
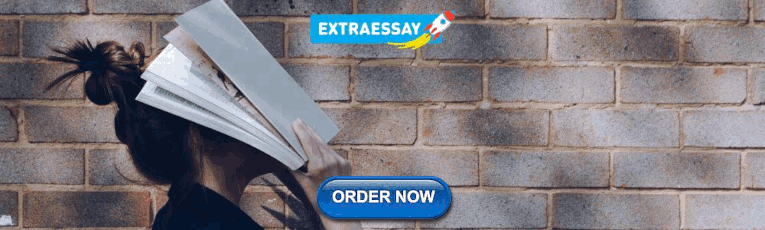
JAVASCRIPT ASSIGNMENT OPERATORS
In this tutorial, you will learn about all the different assignment operators in javascript and how to use them in javascript.
Assignment Operators
In javascript, there are 16 different assignment operators that are used to assign value to the variable. It is shorthand of other operators which is recommended to use.
The assignment operators are used to assign value based on the right operand to its left operand.
The left operand must be a variable while the right operand may be a variable, number, boolean, string, expression, object, or combination of any other.
One of the most basic assignment operators is equal = , which is used to directly assign a value.
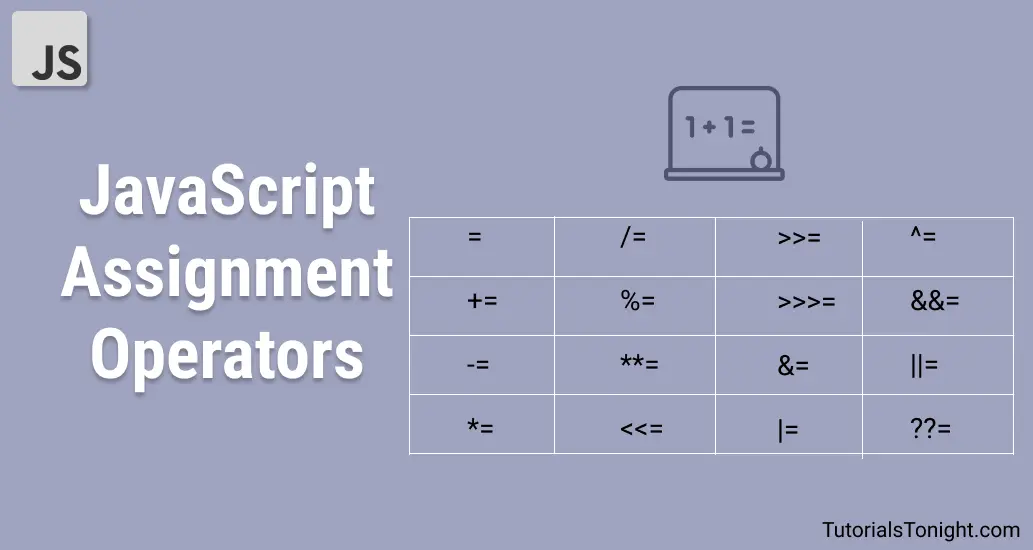
Assignment Operators List
Here is the list of all assignment operators in JavaScript:
In the following table if variable a is not defined then assume it to be 10.
Assignment operator
The assignment operator = is the simplest value assigning operator which assigns a given value to a variable.
The assignment operators support chaining, which means you can assign a single value in multiple variables in a single line.
Addition assignment operator
The addition assignment operator += is used to add the value of the right operand to the value of the left operand and assigns the result to the left operand.
On the basis of the data type of variable, the addition assignment operator may add or concatenate the variables.
Subtraction assignment operator
The subtraction assignment operator -= subtracts the value of the right operand from the value of the left operand and assigns the result to the left operand.
If the value can not be subtracted then it results in a NaN .
Multiplication assignment operator
The multiplication assignment operator *= assigns the result to the left operand after multiplying values of the left and right operand.
Division assignment operator
The division assignment operator /= divides the value of the left operand by the value of the right operand and assigns the result to the left operand.
Remainder assignment operator
The remainder assignment operator %= assigns the remainder to the left operand after dividing the value of the left operand by the value of the right operand.
Exponentiation assignment operator
The exponential assignment operator **= assigns the result of exponentiation to the left operand after exponentiating the value of the left operand by the value of the right operand.
Left shift assignment
The left shift assignment operator <<= assigns the result of the left shift to the left operand after shifting the value of the left operand by the value of the right operand.
Right shift assignment
The right shift assignment operator >>= assigns the result of the right shift to the left operand after shifting the value of the left operand by the value of the right operand.
Unsigned right shift assignment
The unsigned right shift assignment operator >>>= assigns the result of the unsigned right shift to the left operand after shifting the value of the left operand by the value of the right operand.
Bitwise AND assignment
The bitwise AND assignment operator &= assigns the result of bitwise AND to the left operand after ANDing the value of the left operand by the value of the right operand.
Bitwise OR assignment
The bitwise OR assignment operator |= assigns the result of bitwise OR to the left operand after ORing the value of left operand by the value of the right operand.
Bitwise XOR assignment
The bitwise XOR assignment operator ^= assigns the result of bitwise XOR to the left operand after XORing the value of the left operand by the value of the right operand.
Logical AND assignment
The logical AND assignment operator &&= assigns value to left operand only when it is truthy .
Note : A truthy value is a value that is considered true when encountered in a boolean context.
Logical OR assignment
The logical OR assignment operator ||= assigns value to left operand only when it is falsy .
Note : A falsy value is a value that is considered false when encountered in a boolean context.
Logical nullish assignment
The logical nullish assignment operator ??= assigns value to left operand only when it is nullish ( null or undefined ).
Home » JavaScript Tutorial » JavaScript Assignment Operators
JavaScript Assignment Operators
Summary : in this tutorial, you will learn how to use JavaScript assignment operators to assign a value to a variable.
Introduction to JavaScript assignment operators
An assignment operator ( = ) assigns a value to a variable. The syntax of the assignment operator is as follows:
In this syntax, JavaScript evaluates the expression b first and assigns the result to the variable a .
The following example declares the counter variable and initializes its value to zero:
The following example increases the counter variable by one and assigns the result to the counter variable:
When evaluating the second statement, JavaScript evaluates the expression on the right-hand first ( counter + 1 ) and assigns the result to the counter variable. After the second assignment, the counter variable is 1 .
To make the code more concise, you can use the += operator like this:
In this syntax, you don’t have to repeat the counter variable twice in the assignment.
The following table illustrates assignment operators that are shorthand for another operator and the assignment:
Chaining JavaScript assignment operators
If you want to assign a single value to multiple variables, you can chain the assignment operators. For example:
In this example, JavaScript evaluates from right to left. Therefore, it does the following:
- Use the assignment operator ( = ) to assign a value to a variable.
- Chain the assignment operators if you want to assign a single value to multiple variables.
Popular Tutorials
Popular examples, reference materials, learn python interactively, js introduction.
- Getting Started
- JS Variables & Constants
- JS console.log
- JavaScript Data types
JavaScript Operators
- JavaScript Comments
- JS Type Conversions
JS Control Flow
- JS Comparison Operators
- JavaScript if else Statement
- JavaScript for loop
- JavaScript while loop
- JavaScript break Statement
- JavaScript continue Statement
- JavaScript switch Statement
JS Functions
- JavaScript Function
- Variable Scope
- JavaScript Hoisting
- JavaScript Recursion
- JavaScript Objects
- JavaScript Methods & this
- JavaScript Constructor
- JavaScript Getter and Setter
- JavaScript Prototype
- JavaScript Array
- JS Multidimensional Array
- JavaScript String
- JavaScript for...in loop
- JavaScript Number
- JavaScript Symbol
Exceptions and Modules
- JavaScript try...catch...finally
- JavaScript throw Statement
- JavaScript Modules
- JavaScript ES6
- JavaScript Arrow Function
- JavaScript Default Parameters
- JavaScript Template Literals
- JavaScript Spread Operator
- JavaScript Map
- JavaScript Set
- Destructuring Assignment
- JavaScript Classes
- JavaScript Inheritance
- JavaScript for...of
- JavaScript Proxies
JavaScript Asynchronous
- JavaScript setTimeout()
- JavaScript CallBack Function
- JavaScript Promise
- Javascript async/await
- JavaScript setInterval()
Miscellaneous
- JavaScript JSON
- JavaScript Date and Time
- JavaScript Closure
- JavaScript this
- JavaScript use strict
- Iterators and Iterables
- JavaScript Generators
- JavaScript Regular Expressions
- JavaScript Browser Debugging
- Uses of JavaScript
JavaScript Tutorials
