Q30 : How do I update state on a nested object with useState() ?
I have a component that receives a prop that looks like this:
How to update only the align property?
You need to use spread syntax . Also while trying to update current state based on previous, use the callback pattern os setState :
Q31 : How to conditionally add attributes to React components?
Is there a way to only add attributes to a React component if a certain condition is met?
For certain attributes, React is intelligent enough to omit the attribute if the value you pass to it is not truthy . For example:
will result in:
Another possible approach is:
Q32 : How to apply validation on props in ReactJS?
When the application is running in development mode, React will automatically check for all props that we set on components to make sure they must right correct and right data type. For incorrect type, it will generate warning messages in the console for development mode whereas it is disabled in production mode due performance impact. The mandatory prop is defined with isRequired.
The set of predefined prop types are below
- React.PropTypes.string
- React.PropTypes.number
- React.PropTypes.func
- React.PropTypes.node
- React.PropTypes.bool
For example, we define propTypes for user component as below,
Q33 : How would you go about investigating slow React application rendering?
One of the most common issues in React applications is when components re-render unnecessarily . There are two tools provided by React that are helpful in these situations:
- React.memo() : This prevents unnecessary re-rendering of function components
- PureComponent : This prevents unnecessary re-rendering of class components
Both of these tools rely on a shallow comparison of the props passed into the component—if the props have not changed, then the component will not re-render. While both tools are very useful, the shallow comparison brings with it an additional performance penalty, so both can have a negative performance impact if used incorrectly. By using the React Profiler, performance can be measured before and after using these tools to ensure that performance is actually improved by making a given change.
Q34 : What is the difference between using constructor vs getInitialState in React?
The difference between constructor and getInitialState is the difference between ES6 and ES5 itself. You should initialize state in the constructor when using ES6 classes, and define the getInitialState method when using React.createClass .
is equivalent to
Q35 : What is wrong with this code?
What is wrong with this code?
Nothing is wrong with it. It’s rarely used and not well known, but you can also pass a function to setState that receives the previous state and props and returns a new state, just as we’re doing above. And not only is nothing wrong with it, but it’s also actively recommended if you’re setting state based on previous state.
Q36 : What's the difference between useCallback and useMemo in practice?
With useCallback you memoize functions , useMemo memoizes any computed value :
(1) will return a memoized version of fn - same reference across multiple renders, as long as dep is the same. But every time you invoke memoFn , that complex computation starts again .
(2) will invoke fn every time dep changes and remember its returned value ( 42 here), which is then stored in memoFnReturn .
Q37 : When is it important to pass props to super() , and why?
The only one reason when one needs to pass props to super() is when you want to access this.props in constructor:
Not passing:
Note that passing or not passing props to super has no effect on later uses of this.props outside constructor.
Q38 : When to use useState vs useReducer ?
The decision of whether to use useState or useReducer isn't always black and white; there are many shades of grey. But,
use useState if you have:
- JavaScript primitives as state
- Simple state transitions
- Business logic within your component
- Different properties that don't change in any correlated way and can be managed by multiple useState hooks
use useReducer if you have:
- JavaScript objects or arrays as state
- Complex state transitions
- Complicated business logic more suitable for a reducer function (to separate concern of it)
- Different properties tied together that should be managed in one state object (when state depends on state)
Q39 : When would you use StrictMode component in React?
I've found it especially useful to implement strict mode when I'm working on new code bases and I want to see what kind of code/components I'm facing. Also if you're on bug hunting mode, sometimes it's a good idea to wrap with <StrictMode /> the components/blocks of code you think might be the source of the problem.
Q40 : Why would you need to bind event handlers to this ?
Binding is not something that is specifc to React, but rather how this works in Javascript. When you define a component using an ES6 class, a common pattern is for an event handler to be a method on the class. In JavaScript, class methods are not bound by default. If you forget to bind this.someEventHandler and pass it to onChange , this will be undefined when the function is actually called.
Generally, if you refer to a method without () after it, such as onChange={this.someEventHandler} , you should bind that method.
Q41 : How does React renderer work exactly when we call setState ?
There are two steps of what we may call render :
Virtual DOM render: when render method is called it returns a new virtual dom structure of the component. This render method is called always when you call setState() , because shouldComponentUpdate always returns true by default. So, by default, there is no optimisation here in React.
Native DOM render: React changes real DOM nodes in your browser only if they were changed in the Virtual DOM and as little as needed - this is that great React's feature which optimizes real DOM mutation and makes React fast.
Q42 : How to avoid the need for binding in React?
There are several common approaches used to avoid methods binding in React:
- Define Your Event Handler as an Inline Arrow Function
- Define Your Event Handler as an Arrow Function Assigned to a Class Field
- Use a Function Component with Hooks
Q43 : What is React Fiber?
React Fiber is an ongoing reimplementation of React's core algorithm. The main difference between react and react fiber are these new features :-
Incremental Rendering :- React v16.0 includes a completely rewritten server renderer. It’s really fast. It supports streaming, so you can start sending bytes to the client faster
Handle errors in the render API : To make class component an error boundary we define a new lifecycle method called componentDidCatch(error, info).
Return multiple elements from render : With this new feature in React v16.0 now we can also return an array of elements, and string from component’s render method.
Portals : Portals provide a first-class way to render children into a DOM node that exists outside the DOM hierarchy of the parent component.
Fragments : A common pattern in React is for a component to return multiple elements. Fragments let you group a list of children without adding extra nodes to the DOM.
Q44 : What is a Pure Function ?
A Pure function is a function that doesn't depend on and doesn't modify the states of variables out of its scope . Essentially, this means that a pure function will always return the same result given same parameters.
Q45 : What is the key architectural difference between a JavaScript library such as React and a JavaScript framework such as Angular?
React enables developers to render a user interface. To create a full front-end application, developers need other pieces, such as state management tools like Redux.
Like React, Angular enables developers to render a user interface, but it is a “batteries included” framework that includes prescriptive, opinionated solutions to common requirements like state management.
While there are many other considerations when comparing React and Angular specifically, this key architectural difference means that:
- Using a library such as React can give a project a greater ability to evolve parts of the system—again for example, state management—over time, when new solutions are created by the open source community.
- Using a framework such as Angular can make it easier for developers to get started and can also simplify maintenance.
Rust has been Stack Overflow’s most loved language for four years in a row and emerged as a compelling language choice for both backend and system developers, offering a unique combination of memory safety, performance, concurrency without Data races...
Clean Architecture provides a clear and modular structure for building software systems, separating business rules from implementation details. It promotes maintainability by allowing for easier updates and changes to specific components without affe...
Azure Service Bus is a crucial component for Azure cloud developers as it provides reliable and scalable messaging capabilities. It enables decoupled communication between different components of a distributed system, promoting flexibility and resili...
FullStack.Cafe is a biggest hand-picked collection of top Full-Stack, Coding, Data Structures & System Design Interview Questions to land 6-figure job offer in no time.
Coded with 🧡 using React in Australia 🇦🇺
by @aershov24 , Full Stack Cafe Pty Ltd 🤙, 2018-2023
Privacy • Terms of Service • Guest Posts • Contacts • MLStack.Cafe

Tutorial Playlist
Reactjs tutorial: a step-by-step guide to learn react, the best guide to know what is react, a beginners guide to react props, reactjs state: setstate, props and state explained, reactjs components: type, nesting, and lifecycle, react with redux tutorial: learn the basics, flutter vs. react native : which one to choose in 2024, top 40 reactjs interview questions and answers for 2024, the best guide to understanding react and express, introduction to typescript with react: the most informative guide, all you need to know about how to create a facebook clone using react, how to create a youtube clone using react, all you need to know about react 18: new features and improvements.
Lesson 7 of 12 By Taha Sufiyan
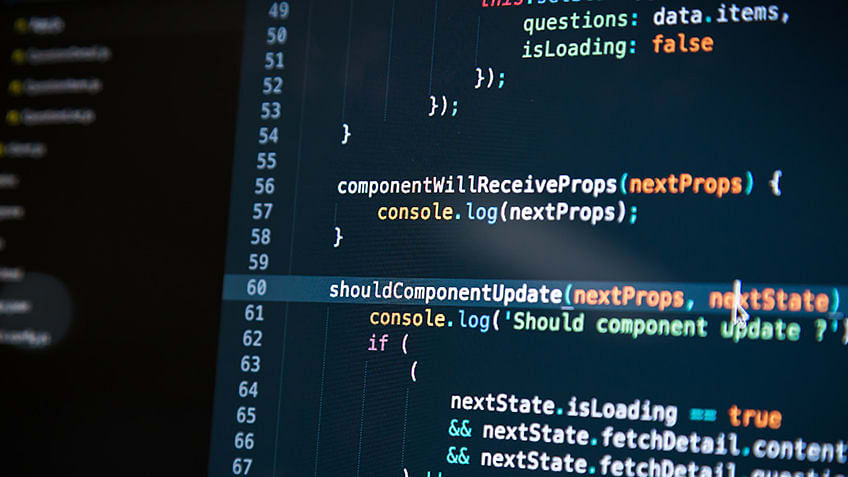
Table of Contents
React is quite the buzzword in the industry these days. As of now, React is the most popular front-end technology that more and more companies are using, and if you are preparing for a job interview , this is ReactJS interview questions tutorial is just the right one for you. Here's a comprehensive list of all the common ReactJS interview questions from basic to advanced levels that are frequently asked in interviews.
Most Asked ReactJS Interview Questions
- What is ReactJS?
- Why ReactJS is Used?
- How Does ReactJS work?
- What are the features of ReactJS?
- What is JSX?
- How to create components in ReactJS?
- What are the advantages of ReactJS?
- Differentiate between real DOM and virtual DOM?
- What are forms in ReactJS?
- How is React different from React Native?
Basic Level - ReactJS Interview Questions
Here are some React Interview Questions on basic concepts.
1. What are the features of React?
Want a top software development job start here.
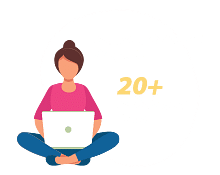
2. What is JSX?
JSX is a syntax extension of JavaScript. It is used with React to describe what the user interface should look like. By using JSX, we can write HTML structures in the same file that contains JavaScript code.
3. Can web browsers read JSX directly?
- Web browsers cannot read JSX directly. This is because they are built to only read regular JS objects and JSX is not a regular JavaScript object
- For a web browser to read a JSX file, the file needs to be transformed into a regular JavaScript object. For this, we use Babel
4. What is the virtual DOM?
DOM stands for Document Object Model. The DOM represents an HTML document with a logical tree structure. Each branch of the tree ends in a node, and each node contains objects.
React keeps a lightweight representation of the real DOM in the memory, and that is known as the virtual DOM. When the state of an object changes, the virtual DOM changes only that object in the real DOM, rather than updating all the objects. The following are some of the most frequently asked react interview questions.
5. Why use React instead of other frameworks, like Angular?
6. what is the difference between the es6 and es5 standards.
This is one of the most frequently asked react interview questions.
These are the few instances where ES6 syntax has changed from ES5 syntax:
Components and Function
- exports vs export
- require vs import
7. How do you create a React app?
These are the steps for creating a React app:
- Install NodeJS on the computer because we need npm to install the React library. Npm is the node package manager that contains many JavaScript libraries, including React.
- Install the create-react-app package using the command prompt or terminal.
- Install a text editor of your choice, like VS Code or Sublime Text.
8. What is an event in React?
An event is an action that a user or system may trigger, such as pressing a key, a mouse click, etc.
- React events are named using camelCase, rather than lowercase in HTML.
- With JSX, you pass a function as the event handler, rather than a string in HTML.
9. How do you create an event in React?
A React event can be created by doing the following:
10. What are synthetic events in React?
- Synthetic events combine the response of different browser's native events into one API, ensuring that the events are consistent across different browsers.
- The application is consistent regardless of the browser it is running in. Here, preventDefault is a synthetic event.
11. Explain how lists work in React
- We create lists in React as we do in regular JavaScript. Lists display data in an ordered format
- The traversal of lists is done using the map() function
12. Why is there a need for using keys in Lists?
Keys are very important in lists for the following reasons:
- A key is a unique identifier and it is used to identify which items have changed, been updated or deleted from the lists
- It also helps to determine which components need to be re-rendered instead of re-rendering all the components every time. Therefore, it increases performance, as only the updated components are re-rendered
13. What are forms in React?
React employs forms to enable users to interact with web applications.
- Using forms, users can interact with the application and enter the required information whenever needed. Form contain certain elements, such as text fields, buttons, checkboxes, radio buttons, etc
- Forms are used for many different tasks such as user authentication, searching, filtering, indexing, etc
Preparing Your Blockchain Career for 2024
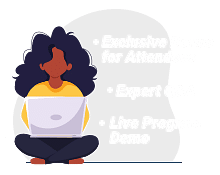
14. How do you create forms in React?
We create forms in React by doing the following:
The above code will yield an input field with the label Name and a submit button. It will also alert the user when the submit button is pressed.
15. How do you write comments in React?
There are basically two ways in which we can write comments:
- Single-line comments
- Multi-line comments
16. What is an arrow function and how is it used in React?
- An arrow function is a short way of writing a function to React.
- It is unnecessary to bind ‘this’ inside the constructor when using an arrow function. This prevents bugs caused by the use of ‘this’ in React callbacks.
Kickstart Your UI/UX Career Right Here!
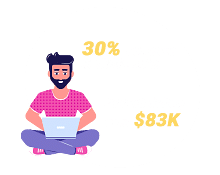
17. How is React different from React Native?
18. how is react different from angular.
In case you have any doubts about these Basic React interview questions and answers, please leave your questions in the comment section below.
ReactJS Interview Questions on Components
Here are some React Interview Questions on components.
19. What are the components in React?
Components are the building blocks of any React application, and a single app usually consists of multiple components. A component is essentially a piece of the user interface. It splits the user interface into independent, reusable parts that can be processed separately.
There are two types of components in React:
- Functional Components: These types of components have no state of their own and only contain render methods, and therefore are also called stateless components . They may derive data from other components as props (properties).
- Class Components: These types of components can hold and manage their own state and have a separate render method to return JSX on the screen. They are also called Stateful components as they can have a state.
20. What is the use of render() in React?
- It is required for each component to have a render() function. This function returns the HTML, which is to be displayed in the component.
- If you need to render more than one element, all of the elements must be inside one parent tag like <div>, <form>.
21. What is a state in React?
- The state is a built-in React object that is used to contain data or information about the component. The state in a component can change over time, and whenever it changes, the component re-renders.
- The change in state can happen as a response to user action or system-generated events. It determines the behavior of the component and how it will render.
22. How do you implement state in React?
23. How do you update the state of a component?
We can update the state of a component by using the built-in ‘setState()’ method:
24. What are props in React?
- Props are short for Properties. It is a React built-in object that stores the value of attributes of a tag and works similarly to HTML attributes.
- Props provide a way to pass data from one component to another component. Props are passed to the component in the same way as arguments are passed in a function.
25. How do you pass props between components?
26. What are the differences between state and props?
27. what is a higher-order component in react.
A higher-order component acts as a container for other components. This helps to keep components simple and enables re-usability. They are generally used when multiple components have to use a common logic.
28. How can you embed two or more components into one?
We can embed two or more components into one using this method:
29. What are the differences between class and functional components?
- Class components example:
- Functional components example:
30. Explain the lifecycle methods of components.
- getInitialState(): This is executed before the creation of the component.
- componentDidMount(): Is executed when the component gets rendered and placed on the DOM.
- shouldComponentUpdate(): Is invoked when a component determines changes to the DOM and returns a “true” or “false” value based on certain conditions.
- componentDidUpdate(): Is invoked immediately after rendering takes place.
- componentWillUnmount(): Is invoked immediately before a component is destroyed and unmounted permanently.
So far, if you have any doubts about the above React interview questions and answers, please ask your questions in the section below.
ReactJS Redux Interview Questions
Here are some ReactJS Interview Questions on the ReactJS Redux concept.
31. What is Redux?
Redux is an open-source, JavaScript library used to manage the application state. React uses Redux to build the user interface. It is a predictable state container for JavaScript applications and is used for the entire application’s state management.
32. What are the components of Redux?
- Store: Holds the state of the application.
- Action: The source information for the store.
- Reducer: Specifies how the application's state changes in response to actions sent to the store.
33. What is the Flux?
- Flux is the application architecture that Facebook uses for building web applications. It is a method of handling complex data inside a client-side application and manages how data flows in a React application.
- There is a single source of data (the store) and triggering certain actions is the only way way to update them.The actions call the dispatcher, and then the store is triggered and updated with their own data accordingly.
- When a dispatch has been triggered, and the store updates, it will emit a change event that the views can rerender accordingly.
34. How is Redux different from Flux?
So far, if you have any doubts about these React interview questions and answers, please leave your questions in the section below.
ReactJS Router Questions
Here are some ReactJS Interview Questions on React Router concepts.
35. What is React Router?
React Router is a routing library built on top of React, which is used to create routes in a React application. This is one of the most frequently asked react interview questions.
36. Why do we need to React Router?
- It maintains consistent structure and behavior and is used to develop single-page web applications.
- Enables multiple views in a single application by defining multiple routes in the React application.
37. How is React routing different from conventional routing?
38. how do you implement react routing.
We can implement routing in our React application using this method:
Considering we have the components App , About , and Contact in our application:
Hope you have no doubts about this ReactJS interview questions article, in case of any difficulty, please leave your problems in the section below.
ReactJS Styling Questions
Here are some ReactJS Interview Questions on Styling concept ReactJS.
39. How do you style React components?
There are several ways in which we can style React components:
- Inline Styling
- JavaScript Object
- CSS Stylesheet
40. Explain the use of CSS modules in React.
- The CSS module file is created with the .module.css extension
- The CSS inside a module file is available only for the component that imported it, so there are no naming conflicts while styling the components.
These are all the basic to advanced ReactJS interview questions that are frequently asked in interviews. We hope these ReactJS interview questions will be helpful in clearing your interview round. All the best for your upcoming job interview! Suppose you want to learn more about ReactJS components, I suggest you click here!
Choose The Right Software Development Program
This table compares various courses offered by Simplilearn, based on several key features and details. The table provides an overview of the courses' duration, skills you will learn, additional benefits, among other important factors, to help learners make an informed decision about which course best suits their needs.
Program Name Full Stack Java Developer Automation Testing Masters Program Geo IN All University Simplilearn Simplilearn Course Duration 6 Months 11 Months Coding Experience Required Basic Knowledge Basic Knowledge Skills You Will Learn 15+ Skills Including Core Java, SQL, AWS, ReactJS, etc. Java, AWS, API Testing, TDD, etc. Additional Benefits Interview Preparation Exclusive Job Portal 200+ Hiring Partners Structured Guidance Learn From Experts Hands-on Training Cost $$ $$ Explore Program Explore Program
Hope this article was able to give you a better understanding about the different ReactJS interview questions that can be asked in an interview and help you prepare for it better. If you are looking to enhance your software development skills further, we would highly recommend you to check Simplilearn's Full Stack Java Developer Course . This program can help you hone the right skills and make you job-ready in no time.
Preparing for a ReactJS interview involves not only understanding the intricacies of this popular JavaScript library but also ensuring that your user interface (UI) design skills are up to par. ReactJS is widely used for building dynamic and interactive user interfaces, making a strong grasp of UI design principles crucial for creating engaging web applications.
If you have any questions or queries, feel free to post them in the comments section below. Our team will get back to you at the earliest.
About the Author
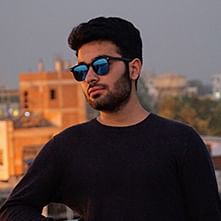
Taha is a Research Analyst at Simplilearn. He is passionate about building great user interfaces and keeps himself updated on the world of Artificial Intelligence. Taha is also interested in gaming and photography.
Recommended Programs
Full Stack Java Developer
Full Stack Web Developer - MEAN Stack
Automation Testing Masters Program
*Lifetime access to high-quality, self-paced e-learning content.
Recommended Resources

Implementing Stacks in Data Structures
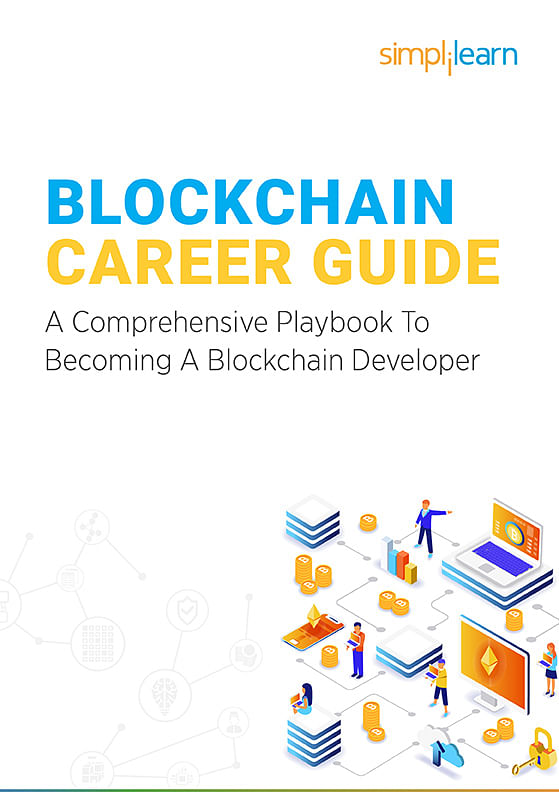
Blockchain Career Guide: A Comprehensive Playbook To Becoming A Blockchain Developer
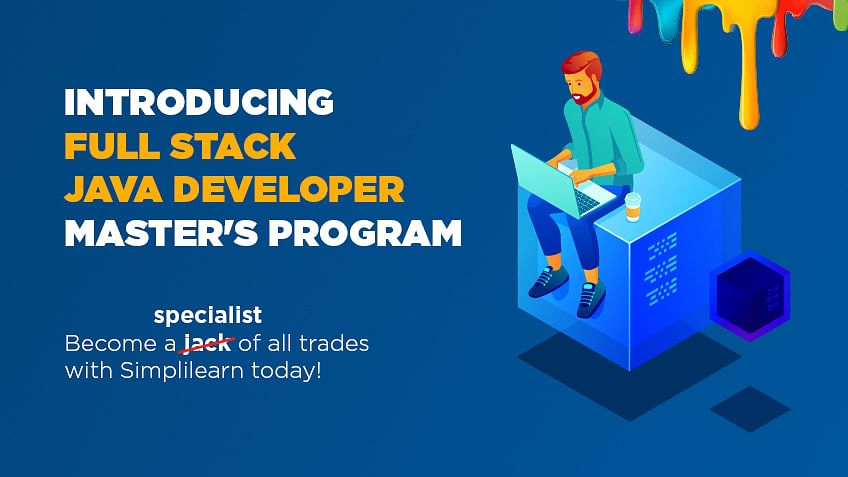
Introducing Simplilearn’s Full Stack Java Developer Master’s Program
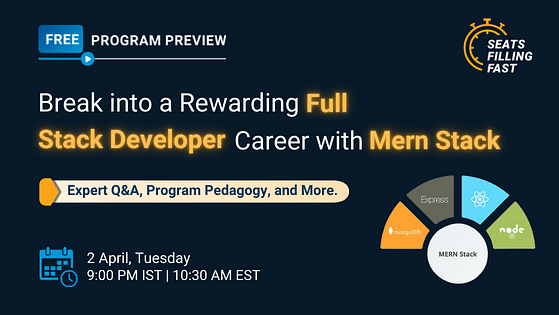
Break into a Rewarding Full Stack Developer Career with Mern Stack
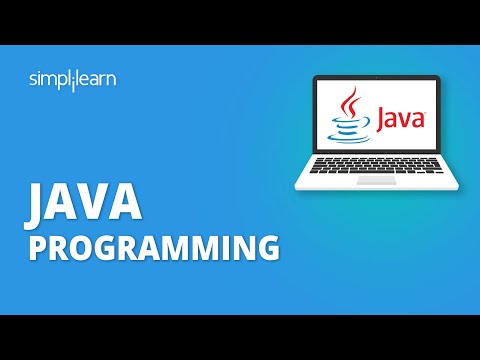
Java Programming: The Complete Reference You Need
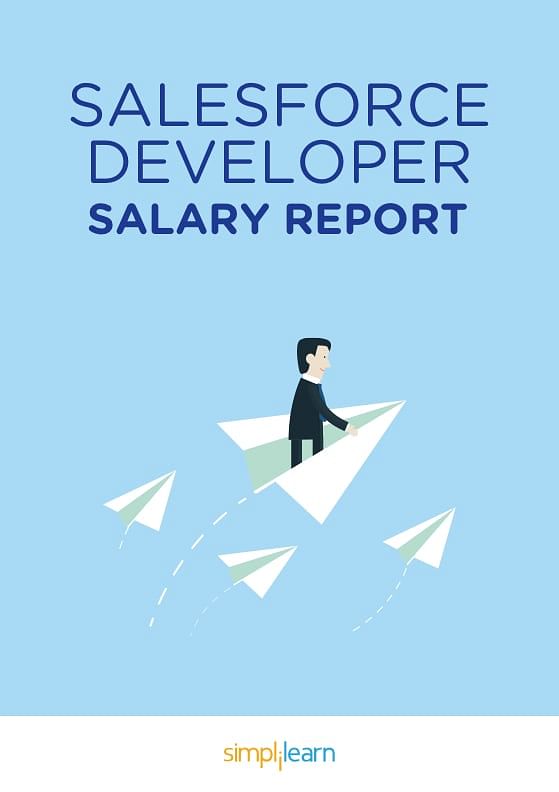
Free eBook: Salesforce Developer Salary Report
- PMP, PMI, PMBOK, CAPM, PgMP, PfMP, ACP, PBA, RMP, SP, and OPM3 are registered marks of the Project Management Institute, Inc.
Real-World React Coding Challenges For Your Interview Preparation
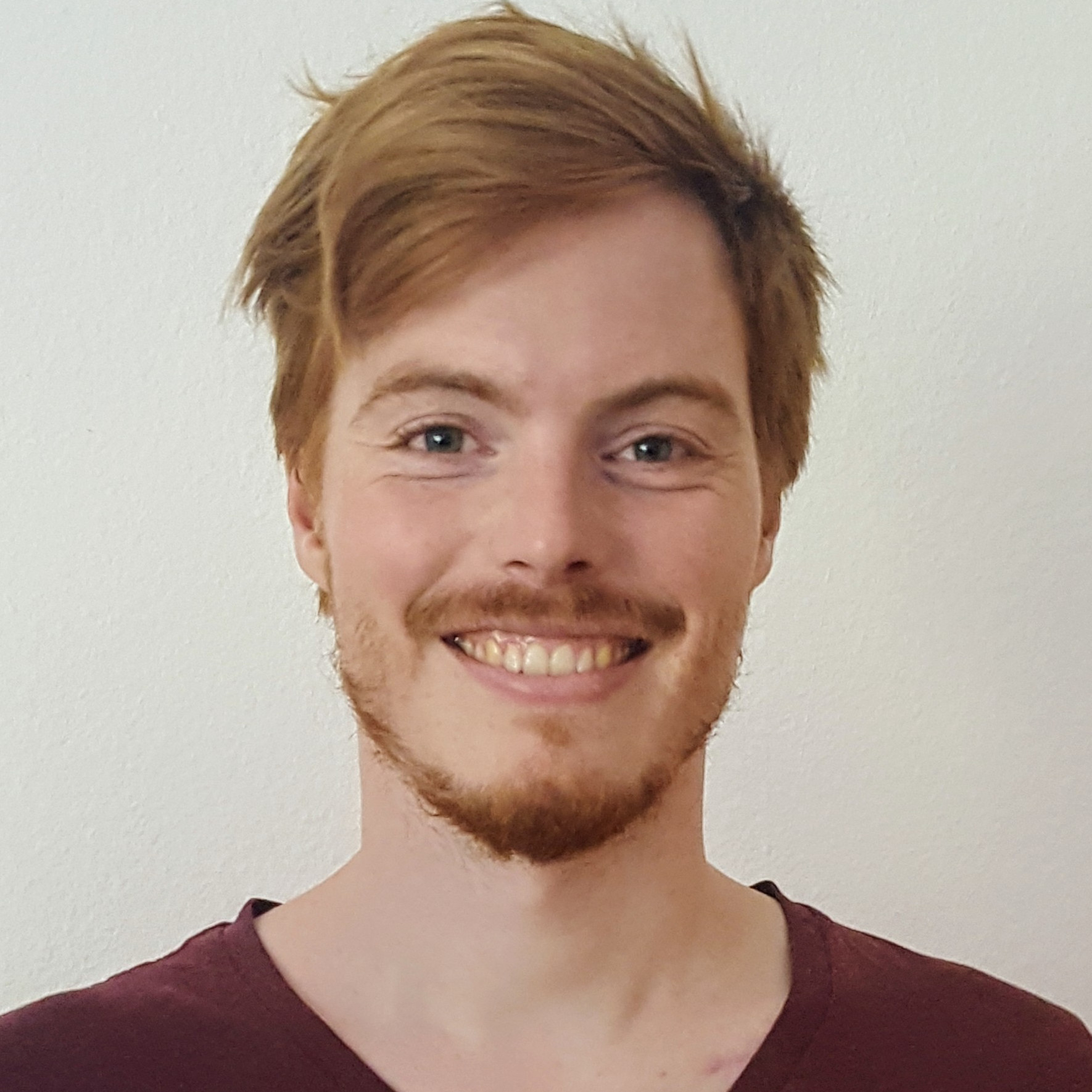
Navigating coding challenges is a crucial part of the interview process, particularly for aspiring React developers lacking professional experience.
Unfortunately, these coding assignments can be daunting for entry-level devs with limited job opportunities, making a failed test disheartening and even humiliating.
To help you prepare for code challenges, this article offers:
- 4 practical React coding challenges covering skills like CSS, simple state management, API data handling, and debugging existing codebases.
- Insight into employers' objectives for coding challenges.
- Guidance on tackling coding challenges during interviews.
React Code Challenges
Before diving in, it's recommended to read the entire article. However, let's kick off with the most exciting part: coding challenges you might encounter during a job interview or in your daily work as a developer.
These challenges stem from the codebase and designs of the React Job Simulator ( source code on GitHub ). They vary in difficulty and assess a range of skills.
Now, let's begin with an easy task.
1. Style A Header Based on Designs
This simple UI challenge involves creating a header component for the app's landing page using Figma designs.
Skills tested : CSS
Difficulty: Easy
- Implement the header according to designs.
- Navigation links should target / , /products , /documentation , and /pricing .
- Adjust the existing "Open Dashboard" link to match the designs.
- Edit pages/index.jsx in the repository.
- styled-components is used for CSS; you may use this or alternatives like CSS modules or inline styles.
- The project is in TypeScript, but the challenge file is JSX. Feel free to change to .tsx if preferred.
- For help with designs or achieving pixel-perfect results, provide your email below for additional resources.
The solution for this task is not the cleanest, but it's sufficient for this challenge without separating the header into a new component file, using TypeScript, or writing tests.
You can get a more elaborate refactoring of above solution by providing your email below.
- a production-grade code base
- realistic tasks & workflows
- high-end tooling setup
- professional designs.
2. Toggle A Modal
This challenge is more dynamic, requiring you to create a modal that opens when users click the contact button.
Skills tested : CSS, simple state management, React APIs
Difficulty: Easy - Medium
- Implement the modal UI.
- The "Cancel" button should close the modal, while "Open Email App" should open users' email clients.
- The modal should open upon clicking the contact button.
- Don't use a modal library.
- It's fine to add code to the file mentioned above.
- There are two modal implementation methods: a simple one and a more advanced one. For both solutions, provide your email below.
For a tougher challenge:
- Integrate your code with the existing codebase.
- Add a Cypress test for this challenge to the existing test suite.
- Add a Storybook story for the modal component.
These tasks are beyond the scope of this post. For more, check out React Job Simulator .
3. Render Elements Based on API Data from a CMS
This more advanced challenge involves connecting the website to a Content Management System (CMS) like Contentful or Strapi. Marketers can use a CMS to easily create content without developer assistance.
In this challenge, you'll create a content element controlled by API data.
Skills tested : Working with API data, CSS
Difficulty: Medium
- Fetch data from the endpoint [https://prolog-api.profy.dev/content-page/home](https://prolog-api.profy.dev/content-page/home) , where home is a "slug" identifying the page.
- Render the hero section (highlighted in the design) using that data.
- Ensure the hero section matches the design.
- Fetch data using any method.
- Add code to pages/index.jsx .
- API Swagger documentation is available here . (Provide your email below for more information)
These tasks are beyond this post's scope. For more, check out React Job Simulator .
4. Find The Bug
This challenge focuses on debugging, a crucial skill for developers. A bug from the React Job Simulator is provided for this exercise.
On the application's issue page , either the "Events" or "Users" column displays incorrect data.
Skills tested : Debugging an existing codebase
- Identify the cause of the bug.
- Fix the bug.
- Adopt a structured approach rather than diving headfirst into the codebase. Use your browser's debugging tools instead of randomly probing the code.
The (Lesser Known) Purpose of Coding Challenges in the Interview Process
Practicing coding challenges is essential, but understanding what interviewers are looking for is equally important.
Employers want proof of a candidate's skills to minimize risks associated with hiring and training. Coding challenges are one way to assess these skills, testing both technical and soft skills.
While technical skills are crucial, don't overlook the importance of soft skills. Coding challenges can demonstrate your communication and problem-solving abilities, allowing interviewers to follow your thought process and observe your attitude towards critique.
This is particularly true for live coding challenges or when discussing your take-home assignment in a follow-up interview. Be prepared for questions on both technical and soft skills.
6 Tips for Coding Challenges in Job Interviews
Coding challenges can be stressful, especially live assignments with the interviewer.
Here are some tips on how to approach them:
Ask for Clarification
One common mistake, particularly among juniors, is diving straight into the task without fully understanding the requirements. Instead, take time to re-read the assignment and ask for clarification if something is unclear.
Asking clarifying questions is not a weakness. It demonstrates a clear mind and a structured approach, which employers value. On the job, building a solution without fully understanding the problem can lead to wasted time and effort.
Talk While You're Coding (For Live Challenges)
Live coding challenges can be intimidating but offer a unique opportunity to showcase your thought process. Talking out loud while writing code allows interviewers to see your problem-solving and communication skills in action.
However, thinking and talking simultaneously isn't easy for everyone. To improve, practice techniques like rubber duck debugging , where you explain your plans as you work on a task. The coding challenges mentioned earlier are excellent opportunities for practicing this skill.
Clean Code Format
Many inexperienced developers overlook the importance of clean code formatting. Inconsistencies in indentation, semicolons, or variable naming can be easily noticed by senior developers or interviewers.
To avoid these issues, use a code formatter like Prettier for your (take-home) coding assignments. This helps maintain consistency and demonstrates your attention to detail.
Use TypeScript and Write Tests If You Can
Experienced developers value the reliability provided by TypeScript and automated tests. If you're comfortable with it, use types in your code and add tests. The choice of tool doesn't matter – you can use Jest, React Testing Library, or Cypress. Even one or two tests can be enough to demonstrate your professionalism and commitment to code quality.
Add a README with Clear Instructions
Your interviewers may not run your code on their local machine, but if they do, you want to avoid confusion.
Include clear instructions on how to install and run your code, and double-check that it's working. If you have extra time, consider adding additional information to the README, such as:
- Your approach to solving the assignment and the reasons behind it.
- Any assumptions you made during the process.
- Improvements you would make if given more time.
Be Prepared for Questions
Adding the information mentioned above to your README not only helps your interviewer understand your thought process, but it also prepares you for the next step: discussing your code.
If you have the opportunity (e.g., with a take-home test), let the code rest for a bit after submission. If you're invited to a follow-up interview, review your code closely:
- What seems strange or confusing in hindsight?
- Are there parts that are particularly difficult to understand?
- How could you improve or refactor your code?
- Is there anything you would do differently now?
Reevaluating your code can help you prepare for the next stage of the interview process, as it's likely you'll face questions about your code assignment.
21 Essential React.js Interview Questions *
Toptal sourced essential questions that the best react.js developers and engineers can answer. driven from our community, we encourage experts to submit questions and offer feedback..