JavaScript Comparison and Logical Operators
JavaScript Booleans
JavaScript Bitwise Operators
JavaScript Ternary Operator
- JavaScript Object.is()
- JavaScript console.log()
JavaScript operators are special symbols that perform unique operations on one or more operands (values). For example,
The + operator performs addition, we have used it here to add the operands 2 and 3 .
JavaScript Operator Types
Here is a list of different JavaScript operators you will learn in this tutorial:
- Arithmetic Operators
- Assignment Operators
- Comparison Operators
- Logical Operators
- Bitwise Operators
- String Operators
- Miscellaneous Operators
1. JavaScript Arithmetic Operators
We use arithmetic operators to perform arithmetic calculations like addition, subtraction, etc. For example,
Here, we used the - operator to subtract 3 from 5 .
Commonly Used Arithmetic Operators
Example 1: arithmetic operators in javascript, example 2: javascript increment and decrement operators.
To learn more about the increment and decrement operators, visit Increment ++ and Decrement -- Operators .
2. JavaScript Assignment Operators
We use assignment operators to assign values to variables. For example,
Here, we used the = operator to assign the value 5 to variable x .
Commonly Used Assignment Operators
Example 3: assignment operators in javascript, 3. javascript comparison operators.
We use comparison operators to compare two values and return a boolean value ( true or false ). For example,
Here, we have used the > comparison operator to check whether a (whose value is 3 ) is greater than b (whose value is 2 ).
Since 3 is greater than 2 , we get true as output.
Commonly Used Comparison Operators
Example 4: comparison operators in javascript.
The equality operators ( == and != ) convert both operands to the same type before comparing their values. For example,
Here, we used the == operator to compare the number 3 and the string 3 .
By default, JavaScript converts string 3 to number 3 and compares the values.
However, the strict equality operators ( === and !== ) do not convert operand types before comparing their values. For example,
Here, JavaScript didn't convert string 4 to number 4 before comparing their values.
Thus, the result is false , as number 4 isn't equal to string 4 .
4. JavaScript Logical Operators
We use logical operators to perform logical operations on boolean values. For example,
Here, && is the logical operator AND . Since both x < 6 and y < 5 are true , the combined result is true .
Commonly Used Logical Operators
Example 5: logical operators in javascript.
Note: We use comparison and logical operators in decision-making and loops. You will learn about them in detail in later tutorials.
We use bitwise operators to perform binary operations on integers.
Note: We rarely use bitwise operators in everyday programming. If you are interested, visit JavaScript Bitwise Operators to learn more.
6. JavaScript String Operators
In JavaScript, you can use the + operator to concatenate (join) two or more strings . For example,
Here, we used + on strings to perform concatenation. However, when we use + with numbers, it performs addition.
7. JavaScript Miscellaneous Operators
JavaScript has many more operators besides the ones we listed above.
Thus, we have categorized them as miscellaneous operators. You will learn them in detail in later tutorials.
Commonly Used Miscellaneous Operators
- JavaScript typeof Operator
Table of Contents
- Introduction
- JavaScript Arithmetic Operators
- JavaScript Assignment Operators
- JavaScript Comparison Operators
- JavaScript Logical Operators
- JavaScript String Operators
- JavaScript Miscellaneous Operators
Video: JavaScript Operators
Sorry about that.
Related Tutorials
JavaScript Tutorial
- Skip to main content
- Select language
- Skip to search
- Expressions and operators
- Operator precedence
Left-hand-side expressions
« Previous Next »
This chapter describes JavaScript's expressions and operators, including assignment, comparison, arithmetic, bitwise, logical, string, ternary and more.
A complete and detailed list of operators and expressions is also available in the reference .
JavaScript has the following types of operators. This section describes the operators and contains information about operator precedence.
- Assignment operators
- Comparison operators
- Arithmetic operators
- Bitwise operators
Logical operators
String operators, conditional (ternary) operator.
- Comma operator
Unary operators
- Relational operator
JavaScript has both binary and unary operators, and one special ternary operator, the conditional operator. A binary operator requires two operands, one before the operator and one after the operator:
For example, 3+4 or x*y .
A unary operator requires a single operand, either before or after the operator:
For example, x++ or ++x .
An assignment operator assigns a value to its left operand based on the value of its right operand. The simple assignment operator is equal ( = ), which assigns the value of its right operand to its left operand. That is, x = y assigns the value of y to x .
There are also compound assignment operators that are shorthand for the operations listed in the following table:
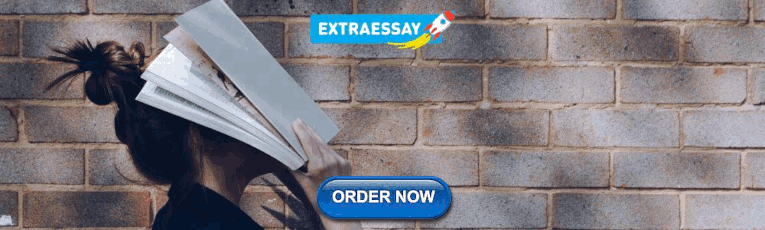
Destructuring
For more complex assignments, the destructuring assignment syntax is a JavaScript expression that makes it possible to extract data from arrays or objects using a syntax that mirrors the construction of array and object literals.
A comparison operator compares its operands and returns a logical value based on whether the comparison is true. The operands can be numerical, string, logical, or object values. Strings are compared based on standard lexicographical ordering, using Unicode values. In most cases, if the two operands are not of the same type, JavaScript attempts to convert them to an appropriate type for the comparison. This behavior generally results in comparing the operands numerically. The sole exceptions to type conversion within comparisons involve the === and !== operators, which perform strict equality and inequality comparisons. These operators do not attempt to convert the operands to compatible types before checking equality. The following table describes the comparison operators in terms of this sample code:
Note: ( => ) is not an operator, but the notation for Arrow functions .
An arithmetic operator takes numerical values (either literals or variables) as their operands and returns a single numerical value. The standard arithmetic operators are addition ( + ), subtraction ( - ), multiplication ( * ), and division ( / ). These operators work as they do in most other programming languages when used with floating point numbers (in particular, note that division by zero produces Infinity ). For example:
In addition to the standard arithmetic operations (+, -, * /), JavaScript provides the arithmetic operators listed in the following table:
A bitwise operator treats their operands as a set of 32 bits (zeros and ones), rather than as decimal, hexadecimal, or octal numbers. For example, the decimal number nine has a binary representation of 1001. Bitwise operators perform their operations on such binary representations, but they return standard JavaScript numerical values.
The following table summarizes JavaScript's bitwise operators.
Bitwise logical operators
Conceptually, the bitwise logical operators work as follows:
- The operands are converted to thirty-two-bit integers and expressed by a series of bits (zeros and ones). Numbers with more than 32 bits get their most significant bits discarded. For example, the following integer with more than 32 bits will be converted to a 32 bit integer: Before: 11100110111110100000000000000110000000000001 After: 10100000000000000110000000000001
- Each bit in the first operand is paired with the corresponding bit in the second operand: first bit to first bit, second bit to second bit, and so on.
- The operator is applied to each pair of bits, and the result is constructed bitwise.
For example, the binary representation of nine is 1001, and the binary representation of fifteen is 1111. So, when the bitwise operators are applied to these values, the results are as follows:
Note that all 32 bits are inverted using the Bitwise NOT operator, and that values with the most significant (left-most) bit set to 1 represent negative numbers (two's-complement representation).
Bitwise shift operators
The bitwise shift operators take two operands: the first is a quantity to be shifted, and the second specifies the number of bit positions by which the first operand is to be shifted. The direction of the shift operation is controlled by the operator used.
Shift operators convert their operands to thirty-two-bit integers and return a result of the same type as the left operand.
The shift operators are listed in the following table.
Logical operators are typically used with Boolean (logical) values; when they are, they return a Boolean value. However, the && and || operators actually return the value of one of the specified operands, so if these operators are used with non-Boolean values, they may return a non-Boolean value. The logical operators are described in the following table.
Examples of expressions that can be converted to false are those that evaluate to null, 0, NaN, the empty string (""), or undefined.
The following code shows examples of the && (logical AND) operator.
The following code shows examples of the || (logical OR) operator.
The following code shows examples of the ! (logical NOT) operator.
Short-circuit evaluation
As logical expressions are evaluated left to right, they are tested for possible "short-circuit" evaluation using the following rules:
- false && anything is short-circuit evaluated to false.
- true || anything is short-circuit evaluated to true.
The rules of logic guarantee that these evaluations are always correct. Note that the anything part of the above expressions is not evaluated, so any side effects of doing so do not take effect.
In addition to the comparison operators, which can be used on string values, the concatenation operator (+) concatenates two string values together, returning another string that is the union of the two operand strings.
For example,
The shorthand assignment operator += can also be used to concatenate strings.
The conditional operator is the only JavaScript operator that takes three operands. The operator can have one of two values based on a condition. The syntax is:
If condition is true, the operator has the value of val1 . Otherwise it has the value of val2 . You can use the conditional operator anywhere you would use a standard operator.
This statement assigns the value "adult" to the variable status if age is eighteen or more. Otherwise, it assigns the value "minor" to status .
The comma operator ( , ) simply evaluates both of its operands and returns the value of the last operand. This operator is primarily used inside a for loop, to allow multiple variables to be updated each time through the loop.
For example, if a is a 2-dimensional array with 10 elements on a side, the following code uses the comma operator to update two variables at once. The code prints the values of the diagonal elements in the array:
A unary operation is an operation with only one operand.
The delete operator deletes an object, an object's property, or an element at a specified index in an array. The syntax is:
where objectName is the name of an object, property is an existing property, and index is an integer representing the location of an element in an array.
The fourth form is legal only within a with statement, to delete a property from an object.
You can use the delete operator to delete variables declared implicitly but not those declared with the var statement.
If the delete operator succeeds, it sets the property or element to undefined . The delete operator returns true if the operation is possible; it returns false if the operation is not possible.
Deleting array elements
When you delete an array element, the array length is not affected. For example, if you delete a[3] , a[4] is still a[4] and a[3] is undefined.
When the delete operator removes an array element, that element is no longer in the array. In the following example, trees[3] is removed with delete . However, trees[3] is still addressable and returns undefined .
If you want an array element to exist but have an undefined value, use the undefined keyword instead of the delete operator. In the following example, trees[3] is assigned the value undefined , but the array element still exists:
The typeof operator is used in either of the following ways:
The typeof operator returns a string indicating the type of the unevaluated operand. operand is the string, variable, keyword, or object for which the type is to be returned. The parentheses are optional.
Suppose you define the following variables:
The typeof operator returns the following results for these variables:
For the keywords true and null , the typeof operator returns the following results:
For a number or string, the typeof operator returns the following results:
For property values, the typeof operator returns the type of value the property contains:
For methods and functions, the typeof operator returns results as follows:
For predefined objects, the typeof operator returns results as follows:
The void operator is used in either of the following ways:
The void operator specifies an expression to be evaluated without returning a value. expression is a JavaScript expression to evaluate. The parentheses surrounding the expression are optional, but it is good style to use them.
You can use the void operator to specify an expression as a hypertext link. The expression is evaluated but is not loaded in place of the current document.
The following code creates a hypertext link that does nothing when the user clicks it. When the user clicks the link, void(0) evaluates to undefined , which has no effect in JavaScript.
The following code creates a hypertext link that submits a form when the user clicks it.
Relational operators
A relational operator compares its operands and returns a Boolean value based on whether the comparison is true.
The in operator returns true if the specified property is in the specified object. The syntax is:
where propNameOrNumber is a string or numeric expression representing a property name or array index, and objectName is the name of an object.
The following examples show some uses of the in operator.
The instanceof operator returns true if the specified object is of the specified object type. The syntax is:
where objectName is the name of the object to compare to objectType , and objectType is an object type, such as Date or Array .
Use instanceof when you need to confirm the type of an object at runtime. For example, when catching exceptions, you can branch to different exception-handling code depending on the type of exception thrown.