Interview Questions
Explain the Virtual DOM, and a pragmatic overview of how React renders it to the DOM.
The Virtual DOM is an interesting concept; it’s a complex idea that boils down into a much simpler algorithm.
In React, if we create simple ES6 class and print it out, we have a function (as all functions can be used as a constructor in JavaScript):
The console.log(Comments) gives us something that looks like this (after compiled by Babel from ES6 to ES5):
When we write something to draw a React Component to the screen, we might have something like the following:
The JSX gets transpiled into ES5 by Babel as well:
We can see that <Comments /> is transpiled directly into React.createElement(Comments, null) . This is where we can see what a Virtual DOM object actually is : a plain JavaScript Object that represents the tag to be rendered onto the screen.
Let’s inspect the output of React.createElement() :
This gives us:
See how the type is a string? DOM.render({...}) gets this object above and looks at the type , and decides whether or not to reuse an existing <div> element on the DOM or create a new <div> and append it.
The Virtual DOM is not a simple Object – it is a recursive structure. For example, if we add two elements beneath the <div/> :
What we get is a nested Object-tree:
This is why, in a React Component’s code, we can access the child and ancestor elements via this.props.children . What React will do is walk down a very deep tree of nested Objects (depending on your UI complexity), each sitting in their parent element’s children .
One thing to note is that the type so far has just been a string. When a React Element is made from a custom Component (like Comments above), the type is a function:
You can play around with a web version of this code at Matthew Keas’ github .
Explain the standard JavaScript toolchain, transpilation (via Babel or other compilers), JSX, and these items’ significance in recent development. What sort of tools might you use in the build steps to optimize the compiled output React code?
The bleeding edge JavaScript toolchain can seem quite complex, and it’s very important to feel confident in the toolchain and to have a mental picture of how the pieces fit together.
There are a couple primary pillars in the JavaScript toolchain: Dependency Management, Linting, Style-checking, Transpilation, and Compilation, Minification, Source-Mapping.
Typically, we use build tools like Gulp, Watchify/Browserify, Broccoli, or Webpack to watch the filesystem for file events (like when you add or edit a file). After this occurs, the build tool is configured to carry out a group of sequential or parallel tasks .
This part is the most complex piece, and is the center of the development process.
The rest of the tools belong in that group of sequential or parallel tasks:
- Style linting - typically a linter like JSCS is used to ensure the source code is following a certain structure and style
- Dependency Management - for JavaScript projects, most people use other packages from npm; some plugins exist for build systems (e.g. Webpack) and compilers (e.g. Babel) that allow automatic installation of packages being import ed or require() ‘d
- Transpilation - a specific sub-genre of compilation, transpilation involves compiling code from one source version to another, only to a similar runtime level (e.g. ES6 to ES5)
- Compilation - specifically separate from transpiling ES6 and JSX to ES5, is the act of including assets, processing CSS files as JSON, or other mechanisms that can load and inject external assets and code into a file. In addition, there are all sorts of build steps that can analyze your code and even optimize it for you.
- Minification and Compression - typically part of – but not exclusively controlled by – compilation, is the act of minifying and compressing a JS file into fewer and/or smaller files
- Source-Mapping - another optional part of compilation is building source maps, which help identify the line in the original source code that corresponds with the line in the output code (i.e. where an error occurred)
For React, there are specific build tool plugins, such as the babel-react-optimize presets that involves compiling code into a format that optimizes React, such as automatically compiling any React.createElement() calls into a JavaScript Object that inlines right into the source code:
- How compilers can help optimize React
- How to bootstrap a modern toolchain with Create React App
- The Next.js and GatsbyJS frameworks built on top of React
How would you create Higher Order Components (HOCs) in React?
Higher Order Components (HOCs) are the coined term for a custom Component that accepts dynamically provided children. For example, let’s make <LazyLoad /> Component that takes child image tags as children , waits until the <LazyLoad /> Component is scrolled into view, and then loads the images they point to in the background (before rendering them to the DOM).
An HOC accepts children via props:
Creating an HOC means handling this.props.children in the Component’s code:
interactive example can be found at https://goo.gl/ns0B6j
Noting a few things about this code:
- We set up initial state ( this.state = {loaded: 0} ) in the constructor() . This will be set to 1 when the parent container is scrolled into view.
- The render() returns the props.children as child elements when this occurs. Extract the src by using ES6 destructuring, where {props:{src}} creates a variable src with the appropriate value.
- We used a single componentDidMount() lifecycle method. This is used because on mount, we’d like the component to check if the HOC is visible.
- The largest function of our component, _scroll() , grabs the HOC Component’s DOM element with DOM.findDOMNode() and then gets the elements position. This position is compared to the height of the browser window, and if it is less than 100px from the bottom, then the scroll listener is removed and loaded is set to 1 .
This technique is called HOC (Higher Order Component) because we pass in elements as this.props.children when we nest those elements inside the container component:
All of these nested elements (which can be custom components) are nested under <HOC/> , thus HOC ’s code will be able to access them as this.props.children .
Apply to Join Toptal's Development Network
and enjoy reliable, steady, remote Freelance React.js Developer Jobs
What is the significance of keys in React?
Keys in React are used to identify unique VDOM Elements with their corresponding data driving the UI; having them helps React optimize rendering by recycling existing DOM elements. Let’s look at an example to portray this.
We have two <TwitterUser> Components being rendered to a page, drawn in decreasing order of followers:
Let’s say that B gets updated with 105 Twitter followers, so the app re-renders, and switches the ordering of A and B:
Without keys, React would primarily re-render both <TwitterUser> Elements in the DOM. It would re-use DOM elements, but React won’t re-order DOM Elements on the screen.
With keys, React would actually re-order the DOM elements, instead of rendering a lot of nested DOM changes. This can serve as a huge performance enhancement, especially if the DOM and VDOM/React Elements being used are costly to render.
Keys themselves should be a unique number or string; so if a React Component is the only child with its key, then React will repurpose the DOM Element represented by that key in future calls to render() .
Let’s demonstrate this with a simple list of todos rendered with React:
Interactive code sample available on Matthew Keas’ github .
The setInterval() occurring on mount reorders the items array in this.state every 20ms. Computationally, if React is reordering the items in state, then it would manipulate the DOM elements themselves instead of “dragging” them around between positions in the <ul> .
It is worth noting here that if you render a homogenous array of children – such as the <li> ’s above – React will actually console.warn() you of the potential issue, giving you a stack trace and line number to debug from. You won’t have to worry about React quietly breaking.
What is the significance of refs in React?
Similarly to keys, refs are added as an attribute to a React.createElement() call, such as <li ref="someName"/> . The ref serves a different purpose, it provides us quick and simple access to the DOM Element represented by a React Element.
Refs can be either a string or a function. Using a string will tell React to automatically store the DOM Element as this.refs[refValue] . For example:
this.refs.someThing inside componentDidUpdate() used to refer to a special identifier that we could use with React.findDOMNode(refObject) – which would provide us with the DOM node that exists on the DOM at this very specific instance in time. Now, React automatically attaches the DOM node to the ref, meaning that this.refs.someThing will directly point to a DOM Element instance.
Additionally, a ref can be a function that takes a single input. This is a more dynamic means for you assign and store the DOM nodes as variables in your code. For example:
[Legacy projects only, < circa 2016] In a general overview, how might React Router and its techniques differ from more traditional JavaScript routers like Backbone’s Router?
“Traditional” routers like the ever-popular Backbone.Router establish a predefined set of routes, in which each route defines a series of actions to take when a route is triggered. When combining Backbone.Router with React, you may have to mount and unmount React Components when the route changes:
The router exists externally of the React Components, and the VDOM has to mount and unmount potentially frequently, introducing a possible slew of problems. React Router focuses on not just “single-level” routing, but enables - nay, empowers - the creation of HOCs that can “decide for themselves” what to render within them.
This is where the advanced HOC implementations can really help simplify a seemingly complex notion. Let’s look at using a tiny router to assess some of the beauty of embedding application routers inside React HOCs. Here, we define a Component that wraps it’s own routing mechanism ( router() not provided here, see universal-utils ):
This Router Component opts for parsing the routes object passed into this.props instead of reading over an array of React Components passed as this.props.children . React Router opts for the latter technique. Need proof? Take a look at this example code provided by React Router’s docs :
A <Router /> Component has one or more <Route /> Components as items in this.props.children , and <Route /> s can have sub- <Route /> s. React Router’s code recursively walks down the tree of children until there are no more to process, allowing the developer to recursively declare routes in a structure that encapsulates sub-routes, instead of having to implement a Backbone-esque flat list of routes (i.e. "/" , "/about" , "/users" , "/users/:id" , etc).
Why do class methods need to be bound to a class instance, and how can you avoid the need for binding?
In JavaScript, the value of this changes depending on the current context. Within React class component methods, developers normally expect this to refer to the current instance of a component, so it is necessary to bind these methods to the instance. Normally this is done in the constructor—for example:
There are several common approaches used to avoid this binding:
1. Define Your Event Handler as an Inline Arrow Function
For example:
Using an arrow function like this works because arrow functions do not have their own this context. Instead, this will refer to the context in which the arrow function was defined—in this case, the current instance of SubmitButton .
2. Define Your Event Handler as an Arrow Function Assigned to a Class Field
Note: As of September 2019, class fields are a Stage 3 ECMAScript proposal and are not yet part of the published ECMAScript specification. However, they are available for use in both Google Chrome and Mozilla Firefox and are commonly used in React projects.
3. Use a Function Component with Hooks
Using the hooks functionality in React it is possible to use state without using this , which simplifies component implementation and unit testing.
Explain the positives and negatives of shallow rendering components in tests.
- It is faster to shallow render a component than to fully render it. When a React project contains a large number of components, this performance difference can have a significant impact on the total time taken for unit tests to execute.
- Shallow rendering prevents testing outside the boundaries of the component being tested—a best practice of unit testing.
- Shallow rendering is less similar to real-world usage of a component as part of an application, so it may not catch certain problems. Take the example of a <House /> component that renders a <LivingRoom /> component. Within a real application, if the <LivingRoom /> component is broken and throws an error, then <House /> would fail to render. However, if the unit tests of <House /> only use shallow rendering, then this issue will not be identified unless <LivingRoom /> is also covered with unit tests.
If you wanted a component to perform an action only once when the component initially rendered—e.g., make a web analytics call—how would you achieve this with a class component? And how would you achieve it with a function component?
Using a Class Component
The componentDidMount() lifecycle hook can be used with class components:
Any actions defined within a componentDidMount() lifecycle hook are called only once when the component is first mounted.
Using a Function Component
The useEffect() hook can be used with function components:
The useEffect() hook is more flexible than the lifecycle methods used for class components. It receives two parameters:
- The first parameter it takes is a callback function to be executed.
- The optional second parameter it takes is an array containing any variables that are to be tracked.
The value passed as the second argument controls when the callback is executed:
- If the second parameter is undefined , the callback is executed every time that the component is rendered.
- If the second parameter contains an array of variables , then the callback will be executed as part of the first render cycle and will be executed again each time an item in the array is modified.
- If the second parameter contains an empty array , the callback will be executed only once as part of the first render cycle. The example above shows how passing an empty array can result in similar behaviour to the componentDidMount() hook within a function component.
What are the most common approaches for styling a React application?
CSS Classes
React allows class names to be specified for a component, like class names are specified for a DOM element in HTML.
When developers first start using React after developing traditional web applications, they often use CSS classes for styling because they are already familiar with the approach.
Styling React elements using inline CSS allows styles to be completely scoped to an element using a well-understood, standard approach. However, there are certain styling features that are not available with inline styles. For example, the styling of :hover pseudo-classes.
Pre-processors Such as Sass, Stylus, and Less
Pre-processors are often used on React projects. This is because, like CSS, they are well understood by developers and are often already in use if React is being integrated into a legacy application.
CSS-in-JS Modules Such as Styled Components, Emotion, and Styled-jsx
CSS-in-JS modules are a popular option for styling React applications because they integrate closely with React components. For example, they allow styles to change based on React props at runtime. Also, by default, most of these systems scope all styles to the respective component being styled.
If you were working on a React application that was rendering a page very slowly, how would you go about investigating and fixing the issue?
If a performance issue such as slow rendering is seen within a React app, the first step is to use the Profiler tool provided within the React Developer Tools browser plugin, which is available for Google Chrome and Mozilla Firefox. The Profiler tool allows developers to find components that take a long time to render or are rendering more frequently than necessary.
One of the most common issues in React applications is when components re-render unnecessarily. There are two tools provided by React that are helpful in these situations:
- React.memo() : This prevents unnecessary re-rendering of function components
- PureComponent : This prevents unnecessary re-rendering of class components
Both of these tools rely on a shallow comparison of the props passed into the component—if the props have not changed, then the component will not re-render. While both tools are very useful, the shallow comparison brings with it an additional performance penalty, so both can have a negative performance impact if used incorrectly. By using the React Profiler, performance can be measured before and after using these tools to ensure that performance is actually improved by making a given change.
At a high level, what is the virtual DOM (VDOM) and how does React use it to render to the DOM?
The VDOM is a programming concept, providing a critical part of the React architecture. Rather than interacting directly with the DOM, changes are instead first rendered to the VDOM—a lightweight representation of the target state of the DOM.
Changes made to the VDOM are batched together to avoid unnecessary frequent changes to the DOM. Each time these batched changes are persisted to the DOM, React creates a diff between the current representation and the previous representation persisted to the DOM, then applies the diff to the DOM.
This abstraction layer for the DOM provides a simple interface for developers while allowing React to update the DOM in an efficient and performant manner.
What is prop drilling and how can you avoid it?
When building a React application, there is often the need for a deeply nested component to use data provided by another component that is much higher in the hierarchy.
Consider the following example components:
- <EditUsersPage /> , which includes selectedUserAddress in its component state and renders a <User /> component
- <User /> , which renders a <UserDetails /> component
- <UserDetails /> , which renders a <UserAddress /> component
- A <UserAddress /> component that requires the selectedUserAddress property stored in the <EditUsersPage /> state
The simplest approach is to simply pass a selectedUserAddress prop from each component to the next in the hierarchy from the source component to the deeply nested component. This is called prop drilling.
The primary disadvantage of prop drilling is that components that should not otherwise be aware of the data—in this case <User /> and <UserDetails /> —become unnecessarily complicated and are harder to maintain.
To avoid prop drilling, a common approach is to use React context. This allows a Provider component that supplies data to be defined, and allows nested components to consume context data via either a Consumer component or a useContext hook.
While context can be used directly for sharing global state, it is also possible to use context indirectly via a state management module, such as Redux.
What is the StrictMode component and why would you use it?
<StrictMode /> is a component included with React to provide additional visibility of potential issues in components. If the application is running in development mode, any issues are logged to the development console, but these warnings are not shown if the application is running in production mode.
Developers use <StrictMode /> to find problems such as deprecated lifecycle methods and legacy patterns, to ensure that all React components follow current best practices.
<StrictMode /> can be applied at any level of an application component hierarchy, which allows it to be adopted incrementally within a codebase.
What is the key architectural difference between a JavaScript library such as React and a JavaScript framework such as Angular? How would that impact the decision for a project to use one versus the other?
React enables developers to render a user interface. To create a full front-end application, developers need other pieces, such as state management tools like Redux.
Like React, Angular enables developers to render a user interface, but it is a “batteries included” framework that includes prescriptive, opinionated solutions to common requirements like state management.
While there are many other considerations when comparing React and Angular specifically, this key architectural difference means that:
- Using a library such as React can give a project a greater ability to evolve parts of the system—again for example, state management—over time, when new solutions are created by the open source community.
- Using a framework such as Angular can make it easier for developers to get started and can also simplify maintenance.
How can automated tooling be used to improve the accessibility of a React application?
There are two main categories of automated tools that can be used to identify accessibility issues:
Static Analysis Tools
Linting tools like ESLint can be used with plugins such as eslint-plugin-jsx-a11y to analyse React projects at a component level. Static analysis tools run very quickly, so they bring a good benefit at a low cost.
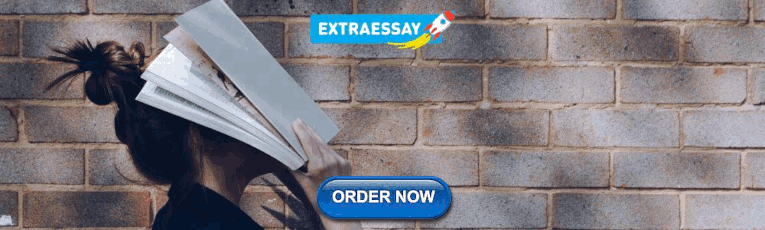
Browser Tools
Browser accessibility tools such as aXe and Google Lighthouse perform automated accessibility at the app level. This can discover more real-world issues, because a browser is used to simulate the way that a user interacts with a website. It is possible for many of these tools to run in a continuous integration environment such as Travis or Jenkins. Since these tools take longer to execute, many developers just run these tools within their local browser on an occasional basis, such as when reaching project milestones.
[Legacy projects only: React < 16.8] What are pure functional Components?
Traditional React Components as we have seen thus far are creating a class with class Example extends React.Component or React.createClass() . These create stateful components if we ever set the state (i.e. this.setState() , getInitialState() , or this.state = {} inside a constructor() ).
If we have no intention for a Component to need state, or to need lifecycle methods, we can actually write Components with a pure function, hence the term “pure functional Component”:
This function that returns a React Element can be used whereever we see fit:
You might notice that <Date/> also takes a prop – we can still pass information into the Component.
How might React handle or restrict Props to certain types, or require certain Props to exist?
You may recall a previous example that looked like the following (some parts of the code left out):
When rendering the <LazyLoad/> , we can pass in props (i.e. <LazyLoad top={0}/> ). Props are essentially inputs or values being passed down to one Component from the parent rendering context, and the code that passes the props to the element may not be compliant with your code. For example, top here seems to be just a number, but would I be able to verify that the prop is in-fact a number before my component is rendered? It’s certainly possible to write this code in each and every Component that uses props . However, React provides us a much simpler and shorter solution: Prop Types.
When using React’s non-minified development version (i.e. when building and testing in development), React will throw an error to alert you of any instances where a Prop is either missing or the wrong type. Above, top should always be a number .
We can make top a required prop by adding:
PropTypes can be used to test Props for any kind of value . Here’s a few quick type-checkers React has for JavaScript’s built-in types:
- React.PropTypes.array ,
- React.PropTypes.bool ,
- React.PropTypes.func ,
- React.PropTypes.number ,
- React.PropTypes.object ,
- React.PropTypes.string ,
- React.PropTypes.symbol ,
We can also test that props are React and DOM types:
- React.PropTypes.node ,
- React.PropTypes.element ,
And we have the ability to test more complex types, such as “shapes”, “instances of”, or “collections of”:
- React.PropTypes.instanceOf(Message) ,
- React.PropTypes.oneOf(['News', 'Photos']) ,
- React.PropTypes.oneOfType([ React.PropTypes.string, React.PropTypes.number, React.PropTypes.instanceOf(Message)])
- React.PropTypes.arrayOf(React.PropTypes.number) ,
- React.PropTypes.shape({ color: React.PropTypes.string, fontSize: React.PropTypes.number })
Use these PropTypes to produce errors and track down bugs. When used effectively, PropTypes will prevent your team from losing too much time in the debugging and documentation process, ensuring stricter standards and understanding of your growing library of Components.
[Legacy projects only: React < 15.5] Compare and contrast creating React Components in ES5 and ES2015 (also known as ES6). What are the advantages and disadvantages of using one or the other? Include notes about default props, initial state, PropTypes, and DisplayName.
Creating React Components the ES5 way involves using the React.createClass() method:
This Comments Component can now be rendered either inside another React Component or directly in the call to ReactDOM.render() :
ES5 Components have some particular qualities, which we’ll note:
- Like the above example, to set the state to an initial value, create the getInitialState() function on the Component. What it returns will be the initial state for a Component when rendered.
- Additionally, you can set the default props for the component to have a certain value with the getDefaultProps() method on the ES5 version.
- The displayName is used in debugging and error reporting by React. If you use JSX, then the displayName is automatically filled out.
- For some, it is common practice to denote a custom method added to a React Component by prefixing it with an underscore, hence _handleClick . _handleClick is passed as the onClick callback for a button in the code above. We can’t do this so easily in the ES6 API of React, because the ES5 version has autobinding , but the ES6 does not. Let’s take a look at what autobinding provides:
Auto-binding
Consider the following piece of code:
Invoking thing.speak() in the console will log "jen" , but pressing a key will log undefined because the context of the callback is the global object. The browser’s global object – window – becomes this inside the speak() function, so this.name becomes window.name , which is undefined .
React in ES5 automatically does autobinding, effectively doing the following:
Autobinding automatically binds our functions to the React Component instance so that passing the function by reference in the render() works seamlessly.
Creating React Components the ES6 way works a little differently, favoring the ES6 class ... extends ... syntax, and no autobinding feature:
- Notice that in ES6, we have a constructor() that we use to set the initial state,
- We can add default props and a display name as properties of the new class created, and
- The render() method, which works as normal, but we’ve had to alter how we pass in the callback function. This current approach ( <button onClick={() => this._handleClick}>click me!</button> ) will create a new function each time the component is re-rendered; so if it becomes a performance bottleneck, you can always bind manually and store the callback:
Or with class fields syntax:
Many React utility libraries on npm provide a single function to bind all handlers in the constructor, just like React does.
[Legacy projects only: React < 15.5] Compare and contrast incorporating mixins and enforcing modularity in React Components. ( extend , createClass and mixins, HOCs) Why would you use these techniques, and what are the drawbacks of each?
Modularity is – in effect – something partially done with intention while coding, and partially done when refactoring afterwards.
Let’s first paint a scenario which we’ll model using each method above. Imagine we have three React Components: onScrollable , Loadable , and Loggable .
- an onScrollable Component will listen to the window.onscroll event, and use a logging mechanism to record it
- a Loadable Component will not render until one or more async requests have finished loading, and will use a logging mechanism to record when this occurs
- a Loggable Component provides a logging mechanism, be it a console , a Winston Node.js logging setup on our own server, or some 3rd party logging service that records logs via JSON requests
First, let’s model this with React’s ES5 API and mixins .
Interactive code sample at Matthew Keas’ github .
Let’s note a few things about the above code:
- There are three POJOs (Plain ol’ JS Objects) created, which hold lifecycle and/or custom methods.
- When creating the Example Component, we add mixins: [Loggable, Loadable, onKeypress] , meaning that any functions from all three objects are included in the Example class.
- Both onKeypress and Loadable add a componentDidMount() , but this doesn’t mean the latter cancels out the prior. In fact, all componentDidMount() functions from each mixin will be invoked when the event occurs. The same is true for all lifecycle methods added to mixins. This way, both the onKeypress and Loadable mixins will work simultaneously!
Mixins are possible, but not built-in to React’s ES6 API. However, the ES6 API makes it easier to create a custom Component that extends another custom Component.
So our Components’ prototype chains would look like the following:
This would result from Components written as such:
Creating anonymous classes would help here, because then Loggable would not have to extend Loadable and onKeypress.
With a mixin() function, this could look more like:
Let’s try to write mixin() by building a chain of anonymous classes that extend Loggable , Loadable , and onKeypress :
There’s a caveat, though – if Loadable extends onKeypress and both implement componentDidMount() , Loadable ’s version will be lower on the prototype chain, which means the function from onKeypress will never be invoked.
The takeaway here is that the mixin pattern isn’t easily implemented by relying only on the ES6 extends approach. Let’s try to implement mixin() again, but build a more robust function:
This new mixin() implementation maps over each class, and cascades function calls from a parent class’s componentDidMount() alongside the child’s componentDidMount() .
There are similar implementations of mixin() available on npm, using packages like react-mixin and es6-react-mixins .
We use mixin() from above like so:
interactive code sample available at https://goo.gl/VnQ21R
Recently, React provided support for – and documented its preference of – React Components declared with ES6 classes. ES6 classes allow us to create component heirarchies with less code, however this makes it more difficult to create a single Component that inherits properties from several mixins, instead forcing us to create prototype chains.
[Legacy projects only: React < 16] Compare and contrast the various React Component lifecycle methods. How might understanding these help build certain interfaces/features?
There are several React lifecycle methods that help us manage the asynchronous and non-determinate nature of a Component during it’s lifetime in an app – we need provided methods to help us handle when a component is created, rendered, updates, or removed from the DOM.
Let’s first classify and define the life-cycle methods:
The “Will’s” - invoked right before the event represented occurs.
- componentWillMount() - Invoked once, both on the client and server, immediately before the initial rendering occurs. If you call setState within this method, render() will see the updated state and will be executed only once despite the state change.
- componentWillReceiveProps(object nextProps) - Invoked when a component is receiving new props. This method is not called for the initial render. Calling this.setState() within this function will not trigger an additional render. One common mistake is for code executed during this lifecycle method to assume that props have changed.
- componentWillUnmount() - Invoked immediately before a component is unmounted from the DOM. Perform any necessary cleanup in this method, such as invalidating timers or cleaning up any DOM elements that were created in componentDidMount.
- componentWillUpdate(object nextProps, object nextState) - Invoked immediately before rendering when new props or state are being received. This method is not called for the initial render.
The “Did’s”
- componentDidMount() - Invoked once, only on the client (not on the server), immediately after the initial rendering occurs. At this point in the lifecycle, you can access any refs to your children (e.g., to access the underlying DOM representation). The componentDidMount() method of child components is invoked before that of the parent component.
- componentDidUpdate(object prevProps, object prevState) - Invoked immediately after the component’s updates are flushed to the DOM. This method is not called for the initial render. Use this as an opportunity to operate on the DOM when the component has been updated.
The “Should’s”
- shouldComponentUpdate(object nextState, object nextProps) - Invoked before rendering when new props or state are being received. This method is not called for the initial render or when forceUpdate() is used. Use this as an opportunity to return false when you’re certain that the transition to the new props and state will not require a component update.
Having a strong understanding of how these fit together – and how setState() or forceUpdate() affect the lifecycle – will help the conscious React developer build robust UIs.
There is more to interviewing than tricky technical questions, so these are intended merely as a guide. Not every “A” candidate worth hiring will be able to answer them all, nor does answering them all guarantee an “A” candidate. At the end of the day, hiring remains an art, a science — and a lot of work .
Tired of interviewing candidates? Not sure what to ask to get you a top hire?
Let Toptal find the best people for you.
Our Exclusive Network of React.js Developers
Looking to land a job as a React.js Developer?
Let Toptal find the right job for you.
Job Opportunities From Our Network
Submit an interview question
Submitted questions and answers are subject to review and editing, and may or may not be selected for posting, at the sole discretion of Toptal, LLC.
Looking for React.js Developers?
Looking for React.js Developers ? Check out Toptal’s React.js developers.
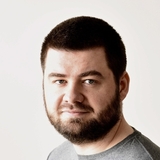
Sergii Petryk
Sergii is a senior full-stack developer with over 10 years of experience in software development across various industries, with the five most recent years focused on React and TypeScript. He is well-respected among industry-leading companies as a skilled developer who truly understands the client infrastructure and pain points. Sergii also has the hands-on technical aptitude needed to design and build solutions.
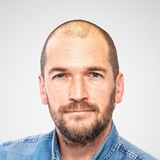
Matt Reynolds
Matt is a seasoned full-stack software engineer with nearly 10 years of experience in web development, most recently with a successful eCommerce startup. He specializes in Next.js, React, and React Native web, mobile, and TV apps. Matt has also worked with major global media companies on APIs and database integrations for SaaS applications.
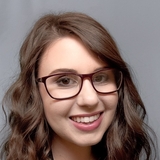
Maria Szubski
Maria is a front-end developer specializing in React and GraphQL. She has five years of experience building B2C and B2B sites and three years mentoring junior developers through local tech workshops. She has also contributed as a solo/lead developer and as part of large dev teams across various technologies. Maria's UX design background helps her quickly translate mockups into responsive UIs, delivering best practices in accessibility, user experience, and maintainable code standards.
Toptal Connects the Top 3% of Freelance Talent All Over The World.
Join the Toptal community.
React Interview Questions – Interview Prep with Answers and Examples