For example, the following code uses instanceof to determine whether theDay is a Date object. Because theDay is a Date object, the statements in the if statement execute.
The precedence of operators determines the order they are applied when evaluating an expression. You can override operator precedence by using parentheses.
The following table describes the precedence of operators, from highest to lowest.
A more detailed version of this table, complete with links to additional details about each operator, may be found in JavaScript Reference .
- Expressions
An expression is any valid unit of code that resolves to a value.
Every syntactically valid expression resolves to some value but conceptually, there are two types of expressions: with side effects (for example: those that assign value to a variable) and those that in some sense evaluates and therefore resolves to value.
The expression x = 7 is an example of the first type. This expression uses the = operator to assign the value seven to the variable x . The expression itself evaluates to seven.
The code 3 + 4 is an example of the second expression type. This expression uses the + operator to add three and four together without assigning the result, seven, to a variable. JavaScript has the following expression categories:
- Arithmetic: evaluates to a number, for example 3.14159. (Generally uses arithmetic operators .)
- String: evaluates to a character string, for example, "Fred" or "234". (Generally uses string operators .)
- Logical: evaluates to true or false. (Often involves logical operators .)
- Primary expressions: Basic keywords and general expressions in JavaScript.
- Left-hand-side expressions: Left values are the destination of an assignment.
Primary expressions
Basic keywords and general expressions in JavaScript.
Use the this keyword to refer to the current object. In general, this refers to the calling object in a method. Use this either with the dot or the bracket notation:
Suppose a function called validate validates an object's value property, given the object and the high and low values:
You could call validate in each form element's onChange event handler, using this to pass it the form element, as in the following example:
- Grouping operator
The grouping operator ( ) controls the precedence of evaluation in expressions. For example, you can override multiplication and division first, then addition and subtraction to evaluate addition first.
Comprehensions
Comprehensions are an experimental JavaScript feature, targeted to be included in a future ECMAScript version. There are two versions of comprehensions:
Comprehensions exist in many programming languages and allow you to quickly assemble a new array based on an existing one, for example.
Left values are the destination of an assignment.
You can use the new operator to create an instance of a user-defined object type or of one of the built-in object types. Use new as follows:
The super keyword is used to call functions on an object's parent. It is useful with classes to call the parent constructor, for example.
Spread operator
The spread operator allows an expression to be expanded in places where multiple arguments (for function calls) or multiple elements (for array literals) are expected.
Example: Today if you have an array and want to create a new array with the existing one being part of it, the array literal syntax is no longer sufficient and you have to fall back to imperative code, using a combination of push , splice , concat , etc. With spread syntax this becomes much more succinct:
Similarly, the spread operator works with function calls:
Document Tags and Contributors
- l10n:priority
- JavaScript basics
- JavaScript first steps
- JavaScript building blocks
- Introducing JavaScript objects
- Introduction
- Grammar and types
- Control flow and error handling
- Loops and iteration
- Numbers and dates
- Text formatting
- Regular expressions
- Indexed collections
- Keyed collections
- Working with objects
- Details of the object model
- Iterators and generators
- Meta programming
- A re-introduction to JavaScript
- JavaScript data structures
- Equality comparisons and sameness
- Inheritance and the prototype chain
- Strict mode
- JavaScript typed arrays
- Memory Management
- Concurrency model and Event Loop
- References:
- ArrayBuffer
- AsyncFunction
- Float32Array
- Float64Array
- GeneratorFunction
- InternalError
- Intl.Collator
- Intl.DateTimeFormat
- Intl.NumberFormat
- ParallelArray
- ReferenceError
- SIMD.Bool16x8
- SIMD.Bool32x4
- SIMD.Bool64x2
- SIMD.Bool8x16
- SIMD.Float32x4
- SIMD.Float64x2
- SIMD.Int16x8
- SIMD.Int32x4
- SIMD.Int8x16
- SIMD.Uint16x8
- SIMD.Uint32x4
- SIMD.Uint8x16
- SharedArrayBuffer
- StopIteration
- SyntaxError
- Uint16Array
- Uint32Array
- Uint8ClampedArray
- WebAssembly
- decodeURI()
- decodeURIComponent()
- encodeURI()
- encodeURIComponent()
- parseFloat()
- Array comprehensions
- Conditional (ternary) Operator
- Destructuring assignment
- Expression closures
- Generator comprehensions
- Legacy generator function expression
- Logical Operators
- Object initializer
- Property accessors
- Spread syntax
- async function expression
- class expression
- delete operator
- function expression
- function* expression
- in operator
- new operator
- void operator
- Legacy generator function
- async function
- for each...in
- function declaration
- try...catch
- Arguments object
- Arrow functions
- Default parameters
- Method definitions
- Rest parameters
- constructor
- element loaded from a different domain for which you violated the same-origin policy.">Error: Permission denied to access property "x"
- InternalError: too much recursion
- RangeError: argument is not a valid code point
- RangeError: invalid array length
- RangeError: invalid date
- RangeError: precision is out of range
- RangeError: radix must be an integer
- RangeError: repeat count must be less than infinity
- RangeError: repeat count must be non-negative
- ReferenceError: "x" is not defined
- ReferenceError: assignment to undeclared variable "x"
- ReferenceError: can't access lexical declaration`X' before initialization
- ReferenceError: deprecated caller or arguments usage
- ReferenceError: invalid assignment left-hand side
- ReferenceError: reference to undefined property "x"
- SyntaxError: "0"-prefixed octal literals and octal escape seq. are deprecated
- SyntaxError: "use strict" not allowed in function with non-simple parameters
- SyntaxError: "x" is a reserved identifier
- SyntaxError: JSON.parse: bad parsing
- SyntaxError: Malformed formal parameter
- SyntaxError: Unexpected token
- SyntaxError: Using //@ to indicate sourceURL pragmas is deprecated. Use //# instead
- SyntaxError: a declaration in the head of a for-of loop can't have an initializer
- SyntaxError: applying the 'delete' operator to an unqualified name is deprecated
- SyntaxError: for-in loop head declarations may not have initializers
- SyntaxError: function statement requires a name
- SyntaxError: identifier starts immediately after numeric literal
- SyntaxError: illegal character
- SyntaxError: invalid regular expression flag "x"
- SyntaxError: missing ) after argument list
- SyntaxError: missing ) after condition
- SyntaxError: missing : after property id
- SyntaxError: missing ; before statement
- SyntaxError: missing = in const declaration
- SyntaxError: missing ] after element list
- SyntaxError: missing formal parameter
- SyntaxError: missing name after . operator