React is a JavaScript library for creating user interfaces. It's used in over 30,000 live websites and has over 70,000 GitHub stars.
According to the 2021 Stack Overflow developer survey , React has surpassed jQuery as the most popular web framework, and holds approximately 40.14% of the market share. React was also the most sought-after, with one out of every four developers using it.
Over 8,000 industry leaders, including LinkedIn, Twitter, and AirBnB, use React.
A React developer's average annual salary in the United States is $120,000. Many businesses and companies use React, which forces them to constantly look for new talent.
In this article, we will go over some React basics and then look at some interview questions and their proper answers to help you succeed in any React interview you may have.
What is React?
React is an open-source front-end JavaScript library for creating user interfaces. It is declarative, efficient, and flexible, and it adheres to the component-based approach, which allows us to create reusable UI components.
Developer primarily use React to create Single Page Applications and the library focuses solely on the view layer of MVC. It is also extremely fast.
Features of React
React has many features that set it apart, but here are a few highlights:
- React employs the Virtual DOM as opposed to a real/browser DOM.
- React uses unidirectional one-way data binding.
- It is used to develop web applications as well as mobile applications using React Native , which allows us to build cross-platform applications.
How to Start a New Project in React
We can create a React app from scratch by initalizing a project and installing all dependencies. But the easiest way is by using create-react-app via the command below:
Note: my-app is the name of the application we are creating, but you can change it to any name of your choice.
You can read more on how to get started with React in this article .
What Does DOM Stand For?
The term "DOM" stands for D ocument O bject M odel and refers to the representation of the entire user interface of a web application as a tree data structure.
Types of DOM
We have two types of DOM which are the Virual DOM and the Real DOM.
Advantages and Disadvantages of React
Here are some of the advantages and disadvantages of React:
Advantages of React
- It has a shorter learning curve for JavaScript developers and a large number of manuals, tutorials, and training materials are available because of its active community.
- React is search engine friendly
- It makes it easier to create rich UI and custom components.
- React has quick rendering
- The use of JSX allows us to write code that is simpler, more appealing, and easier to understand.
Disadvantages of React
- Because React is a frontend library, other languages and libraries are required to build a complete application.
- It can be difficult for inexperienced programmers to understand because it employs JSX.
- Existing documentation is quickly out of date due to the short development cycles.
What is JSX?
JavaScript XML is abbreviated as JSX. JSX enables and simplifies the creation of HTML in React, resulting in more readable and understandable markup.
For example:
You can read more about JSX in React in this article .
Why can't Browsers Read JSX?
JSX is not a valid JavaScript code, and there is no built-in implementation that allows the browser to read and understand it. We need to transpile the code from JSX into valid JavaScript code that the browser can understand, and we use Babel , a JavaScript compiler/transpiler, to accomplish this.
Note: create-react-app uses Babel internally for the JSX to JavaScript conversion, but you can also set up your own babel configuration using Webpack.
What are Components?
A component is a self-contained, reusable code block that divides the user interface into smaller pieces rather than building the entire UI in a single file.
There are two kinds of components in React: functional and class components.
What is a Class Component?
Class components are ES6 classes that return JSX and necessitate the use of React extensions. Because it was not possible to use state inside functional components in earlier versions of React (16.8), functional components were only used for rendering UI.
Read more about React components and the types of components in this article.
What is a Functional Component?
A JavaScript/ES6 function that returns a React element is referred to as a functional component (JSX).
Since the introduction of React Hooks, we have been able to use states in functional components, which has led to many people adopting them due to their cleaner syntax.
Difference between Functional and Class components
How to use css in react.
There are 3 ways to style a react application with CSS:
- Inline Styles
- External Styling
Read more on how to style a React application in this article .
What is the Use of render() in React?
Render() is used in the class component to return the HTML that will be displayed in the component. It is used to read props and state and return our JSX code to our app's root component.
What are Props?
Props are also referred to as properties. They are used to transfer data from one component to the next (parent component to child component). They are typically used to render dynamically generated data.
Note: A child component can never send props to a parent component since this flow is unidirectional (parent to child).
Read more about how props works in React here .
What is State in React?
State is a built-in React Object that is used to create and manage data within our components. It differs from props in that it is used to store data rather than pass data.
State is mutable (data can change) and accessible through this.state() .
How to Update the State of a Component in React
It is important to know that when we update a state directly it won’t re-render the component – meaning we don’t get to see the update.
If we want it to re-render, then we have to use the setState() method which updates the component’s state object and re-reders the component.
You can learn more here .
How to Differentiate Between State and Props
State and props are JavaScript objects with distinct functions.
Props are used to transfer data from the parent component to the child component, whereas state is a local data storage that is only available to the component and cannot be shared with other components.
What is an Event in React?
In React, an event is an action that can be triggered by a user action or a system generated event. Mouse clicks, web page loading, key press, window resizes, scrolls, and other interactions are examples of events.
How to Handle Events in React
Events in React are handled similarly to DOM elements. One difference we must consider is the naming of our events, which are named in camelCase rather than lowercase.
Class Component
Function component, how to pass parameters to an event handler.
In functional components, we can do this:
And in class components we can do this:
What is Redux?
Redux is a popular open-source JavaScript library for managing and centralizing application state. It is commonly used with React or any other view-library.
Learn more about redux here .
What are React Hooks?
React hooks were added in v16.8 to allow us to use state and other React features without having to write a class.
They do not operate within classes, but rather assist us in hooking into React state and lifecycle features from function components.
When Did We Begin to Use Hooks in React?
The React team first introduced React Hooks to the world in late October 2018, during React Conf, and then in early February 2019, with React v16. 8.0.
Explain the useState() Hook
The useState Hook is a store that enables the use of state variables in functional components. You can pass the initial state to this function, and it will return a variable containing the current state value (not necessarily the initial state) and another function to update this value.
Explain the useEffect() Hook
The useEffect Hook allows you to perform side effects in your components like data fetching, direct DOM updates, timers like setTimeout(), and much more.
This hook accepts two arguments: the callback and the dependencies, which allow you to control when the side effect is executed.
Note: The second argument is optional.
What's the Use of the useMemo() Hook?
The useMemo() hook is used in functional components to memoize expensive functions so that they are only called when a set input changes rather than every render.
This is similar to the useCallback hook, which is used to optimize the rendering behavior of your React function components. useMemo is used to memoize expensive functions so that they do not have to be called on every render.
What Is the useRefs Hook?
The useRefs() hook, also known as the References hook, is used to store mutable values that do not require re-rendering when they are updated. It is also used to store a reference to a specific React element or component, which is useful when we need DOM measurements or to directly add methods to the components.
When we need to do the following, we use useRefs:
- Adjust the focus, and choose between text and media playback.
- Work with third-party DOM libraries.
- Initiate imperative animations
What are Custom Hooks?
Custom Hooks are a JavaScript function that you write to allow you to share logic across multiple components, which was previously impossible with React components.
If you're interested in learning how this works, here is a step by step guide to help you build your own Custom React Hook .
What is Context in React?
Context is intended to share "global" data for a tree of React components by allowing data to be passed down and used (consumed) in whatever component we require in our React app without the use of props. It allows us to share data (state) more easily across our components.
Read more about React Context in this guide .
What is React Router?
React router is a standard library used in React applications to handle routing and allow navigation between views of various components.
Installing this library into your React project is as simple as typing the following command into your terminal:
In this tutorial, we went over some React interview questions to help you prepare for your interviews. All of us at FreeCodeCamp wish you the best of luck and success in your interview.
Instead of attempting to take several courses at once, find a helpful tutorial and complete it if you want to learn more and master React so you can perform well in practical interview sessions. Check out freeCodeCamp's Free React Course for 2022 or Learn React - Full Course for Beginners .
Frontend Developer & Technical Writer
If you read this far, thank the author to show them you care. Say Thanks
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
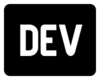
DEV Community