- SyntaxError: missing variable name
- SyntaxError: missing } after function body
- SyntaxError: missing } after property list
- SyntaxError: redeclaration of formal parameter "x"
- SyntaxError: return not in function
- SyntaxError: test for equality (==) mistyped as assignment (=)?
- SyntaxError: unterminated string literal
- TypeError: "x" has no properties
- TypeError: "x" is (not) "y"
- TypeError: "x" is not a constructor
- TypeError: "x" is not a function
- TypeError: "x" is not a non-null object
- TypeError: "x" is read-only
- TypeError: More arguments needed
- TypeError: can't access dead object
- TypeError: can't define property "x": "obj" is not extensible
- TypeError: can't delete non-configurable array element
- TypeError: can't redefine non-configurable property "x"
- TypeError: cyclic object value
- TypeError: invalid 'in' operand "x"
- TypeError: invalid Array.prototype.sort argument
- TypeError: invalid arguments
- TypeError: invalid assignment to const "x"
- TypeError: property "x" is non-configurable and can't be deleted
- TypeError: setting getter-only property "x"
- TypeError: variable "x" redeclares argument
- URIError: malformed URI sequence
- Warning: -file- is being assigned a //# sourceMappingURL, but already has one
- Warning: 08/09 is not a legal ECMA-262 octal constant
- Warning: Date.prototype.toLocaleFormat is deprecated
- Warning: JavaScript 1.6's for-each-in loops are deprecated
- Warning: String.x is deprecated; use String.prototype.x instead
- Warning: expression closures are deprecated
- Warning: unreachable code after return statement
- JavaScript technologies overview
- Lexical grammar
- Enumerability and ownership of properties
- Iteration protocols
- Transitioning to strict mode
- Template literals
- Deprecated features
- ECMAScript 2015 support in Mozilla
- ECMAScript 5 support in Mozilla
- ECMAScript Next support in Mozilla
- Firefox JavaScript changelog
- New in JavaScript 1.1
- New in JavaScript 1.2
- New in JavaScript 1.3
- New in JavaScript 1.4
- New in JavaScript 1.5
- New in JavaScript 1.6
- New in JavaScript 1.7
- New in JavaScript 1.8
- New in JavaScript 1.8.1
- New in JavaScript 1.8.5
- Documentation:
- All pages index
- Methods index
- Properties index
- Pages tagged "JavaScript"
- JavaScript doc status
- The MDN project
Best Programming Guides with Examples
Subscribe to Blog via Email
Enter your email address to subscribe to this blog and receive notifications of new posts by email.
Email Address
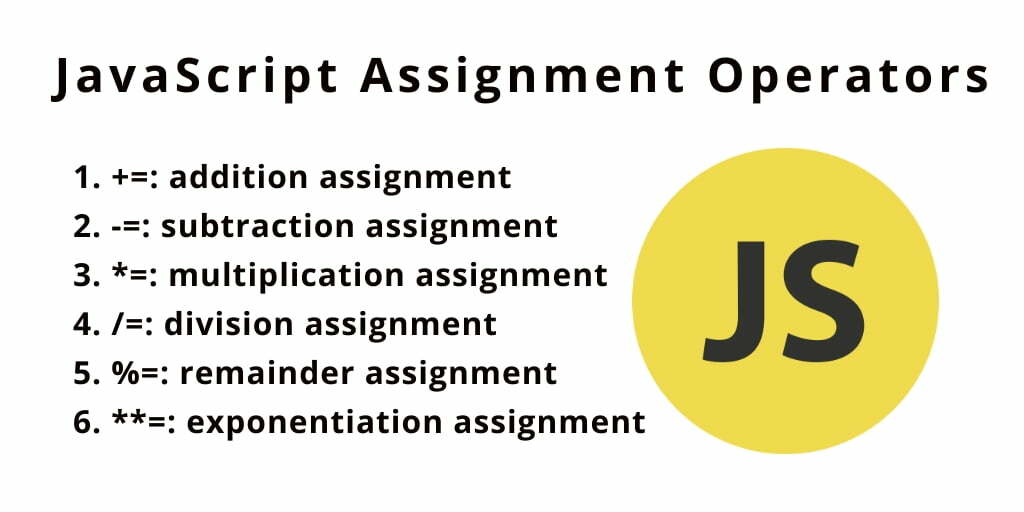
JavaScript Assignment Operators Example
Assignment operator in javascript with example; In this tutorial, you will learn about JavaScript assignment operators with the help of examples. And as well as learn how to assign a value of the right operand to its left operand in js using these assignment operators.
List of JS Assignment Operators
If you want to assign a value of the right(first) operand to its left(second) operand, you can use equal operator in js.
See the example of assignment operator is equal (=):
In this example, Assigned the number 15 to the variable x.
See another example for better understanding:
It’s equivalent to the above example:
JS assignment operator has several shortcuts for performing all the arithmetic operations , which let you assign to the right operand the left operand.
Here, you can see that examples of the following assignment operators:
- += : addition assignment
- -= : subtraction assignment
- *= : multiplication assignment
- /= : division assignment
- %= : remainder assignment
- **= : exponentiation assignment
See the following example:
In this tutorial, you have learned how to use JavaScript assignment operators.
Recommended JavaScript Tutorial
Author Admin
Greetings, I'm Devendra Dode, a full-stack developer, entrepreneur, and the proud owner of Tutsmake.com. My passion lies in crafting informative tutorials and offering valuable tips to assist fellow developers on their coding journey. Within my content, I cover a spectrum of technologies, including PHP, Python, JavaScript, jQuery, Laravel, Livewire, CodeIgniter, Node.js, Express.js, Vue.js, Angular.js, React.js, MySQL, MongoDB, REST APIs, Windows, XAMPP, Linux, Ubuntu, Amazon AWS, Composer, SEO, WordPress, SSL, and Bootstrap. Whether you're starting out or looking for advanced examples, I provide step-by-step guides and practical demonstrations to make your learning experience seamless. Let's explore the diverse realms of coding together.
View all posts by Admin
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
Assignment Operator in JavaScript with Example
An operator that is used to assign/update the value of a variable is called assignment operator in JavaScript .
The most common assignment operator is equal operator “=”, which assigns the right-hand value to the left-hand variable.
The general syntax is as follows:
We can assign a primitive type variable using an integer literal value or the result of an expression.
Types of Assignment Operator in JavaScript
We can classify an assignment operator in JavaScript into three major categories. They are as follows:
- Simple assignment
- Compound assignment
- Assignment as expression
Simple Assignment
A simple assignment operator can be used to store or assign a value into a variable or to store a value of a variable into another variable. The general form to represent a simple assignment is given below:
In the above syntax,
- variable: It is the name of a variable that represents a memory location where a value may be stored.
- expression: It may be a constant, variable, or a combination of constants, variables, and operators.
- = is an assignment operator.
Let’s understand it with the help of some examples.
Compound Assignment Operators
The compound assignment operators consist of a simple assignment operator with another binary operator. The compound assignment operator is also known as shorthand operator in JavaScript. The general syntax is as:
1. Addition assignment (+=):
2. Subtraction assignment (-=):
3. Multiplication assignment (*=):
4. Division assignment (/=):
5. Remainder assignment (%=):
Let’s create a JavaScript program based on the compound assignment operators.
Program code 8:
1. The expression x += y; will be evaluated in the following steps:
2. The expression y -= x + z; is equivalent to y = y – (x + z);
3. The expression z *= x * y; is equivalent to z = z * (x * y);
Assignment as Expression
In JavaScript, we can also consider an assignment operation an expression because the operation has a result. The outcome (or result) of an expression is a value that is stored in a variable. For example:
Here, the expression b – c + 4 is evaluated first, and then its result is stored into the variable a.
Program code 9:
Explanation :
The following steps involved to evaluate the above expressions of the JavaScript program.
1. Evaluation of expression: a += 1;
2. Evaluation of expression: b -= 1;
3. Evaluation of expression: c *= 2;
4. Evaluation of x = (10 + a);
5. Evaluation of y = x + 100;
6. Evaluation of z = x + y + c;
Try It Yourself
Program code 10:
Program code 11:
In this tutorial, you learned assignment operators in JavaScript and its types with example programs. Hope that you will have understood the basic points of assignment operators.
In the next tutorial, we will learn comparison operators in JavaScript with different example programs. Thanks for reading!!! Next ⇒ Comparison Operators in JavaScript ⇐ Prev Next ⇒

JavaScript Tutorial
Javascript basics, javascript objects, javascript bom, javascript dom, javascript validation, javascript oops, javascript cookies, javascript events, exception handling, javascript misc, javascript advance, differences.
Interview Questions
Logical AND assignment operator (&&=)
The &&= symbol is a "Logical AND assignment operator" and connects two variables. If the initial value is correct, the second value is used. It is graded from left to right.
The following syntax shows the Logical AND assignment operator with the two values.
The examples work with multiple values using the "Logical AND assignment operator" in the javascript.
The following example shows basic values for the "AND assignment operator" in javascript.
The image shows the "logical AND assignment operator's data" as an output.
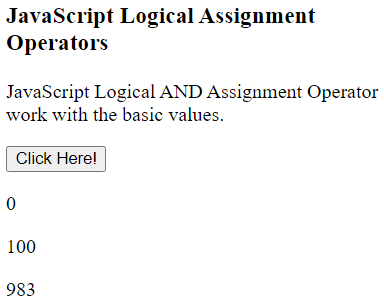
The following example shows the "AND assignment operator" hash in javascript.
The image shows the "logical and assignment operator's data" as an output.
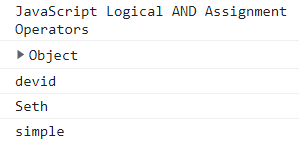
The following example shows an array for the "AND assignment operator" in javascript.
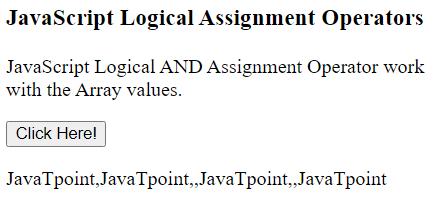
Logical OR assignment operator (||=)
The ||= is a "logical OR assignment operator" between two values. If the initial value is incorrect, the second value is used. It is graded from left to right.
The following syntax shows the Logical OR assignment operator with the two values.
The examples work with multiple values using the " Logical OR assignment operator" in the javascript.
The following example shows basic values for the "Logical OR assignment" in javascript. Here, We can use simple numeric values.
The image shows the "logical OR assignment operator's data" as an output.
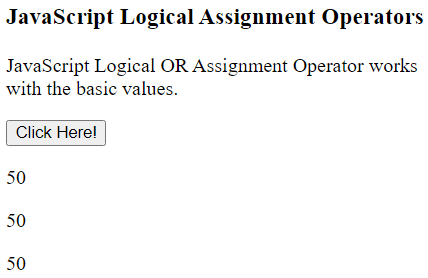
The following example shows the "OR assignment operator" hash in javascript.
The image shows the " Logical OR assignment operator's data" as an output.
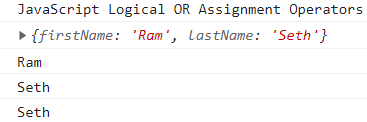
The following example shows arrays for the "OR assignment operator" in javascript.
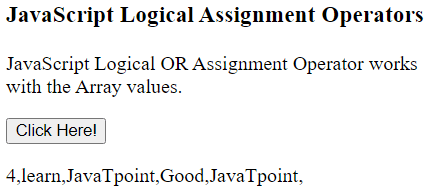
Nullish coalescing assignment operator (??=)
The ??= is a "Nullish coalescing assignment operator" between two values. The second value is assigned if the first value is undefined or null. It is graded from left to right.
The following syntax shows the Logical nullish assignment operator with the two values.
The examples work with multiple values using the " Logical nullish assignment operator" in the javascript.
The following example shows basic values for the "Logical nullish assignment" in javascript. Here, We can use simple numeric values.
The image shows the "logical nullish assignment operator's data" as an output.
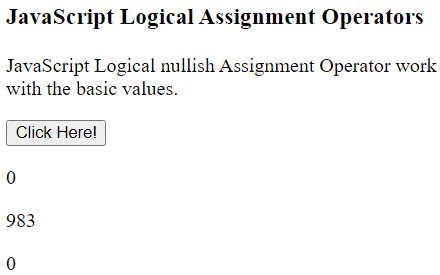
The following example shows hash values for the "Logical nullish assignment" in javascript.
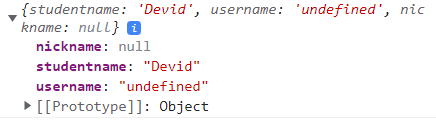
The following example shows array values for the "Logical nullish assignment" in javascript. The nullish operator uses the null and the "jtp" value to show functionality.
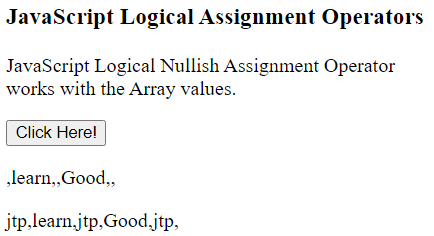
The javascript logical assignment operators help to operate logical conditions between two inputs in all data formats. The "and", "or", and nullish logical operation works by these operators in the javascript language.