Posted on Jul 10, 2022 • Updated on Oct 24, 2023
11 Advanced React Interview Questions you should absolutely know (with detailed answers)
1. what is the react virtual dom .
Virtual DOM is a concept where a virtual representation of the real DOM is kept inside the memory and is synced with the actual DOM by a library such as ReactDOM .
The virtual DOM is an object that represents the real DOM in the memory. Since DOM updates are an integral part of any web app but are the costliest operation in the world of frontend , the virtual DOM is utilized to check for parts of the app that need to be updated & update only those parts, thus significantly boosting performance .
2. Why do we need to transpile React code?
React code is written in JSX , but no browser can execute JSX directly as they are built to read-only regular JavaScript .
Thus we require to use tools like Babel to transpile JSX to JavaScript so that the browser can execute it.
3. What is the significance of keys in React ?
Keys in React is used to identify unique VDOM Elements with their corresponding data driving the UI ; having them helps React optimize rendering by recycling existing DOM elements.
Key helps React identify which items have changed , are added , or are removed , enabling it to reuse already existing DOM elements, thus providing a performance boost .
For example:
This would cause new DOM Elements to be created everytime todos change, but adding the key prop ( <li key={todo.id}>{todo.text}</li> ) would result in "dragging" around the DOM Elements inside the ul tag & updating only the necessary li s.
4. What is the significance of refs in React ?
Refs are variables that allow you to persist data between renders , just like state variables, but unlike state variables, updating refs does NOT cause the component to re-render .
Refs are usually used to, but not restricted to, store reference to DOM elements .
5. What are the most common approaches for styling a React application?
CSS Classes
React allows class names to be specified for a component like class names are set for a DOM element in HTML .
When developers first start using React after developing traditional web applications, they often opt for CSS classes as they are already familiar with the approach.
Styling React elements using inline CSS allows styles to be completely scoped to an element. However, certain styling features are not available with inline styles . For example, the styling of pseudo-classes like :hover .
Pre-processors (Sass, Stylus, and Less)
Pre-processors are often used on React projects. This is because, like CSS , they are well understood by developers and are often already in use if React is being integrated into a legacy application.
CSS-in-JS Modules (Styled Components, Emotion, and Styled-jsx)
CSS-in-JS modules are a popular option for styling React applications because they integrate closely with React components. For example, they allow styles to change based on React props at runtime. Also, by default, most of these systems scope all styles to the respective component being styled .
6. What are some of the performance optimization strategies for React ?
Using useMemo
useMemo is a React hook that is used for caching CPU-Expensive functions . A CPU-Expensive function called repeatedly due to re-renders of a component , can lead to slow rendering .
useMemo hook can be used to cache such functions. By using useMemo , the CPU-Expensive function gets called only when it is needed.
useCallback can be used to obtain a similar result.
Lazy Loading
Lazy loading is a technique used to reduce the load time of a React app . It helps reduce the risk of web app performances to a minimum, by loading up the components as the user navigates through the app .
7. What is prop drilling and how to avoid it?
Sometimes while developing React applications, there is a need to pass data from a component that is higher in the hierarchy to a component that is deeply nested . To pass data between such components, we pass props from a source component and keep passing the prop to the next component in the hierarchy till we reach the deeply nested component .
The disadvantage of using prop drilling is that the components that should otherwise be not aware of the data have access to the data, moreover, the code becomes harder to maintain .
Prop drilling can be avoided using the Context API or some form of State Management library.
8. What is the StrictMode component and why would you use it?
<StrictMode /> is a component included with React to provide additional visibility of potential issues in components. Suppose the application is running in development mode . In that case, any issues are logged to the development console , but these warnings are not shown if the application is running in production mode .
Developers use <StrictMode /> to find problems such as deprecated lifecycle methods and legacy patterns , to ensure that all React components follow current best practices.
<StrictMode /> can be applied at any level of an application component hierarchy , which allows it to be adopted incrementally within a codebase.
9. What are synthetic events in React ?
Synthetic events combine the response of different browser's native events into one API , ensuring that the events are consistent across different browsers . The application is consistent regardless of the browser it is running in.
10. Why is it not advisable to update state directly, but use the setState call?
The conventional way to update state is to use the setState call. Without using it, the user would still be able to modify the state, but it would not update the DOM to reflect the new state.
11. What are portals in React ?
Portal is a recommended way to render children into a DOM node that exists outside the DOM hierarchy of the parent component.
Finding personal finance too intimidating? Checkout my Instagram to become a Dollar Ninja
Thanks for reading
Need a Top Rated Front-End Development Freelancer to chop away your development woes? Contact me on Upwork
Want to see what I am working on? Check out my Personal Website and GitHub
Want to connect? Reach out to me on LinkedIn
Follow me on Instagram to check out what I am up to recently.
Follow my blogs for Weekly new Tidbits on Dev
These are a few commonly asked questions I get. So, I hope this FAQ section solves your issues.
I am a beginner, how should I learn Front-End Web Dev? Look into the following articles:
- Front End Development Roadmap
- Front End Project Ideas
Would you mentor me? Sorry, I am already under a lot of workload and would not have the time to mentor anyone.
Top comments (17)
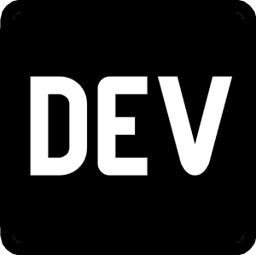
Templates let you quickly answer FAQs or store snippets for re-use.

- Joined Feb 20, 2022
Cooncidentally this is actually a pretty good article for people to get started with React haha.
I'm not sure if I would appreciate being asked these questions in an interview though... Answering these kind of higher level concepts is really difficult in a person-to-person conversation, if there's doubt about the candidates capabilities as a React developer it's better to give them a small assignment to see how they deal with prop drilling or a broken example that they need to fix/optimize by memoizing a value etc...
On the other hand these are also things that can be taught pretty quickly through code reviews, so if a company starts asking these kind of questions it's an indication that they're concerned about the wrong things.

- Joined Nov 19, 2021
That's only shows you know how to to use jsx not why react has implemented the features which it has this results in in dev's not knowing how things work means that when the next major update comes out the Dev in questions performance shall drop
What exactly are you talking about? Knowing how what things work? Knowing how the virtual dom works does not help you to write better performing code. The virtual dom is encapsulated in the React framework black box which is a black box for a reason. Unless you want to start working at React, there's no reason to increase your knowledge of what the virtual dom is and how it works, thats why you use React, to abstract that complexity for you, so you can focus on implementing business logic in the best way possible.
My examples would be that Understanding how the virtual react stores and updates state has a marked importance for performance accessibility and seo. That is what my main focus is and programming people to spit out JSX has also a recognised issue when it comes to just using libraries on top of dependencies or not considering the issues with purely client side rendering.
I completely agree that it is easy to teach JSX but understanding where and why it has emerged and the practicality of a framework built for Facebook is important and often overlooked. If you are down to using the is odd lib/ dependency and using bootstrap without purging or treeshaking why not just use a cms with plugins. It is often not right package for the job even though it works. You need to understand it quite deeply to make your own reactivity optimal abstraction aside.
For many use cases React has a great community but is very average while WordPress is excellent.
I hope this clarifies my position. A programmer should be able to look at patterns, recognise what they need and have a work flow that removes what they don't another reason I used WordPress as an example.
If the global [you] don't understand why your framework is widely used but just know how than can [you] really claim to be full stack. Coincidentally this has been discussed and dissected here although a quote about javascript in 2008 is quoting a different language the future was predicted unsurprisingly well.
The virtual DOM does not store and update state, React does. those state updates are reflected in the virtual DOM by React and then it decides which parts of the actual DOM need to be rerendered. The exact logic behind this is not relevant for any business logic that I've had to implement in the last 10 years.
A developer needs to recognize what the problem is they're trying to solve. React helps developers to solve a lot of problems that would previously take a lot more effort to solve, which is why it became a popular framework.
Comparing a CMS and a frontend framework is somewhat farfetched... There's good CMSes built on top of React or other modern frontend frameworks also. No need to stick with something archeic like Wordpress. And yes, if you have a simple use-case that doesnt require much custom business logic, you can use a CMS. Knowing how the virtual DOM works has no influence on that decision. The question is: "Is my data model simple enough that I can use a pre-built CMS, or is there so much custom business logic that I require something custom."
Full stack has nothing to do with what framework you use. Full stack is about knowing how to work on both frontend and backend code.
If your goal is to come up with your own framework thats better than React, sure then you can deep dive in how React is actually manipulation the virtual DOM internally. However this is not required knowledge to become a good developer. Knowing how to properly use the tools that you need, is what makes you a good developer. Similarly, a carpenter doesn't need to know what happens internally in a hammer when they use it to whack a nail, they just need to know how to whack properly. Unless the carpenter comes to the conclusion they can't find a proper hammer to do the job and decides to built their own hammer.
To clarify I mean updates as in literally as in FPS, ticks and reconciliation. Your hammer analogy is good, I was not referring to framework* s * though I was referring to React and it is fairly blunt :p. I have a lot of respect for the CRA team and how they have approached things. I will quickly say that a carpenter should know their tools.
Like I said if you have just been programmed in how to use JSX for React are you truly full-stack. Can you add the layers and sometimes complex workaround which are required (for instance when updating legacy versions -- I guess that's a rock to a hammer), or solve a mess of a modal window somebody hashed together years ago because React was the only option.
You need to understand how React works in order to justify using it for a lot of the small SPAs which it has been used for which have literally bloated the internet. I am not saying that React doesn't have use-cases, I am not saying React can't be SEO friendly but largely that is overlooked without additional plugins .
To me it feels like when a CS student first discovers bootstrap. They have a whole library of problems to cut corners and leave all the unnecessary functionality.
We have gone off topic. React is good enough because it is flexible, Solid is fast and considers virtual doms to be bloat, Svelte is loved for intuity and ingenuity, Ruby on Rails has a different kind of battle tested simplicity. Likewise Angular is the full package and extends the dom but is perceived as complex.
A dev wants the right tool for the job and there are plenty of good enough's out there. If you want reactivity from react you need some understanding of the virtual dom implimentation. I have thrown you some edge cases but programming is solving problems through automation and hammering out some JSX which misses a tick isn't that.
This reads like a long rant. There are some excellent devs out there who use react, there are less excellent react devs - I won't say they are unicorns but I will say they are limiting themselves.
Edit - had a quick snoop at some of your answers and liked the one where you are basically agreeing with my overall point
Edit 2 -- On revisit my first post wasn't clear 👍 I hope I have clarified a bit?

- Email [email protected]
- Work Software Engineer
- Joined Nov 14, 2018
If you're interviewing and you see questions like this: Run! Take it as a 🚩 red flag 🚩. If you're an interviewer, then make questions that actually matter, that actually let you know if that person will enjoy working with you in your project.
Cool article tho!

- Education AccuTech Career Institute
- Work Day: IT Manager in Insurance, Night: dev@ my own private firm
- Joined May 13, 2022
Oh this is so good for beginners! Very concise and well put together 👍🏼

- Work Junior Frontend Engineer at freelancer
- Joined Feb 23, 2021
Nice compilation, thanks

- Work Software Developer
- Joined Aug 26, 2021
THX for that.

- Location Kolkata, West Bengal, India
- Joined Dec 4, 2020
I appreciate you spending your precious time to write down such lengthy thoughts!

- Location Planet Earth
- Joined Jun 11, 2019
I want to work with this guy! 👆
I've also done my fair share of technical interviews, and it doesn't matter if they know what a Portal is, because they can always go read the documentation and learn it in a couple of minutes.

- Joined Sep 21, 2022
I'm always wary of people with this attitude. The thing is its hard to find strong engineers. If you are an engineer lacking knowledge in these areas then its possible that you will not be able to contribute at the same level as someone who does. You don't need to use this knowledge day-in-day-out, but when tricky problems come up, you should be able to easily know what options you have for solving them.
To use the same car driver anology. Yes, to be a good driver, you don't need to know the composition of oil. But if your car breaks down, are you going to have the skills to fix it? Or will you need to hire a mechanic? Maybe you can spend 5 hours googling what the car is doing with the oil you are putting in to maybe understand what is going wrong.
Point is, if you are going to use a tool, as an engineer, you can probably go far knowing the bare minimum (how to drive), until you are working on complex systems that rely on the core functionality of those tools; if you don't know anything beyond the bare minimum, there's going to be a limit to how much you can help.
And specifically speaking about React, the react core is REALLY small, so expecting that engineers know many of these topics mentioned in this blog is not outragous.
But even if you don't know the answer to these questions, you should be able to participate in a conversation about these things, which is what the interviews should be ultimately. If you see these questions as a red flag, honestly I think you as a candidate are a red flag -- it just shows that have no interest in having a deep understanding of the tools you use daily, and/or you are not a great communicator (not able to have conversations about things you don't know about). I'm not speaking in behalf of all interviewers here, but myself, as someone who carries out interviews as a conversation more so than a test.
Also, In my opinion, the culture of "I'll google it when I need it" leads to many engineers building sub-optimal solutions, (not just in terms of performance, but speaking generally), and many times, code that is coppied-and-pasted from blogs or stack overflow without the author thinking critically about what is needed and not needed for their specific problem. Ultimately this is what interviewing is about for me. Getting indicators that the candidate has the ability to think for himself and solve a problem using (hopefully) a wide range of technical skills in their respective area. And there just isn't a way around that other than making them solve some problems and start conversations about these topics.
Lastly I'll say, the reason why I'm so moved by this is that it does no good to encourage people to not spend the time learning these things. By learning these things, they are able to contribute at a higher-level than someone who doesn't, and can become a better asset for their teams. Is your opinion that this may be a red flag, but in my opinion, it only HELPS to spend time learning these things, if you are planning to work with React daily.

- Location Boston
- Education BS Computer Science, UMass Lowell
- Work Staff Software Engineer at Synopsys
- Joined Jun 4, 2019
Most of these questions are pretty vague and honestly do not seem like "absolutely should know" questions.
Like StrictMode is definitely useful but to consider it a must-know for a React job interview is a little silly IMO.
I agree with much of what you said I think I misunderstood your stance. But I didn't appreciate your tone which seemed a bit condescending (at times) 😏
I agree, interviews should not be carried out like exams with close ended questions, but there's nothing wrong with digging into these topics in an interview. I think its totally fair to ask someone to explain their mental model for understanding what react does, it doesn't have to be a text book definition, but I think its completely reasonable to expect that developers have SOME mental model for what react is doing under the hood, and asking them to explain that is reasonable in my opinion.
Also, on the topic of googling, OBVIOUSLY every engineer googles things, like API documentation, common approaches for a given problem etc. Research is part of the job. But also is internalizing the research and making a decision on what you are going to do. What I was speaking about was about a breed of developers that google then copy and paste solutions without thinking critically about the code they are committing. My opinion (and one of my points) is that this group of developers overlap with the developers that do not take the time to learn the fundamentals of the technologies they work with (like virtual dom, and core react APIs if you work with react). I was projecting an assumption -- I may be wrong, but I've seen this correlation before.