- Send your Feedback to [email protected]
Help Others, Please Share

Learn Latest Tutorials

Transact-SQL

Reinforcement Learning

R Programming

React Native

Python Design Patterns

Python Pillow

Python Turtle

Preparation

Verbal Ability

Company Questions
Trending Technologies

Artificial Intelligence

Cloud Computing

Data Science

Machine Learning

B.Tech / MCA

Data Structures

Operating System

Computer Network

Compiler Design

Computer Organization

Discrete Mathematics

Ethical Hacking

Computer Graphics

Software Engineering

Web Technology

Cyber Security

C Programming

Control System

Data Mining

Data Warehouse

- Implementing Joint Venture Management
Example of Setting Up and Processing Assignment Rules
This example shows how assignment rules can be set up to assign different ownership definitions to different types of transactions in a joint venture.
In this example, a joint operating agreement includes terms for splitting costs and revenue among the managing partner and the three partners of a joint venture. Most of the expenses and revenue have the same terms for the split, except for costs related to site materials, maintenance, and labor. In the agreement, each of these types of costs has a different percentage split.
The managing partner creates a joint venture definition with the following details:
- Identification of distributable accounts.
Before creating assignment rules to assign ownership definitions to cost transactions, the managing partner sets up account sets to use in the assignment rules. The account sets identify the following accounts:
- Material accounts: 515600–515950
- Maintenance accounts: 525400–525410
- Labor accounts: 536051–536100
Next, the managing partner creates the following assignment rules, associating each account set to the appropriate ownership definition:
After activating the joint venture definition, account sets, and assignment rules, the managing partner sets up the Identify Joint Venture Transactions process to process transactions for the joint venture. The process uses the primary segment values in the joint venture definition to identify all joint venture costs and revenue for distribution. It then processes the assignment rules according to the sequence number and assigns ownership definitions to each type of cost transaction according to the details in the assignment rules.
This is a simplified scenario, but you can also set up assignment rules to accommodate more complex joint operating agreements. You can set up an assignment rule to assign a single stakeholder to a set of transactions. Or if your implementation of Oracle Joint Venture Management is integrated with Oracle Project Costing, you can set up assignment rules to assign ownership definitions or stakeholders to transactions based on particular project details in transactions.
- DSA with JS - Self Paced
- JS Tutorial
- JS Exercise
- JS Interview Questions
- JS Operator
- JS Projects
- JS Examples
- JS Free JS Course
- JS A to Z Guide
- JS Formatter
JS Arithmetic Operators
- Addition(+) Arithmetic Operator in JavaScript
- Subtraction(-) Arithmetic Operator in JavaScript
- Multiplication(*) Arithmetic Operator in JavaScript
- Division(/) Arithmetic Operator in JavaScript
- Modulus(%) Arithmetic Operator in JavaScript
- Exponentiation(**) Arithmetic Operator in JavaScript
- Increment(+ +) Arithmetic Operator in JavaScript
- Decrement(--) Arithmetic Operator in JavaScript
- JavaScript Arithmetic Unary Plus(+) Operator
- JavaScript Arithmetic Unary Negation(-) Operator
JS Assignment Operators
- Addition Assignment (+=) Operator in Javascript
- Subtraction Assignment( -=) Operator in Javascript
- Multiplication Assignment(*=) Operator in JavaScript
- Division Assignment(/=) Operator in JavaScript
- JavaScript Remainder Assignment(%=) Operator
- Exponentiation Assignment(**=) Operator in JavaScript
- Left Shift Assignment (<<=) Operator in JavaScript
Right Shift Assignment(>>=) Operator in JavaScript
- Bitwise AND Assignment (&=) Operator in JavaScript
- Bitwise OR Assignment (|=) Operator in JavaScript
- Bitwise XOR Assignment (^=) Operator in JavaScript
- JavaScript Logical AND assignment (&&=) Operator
- JavaScript Logical OR assignment (||=) Operator
- Nullish Coalescing Assignment (??=) Operator in JavaScript
JS Comparison Operators
- Equality(==) Comparison Operator in JavaScript
- Inequality(!=) Comparison Operator in JavaScript
- Strict Equality(===) Comparison Operator in JavaScript
- Strict Inequality(!==) Comparison Operator in JavaScript
- Greater than(>) Comparison Operator in JavaScript
- Greater Than or Equal(>=) Comparison Operator in JavaScript
- Less Than or Equal(
JS Logical Operators
- NOT(!) Logical Operator inJavaScript
- AND(&&) Logical Operator in JavaScript
- OR(||) Logical Operator in JavaScript
JS Bitwise Operators
- AND(&) Bitwise Operator in JavaScript
- OR(|) Bitwise Operator in JavaScript
- XOR(^) Bitwise Operator in JavaScript
- NOT(~) Bitwise Operator in JavaScript
- Left Shift (
- Right Shift (>>) Bitwise Operator in JavaScript
- Zero Fill Right Shift (>>>) Bitwise Operator in JavaScript
JS Unary Operators
- JavaScript typeof Operator
- JavaScript delete Operator
JS Relational Operators
- JavaScript in Operator
- JavaScript Instanceof Operator
JS Other Operators
- JavaScript String Operators
- JavaScript yield Operator
- JavaScript Pipeline Operator
- JavaScript Grouping Operator
The Right Shift Assignment Operator is represented by “>>=”. This operator shifts the first operand to the right and assigns the result to the variable. It can also be explained as shifting the first operand to the right in a specified amount of bits which is the second operand integer and then assigning the result to the first operand.
Where –
- a is the first operand, and
- b is the second operand.
Example 1: In this example, we will see the implementation of the right shift assignment.
Example 2: In this example, we will see assigning the right shift operator to the variable.
We have a complete list of Javascript Assignment Operators, Please check this article Javascript Assignment Operator .
Supported Browser:
Please Login to comment...
Similar reads.
- Web Technologies
- Otter.ai vs. Fireflies.ai: Which AI Transcribes Meetings More Accurately?
- Google Chrome Will Soon Let You Talk to Gemini In The Address Bar
- AI Interior Designer vs. Virtual Home Decorator: Which AI Can Transform Your Home Into a Pinterest Dream Faster?
- Top 10 Free Webclipper on Chrome Browser in 2024
- 30 OOPs Interview Questions and Answers (2024)
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Go Tutorial
Go exercises, go assignment operators, assignment operators.
Assignment operators are used to assign values to variables.
In the example below, we use the assignment operator ( = ) to assign the value 10 to a variable called x :
The addition assignment operator ( += ) adds a value to a variable:
A list of all assignment operators:

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
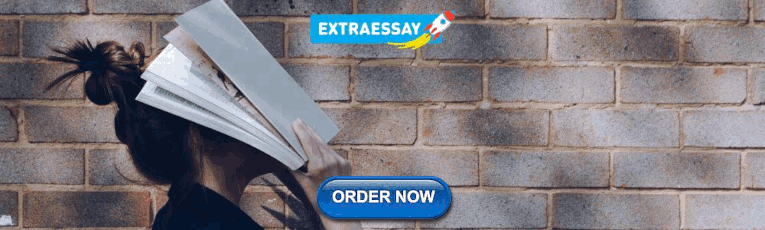
IMAGES
VIDEO
COMMENTS
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. ... The Bitwise AND Assignment Operator does a bitwise AND operation on two operands and assigns the result to the the variable.
The assignment operator is completely different from the equals (=) sign used as syntactic separators in other locations, which include:Initializers of var, let, and const declarations; Default values of destructuring; Default parameters; Initializers of class fields; All these places accept an assignment expression on the right-hand side of the =, so if you have multiple equals signs chained ...
Division Assignment Operator (/=) The Division Assignment operator divides a variable by the value of the right operand and assigns the result to the variable. Example: Javascript. let yoo = 10; const moo = 2; // Expected output 5 console.log(yoo = yoo / moo); // Expected output Infinity console.log(yoo /= 0); Output:
An assignment operator assigns a value to its left operand based on the value of its right operand.. Overview. The basic assignment operator is equal (=), which assigns the value of its right operand to its left operand.That is, x = y assigns the value of y to x.The other assignment operators are usually shorthand for standard operations, as shown in the following definitions and examples.
In this tutorial, you will learn about all the different assignment operators in javascript and how to use them in javascript. Assignment Operators. In javascript, there are 16 different assignment operators that are used to assign value to the variable. It is shorthand of other operators which is recommended to use.
An assignment operator ( =) assigns a value to a variable. The syntax of the assignment operator is as follows: let a = b; Code language: JavaScript (javascript) In this syntax, JavaScript evaluates the expression b first and assigns the result to the variable a. The following example declares the counter variable and initializes its value to zero:
Javascript operators are used to perform different types of mathematical and logical computations. Examples: The Assignment Operator = assigns values. The Addition Operator + adds values. The Multiplication Operator * multiplies values. The Comparison Operator > compares values
2. JavaScript Assignment Operators. We use assignment operators to assign values to variables. For example, let x = 5; Here, we used the = operator to assign the value 5 to variable x. Commonly Used Assignment Operators
The shorthand assignment operator += can also be used to concatenate strings. For example, var mystring = 'alpha'; mystring += 'bet'; // evaluates to "alphabet" and assigns this value to mystring. Conditional (ternary) operator. The conditional operator is the only JavaScript operator that takes three operands. The operator can have one of two ...
The JavaScript Assignment operators are used to assign values to the declared variables. Equals (=) operator is the most commonly used assignment operator. For example: var i = 10; The below table displays all the JavaScript assignment operators. JavaScript Assignment Operators. Example. Explanation. =.
@Alexander The first expression that is evaluated is the outer assignment, which then has to evaluate the -operation, which then has to evaluate its left operand (the sum) and after that its right operand (the inner assignment). Outside-in, left-to-right.
x += 45; JS assignment operator has several shortcuts for performing all the arithmetic operations, which let you assign to the right operand the left operand. Here, you can see that examples of the following assignment operators: +=: addition assignment. -=: subtraction assignment. *=: multiplication assignment. /=: division assignment.
Assignment operators are used in programming to assign values to variables. We use an assignment operator to store and update data within a program. They enable programmers to store data in variables and manipulate that data. The most common assignment operator is the equals sign (=), which assigns the value on the right side of the operator to ...
Learn to structure web content with HTML. CSS. Learn to style content using CSS. JavaScript. Learn to run scripts in the browser ... The right shift assignment (>>=) operator performs right shift on the two operands and assigns the result to ... x >>= y is equivalent to x = x >> y, except that the expression x is only evaluated once. Examples ...
An operator that is used to assign/update the value of a variable is called assignment operator in JavaScript. The most common assignment operator is equal operator "=", which assigns the right-hand value to the left-hand variable. The general syntax is as follows: variable = value; // Here, a value can be a constant, a variable, or an ...
The &&= symbol is a "Logical AND assignment operator" and connects two variables. If the initial value is correct, the second value is used. It is graded from left to right. Syntax. The following syntax shows the Logical AND assignment operator with the two values. Value1 &&= Value2. Value1 &&= Value2. Examples.
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. ... In the example below, we use the assignment operator (=) to assign the value 10 to a variable called x:
Multiplication Assignment Operator (*=) in JavaScript is used to multiply two operands and assign the result to the right operand. Syntax: variable1 *= variable2. // variable1 = variable1 * variable2. Example 1: In this example, we multiply two numerical values using the Multiplication Assignment Operator (*=) and assign the result variable in ...
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. ... Python Assignment Operators. Assignment operators are used to assign values to variables: Operator Example Same As
Example 1: User-Defined Real Estate Application Account Rule Condition Example. This example defines an account rule for assignment to a loan journal line. The account rule has two priorities, a mapping set and a constant. The first priority creates an output for an account based on the mapping set rule definition.
Example of Setting Up and Processing Assignment Rules. This example shows how assignment rules can be set up to assign different ownership definitions to different types of transactions in a joint venture. In this example, a joint operating agreement includes terms for splitting costs and revenue among the managing partner and the three ...
1. "=": This is the simplest assignment operator. This operator is used to assign the value on the right to the variable on the left. Example: a = 10; b = 20; ch = 'y'; 2. "+=": This operator is combination of '+' and '=' operators. This operator first adds the current value of the variable on left to the value on the right and ...
In C++, the addition assignment operator (+=) combines the addition operation with the variable assignment allowing you to increment the value of variable by a specified expression in a concise and efficient way. Syntax. variable += value; This above expression is equivalent to the expression: variable = variable + value; Example.
The Right Shift Assignment Operator is represented by ">>=". This operator shifts the first operand to the right and assigns the result to the variable. It can also be explained as shifting the first operand to the right in a specified amount of bits which is the second operand integer and then assigning the result to the first operand. Syntax:
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, PHP, Python, Bootstrap, Java and XML. ... In the example below, we use the assignment operator (=) to assign the value 10 to a variable called x: Example. package main import ("fmt")