- Location Pakistan
- Education Computer Science
- Work Full Stack Developer at self-employed
- Joined Jun 19, 2020
Advanced??? Really. All of these are basic concepts, good for beginners.
Some comments may only be visible to logged-in visitors. Sign in to view all comments.
Are you sure you want to hide this comment? It will become hidden in your post, but will still be visible via the comment's permalink .
Hide child comments as well
For further actions, you may consider blocking this person and/or reporting abuse
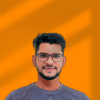
Array methods in react.js
Anil - Mar 18
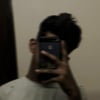
11 BEST JavaScript Animation Libraries 🎨✨
Arjun Vijay Prakash - Mar 29
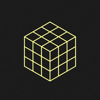
How to Fix K8S Exit Code 137?
CiCube Team - Mar 27
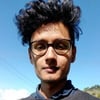
Rotating Images with Node.js Made Easy: Automate BoreStuffs
Akshay S - Mar 27
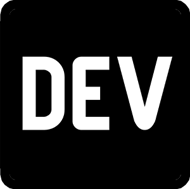
We're a place where coders share, stay up-to-date and grow their careers.
- React Tutorial
- React Exercise
- React Basic Concepts
- React Components
- React Props
- React Hooks
- React Advanced
- React Examples
- React Interview Questions
- React Projects
- Next.js Tutorial
- React Bootstrap
- React Material UI
- React Ant Design
- React Desktop
- React Rebass
- React Blueprint
- Web Technology
- React Introduction
- React Environment Setup
React Fundamentals
- ReactJS Babel Introduction
- ReactJS Virtual DOM
- React JS ReactDOM
- ReactJS Lists
- React Forms
- ReactJS Keys
- ReactJS Refs
- ReactJS Rendering Elements
- React Conditional Rendering
- Code Splitting in React
- ReactJS | Components - Set 2
- ReactJS Pure Components
- ReactJS Functional Components
- React Lifecycle
- Differences between Functional Components and Class Components
- ReactJS Container and Presentational Pattern in Components
React Props & States
- ReactJS Methods as Props
- ReactJS PropTypes
- ReactJS Props - Set 1
- ReactJS Props - Set 2
- ReactJS Unidirectional Data Flow
- ReactJS State
- ReactJS State vs Props
- Implementing State in React Components
- React useState Hook
- ReactJS useEffect Hook
- Context in React
- React Router
- React JS Types of Routers
- ReactJS Fragments
- Create ToDo App using ReactJS
- Create a Quiz App using ReactJS
- Create a Coin Flipping App using ReactJS
- How to create a Color-Box App using ReactJS?
- Dice Rolling App using ReactJS
- Guess the number with React
React Connection & Deployment
- How to Deploy Your React Websites on GitHub?
- How to Deploy React project on Firebase?
- How to deploy React app to Heroku?
- How to deploy React app to Surge ?
- How to deploy simple frontend server-less (static) React applications on Netlify
React Exercises
React exercises, practice questions and solutions, react questions.
- How to connect Django with Reactjs ?
- 7 React Best Practices Every Web Developer Should Follow
- 8 Ways to Style React Components
- How to add Stateful component without constructor class in React?
- How to display a PDF as an image in React app using URL?
- React JS Toast Notification
- What is the use of data-reactid attribute in HTML ?
- How to zoom-in and zoom-out image using ReactJS?
- How to avoid binding by using arrow functions in callbacks in ReactJS?
- How to bind 'this' keyword to resolve classical error message 'state of undefined' in React?
- How to get the height and width of an Image using ReactJS?
- How to handle multiple input field in react form with a single function?
- How to handle states of mutable data types?
- How to change state continuously after a certain amount of time in React?
- How to change the state of react component on click?
React Exercise: Explore interactive quizzes, track progress, and enhance coding skills with our engaging portal. Ideal for beginners and experienced developers, Level up your React proficiency at your own pace. Start coding now!
A step-by-step React practice guide for beginner to advance.
Benefits of React Exercises
- Interactive Quizzes: Engage in hands-on React quizzes.
- Progress Tracking: Monitor your learning journey.
- Skill Enhancement: Sharpen coding skills effectively.
- Flexible Learning: Practice at your own pace.
- Immediate Feedback: Receive instant results and feedback.
- Convenient Accessibility: Accessible online, anytime.
- Real-world Application: Apply React concepts practically.
- Comprehensive Learning: Cover a range of React topics.
How to Start Practice ?
Embark on your React learning journey with our online practice portal. Start by selecting quizzes tailored to your skill level. Engage in hands-on coding exercises, receive real-time feedback, and monitor your progress. With our user-friendly platform, mastering React becomes an enjoyable and personalized experience. Elevate your coding expertise today.
React Best Practice Guide
Dive into React excellence with our Best Practice Guide. Explore coding standards, optimization techniques, and industry-recommended approaches. Elevate your skills through insightful tips, real-world examples, and interactive challenges. Unlock the secrets of efficient and maintainable React coding, ensuring your projects stand out with clarity and performance.
Why Practice React Online ?
Elevate your React online test experience with categorized fill-in-the-blank questions. This structured format guides your learning, providing targeted challenges for hands-on improvement. Engage in focused practice as it reinforce coding concepts, receive instant feedback, and enhance your skills incrementally. Moreover, by combining theoretical understanding with practical application, you’ll enjoy a comprehensive and effective approach to mastering React and as a result optimizing your performance in real-world scenarios.
React Online Practice Rules
- Be Honest: Complete challenges independently, refrain from plagiarism or seeking unauthorized help.
- Manage Time: Adhere to assessment time limits, simulating real-world scenarios.
- Code Quality : Prioritize clean, efficient, and well-documented code.
- Follow Guidelines : Adhere to platform-specific instructions, including input/output formats and code submission procedures.
- No Cheating: Avoid external resources during assessments, unless explicitly allowed.
- Utilize Feedback: Learn from automated or mentor/community feedback.
- Participate Actively: Engage in forums, discussions, and share insights with fellow learners.
- Continuous Improvement: Use assessments to identify and improve areas of weakness for ongoing growth.
Features of Practice Portal :
- Instant Mistake Correction: Receive immediate feedback on errors to facilitate quick learning.
- Unlimited Tries: Allow learners to attempt questions multiple times for mastery.
- Time Display for Each Category: Show elapsed time for each set of 5-6 fill-in-the-blank questions to manage time effectively.
- Table View of Scenarios: Provide a comprehensive table view displaying all categories, their status (completed or pending), and corresponding scores.
- Performance Analytics: Track progress with detailed analytics, highlighting strengths and areas for improvement.
- Interactive Code Editor: Offer a responsive code editor for an immersive hands-on experience.
- Hints and Solutions: Include hints for guidance and solutions to foster self-learning.
- Community Integration: Facilitate collaboration through forums and discussions for peer learning.
- Adaptive Difficulty: Adjust difficulty levels based on user performance for personalized challenges.
- Gamification Elements: Incorporate scoring, achievements, or badges to make learning engaging.
Please Login to comment...
- Web Technologies
- How to Delete Whatsapp Business Account?
- Discord vs Zoom: Select The Efficienct One for Virtual Meetings?
- Otter AI vs Dragon Speech Recognition: Which is the best AI Transcription Tool?
- Google Messages To Let You Send Multiple Photos
- 30 OOPs Interview Questions and Answers (2024)
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Not sure where to start?
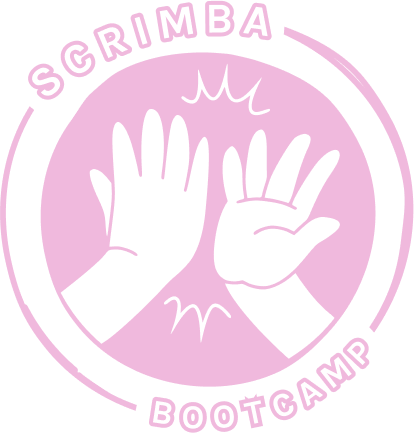
Study group
Collaborate with peers in your dedicated #study-group channel.
Code reviews
Submit projects for review using the /review command in your #code-reviews channel
React Challenges
Join 1900 other students
Log in to get
Subscribe to access to this course and ALL other courses. You get a 30-day money-back guarantee, no questions asked.
Subscription includes
with Daniel Rose
Course level: Intermediate
Transform your coding skills and unlock your success through real-world problem-solving across 40 immersive challenges.
What's inside
This course contains 82 interactive scrims
82 lessons 9 hours 45 min
You'll learn
DOM manipulation
Conditionals
State setting
Objects and arrays
Forms and inputs
Default Form Data
Mouse interactions
UX optimizations
preventDefault()
useEffect()
Converting JS to React
Code organization
Array methods
Writing tests
Destructuring
Writing efficient code
Prop reduction
State verification
localStorage
Dynamically-generated values
Iterative code
Dynamic components
Styles in React
Dealing with duplication
APIs in React
Submitting data
Filtering data
Audio in React
Working with times
Prerequisites
Before taking this course, you should have a basic understanding of HTML, CSS, JavaScript, and React. Below are our suggested resource to get you up to speed.
Meet your teacher
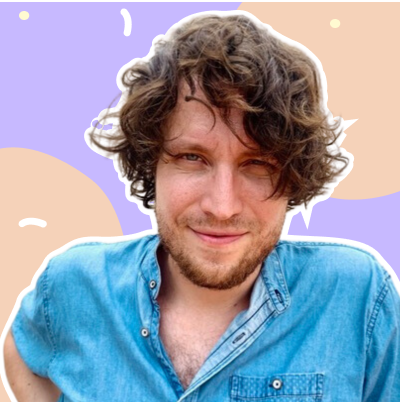
Daniel Rose
React enthusiast, vanilla JS appreciator, CSS aficionado, and I’ve run out of words in my thesaurus, so I’ll leave it that! Outside of coding, my passions are music, books, education, dogs, and everything else with fur, feathers, or scales. I'm a big believer in active, hands-on learning, which is what drew me to Scrimba as both a former student and now a teacher! I remember feeling bad about myself and doubtful of my abilities when I first started trying to learn to code elsewhere, and I really want to help other people avoid that experience and feel empowered and confident with this stuff!
Why this course rocks
This course is designed to elevate you from React learner to React developer. With more than 40 interactive, real-world challenges, you will test your understanding of React's fundamental concepts and enhance your ability to solve problems. Every challenge in the series is designed to mimic a real coding scenario, providing a practical and hands-on approach for an effective learning experience. These include a consent form, calculator, dashboard, blog, tracker, file uploader, weather app, clock, etch-a-sketch, and various games. This course provides immersive tasks that will give you the necessary knowledge, confidence, and experience to work effectively with production codebases and projects utilizing React. The challenges included in the course cover a huge range of topics which are specifically designed to prepare you for success with React and in your coding career. After completing each challenge, you can compare your solution with the one provided by our React expert. This will give you the opportunity to learn from their expertise and experience, as if you were programming together as a pair. By the end of the course, you will have a comprehensive understanding of React and its many capabilities, as well as practical experience in using React to develop games, apps, features, and tests. React knowledge at the ready? Let's get started.
Built with Imba
Featured articles
Build a paginated pokemons list with a "load more" button - starting from failing unit tests, practice react by fixing tests - check your jsx knowledge, build a button using test driven development, build a simple shopping cart using react-query, create a custom hook that allows saving items to the local storage, create a simple contact book app, create a movie search page.
Acing the ReactJS Interview: Common Questions and Sample Answers
Prepare for your ReactJS interview with our detailed Q&A. Understand Virtual DOM, component lifecycle, state management, and more for interview success.
Avinash Bidkar
Introduction.
In the dynamic landscape of modern web development, ReactJS has emerged as a cornerstone technology, revolutionizing how user interfaces are built and maintained. As React gains prominence, so does the demand for skilled ReactJS developers who can harness its power to craft seamless, interactive web applications. The ReactJS interview process is a pivotal gateway to these opportunities, testing candidates on their theoretical knowledge, coding prowess, and problem-solving acumen. This blog is your ultimate guide to facing and acing your ReactJS interview.
Common ReactJS Interview Questions and Sample Answers
Here are some common and frequently asked ReactJS interview questions and their answers.
1. What is ReactJS, and how does it differ from other JavaScript frameworks?
ReactJS is an open-source JavaScript library designed for building user interfaces. Unlike traditional frameworks, React focuses solely on the view layer, making it highly efficient for creating dynamic UIs. Its component-based architecture promotes reusability and modularity, allowing developers to compose complex interfaces from smaller, self-contained building blocks. This contrasts with frameworks like Angular, which offer a more comprehensive structure encompassing data management and routing. Additionally, React's virtual DOM optimizes rendering, updating only the necessary parts of the actual DOM, which enhances performance compared to other frameworks that might re-render entire sections.
2. Explain the concept of JSX.
JSX, or JavaScript XML, is a syntax extension used in React to describe the structure of UI components. It resembles HTML but seamlessly integrates JavaScript expressions. JSX simplifies component rendering by allowing developers to write expressive, declarative code. Under the hood, JSX gets transpiled into standard JavaScript using tools like Babel. For instance, <Button color="blue" /> in JSX corresponds to React.createElement(Button, {color: 'blue'}) in JavaScript. This blend of HTML-like syntax with JavaScript logic streamlines UI development and enhances code readability.
3. What is the significance of the virtual DOM?
Virtual DOM is a crucial concept in React that boosts performance and efficiency. It's an abstraction of the actual DOM, a lightweight representation of UI components and their structure. When changes occur, React compares the virtual DOM with the real DOM, computing the minimal necessary updates. This leads to fewer direct manipulations of the actual DOM, reducing rendering overhead and enhancing speed. Consequently, applications built with React deliver a smoother user experience as updates are processed optimally, minimizing unnecessary reflows and repaints.
4. How do React props work?
React props, short for properties, pass data from a parent component to its child components. They enable communication and allow components to be reusable and modular. Props are immutable and provide a way to share information between components without directly modifying the component's state. By defining attributes within JSX tags, you can pass data down the component hierarchy. For instance, <UserProfile name="John" age={25} /> sends the name and age values as props to the UserProfile component. Inside the component, these values are accessible as this.props.name and this.props.age.
5. What is React state, and how is it different from props?
React state is a built-in feature that allows components to store and manage their internal data. While similar to props, which are passed from parent to child components, the state is local and mutable, whereas props are immutable. The state is primarily used for dynamic data, like user input or component-specific values, that can change over time. Unlike props, which are externally controlled, a component manages its state. Using the setState method, you can modify the state, prompting React to re-render the component and update the UI based on the changes.
6. Explain the component lifecycle in React.
The React component lifecycle consists of three main phases: mounting, updating, and unmounting. The component is initialized during mounting, and methods like constructor and componentDidMount are executed. In the updating phase, changes trigger methods like shouldComponentUpdate to determine if re-rendering is needed, followed by componentDidUpdate after rendering. Finally, in the unmounting phase, the component is removed from the DOM, and the component will unmount method is called. Understanding these phases helps manage component behavior, optimize rendering, and perform cleanup tasks.
7. How does React handle forms?
React provides two approaches for handling forms: controlled components and uncontrolled components. Controlled components maintain form data in the component's state, enabling dynamic updates and validation. Uncontrolled components, on the other hand, rely on the DOM for form data storage. Developers can access form values using references after user input. Controlled components offer better control and validation, making them a preferred choice for complex forms, while uncontrolled components are suitable for simpler scenarios.
8. What is the significance of keys in React lists?
Keys are essential when rendering lists of elements in React. They provide a unique identifier for each item in a list, enabling React to track and manage updates efficiently. When items are added, removed, or rearranged, keys ensure that only the necessary elements are modified in the DOM. React might re-render the entire list without keys, resulting in performance issues and unintended side effects. Assigning a key to each list item ensures stable and predictable rendering behavior.
9. How can you optimize a React application's performance?
Optimizing a React application's performance involves techniques like code splitting, lazy loading, and memoization. Code splitting divides the application into smaller chunks, loaded on-demand, reducing the initial load time. Lazy loading defers loading non-essential components until they are needed. Memoization optimizes rendering by caching the results of expensive function calls using React. memo and useMemo, preventing unnecessary recalculations.
10. Describe the concept of "lifting state."
"Lifting state up" is a pattern in React where the shared state is managed at a higher level in the component hierarchy and passed down as props to child components. This pattern enhances data consistency and synchronization between components. By centralizing state management, you prevent inconsistencies and improve maintainability. For instance, when multiple child components need access to the same data, lifting the state to a common ancestor simplifies data management, reduces redundancy, and enhances component interaction.
In the ever-evolving realm of web development, ReactJS is a beacon of innovation, shaping the digital landscape with its dynamic capabilities. As you embark on your ReactJS interview journey , remember that success goes beyond mastering technical concepts – it encompasses effective communication and the ability to showcase your skills with clarity. By embracing the insights shared in this guide, you can navigate the diverse ReactJS interview questions confidently. Your journey to ReactJS excellence starts here. Best of luck!
What should I anticipate from a ReactJS job interview?
Your theoretical knowledge, technical skills, and problem-solving talents are evaluated during ReactJS interviews. React principles, JSX, virtual DOM, state management, and component lifecycle are topics that interviewers frequently probe.
What distinguishes ReactJS from other JavaScript frameworks?
A JavaScript library devoted to creating user interfaces is called ReactJS. It excels at building dynamic user interfaces by quickly drawing just the required bits of the view. React's component-based architecture, in contrast, to complete frameworks like Angular, encourages reusability and modularity, making it a popular option for front-end development.
Can you describe JSX and how it is used to create React?
React uses the grammar extension known as JSX, or JavaScript XML, to express UI components. It simplifies component rendering and improves code readability by combining JavaScript expressions with syntax similar to HTML. The declarative nature of React programming is enhanced by translating JSX into regular JavaScript code.
In React, what role does the virtual DOM play?
React's virtual DOM is a key idea that improves performance. It optimizes UI rendering by computing the fewest updates possible and is a lightweight approximation of the actual DOM.
How are props and React states different?
React state is mutable and used to manage internal data within components. Props, on the other hand, are immutable and handed from parent to child components. Props enable communication between components without directly affecting their internal state, whereas the state is appropriate for maintaining dynamic data that might change over time
Our Courses
Practice-Based Learning Tracks, Supercharged By A.I.
React Online Coding Tests
Create and send React coding tests in 60 seconds, even if you’re not techy. Hand over qualified React candidates for your engineering team to interview.
Back to online coding tests
About our React coding tests
We provide React code exams for your team that are realistic and useful, giving a precise assessment of candidates’ React skills. Even if you don’t have a technical background, you can develop React code tests in under a minute using tools like CoderPad Screen.
We have created our tests to precisely cover important ideas like object-oriented programming, data structures, algorithms, and more. Our industry specialists have carefully selected them to ensure their applicability to real-world situations and their efficacy in evaluating candidates’ talents.
There is an ability to modify the tests and add their own coding activities for hiring managers who wish to go further.
- Recommended duration: 20-60 min
- Average number of questions: 20
- Type of exercises: Multiple choice, coding exercises
- Test levels : Junior, Senior, Expert
ℹ️ Explore our questions bank and test our candidate experience yourself
React skills to assess
- Component lifecycle
- React hooks
- React context API
- State management
Jobs using React
- Front-End developer
React sample questions
Example question 1.
What is the difference between a class component and a functional component in React? What is the difference between a class component and a functional component in React?
Example Question 2
Your task is to create a working todo list with persistent data storage.
Requirements
- Clicking on a todo item should toggle the “checked” state.
- The todo list state should be saved and loaded from local storage.
- Checked items should sink to the bottom of the list automatically
ℹ️ Preview a sample coding test report
Explore Code Playback
Obtain a deeper understanding of the problem solving thought process of your developer applicants.
- Witness their proficiency in building algorithms and evaluate their approach to solving problems with them.
- Jot down feedback from different segments of the interview to discuss in subsequent interview rounds.
- Pay attention to detect and prevent any distasteful actions.
How to test React Skills to hire for your team?
Relying only on resumes may not accurately paint a broad picture of a React developer’s skills and abilities, and self-reported skills may be untrustworthy.
Here are five ways to assess React developers’ coding skills:
- Examine their body of work. This provides you with more information about the kinds of React projects they have worked on and their level of language expertise.
- Examine their GitHub account to judge the quality of their React code, their projects, and their participation in React-related projects.
- Ask about their use of Stack Overflow or React communities in their day-to-day development to understand their knowledge level and participation in the React development community.
- Employ the use of practical React focused programming exercises for React developers to allow them assess and improve their coding skills.
- Conduct React-focused live coding interviews in which candidates can exhibit their coding skills, problem-solving talents, and understanding of React fundamentals.
💡 To dig deeper: 5 ways to test developers’ skills before hiring
How to approach initial candidate screening effectively?
Some recruiters are concerned that putting coding tests on candidates early in the interview process will intimidate potential prospects and discourage applications. However, good scenario management, such as clarifying the interview process and why it is vital, will help candidates comprehend the goal and feel at ease.
Here are some guidelines for you to ensure a positive candidate experience during early testing:
- Job Ad : Make certain that the advertisement includes every phase and expectation of the hiring process. As a result, the candidate will not feel singled out and will be able to fully prepare for the interview. It is also a good idea to convey the time limits involved in technical interviews ahead of time.
- Highlight Equal Opportunities and Diversity: Emphasize your company’s commitment to promote diversity and equal opportunity through the testing process. It must to be obvious that the major objective of the assessment is to fairly evaluate the candidate talents, regardless of history.
- Concise Assessment: Choose quality over quantity by keeping the assessment brief and focused on the skills required for the job. This is to eliminate any time wasting and ensure the candidates can attempt the exercise hitch-free. Ideally, aim for a test that can be completed within an hour or less without compromising any requirements.
- Relevance: By ensuring that the examinations only focus on the abilities that are specifically relevant to the position, redundancies can be avoided. Developers value candidates that show pragmatism by emphasizing practical abilities that have an impact on daily work.
- Feedback and Results : Provide applicants with performance comments after the testing phases. You may improve candidates’ experiences by using a product like CoderPad Screen, which automatically creates a concise report and sends it to them. Candidates who do poorly will be able to understand why they were passed over for the following round in this way.
We had no unified approach or tool to assess technical ability. Today, CoderPad Screen enables us to test against a wide selection of languages and is continually updated.
1,000 Companies use CoderPad to Screen and Interview Developers
- Knowledge Base
- Free Resume Templates
- Resume Builder
- Resume Examples
- Free Resume Review
React.js, often referred to as React has emerged as one of the most popular JavaScript libraries for building user interfaces in modern web development.
And in today's fast-paced digital landscape, where user experience plays a crucial role in the success of web applications, React.js has gained significant traction. Its simplicity, flexibility, and performance have made it a go-to choice for developers and organizations worldwide.
Along with that, the demand for React.js developers has also been skyrocketing, driven by the increasing adoption of React.js in the industry.
JavaScript developers are currently in high demand nationwide in the US, and the skills associated with this role such as React.js are highly sought after.
This surge in demand has made the interview process for React.js positions highly competitive. To stand out from the crowd and land their dream job, aspiring React.js developers need to be well-prepared for the interview process.
This guide will explore a comprehensive list of React.js interview questions covering various aspects of React.js development.
By familiarizing yourself with these questions and their answers, you can boost your confidence, improve your interview performance, and increase your chances of securing a React.js development role in today's highly competitive job market.
Let's dive in!
- What are some common React.js interview questions and answers?
- What are some React.js coding interview questions?
- What are some commonly asked React.js interview questions for senior developers?
- What are some top React.js interview questions and answers for freshers?
- How to answer React.js interview questions the right way?
Also Read: How to prepare for c# interviews in 2023?
Basic React.js Interview Questions and Sample Answers
Basic React.js interview questions typically refer to fundamental questions that assess a candidate's understanding of the core concepts and principles of React.js.
These questions aim to gauge the candidate's foundational knowledge of React.js and their ability to explain fundamental concepts clearly and concisely.
By asking these basic questions, interviewers can assess a candidate's familiarity with react.js and their understanding of various React.js terminology and essential concepts.
Given below are some basic React.js interview questions with sample answers that you can refer to:
What is React.js and what are its core features?
Sample Answer: React.js is a JavaScript library used for building user interfaces. Its core features include a virtual DOM for efficient rendering, component-based architecture for reusability, JSX syntax for combining JavaScript and HTML, and a unidirectional data flow for managing the application state.
What are the differences between React components and HTML elements?
Sample Answer: React components are reusable and self-contained pieces of code that encapsulate their own logic and rendering. They allow for more dynamic and interactive behavior. On the other hand, HTML elements are pre-defined tags provided by the browser that represent different parts of a web page. While React components can be used to render HTML elements, they offer additional functionality and flexibility.
Explain the concept of state and props in React.js.
Sample Answer: In React.js, the state represents the internal data of a component that can change over time. It allows components to manage and update their own data. Props, short for properties, are read-only and passed to a component from its parent component. Props allow for the transfer of data and behavior from one component to another.
What are functional components and class components? When would you use each?
Sample Answer: Functional components are JavaScript functions that return React elements. They are simpler, easier to read, and recommended for most scenarios. Class components, on the other hand, are created using ES6 classes and extend the React.Component class. They have additional features like lifecycle methods and state management. Class components are suitable when you need more advanced functionality like local state or lifecycle methods.
How do you pass data between parent and child components?
Sample Answer: Data can be passed from a parent component to a child component by using props. The parent component can pass data as attributes to the child component when rendering it. The child component can then access this data through its props. If the child component needs to communicate back to the parent, it can do so by using callback functions passed as props.
Also Read: How to make an outstanding React.js resume?
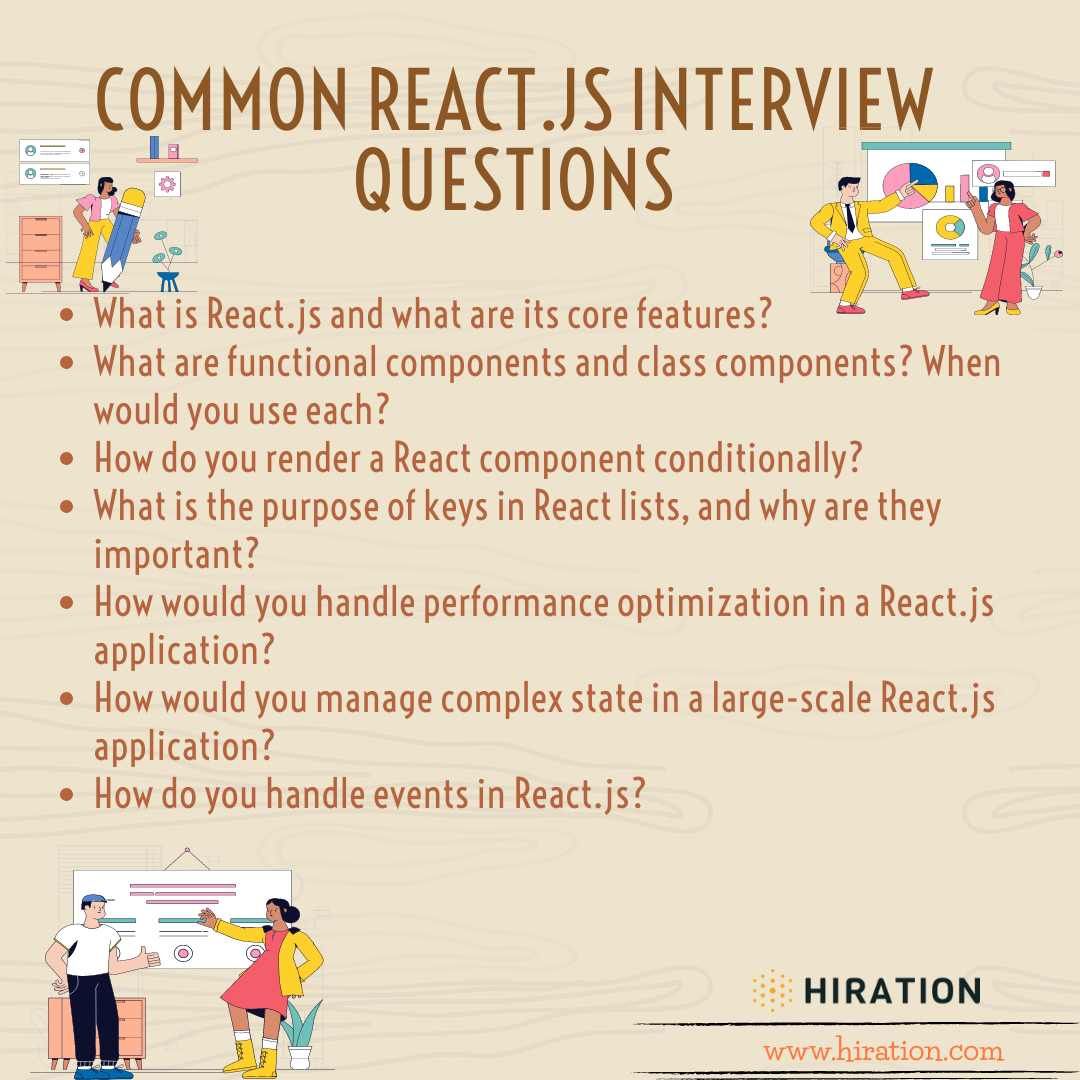
Top React.js Coding Interview Questions and Answers
React.js coding interview questions refer to the most commonly asked coding-related queries in interviews for React.js development positions, covering a range of topics.
Interviewers ask top React.js coding interview questions to assess a candidate's technical proficiency, problem-solving abilities, and familiarity with React.js best practices.
By asking these questions, interviewers can evaluate a candidate's suitability for the role and assess their potential to contribute effectively to React.js development projects.
Listed below are some of the most commonly asked coding interview questions on React.js:
How do you render a React component conditionally?
Sample Answer: To conditionally render a React component, you can use JavaScript's conditional statements or the ternary operator within the component's render method. For example, you can use an if statement or a ternary operator to check a condition and return different JSX based on the result.
How can you manage state in a functional component?
Sample Answer: In React, you can use the useState hook to manage the state in functional components. By importing the useState hook from the 'react' package, you can declare a state variable and a corresponding setter function. This allows you to update and access the state value within the functional component.
How do you handle forms in React?
Sample Answer: In React, you can handle forms by attaching event handlers to form elements and managing the form data in the component's state. By using controlled components, you can bind form elements to state variables and update the state as the user interacts with the form.
What is the purpose of keys in React lists, and why are they important?
Sample Answer: Keys are used in React lists to help identify each item uniquely and optimize the rendering process. They enable React to efficiently update and reorder elements in lists by providing a stable identity for each item. Keys help improve performance and avoid unnecessary re-rendering of list items.
How can you optimize the performance of a React application?
Sample Answer: To optimize React application performance, you can implement techniques like code splitting, memoization, and shouldComponentUpdate or React.memo to prevent unnecessary re-rendering. Additionally, you can leverage React's PureComponent or useMemo hook to optimize the rendering of components and avoid unnecessary computations.
Remember, that it's essential to elaborate and provide additional context during an actual interview. Interviewers may have follow-up questions or variations of these questions to further assess your understanding of React.js concepts and your ability to apply them in practice.
Also Read: What is the job description of a Java developer in 2023?
React.js Advanced Interview Questions for Senior Developers
React.js advanced interview questions are more in-depth and challenging questions specifically designed to evaluate the comprehensive knowledge, expertise, and problem-solving skills of experienced React.js developers.
They aim to assess a senior developer's ability to apply advanced techniques, make informed decisions, and demonstrate a deep understanding of React.js principles and best practices.
Here are some advanced React.js interview questions with sample answers for seasoned professionals:
How would you handle performance optimization in a React.js application?
Sample Answer: Performance optimization in React.js can be achieved through techniques like code splitting, memoization, virtualization, and using the shouldComponentUpdate method or React.memo to prevent unnecessary re-rendering. Additionally, optimizing network requests, utilizing the React Profiler, and employing lazy loading can further enhance performance.
Explain the concept of higher-order components (HOC) and how they can be used in React.js.
Sample Answer: Higher-order components are functions that take a component and return an enhanced version of that component. They enable code reuse, separation of concerns, and provide additional functionality to wrapped components. HOCs can be used for tasks like handling authentication, implementing data fetching, or adding additional props to components.
How would you manage complex state in a large-scale React.js application?
Sample Answer: In a large-scale application, state management libraries like Redux or MobX can be used to handle complex state. These libraries provide a centralized state store, reducers, and actions to manage and update state across components. By adopting a predictable state management approach, it becomes easier to track, test, and debug application state.
Explain the differences between controlled and uncontrolled components in React.js.
Sample Answer: Controlled components in React.js are those where form data is controlled by React state. The component's state is updated with every user input. In contrast, uncontrolled components allow form data to be handled by the DOM itself, and the component doesn't manage its own state. Controlled components offer more control and validation, while uncontrolled components can be useful for simple forms.
How would you implement server-side rendering (SSR) in a React.js application?
Sample Answer: Server-side rendering in React.js involves rendering components on the server and sending the HTML to the client, providing faster initial page load and improved SEO. Implementing SSR requires setting up a server-side rendering framework, handling data fetching on the server, and synchronizing the client and server renderings to ensure a consistent UI.
Also Read: How to become a Java developer in 2023?
React.js Interview Questions and Answers for Freshers
Interviewers often ask basic React.js questions to freshers that cover fundamental concepts, syntax, and core principles of React.js.
Given below are some of the most common React.js interview questions asked by recruiters to freshers:
How do you handle events in React.js?
Sample Answer: In React.js, event handling involves attaching event handlers to elements and defining corresponding callback functions. We can use the onClick attribute (or other event attributes) to specify the event and provide a function that will be executed when the event occurs. For example, onClick={handleClick} would call the handleClick function when the element is clicked.
Explain the concept of conditional rendering in React.js and provide an example.
Sample Answer: Conditional rendering in React.js allows you to show or hide components or elements based on certain conditions. You can use JavaScript's conditional statements or the ternary operator within the component's render method to conditionally render content. For example, you can use an if statement or a ternary operator to check a condition and return different JSX based on the result.
How would you handle component communication in React.js?
Sample Answer: React.js offers several ways to handle component communication. Parent-to-child communication can be achieved by passing data through props. Child-to-parent communication can be accomplished by passing callback functions as props. For complex scenarios involving communication between unrelated components, you can use state management libraries like Redux or Context API.
Also Read: What are some of the best entry-level Java developer jobs?
How to Answer React.js Interview Questions?
When it comes to answering React.js interview questions, preparation, and the right approach are key.
Here are some tips to help you tackle React.js interview questions the right way and increase your chances of success:
Understand the Question: Take the time to fully comprehend the question before jumping into an answer. Pay attention to any specific requirements or constraints mentioned.
Provide Clear and Concise Responses: Aim to provide clear and concise answers that directly address the question. Avoid going off on tangents or providing unnecessary information.
Showcase Your Knowledge: Demonstrate a deep understanding of React.js concepts, such as virtual DOM, component-based architecture, and state management. Use relevant examples or real-life scenarios to support your answers.
Practice Hands-on Coding: Some interviews may include coding exercises or challenges. Practice coding React.js components and solving common problems to enhance your coding skills and efficiency.
Explain Your Thought Process: When solving a problem or answering a complex question, explain your thought process step by step. This allows the interviewer to understand your approach and problem-solving abilities.
Be Honest About Your Limitations: It's okay if you don't know the answer to every question. Instead of guessing or making up an answer, be honest about your limitations and express your willingness to learn and improve.
Showcase Your Experience: Share relevant examples from your previous React.js projects or contributions to open-source projects. Discuss the challenges you faced and how you overcame them, highlighting your problem-solving abilities.
Stay Up-to-Date: Keep yourself updated with the latest trends, best practices, and new features in React.js. Stay informed about React.js ecosystem tools and libraries, as well as any updates in the React.js community.
Ask Questions: At the end of the interview, don't hesitate to ask thoughtful questions about the company's React.js development process, team structure, or future projects. This shows your interest and engagement.
Also Read: How much do java developers make?
FAQs on React.js Interview Questions
How do i take a reactjs interview.
To conduct a React.js interview, you can assess a candidate's knowledge by asking a combination of theoretical questions to gauge their understanding of React.js concepts and practical questions that require them to write code or solve problems related to React.js development.
What is DOM in React?
In React, the DOM (Document Object Model) represents the structure of the web page, and React uses a virtual DOM to efficiently update and render the actual DOM based on changes in the application's state.
What questions are asked in the TCS interview on ReactJS?
In TCS interviews for React.js, you can expect questions related to React.js concepts such as components, state management, lifecycle methods, JSX syntax, virtual DOM, and the usage of React.js libraries and tools. Additionally, TCS may also inquire about your experience with React.js projects, problem-solving abilities, and your understanding of best practices in React.js development.
What are props in React?
In React, props (short for properties) are used to pass data from a parent component to a child component, allowing the child component to access and use that data within its own scope.
Want to prepare for your upcoming React.js interview questions with professional help? Use Hiration’s ChatGPT-powered Interview Preparation Tool with 24x7 chat support.
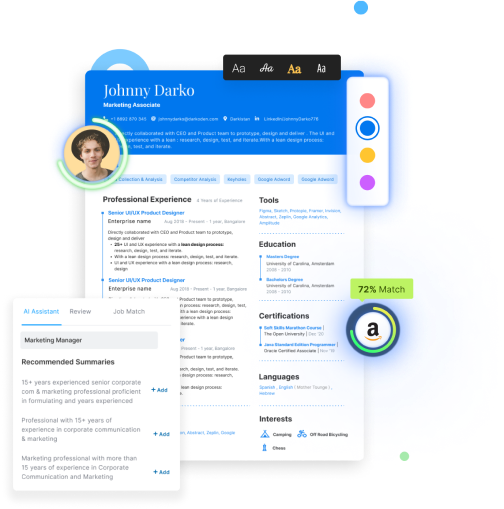
Share this blog
Subscribe to Free Resume Writing Blog by Hiration
Get the latest posts delivered right to your inbox
Stay up to date! Get all the latest & greatest posts delivered straight to your inbox
Is Your Resume ATS Friendly To Get Shortlisted?
Upload your resume for a free expert review.

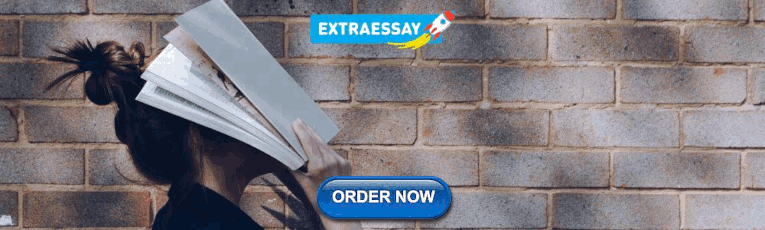
IMAGES
VIDEO
COMMENTS
Job Interview Preparation Materials (22 Part Series) 1 JavaScript Interview Questions and Answers you should know - Junior and Senior 2 CSS and HTML Interview Questions and Answers [2022] ... 18 more parts... 21 CSS Interview Question: Create Responsive Design (1 Column / 3 Column) 22 CSS Interview Question: Center HTML Element (3 Approaches ...
Question 4. Develop a currency converter application that allows users to input an amount in one currency and convert it to another. For the sake of this challenge, you can use a hard-coded exchange rate. Take advantage of React state and event handlers to manage the input and conversion calculations. Question 5
3. Display a list in React. React code to print each item from the list on the page using Array.map() function to display each item on the page. The list of items is displayed using React JS through the following steps: Declare list of items as JS Array. Access each item using Array.map() Return JSX code in callback function for every item.
React has been on a roll for a good 5 years now, and currently there is no end in sight. It keeps evolving. The average React Js Developer salary in USA is $125,000 per year or $64.10 per hour. Entry level positions start at $63,050 per year while most experienced workers make up to $195,000 per year. Follow along to learn most advanced React Interview Questions for your next tech interview.
To master React, it's crucial to practice your skills with real-world scenarios and practical coding exercises. In this blog, we present 50 practical practice questions that range from beginner to advanced levels. These questions will help you hone your React skills and enhance your problem-solving abilities while working with code.
21. What is a state in React? The state is a built-in React object that is used to contain data or information about the component. The state in a component can change over time, and whenever it changes, the component re-renders. The change in state can happen as a response to user action or system-generated events.
10. Explain what a mounted component is. A mounted component is one that has been initialized, added to the DOM, and is visible to the user. 11. Explain what useState is. useState is a Hook in React used to add state to functional components, enabling them to manage and display dynamic data. 12.
To help you prepare for code challenges, this article offers: 4 practical React coding challenges covering skills like CSS, simple state management, API data handling, and debugging existing codebases. Insight into employers' objectives for coding challenges. Guidance on tackling coding challenges during interviews.
Explain the Virtual DOM, and a pragmatic overview of how React renders it to the DOM. View answer. 2. Explain the standard JavaScript toolchain, transpilation (via Babel or other compilers), JSX, and these items' significance in recent development.
React is a JavaScript library for creating user interfaces. It's used in over 30,000 live websites and has over 70,000 GitHub stars. According to the 2021 Stack Overflow developer survey, React has surpassed jQuery as the most popular web framework, and holds approximately 40.14% of the market share. React was also the most sought-after, with ...
Problem-solving: Expect problem-solving scenarios or algorithmic questions. Approach these challenges systematically, explaining your approach and considering trade-offs.
This is because, like CSS, they are well understood by developers and are often already in use if React is being integrated into a legacy application. CSS-in-JS Modules (Styled Components, Emotion, and Styled-jsx) CSS-in-JS modules are a popular option for styling React applications because they integrate closely with React components.
React, a JavaScript library for building user interfaces, provides several hooks to manage state in functional components. One of the most commonly used hooks is useState, which allows developers to add state to their functional components. In this blog post, we will explore 20 practical practice questions that cover a range of scenarios, from ...
Benefits of React Exercises. Interactive Quizzes: Engage in hands-on React quizzes. Progress Tracking: Monitor your learning journey. Skill Enhancement: Sharpen coding skills effectively. Flexible Learning: Practice at your own pace. Immediate Feedback: Receive instant results and feedback. Convenient Accessibility: Accessible online, anytime.
You signed in with another tab or window. Reload to refresh your session. You signed out in another tab or window. Reload to refresh your session. You switched accounts on another tab or window.
With more than 40 interactive, real-world challenges, you will test your understanding of React's fundamental concepts and enhance your ability to solve problems. Every challenge in the series is designed to mimic a real coding scenario, providing a practical and hands-on approach for an effective learning experience.
Build a paginated Pokemons list with a "Load more" button - starting from failing unit tests! Practice React by fixing tests - Check your JSX knowledge! Coding exercises to improve your React skills. Detailed solutions to check your work and get feedback, fast.
Home; Challenges; Author; Contribute; Explore practical challenges and more Explore Challenges;;
As React gains prominence, so does the demand for skilled ReactJS developers who can harness its power to craft seamless, interactive web applications. The ReactJS interview process is a pivotal gateway to these opportunities, testing candidates on their theoretical knowledge, coding prowess, and problem-solving acumen.
Through a series of coding challenges, you'll develop the problem-solving skills crucial for interview success. Whether you're aiming for a junior, mid-level, or senior React.js position, this course will empower you with the skills and confidence to tackle any React.js interview question that comes your way.
Employ the use of practical React focused programming exercises for React developers to allow them assess and improve their coding skills. Conduct React-focused live coding interviews in which candidates can exhibit their coding skills, problem-solving talents, and understanding of React fundamentals.
React.js advanced interview questions are more in-depth and challenging questions specifically designed to evaluate the comprehensive knowledge, expertise, and problem-solving skills of experienced React.js developers. They aim to assess a senior developer's ability to apply advanced techniques, make informed decisions, and demonstrate a deep ...
Boost your coding interview skills and confidence by practicing real interview questions with LeetCode. Our platform offers a range of essential problems for practice, as well as the latest questions being asked by top-tier companies. ... Interview. Store Study Plan. See all. Array 1600. String 681. Hash Table 570. Dynamic Programming 487. Math ...